Sending Emails with Postmark API and Node.js
Streamline your email communication with Postmark's user-friendly API, ensuring your messages land in inboxes, not the spam folder. Gain valuable insights with detailed analytics and simplify development with seamless Node.js integration. Supercharge your email marketing today!
In the age of instant messaging and social media, it's easy to underestimate the power of email. However, email remains a critical communication channel for businesses and individuals alike. It fosters brand engagement, delivers important updates, and facilitates personalized interactions. Yet, the effectiveness of email hinges on one crucial factor: deliverability. Traditional email sending methods often struggle with spam filters and low inbox rates, leaving your message languishing in the digital abyss.
This is where Postmark steps in. As a developer-friendly transactional email API service, Postmark empowers you to send emails with confidence. It streamlines the process, ensuring your messages reach their intended recipients while providing valuable insights into email performance.
What is Postmark?
Postmark is a cloud-based transactional email API service. This means it operates entirely online, eliminating the need to manage your own email servers or software. Here's a breakdown of the key terms:
Cloud-based: Postmark's infrastructure resides on remote servers, accessible through the internet. You don't need to install or maintain any software on your own machines.
Transactional Email: Transactional emails are emails sent to users depending on the action they take on your website or application. Postmark focuses on emails triggered by specific user actions within an application. These emails are typically one-to-one, personalized messages, such as:
- Password reset confirmations
- Order confirmations and receipts
- Account verification emails
- Notification emails (e.g., security alerts, appointment reminders)
API Service: API stands for Application Programming Interface. It's a set of tools and instructions that allows your application to communicate with Postmark. Using the Postmark API, you can easily integrate email sending functionality into your Node.js application with just a few lines of code.
Why Choose Postmark?
In essence, Postmark simplifies and streamlines email sending for developers and businesses by:
- When it comes to sending transactional emails, traditional methods like webmail or your own server can be cumbersome and unreliable. Here's where Postmark shines. It offers a plethora of advantages compared to these methods:
- Improved Deliverability: This is arguably Postmark's biggest strength. Traditional email sending methods often struggle with spam filters and low inbox rates. Postmark boasts industry-leading deliverability thanks to its:
- Advanced routing techniques: Postmark intelligently routes your emails through a network optimized for deliverability.
- Reputation management: Postmark maintains a pristine reputation with email providers, ensuring your emails are less likely to be flagged as spam.
Detailed Analytics: Gone are the days of blindly sending emails without knowing their impact. Postmark provides comprehensive reports and insights on your email performance. You can track metrics like Open Rates CTR, and Bounce Rates.
These analytics empower you to optimize your email content, improve engagement, and identify any delivery problems that might need addressing.
Simplified Development: Integrating email functionality into your application can be a headache with traditional methods. Postmark's user-friendly API makes this process a breeze. With just a few lines of code, you can leverage Postmark's robust email-sending capabilities within your application. This allows developers to focus on core functionalities without getting bogged down in email complexities.
Enhanced Security: Security is paramount when dealing with user data. Postmark prioritizes data security by employing robust security measures to protect your information and that of your recipients. They adhere to industry standards and regulations to ensure your data remains safe.
In summary, Postmark offers a compelling package for businesses and developers who need a reliable, secure, and data-driven way to send transactional emails. It simplifies the process, increases deliverability, and provides valuable insights to optimize your email marketing efforts.
Enough of the long talk, let's get started with sending emails with Postmark API & Node.js!
Sending Emails with Postmark API and Node.js
Prerequisites: About Postmark API Key
Before we can continue, it's vital that you already have an account with Postmark. At the time of writing this article, it's unfortunate that Postmark doesn't allow creating an account using Google, Yahoo, or any other public domain. This means that you MUST use your domain name to create an account. E.j sam@samuelblog.com
So make sure to create your account, verify your domain, and obtain your API key. We need that key to send emails.
When you create your account, it'll be in test mode. You'll then need to request approval to enjoy all their services.
We also need a tool to test the API that we'll create. We need to know what the response and the status is. For this, I'll use an API testing tool called Apidog to test this setup.
When you create your account, you'll be assigned an automatic project folder where you will be able to test different APIs. You can refer to this documentation to learn about Apidog and its work.
Writing The Codes
If you're already working on a Node.js application, you need to install the postmark Node.js SDK using npm: npm install postmark --save
and that will install the package for us.
If you don't have a working directory yet, please create one using mkdir
. Now run npm init -y
to initialize npm. Once you've done that, run npm install express postmark --save
and open that folder in your favorite code editor - vsCode for most of us.
Now create an index.js or server.js file. That file would hold all our code logic to send emails using the postmark API.
Open that server.js
file and paste the following codes;
const express = require("express");
const app = express();
app.disable("x-powered-by"); // hides express server.
app.use(express.json());
app.post("/send-email", (req, res) => {
res.send("Send email endpoint hit!");
});
app.listen(8080, () => {
console.log(`Backend server listening on port 8080`);
});
The codes above are just a simple node.js setup. Make sure to save it, and start the node.js server using node server.js
.
We need to test the route that was created and to do this, I'll use Apidog. Open the app, either on your browser or your computer, and click on New project
to create a new project, or click on the default project assigned to you.
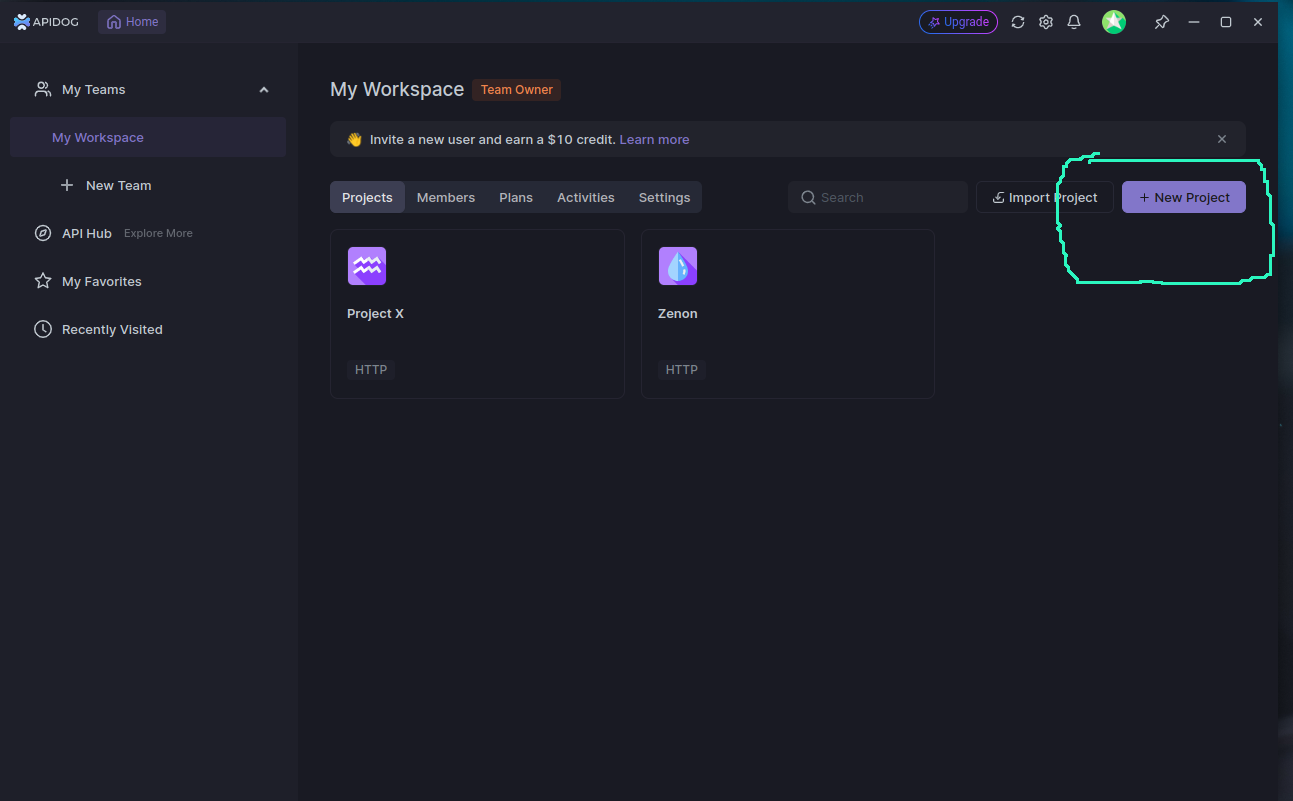
Click on the project you created or the one assigned to you and click on the "New Request" button to send your first request.
In Apidog, type the route in the input field, and click " Run " to see a response.
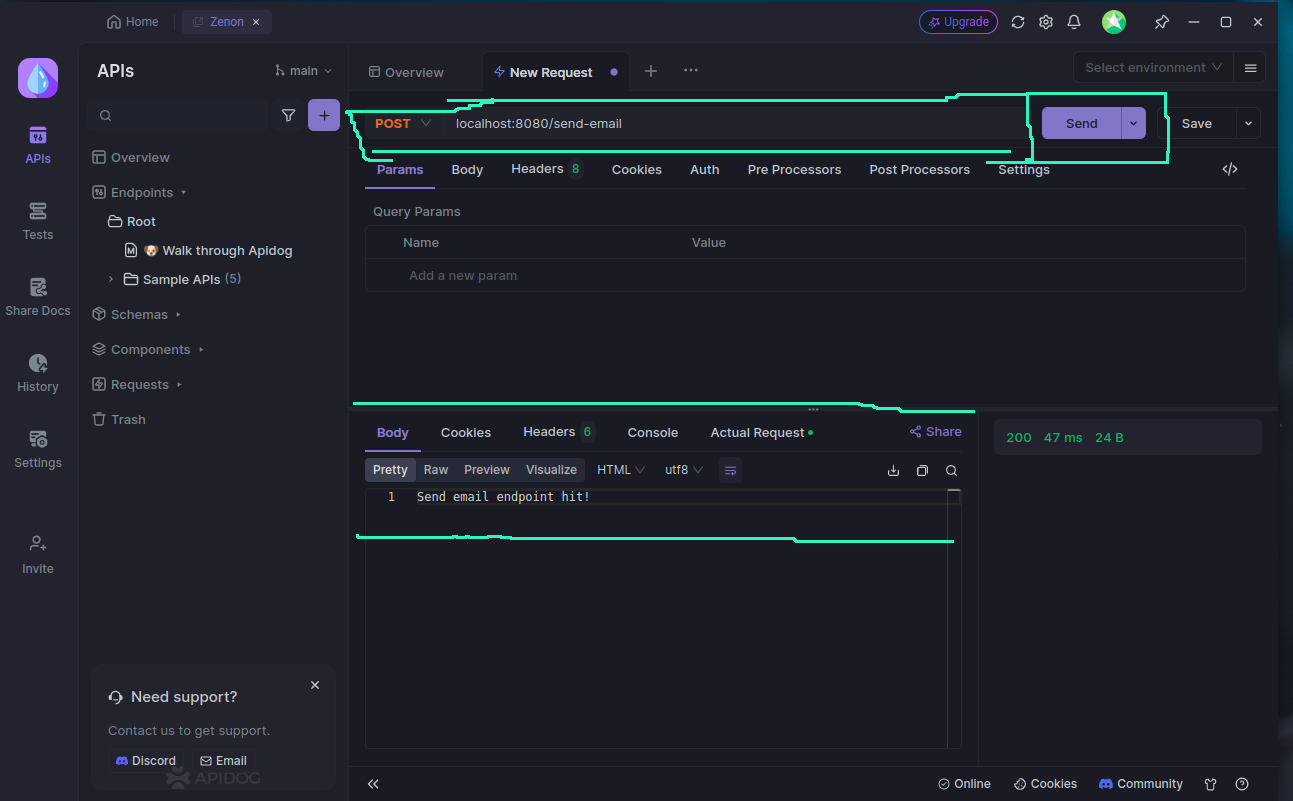
You can see that the response in the body matches with the response we sent in the route we defined. That shows that our application is working, and now we need to go ahead send emails!
Next up, open the server.js file and and update the codebase with the following;
const express = require("express");
const postmark = require("postmark");
const app = express();
app.disable("x-powered-by"); // hides express server.
app.use(express.json());
const client = new postmark.ServerClient(
"acXXXXXXX_XXX" // you can use .env file
);
app.post("/send-email", async (req, res) => {
try {
await client.sendEmail({
From: "iroro@yourdomain.com",
To: "test@blackhole.postmarkapp.com",
Subject: "Hello from Postmark",
HtmlBody: "<strong>Hello</strong> dear Postmark user.",
TextBody: "Hello from Postmark!",
MessageStream: "outbound",
});
res.send("Email sent successfully!");
} catch (error) {
console.error("Error sending email:", error);
res.status(500).send("Error sending email"); // Handle error response
}
});
app.listen(8080, () => {
console.log(`Backend server listening on port 8080`);
});
The code is updated with Postmark SDK. We're using our API key to send the request to Postmark to verify we're making the request. We're also using try catch
should incase we run into an error, log the error and see where the error is coming from.
If you have these codes, save the file and restart your node server. After that, held back to Apidog and send a new request to the /send-email
endpoint.
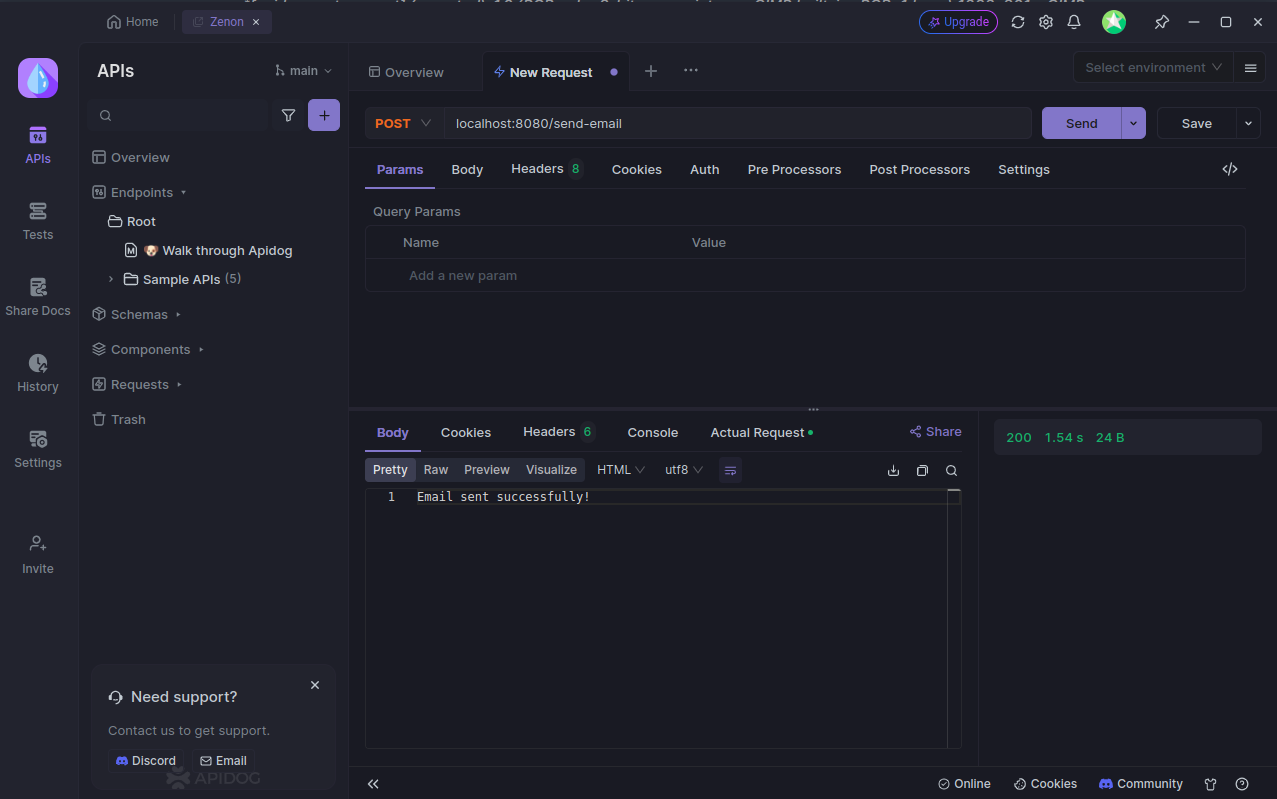
If everything goes well for you, you should be able to send email using the Postmark API directly from your Node.js application!
To confirm this worked, you can view your logs or check the email you sent to, if you've verified your account and it should work well for you.
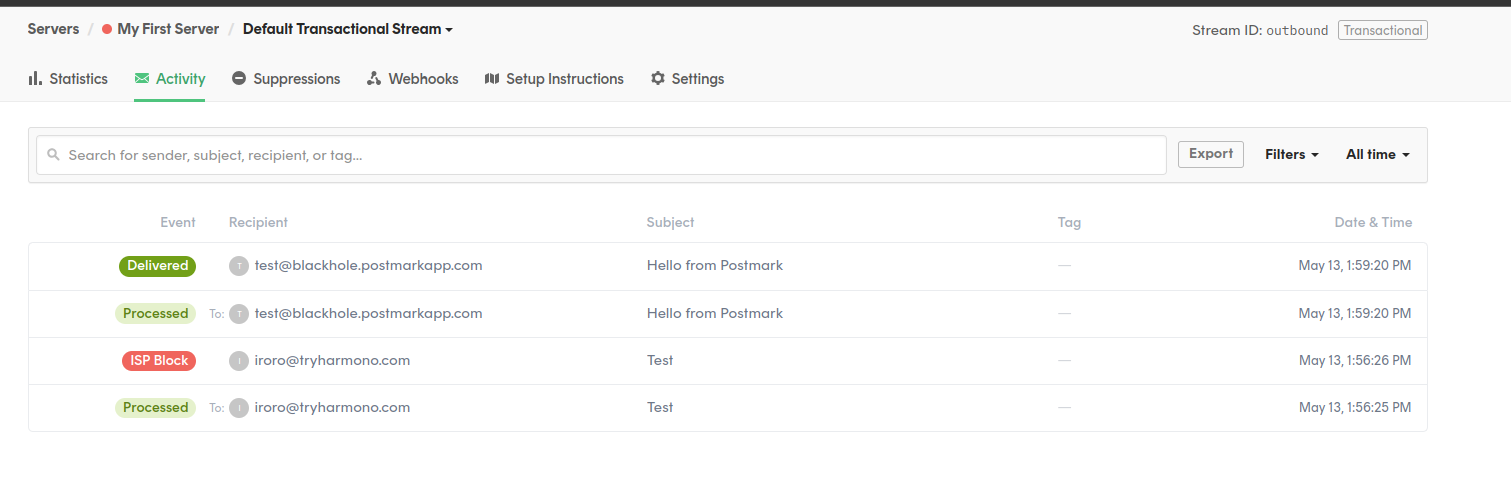
Postmark API Sending Limits
Postmark prioritizes smooth and reliable email delivery for all users. While they don't impose a strict daily sending limit for broadcast emails, they do have measures in place to ensure responsible sending practices. Here's a breakdown:
Batch Sending:
- Maximum Messages: Up to 500 emails can be sent in a single API call using the batch endpoint.
- Payload Size: The total payload size, including attachments, cannot exceed 50 MB per API call.
Broadcast Message Streams:
- Focus on Reputation: Unlike daily limits, Postmark monitors the health of your Broadcast Message Streams based on two key metrics:
- Spam Complaint Rate: Maintain a spam complaint rate below 0.1% to avoid warnings.
- Bounce Rate: Keep your bounce rate under 10% to ensure optimal delivery.
Monitoring and Warnings:
- Color-Coded Indicators: Postmark uses a color-coding system within your account to visually represent the health of your Broadcast Message Streams:
- Orange: Your spam complaint rate or bounce rate is approaching the limit.
- Red: Your spam complaint rate or bounce rate has exceeded the limit. This can potentially impact your sending ability.
Best Practices for Optimal Sending:
- Distribute Sending: Spreading emails throughout the day in bursts is generally preferred over sending large volumes at once.
- Monitor Your Volume: Track your daily and hourly email sending volume. If you anticipate a significant increase, contact Postmark support to discuss your needs.
- Optimize Your Emails: Ensure proper email formatting and avoid spammy content. High bounce rates or spam complaints can affect your account health.
By following these guidelines and maintaining responsible sending practices, you can leverage Postmark's email API to its full potential and ensure your emails reach their intended audience.
Conclusion
In conclusion, Postmark APIs and Node.js offer a powerful and user-friendly solution for sending emails. With its focus on deliverability, insightful analytics, and simplified development, Postmark empowers you to streamline your email communication and ensure your messages reach their intended audience. Explore Postmark today and unlock the potential of reliable and effective email sending.
Happy Coding!