Modern web apps rely on back-and-forth data exchange between users and servers. This is what makes web interactions work. Luckily, NodeJS developers can use ExpressJS to easily handle this communication.
However, if you are considering using Apidog, you no longer have to worry about learning a whole new language! With Apidog, you get to enjoy a code generation feature that can help you with JavaScript and other programming languages!
To understand what Apidog can offer you, make sure to click the button below!
The phrase "NodeJS Express POST Requests" contains a couple of important terms. Without understanding them individually, you may easily get confused, so let us split them apart.
What is NodeJS?
NodeJS is an open-source, cross-platform runtime environment specifically designed for executing JavaScript code outside of the traditional web browser context. This document provides a comprehensive exploration of NodeJS, delving into its core functionalities and the paradigm shift it has introduced within the software development landscape.
NodeJS Key Features
Asynchronous and Event-Driven
NodeJS employs a single thread, but don't be fooled by the simplicity. It uses a clever approach called event-driven architecture. This means NodeJS efficiently juggles multiple requests like a waiter handling a busy restaurant. When a request arrives, it's registered as an event. NodeJS then keeps track of these events and triggers functions (callbacks) to process them as they become ready. This asynchronous nature allows NodeJS to excel at handling tasks that involve waiting, like network requests, without slowing down the entire system.
JavaScript on the Server
Traditionally, JavaScript's playground was the web browser, where it made web pages interactive. NodeJS breaks down this barrier by establishing a robust runtime environment that empowers JavaScript code execution outside the browser – on the server. This innovation is a game-changer for developers. They can now leverage their existing JavaScript knowledge for both front-end (user interface) and back-end (server-side logic) development, streamlining the entire process.
NPM
NPM, a built-in feature of NodeJS, acts as a central repository housing a vast collection of pre-built code modules and libraries. Developers can leverage NPM to search for specific functionalities they need, download them with ease, and integrate them into their applications. This extensive library ecosystem significantly accelerates development and empowers developers to incorporate a wide range of features without starting from scratch.
Single-Threaded Scalability with Non-Blocking I/O
While NodeJS utilizes a single thread, it achieves impressive scalability through a technique called non-blocking I/O. This means that NodeJS doesn't wait for slow tasks (like network requests) to finish before processing other requests. Instead, it cleverly moves on to the next request while keeping track of the pending I/O operation. Once the slow task is done, NodeJS circles back and handles the response.
Built-in HTTP Muscle
NodeJS comes pre-equipped with all the necessary tools for building web servers. Built-in HTTP modules simplify the development process by allowing developers to create robust HTTP servers and handle various HTTP methods (GET, POST, PUT, DELETE) with ease.
Thriving Open-Source Community
NodeJS benefits from a large and active open-source community. This translates to a wealth of resources readily available for developers. Extensive documentation, tutorials, and forums are just a few examples of the support offered by the community. Additionally, the open-source nature allows anyone to contribute to the project's growth and benefit from the collective knowledge and expertise of the community.
What is Express?
Express, also known as ExpressJS, is a prominent web application framework built upon the foundation of NodeJS. It serves as a powerful abstraction layer, offering a higher level of development compared to the raw capabilities of NodeJS. This abstraction translates to a significant reduction in boilerplate code, accelerating the development process for web applications and APIs.

ExpressJS Key Features
Simplified Development with Abstractions
NodeJS offers a powerful platform for server-side JavaScript execution, but it can involve writing low-level code for common web application functionalities. ExpressJS acts as an abstraction layer, providing pre-built features and structures that streamline development. This translates to less boilerplate code and a focus on the core logic of your application.
Elegant Routing System
ExpressJS boasts a robust routing system that allows developers to define routes using clear and concise methods. You can define routes for different URLs (e.g., "/products", "/about-us") and associate them with specific functions (handlers) that handle the user's request for that particular page. This clear separation of concerns promotes code organization and maintainability.
Modular Functionality with Middleware
Middleware a powerful concept that allows developers to define functions that act as intermediaries between incoming requests and outgoing responses. Each middleware function can perform specific tasks like logging requests, parsing data from the request body (e.g., form data, JSON), or implementing authentication checks. Middleware functions are chained together, and requests pass through this chain, allowing for modular and reusable functionality across your application.
Dynamic Content with Templating Engine
Web applications often require the generation of dynamic content based on user input or data retrieval. ExpressJS integrates seamlessly with various templating engines like Pug (formerly Jade) or EJS (Embedded JavaScript). These engines provide developers with a way to define templates that act as blueprints for HTML pages. The templates can contain placeholders for dynamic content. At runtime, ExpressJS fills these placeholders with data from your application, generating the final HTML content that gets delivered to the user's browser.
Built-in HTTP Utilities
ExpressJS leverages the built-in HTTP modules that come with Node.js. This inheritance empowers developers to readily create web servers, handle HTTP requests and responses, and manage various HTTP methods (GET, POST, PUT, DELETE) with ease. You don't need to write complex code from scratch to set up basic server functionalities.
Extensive Third-Party Library Ecosystem
Just like Node.js thrives with NPM (Node Package Manager), ExpressJS benefits from a vast ecosystem of third-party libraries and tools. These pre-built modules can be easily integrated into your ExpressJS application, extending its functionality and adding features like user authentication, database interactions, file uploads, or real-time messaging.
How to Make NodeJS Express POST Requests?
This section will guide you on how to start making POST requests with NodeJS Express!
Prerequisites
Firstly, you have to ensure NodeJS and npm (Node Package Manager) are installed on your system. You can verify their installation by running node -v
and npm -v
in your terminal.
- Create Project Directory: Create a new directory for your project using your terminal. Navigate to the desired location and run
mkdir my-express-app
to create the directory. - Initialize Project: Inside the newly created directory, initialize a new project using npm by running
npm init -y
. This will create apackage.json
file for your project.
Step 1 - Install ExpressJS
Now that your project is set up, let's install ExpressJS. In your terminal, within the project directory, run the following line of code. This will download and install ExpressJS as a dependency for your project.
npm install express
Step 2 - Create a Server File
Create a new JavaScript file named server.js
inside your project directory. This will hold the code for your Express server.
Step 3 - Import Express and Define the App
Open your server.js
file in your code editor and include the following line of code:
const express = require('express');
Then, create an Express application instance:
const app = express();
Step 4 - Define the Route and Handle POST Requests:
Use the app.post
method to specify the URL path and the function that will handle the request:
app.post('/your-endpoint', (req, res) -> {
// Handle POST request logic here
});
When implementing the code snippets, make sure to replace /your-endpoint
with the actual URL path where you intend to receive POST requests.
Functions passed to app.post
takes two arguments:
req
: The request object that contains information about the incoming request, including the data sent in the body.res
: The response object that you can use to send a response back to the client.
Step 5 - Accessing POST Data
Inside the function handling the POST request, you can access the data sent in the request body using the req.body
object - it is a JavaScript object containing the key-value pairs of the data sent in the request body.
app.post('/your-endpoint', (req, res) => {
const name = req.body.name; // Assuming data has a key 'name'
const message = req.body.message; // Assuming data has a key 'message'
// Process the data here (e.g., store in database, send an email)
res.send('POST request received successfully!');
});
Step 6 - Sending a Response
After processing the data from the POST request, you can send a response back tot he client using the res
object.
app.post('/your-endpoint', (req, res) => {
// ... (process data)
res.send('POST request received successfully!'); // Send a simple message
// OR
res.json({ message: 'Data received and processed successfully!' }); // Send JSON data
});
Step 7 - Start the Server
Lastly, start the server by listening on a specific port using the app.listen
method. Usually, the port number used for development is 3000, as shown in the code sample below:
app.listen(3000, () => {
console.log('Server listening on port 3000!');
});
Apidog - Speed Up Express GET Requests With Code Generation
Speed up API and app development by considering using Apidog, a comprehensive API development platform that has a code generation feature for the JavaScript programming language.
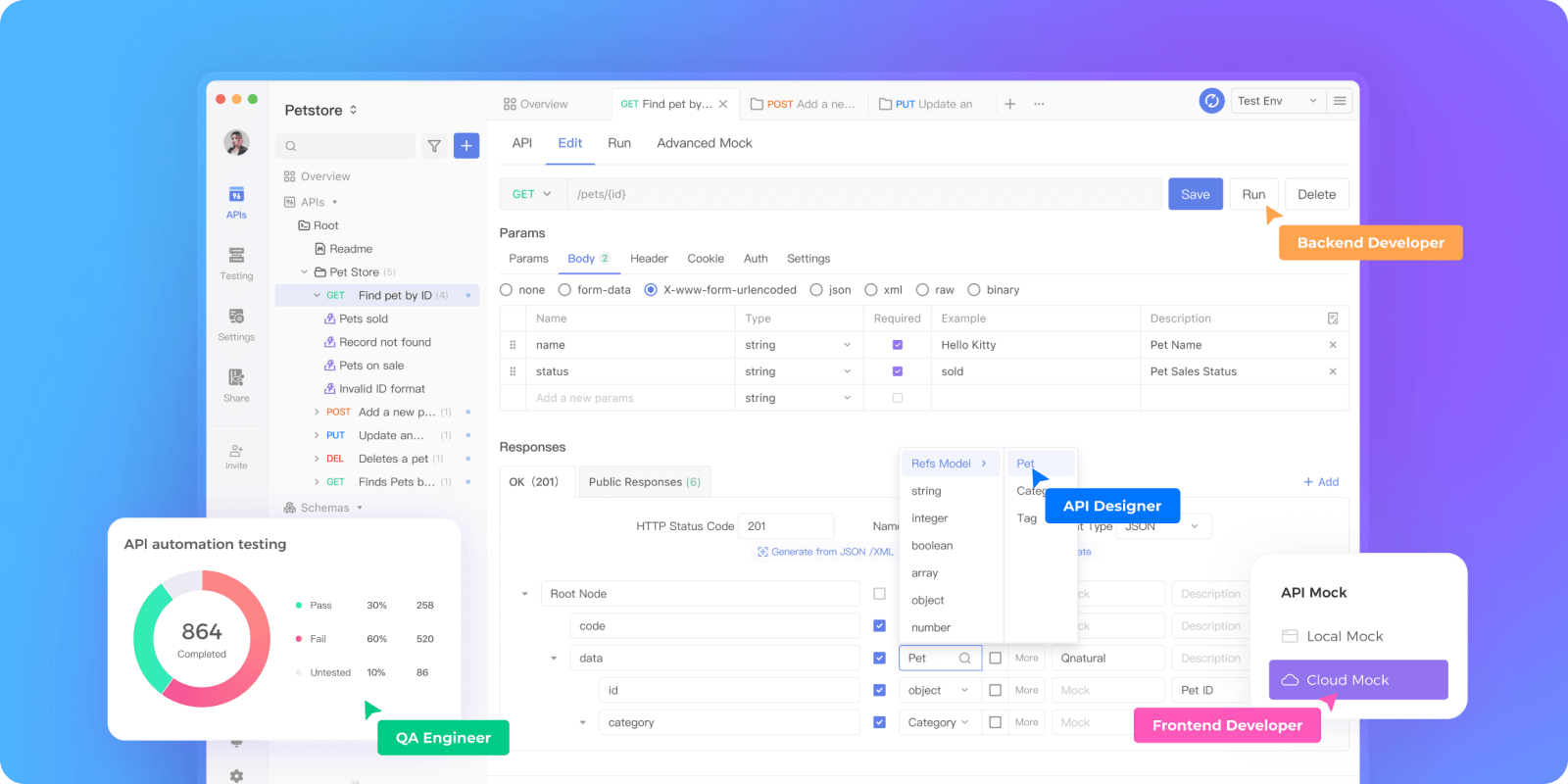
Let's take a look at how you can generate JavaScript client code in just a few clicks.
Quickly Generate JavaScript Code for Your App with Apidog
This section will highlight how you can generate JavaScript (or other available programming languages available) code for your app.
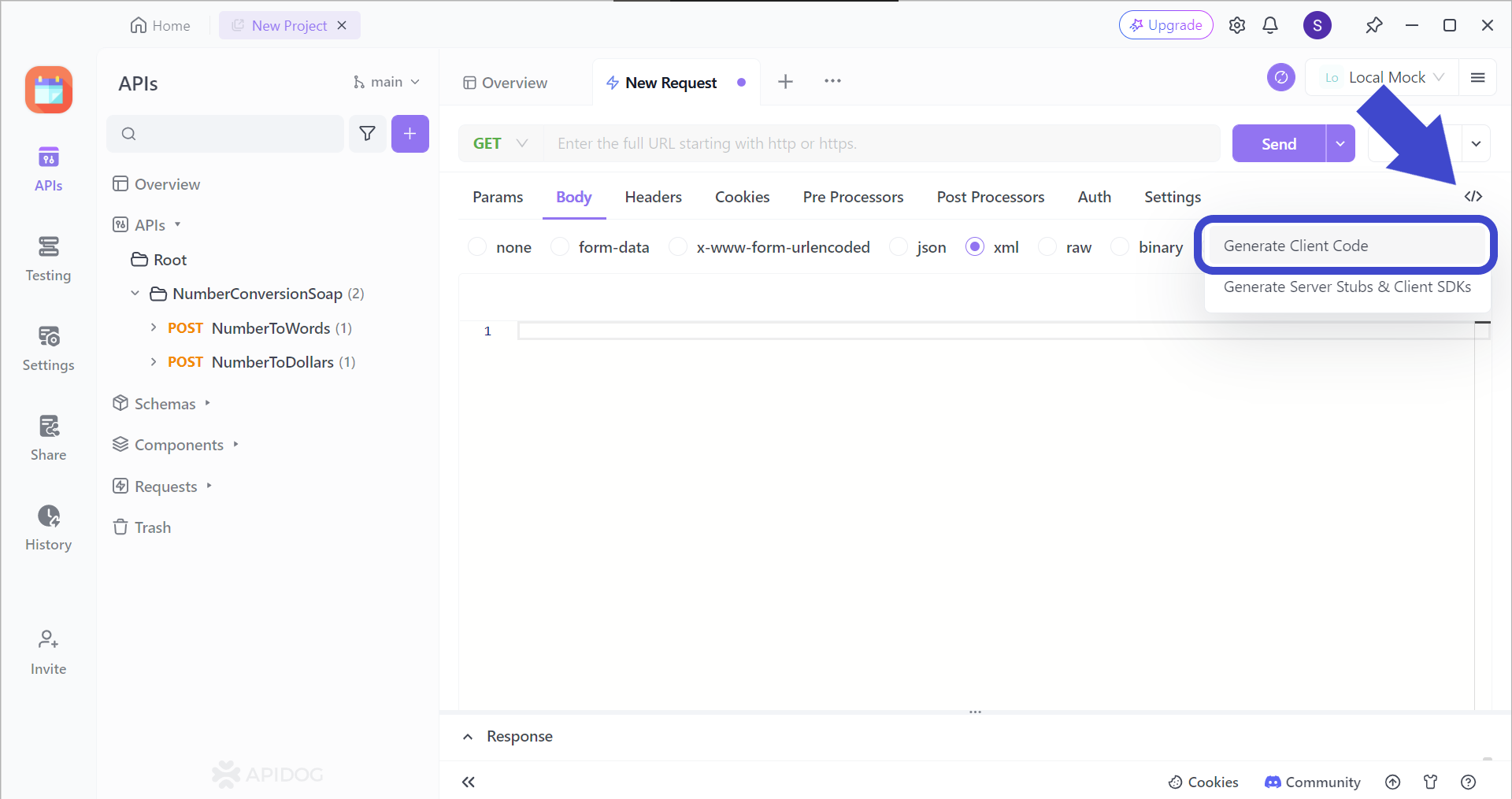
First, locate the </>
button found around the top right corner of the screen. If you are struggling to locate the button, you can refer to the image above.
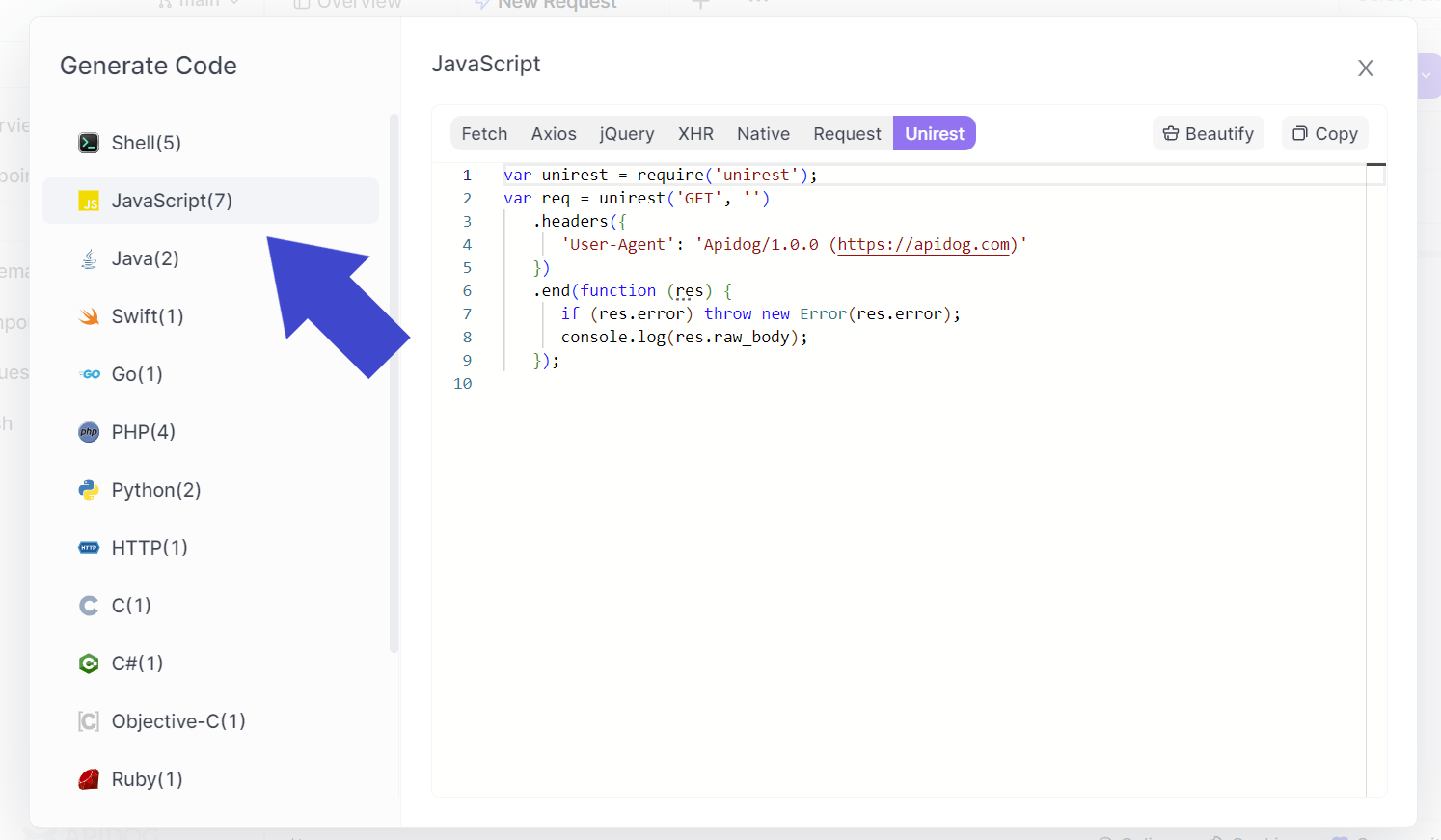
Proceed by selecting the client-side programming language that you need. You have the power to choose which JavaScript library you are more comfortable with.
All you need to do is copy and paste the code to your IDE, and continue with editing to ensure it fits your NodeJS app! However, if you're keen on using Unirest as shown in the image above, you can refer to the article here:
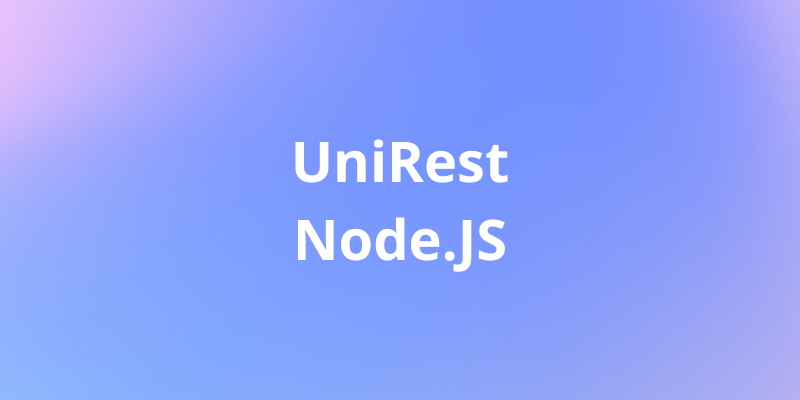
Test Out APIs with Apidog
Apidog provides developers with a comfortable yet beautiful user interface for debugging APIs.
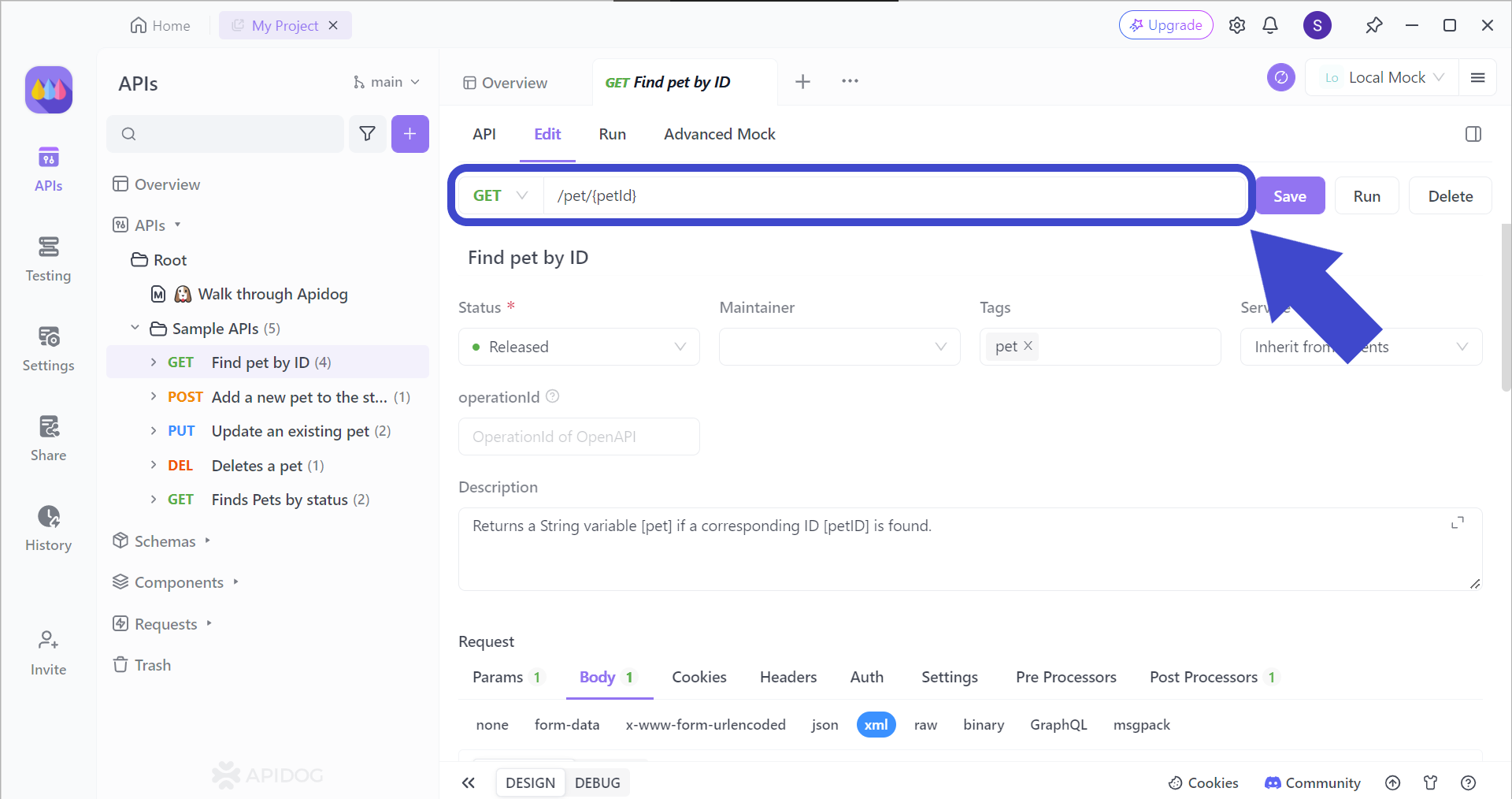
Provide the API endpoint in the bar highlighted by the arrow in the image above.
If you're unsure about using multiple parameters in a URL, this article can guide you on how to hit the exact resource within larger datasets!
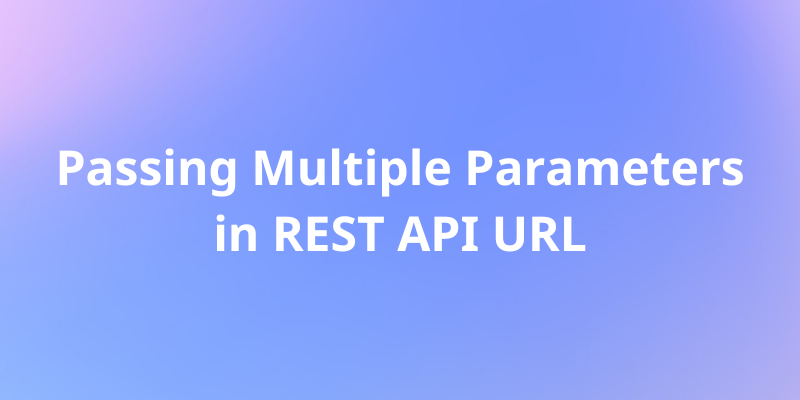
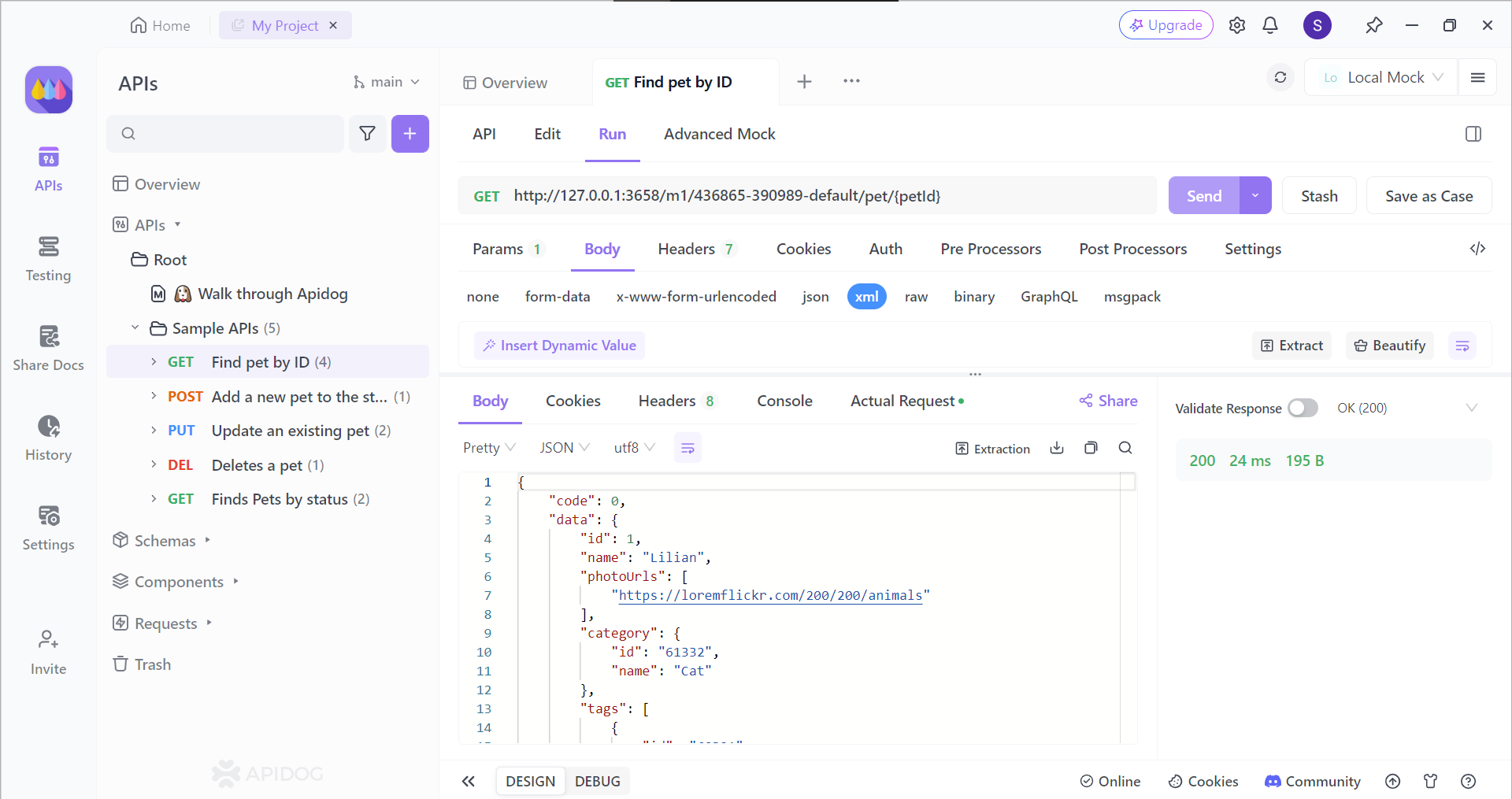
Once you are sure of your API, you can click Send
, which sends the request to the API. You should expect a complete response. A glance at the status code tells you if it was successful. You can also examine the raw response, which shows the exact data structure your code needs to understand the information from the API's servers.
Conclusion
NodeJS and Express provide a powerful combination for building modern web applications that interact with data. By leveraging Express's routing system and middleware capabilities, developers can efficiently handle POST requests, the workhorse of form submissions, and data exchange on the web. With tools like body-parser
for parsing request bodies, accessing and processing data becomes straightforward.
This empowers developers to create applications that can collect user input, store data in databases, or trigger actions based on received information. The ease of use and flexibility offered by NodeJS and Express make them a compelling choice for developers looking to build dynamic and data-driven web applications.