Posting JSON Data with PHP: A Practical Guide
Learn how to master the art of posting JSON data with PHP in this practical guide. Discover the importance of JSON, prepare your environment, and explore scenarios for sending and handling JSON data using PHP.
Hey there, fellow developers! Today, we're going to dive into the exciting world of posting JSON data using PHP. Whether you're building a robust API, integrating with third-party services, or simply handling data exchanges, understanding how to work with JSON in PHP is an essential skill. So, let's roll up our sleeves and get ready to unlock the power of JSON communication!
Understanding JSON
JSON, which stands for JavaScript Object Notation, is a lightweight data interchange format that’s easy to read and write for humans, and easy to parse and generate for machines. It’s based on a subset of JavaScript language, but it’s used across many programming languages due to its simplicity and language-independent design.
Here’s a basic overview of JSON:
Syntax: JSON is written as key-value pairs, much like a JavaScript object. The keys are strings, and the values can be strings, numbers, objects, arrays, true, false, or null.
Data Types: The value of a key-value pair in JSON can be a string (in double quotes), number, object (another set of key-value pairs), array, true, false, or null.
Objects: JSON objects are enclosed in curly braces {}
and can contain multiple key-value pairs.
Arrays: JSON arrays are enclosed in square brackets []
and can contain a list of values or objects.
Nesting: JSON can have nested structures, meaning an object can contain another object or an array, and arrays can contain objects.
Here’s an example of a JSON object:
{
"name": "John Doe",
"age": 30,
"isEmployed": true,
"address": {
"street": "123 Main St",
"city": "Anytown"
},
"phoneNumbers": ["123-456-7890", "987-654-3210"]
}
In this example, name
, age
, and isEmployed
are simple key-value pairs, address
is a nested object, and phoneNumbers
is an array of strings.
JSON is widely used in web applications for sending data from a server to a client and vice versa. It’s also commonly used for configuration files and data storage.
Why Post JSON Data with PHP?
Posting JSON data with PHP is common when dealing with web services and APIs, especially in scenarios where you’re sending data from a client to a server. PHP provides several functions that make it easy to handle JSON data, allowing you to encode, transmit, and decode it.
Here are some reasons why you might want to post JSON data with PHP:
- Interoperability: JSON is a language-independent data format. It’s widely supported across different programming languages, making it ideal for web services that interact with various systems.
- Human-readable: JSON is easily readable by humans, which simplifies debugging and development.
- Efficiency: JSON is a lightweight data format, which means it can reduce the amount of data that needs to be transferred compared to other formats like XML.
- Flexibility: JSON can represent complex data structures, including nested arrays and objects, which is useful for passing structured data.
- Compatibility: Most modern web APIs use JSON as their communication format, so using PHP to post JSON data ensures compatibility with these services.
By mastering the art of posting JSON data with PHP, you'll be able to seamlessly integrate your applications with a plethora of third-party services and APIs. From social media platforms to payment gateways, and everything in between, JSON is the language that keeps the digital world connected.
Moreover, JSON is incredibly versatile. It can be used for various purposes, such as sending data to a server, retrieving data from an API, or even storing data in a database (although, for that, you might want to consider using a more suitable format like SQL or NoSQL databases).
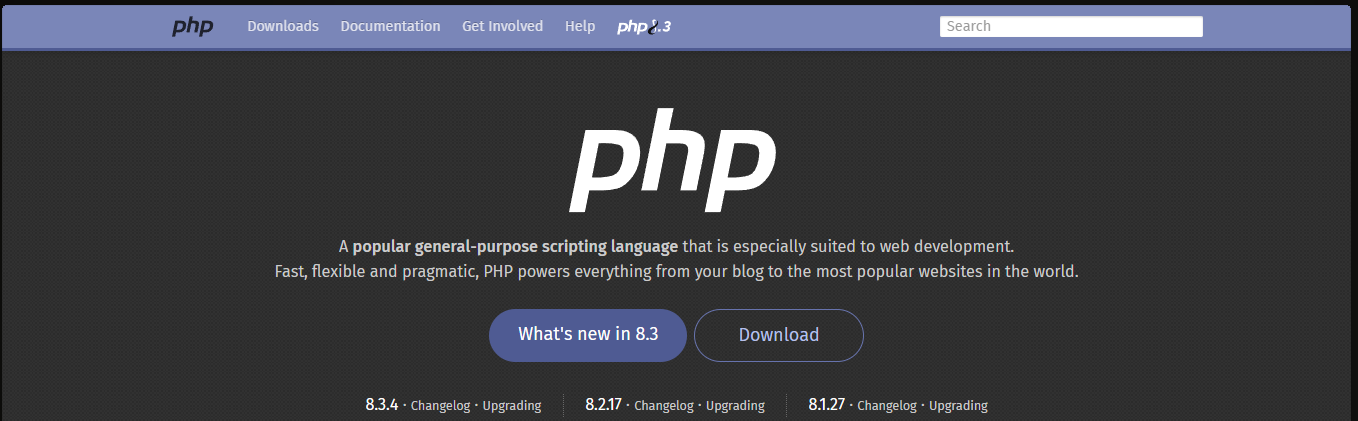
Setting the Stage (Preparing to Post JSON Data with PHP)
Alright, now that we've covered the "why" and the "what" of JSON in PHP, let's get our hands dirty and prepare for the actual posting process. First things first, we need to make sure our PHP environment is set up correctly.
If you haven't already, install the latest version of PHP on your development machine or server. You can check if PHP is installed and which version you're running by executing the following command in your terminal or command prompt:
php --version
Once you've confirmed that PHP is installed and ready to go, it's time to familiarize yourself with a few key PHP functions that will help us work with JSON data:
json_encode()
: This function is used to convert a PHP array or object into a JSON string.json_decode()
: Conversely, this function is used to convert a JSON string into a PHP array or object.
These two functions will be our best friends throughout the JSON posting process.
How to Post JSON Data with PHP
Posting JSON data with PHP typically involves using the cURL
library or PHP’s built-in functions to send an HTTP POST request. Here’s a step-by-step guide on how to do it:
- Create the JSON data: You’ll need to create an array with your data and then convert it into a JSON string using
json_encode()
.
$data = array(
'key1' => 'value1',
'key2' => 'value2'
);
$json_data = json_encode($data);
- Initialize cURL: Set up a cURL session and provide the URL of the API endpoint you’re sending data to.
$url = 'http://example.com/api/endpoint';
$ch = curl_init($url);
- Set cURL Options: Configure the cURL options to send a POST request with your JSON data and include the necessary headers.
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $json_data);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json',
'Content-Length: ' . strlen($json_data)
));
- Execute the Request: Send the request and store the response.
$response = curl_exec($ch);
- Check for Errors: It’s good practice to check for errors in the cURL request.
if (curl_errno($ch)) {
throw new Exception(curl_error($ch));
}
- Close cURL Session: Once you’ve got your response, don’t forget to close the cURL session.
curl_close($ch);
- Decode the Response: If the response is in JSON format, use
json_decode()
to convert it into a PHP array.
$result = json_decode($response, true);
And that’s it! You’ve successfully posted JSON data with PHP. Remember to replace 'http://example.com/api/endpoint'
with the actual URL you’re sending data to and $data
with your actual data array.
Posting JSON Data with PHP using Apidog
Apidog provides a user-friendly interface that simplifies the process of crafting POST requests with JSON payloads, making it a helpful tool for testing and developing APIs that require JSON data within the request body.
To post JSON data with PHP using Apidog, you can follow these general steps:
Establish a New Project: In your project, initiate a new request
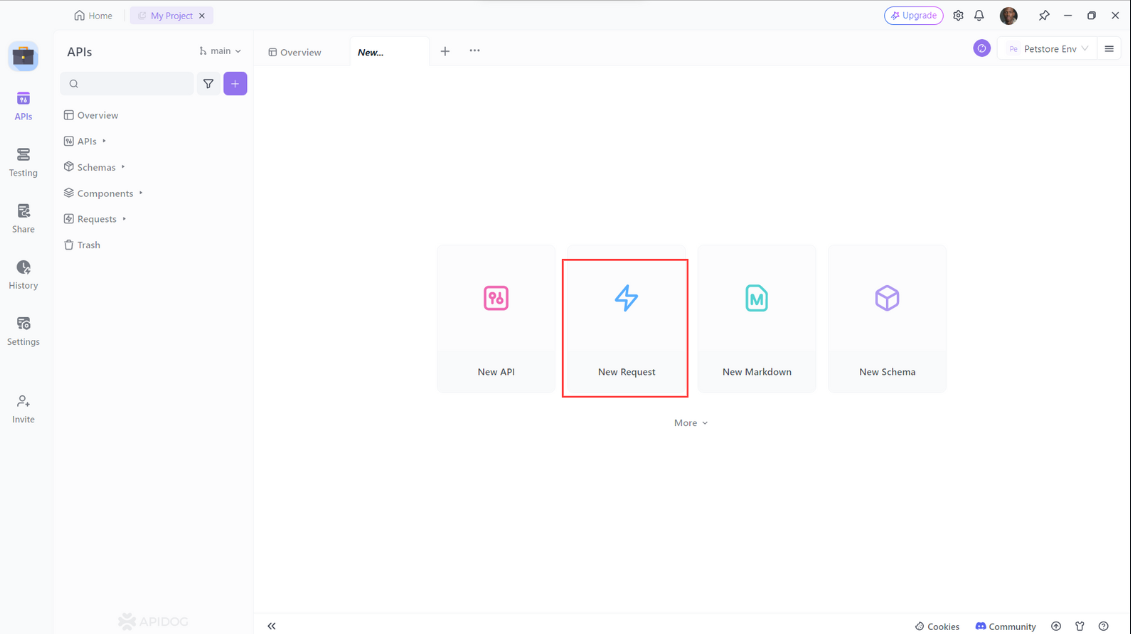
Enter POST Request Details: Select the request type as POST.
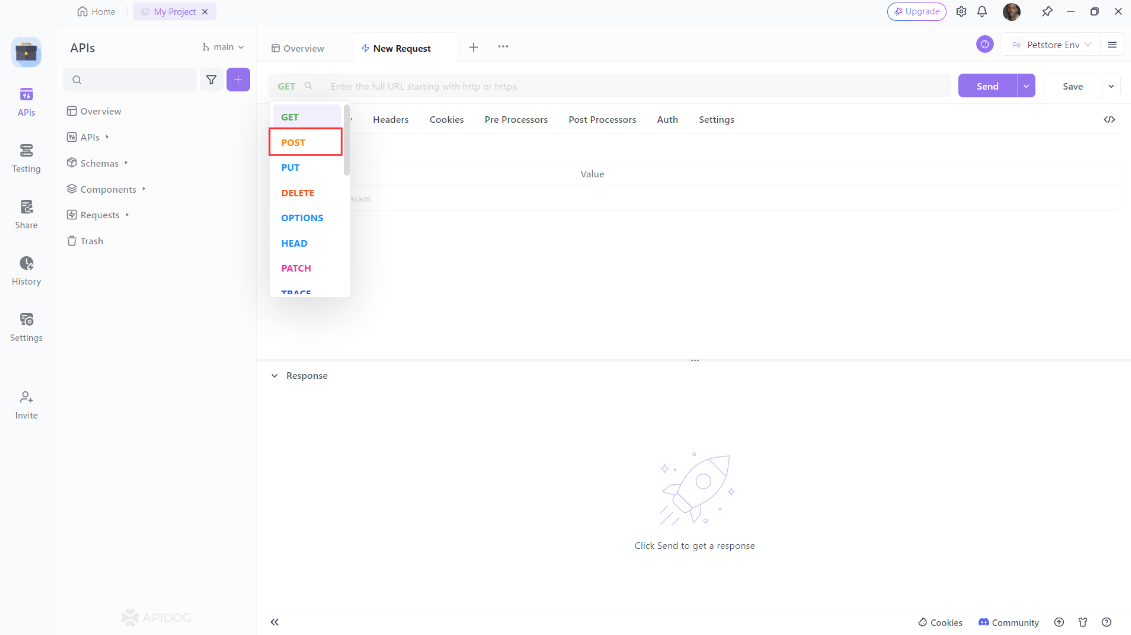
Input JSON Data: Navigate to the “Body” tab in your request setup, choose “json” as the format, and input the JSON data you wish to send.
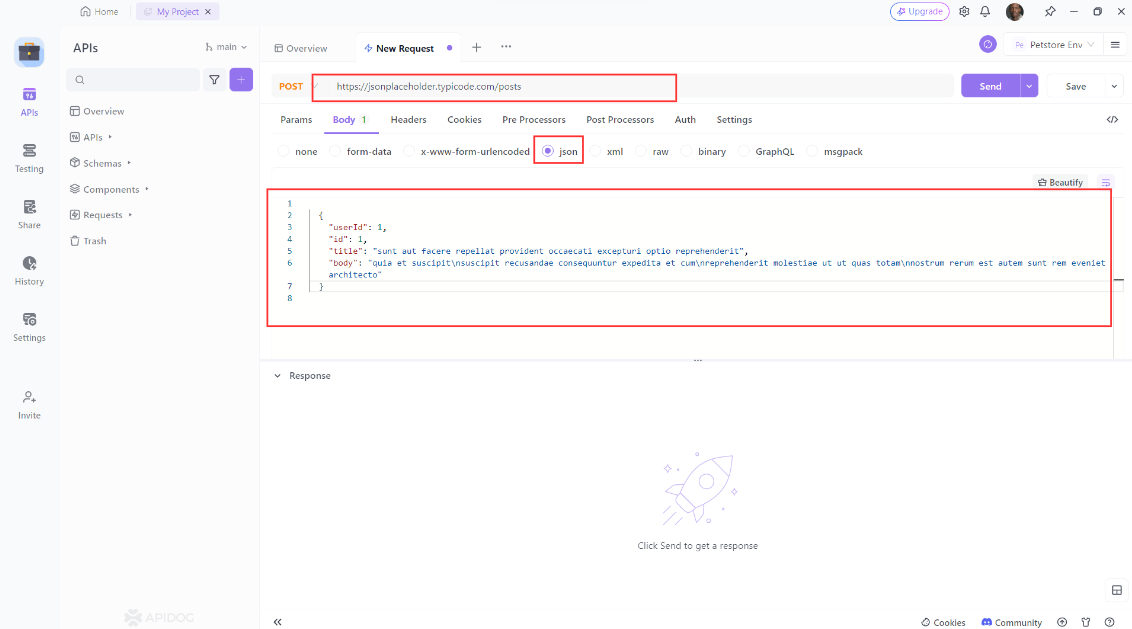
Send Post Request: After setting up your request with the JSON data, you can send the POST request and observe the response from the server.
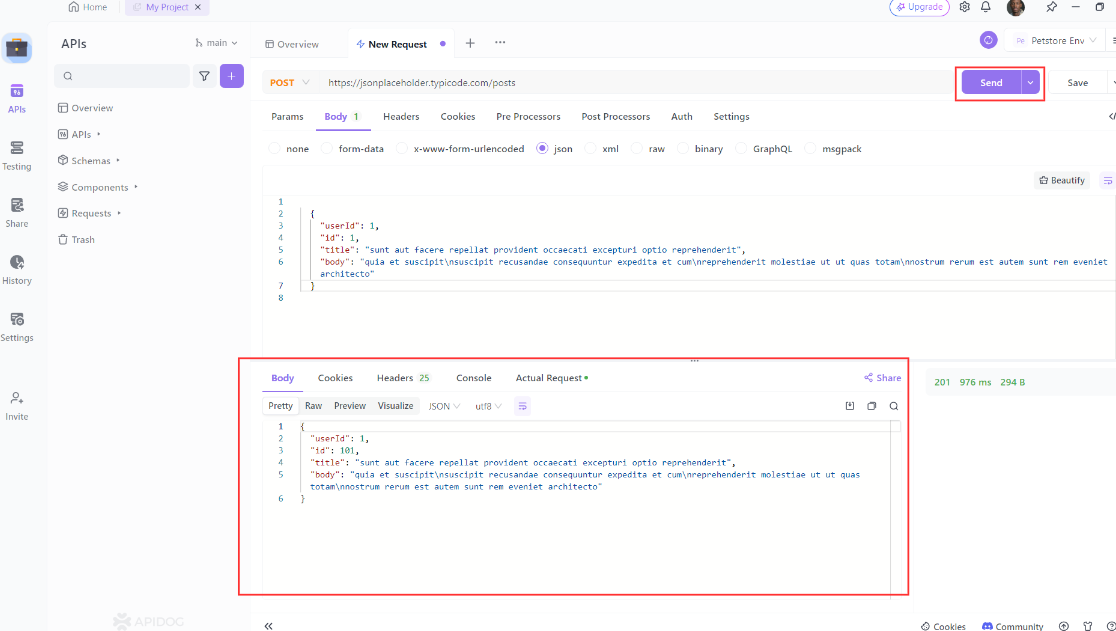
Conclusion:
In conclusion, JSON is a versatile and widely-used format for data interchange, particularly in web applications. Posting JSON data with PHP is a fundamental skill for web developers, enabling server-client communication and interaction with various APIs. Tools like Apidog can further simplify and streamline the process of testing and developing APIs that utilize JSON.
Remember, when working with JSON and PHP:
- JSON is text-based and language-independent, making it ideal for data interchange.
- PHP offers built-in functions for encoding and decoding JSON, as well as sending HTTP requests.
- Tools like Apidog provide a user-friendly interface for crafting and testing API requests.
Whether you’re building a new application or integrating with existing services, understanding how to work with JSON and PHP is crucial for modern web development. Keep exploring and practicing, and you’ll find these skills invaluable for your projects. Happy coding! 🚀