JavaScript - Variables: A Deep Dive
In JavaScript, variables are fundamental building g blocks that allow developers to store and manipulate data. A variable is essentially a named container that holds a value, which can be of various types, such as numbers, strings, objects, and functions.
JavaScript, often referred to as the "language of the web," is a versatile and widely-used programming language that plays a pivotal role in web development. It enables developers to create dynamic, interactive web pages and applications. At the core of JavaScript lies the concept of variables, which serve as containers for storing and managing data. In this comprehensive blog post, we will take a deep dive into JavaScript variables, exploring their types, scope, best practices, and some advanced use cases.
What are the Variables in JavaScript?
In JavaScript, variables are fundamental building g blocks that allow developers to store and manipulate data. A variable is essentially a named container that holds a value, which can be of various types, such as numbers, strings, objects, and functions. Variables are like labeled boxes in which you can place different items (data) and change their contents as needed.
JavaScript's power lies not only in variables but also in its ability to work with arrays, making it fundamental for front-end development. Arrays allow you to store and manipulate collections of data efficiently. However, as your codebase grows, debugging becomes crucial. This is where the best 7 JavaScript Debugging Tools' step in, simplifying the process and helping you identify and resolve issues quickly. If you're new to front-end development, the 'JavaScript Online Debugging Front-end Beginner's Guide' is a valuable resource that offers insights into both variables and arrays, bridging the gap for effective coding practices.
Declaring Variables
In JavaScript, you declare a variable using the var
, let
, or const
keyword, followed by the variable's name. Here's a brief overview of these keywords:
var
: Historically,var
was the primary keyword used to declare variables in JavaScript. However, it has some quirks and limitations, particularly related to variable scope, which we will discuss later.let
: Introduced in ECMAScript 6 (ES6),let
provides better scoping rules and is generally preferred overvar
for declaring variables within a block scope.const
: Also introduced in ES6,const
is used to declare variables that should never be reassigned after their initial value is assigned. It is commonly used for declaring constants.
Variable Naming Rules
When naming variables in JavaScript, you need to adhere to certain rules and conventions:
- Variable names are case-sensitive, so
myVariable
andmyvariable
are treated as different variables. - Variable names can only consist of letters (a-z, A-Z), digits (0-9), underscores (_), or dollar signs ($). They must start with a letter, underscore, or dollar sign.
- JavaScript reserves certain keywords that cannot be used as variable names (e.g.,
if
,else
,while
,function
,return
, etc.). - Variable names should be descriptive and meaningful to enhance code readability. For example, use
totalAmount
instead ofx
to store a total amount.
Data Types
JavaScript is a dynamically typed language, which means variables can hold values of different data types. The main data types in JavaScript include:
Primitive Data Types:
Number
: Represents numeric values, both integers and floating-point numbers (e.g., 42
, 3.14
).
String
: Represents text data enclosed in single ('') or double ("") quotes (e.g., 'Hello, World!'
).
Boolean
: Represents either true
or false
, often used for conditional logic.
Undefined
: Represents a variable that has been declared but has not been assigned a value.
Null
: Represents the intentional absence of any object value.
Symbol
(ES6): Represents a unique and immutable value, primarily used as object property keys.
BigInt
(ES11): Represents large integers, useful for handling extremely large numbers.
Reference Data Types:
Object
: Represents complex data structures and objects.
Array
: A special type of object used to store ordered lists of values.
Function
: Represents reusable blocks of code that can be executed.
Understanding data types is crucial when working with variables, as it determines how the data is stored and processed.
Type of Variables JavaScript
Variable scope defines where in your code a variable can be accessed. JavaScript has two main types of variable scope: global scope and local scope.
Global Variable JavaScript
Variables declared outside of any function or code block have global scope. They can be accessed from anywhere in your code, including within functions and code blocks.
javascriptCopy code
var globalVar = 42;
function myFunction() {
console.log(globalVar); // Accessible
}
While global scope provides accessibility, it comes with some potential issues, such as variable name clashes and reduced code encapsulation.
Local Scope
Variables declared inside a function or a code block have local scope. They can only be accessed within that specific function or block.
javascriptCopy code
function myFunction() {
var localVar = 'Local variable';
console.log(localVar); // Accessible
}
console.log(localVar); // Not accessible (throws an error)
Local scope offers better encapsulation, as variables are confined to a specific context, reducing the risk of unintended side effects and name conflicts.
Block Scope
Block scope was introduced with the let
and const
keywords in ES6. Unlike, which has function scope, let
and const
have block scope. This means they are limited to the code block they are declared in, such as loops or conditional statements.
javascriptCopy code
if (true) {
let blockVar = 'Block variable';
console.log(blockVar); // Accessible
}
console.log(blockVar); // Not accessible (throws an error)
Block scope enhances code predictability by limiting the visibility of variables to the specific block where they are declared.
Hoisting
Hoisting is a JavaScript behavior where variable declarations (not their assignments) are moved to the top of their containing scope during compilation. This can lead to unexpected results if not understood correctly.
javascriptCopy code
console.log(hoistedVar); // undefinedvar hoistedVar = 'I am hoisted';
In the example above, hoistedVar
is declared using var
and is hoisted to the top of its containing function or global scope. However, its assignment ('I am hoisted'
) remains in place. As a result, the variable is declared but uninitialized, leading to undefined
.
To avoid issues related to hoisting, it's a good practice to always declare variables at the beginning of their containing scope and initialize them with values as needed.
Apidog: Visual Interface for Variable Editing and Retrieval
Apidog is not just your run-of-the-mill testing tool; it goes the extra mile to provide developers with a user-friendly and feature-rich visual interface. Among its many capabilities, Apidog offers a visual interface that simplifies the process of editing and retrieving variables, making it an invaluable asset in your development toolkit.
Streamlined Variable Editing
Apidog's visual interface offers a streamlined approach to variable editing. Whether you're working with simple data types like strings and numbers or complex objects and arrays, Apidog's intuitive interface makes it easy to modify variables on the fly.
1. User-Friendly Interface: Apidog's interface is designed with developers in mind. It provides a clean and user-friendly environment where you can view and edit variables effortlessly.
2. Real-Time Updates: As you make changes to variables, Apidog provides real-time updates. You can see the effects of your edits immediately, which is particularly handy for debugging and experimentation.
3. Variable History: Apidog keeps a record of variable changes, allowing you to revisit and revert to previous states if needed. This feature is a lifesaver when troubleshooting complex scenarios.
Effortless Variable Retrieval
In addition to editing variables, Apidog excels at retrieving and displaying variable values. Whether you're working with variables in your code or inspecting the responses from API calls, Apidog simplifies the process of accessing and utilizing your data.
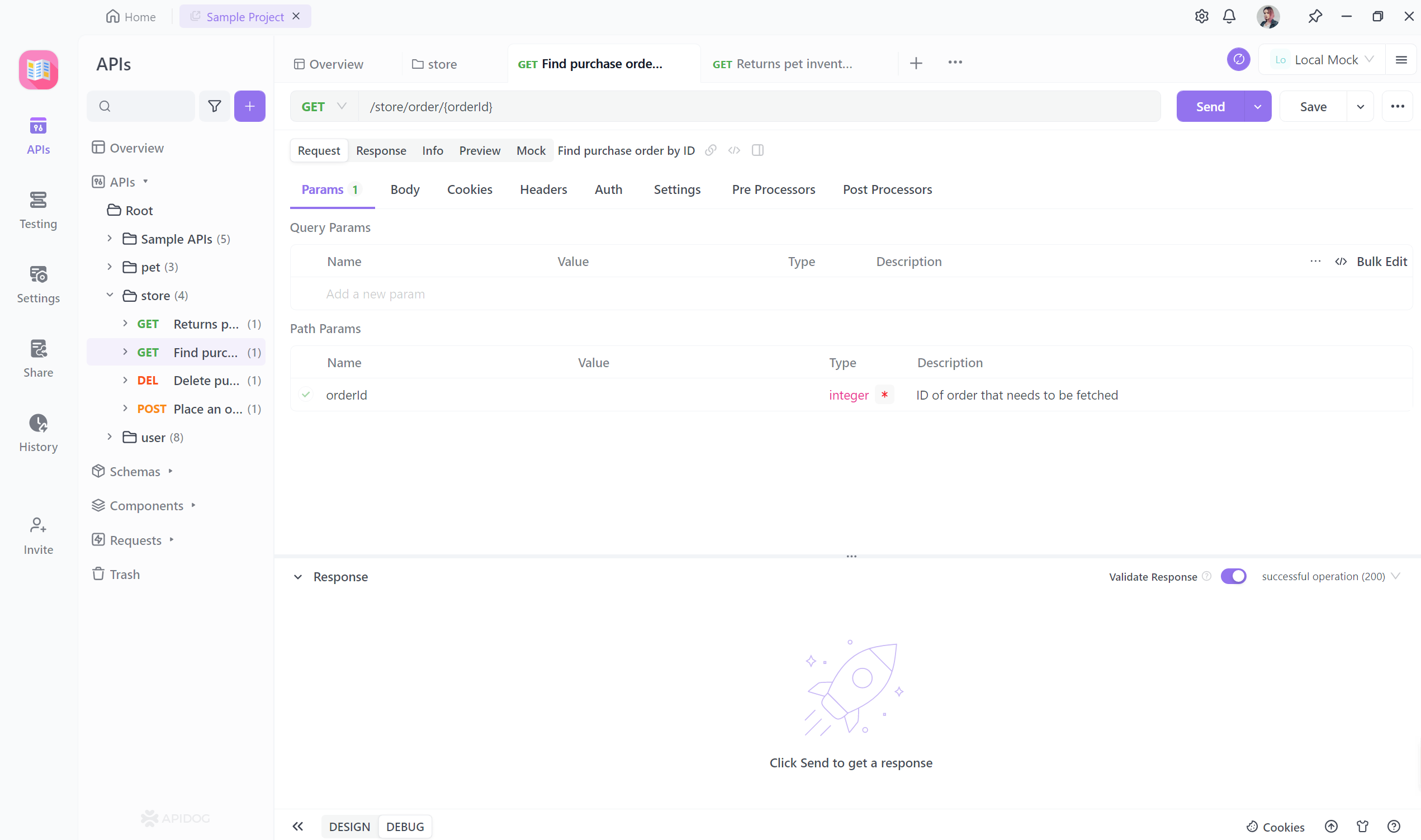
1. Variable Inspections: Apidog's interface includes dedicated tools for inspecting variables within your code. You can easily view variable values, data types, and structures without sifting through your script manually.
2. Variable Extraction: When working with API responses, Apidog's visual interface allows you to extract specific variables effortlessly. You can select the desired variables and copy them for further use in your application.
3. Interactive Debugging: Debugging becomes a breeze with Apidog's interactive variable retrieval. You can set breakpoints, inspect variables at runtime, and even modify them on the fly to troubleshoot issues effectively.
4. Data Visualization: For complex data structures like arrays and objects, Apidog offers data visualization tools. You can expand and collapse nested elements, making it easy to explore data hierarchies.
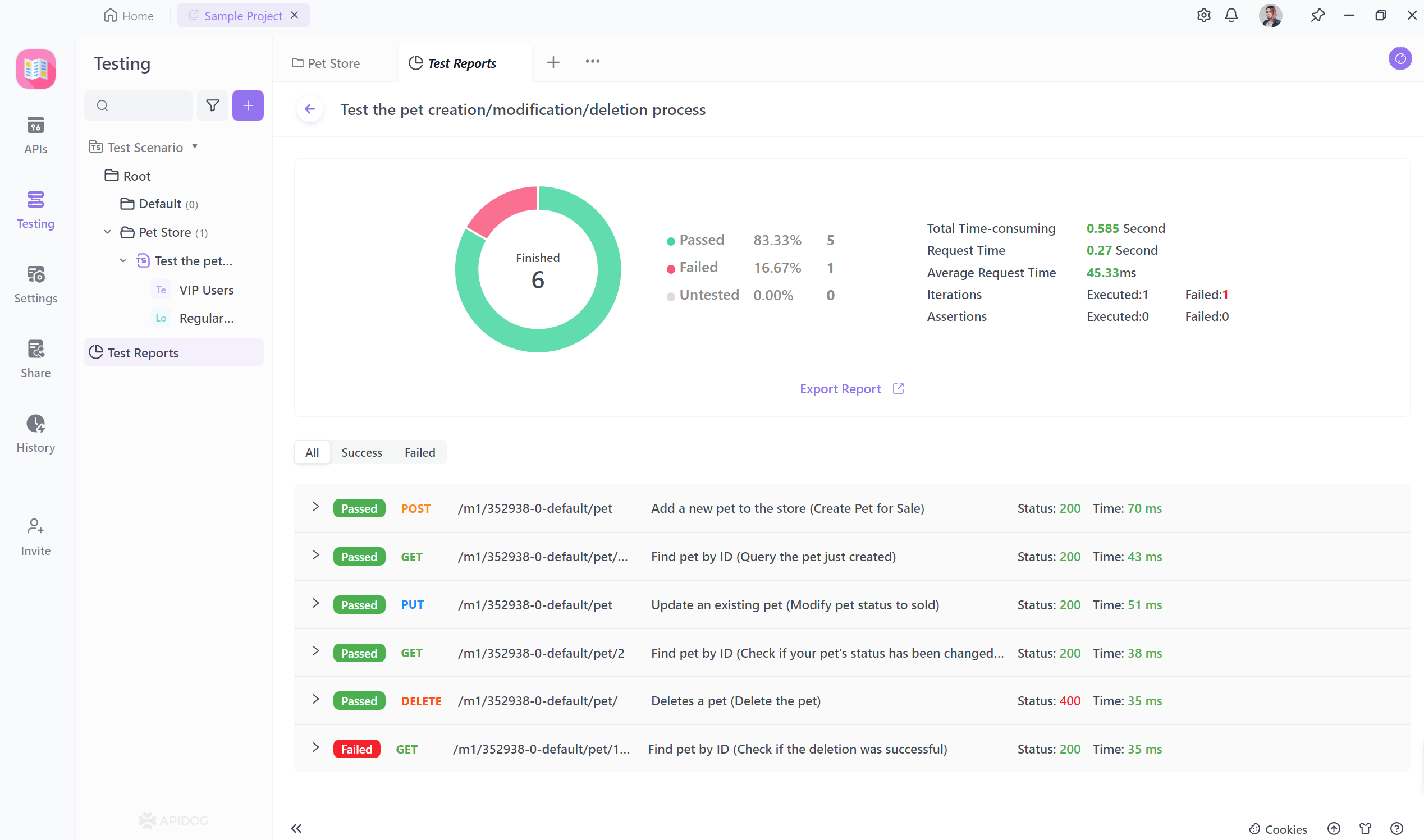
Best Practices
When working with variables in JavaScript, there are several best practices to follow to ensure clean, maintainable, and error-free code:
Use let
and const
Over var
Prefer using let
and const
for variable declarations over the older var
. They offer better scoping and are less prone to unexpected behavior.
Avoid Global Variables
Minimize the use of global variables to prevent naming conflicts and maintain code encapsulation. If a variable is only needed within a specific function or block, declare it with local scope.
Descriptive Variable Names
Choose meaningful and descriptive variable names that reflect their purpose. This improves code readability and makes it easier for other developers to understand your code.
Initialize Variables
Always initialize variables when declaring them. This helps prevent unexpected behavior due to hoisting and ensures variables have valid initial values.
Use Constants for Unchanging Values
When a variable's value should never change after assignment, declare it using const
to indicate that it is a constant. This enhances code clarity and prevents accidental reassignment.
Be Mindful of Variable Shadowing
Variable shadowing occurs when a variable in an inner scope has the same name as a variable in an outer scope, effectively hiding the outer