Troubleshooting “axios.request is not a function”: A Comprehensive Guide
Learn how to troubleshoot the “axios.request is not a function” error in your JavaScript projects. Understand Axios, common causes of the issue, and practical solutions. Get your APIs back on track!
Let's take a moment to appreciate the versatility of the axios
library. This powerful JavaScript tool simplifies the process of making HTTP requests, allowing developers to effortlessly communicate with APIs and retrieve data. Its sleek and intuitive interface has made it a staple in the modern web development ecosystem.
APIs play a crucial role in web development. They allow applications to communicate with external services, retrieve data, and perform various tasks. One popular library for making HTTP requests in JavaScript is Axios. However, encountering the error message “axios.request is not a function” can be frustrating. Fear not! In this blog post, we’ll delve into the causes of this issue and provide practical solutions.
Understanding Axios and Its Role in API Requests
What Is Axios?
Axios is a promise-based HTTP client for the browser and Node.js. It simplifies making HTTP requests, handling responses, and managing errors. Developers love it for its clean syntax and flexibility. Whether you’re fetching data from an API, posting form data, or handling file uploads, Axios has your back.
The "axios.request is not a function" error often rears its ugly head when there's a mismatch between the version of axios
you're using and the way you're importing or requiring it in your code. This dissonance can occur due to various reasons, such as outdated dependencies, incorrect import statements, or even conflicts with other libraries.
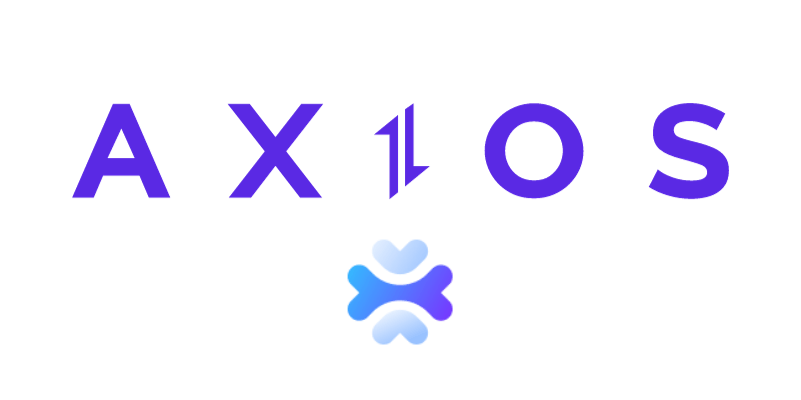
The Anatomy of an Axios Request
Before diving into troubleshooting, let’s review the basic structure of an Axios request:
Creating an Instance:To use Axios, you create an instance of it. This allows you to set default configuration options, such as the base URL, headers, and timeout.
Making Requests:You can make various types of requests using Axios:
axios.get(url, config)
: Fetch data.axios.post(url, data, config)
: Send data to the server.- And more!
Handling Responses:Axios returns promises, which you can chain with .then()
and .catch()
to handle successful and failed requests.
Common Causes of “axios.request is not a function”
1. Typo in Method Name:
Double-check that you’re using the correct method. Instead of axios.request()
, use axios.get()
or axios.post()
.
2. Incorrect Axios Import:
Ensure that you’ve imported Axios correctly. The following import statement is standard:
import axios from 'axios';
3. Version Mismatch:
Sometimes, the error can stem from an outdated version of axios
or its associated dependencies. Run npm update
or yarn upgrade
to bring your project up to speed with the latest versions.
4. Check for Conflicting Libraries:
Certain libraries or plugins may cause conflicts with axios
, resulting in the dreaded error. Review your project's dependencies and remove any potential conflicts.
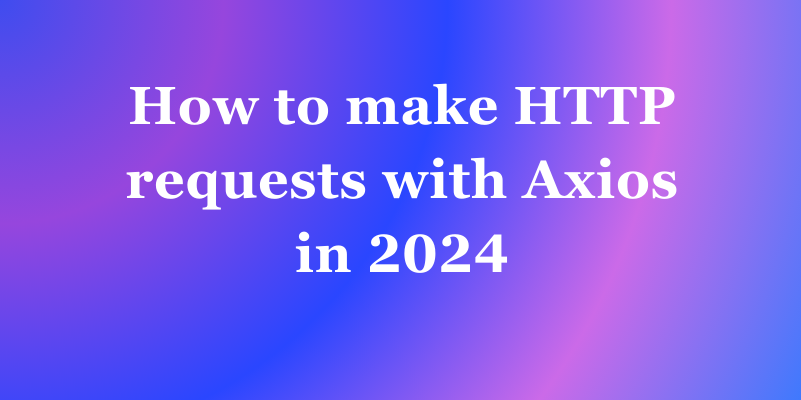
Solutions and Workarounds
1. Method Aliases:
Axios provides aliases for common HTTP methods. For example:
axios.request(config)
is equivalent toaxios(config)
.axios.head(url, config)
is equivalent toaxios({ method: 'head', url, ... })
.
2. Check Your Codebase:
Search your codebase for any instances of axios.request()
. Replace them with the appropriate method (e.g., axios.get()
or axios.post()
).
3. Inspect Dependencies:
If you’re using Axios within a larger project, ensure that other libraries or plugins aren’t interfering. Sometimes conflicting dependencies can cause unexpected behavior.
4. Alternative Solutions for Persistent Issues
If the steps above fail to resolve the issue, fear not, for we have a few more tricks up our sleeves. Consider the following alternatives:
- Reinstall
axios
: Sometimes, a fresh installation can work wonders. Removeaxios
from your project's dependencies and reinstall it usingnpm install axios
oryarn add axios
. - Try a Different Version of
axios
: While it's generally advisable to use the latest stable version, some projects may require a specific version ofaxios
. Experiment with different versions until you find the one that works seamlessly with your codebase. - Explore Alternative Libraries: If all else fails, you might consider exploring alternative HTTP request libraries, such as
fetch
orsuperagent
. While they may require some adjustment, they could provide a workaround for your project.
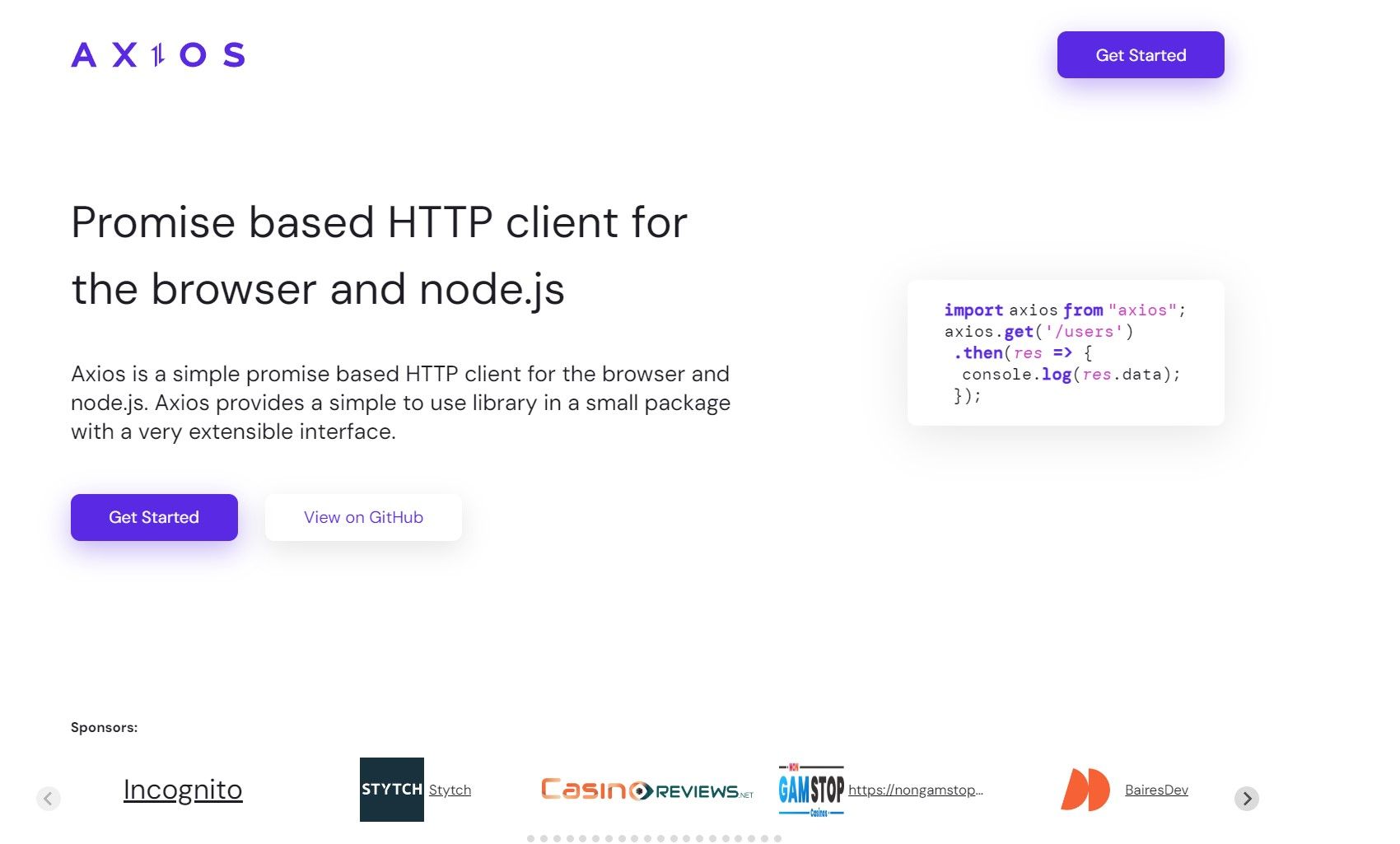
Mastering axios.request
for Seamless API Interactions
To harness the full potential of axios.request
, you'll need to understand its various configuration options. Here's a glimpse into some of the most commonly used options:
method
: Specifies the HTTP method (GET, POST, PUT, DELETE, etc.) for the request.url
: The URL endpoint to which the request should be sent.headers
: An object containing custom headers for the request.params
: An object containing query parameters to be appended to the URL.data
: The data payload to be sent in the request body (for POST, PUT, and PATCH requests).
By mastering these options, you'll be able to craft API calls tailored to your specific needs, ensuring seamless data exchange and optimal performance.
Useasync/await
for Elegant Asynchronous Code
One of the beauties of working with axios
is its seamless integration with modern JavaScript asynchronous patterns, such as async/await. By leveraging these constructs, you can write asynchronous code that reads like synchronous code, greatly enhancing code readability and maintainability.
async function fetchData() {
try {
const response = await axios.request({
method: 'GET',
url: 'https://api.example.com/data',
});
console.log(response.data);
} catch (error) {
console.error(error);
}
}
In the example above, we're using an async
function to make a GET request to an API endpoint using axios.request
. The await
keyword ensures that the code execution pauses until the API response is received, allowing us to handle the response data or any potential errors elegantly.
Optimizing Performance with axios.request
Configurations
While axios.request
is powerful out of the box, there are various configurations and settings you can tweak to optimize performance and ensure your API calls are as efficient as possible. Here are a few tips:
Timeout Settings: Use the timeout
option to set a maximum time for the request to complete before throwing an error. This can prevent your application from hanging indefinitely while waiting for a slow or unresponsive API.
Response Transformations: axios
allows you to define custom response transformations using the transformResponse
option. This can be useful for automatically parsing JSON responses or performing other data manipulations before returning the response data.
Caching Strategies: If your API responses are cacheable, you can implement caching strategies to reduce redundant API calls and improve overall performance. axios
provides built-in support for caching via the cache
option or integrations with third-party caching libraries.
Using Apidog to Send Requests
Apidog offers several advanced features that further enhance its ability to automate requests . These features allow you to customize your requests and handle more complex scenarios effortlessly. For example You can easily send GET requests with params.
Here’s how to use Apidog to send GET requests with params:
- Open Apidog, Click on the New Request button.
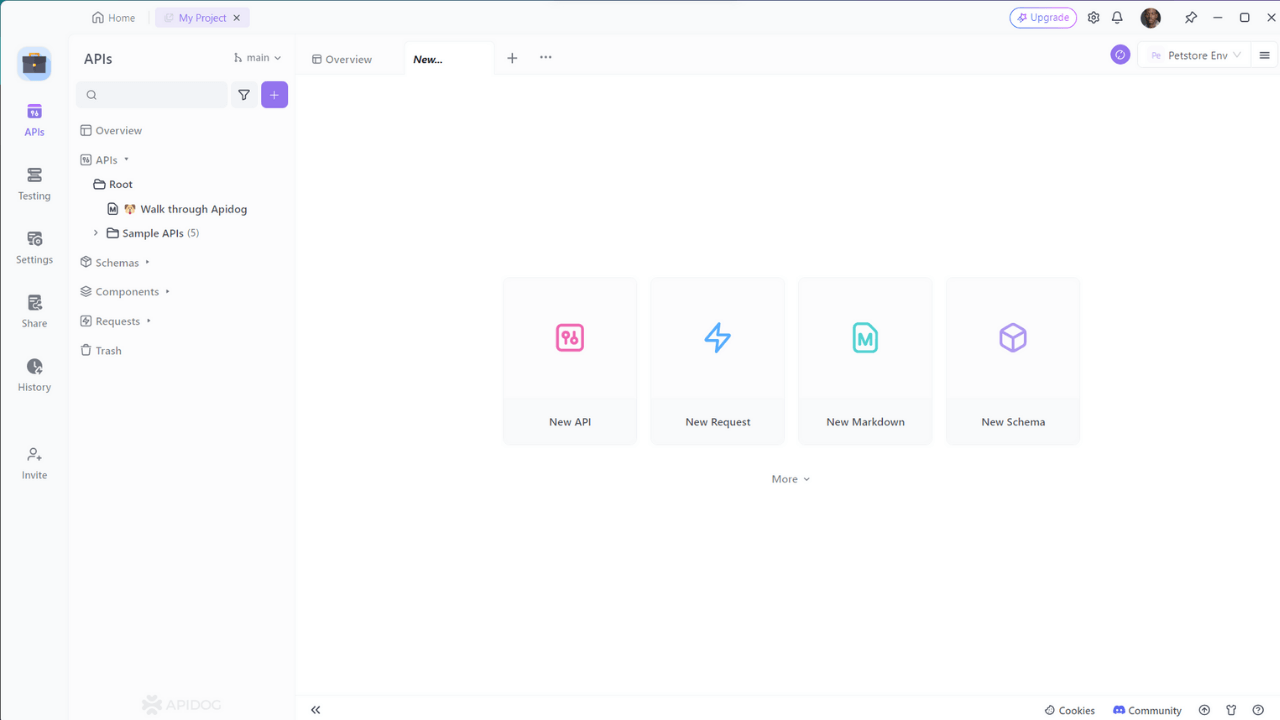
2. Enter the URL of the API endpoint you want to send a GET request to, then click on the Query Params tab and enter the query string parameters you want to send with the request and enter the query string parameters.
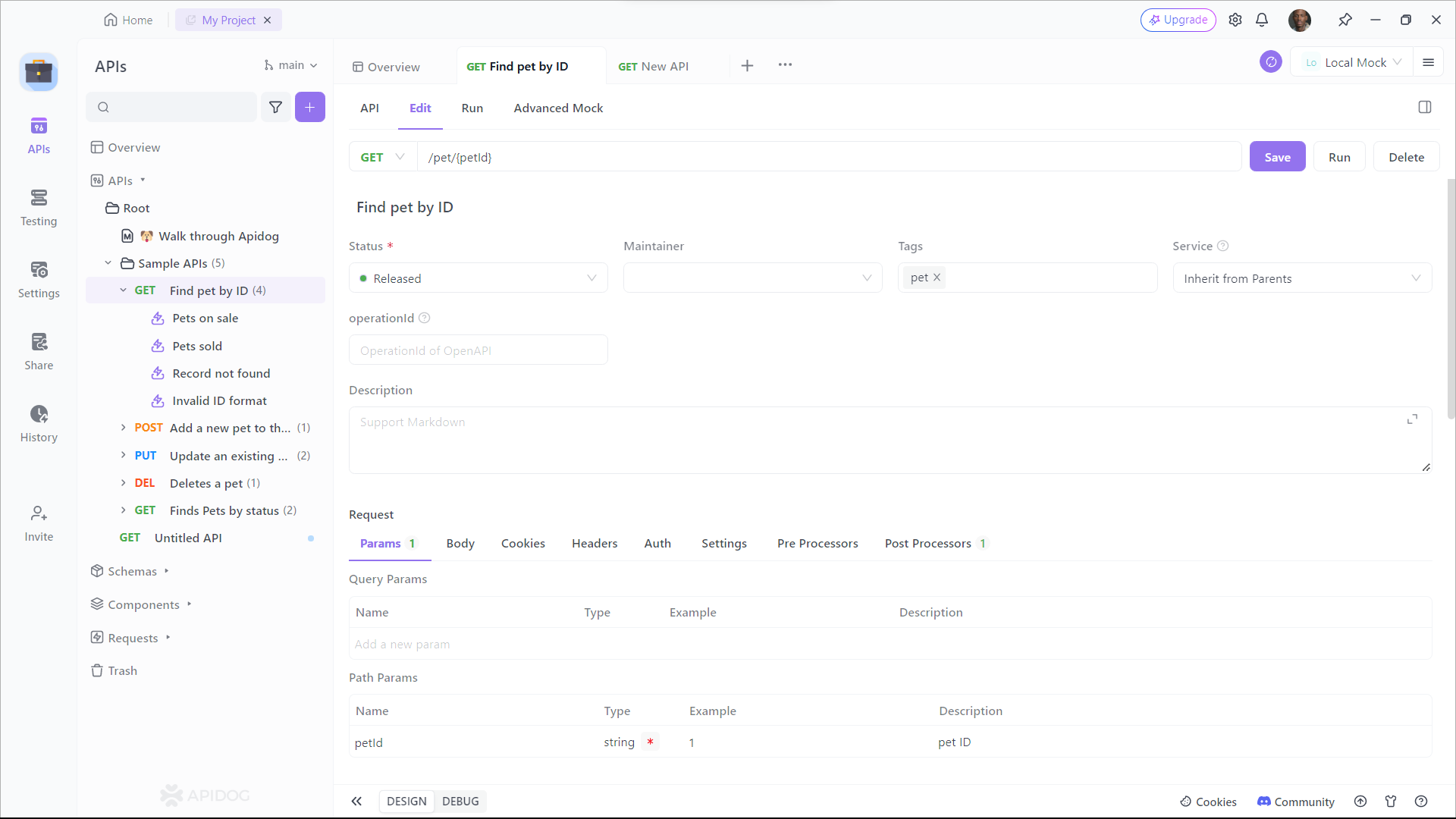
Using Apidog to Automatically Generate Axios Code
Apidog also allows you to automatically generate Axios code for making HTTP requests. Here’s how to use Apidog to generate Axios code:
- Enter any headers or query string parameters you want to send with the request, then click on the Generate Code button.
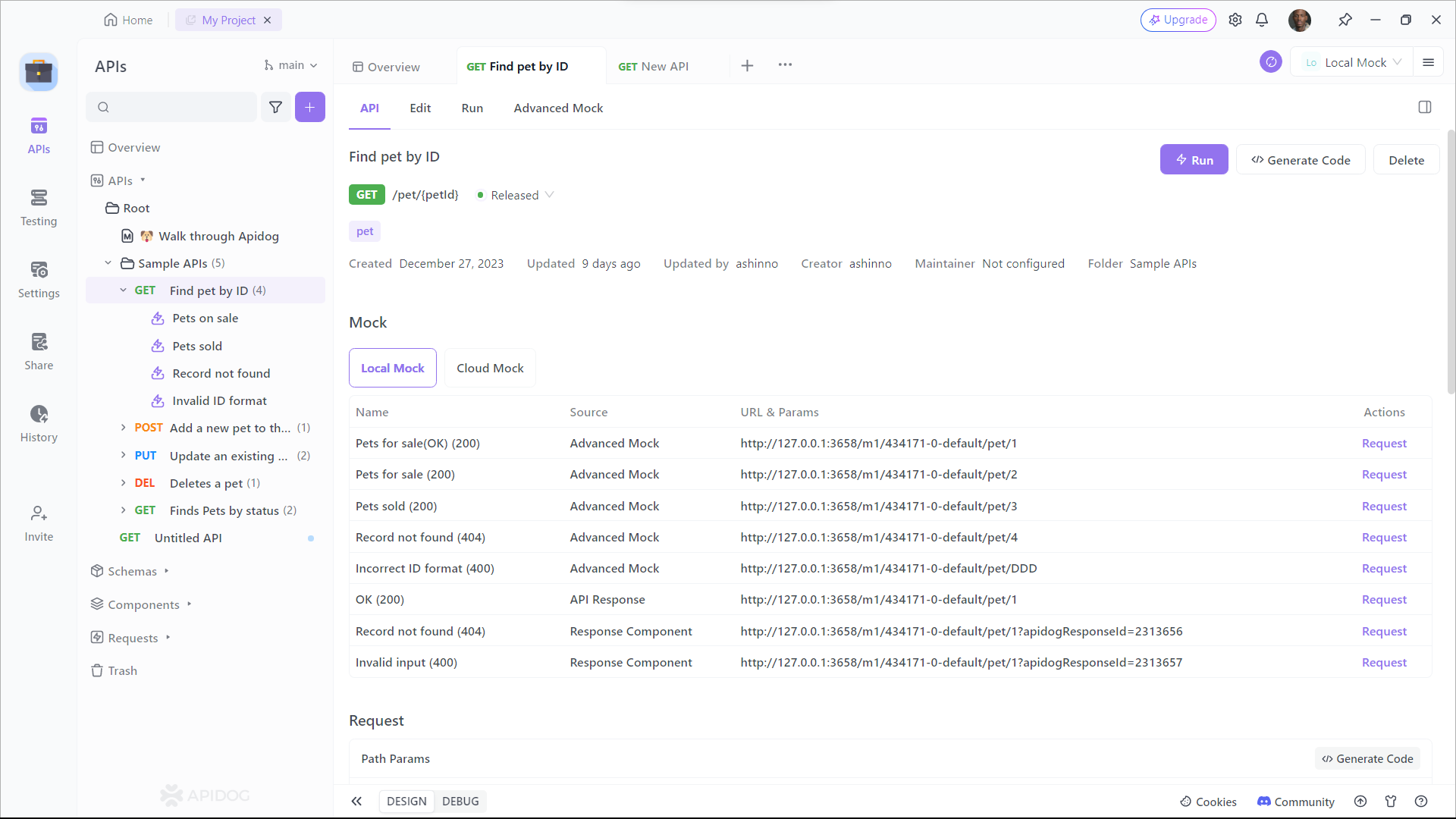
2. Copy the generated Axios code and paste it into your project.
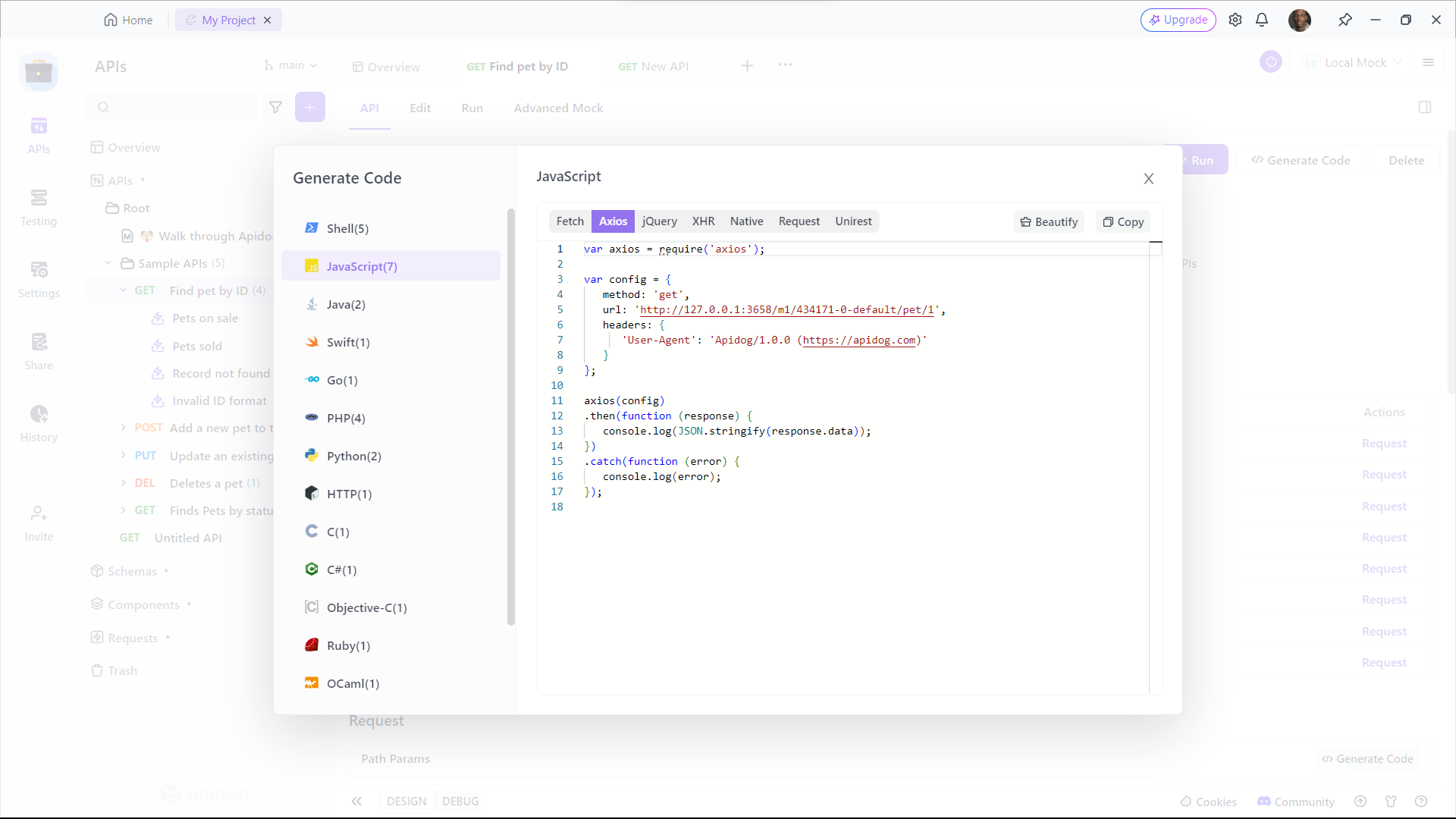
Best Practices for Robust API Communication
As you embark on your journey of mastering axios.request
and API communication, it's essential to embrace best practices to ensure your code is robust, maintainable, and resilient. Here are some guidelines to keep in mind:
Error Handling: Implement proper error handling mechanisms to gracefully handle API errors, network failures, and other unexpected scenarios. This will ensure your application remains stable and provides a smooth user experience.
Code Organization: Keep your API-related code organized and modularized. Separate concerns by creating dedicated modules or services for handling API requests, responses, and data manipulation.
Testing: Write comprehensive tests to ensure your API communication code works as expected under various conditions. Test edge cases, error scenarios, and different response payloads to catch bugs early and maintain code quality.
Documentation: Document your API communication code, including the purpose of each request, expected responses, and any quirks or gotchas. This will streamline collaboration and make it easier for other developers to understand and maintain your codebase.
By following these best practices, you'll not only conquer the "axios.request is not a function" error but also establish a solid foundation for robust and efficient API communication in your web applications.
Conclusion
Mastering Axios is essential for seamless API interactions. Remember to double-check your code, update your dependencies, and choose the right method. With these tips, you’ll conquer the “axios.request is not a function” error and continue building amazing web applications.