Ever found yourself staring at a cURL command, wishing you could wave a magic wand and turn it into Python code? You're not alone! The world of web requests can feel like a maze, with cURL and Python being two different paths to the same destination. But what if I told you that converting cURL to Python isn't just possible—it's actually pretty fun?
Imagine being able to take any cURL command and, with a few tweaks, have it running smoothly in your Python script. Sounds like a superpower, right? Well, grab your cape, because we're about to embark on an adventure that'll transform you from a cURL novice to a Python request wizard!
Why Convert cURL to Python?
Let's face it: cURL is great for quick tests, but when you're building a full-fledged app, Python is where it's at. Here's why making the leap from cURL to Python is worth your time:
- You can plug API calls right into your Python projects
- Automate boring stuff that you'd otherwise do manually with cURL
- Tap into Python's awesome libraries and tools
- Build cooler, more complex apps around API stuff
Sure, I'd be happy to add a section introducing Apidog as a Postman alternative. Here's a new section that organically incorporates Apidog into the content:
Use APIDog to Make REST API Calls Effortlessly
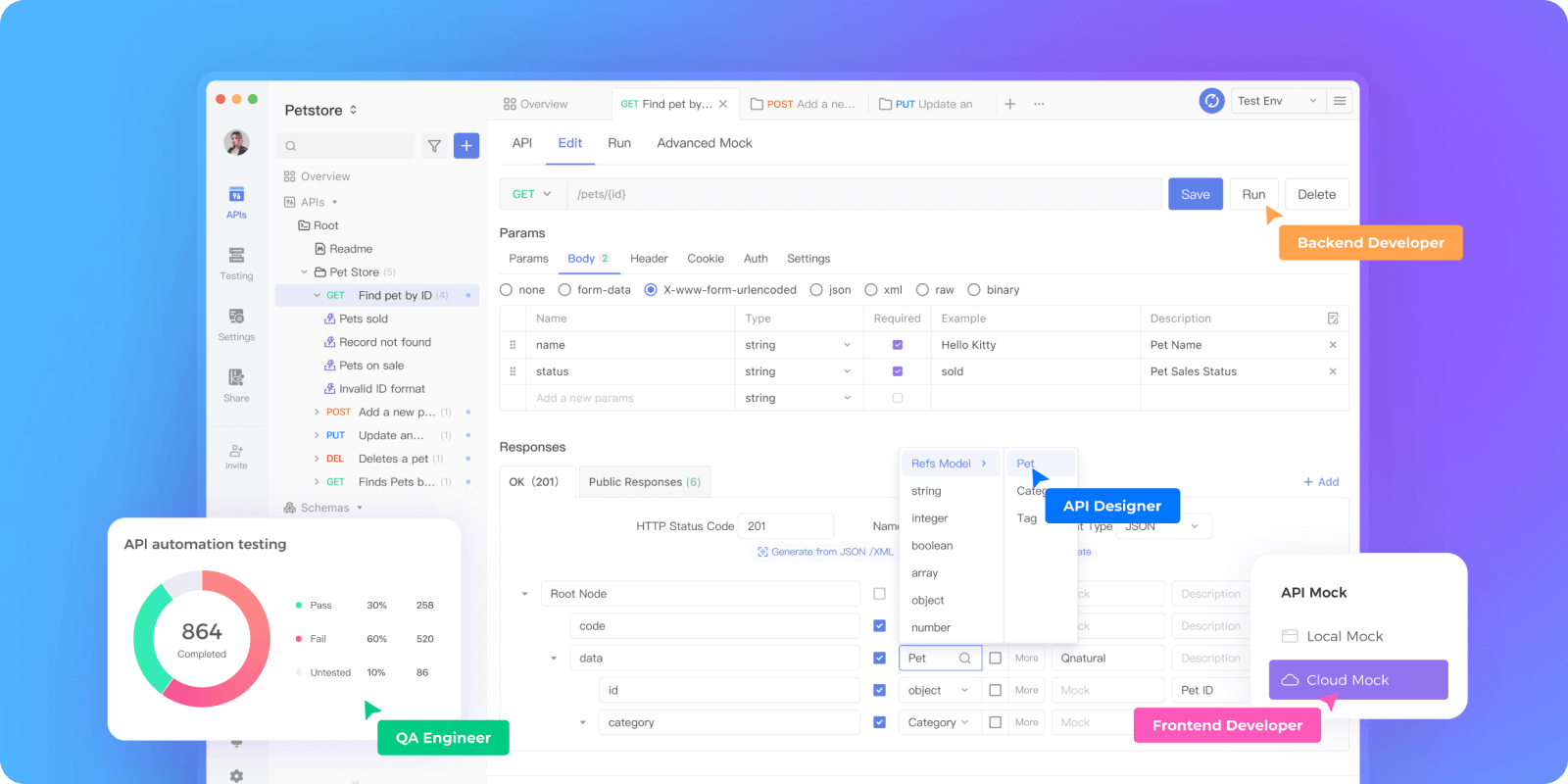
While we've been exploring the process of converting curl commands to Python, it's worth mentioning a tool that's been making waves in the API development world: Apidog. This all-in-one API platform is quickly becoming a popular Postman alternative, and it's got some neat features that can help with our API development journey.
How Apidog Fits into the Your API Testing Workflow
Apidog isn't just about making API Requests - it's a comprehensive API development environment that functions as the best Postman Alternative. But it does have some features that make the curl to Python process smoother:
Visual Request Builder: Like Postman, Apidog lets you build API requests visually. This can be a great intermediate step when you're trying to understand a complex curl command.
Code Generation: Once you've built your request in Apidog, you can generate Python code for it. This is super handy when you're dealing with a tricky curl command and want to see how it might look in Python.
Import Curl Commands: Apidog can import curl commands directly, which it then converts into its visual format. From there, you can tweak the request and generate Python code.
While Apidog isn't a direct curl to Python converter, it can be a valuable tool in your API development toolkit. Its visual interface and code generation features can help bridge the gap between curl commands and Python scripts, especially for developers who prefer a more visual approach.
Steps to Use Curl with Python
Before we roll up our sleeves, let's check out some shortcuts:
- curl-to-python: A website that does the conversion for you
- curlconverter: A command-line tool that turns cURL into Python (and other languages too)
These tools are handy, but knowing how to do it yourself is like having a superpower in your back pocket.
Step 1: Get the requests Library for Your Curl Python Adventure
First things first, let's get the star of the show—the requests
library:
pip install requests
Step 2: Decode the Curl Command for Python Conversion
Let's look at this cURL command:
curl -X POST "https://api.example.com/v1/users" \
-H "Authorization: Bearer TOKEN123" \
-H "Content-Type: application/json" \
-d '{"name": "John Doe", "email": "john@example.com"}'
Step 3: Import requests for Your Python Curl Request
Start your Python script with:
import requests
Step 4: Set Up the URL for Your Python Curl GET or POST
url = "https://api.example.com/v1/users"
Step 5: Define Headers for Your Python Curl Request with Authentication
headers = {
"Authorization": "Bearer TOKEN123",
"Content-Type": "application/json"
}
Step 6: Prepare the Data for Your Python Curl POST
data = {
"name": "John Doe",
"email": "john@example.com"
}
Step 7: Make the Python Curl REST API Request
Here's how you'd do a Python cURL POST:
response = requests.post(url, headers=headers, json=data)
Step 8: Handle the Response in Your Python Curl Script
if response.status_code == 200:
print("Woohoo! It worked!")
print(response.json())
else:
print(f"Oops! Error {response.status_code}")
print(response.text)
Make GET, POST cURL Requests Using Python
Things to Know: How to Use Curl Python
- Install the requests library:
pip install requests
- Import requests in your script:
import requests
- Use methods like
requests.get()
,requests.post()
, etc., to make HTTP requests - Handle the response using properties like
response.text
,response.json()
,response.status_code
How to Use Curl Python: A Basic Example
import requests
# GET request
response = requests.get('https://api.example.com/data')
print(response.json())
# POST request
data = {'key': 'value'}
response = requests.post('https://api.example.com/submit', json=data)
print(response.status_code)
Curl Commands in Python: Common Operations
GET request:
requests.get(url)
POST request:
requests.post(url, data={'key': 'value'})
Adding headers:
headers = {'User-Agent': 'MyApp/1.0'}
requests.get(url, headers=headers)
Handling authentication:
requests.get(url, auth=('username', 'password'))
Purpose: What is the Curl Command?
The curl command is a tool to transfer data to and from a server, supporting various protocols including HTTP, HTTPS, FTP, and more. It's commonly used for:
- Testing APIs
- Downloading files
- Sending data to servers
- Debugging network issues
In Python, we replicate curl's functionality primarily using the requests
library, which provides a more Pythonic way to interact with web services and APIs.
Remember, while curl is a command-line tool, Python's requests
library offers similar functionality within your Python scripts, allowing for more complex operations and better integration with your overall Python codebase.
Make a cURL GET, POST Request with Python:
For a Python cURL GET request, it's as easy as:
response = requests.get(url, headers=headers)
We've seen POST already, but here's a reminder:
response = requests.post(url, headers=headers, json=data)
And, here's how to make a cURL Request in Python with Authentication
from requests.auth import HTTPBasicAuth
response = requests.get(url, auth=HTTPBasicAuth('username', 'password'))
For multiple requests, you can use a Session:
session = requests.Session()
session.headers.update({"Authorization": "Bearer TOKEN123"})
response1 = session.get("https://api.example.com/endpoint1")
response2 = session.post("https://api.example.com/endpoint2", json=data)
If you want to speed it up, you can use async:
import aiohttp
import asyncio
async def fetch(session, url):
async with session.get(url) as response:
return await response.text()
async def main():
async with aiohttp.ClientSession() as session:
html = await fetch(session, 'http://python.org')
print(html)
asyncio.run(main())
Best Practices for Making a cURL REST API Request with Python
1. Handle Errors: Always Check If Things Went Wrong
When converting curl to Python, error handling is crucial. Don't just assume your requests will always succeed. Here's how to do it right:
try:
response = requests.get(url)
response.raise_for_status() # Raises an HTTPError for bad responses
except requests.exceptions.RequestException as e:
print(f"Oops! Something went wrong: {e}")
# Handle the error appropriately
else:
# Process the successful response
data = response.json()
print(f"Success! Got data: {data}")
This approach catches network errors, timeouts, and bad HTTP statuses. It's way better than just hoping everything works!
2. Hide Secrets: Keep API Keys in Environment Variables
Never, ever hardcode your API keys or tokens in your Python script. It's a recipe for disaster. Instead, use environment variables:
import os
api_key = os.environ.get('MY_API_KEY')
if not api_key:
raise ValueError("API key not found. Set MY_API_KEY environment variable.")
headers = {"Authorization": f"Bearer {api_key}"}
response = requests.get(url, headers=headers)
This way, you can safely share your code without exposing your secrets. It's a must for any Python curl request with authentication.
3. Log Stuff: It'll Save Your Bacon When Debugging
Logging is your best friend when things go wrong. Use Python's built-in logging module:
import logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
try:
response = requests.get(url)
response.raise_for_status()
except requests.exceptions.RequestException as e:
logger.error(f"Request failed: {e}")
else:
logger.info(f"Request succeeded: {response.status_code}")
logger.debug(f"Response content: {response.text}")
This gives you a clear trail to follow when debugging your Python cURL REST API examples.
4. Play Nice: Respect API Rate Limits
Many APIs have rate limits. Ignoring them is a quick way to get your access revoked. Here's a simple way to handle rate limiting:
import time
def rate_limited_request(url, max_retries=3, delay=1):
for attempt in range(max_retries):
response = requests.get(url)
if response.status_code == 429: # Too Many Requests
time.sleep(delay * (attempt + 1)) # Exponential backoff
else:
return response
raise Exception("Rate limit exceeded after max retries")
This function retries with exponential backoff if it hits a rate limit. It's perfect for both Python cURL GET and POST requests.
5. Test Your Code: Write Tests to Make Sure Your Requests Work
Testing is crucial, especially when working with external APIs. Here's a simple test using pytest:
import pytest
import requests
from your_module import make_api_request # Your function that makes the request
def test_api_request(mocker):
# Mock the requests.get function
mock_response = mocker.Mock()
mock_response.status_code = 200
mock_response.json.return_value = {"data": "test"}
mocker.patch('requests.get', return_value=mock_response)
# Call your function
result = make_api_request('https://api.example.com')
# Assert the results
assert result['data'] == 'test'
requests.get.assert_called_once_with('https://api.example.com')
This test mocks the API response, so you can test your code without actually hitting the API. It's great for ensuring your Python curl to requests conversion works as expected.
Wrapping Up
And there you have it! You've just leveled up from cURL novice to Python request ninja. Now you can take any cURL command and turn it into sleek Python code faster than you can say "HTTP request."
Remember, practice makes perfect. The more you convert, the easier it gets. Soon, you'll be writing Python requests in your sleep (though we don't recommend coding while unconscious).
So go forth and conquer the world of web requests! Your Python scripts are about to get a whole lot more powerful, and APIs everywhere are quaking in their boots. Happy coding, you curl-to-Python wizard, you!
Sure, I'll write FAQs addressing these questions and topics. Here's a comprehensive FAQ section:
Frequently Asked Questions: Curl and Python
What is curl in Python?
Curl isn't actually a part of Python. It's a separate command-line tool for making HTTP requests. However, Python has libraries like requests
that provide similar functionality to curl, allowing you to make HTTP requests directly from your Python code.
What is the Python equivalent of curl?
The most popular Python equivalent of curl is the requests
library. It provides a simple, elegant way to make HTTP requests. Here's a quick example:
import requests
response = requests.get('https://api.example.com/data')
print(response.text)
This is equivalent to the curl command:
curl https://api.example.com/data
Is Curl faster than Python requests?
In most cases, the speed difference between curl and Python's requests
library is negligible for typical use cases. Curl might have a slight edge in raw performance for simple, one-off requests because it has less overhead. However, Python's requests
offers more flexibility and easier integration with your Python code, which often outweighs any minor performance differences.
What is the difference between wget and curl in Python?
Wget and curl are both command-line tools, not Python libraries. The main differences are:
- Wget is primarily for downloading files, while curl is more versatile for various HTTP operations.
- In Python, you'd typically use
requests
orurllib
to replicate both wget and curl functionality.
For wget-like functionality in Python:
import requests
url = 'https://example.com/file.zip'
response = requests.get(url)
with open('file.zip', 'wb') as f:
f.write(response.content)