Understanding async/await in Node.js
Explore the essentials of async/await in Node.js with our comprehensive guide. Understand promises, async functions, error handling, and more to write efficient, clean code.
Asynchronous programming in Node.js is a core part of its functionality, enabling non-blocking operations and efficient performance. The async/await syntax introduced in ES2017 has revolutionized how developers write asynchronous code.
Understand NodeJs
Node.js is a JavaScript runtime environment that allows you to run JavaScript code outside of a web browser. Node.js is based on the Google Chrome V8 JavaScript engine, and it is used for building web applications, especially data-intensive and real-time ones. Node.js also has a large library of modules and packages that you can use to add functionality to your projects. Some of the benefits of Node.js are:
- It is fast and scalable, thanks to its asynchronous and event-driven nature.
- It is cross-platform and open-source, meaning you can run it on various operating systems and contribute to its development.
- It is consistent and unified, as you can use the same language for both the front-end and the back-end of your web application.
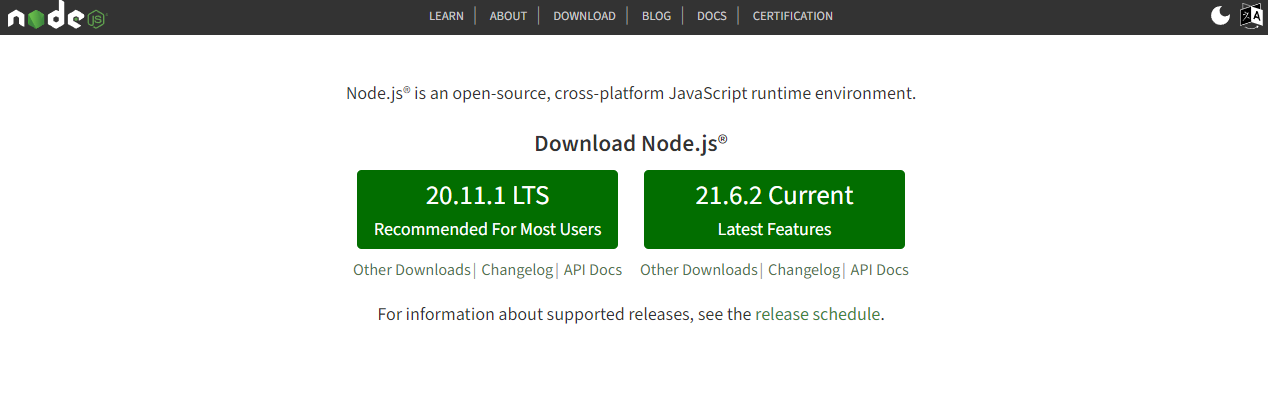
What are Promises?
In JavaScript, a Promise is an object that represents the eventual completion (or failure) of an asynchronous operation and its resulting value. It allows you to work with an asynchronous operation more synchronously. Here’s a breakdown of what a Promise is and how it works:
States: A Promise is in one of these states:
pending
: Initial state, neither fulfilled nor rejected.fulfilled
: The operation was completed successfully.rejected
: The operation failed.- Usage: You can associate handlers with an asynchronous action’s eventual success value or failure reason. This lets asynchronous methods return values like synchronous methods: instead of immediately returning the final value, the asynchronous method returns a promise to supply the value at some point in the future.
- Settling: A promise is said to be settled if it is either fulfilled or rejected, but not pending. The eventual state of a pending promise can either be fulfilled with a value or rejected with a reason (error).
How to Declare an Async Function
Declaring an async
function in JavaScript is quite straightforward. You simply prefix the function declaration with the async
keyword. This signals that the function is asynchronous and can contain one or more await
expressions. Here’s the basic syntax:
async function functionName(parameters) {
// function body
}
Here’s an example of an async
function that fetches data from an API:
async function fetchData(url) {
try {
const response = await fetch(url);
const data = await response.json();
return data;
} catch (error) {
console.error('An error occurred:', error);
}
}
In this example, fetchData
is an async
function that uses await
to wait for the fetch
call to resolve before proceeding. If the fetch
call is successful, it processes the response and returns the data. If there’s an error, it’s caught and logged to the console.
What is Await?
The await
keyword in JavaScript is used within an async
function to pause the execution of the function until a Promise
is resolved. When you use await
, the function waits for the Promise
to settle, and then resumes execution with the result of the Promise
. If the Promise
is rejected, the await
expression throws the rejected value, allowing you to handle it with try
/catch
blocks.
Here’s a simple example to demonstrate await
:
async function getUserData() {
try {
let response = await fetch('/user/data');
let data = await response.json();
console.log(data);
} catch (error) {
console.error('An error occurred:', error);
}
}
getUserData();
In this example, getUserData
is an async
function that fetches user data. The await
keyword is used to wait for the fetch
call to resolve before proceeding to the next line, which also uses await
to wait for the response.json()
call to resolve. If any of these promises are rejected, the error will be caught in the catch
block.
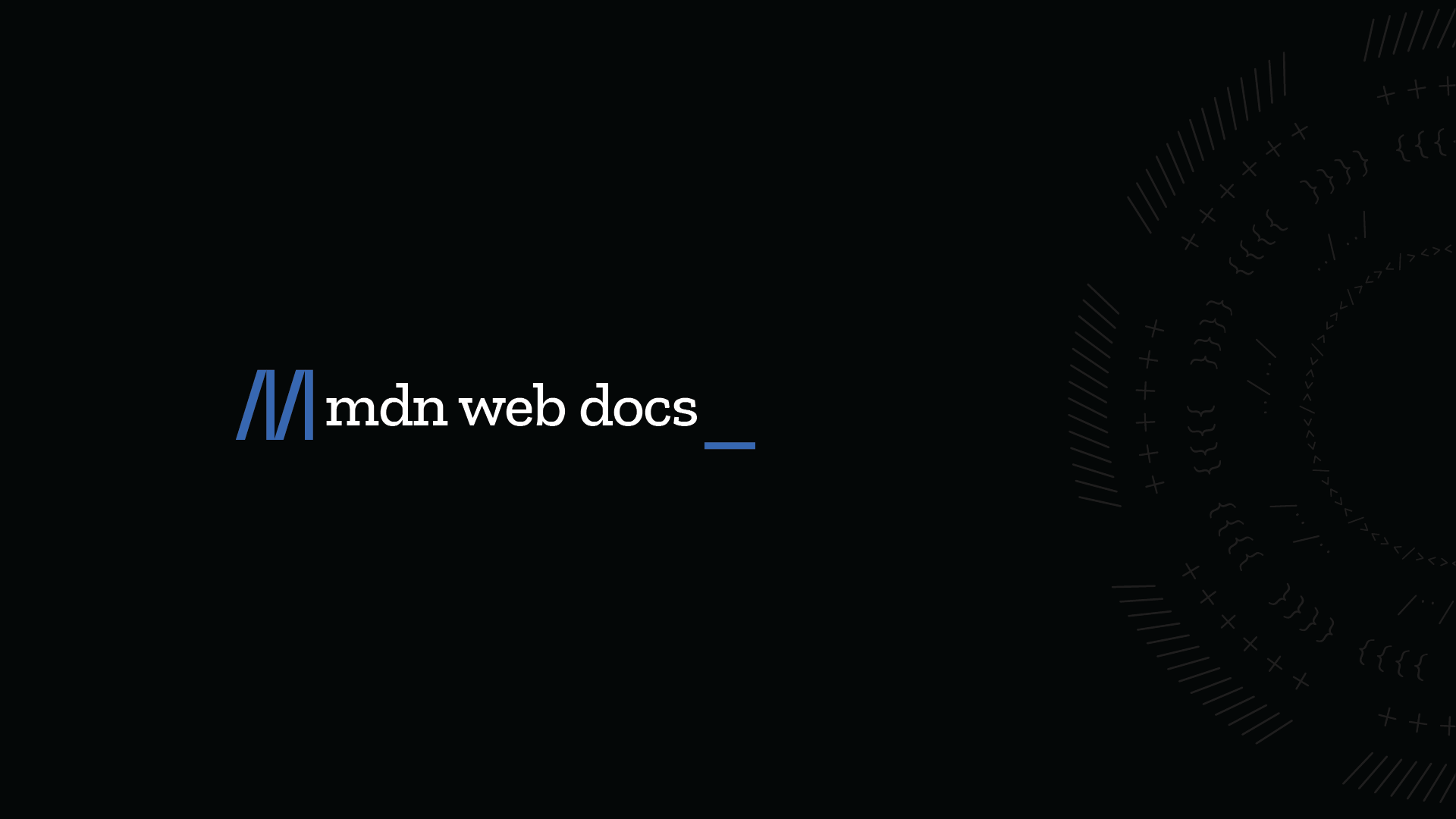
Why Use Async/Await?
Using async
and await
in programming, particularly in NodeJs, provides several advantages:
- Simplifies Asynchronous Code: It allows you to write asynchronous code that looks and behaves more like synchronous code, making it easier to understand and maintain.
- Improves Readability: By avoiding the “callback hell” or “pyramid of doom” scenario, where you have multiple nested callbacks, the code becomes cleaner and more readable.
- Error Handling: It enables better error handling with try/catch blocks, similar to synchronous code, which is not as straightforward with traditional callback-based approaches.
- Control Flow: It gives you better control over the flow of asynchronous operations, allowing you to write code that executes in a more predictable manner.
- Non-Blocking:
await
pauses the execution of the async function and waits for the Promise to resolve without blocking the main thread, allowing other operations to continue running in the background.
Error Handling with Async/Await
Error handling with async
/await
in JavaScript is straightforward and similar to synchronous error handling using try
/catch
blocks. Here’s how you can handle errors in an async
function:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error fetching data:', error);
}
}
fetchData();
In the example above, if the fetch
call fails or if there’s an error parsing the JSON, the error will be caught in the catch
block. This allows for centralized error handling, which is particularly useful when dealing with multiple asynchronous operations.
Remember, unhandled promise rejections can still occur if errors are not properly caught. It’s important to ensure that every promise has a .catch()
handler or is within a try
/catch
block in an async
function to avoid potential issues in your application.
Async/Await with Apidog
Apidog is not just an API development platform; it’s a comprehensive suite that streamlines the entire API lifecycle. With its design-first approach, Apidog ensures that your APIs are not only functional but also intuitive and user-friendly.
Using async/await with Apidog elevates the efficiency of handling API requests and responses. It allows developers to write code that’s clean and easy to read, making debugging a breeze.
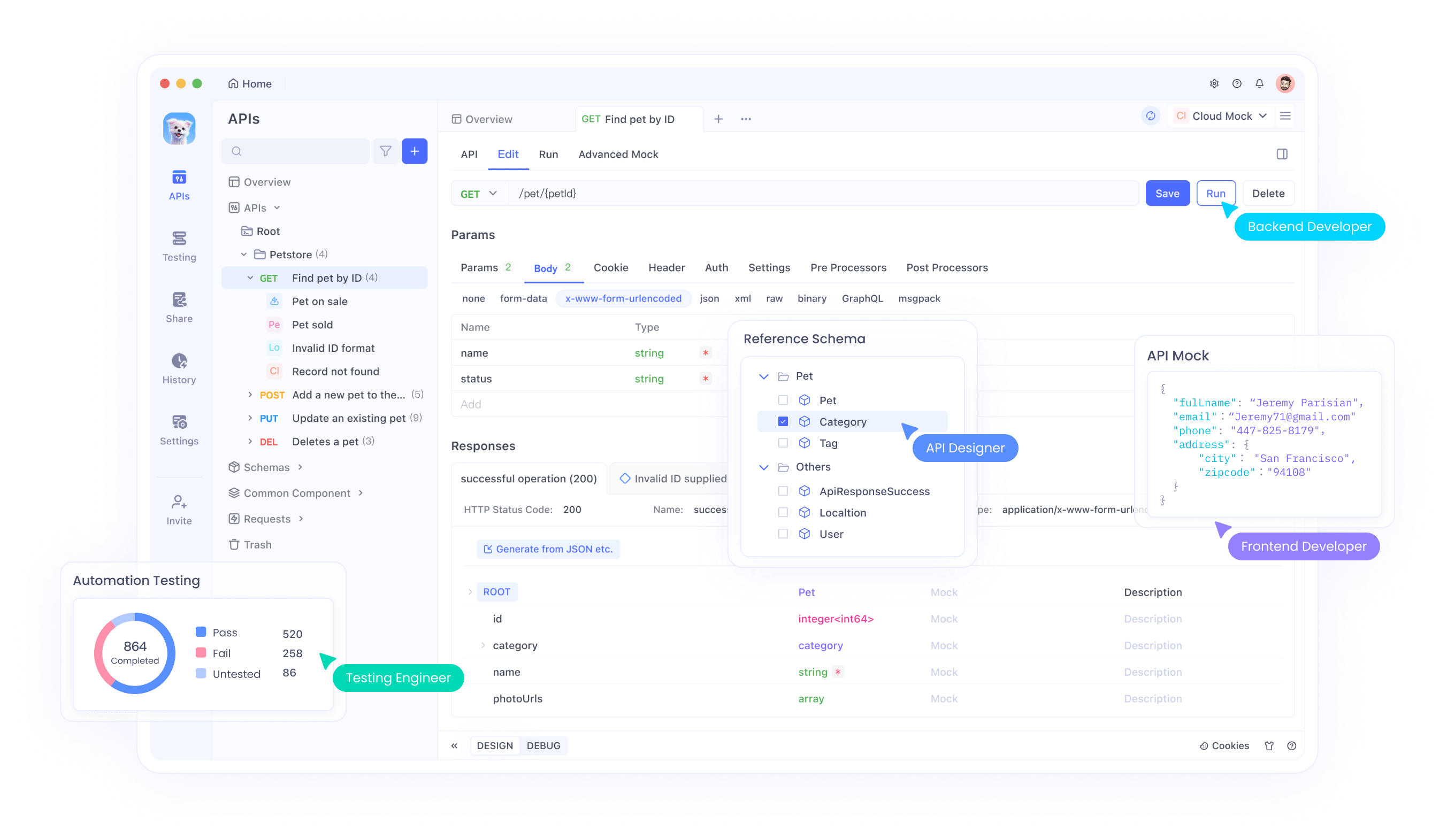
Conclusion
Async/await in Node.js is a powerful feature that simplifies writing asynchronous code. By understanding and utilizing this syntax, developers can write more efficient, readable, and maintainable applications.