If you're diving into the world of modern web development, chances are you've heard of FastAPI. It's one of the fastest-growing frameworks for building APIs in Python. In this blog post, we're going to explore how to use FastAPI to create and handle POST requests. Whether you're a seasoned developer or just starting out, this guide will help you understand the basics and beyond.
What is FastAPI?
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.7+ based on standard Python type hints. It is designed to be easy to use and to provide high performance on par with Node.js and Go. The framework is also very intuitive and straightforward, making it an excellent choice for beginners and experts alike.
Why Use FastAPI?
There are several reasons why developers are choosing FastAPI over other frameworks:
- Speed: FastAPI is fast. In fact, it's one of the fastest web frameworks available today.
- Ease of Use: With automatic interactive documentation (thanks to Swagger UI and ReDoc), it's incredibly easy to test your APIs.
- Data Validation: FastAPI leverages Pydantic for data validation, which ensures that the data your API receives is valid.
- Async Ready: It supports asynchronous programming out of the box, making it suitable for modern, high-performance web applications.
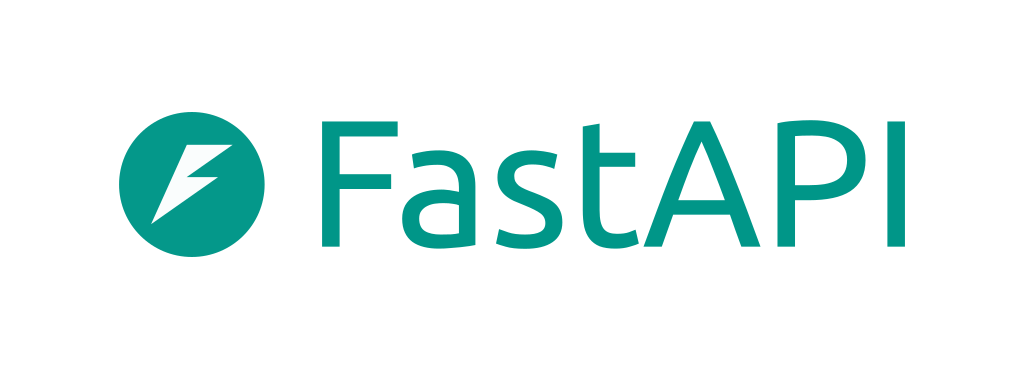
Setting Up FastAPI
Before we dive into POST requests, let's quickly set up FastAPI. You'll need Python installed on your system. Then, you can install FastAPI and an ASGI server, such as Uvicorn, using pip:
pip install fastapi uvicorn
Once you have FastAPI installed, you can create a simple FastAPI application. Let's start by creating a file named main.py
:
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def read_root():
return {"Hello": "World"}
To run the application, use Uvicorn:
uvicorn main:app --reload
Open your browser and navigate to http://127.0.0.1:8000
. You should see a JSON response: {"Hello": "World"}
.
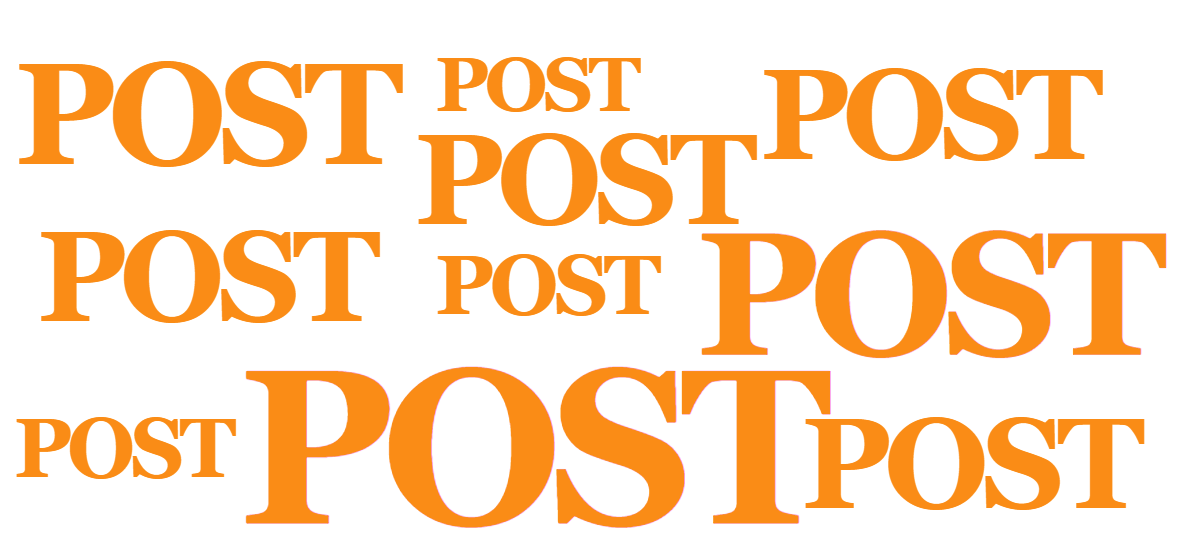
Creating a POST Endpoint
Now that we have a basic FastAPI app running, let's add a POST endpoint. POST requests are used to submit data to be processed to a specified resource. To handle a POST request in FastAPI, you use the @app.post
decorator.
Here's a simple example of how to handle a POST request:
from fastapi import FastAPI
from pydantic import BaseModel
app = FastAPI()
class Item(BaseModel):
name: str
description: str = None
price: float
tax: float = None
@app.post("/items/")
def create_item(item: Item):
return item
In this example:
- We define a
FastAPI
instance. - We create a Pydantic model
Item
that describes the data structure of the request body. - We use the
@app.post
decorator to define a POST endpoint/items/
. - The
create_item
function takes anItem
object as input and returns it.
To test this, you can use an API client like apidog or simply use the interactive Swagger UI provided by FastAPI at http://127.0.0.1:8000/docs
.
Handling Data and Validations
FastAPI automatically validates the request data against the Pydantic model. If the data does not conform to the model, FastAPI returns a 422 status code with details about the validation errors.
For example, if you send a POST request to /items/
with the following JSON body:
{
"name": "Item name",
"price": 25.5
}
FastAPI will accept this request because it meets the requirements of the Item
model. However, if you omit the price
field, FastAPI will reject the request and return an error.
Using Apidog to Test Your FastAPI POST Request
Apidog is a powerful tool for testing APIs. It allows you to create and save API requests, organize them into collections, and share them with your team.
Here is how you can use Apidog to test your POST request:
- Open Apidog and create a new request.
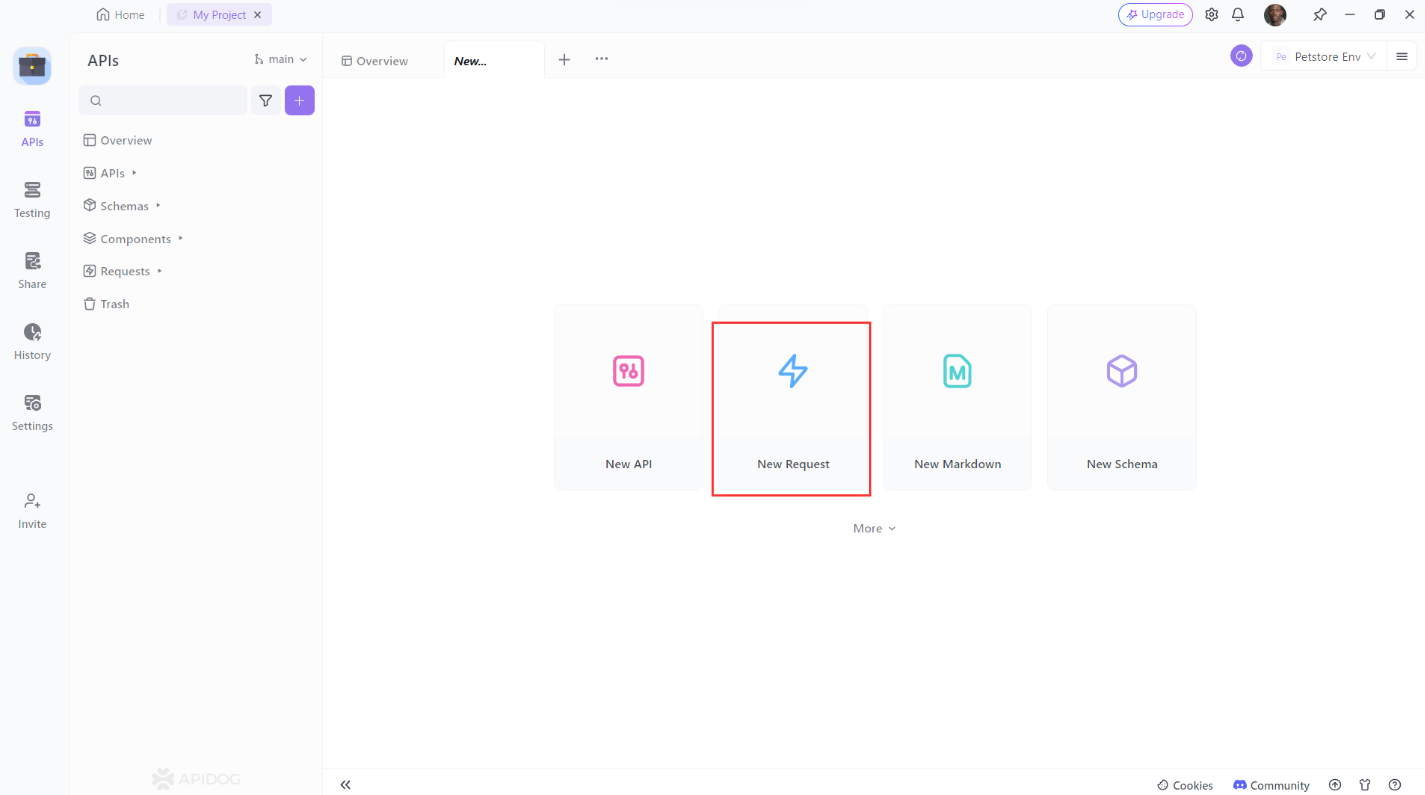
2. Set the request method to POST.
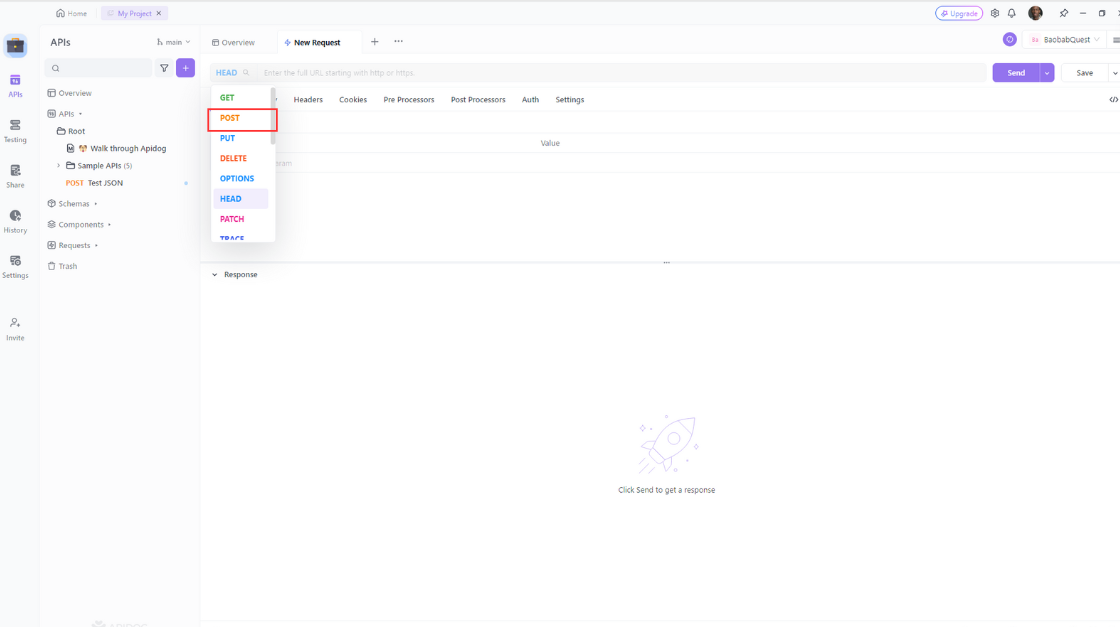
3. Enter the URL of the resource you want to update. Add any additional headers or parameters you want to include then click the “Send” button to send the request.
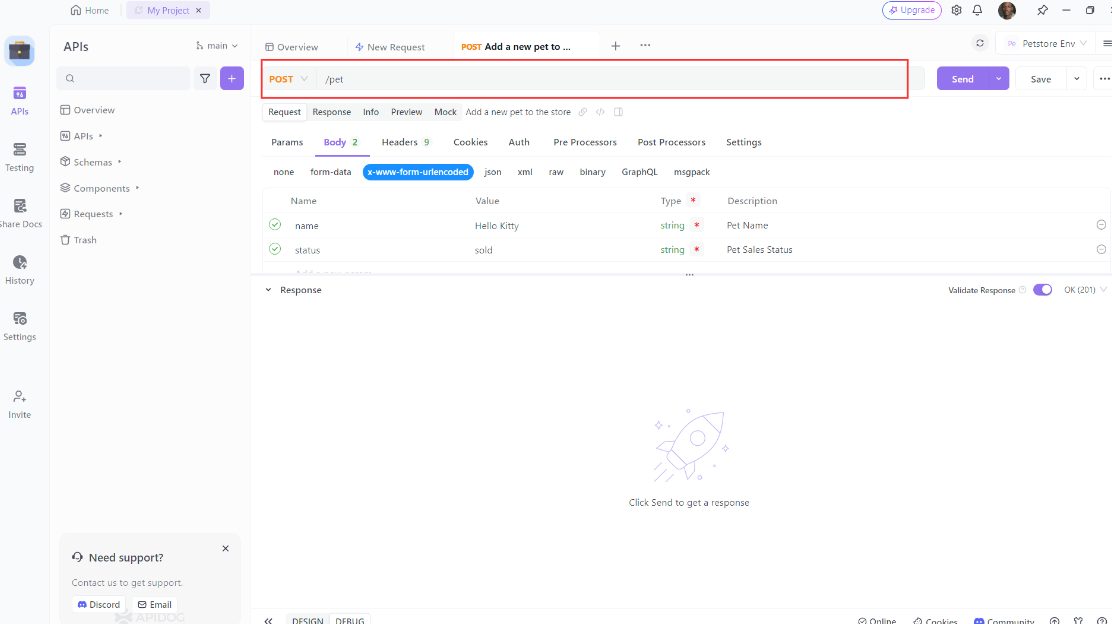
4. Verify that the response is what you expected.
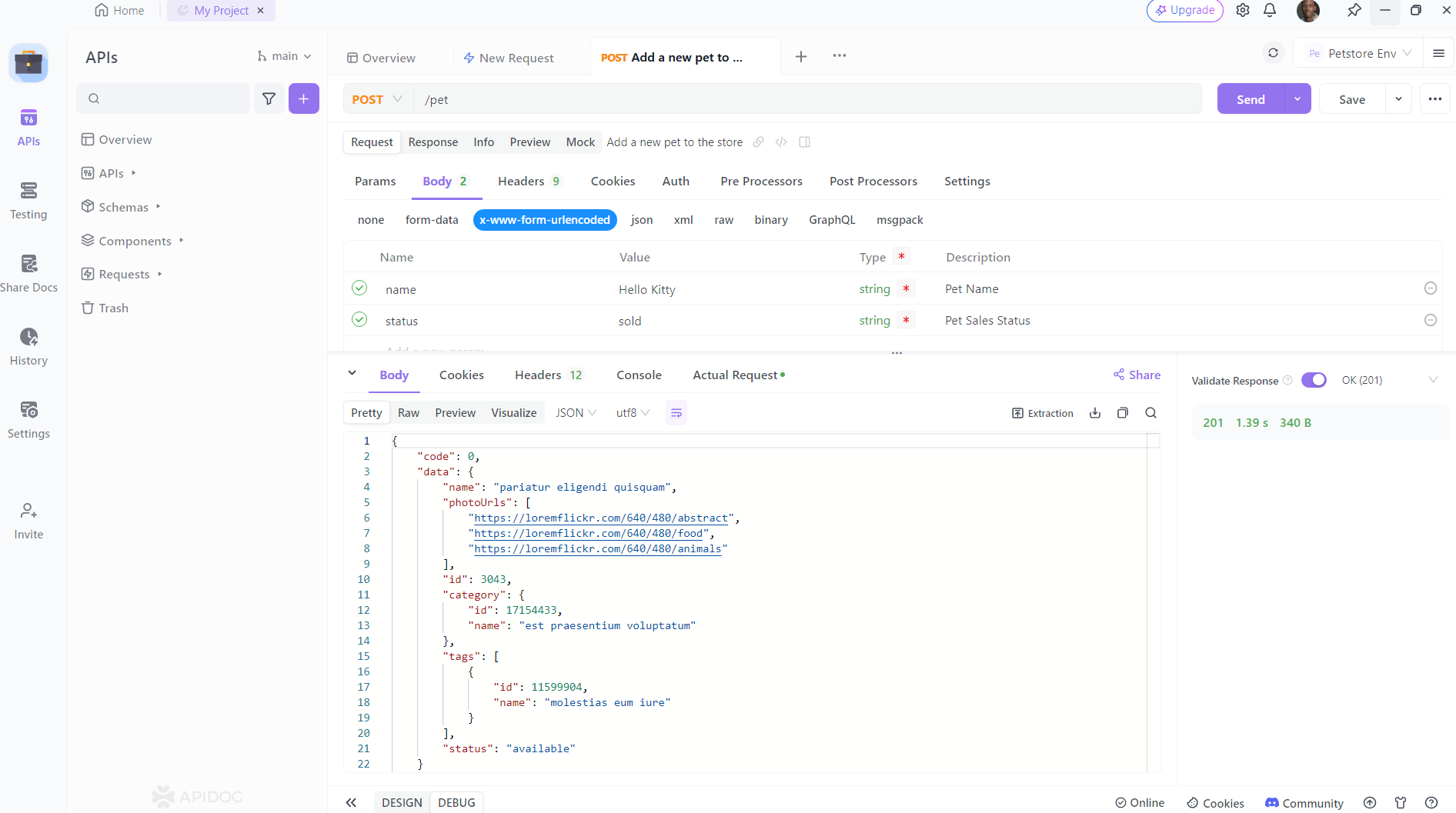
Advanced POST Requests
Now, let's explore some more advanced scenarios. FastAPI allows you to do a lot more with POST requests. For instance, you can:
- Handle File Uploads: Use
File
andUploadFile
fromfastapi
. - Process Form Data: Use
Form
fromfastapi
. - Use Path Parameters: Combine
path parameters
with POST requests.
Handling File Uploads
Here's an example of handling file uploads with FastAPI:
from fastapi import FastAPI, File, UploadFile
app = FastAPI()
@app.post("/uploadfile/")
async def create_upload_file(file: UploadFile):
return {"filename": file.filename}
This endpoint will accept a file and return the filename. You can test this with apidog or Swagger UI.
Processing Form Data
To process form data, use the Form
class from FastAPI:
from fastapi import FastAPI, Form
app = FastAPI()
@app.post("/login/")
def login(username: str = Form(...), password: str = Form(...)):
return {"username": username}
This example creates a login endpoint that accepts form data. You can test this using a form submission in your browser or an API testing tool.
Using Path Parameters
You can also combine path parameters with POST requests:
from fastapi import FastAPI
app = FastAPI()
@app.post("/users/{user_id}/items/")
def create_item_for_user(user_id: int, item: Item):
return {"user_id": user_id, "item": item}
This endpoint creates an item for a specific user, identified by the user_id
path parameter.
Conclusion
FastAPI is a powerful framework that makes it easy to build robust APIs with Python. Its support for data validation, interactive documentation, and high performance makes it an excellent choice for modern web development.
In this blog post, we've covered how to set up a FastAPI application and handle POST requests. We've also explored more advanced topics like file uploads, form data processing, and combining path parameters with POST requests. By now, you should have a solid understanding of how to use FastAPI to create and handle POST requests.
Remember, whether you're building a simple API or a complex application, FastAPI has the tools you need to succeed. Happy coding!