The Best AI for Solving Coding Problems: A Comprehensive Guide
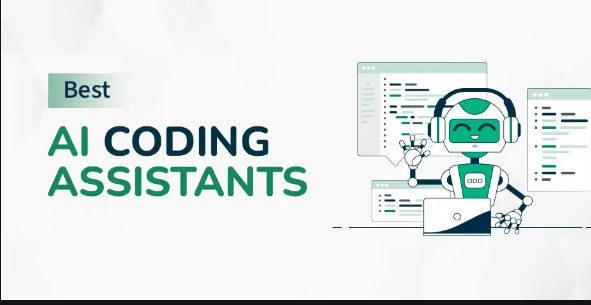
In recent years, artificial intelligence has made significant strides in various domains, including software development. One area where AI has shown remarkable progress is in solving coding problems. As the demand for efficient and accurate code solutions continues to grow, AI-powered tools have emerged as valuable assets for developers, offering assistance in everything from code generation to bug fixing and optimization. In this article, we’ll explore the landscape of AI coding assistants and determine which ones stand out as the best for solving coding problems.
Understanding AI in Coding
Before diving into specific tools, it’s essential to understand how AI is applied to coding problems. AI in coding typically leverages machine learning algorithms, particularly natural language processing (NLP) and deep learning models. These models are trained on vast datasets of code repositories, documentation, and programming patterns to understand and generate human-like code.
The primary goals of AI in coding include:
- Code generation
- Code completion
- Bug detection and fixing
- Code optimization
- Natural language to code translation
With these capabilities, AI coding assistants aim to enhance developer productivity, reduce errors, and streamline the coding process.
Criteria for Evaluating AI Coding Tools
To determine the best AI for solving coding problems, we need to consider several key factors:
- Accuracy of code generation
- Language and framework support
- Integration with development environments
- Learning curve and ease of use
- Customization options
- Performance and speed
- Community support and updates
- Privacy and security considerations
With these criteria in mind, let’s explore some of the top contenders in the AI coding assistant space.
Top AI Coding Assistants
GitHub Copilot
GitHub Copilot, developed in collaboration with OpenAI, has quickly become one of the most popular AI coding assistants. Powered by OpenAI’s Codex model, Copilot offers impressive code generation capabilities across a wide range of programming languages.
Key features:
- Seamless integration with popular IDEs
- Context-aware code suggestions
- Support for multiple programming languages
- Ability to generate entire functions from comments
GitHub Copilot excels in understanding the context of your code and providing relevant suggestions. It’s particularly useful for generating boilerplate code and helping developers explore new languages or frameworks.
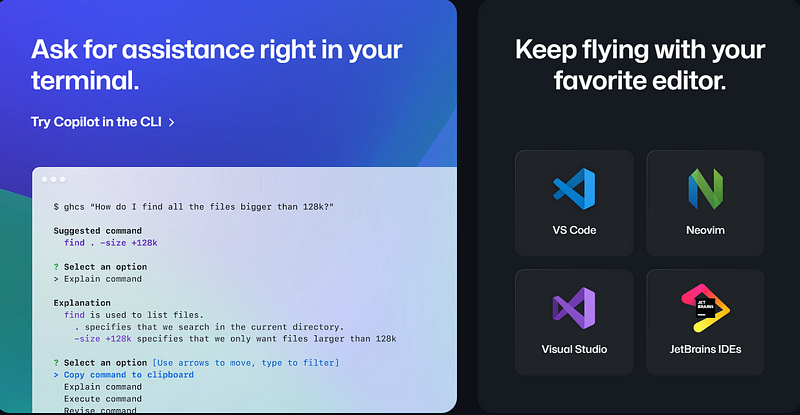
OpenAI Codex
While not a standalone tool, OpenAI Codex is the underlying model that powers GitHub Copilot and other AI coding assistants. It’s worth mentioning separately due to its versatility and potential for integration into various development tools.
Key features:
- Trained on a vast corpus of code from GitHub
- Supports dozens of programming languages
- Can translate natural language into code
- Offers an API for custom integrations
OpenAI Codex’s strength lies in its ability to understand and generate code across multiple languages, making it a powerful foundation for AI coding tools.
Tabnine
Tabnine is another popular AI coding assistant that uses deep learning to provide code completions and suggestions. It stands out for its ability to learn from your coding style and project-specific patterns.
Key features:
- Local and cloud-based options for privacy
- Supports over 30 programming languages
- Learns from your code to provide personalized suggestions
- Offers both free and paid versions
Tabnine’s adaptive learning capabilities make it particularly useful for developers working on long-term projects, as it becomes more accurate and helpful over time.
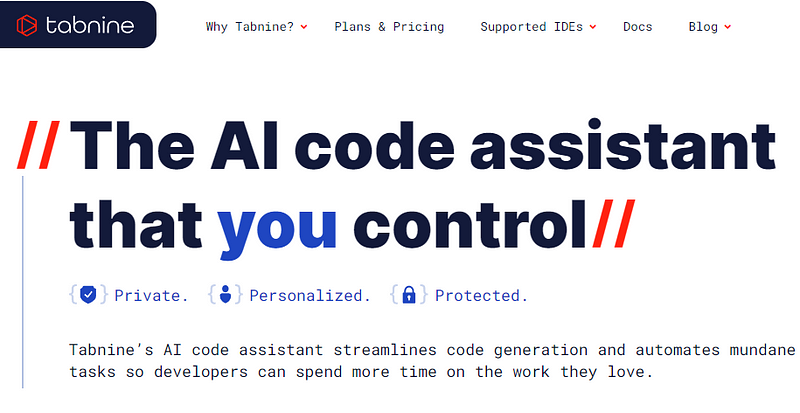
IBM AI for Code
IBM’s AI for Code initiative includes several tools aimed at enhancing the software development process. While not a single product, IBM’s suite of AI coding tools offers various capabilities for solving coding problems.
Key features:
- Code pattern recognition
- Automated code review
- Bug prediction and prevention
- Natural language to code translation
IBM’s tools leverage the company’s expertise in AI and machine learning to provide enterprise-grade solutions for code analysis and generation.
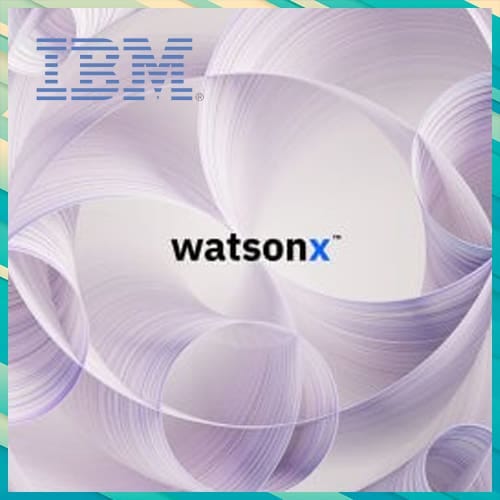
DeepCode
DeepCode, now part of Snyk, uses AI to provide advanced code review and bug detection capabilities. While it’s not primarily focused on code generation, its ability to identify and solve complex coding problems makes it a valuable tool for developers.
Key features:
- AI-powered static code analysis
- Identifies security vulnerabilities and bugs
- Provides fix suggestions
- Integrates with popular version control systems
DeepCode’s strength lies in its ability to detect subtle bugs and security issues that might be missed by traditional static analysis tools.
Comparing AI Coding Assistants
When it comes to determining the best AI for solving coding problems, it’s important to note that different tools excel in different areas. Here’s a brief comparison of the mentioned tools based on our evaluation criteria:
- Accuracy of code generation: GitHub Copilot and OpenAI Codex generally lead in this area, providing highly accurate and contextually relevant code suggestions.
- Language and framework support: OpenAI Codex and Tabnine offer the broadest language support, while Kite specializes in Python.
- Integration with development environments: Most tools offer good integration, with GitHub Copilot and Tabnine having particularly seamless integrations with popular IDEs.
- Learning curve and ease of use: GitHub Copilot and Kite are known for their user-friendly interfaces and easy setup processes.
- Customization options: Tabnine stands out for its ability to learn from your coding style and project-specific patterns.
- Performance and speed: All mentioned tools offer good performance, with local options like Tabnine’s offline mode providing faster response times.
- Community support and updates: GitHub Copilot benefits from the large GitHub community, while IBM AI for Code has strong enterprise support.
- Privacy and security considerations: Tabnine offers local processing options, which can be beneficial for developers working with sensitive code.
The Verdict: Which AI is Best at Solving Coding Problems?
Considering all factors, GitHub Copilot emerges as the top contender for the best AI at solving coding problems. Its combination of accurate code generation, broad language support, and seamless integration with popular development environments makes it a versatile and powerful tool for developers.
However, the “best” tool can vary depending on individual needs:
- For Python specialists, Kite might be the preferred choice.
- Developers concerned about privacy might lean towards Tabnine’s local processing option.
- Those working in enterprise environments might find IBM AI for Code more suitable.
It’s also worth noting that the field of AI coding assistants is rapidly evolving. New tools and improvements to existing ones are constantly emerging, so it’s essential to stay informed about the latest developments.
The Future of AI in Coding
As AI technology continues to advance, we can expect even more sophisticated coding assistants in the future. Some potential developments include:
- More accurate natural language to code translation
- Improved understanding of complex coding patterns and architectures
- Better integration with software development lifecycles
- Enhanced capabilities in code optimization and refactoring
- Increased specialization in specific domains or languages
While AI coding assistants are becoming increasingly powerful, it’s important to remember that they are tools designed to augment human developers, not replace them. The creativity, problem-solving skills, and domain knowledge that human developers bring to the table remain invaluable in the software development process.
Conclusion
The best AI for solving coding problems depends on your specific needs, programming languages, and development environment. GitHub Copilot currently stands out as a top all-around choice, but tools like Tabnine, Kite, and others offer unique strengths that may make them better suited for certain developers or projects.
As you explore AI coding assistants, it’s crucial to experiment with different tools to find the one that best fits your workflow and enhances your productivity. Remember that while these AI tools can significantly streamline the coding process, they should be used as aids to complement your skills and knowledge as a developer.
The field of AI in coding is evolving rapidly, promising even more advanced and helpful tools in the future. By staying informed about these developments and integrating AI coding assistants into your workflow, you can enhance your coding efficiency and focus on solving more complex and creative programming challenges.