How to Use the Event Loop in Node.js?
In this article, we'll take a deep dive into how the event loop works, providing common usage scenarios and methods, as well as practical examples to demonstrate how to effectively utilize the event loop in Node.js.
The Node.js event loop is a very efficient way to handle I/O operations. It allows the program to perform other tasks while waiting for the I/O to complete without blocking the entire process. This makes Node.js ideal for building high-throughput, low-latency web applications.
In this article, we'll take a deep dive into how the event loop works, providing common usage scenarios and methods, as well as practical examples to demonstrate how to effectively utilize the event loop in Node.js.
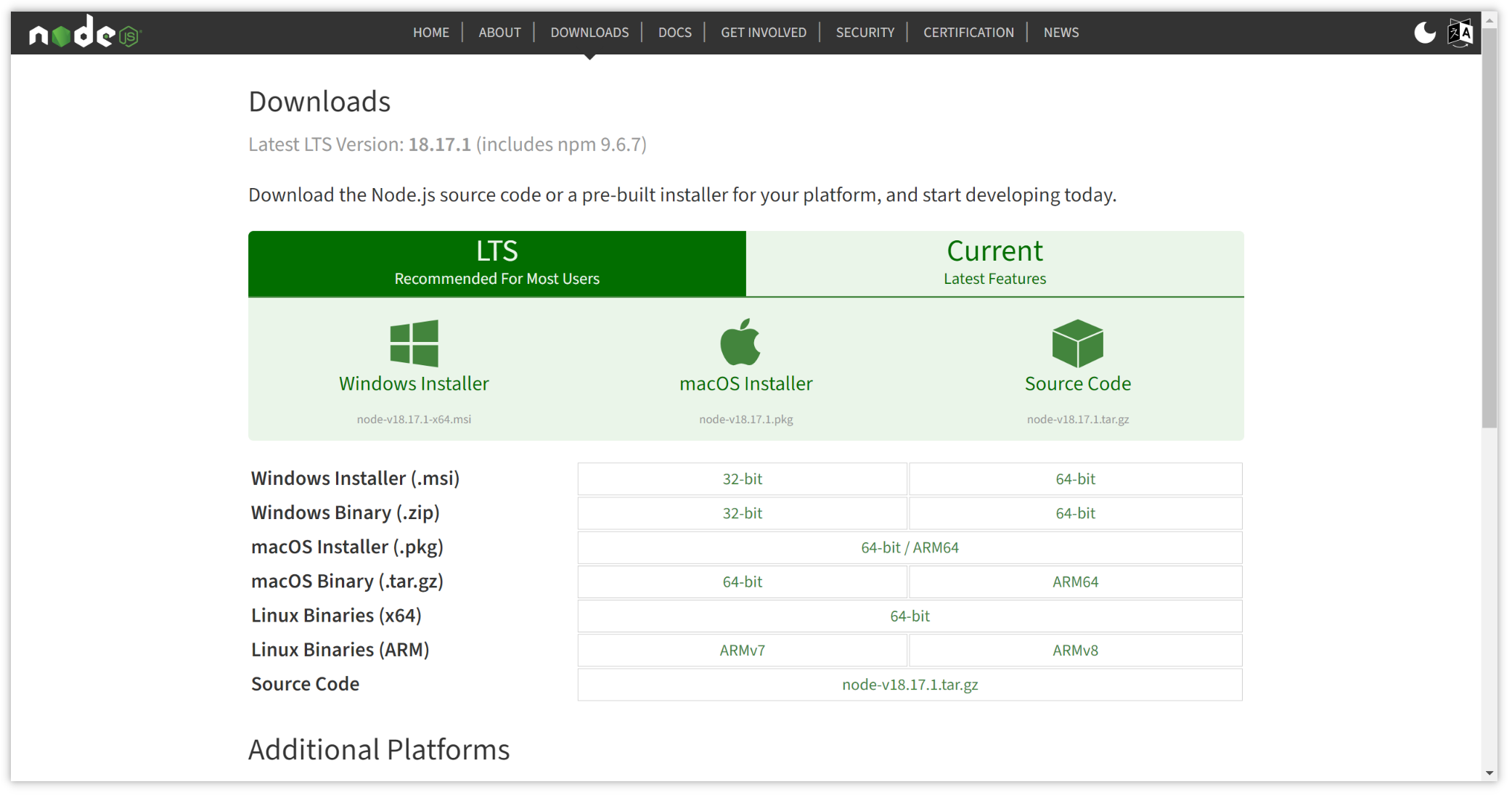
The Principle of Event Loop
The event loop is at the heart of Node.js and is based on a single-threaded execution model but allows asynchronous operations. This is achieved by queuing tasks into an event queue and then executing these tasks at different stages of the event loop. The event loop of Node.js roughly follows the following stages:
- Timers : At this stage, timer tasks will be executed, including callback functions registered through
setTimeout()
and functions.setInterval()
- I/O Callbacks : At this stage, callback functions for all completed I/O operations are executed, such as reading files, network requests, etc.
- Idle, prepare : This is an internal phase and is generally not triggered by user code.
- Poll : In this phase, Node.js will check if there are new I/O events to handle. If so, it will execute the corresponding callback function.
- Check : At this stage,
setImmediate()
the registered callback function is executed. - Close Callbacks : In this phase, some close callback functions are executed,
socket.on('close', ...)
e.g.
The event loop continuously switches between these stages, processing tasks in the queue, until the queue is empty. This non-blocking model enables Node.js to handle large numbers of concurrent connections without blocking because it can perform other tasks while waiting for I/O.
When to Use Node.js Event Loop?
The Node.js event loop is suitable for many application scenarios, especially those that require high throughput and low latency. Here are some common usage scenarios:
- Web Server : Node.js is often used to build high-performance web servers capable of handling large numbers of concurrent requests. The event loop makes it possible to handle multiple requests simultaneously without blocking the processing of other requests.
- Real-time applications: For real-time chat applications, online games, or real-time notification services, Node.js’ event loop makes it easy to handle real-time events and messages.
- Backend API services : Node.js can be used to build backend API services that can efficiently handle HTTP requests through event loops and communicate asynchronously with databases or other services.
- Data stream processing : To handle large amounts of data streams, such as log file processing or data import and export, Node.js's event loop can provide efficient solutions.
- Network proxy : Node.js can be used as a network proxy server, which can handle multiple connections and implement proxy functions.
Basic concepts of event loop
1. setTimeout() and setInterval()
setTimeout()
and setInterval()
are two common methods for scheduling cron tasks in an event loop. They are used as follows:
// One-time scheduled task
setTimeout(() => {
console.log('One-time task executed');
}, 1000); // Executes after 1 second
// Recurring scheduled task
setInterval(() => {
console.log('Recurring task executed');
}, 2000); // Executes every 2 seconds
2. setImmediate()
setImmediate()
Used to execute a callback function in a stage of the event loop Check
. It is executed after the current stage and is usually used for tasks that need to be executed as soon as possible.
setImmediate(() => {
console.log('setImmediate task executed');
});
3. Event listener
The core module of Node.js events
provides event listening and triggering functions. You can create custom events and then trigger them at the appropriate time.
const EventEmitter = require('events');
const myEmitter = new EventEmitter();
myEmitter.on('customEvent', (message) => {
console.log(`Received message: ${message}`);
});
myEmitter.emit('customEvent', 'Hello, Node.js!');
4. Asynchronous file operations
Node.js fs
modules allow you to perform asynchronous file operations, such as reading files or writing files. These operations add callback functions to the event queue and are executed during the I/O Callbacks phase.
const fs = require('fs');
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
Best Practice for Event Loop in Node.js
File reading case using event loop: We will create a Node.js application that reads the file content and displays it in the console. This will involve using an event loop to handle asynchronous file read operations.
Step 1: Initialize the project
First, create a new directory and initialize the Node.js project in it. Open a terminal and run the following command:
mkdir nodejs-event-loop-example
cd nodejs-event-loop-example
npm init -y
Step 2: Create JavaScript file
Create a JavaScript file called in the project directory fileReader.js
and add the following code:
const fs = require('fs');
// Asynchronous file reading
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error occurred while reading the file:', err);
return;
}
console.log('File content:', data);
});
console.log('File reading operation initiated');
This code uses the Node.js fs
module to asynchronously read example.txt
the contents of a text file named. When the read operation is completed, the callback function will be executed to process the file contents. At the same time, we print a message in the console to indicate that the file read operation has started.
Step 3: Run the application
Run the following command in the terminal to start the application:
node fileReader.js
The application will read the contents of the file example.txt
and display it in the console. Then, you will see the message "File reading operation initiated", indicating that the file read operation is asynchronous and does not block other parts of the application.
Case Description:
This example demonstrates how the Node.js event loop operates:
- We use the
fs
module in Node.js to execute asynchronous file reading operations, which are typical event-driven I/O operations that add file read requests to the event queue. - We provide a callback function to
fs.readFile
that will be triggered when the file read operation is completed. - In the console, we first output "File reading operation initiated" and then wait for the file read operation to complete.
- Upon completion of the file reading operation, the callback function is executed, and the file contents are displayed in the console.
This case underscores the essential concept of the event loop, which enables a Node.js application to execute multiple operations concurrently without blocking other tasks. By allowing applications to continue executing other tasks while waiting for I/O operations to finish, the event loop enhances program performance and responsiveness.
Apidog: Your All-in-One Interface Testing Solution
As a Node.js developer, navigating APIs is a daily task essential for ensuring your applications run smoothly. That's where Apidog steps in – a robust interface testing tool designed to streamline your backend management.
Apidog surpasses the capabilities of traditional tools like Postman by integrating features akin to Postman, Swagger, Mock, and JMeter – all in one platform. It boasts extensive support for debugging across various protocols, including http(s), WebSocket, Socket, gRPC. This comprehensive approach makes Apidog an invaluable asset for thorough interface testing.
Moreover, Apidog seamlessly integrates with IDEs through its IDEA plug-in, enhancing collaboration and productivity. Its user-friendly graphical interface simplifies project launch and facilitates efficient interface management and testing. Don't miss out – download Apidog now to elevate your development experience!
Unlock the Potential of Node.js Event Loop
The event loop in Node.js serves as a cornerstone for building high-performance, scalable applications. Mastering its principles, application scenarios, and best practices is paramount for Node.js developers.
This article provides a comprehensive overview of Node.js event loop fundamentals, accompanied by practical examples to empower developers in leveraging this powerful mechanism to craft exceptional applications.
For further exploration of Node.js event loop intricacies, refer to the official documentation. Dive into the world of Apidog and harness the full potential of Node.js development today.
Bonus Tips:
Try to avoid performing long-running synchronization operations in the event loop to avoid blocking the execution of other tasks.
- Use
async/await
andPromises
to better manage asynchronous processes and make code easier to understand and maintain. - Use with caution
setImmediate()
and only when necessary to avoid disrupting the normal execution flow of the event loop.