Mastering API Responses: The Definitive Guide to JSON Formatting
Dive into the essentials of API response JSON format with our expert guide. Discover best practices, parsing techniques, and practical applications to optimize your API development for seamless integration and efficiency.
APIs (Application Programming Interfaces) have emerged as the cornerstone of software development, enabling disparate systems to communicate and share data seamlessly. As we delve into the world of APIs, one aspect that stands out is the format of the responses they return. The JSON (JavaScript Object Notation) format has become the de facto standard for API responses, prized for its simplicity, ease of use, and language-agnostic nature.
In this blog post, we’ll explore the intricacies of API response JSON format, often referred to as the lingua franca of the web. We’ll uncover why developers favor it, how it streamlines the process of data interchange, and the best practices for structuring JSON responses. Whether you’re a seasoned developer or just starting out, understanding the nuances of JSON will enhance your ability to design, consume, and debug APIs.
Understanding APIs and Their Significance
APIs, or Application Programming Interfaces, are the unsung heroes of our connected world. They are the conduits through which different software applications exchange data and functionalities, making them integral to the seamless operation of the digital ecosystem.
At their core, APIs are sets of rules and protocols that dictate how software components should interact. They enable developers to tap into existing services and platforms, leveraging their capabilities without having to reinvent the wheel. This not only saves time and resources but also fosters innovation by allowing for the integration of diverse technologies.
The significance of APIs cannot be overstated. They are the building blocks of modern software development, powering everything from web applications to mobile apps, and from cloud services to IoT devices. APIs facilitate the interoperability between systems, making it possible for your favorite apps to communicate with one another, share data, and offer a cohesive user experience.
The Role of JSON in API Responses
JSON, or JavaScript Object Notation, plays a pivotal role in API responses due to its lightweight nature and easy readability. It serves as a universal language for data interchange between servers and web applications.
Why JSON?
- Human-readable: JSON is self-describing and easy to understand, even for those who are not developers.
- Lightweight: Its simplicity allows for quick parsing and a smaller data footprint compared to other formats like XML.
- Language-agnostic: JSON is supported by most programming languages, making it highly versatile for backend and frontend development.
JSON in Action
When an API is called, the server responds with a JSON-formatted text that represents the data requested. This could be anything from user information to a list of products. The JSON format ensures that this data can be easily parsed by the client application and used as needed.
For example, a simple API response in JSON format might look like this:
{
"userId": 1,
"userName": "apidog",
"email": "apidog@example.com"
}
In this snippet, we see a user object with properties and values encoded in a way that’s both easy to read and easy to process programmatically.
The Impact of JSON on APIs
The adoption of JSON has streamlined the development process, enabling faster, more efficient, and more reliable data exchange. It has become the backbone of RESTful APIs, which are the standard for web services today.
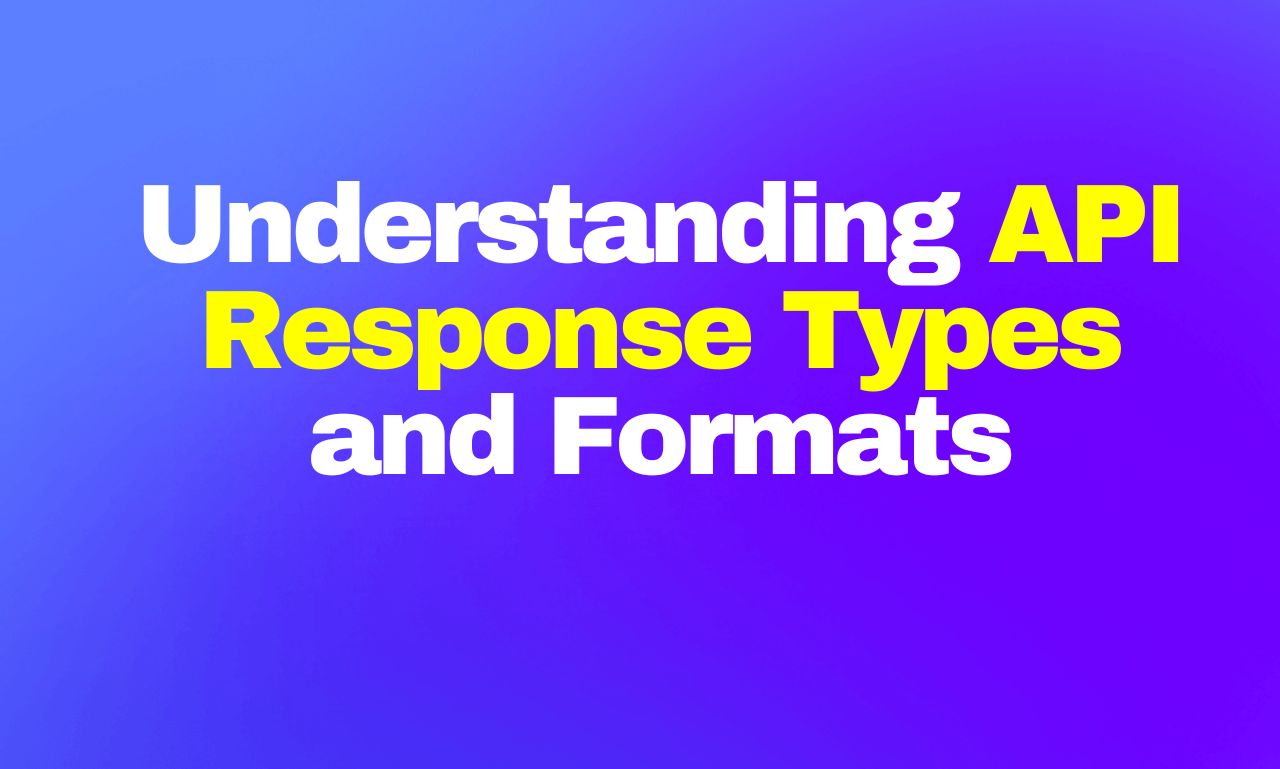
Anatomy of an API Response in JSON Format
The anatomy of an API response in JSON format is akin to the structure of a well-organized document. It consists of key-value pairs that represent data in a structured, hierarchical manner. Let’s dissect this anatomy to understand its components better.
Root Element
At the highest level, a JSON response typically starts with a root element, which can be either an object or an array. An object is denoted by curly braces {}
, while an array is denoted by square brackets []
.
Objects
An object represents a single entity and contains one or more key-value pairs. The keys are strings, and the values can be strings, numbers, objects, arrays, true
, false
, or null
.
Arrays
An array is an ordered collection of values, which can be of any type, including objects and other arrays.
Key-Value Pairs
The key-value pairs within an object are the fundamental units of data representation in JSON. They are separated by commas, and the key and value are separated by a colon.
Example of a JSON API Response:
{
"user": {
"id": 1,
"name": "apidog",
"email": "apidog@example.com",
"roles": ["admin", "user"],
"profile": {
"bio": "Lover of APIs",
"website": "https://apidog.com"
}
},
"status": "success",
"message": "User data retrieved successfully"
}
In this example:
- The root element is an object.
- The object contains three key-value pairs:
user
,status
, andmessage
. - The
user
key contains an object with its own nested key-value pairs. - The
roles
key within theuser
object contains an array of values. - The
profile
key within theuser
object contains another object.
Understanding the structure of a JSON API response is crucial for developers as it allows them to parse the data correctly and integrate it into their applications. It’s the clarity and predictability of this structure that make JSON an invaluable format in the world of APIs.
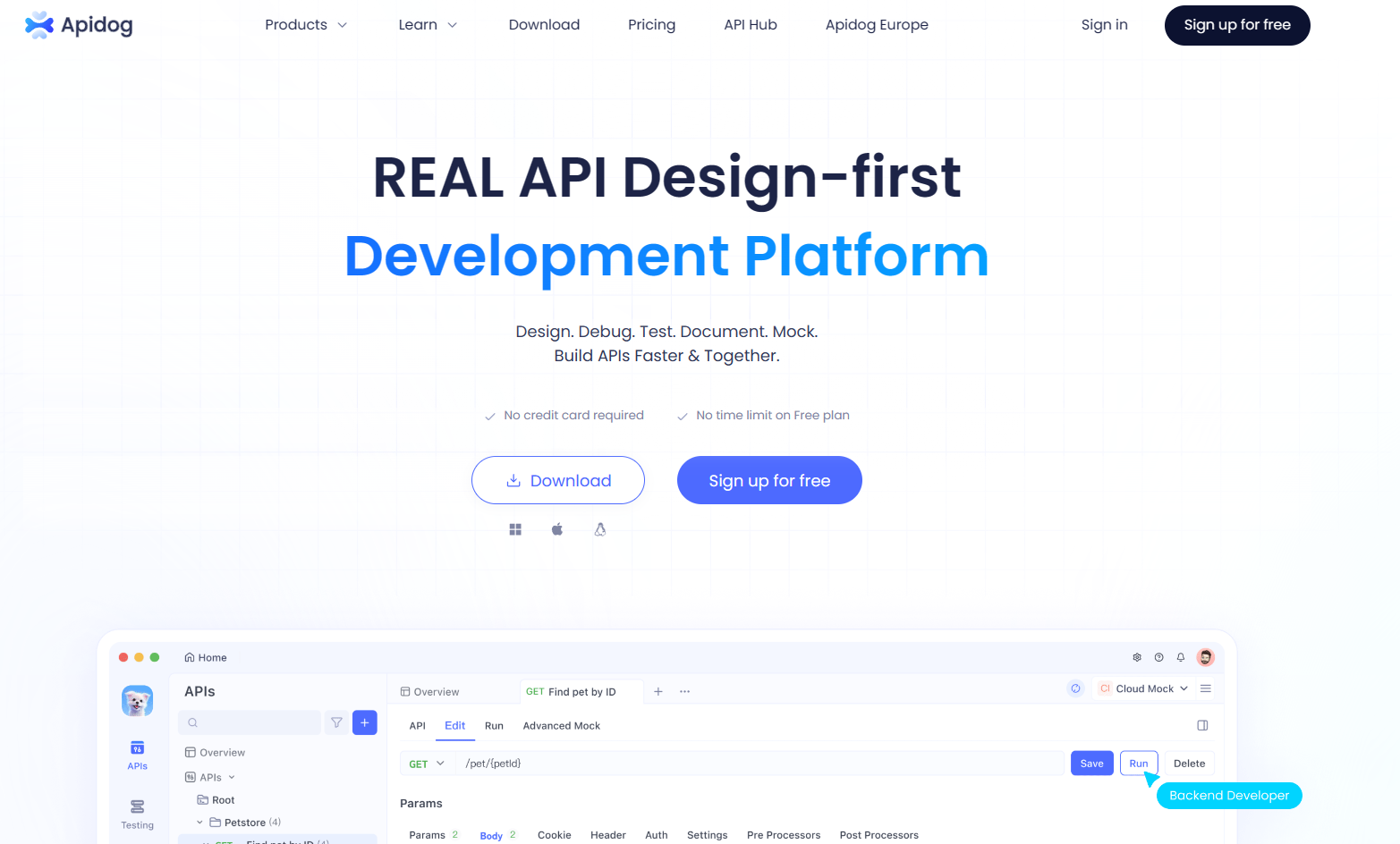
JSON API Responses in Apidog
The API response JSON format in Apidog is designed to be intuitive and easy to work with for developers.
It typically includes the following components:
- Status Code: Indicates the result of the API call, such as success or error.
- Headers: Provide metadata about the response, like content type and cache directives.
- Body: Contains the actual data payload, formatted as a JSON object or array.
For example, a successful response from an API might look like this:
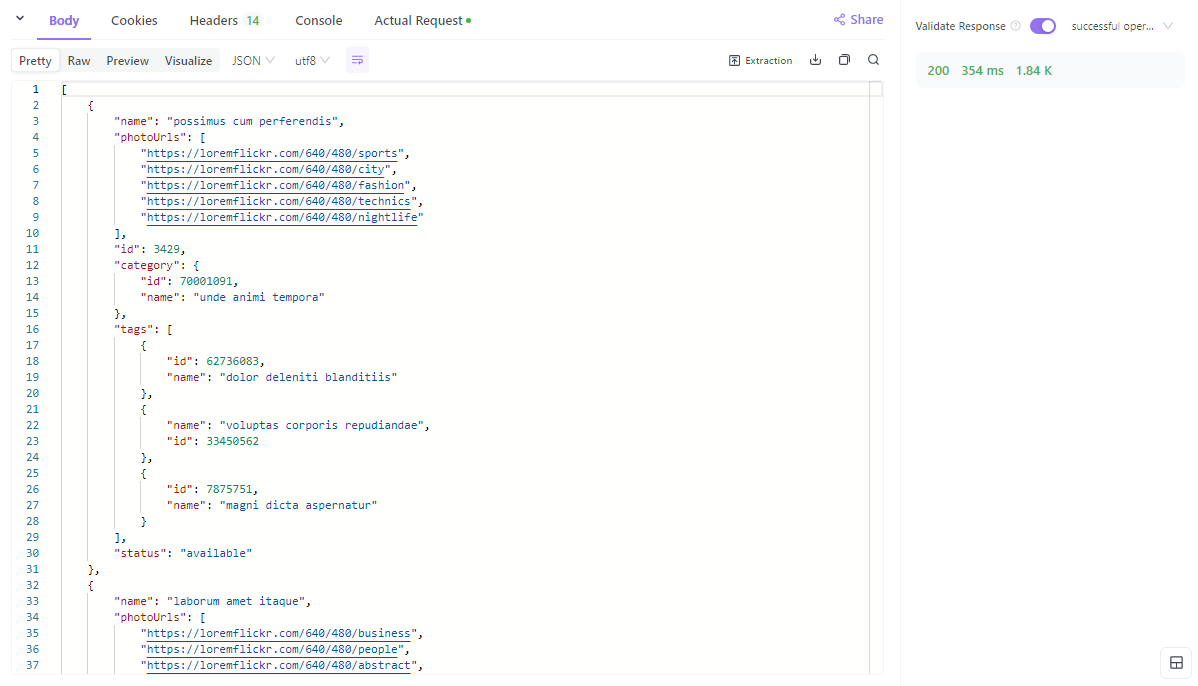
Apidog emphasizes clear documentation and structured responses to ensure efficient data exchange and error handling.
Best Practices for Structuring JSON API Responses
When structuring JSON API responses, adhering to best practices is crucial for ensuring that the data is easily consumable and maintainable. Here are some guidelines to follow:
1. Keep it Intuitive and Consistent
- Use clear, descriptive key names that accurately reflect the data they hold.
- Maintain a consistent structure across all API endpoints to avoid confusion.
2. Nest Sparingly
- While JSON allows for nesting, overdoing it can make the response complex and harder to parse. Limit nesting to what’s necessary for logical grouping.
3. Use HTTP Status Codes
- Leverage HTTP status codes to indicate the success or failure of an API request. This helps clients handle responses appropriately.
4. Provide Meaningful Error Messages
- In case of an error, include a message that explains what went wrong and possibly how to fix it.
5. Pagination for Large Data Sets
- For endpoints that can return large data sets, implement pagination to improve performance and usability.
6. HATEOAS (Hypermedia as the Engine of Application State)
- Consider using HATEOAS principles to include hyperlinks in your API responses, guiding clients through the available actions.
7. Security
- Be mindful of sensitive data. Ensure that private information is not exposed unintentionally in your API responses.
8. Documentation
- Document your API responses thoroughly. Clear documentation is invaluable for developers who will consume your API.
By following these best practices, you can create JSON API responses that are not only functional but also a pleasure to work with. Remember, the goal is to make the data exchange process as smooth and efficient as possible for all parties involved.
Parsing and Utilizing JSON API Responses
Parsing JSON API responses is a fundamental skill for developers working with APIs. It involves converting the JSON-formatted string received from an API into a data structure that can be manipulated and used within an application.
Parsing JSON in Different Languages:
JavaScript:
const jsonResponse = '{"name": "apidog", "age": 5}';
const parsedData = JSON.parse(jsonResponse);
console.log(parsedData.name); // Output: apidog
Python:
import json
json_response = '{"name": "apidog", "age": 5}'
parsed_data = json.loads(json_response)
print(parsed_data['name']) # Output: apidog
Java:
import org.json.JSONObject;
String jsonResponse = "{\"name\": \"apidog\", \"age\": 5}";
JSONObject parsedData = new JSONObject(jsonResponse);
System.out.println(parsedData.getString("name")); // Output: apidog
Utilizing Parsed Data:Once the JSON response is parsed, the data can be used in various ways depending on the application’s requirements. For instance:
- Displaying user information on a profile page.
- Processing a list of products in an e-commerce app.
- Configuring settings in a software application based on user preferences.
Best Practices for Parsing and Utilization:
- Error Handling: Always include error handling when parsing JSON to manage unexpected or malformed data.
- Data Validation: Validate the parsed data to ensure it meets the expected format and type.
- Efficient Data Access: Access the data in a way that is efficient and does not hinder application performance.
Conclusion
In essence, JSON’s role in API responses is a testament to its efficiency and adaptability, making it an industry standard. By following best practices in JSON formatting, developers can ensure their applications are robust and user-centric. Apidog stands as a prime example of these principles in action, providing a clear path for developers to master API responses and build the interconnected applications of tomorrow.