Simple Guide to NodeJS Express DELETE Requests
Considering the development of web applications or APIs? Node.js Express, a widely recognized framework, simplifies and streamlines the development process by offering a consistent and structured approach. Learn the necessary knowledge to implement DELETE requests using NodeJS Express.
Within the realm of web development, the ability to manage and manipulate data effectively is paramount. NodeJS Express, a prominent framework for building NodeJS applications, provides a robust and streamlined approach to constructing web APIs. One crucial aspect of data manipulation lies in the utilization of HTTP methods, each dictating how resources are interacted with. The DELETE method plays a vital role in this context, enabling developers to remove specific resources from a server.
This article explores the practical implementation of DELETE requests within NodeJS Express applications. By following a step-by-step approach with illustrative code examples, you will gain a comprehensive understanding of how to leverage DELETE requests to manage data effectively within your NodeJS Express applications.
Let's take a look at the core frameworks to understand before going further into making DELETE requests in NodeJS Express.
What is NodeJS?
NodeJS (or Node.js) is an open-source, cross-platform runtime environment built on Chrome's V8 JavaScript engine. It allows developers to use JavaScript to write server-side scripting and create scalable network applications.
NodeJS Key Features
JavaScript Everywhere
This is a game-changer for developers. NodeJS allows you to use JavaScript for both front-end and back-end development. Since JavaScript is a widely used and popular language, this eliminates the need to learn and manage separate languages for different parts of your application. It can significantly improve developer productivity and reduce the complexity of web application development.
Event-Driven Architecture
Traditional web servers typically rely on threads to handle multiple requests simultaneously. This approach can be resource-intensive for applications with a high volume of concurrent connections. NodeJS, however, utilizes an event loop for handling requests. This event-driven architecture makes NodeJS ideal for real-time applications, chat applications, and any scenario where you need to handle many concurrent connections efficiently.
Non-Blocking I/O
NodeJS employs a non-blocking I/O model. This means it doesn't wait for slow operations (like reading from a database) to finish before processing other requests. Instead, it moves on to the next request while the slow operation completes in the background. This keeps the server responsive and avoids situations where a single slow request can hold up the entire server.
Rich Ecosystem and NPM (Node Package Manager)
The NodeJS community is vast and active. This translates to a rich ecosystem of tools and libraries available through npm, the Node Package Manager. NPM offers a massive collection of pre-written, reusable modules for various functionalities. This saves developers immense time and effort by providing well-tested and maintained code components that they can integrate into their applications.
Scalability
NodeJS applications are known for their horizontal scalability. This means you can easily add more worker servers to handle increased traffic. As your application grows in user base and complexity, you can seamlessly scale your NodeJS application by adding more servers, making it suitable for building large and complex web applications.
What is Express?
ExpressJS, often simply referred to as Express, stands out as a prominent web application framework built upon the foundation of NodeJS. It functions as a powerful layer of abstraction, offering a higher level of development compared to the raw capabilities of NodeJS itself. This abstraction layer translates to a significant reduction in boilerplate code, the repetitive and predictable code that developers often need to write when building web applications from scratch. By streamlining this process, ExpressJS accelerates the development process for web applications and APIs (Application Programming Interfaces).
Express Key Features
Structured Development
ExpressJS enforces a well-defined structure for your web application code. This structure promotes organization, maintainability, and adherence to best practices, ultimately leading to more robust and scalable applications.
Routing
A core functionality of ExpressJS is its routing system. Routing allows developers to map URLs to specific functions within their application. This mapping establishes a clear relationship between incoming requests and the corresponding actions the application should take, making the code more readable and easier to understand.
Middleware
Middleware acts as an extension point within the ExpressJS request-response cycle. These mini-applications can intercept requests and responses, allowing developers to perform common tasks like logging, authentication, or static file serving before the request reaches its final destination. This modular approach promotes code reusability and simplifies complex functionalities.
Templating Engines
ExpressJS integrates seamlessly with various templating engines like EJS, Pug, or Handlebars. These engines enable developers to create dynamic HTML pages by combining HTML with server-side-generated content. This separation of concerns improves the organization and maintainability of your codebase.
Community and Ecosystem
ExpressJS benefits from a large and active developer community. This translates to a vast amount of readily available middleware, libraries, and resources. Developers can leverage these resources to save time and effort when building web applications, fostering innovation and accelerating development cycles.
How to Make NodeJS Express DELETE Requests?
Before making the DELETE requests, you will have to set up your system.
System Setup Requirements
First, you will need NodeJS and NPM installed on your system. Proceed by creating a project directory:
mkdir nodejs-delete-request
Next, navigate to the project directory:
cd nodejs-delete-request
Follow up by initializing a NodeJS project:
npm init -y
Lastly, install Express by running this code of line in Terminal:
npm install express
Build a DELETE Route
Begin by creating a file named server.js
in your project directory. A code example can be seen below.
const express = require('express');
const app = express();
const port = 3000;
// In-memory data store (replace with a database for real applications)
let data = [
{ id: 1, name: "Item 1" },
{ id: 2, name: "Item 2" },
{ id: 3, name: "Item 3" },
];
// DELETE route to remove data by ID
app.delete('/api/data/:id', (req, res) => {
const id = parseInt(req.params.id); // Parse ID from URL parameter
const index = data.findIndex(item => item.id === id);
// Check if data exists for the ID
if (index === -1) {
return res.status(404).send("Data not found");
}
// Remove data from the array using splice
data.splice(index, 1);
res.json({ message: "Data deleted successfully" });
});
app.listen(port, () => {
console.log(`Server listening on port ${port}`);
});
Code Explanation:
- We import Express and create an Express application instance.
- A sample data array serves as a placeholder (a database would be used in production).
- The DELETE route (
/api/data/:id
) captures an ID parameter from the URL. - We parse the ID to an integer for comparison.
findIndex
is used to locate the data object with the matching ID.- If the data is not found, a 404 error response is sent.
- If found, the
splice
method removes the data object from the array at the specified index. - Finally, a success message is sent back in the response.
Using Apidog to Test Your DELETE Request
Apidog is a comprehensive API development platform that provides users with complete tools for the entire API lifecycle. With Apidog, you can design, debug, mock, and document APIs, all within a single application!
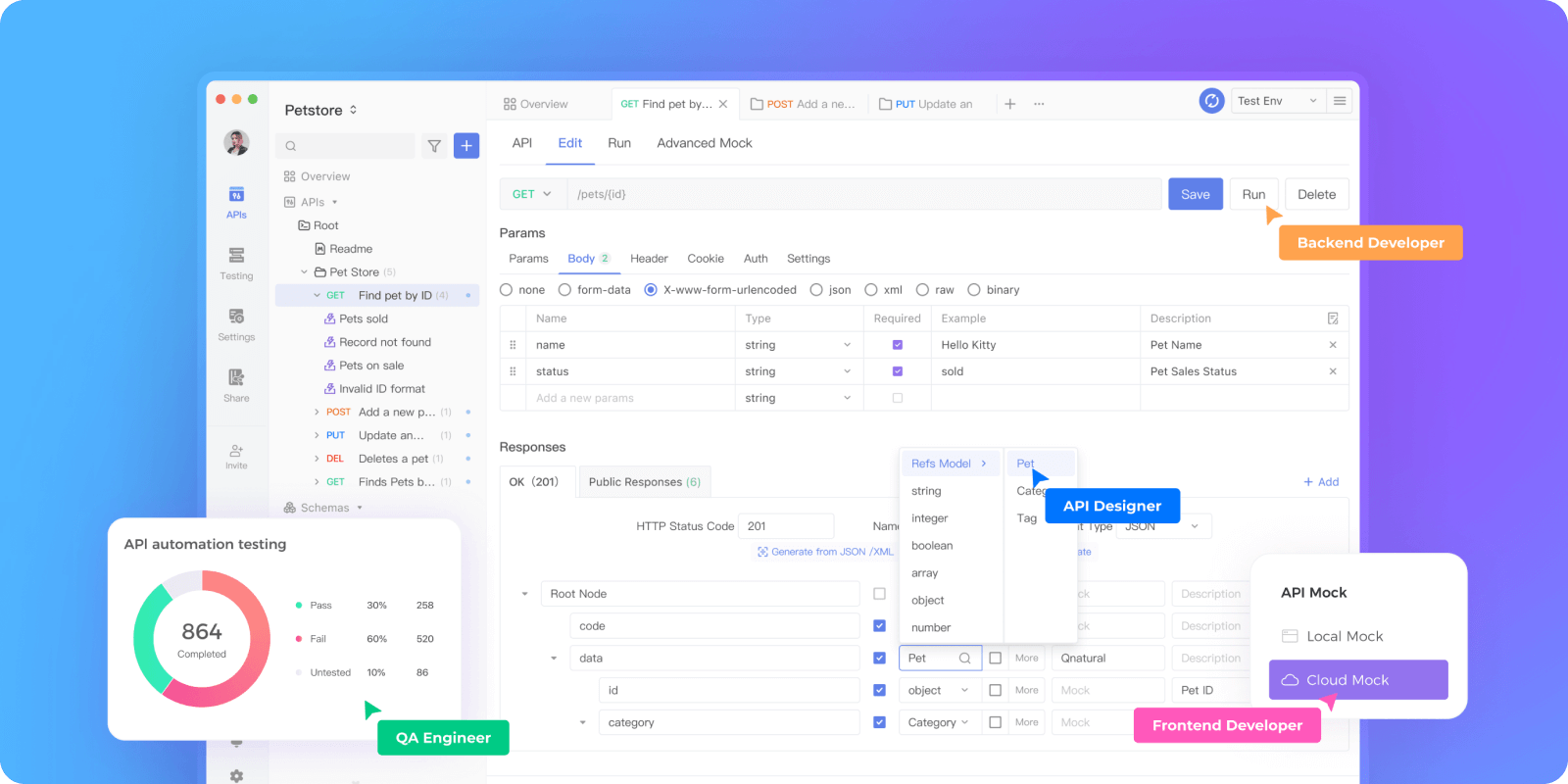
Testing Indiviual APIs Using Apidog
In order to ensure that DELETE requests are made correctly, you can test each request individually.
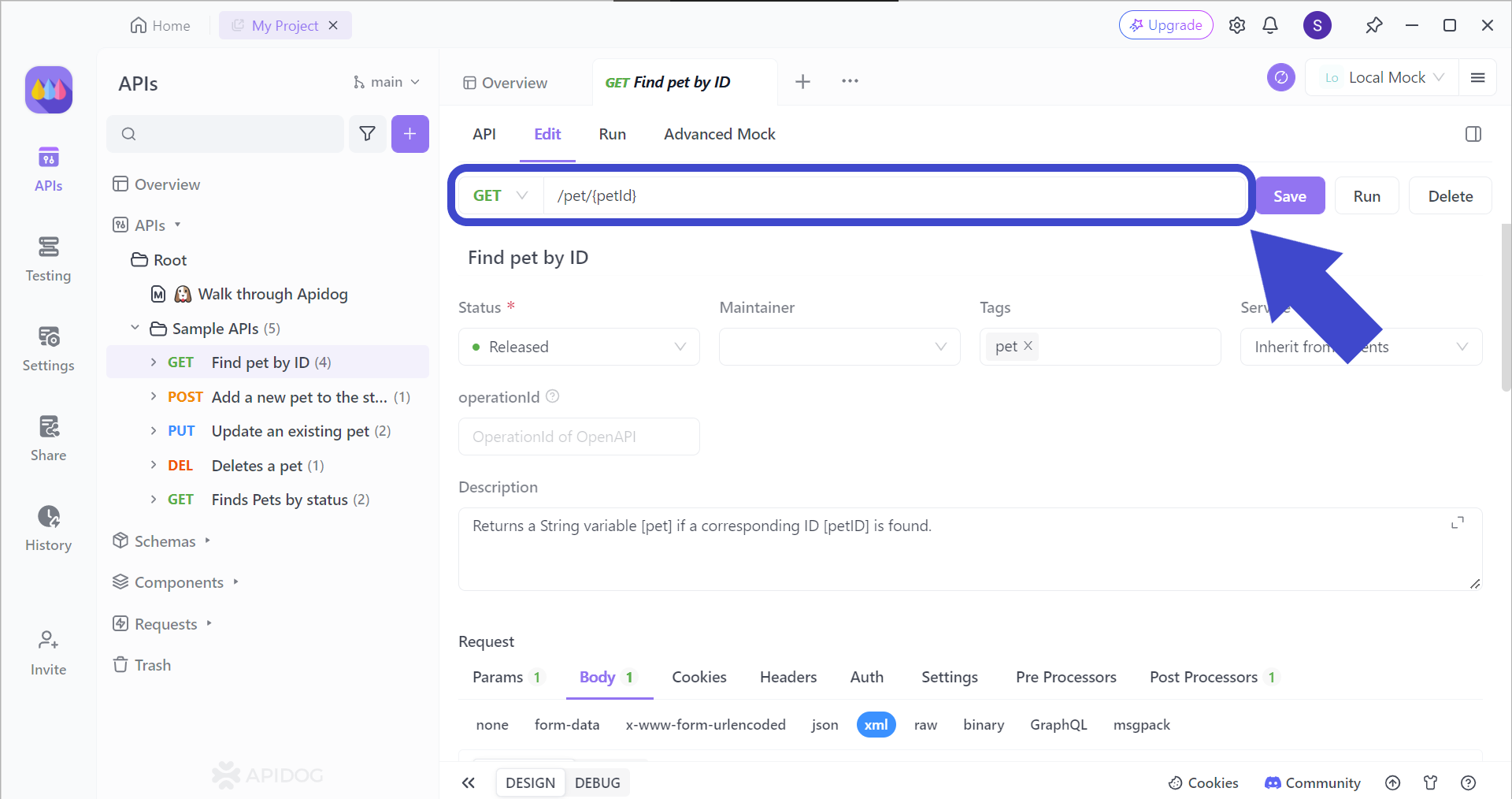
To test an endpoint, simply enter its URL. Include any necessary parameters specific to that endpoint. Feeling unsure about complex URLs with multiple parameters? A separate resource (not included here) offers guidance on how to target specific data within larger datasets.
If you're unsure about using multiple parameters in a URL, this article can guide you on how to hit the exact resource within larger datasets!
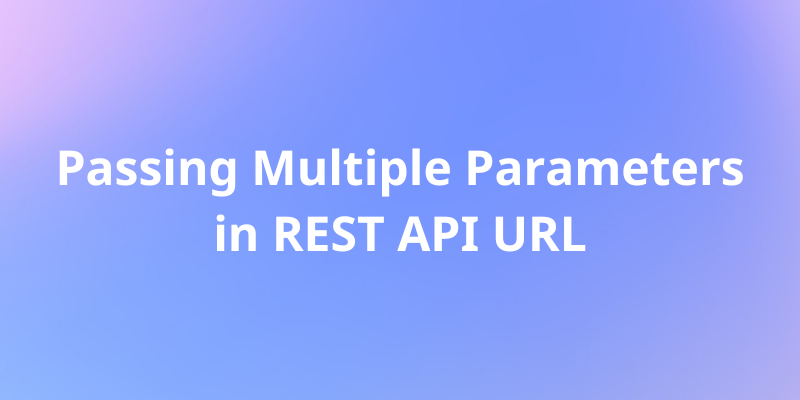
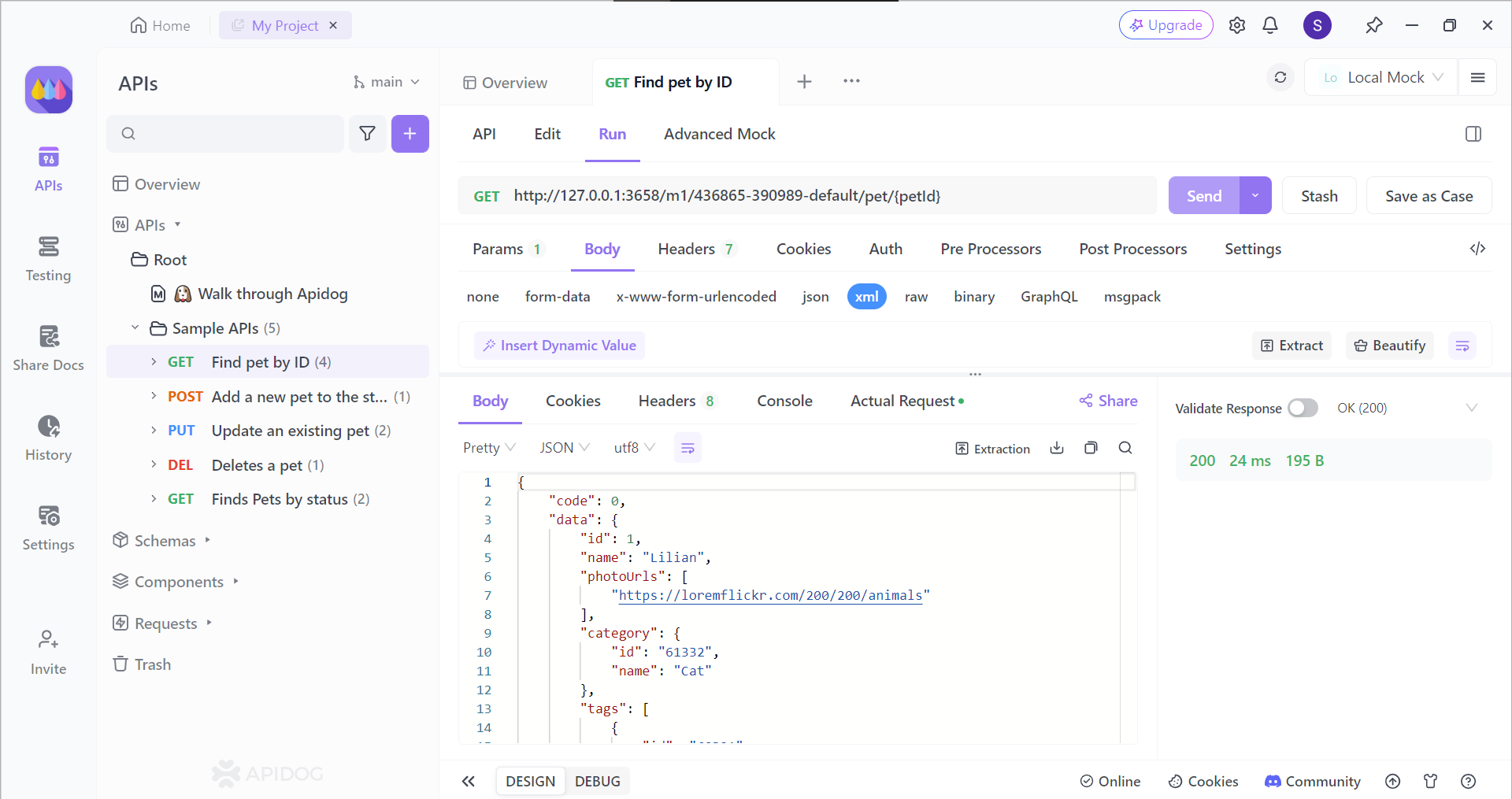
Pressing the Send
button triggers the request and displays the API's response in full detail. The status code quickly indicates success or failure. You can also dive into the raw response, revealing the exact data format your client code needs to process information from the backend servers.
Don't Know JavaScript? Let Apidog Help
Apidog has a code generation feature that's got your back, whether you know JavaScript or not!
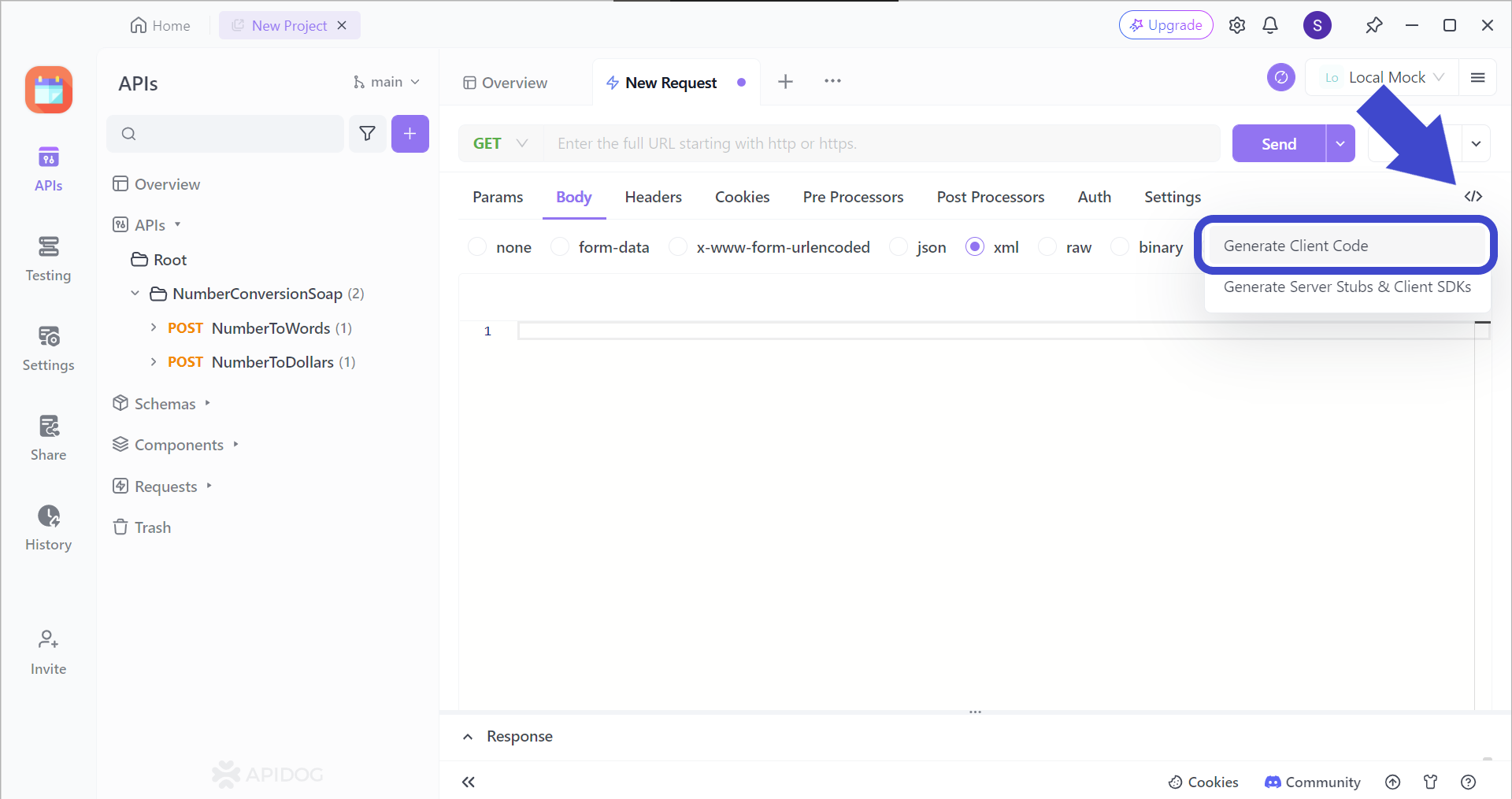
First, locate the </>
button found around the top right corner of the screen. If you are struggling to locate the button, you can refer to the image above.
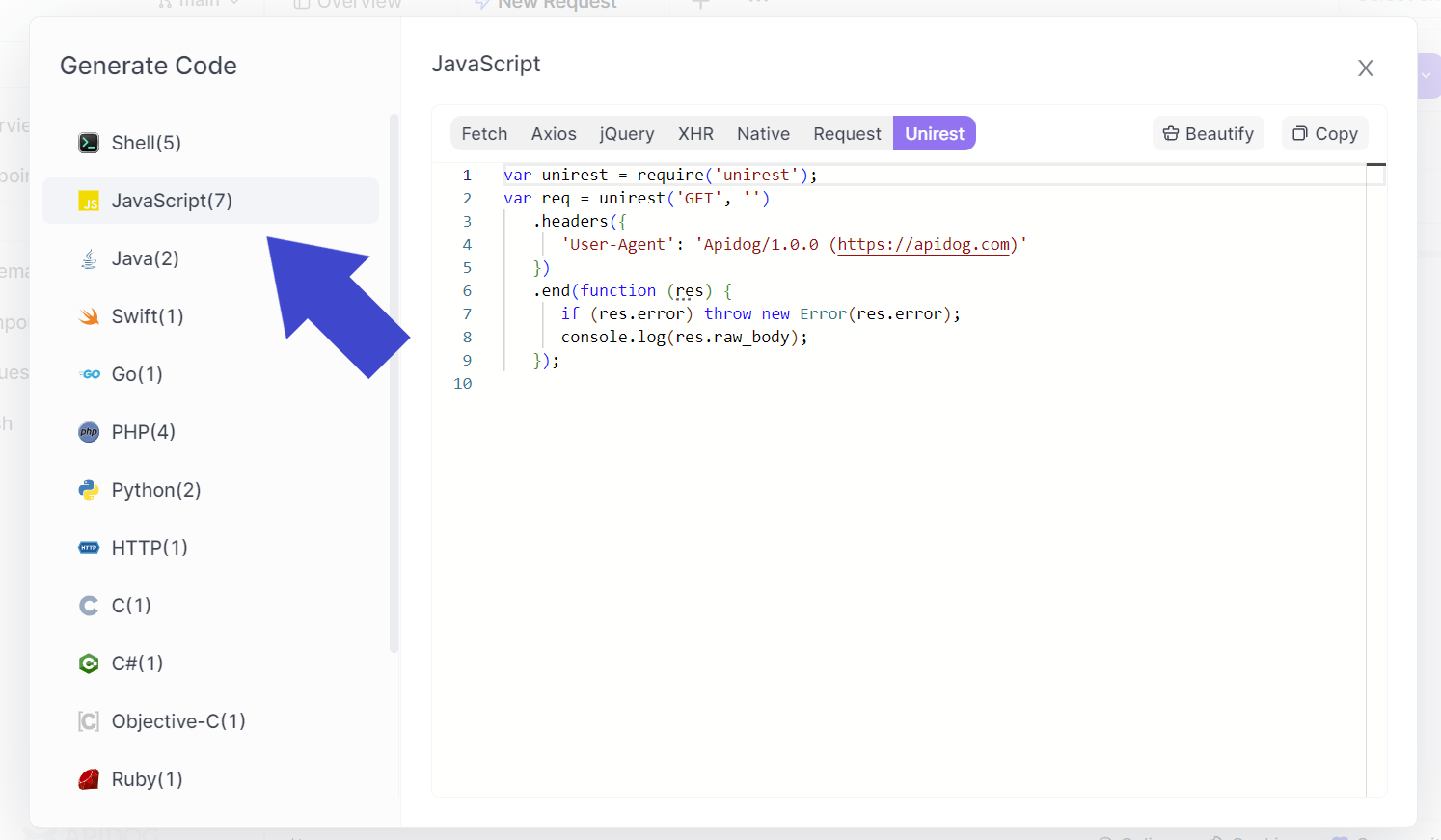
Proceed by selecting the client-side programming language that you need. You have the power to choose which JavaScript library you are more comfortable with.
All you need to do is copy and paste the code to your IDE, and continue with editing to ensure it fits your NodeJS app! However, if you're keen on using Unirest as shown in the image above, you can refer to the article here:
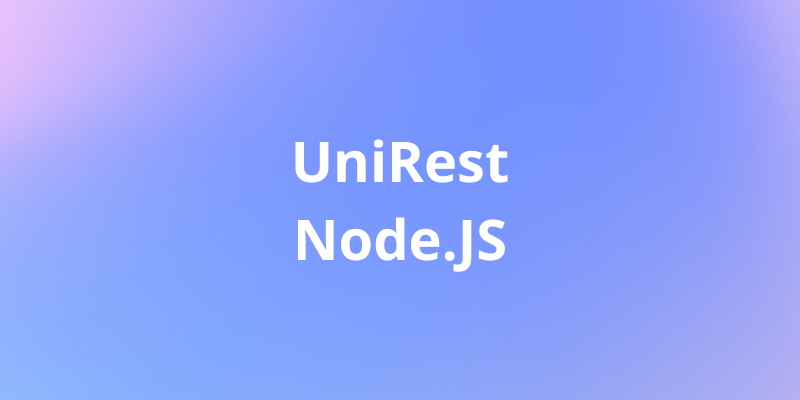
Conclusion
NodeJS Express empowers developers to construct robust APIs that handle resource deletion seamlessly through DELETE requests. This guide explored the core concepts involved in implementing DELETE requests within NodeJS Express applications. We walked you through setting up a project, crafting a DELETE route, and simulating a request using Postman. Remember, this serves as a foundation.
Real-world applications should incorporate best practices like error handling, data validation, database integration, and middleware to ensure a comprehensive and secure development approach. By mastering DELETE requests within NodeJS Express, you can effectively manage and remove data within your web applications and APIs, keeping your data storage efficient and organized.