In the world of web development, making API requests is a fundamental task. Whether you're building a front-end application, a back-end service, or testing an API, knowing how to make these requests efficiently is crucial. Two popular tools for this purpose are curl
and JavaScript fetch
. This guide will take you through the ins and outs of using these tools, providing you with practical examples and tips along the way.
Before we dive in, if you’re looking for an easy way to test your APIs, I highly recommend downloading Apidog for free. Apidog simplifies the process of API testing, making it a breeze to work with complex APIs.
Understanding API Requests
What is an API?
An API (Application Programming Interface) is a set of rules that allows different software entities to communicate with each other. APIs define the methods and data formats that applications can use to interact with external services, databases, or other applications.
Why Use API Requests?
API requests are essential for fetching data from servers, submitting data to be processed, and interacting with various web services. They are the backbone of modern web applications, enabling functionalities like user authentication, data retrieval, and third-party integrations.
Introduction to cURL
What is curl?
cURL is a command-line tool used for transferring data with URLs. It supports a wide range of protocols, including HTTP, HTTPS, FTP, and many more. curl
is widely used for testing APIs, downloading files, and performing web requests.
Installing cURL
cURL is pre-installed on most Unix-based systems, including macOS and Linux. For Windows, you can download it from the official curl website.
To check if cURL is installed on your system, open your terminal or command prompt and run:
curl --version
Basic Usage of cURL
Making a Simple GET Request
To make a basic GET request with cURL , you can use the following command:
curl https://api.example.com/data
This command fetches data from the specified URL.
Adding Headers
Sometimes, you need to add headers to your request. This can be done using the -H
flag:
curl -H "Authorization: Bearer YOUR_ACCESS_TOKEN" https://api.example.com/data
Making a POST Request
To send data to the server, you can use the POST method. Here's how you can do it with curl
:
curl -X POST -H "Content-Type: application/json" -d '{"key":"value"}' https://api.example.com/data
In this command, -X POST
specifies the request method, -H
adds a header, and -d
sends the data.
Introduction to JavaScript fetch
What is JavaScript fetch?
The fetch API is a modern interface that allows you to make HTTP requests from the browser. It is a simpler and more powerful alternative to XMLHttpRequest
and is widely used in front-end development.
Basic Usage of fetch
Making a Simple GET Request
Here’s how you can make a GET request using fetch :
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
This code fetches data from the specified URL and logs it to the console.
Adding Headers
To add headers to your fetch request, you can use the headers
option:
fetch('https://api.example.com/data', {
headers: {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN'
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Making a POST Request
To send data to the server using fetch, you can use the POST method:
fetch('https://api.example.com/data', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ key: 'value' })
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Comparing cURL and JavaScript fetch
Use Cases
cURL is ideal for:
- Command-line tasks
- Automating scripts
- Testing APIs without a graphical interface
JavaScript fetch is perfect for:
- Web applications
- Asynchronous operations in the browser
- Modern front-end frameworks like React and Vue
Syntax and Usability
cURL uses a command-line interface with a syntax that can become complex with advanced options. However, it’s extremely powerful and flexible.
JavaScript fetch
, on the other hand, offers a more readable and promise-based syntax, making it easier to handle asynchronous operations and manage responses.
Error Handling
Error handling in cURL is done using exit codes and parsing responses manually. In JavaScript fetch
, error handling is more straightforward with catch
blocks, which provide a more intuitive way to handle exceptions.
Practical Examples
Example 1: Fetching User Data
Using cURL
curl https://jsonplaceholder.typicode.com/users
This command retrieves a list of users from the placeholder API.
Using JavaScript fetch
fetch('https://jsonplaceholder.typicode.com/users')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Example 2: Submitting a Form
Using cURL
curl -X POST -H "Content-Type: application/json" -d '{"name":"John Doe","email":"john.doe@example.com"}' https://jsonplaceholder.typicode.com/users
Using JavaScript fetch
fetch('https://jsonplaceholder.typicode.com/users', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ name: 'John Doe', email: 'john.doe@example.com' })
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Using Apidog to Simplify API Requests
If you want to streamline your API workflows even further, consider using Apidog. Apidog is a powerful tool designed to make API management easy and efficient. It provides features like API documentation, testing, and monitoring in one place.
Why Use Apidog?
- User-Friendly Interface: Apidog offers an intuitive interface that makes API management a breeze.
- Comprehensive Features: From creating and testing APIs to monitoring their performance, Apidog covers all aspects of API management.
- Free to Use: You can download and use Apidog for free, making it accessible to developers of all levels.
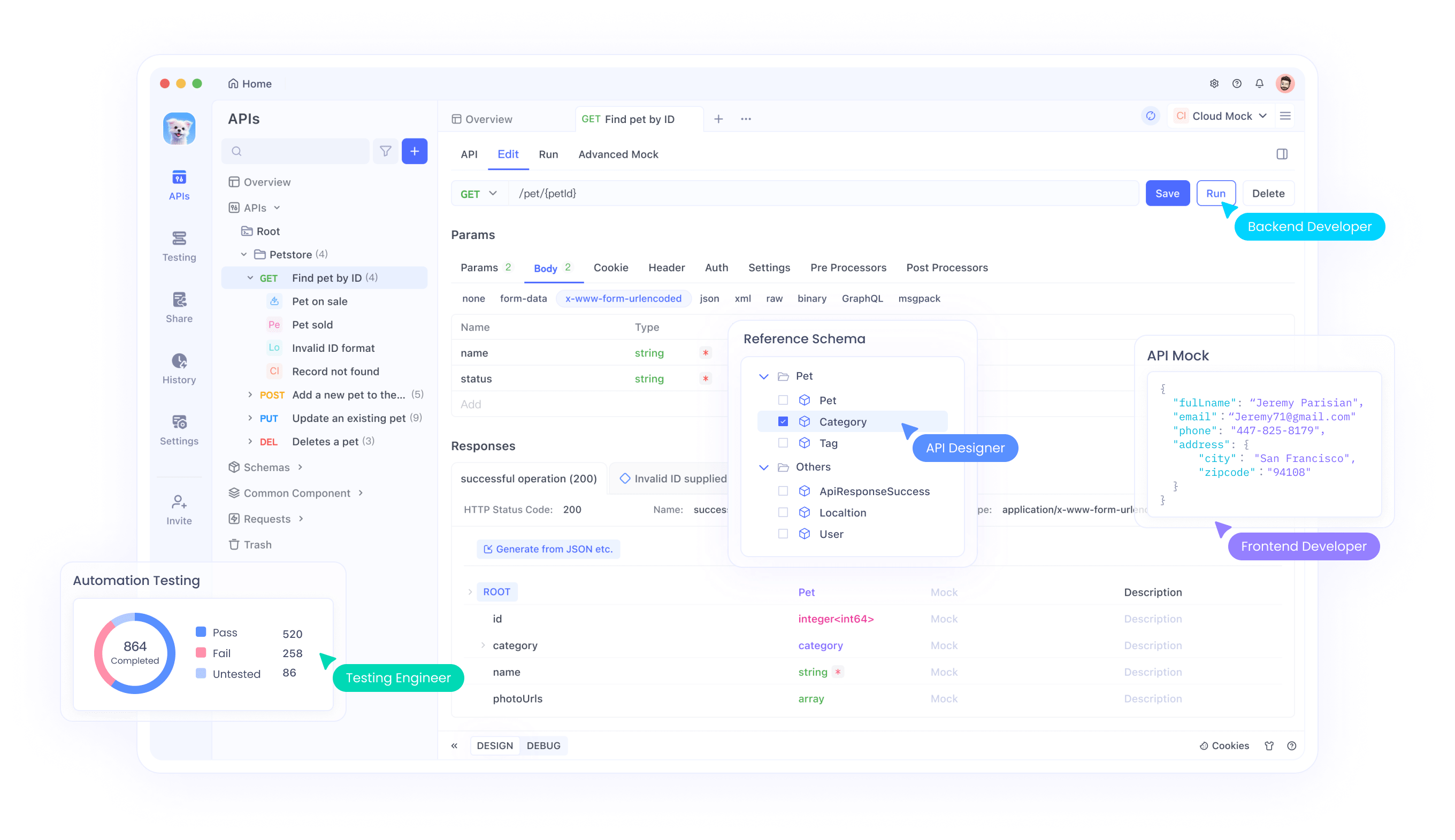
Start Working with cURL APIs by Importing them to Apidog
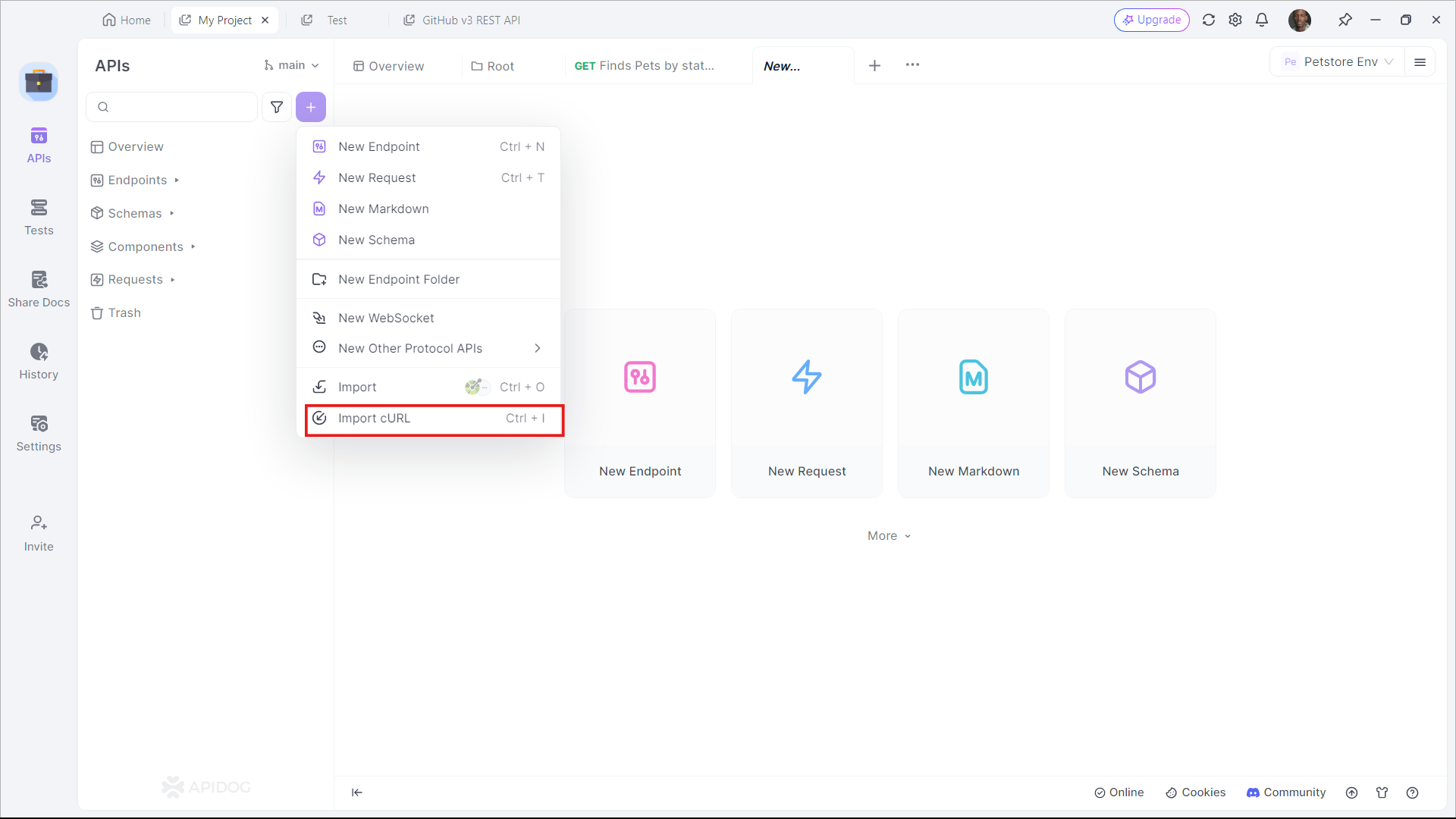
Apidog supports users who wish to import cURL commands to Apidog. In an empty project, click the purple +
button around the top left portion of the Apidog window, and select Import cURL
.
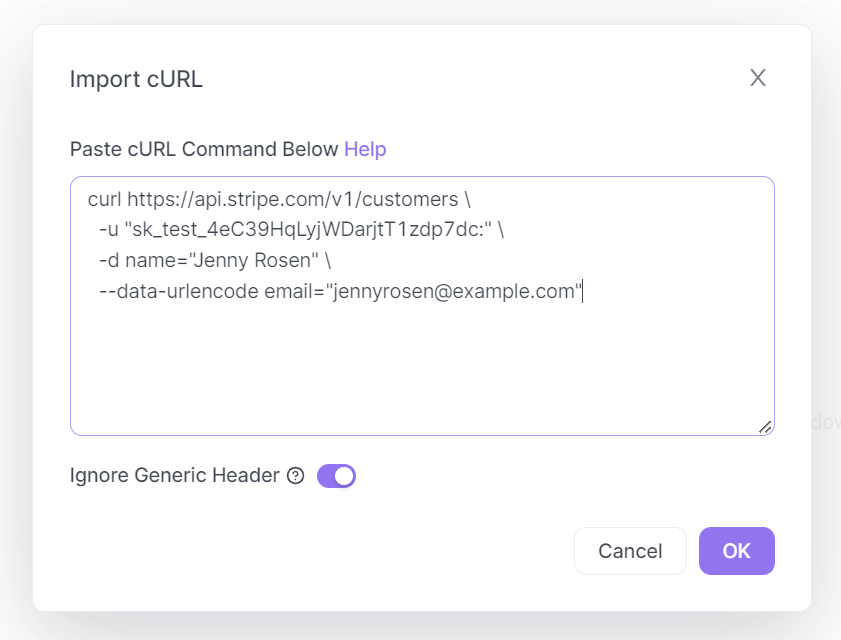
Copy and paste the cURL command into the box displayed on your screen.
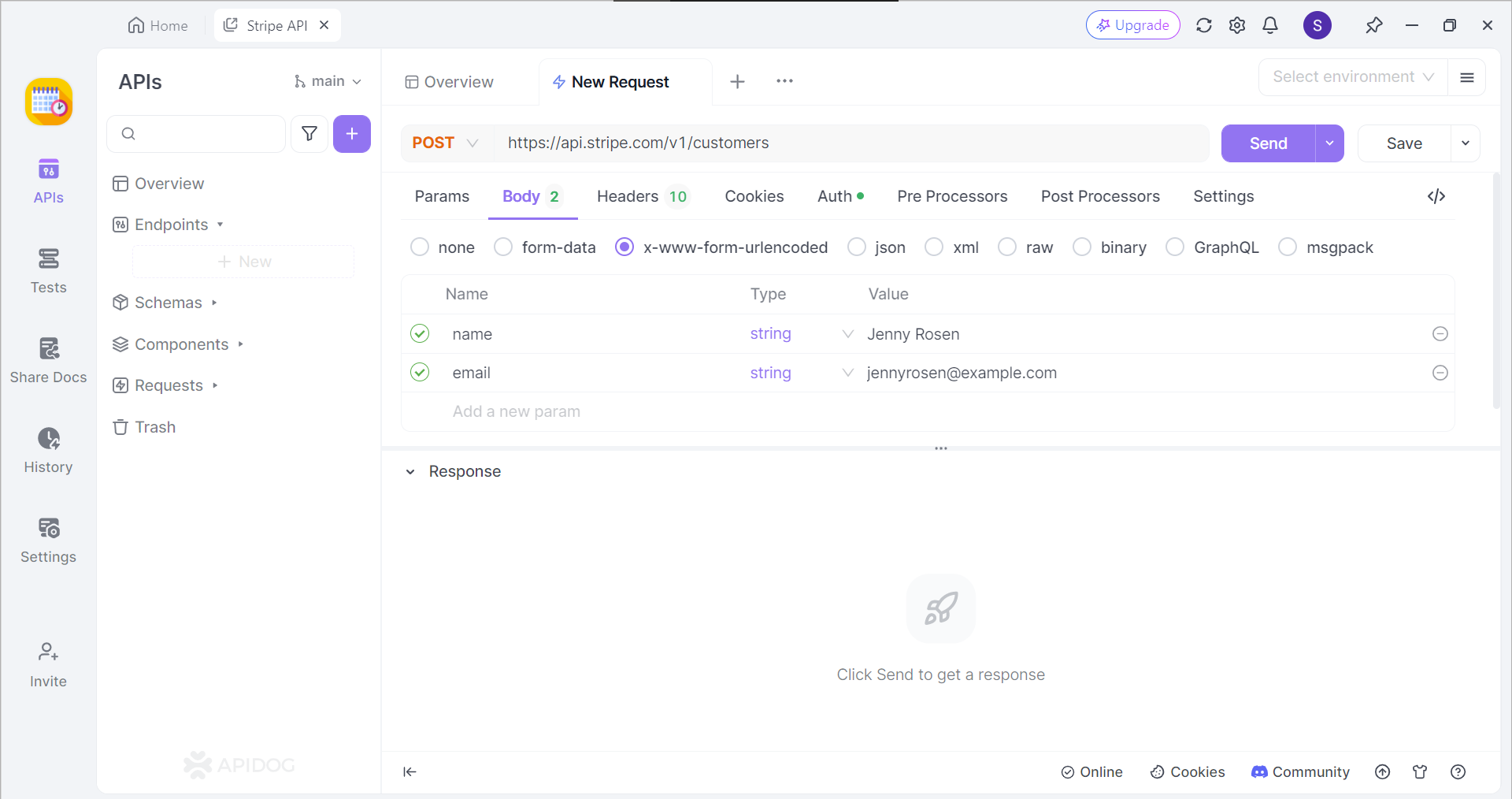
If successful, you should now be able to view the cURL command in the form of an API request.
Generate Javascript Fetch Code Instantly
Apidog can generate the necessary Javascript code for your application within the blink of an eye.
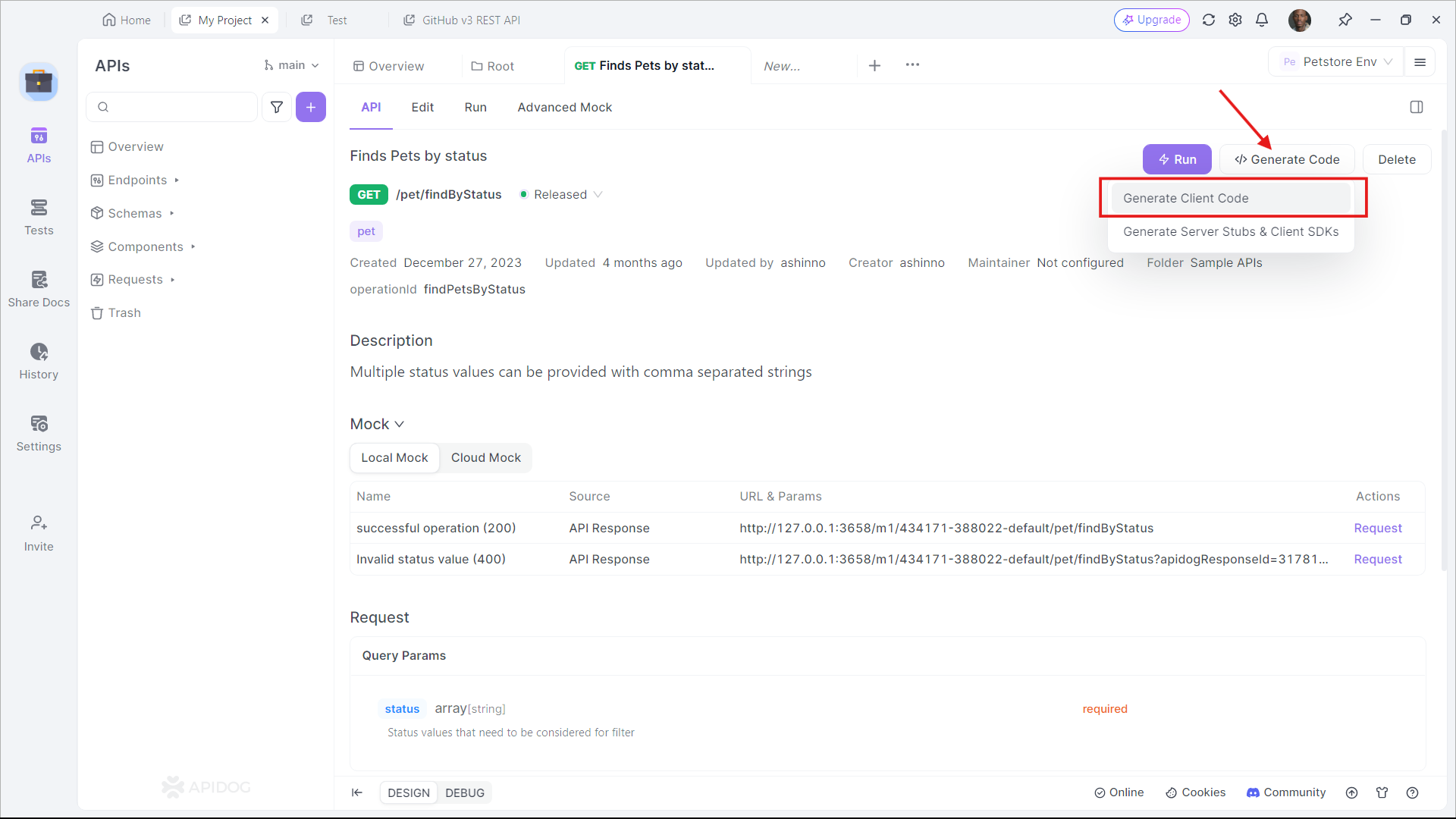
First, locate the </> Generate Code
button on any API or request, and select Generate Client Code
on the drop-down list.
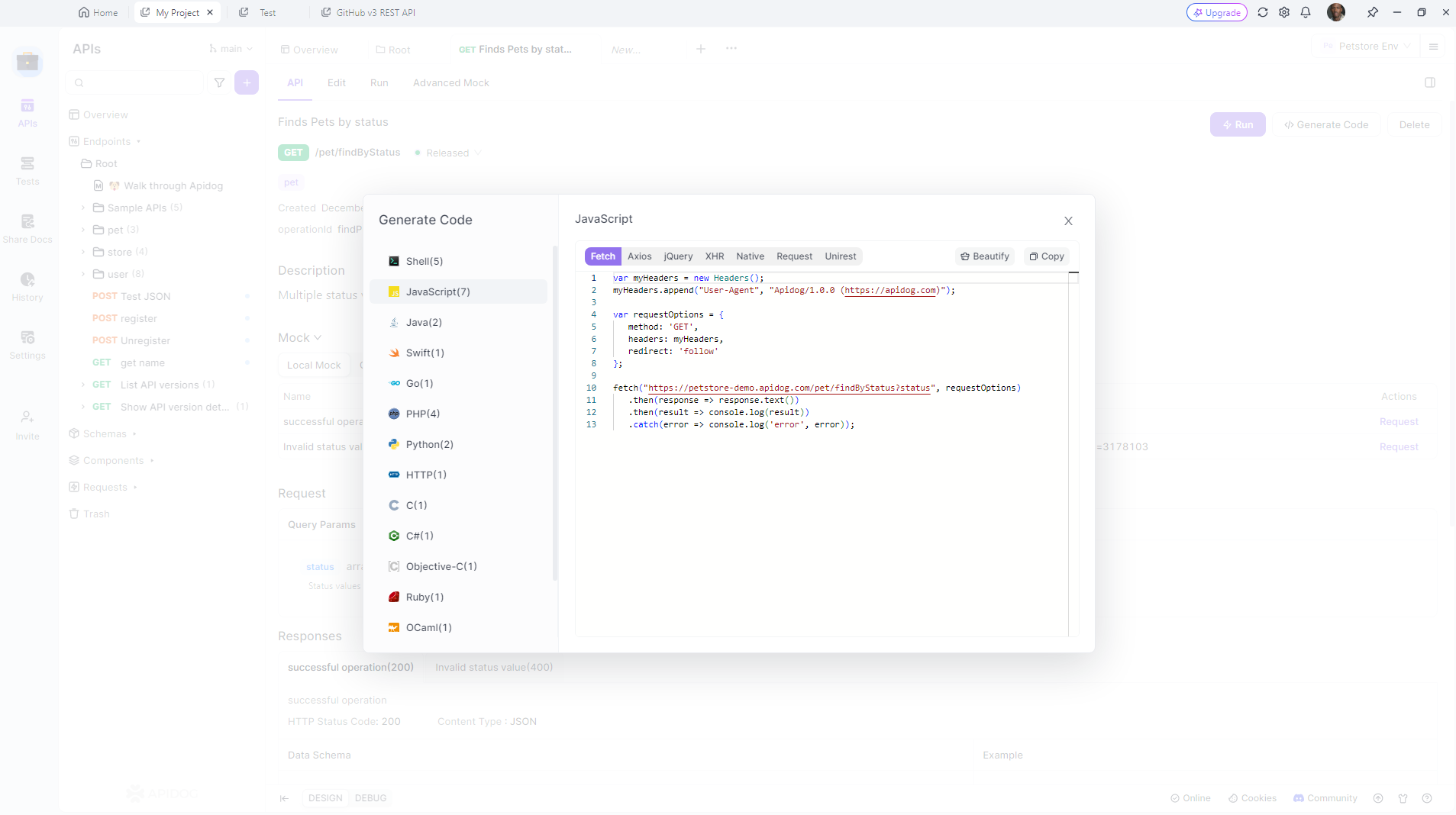
Next, select Javascript, and find the Fetch section. You should now see the generated code. All you have to do is copy and paste it to your IDE (Integrated Development Environment) and continue developing your application.
Best Practices for API Requests
Securing API Keys
Always keep your API keys secure. Avoid hardcoding them in your code, especially in front-end applications. Use environment variables or secure vaults to manage your keys.
Handling Errors
Proper error handling ensures that your application can gracefully handle failures. Always check for response status codes and handle errors appropriately.
Optimizing Performance
For performance optimization, consider:
- Caching responses
- Minimizing the number of API calls
- Using efficient data formats (e.g., JSON)
Advanced Topics
Using cURL with Scripts
You can automate cURL commands using shell scripts. This is useful for repetitive tasks, such as data fetching, batch processing, or testing APIs.
#!/bin/bash
API_URL="https://api.example.com/data"
API_KEY="your_api_key"
response=$(curl -H "Authorization: Bearer $API_KEY" $API_URL)
echo $response
Using fetch with Async/Await
async
and await
make fetch requests even more readable and manageable:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
fetchData();
Conclusion
In this guide, we’ve explored how to make API requests using cURL and JavaScript fetch. Both tools have their strengths and are suited to different tasks. cURL excels in command-line operations and scripting, while fetch is perfect for web applications and asynchronous operations.
Remember, if you want to simplify your API testing process, consider downloading Apidog for free. It’s an excellent tool that makes working with APIs much easier.