API Assertions: Ensuring Integration and Performance
Developers define statements or conditions called API assertions to verify an API's functionality. These assertions are checkpoints throughout testing to ensure the API fulfills requirements and operates as intended.
Application Programming Interfaces (APIs) are essential to the dynamic field of software development because they enable smooth data sharing and communication across disparate software systems. Reliable testing methods become increasingly important as APIs gain prominence.
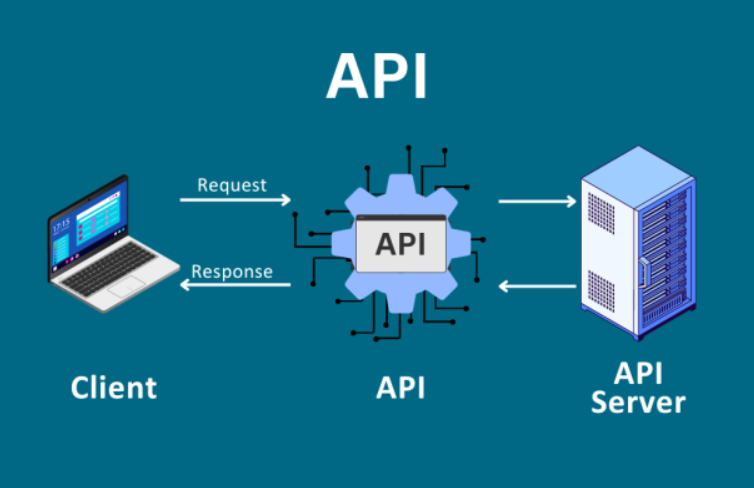
API Assertions: The Backbone of Testing
Developers define statements or conditions called API assertions to verify an API's functionality. These assertions are checkpoints throughout testing to ensure the API fulfills requirements and operates as intended. They are essential to the quality assurance process because they provide an organized way to check if responses from APIs, data formats, and system behavior are accurate.
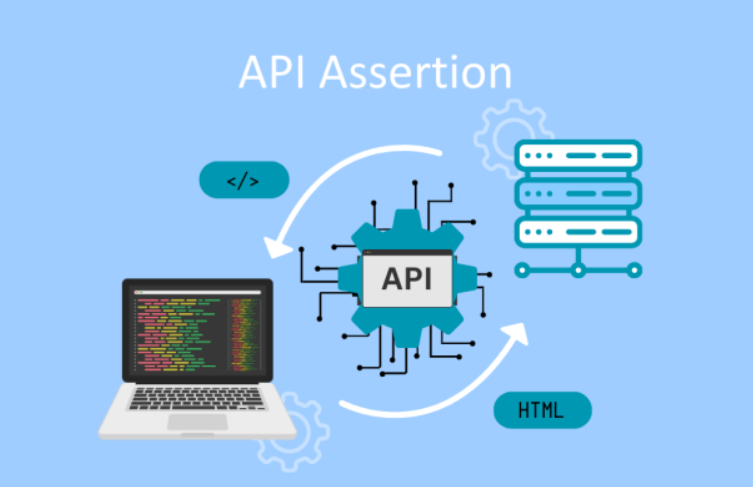
Key Components of API Assertions
Response Validation:
API assertions examine responses that an API produces. This entails verifying that the payload content, response headers, and HTTP status codes are accurate. Developers can identify potential API implementation problems by monitoring deviations from the established expectations for these components.
{
"status": 200,
"contentType": "application/json",
"body": {
"userId": 123,
"username": "example_user",
"email": "user@example.com"
}
}
Data Format Verification:
It is important to guarantee that the data transmitted over the API follows the anticipated format. API assertions assure that the data conforms to set standards or schemas by validating its content and structure.
{
"contentType": "application/json",
"bodyFormat": {
"type": "object",
"properties": {
"name": { "type": "string" },
"age": { "type": "number" },
"email": { "type": "string", "format": "email" }
},
"required": ["name", "age", "email"]
}
}
Performance Metrics Confirmation:
API assertions are capable of assessing an API's performance in addition to its functionality. This includes verifying throughput, latency, and response times to ensure the API satisfies the established performance benchmarks.
{
"responseTime": {
"max": 100, // in milliseconds
"min": 50
}
}
Error Handling Assessment:
A vital component of any API is robust error handling. API assertions can mimic error conditions and confirm that the API reacts correctly, delivering informative error messages and preserving graceful degradation.
{
"status": 400,
"contentType": "application/json",
"body": {
"error": {
"code": "INVALID_PARAMETER",
"message": "Invalid parameter value"
}
}
}
Security Assertion:
To protect sensitive data, an API's security must be guaranteed. Security assertions confirm that the API puts the required security protections in place.
{
"protocol": "https"
}
Concurrency and Scalability Testing:
Evaluating an API's capacity to manage several requests simultaneously and scale under numerous usage scenarios is crucial. In this case, assertions attest to the API's ability to function well even under varying workloads.
{
"responseTime": {
"max": 200 // in milliseconds under high load
}
}
Cross-Browser and Cross-Platform Testing:
A smooth user experience is ensured by confirming that an API operates consistently across various platforms and browsers. Cross-platform and cross-browser assertions validate consistent behavior.
{
"platform": "Windows",
"browser": "Chrome"
}
Mocking and Virtualization:
Controlled testing environments benefit from virtualization and mocking to simulate various scenarios and responses. Assertions confirm that under various simulated scenarios, the API works as intended.
{
"response": {
"statusCode": 200,
"data": "Mocked data for testing"
}
}
Documentation Synchronization:
Maintaining API assertions following documentation is critical to prevent confusion and errors. For proper testing, assertions should be based on the most recent requirements.
{
"documentation": "https://api-documentation.example.com",
"responseFormat": {
"type": "object",
"properties": {
// ... properties as per documentation
},
"required": ["name", "age", "email"]
}
}
Integration with Monitoring Systems:
Monitoring ways with integrated API assertions helps in the early detection of problems. Early problem discovery is ensured by ongoing validation of important components.
{
"monitoring": {
"enabled": true,
"thresholds": {
"responseTime": 150 // in milliseconds
}
}
}
Assertion in Apidog
Apidog empowers developers to validate and confirm the accuracy of API responses within the Apidog platform. With this functionality, users can define specific criteria and conditions that API responses must meet, such as verifying response content, checking status codes, or ensuring the expected format of data.
This robust assertion capability provides developers with a comprehensive toolset for quality assurance in API interactions. By setting up precise assertions, users can catch discrepancies or errors in real-time, ultimately contributing to the creation of more reliable and error-resistant APIs.
The flexibility and customization options within Apidog's assertion framework enable developers to tailor their validation processes to suit the unique requirements of their APIs, fostering a seamless and trustworthy integration experience.
Best Practices for Implementing API Assertions:
Clear Definition of Expectations:
Clearly state what results you expect from API requests. This includes stating the data formats, performance indicators, and expected response codes.
Comprehensive Test Coverage:
Create a wide range of assertions to address different facets of API functioning. To guarantee complete coverage, both positive and negative test scenarios are included.
Parameterized Testing:
Enable parameterized testing by changing the input parameters and seeing the response from the API. This method assists in identifying possible weak points and edge situations.
Regular Update of Assertions:
The relevant assertions should also adapt as APIs change over time. Assumptions should be reviewed and updated often to reflect any API requirements modifications.
Advancing API Assertions: Going Beyond the Basics
Adopting AI-Driven Assertions:
Incorporating artificial intelligence (AI) into API assertions is a novel technique in this age of rapidly changing technology. AI-driven assertions dynamically examine trends in API responses by utilizing machine learning methods. These intelligent assertions anticipate potential issues, adapt to shifting use trends, and offer more precise and flexible testing.
Dynamic Security Assertions:
In today's ever-evolving threat landscape, static security assumptions are no longer enough. Dynamic security assertions adapt constantly to new threats using threat data and ongoing security monitoring. These assertions are essential to preserving an aggressive security posture for APIs.
Globalization Testing Assertions:
Globalization testing assertions become critical when APIs serve a global audience. These assertions guarantee that APIs operate without glitches across various linguistics, geographical, and cultural settings and avoid localization-related problems.
API Governance Assertions:
The major goals of API governance assertions are to ensure uniformity and adherence to organizational API standards. These assertions help create a landscape of APIs that is well-documented and standardized, guaranteeing that APIs follow best practices and established rules.
User Experience Assertions:
User experience assertions evaluate the whole user journey, going beyond functional testing. These assertions guarantee user-friendly error messages, accessibility, and responsiveness, all of which contribute to a more positive and fulfilling user experience.
Compliance Assertions for Industry Standards:
Compliance assertions are useful in industries like healthcare, banking, and e-commerce, subject to certain rules. These assertions reduce the possibility of legal and regulatory problems by guaranteeing that APIs follow industry-specific standards and laws.
Regression Testing with Historical Data Assertions:
Regression testing is improved by using historical data-backed assertions by utilizing previous performance measures. These assertions guarantee continued program dependability by identifying and preventing the return of previous problems during software upgrades.
Collaborative Assertion Repositories:
You may promote cooperation by presenting the idea of collaborative assertion repositories. This method allows development teams to exchange and add to a single library of assertions, encouraging uniformity, knowledge sharing, and quicker onboarding of new team members.
Post-Deployment Assertions:
Expand assertions to include post-deployment monitoring to guarantee continued adherence to performance standards and specified requirements. Post-deployment assertions help with continuous improvement by pointing out problems in real-world production settings.
Cross-Team Collaboration:
To create and maintain assumptions, emphasize how important it is for teams to work together. It is ensured that API testing is done more thoroughly and successfully when developers, QA teams, security specialists, and operational teams are included in the assertion procedures.