Postman response to string handling is a critical skill in API testing. It involves capturing, manipulating, and analyzing text-based responses from API calls. This guide will equip you with the knowledge to effectively work with string responses, enhancing your API testing capabilities regardless of your experience level.
In the world of API development and testing, understanding how to handle string responses is crucial. Whether you're working with RESTful APIs, SOAP services, or GraphQL endpoints, the ability to process and validate string responses forms the backbone of effective testing strategies. This comprehensive guide will delve into the intricacies of Postman response to string handling, providing you with the tools and techniques to master this essential aspect of API testing.
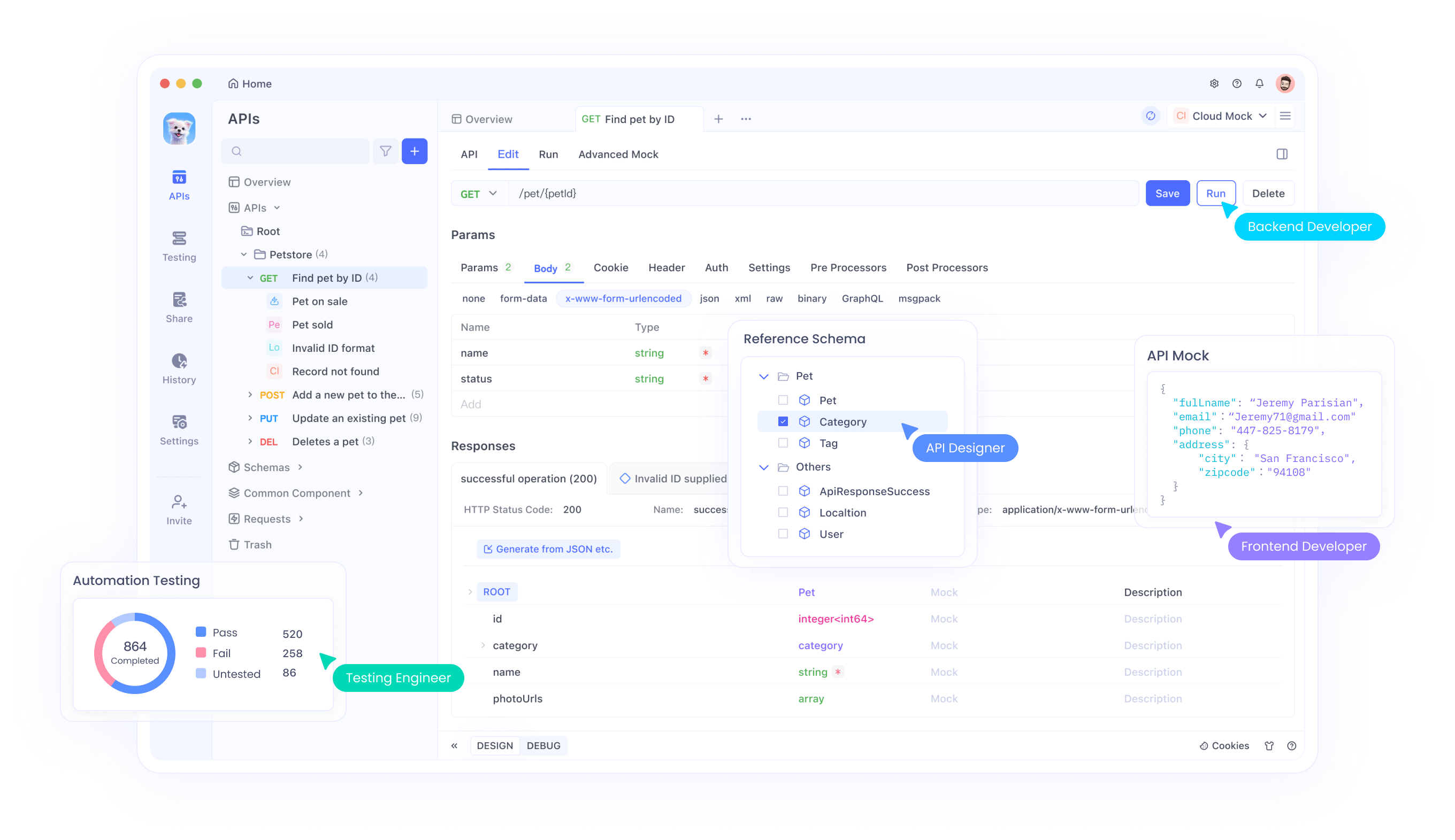
How Do I Get the Response from Postman as a String
A Postman response to string is any text-based output received from an API call. This can range from simple plain text messages to complex data structures like JSON or XML represented as strings.
For example, a weather API might return a string response like "Temperature: 72°F, Condition: Sunny".
Understanding these responses is crucial because they contain the data you need to validate API functionality, extract information for further processing, or chain multiple API calls together in your testing workflows.
String responses are versatile and can accommodate various types of data:
- Plain text: Simple messages or status updates.
- Structured data: CSV, TSV, or custom-formatted data.
- Serialized objects: JSON or XML representations of complex data structures.
- HTML: Web page content or fragments.
- Base64 encoded data: Binary data represented as text.
Each of these types requires different handling techniques, which we'll explore throughout this guide. The flexibility of string responses makes them a universal medium for data exchange in API communications, underlining the importance of mastering their manipulation in Postman.
How to Retrieve Postman Response to String
How do I get the Postman Response as a String?
To retrieve a Postman response as a string, use the following JavaScript code in your Postman tests:
let responseString = pm.response.text();
console.log(responseString);
This method gives you access to the entire response body as a string. It's particularly useful when dealing with plain text responses or when you need to perform custom parsing on the raw response data. For instance, if an API returns a comma-separated list of values, you can easily split this string into an array for further processing:
let responseString = pm.response.text();
let dataArray = responseString.split(',');
console.log(dataArray);
This approach allows you to work with the response data in its most basic form, giving you full control over how you parse and manipulate the information.
It's important to note that while pm.response.text()
is ideal for string responses, Postman provides other methods for different response types:
- For JSON responses:
pm.response.json()
- For XML responses:
pm.response.xml()
- For HTML responses:
pm.response.html()
Choosing the right method ensures that you're working with the response data in the most appropriate format. However, even when dealing with structured data like JSON, there may be cases where you want to work with the raw string response, such as when performing custom parsing or when the response doesn't strictly adhere to JSON format.
Accessing Different Parts of the Postman Response
While the body of the response often contains the most crucial information, other parts of the response can also be valuable for testing and validation:
- Status Code:
let statusCode = pm.response.code;
console.log("Status Code:", statusCode);
- Headers:
let contentType = pm.response.headers.get('Content-Type');
console.log("Content Type:", contentType);
- Response Time:
let responseTime = pm.response.responseTime;
console.log("Response Time (ms):", responseTime);
These additional pieces of information can be crucial for comprehensive API testing. For example, you might want to ensure that your API responds within a certain time frame, or that it's returning the correct content type header for the data it's sending.
Working with Postman Response to String
Common string operations for Postman Response to String
Once you have your response as a string, you can perform various operations to clean, format, or extract specific information. Here are some common operations:
- Trimming whitespace:
let trimmedResponse = pm.response.text().trim();
This is useful for removing any leading or trailing spaces that might interfere with your parsing or comparisons. For example, if you're expecting a specific string value, extra whitespace could cause comparison tests to fail unnecessarily.
- Converting case:
let uppercaseResponse = pm.response.text().toUpperCase();
let lowercaseResponse = pm.response.text().toLowerCase();
Case conversion can be crucial when performing case-insensitive comparisons or when you need to standardize the format of your response data. This is particularly useful when dealing with user input or when comparing against predefined values.
- Searching for substrings:
let responseText = pm.response.text();
if (responseText.includes("success")) {
console.log("Operation successful");
}
This allows you to quickly check for the presence of specific keywords or phrases in your response. It's a simple yet powerful way to validate that certain information is present in the response.
- Extracting information with regular expressions:
let responseText = pm.response.text();
let match = responseText.match(/id: (\d+)/);
if (match) {
let id = match[1];
console.log("Extracted ID:", id);
}
Regular expressions are powerful tools for extracting specific pieces of information from structured string responses. They allow for complex pattern matching and can be used to pull out data from even the most intricate string formats.
- Replacing substrings:
let responseText = pm.response.text();
let sanitizedText = responseText.replace(/sensitive-data/g, "***");
console.log(sanitizedText);
This can be useful for sanitizing sensitive information in logs or for modifying the response for further processing.
- Splitting strings:
let responseText = pm.response.text();
let lines = responseText.split('\n');
console.log("Number of lines:", lines.length);
Splitting strings is particularly useful when dealing with multi-line responses or when you need to process each part of a delimited string separately.
These operations form the foundation of string manipulation in Postman, allowing you to prepare your response data for further processing or validation. By combining these techniques, you can handle a wide variety of string response formats and extract the exact information you need for your tests.
Advanced String Manipulation Techniques
For more complex scenarios, you might need to employ advanced string manipulation techniques:
- Using template literals for dynamic string construction:
let name = "John";
let age = 30;
let formattedString = `Name: ${name}, Age: ${age}`;
console.log(formattedString);
- Parsing structured data within strings:
let responseText = "key1=value1&key2=value2&key3=value3";
let parsedData = {};
responseText.split('&').forEach(pair => {
let [key, value] = pair.split('=');
parsedData[key] = value;
});
console.log(parsedData);
- Using string methods in combination:
let responseText = " User ID: 12345, Name: John Doe ";
let userId = responseText.trim().match(/User ID: (\d+)/)[1];
console.log("Extracted User ID:", userId);
These advanced techniques allow you to handle more complex string responses, giving you the flexibility to extract and manipulate data in sophisticated ways.
Testing Postman Response to String
How to check if a Postman Response to String contains specific text
One of the most common tests is checking for the presence of specific text in your response. This can be done using Postman's built-in testing framework:
pm.test("Response contains expected string", function () {
pm.expect(pm.response.text()).to.include("expected string");
});
This test is versatile and can be used in various scenarios:
- Verifying success messages:
pm.test("Operation successful", function () {
pm.expect(pm.response.text()).to.include("Operation completed successfully");
});
- Checking for error conditions:
pm.test("No error occurred", function () {
pm.expect(pm.response.text()).to.not.include("error");
});
- Validating specific data presence:
pm.test("User data present", function () {
pm.expect(pm.response.text()).to.include("username");
pm.expect(pm.response.text()).to.include("email");
});
These tests ensure that your API is returning the expected content, allowing you to catch issues quickly if the response doesn't contain the anticipated information.
Advanced Testing Techniques
For more complex validation scenarios, you can combine string operations with Postman's testing capabilities:
- Regex-based testing:
pm.test("Response matches expected pattern", function () {
pm.expect(pm.response.text()).to.match(/^User\s\d+:\s[A-Za-z\s]+$/);
});
- Conditional testing based on response content:
pm.test("Appropriate error message for invalid input", function () {
let responseText = pm.response.text();
if (responseText.includes("Invalid input")) {
pm.expect(responseText).to.include("Please provide a valid email address");
} else {
pm.expect(responseText).to.include("User created successfully");
}
});
- Testing multiple conditions:
pm.test("Response contains all required fields", function () {
let responseText = pm.response.text();
pm.expect(responseText).to.include("id");
pm.expect(responseText).to.include("name");
pm.expect(responseText).to.include("email");
pm.expect(responseText).to.include("created_at");
});
- Extracting and testing specific values:
pm.test("User ID is in correct format", function () {
let responseText = pm.response.text();
let match = responseText.match(/User ID: (\d+)/);
pm.expect(match).to.not.be.null;
let userId = match[1];
pm.expect(userId).to.match(/^\d{5}$/); // Expecting a 5-digit user ID
});
These advanced testing techniques allow you to create more robust and comprehensive test suites, ensuring that your API responses meet all the required criteria and contain the expected data in the correct format.
Best Practices for Postman Response to String Handling
When working with string responses in Postman, it's important to follow best practices to ensure your tests are reliable, maintainable, and effective:
- Always validate response format before parsing: Before attempting to extract or manipulate data from a string response, verify that the response is in the expected format. This can prevent errors caused by unexpected response structures.
- Use appropriate methods for different response types: While this guide focuses on string responses, remember to use
pm.response.json()
for JSON responses andpm.response.xml()
for XML responses when appropriate. - Handle potential errors gracefully: Wrap your string operations in try-catch blocks to handle potential errors, such as attempting to access properties of undefined values.
- Use meaningful variable and test names: Clear, descriptive names for variables and tests make your scripts more readable and easier to maintain.
- Comment your code: Especially for complex string manipulations or regex patterns, add comments to explain the logic and purpose of your code.
- Avoid hardcoding expected values: Use environment or global variables to store expected values, making your tests more flexible and easier to update.
- Keep tests focused and atomic: Each test should validate one specific aspect of the response. This makes it easier to identify and fix issues when tests fail.
- Regularly review and update your tests: As APIs evolve, make sure to review and update your tests to ensure they remain relevant and effective.
Conclusion
Mastering Postman response to string techniques is essential for effective API testing. This guide has covered the fundamentals of working with string responses, from basic retrieval to common operations and testing strategies. We've explored how to extract, manipulate, and validate string data, as well as how to create robust tests that ensure your APIs are functioning as expected.
By applying these techniques, you'll be able to handle a wide range of API responses, making your testing more robust and efficient. Remember that practice is key – the more you work with string responses in Postman, the more adept you'll become at crafting effective tests and extracting valuable information from your API calls.
As you continue to develop your skills in Postman response to string handling, consider exploring more advanced topics such as:
- Automating API tests using Postman's Newman CLI tool
- Integrating Postman tests into your CI/CD pipeline
- Using Postman's visualization features to create dynamic dashboards based on API responses
By mastering these techniques and continuously expanding your knowledge, you'll be well-equipped to tackle even the most complex API testing scenarios, ensuring the reliability and effectiveness of your applications and services.