Flask vs Django: How to Choose the Right Python Web Framework for Your Project
Flask and Django are both popular Python web frameworks, but they have very different approaches and philosophies. Learn how to compare and choose the right framework for your project in this blog post.
Python is one of the most widely used programming languages in the world, and for good reason. It’s easy to learn, versatile, and powerful. It can be used for a variety of applications, from data science and machine learning to web development and automation.
But when it comes to web development, Python alone is not enough. You need a web framework to help you create dynamic and interactive web applications without having to deal with the low-level details of protocols, sockets, and security.
There are many web frameworks available for Python, but two of the most popular ones are Flask and Django. Both frameworks have their own strengths and weaknesses, and choosing the right one for your project can make a big difference in your productivity, performance, and satisfaction.
By the end of this post, you should have a clear idea of which framework suits your needs and preferences better. Let’s get started!
What are Flask and Django, and what are their main features?
Flask and Django are both web frameworks for Python, but they have very different approaches and philosophies.
Flask: The microframework
Flask is a microframework, which means it provides only the essential features and tools for web development, such as URL routing, request and response handling, templating, and a development server. Flask does not impose any restrictions or conventions on how you structure your code or your project. You have the freedom and flexibility to choose the components and libraries you want to use for your web application.
Some of the main features of Flask are:
- Minimalist and lightweight: Flask has a small core and few dependencies, which makes it easy to install and run. It also consumes less memory and resources than other frameworks.
- Extensible and modular: Flask supports extensions that can add functionality to the framework, such as database integration, authentication, caching, testing, and more. You can also use any Python library or package you want with Flask, as long as it is compatible with the WSGI standard.
- Developer-friendly and expressive: Flask has a simple and intuitive syntax that makes it easy to write and read code. It also has a built-in debugger and a CLI tool that can help you with development and testing.
- Documentation and community: Flask has a comprehensive and well-written documentation that covers everything you need to know about the framework. It also has a large and active community of developers who can provide support and feedback.
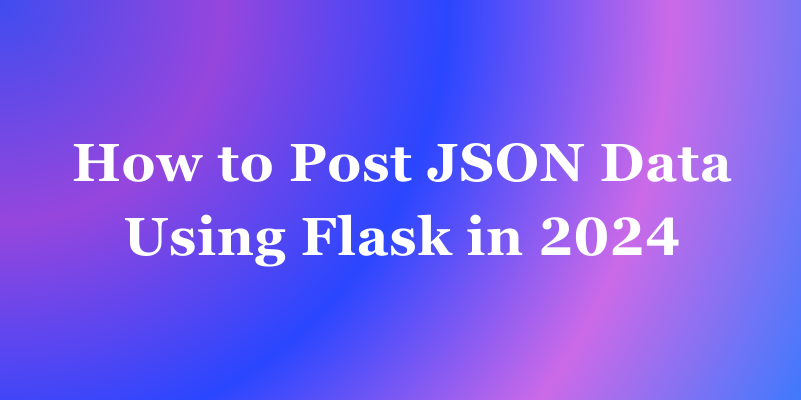
Django: The batteries-included framework
Django is a batteries-included framework, which means it provides everything you need for web development, such as URL routing, request and response handling, templating, database integration, authentication, administration, caching, testing, and more. Django follows the Model-View-Template (MVT) pattern and enforces a strict and consistent project structure. You have to follow the conventions and best practices that Django recommends for your web application.
Some of the main features of Django are:
- Comprehensive and full-featured: Django has a rich set of features and tools that can handle almost any web development scenario. It also has a lot of built-in security measures, such as CSRF protection, XSS prevention, and password hashing.
- Scalable and reliable: Django is designed to handle high-traffic and complex web applications, such as Instagram, Spotify, and The Washington Post. It also has a robust and stable codebase that is regularly updated and maintained.
- Productive and efficient: Django allows you to create web applications quickly and easily, with less code and less hassle. It also has a powerful ORM (Object-Relational Mapper) that can abstract the database operations and simplify the data manipulation.
- Documentation and community: Django has an excellent and detailed documentation that covers everything you need to know about the framework. It also has a huge and vibrant community of developers who can provide support and feedback.
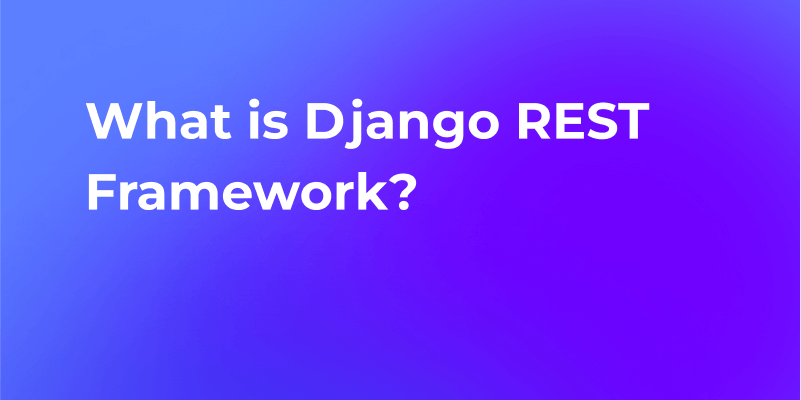
How do Flask and Django work, and what are their architectures?
Flask and Django both use the WSGI (Web Server Gateway Interface) protocol, which is a standard for communication between web servers and web applications in Python. WSGI allows web applications to be compatible with different web servers, such as Apache, Nginx, or Gunicorn.
However, Flask and Django have very different architectures and workflows, which affect how you design and develop your web applications.
Flask: The bottom-up approach
Flask follows a bottom-up approach, which means you start with the bare minimum and add the features and components you need as you go along. You have to decide how to organize your code, how to structure your project, and what libraries and extensions to use for your web application.
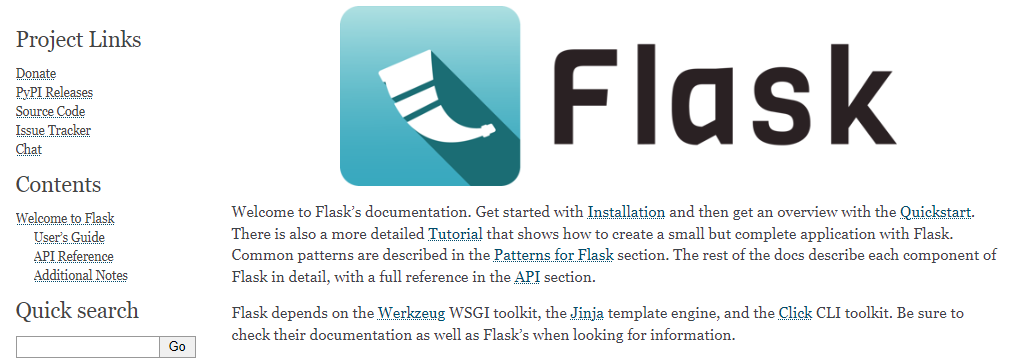
A typical Flask application consists of the following elements:
- A single Python file (usually called app.py) that contains the application instance, the URL routes, and the view functions. The application instance is an object that represents the Flask application and its configuration. The URL routes are the mappings between the URLs and the view functions. The view functions are the functions that handle the requests and return the responses.
- A templates folder that contains the HTML files for the web pages. Flask uses the Jinja2 template engine, which allows you to use variables, expressions, filters, and control structures in your HTML files. You can also use template inheritance and macros to reuse and modularize your code.
- A static folder that contains the static files, such as CSS, JavaScript, and images. You can use the url_for function to generate the URLs for the static files in your templates.
- Optionally, other Python files or modules that contain the models, forms, utilities, or other logic for your web application. You can import and use these files or modules in your app.py file or in your templates.
Here is an example of a simple Flask application that displays a greeting message on the homepage:
from flask import Flask, render_template
app = Flask(__name__)
@app.route("/")
def index():
return render_template("index.html")
if __name__ == "__main__":
app.run(debug=True)
And here is the index.html file in the templates folder:
<!DOCTYPE html>
<html>
<head>
<title>Flask App</title>
</head>
<body>
<h1>Hello, world!</h1>
</body>
</html>
As you can see, Flask is very simple and straightforward to use. You can create a web application with just a few lines of code. However, if you want to add more features and functionality to your web application, such as database integration, authentication, or RESTful API, you have to install and configure the extensions and libraries yourself. This can be a tedious and time-consuming process, and you have to make sure that the components you choose are compatible and secure.
Django: The top-down approach
Django follows a top-down approach, which means you start with a lot of features and components and remove or customize the ones you don’t need. You have to follow the project structure and the coding style that Django provides for your web application.
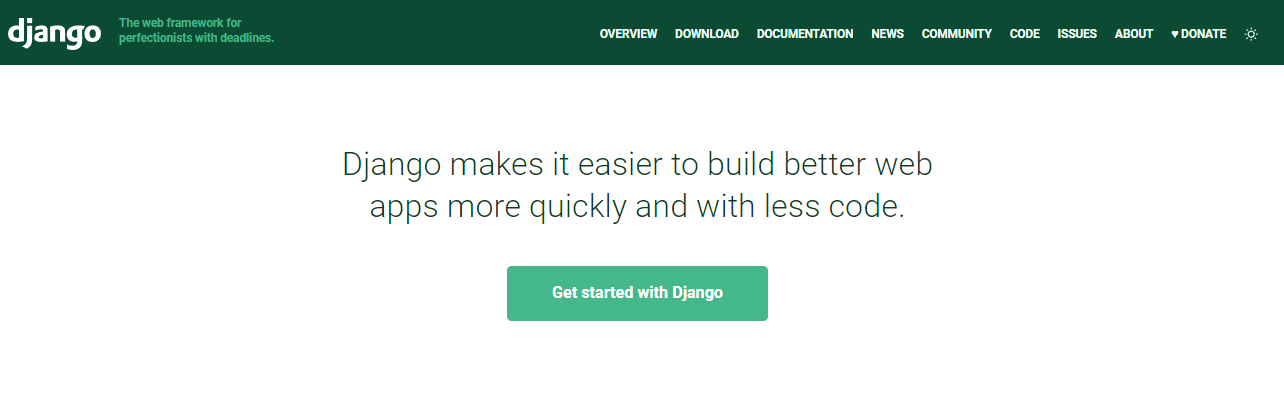
A typical Django project consists of the following elements:
- A project folder that contains the settings, the URLs, and the WSGI configuration for the whole project. The settings file contains the configuration options for the project, such as the installed apps, the database settings, the middleware, the templates, and more. The URLs file contains the URL patterns for the project, which map the URLs to the views. The WSGI file contains the WSGI application object that is used by the web server to communicate with the project.
- One or more app folders that contain the models, the views, the templates, and the tests for each app. An app is a self-contained and reusable component of the project that performs a specific function, such as a blog, a forum, or a shopping cart. The models file contains the classes that define the data structures and the business logic for the app. The views file contains the functions or classes that handle the requests and return the responses for the app. The templates folder contains the HTML files for the web pages for the app. The tests file contains the unit tests or the integration tests for the app.
- A manage.py file that is a command-line utility that allows you to perform various tasks for the project, such as creating apps, running tests, migrating the database, or starting the development server.
Here is an example of a simple Django project that displays a greeting message on the homepage:
# project/settings.py
...
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'app', # the app we created
]
...
# project/urls.py
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('app.urls')), # include the app's URLs
]
# app/models.py
from django.db import models
# Create your models here.
# app/views.py
from django.shortcuts import render
# Create your views here.
def index(request):
return render(request, "index.html")
# app/urls.py
from django.urls import path
from . import views
urlpatterns = [
path('', views.index, name='index'), # the homepage URL
]
# app/templates/index.html
<!DOCTYPE html>
<html>
<head>
<title>Django App</title>
</head>
<body>
<h1>Hello, world!</h1>
</body>
</html>
As you can see, Django is very comprehensive and structured to use. You can create a web application with a lot of features and tools already available for you. However, if you want to customize or modify the features and tools that Django provides, such as the admin interface, the authentication system, or the template engine, you have to learn and understand how Django works internally. This can be a steep and complex learning curve, and you have to follow the conventions and best practices that Django expects from you.
What are the advantages and disadvantages of Flask and Django?
Flask and Django both have their pros and cons, depending on your preferences, goals, and requirements. Here are some of the main advantages and disadvantages of each framework:
Flask: The pros and cons
Pros:
- Flexibility and customizability: Flask gives you the freedom and flexibility to create your web application the way you want it. You can choose the components and libraries that suit your needs and preferences, and you can customize them as much as you want. You can also experiment with different architectures and design patterns, such as MVC, REST, or GraphQL.
- Simplicity and readability: Flask has a simple and expressive syntax that makes it easy to write and read code. It also has a minimalist and lightweight core that makes it easy to understand and debug. You can focus on the logic and functionality of your web application, rather than the boilerplate and configuration.
- Learning curve and beginner-friendliness: Flask is easy to learn and use, especially if you are already familiar with Python. It has a clear and well-written documentation that covers everything you need to know about the framework. It also has a lot of tutorials and examples that can help you get started and learn by doing.
Cons:
- Lack of features and tools: Flask does not provide a lot of features and tools out of the box, such as database integration, authentication, administration, caching, testing, and more. You have to install and configure the extensions and libraries yourself, which can be a tedious and time-consuming process. You also have to make sure that the components you choose are compatible and secure.
- Lack of structure and consistency: Flask does not impose any structure or consistency on how you organize your code or your project. You have to decide how to structure your project, how to name your files and folders, how to separate your concerns, and how to follow the coding standards. This can lead to confusion and inconsistency, especially if you are working on a large or complex project, or if you are collaborating with other developers.
- Lack of support and maintenance: Flask is a relatively young and small framework, compared to Django. It has a smaller and less active community of developers, which means it has less support and feedback. It also has fewer updates and bug fixes, which means it may have more issues and vulnerabilities.
Django: The pros and cons
Pros:
- Features and tools: Django provides a lot of features and tools out of the box, such as database integration, authentication, administration, caching, testing, and more. You don’t have to install and configure the extensions and libraries yourself, which saves you a lot of time and hassle. You also get a lot of security measures, such as CSRF protection, XSS prevention, and password hashing.
- Structure and consistency: Django imposes a structure and consistency on how you organize your code and your project. You have to follow the project structure and the coding style that Django provides for your web application. This makes your code and your project more organized, readable, and maintainable. It also makes it easier to collaborate with other developers, as you follow the same conventions and best practices.
- Support and maintenance: Django is a mature and established framework, compared to Flask. It has a huge and vibrant community of developers, which means it has a lot of support and feedback. It also has frequent updates and bug fixes, which means it is more stable and secure.
Cons:
- Complexity and rigidity: Django has a complex and rigid architecture that makes it hard to customize or modify. You have to learn and understand how Django works internally, which can be a steep and complex learning curve. You also have to follow the conventions and best practices that Django expects from you, which can limit your creativity and flexibility.
- Overhead and bloat: Django has a lot of features and tools that you may not need or use for your web application, which can add unnecessary overhead and bloat. It also consumes more memory and resources than Flask, which can affect the performance and scalability of your web application. You may have to remove or disable the features and tools that you don’t need, which can be a hassle and a waste of time.
- Learning curve and beginner-unfriendliness: Django is hard to learn and use, especially if you are new to Python or web development. It has a lot of concepts and components that you have to master, such as the ORM, the MVT pattern, the middleware, the templates, and more. It also has a lot of documentation that can be overwhelming and confusing. You may have to spend a lot of time and effort to learn and use Django effectively.
When should you use Flask or Django for your project?
There is no definitive answer to this question, as it depends on your preferences, goals, and requirements. However, here are some general guidelines that can help you decide which framework to use for your project:
- Use Flask if you want more flexibility and customizability, if you have a simple or small project, if you want to experiment with different architectures and design patterns, or if you are a beginner who wants to learn Python and web development.
- Use Django if you want more features and tools, if you have a complex or large project, if you want to follow a consistent and structured approach, or if you are an experienced developer who wants to create a robust and scalable web application.
Of course, these are not absolute rules, and you can use either framework for any kind of project, as long as you are comfortable and confident with it. The best way to find out which framework suits you better is to try them both and see for yourself.
How to Use Apidog to send request in Flask or Django?
Apidog is a tool that helps you design, debug, test, and document your APIs in a fast and fun way. Apidog is based on the concept of API design-first, which means that you start by defining the structure and behavior of your API before you write any code. This way, you can ensure that your API is consistent, clear, and easy to use.
Here’s how to use Apidog to send GET requests with params:
- Open Apidog, Click on the New Request button.
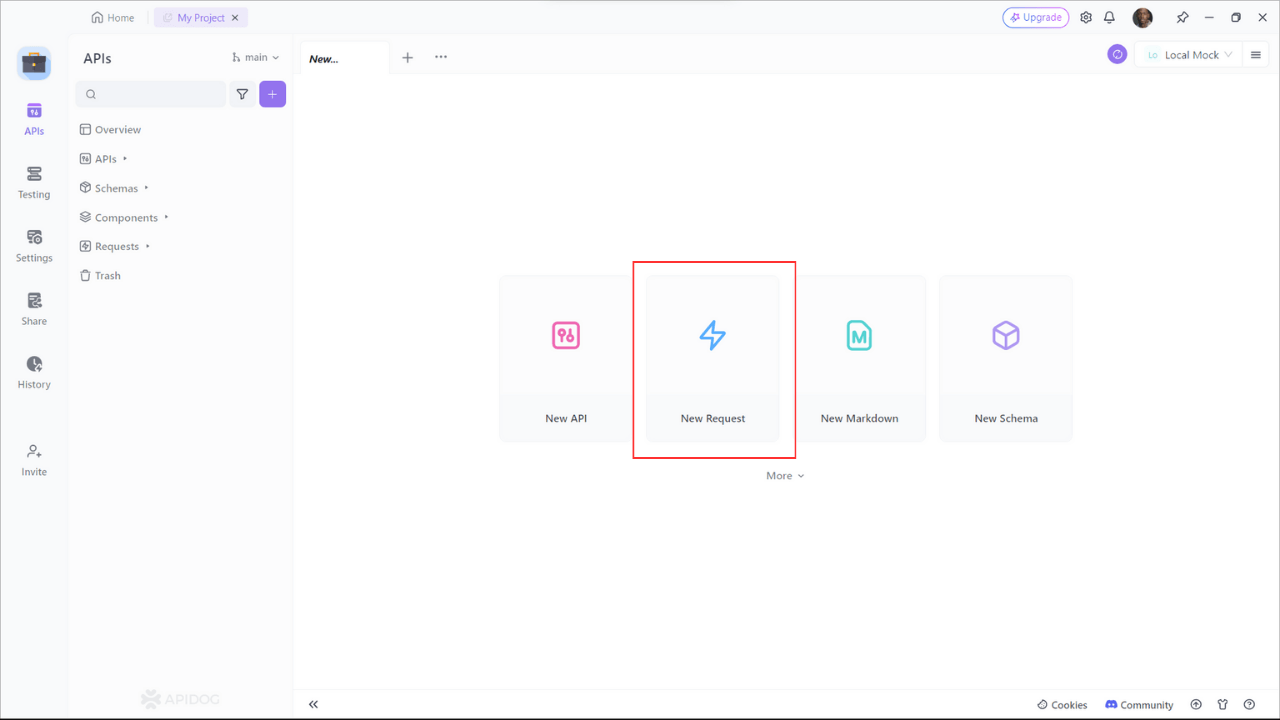
2. Enter the URL of the API endpoint you want to send a GET request to
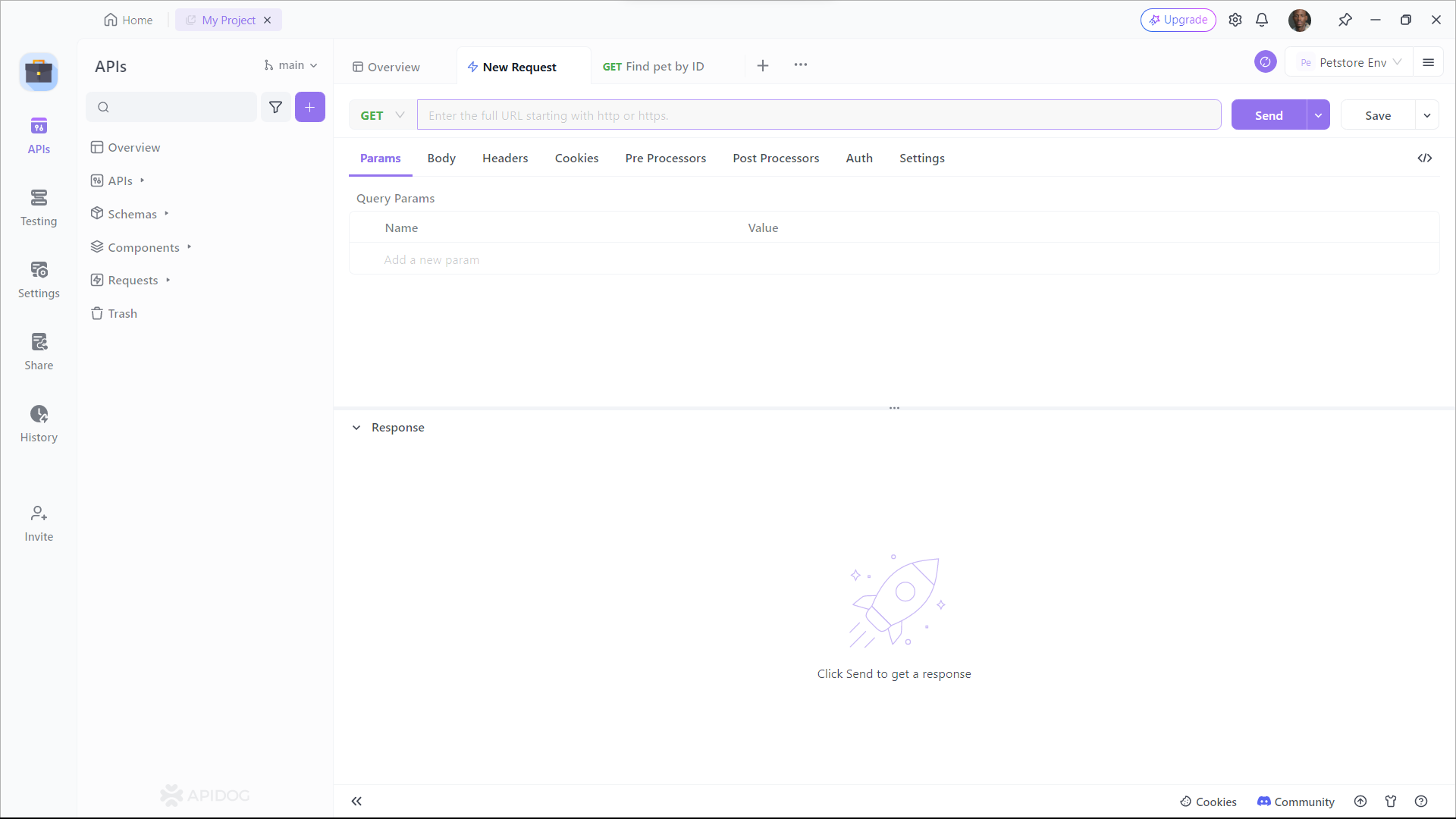
3. Click on the Send button to send the request and get the result
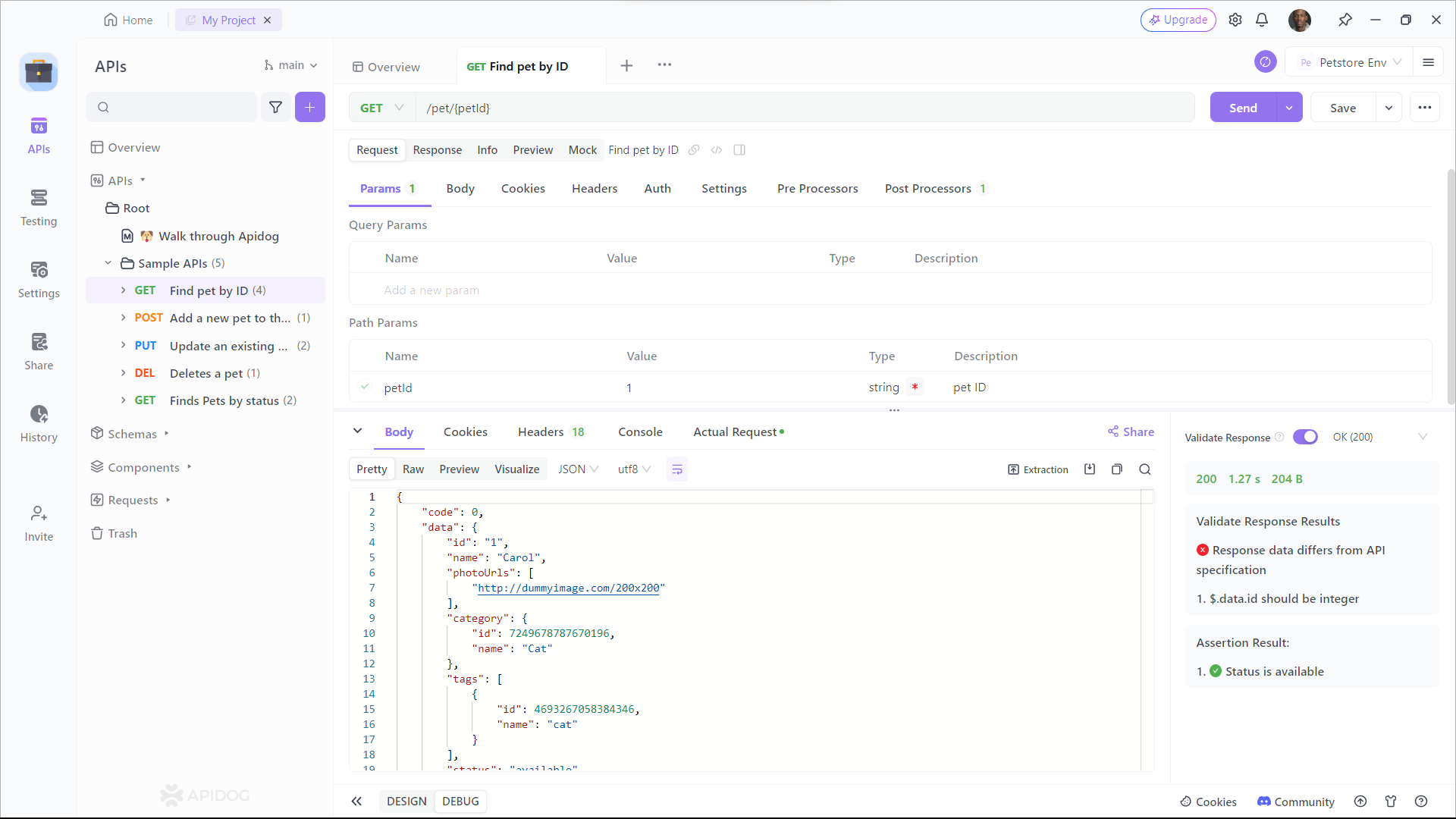
Conclusion
Flask and Django are both excellent web frameworks for Python, but they have very different approaches and philosophies. Flask is a microframework that gives you more flexibility and customizability, but less features and tools. Django is a batteries-included framework that gives you more features and tools, but less flexibility and customizability. Choosing the right framework for your project depends on your preferences, goals, and requirements. The best way to find out which framework suits you better is to try them both and see for yourself.
We hope this blog post has helped you understand the differences and similarities between Flask and Django, and how to choose the right Python web framework for your project.