How to Make a PUT Request with curl_init()?
Utilize the curl_init() function to construct a data payload and ensure secure data delivery for your PUT requests.
The software application cURL, formally known as "Client for URLs," provides developers with a robust toolkit for managing data transfers. It operates in two distinct modes: a user-friendly command-line interface that caters to fundamental interactions and a powerful library designed for seamless integration into programming projects.
To understand Apidog better, try it out for yourself by clicking the button below!
Formal Definition of curl_init()
Based on the official PHP website, the curl_init function initializes a cURL session and returns a cURL handle for use with the curl_setopt(), curl_exec(), and curl_close() functions.
Parameters Involved
url
If you provide a URL, the CURLOPT_URL
option will be set to its value. You can also manually set this option using the curl_setopt() function.
However, do note that the file
protocol is disabled by cURL itself if the open_basedir
has been set.
Return Values
The curl_init() function returns a cURL handle on success, and false
on errors.
What is a PUT Request?
The PUT request within the Hypertext Transfer Protocol (HTTP) acts as a refined tool for managing resources on a web server. It deviates from its counterpart, the GET request (used for data retrieval), by specifically targeting modifications on the server side.
PUT Requests' Function
Unlike its counterpart, the GET request used for retrieving data, a PUT request focuses on modifying information on the server side. This modification can encompass two main actions:
- Updating Existing Resources: If a resource (like a user account or a product in a database) already exists at a specific URL, a PUT request can be used to update its associated data.
- Creating New Resources: In scenarios where a resource doesn't exist at the designated URL, a PUT request can be employed to create a new resource with the provided data.
Data Placement
PUT requests typically transmit data within the request body, similar to POST requests. This data often represents the new or updated state of the resource being targeted.
Idempotence
A defining characteristic of PUT requests is their idempotent nature. This implies that executing a PUT request multiple times with identical data will produce the same outcome. In simpler terms, sending a PUT request once or several times with the same data leads to the same result on the server.
PUT Requests' Common Use Cases
- Updating user profiles or account information
- Modifying existing content in a database (e.g., editing a blog post)
- Uploading a new file to a specific location on the server
Code Examples of Making PUT Requests with curl_init() Function
Here are some PHP coding examples that you can refer to if you wish to make PUT requests with the curl_init()
function.
Example 1 - Updating User Information (JSON Data)
This example updates a user's profile information on a server using JSON data.
<?php
$url = "https://www.example.com/api/users/123"; // Replace with actual user ID
$new_email = "new_email@example.com";
$data = array(
"email" => $new_email
);
$data_json = json_encode($data);
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "PUT"); // Set request method to PUT
curl_setopt($ch, CURLOPT_POSTFIELDS, $data_json); // Set PUT data as JSON
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); // Return the response
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json', // Set content type to JSON
'Authorization: Bearer YOUR_API_KEY' // Add authorization header
));
$response = curl_exec($ch);
curl_close($ch);
if($response) {
echo "User profile updated successfully!";
} else {
echo "Error: " . curl_error($ch);
}
?>
Code explanation:
- We define the target URL (including the user ID) and the new email address.
- We create a
$data
array containing the updated email and convert it to JSON usingjson_encode
. - We initialize the curl handle and set the request method to PUT using
CURLOPT_CUSTOMREQUEST
. - We set the PUT data with
CURLOPT_POSTFIELDS
and set the content type header to JSON. - We add an authorization header with your API key for secure access.
- We execute the request, close the handle, and check for a successful response.
Example 2 - Uploading a File (Raw Data)
This example uploads a file to a server using raw data.
<?php
$url = "https://www.example.com/api/uploads";
$filename = "myfile.txt";
$file_content = file_get_contents($filename); // Read file contents
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "PUT"); // Set request method to PUT
curl_setopt($ch, CURLOPT_PUT, 1); // Set PUT upload mode
curl_setopt($ch, CURLOPT_INFILE, fopen($filename, "rb")); // Set file for upload
curl_setopt($ch, CURLOPT_INFILESIZE, filesize($filename)); // Set file size
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); // Return the response
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/octet-stream' // Set content type for raw data
));
$response = curl_exec($ch);
curl_close($ch);
if($response) {
echo "File uploaded successfully!";
} else {
echo "Error: " . curl_error($ch);
}
?>
Code explanation:
- We define the upload URL and filename.
- We read the file contents using
file_get_contents
. - We initialize the curl handle and set the request method to PUT.
- We enable PUT upload mode with
CURLOPT_PUT
. - We specify the file for upload using
CURLOPT_INFILE
and its size withCURLOPT_INFILESIZE
. - We set the content type header to indicate raw data.
- We execute the request, close the handle, and check for a successful response.
Example 3 - Conditional PUT with ETag
This example demonstrates a conditional PUT request using an ETag for optimistic locking.
<?php
$url = "https://www.example.com/api/posts/123";
$new_title = "Updated Title";
$etag = "some-etag-value"; // Replace with actual ETag
$data = array(
"title" => $new_title
);
$data_json = json_encode($data);
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "PUT"); // Set request method to PUT
curl_setopt($ch, CURLOPT_POSTFIELDS, $data_json); // Set PUT data as JSON
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); // Return the response
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json', // Set content type to JSON
'If-Match: ' . $etag // Add ETag for conditional update
));
$response = curl_exec($ch);
curl_close($ch);
if($response) {
echo "Post updated successfully!";
} else {
$error = curl_error($ch);
if (strpos($error, '412 Precondition Failed') !== false) {
echo "Update failed: ETag mismatch (data may have been modified by another user).";
} else {
echo "Error: " . $error;
}
}
?>
Code explanation:
- We define the target URL (post ID), the new title, and the ETag retrieved from the server.
- We create a
$data
array and convert it to JSON. - We set the request method to PUT and included the PUT data.
- We add an
If-Match
header with the ETag to ensure the update occurs only if the current server-side version matches the ETag. This prevents overwriting changes made by another user (optimistic locking). - We check the response for success and handle a specific error (412 Precondition Failed) indicating an ETag mismatch.
Note to Remember
Please ensure that the code samples above are not copied and pasted into your IDEs, as they are simplified and will require further modifications to fit your application's needs.
For the official documentation, you can check it out at: https://www.php.net/manual/en/book.curl.php
Fast-track Your cURL API Development with Apidog
Apidog is a sophisticated API development platform capable of converting cURL command lines into beautiful and intuitive graphical displays, making it easier for developers to understand and work around APIs.
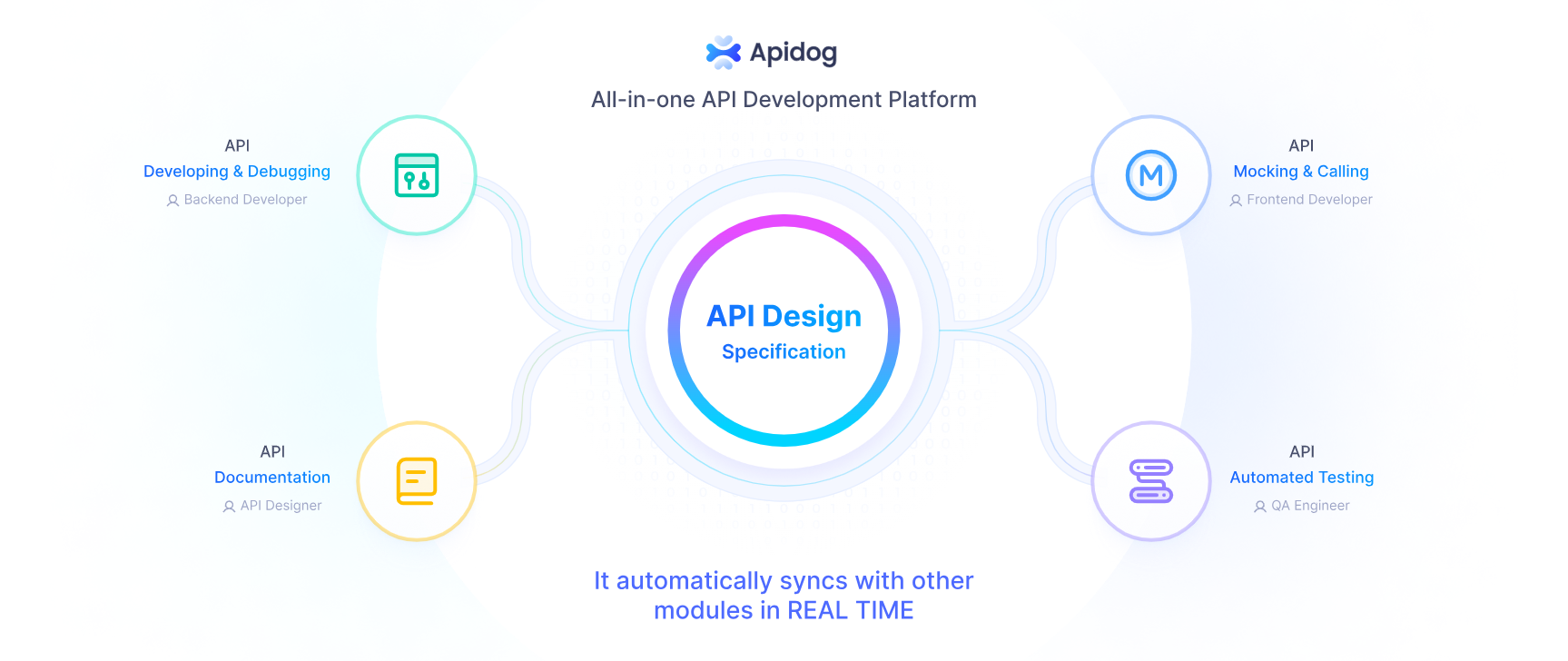
Not only can you import cURL commands, but also build, debug, mock, and document them like any other API! With such convenience, Apidog can support you with an uninterrupted workflow.
Import cURL APIs Within Seconds
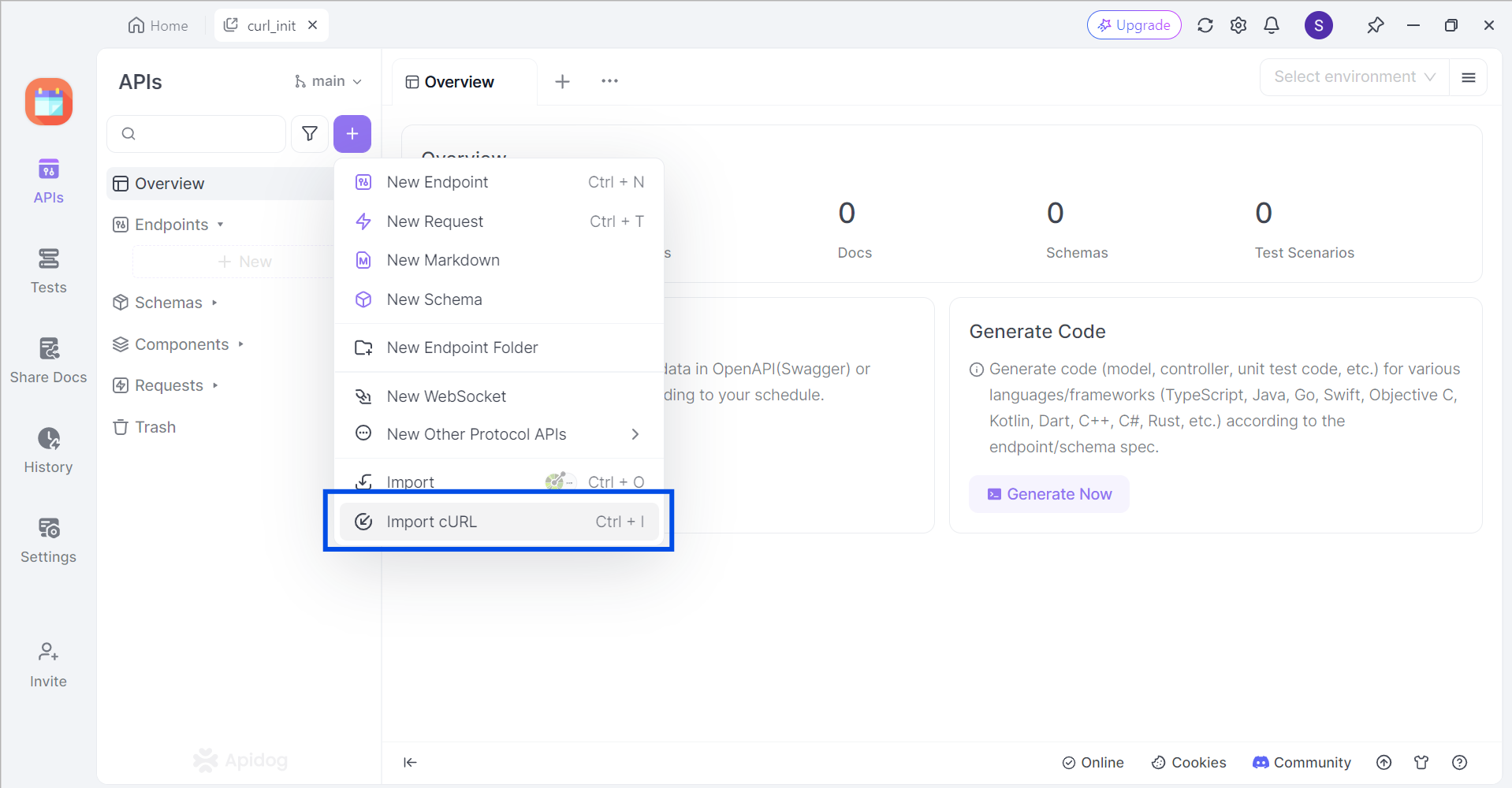
Apidog supports users who wish to import cURL commands to Apidog. In an empty project, click the purple +
button around the top left portion of the Apidog window, and select Import cURL
.
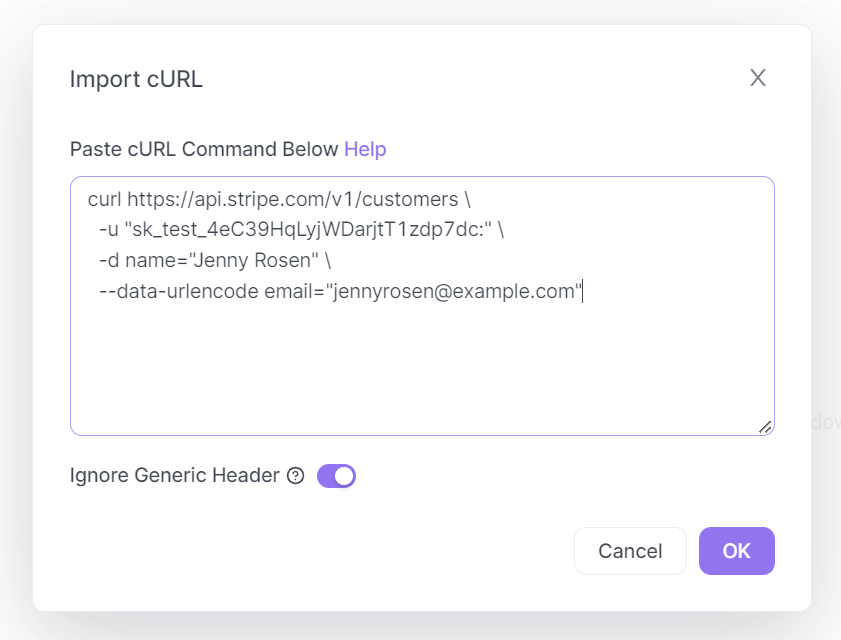
Copy and paste the cURL command into the box displayed on your screen.
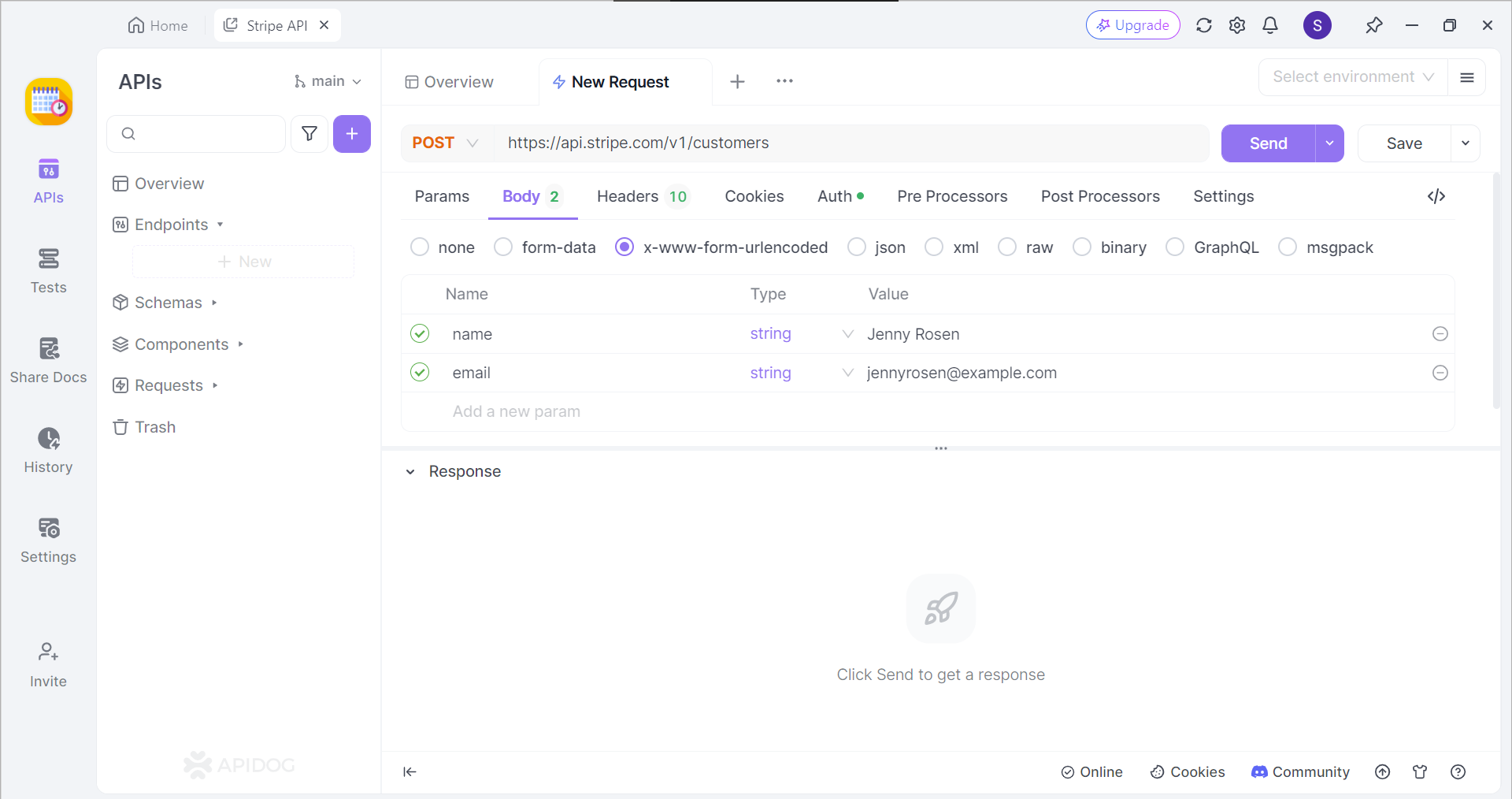
If successful, you should now be able to view the cURL command in the form of an API request.
Unsure of PHP? Generate Code With Apidog
If you do not have prior experience coding in the PHP programming language, fear not! Apidog has a code generation feature that you can rely on, providing you with code frameworks for multiple other programming languages.
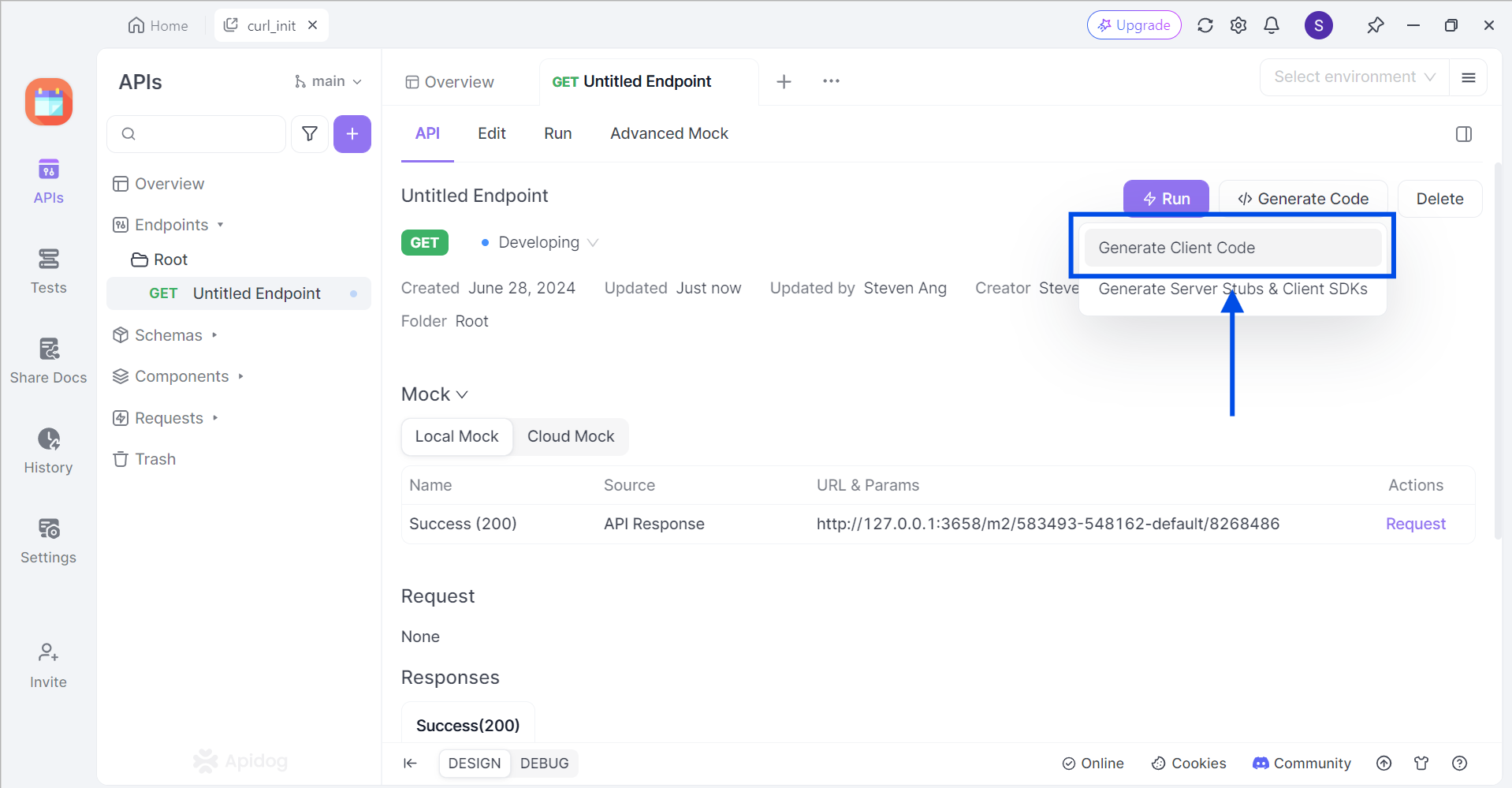
First, locate the </> Generate Code
button on any API or request, and select Generate Client Code
on the drop-down list.
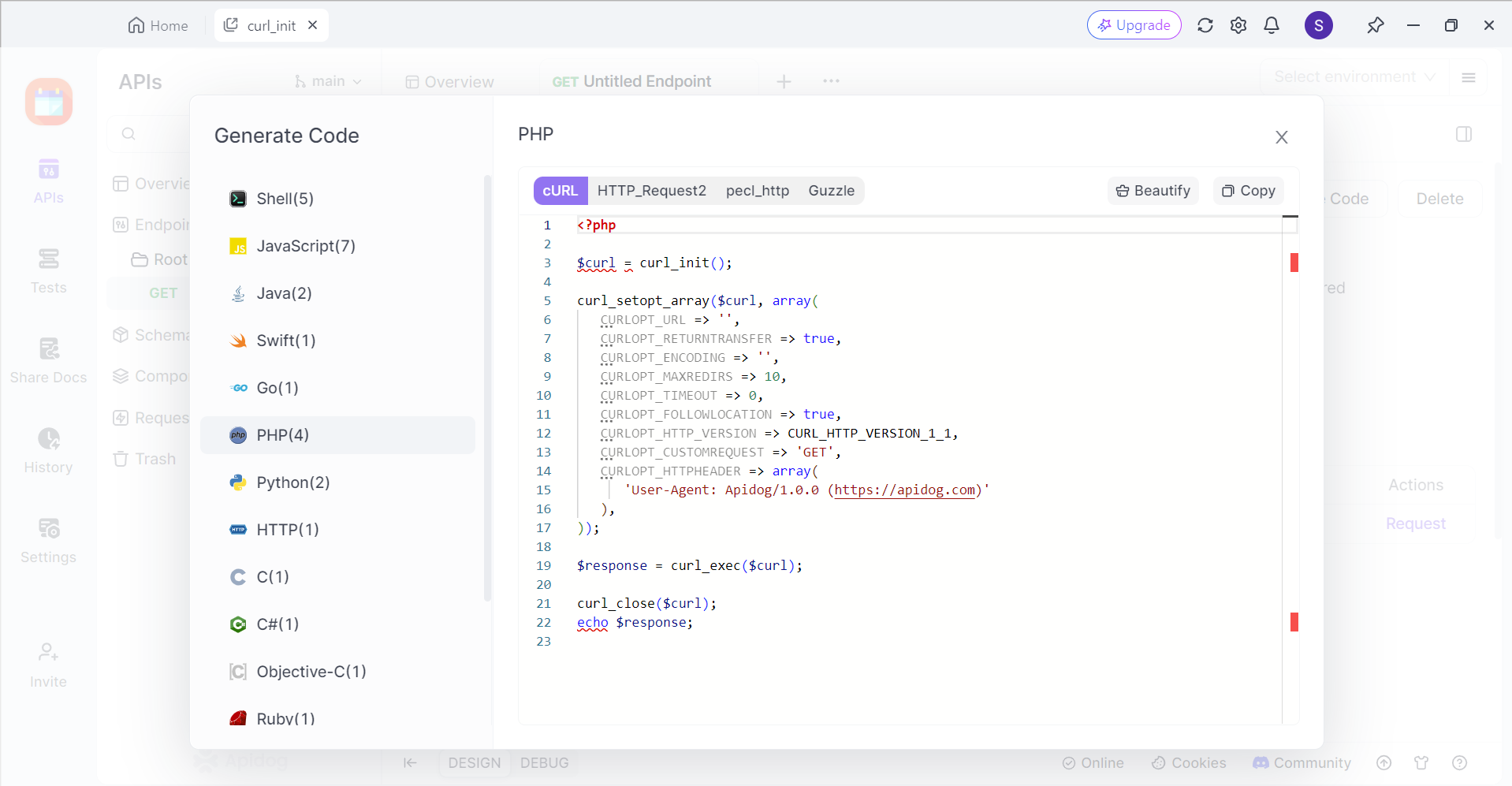
Next, select PHP, and find the cURL section. You should now see the generated code for cURL. All you have to do is copy and paste it to your IDE (Integrated Development Environment) and continue developing your application.
Conclusion
By mastering PUT requests with curl_init()
, you've unlocked a powerful tool for interacting with web servers. This function empowers you to not only update existing resources but also create new ones. Whether you're managing user profiles, modifying database entries, or uploading files, PUT requests offer a precise and efficient method for data manipulation.
Remember,curl_init()
provides a versatile approach, allowing you to specify data formats, set custom headers for secure communication, and even handle scenarios like conditional updates and progress reporting for large file transfers. With this newfound knowledge, you can confidently leverage PUT requests to elevate the functionality and performance of your web applications.