Getting Started with Array JavaScript
Arrays are a fundamental data structure in JavaScript, and they allow you to store and manipulate collections of data. In this blog post, we will explore the concept of arrays in JavaScript and discuss some common array methods and operations.
Arrays are a fundamental data structure in JavaScript, and they allow you to store and manipulate collections of data. In this blog post, we will explore the concept of arrays in JavaScript and discuss some common array methods and operations.
In this blog post, we've explored the concept of arrays in JavaScript and discussed common array methods and object traversal techniques. Arrays are powerful data structures in JavaScript that allow you to store, manipulate, and operate on collections of data efficiently.
What is an Array in JavaScript?
An array in JavaScript is a collection of values that can be of any data type, including numbers, strings, objects, and even other arrays. Arrays are ordered and indexed, which means each element in an array has a position or index, starting from zero.
Here's how you can create a simple array in JavaScript:
const fruits = ["apple", "banana", "orange"];
In this example, fruits
is an array containing three string values. You can access the elements of an array by their index, like this:
console.log(fruits[0]); // Output: "apple"
console.log(fruits[1]); // Output: "banana"
console.log(fruits[2]); // Output: "orange"
Arrays are versatile and can be used for a wide range of tasks, such as storing lists of items, managing data, and performing operations on data sets.
Array Properties
Arrays have some built-in properties, mainly including:
constructor: Returns the Array function that created the array.
length: Reflects the number of elements in an array.
prototype: Allows you to add properties and methods to the array object.
For example:
const fruits = ['apple', 'banana', 'orange'];
fruits.constructor // Array()
fruits.length // 3
fruits.prototype // Array.prototype
The constructor property returns the Array constructor function that created this array.
The length property reflects the number of elements in the array.
The prototype property points to the prototype object of arrays, Array.prototype, allowing you to add custom properties and methods to the array.
Additionally, arrays inherit methods like forEach(), map(), filter() etc from their prototype object Array.prototype.
Through the built-in properties of arrays, we can get basic information about the array; by modifying the prototype property, we can customize the behavior of arrays. The properties of arrays provide the foundation for working with arrays.
The Object Traversal Methods in JavaScript
In JavaScript, one common task is traversing objects, including arrays. We often need to iterate through an object's properties and values to perform various operations. Let's explore some of the methods you can use to traverse objects in JavaScript.
Array Methods in JavaScript with Example
Now that we've discussed the object traversal methods, let's look at some example array methods in JavaScript that allow you to manipulate arrays effectively.
forEach
- forEach() - Executes a provided callback function once for each element in the array. Does not mutate the original array.
js
Copy code
const fruits = ['apple', 'banana', 'orange'];
fruits.forEach(fruit => {
console.log(fruit);
});
The forEach() method iterates over the array and executes the callback function once for each element.
The callback function accepts three arguments:
- The current element
- The current index
- The array itself
You can access these arguments like this:
js
Copy code
fruits.forEach((fruit, index, array) => {
console.log(fruit, index, array);
});
The iteration order is determined by the array indexes.
forEach() is commonly used to execute a operation on each element in the array, like logging:
js
Copy code
fruits.forEach(fruit => {
console.log(fruit);
});
Or mapping to a new array:
js
Copy code
const upperCased = [];
fruits.forEach(fruit => {
upperCased.push(fruit.toUpperCase());
});
join
- join() - Joins all elements of an array into a string. You can optionally specify a separator that will be used between each element.
js
Copy code
const fruits = ['apple', 'banana', 'orange'];
fruits.join(); // 'apple,banana,orange'
fruits.join(' & '); // 'apple & banana & orange'
Push
- push() - Adds one or more elements to the end of an array and returns the new length of the array. This mutates the original array by modifying its length and adding the new elements.
js
Copy code
const fruits = ['apple', 'banana'];
fruits.push('orange'); // 3
fruits.push('mango', 'pear'); // 5
pop
- pop() - Removes the last element from an array and returns that element. This mutates the array by reducing its length by 1 and changing the contents.
js
Copy code
const fruits = ['apple', 'banana', 'orange'];
fruits.pop(); // 'orange'
unshift
- unshift() - Adds one or more elements to the beginning of an array and returns the new length. Mutates the array by increasing length and adding elements.
js
Copy code
const fruits = ['apple', 'banana'];
fruits.unshift('grape'); // 3
fruits.unshift('mango', 'pear'); // 5
- shift() - Removes the first element of an array and returns that element. Mutates the array by removing the first element and decreasing length.
js
Copy code
const fruits = ['apple', 'banana', 'orange'];
fruits.shift(); // 'apple'
- indexOf() - Returns the first index at which a given element can be found in the array, or -1 if it is not present.
js
Copy code
const fruits = ['apple', 'banana', 'orange', 'apple'];
fruits.indexOf('apple'); // 0
fruits.indexOf('grape'); // -1
- includes() - Determines whether an array contains a certain element, returning true or false. Does not mutate the array.
js
Copy code
const fruits = ['apple', 'banana', 'orange'];
fruits.includes('apple'); // true
fruits.includes('grape'); // false
Automated Testing and Array Traversal in Apidog
Apidog is a powerful automation testing tool designed to provide developers with convenient interface testing and data traversal capabilities. In this feature overview, we will focus on Apidog's automated testing capabilities and its ability to traverse arrays.
Automated Testing
Apidog offers a range of automated testing tools to help you easily validate the correctness of your backend interfaces and services. These tools include:
- API Testing
Apidog supports testing for multiple protocols, including HTTP(S), WebSocket, Socket, gRPC, and more. You can use Apidog to send requests, validate responses, check status codes, and handle complex interface testing scenarios.
- Integration Testing
For complex applications or services, integration testing is crucial. Apidog allows you to create and manage test collections for multiple interfaces, ensuring that various interfaces work together as expected.
- Data-Driven Testing
Apidog supports data-driven testing, enabling you to easily load test data and automatically run test cases to ensure that interfaces perform well under different input data scenarios.
- Scripted Testing
For advanced testing scenarios, Apidog provides scripted testing capabilities, allowing you to write custom testing logic using scripts.
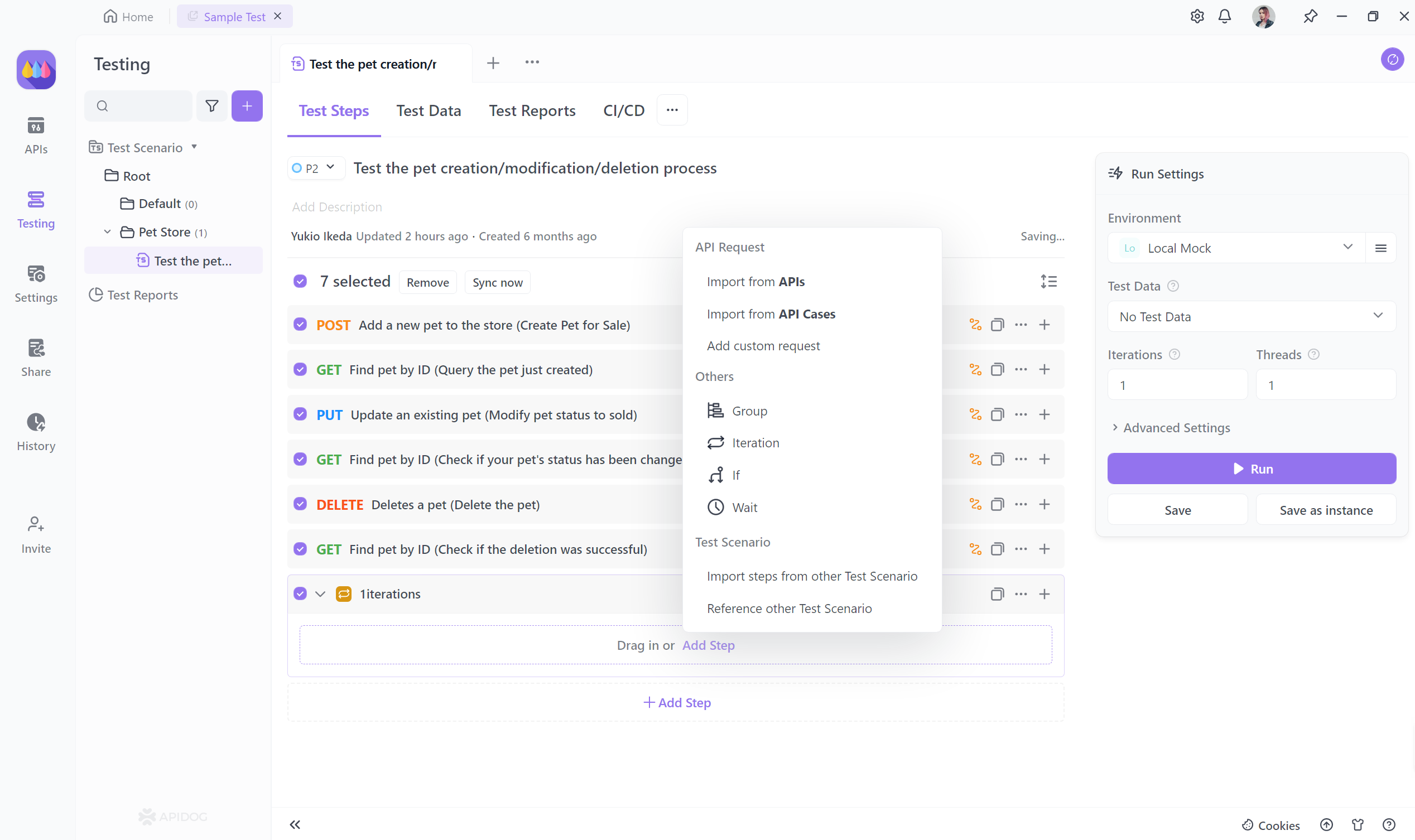
Array Traversal Capability
Apidog also boasts a robust array traversal feature, which empowers you to test and verify interface behavior more efficiently. Here are the key features of Apidog's array traversal:
- Data Generation: Apidog enables you to generate array data to simulate various input scenarios. This is valuable for testing the performance and stability of interfaces under different data loads.
- Data Traversal: With Apidog, you can effortlessly traverse array data and pass it as parameters to interface test cases. This allows you to cover multiple data points in a single test, ensuring that the interface functions correctly under various input conditions.
- Data Assertion: Apidog permits data assertion during array traversal. This means you can verify if the response for each data point meets your expectations, ensuring interface accuracy.
- Loop Testing: If you need to run the same test case multiple times, Apidog supports loop testing, making it easy to test interface performance in consecutive requests.
Conclusion
Understanding how to traverse objects using methods like for...in
, Object.keys()
, Object.values()
, and Object.entries()
is crucial when working with complex data structures. Additionally, array methods like forEach()
, map()
, filter()
, and reduce()
provide powerful tools for manipulating and transforming arrays.