How to Generate API Documentation from Swagger Automatically
In this post, we will provide you with a comprehensive guide on how to generate Swagger documentation for your API. Additionally, we will introduce a valuable tool called Apidog that can help streamline the documentation process.
Most of developers use Swagger to document API automatically, it can provide your team or external collaborators with easy access to your API, enabling users to make accurate calls to your API. To reduce errors caused by manual documentation, many API developers prefer to find good ways to automatically generate API documentation.
In this post, we will provide you with a comprehensive guide on how to generate Swagger documentation for your API. Additionally, we will introduce a valuable tool called Apidog that can help streamline the documentation process.
What is Swagger?
Swagger, or the OpenAPI Specification, is an open-source framework used to define and document RESTful APIs. It provides a standard way of describing RESTful APIs that allows both humans and machines to understand the API's capabilities without accessing its source code, documentation, or network traffic.
Swagger documentation includes critical information about your API, such as endpoints, request and response formats, authentication methods, and more. This information is typically presented in a structured and easy-to-read format, making it an essential resource for developers who need to interact with your API.
How to Create API Documentation in Swagger?
After having a brief knowledge of Swagger, and how to write your API effectively and efficiently using Swagger, let's have a look.
Step 1. Create your new API by clicking the "+" button in the top left of the Swagger interface.
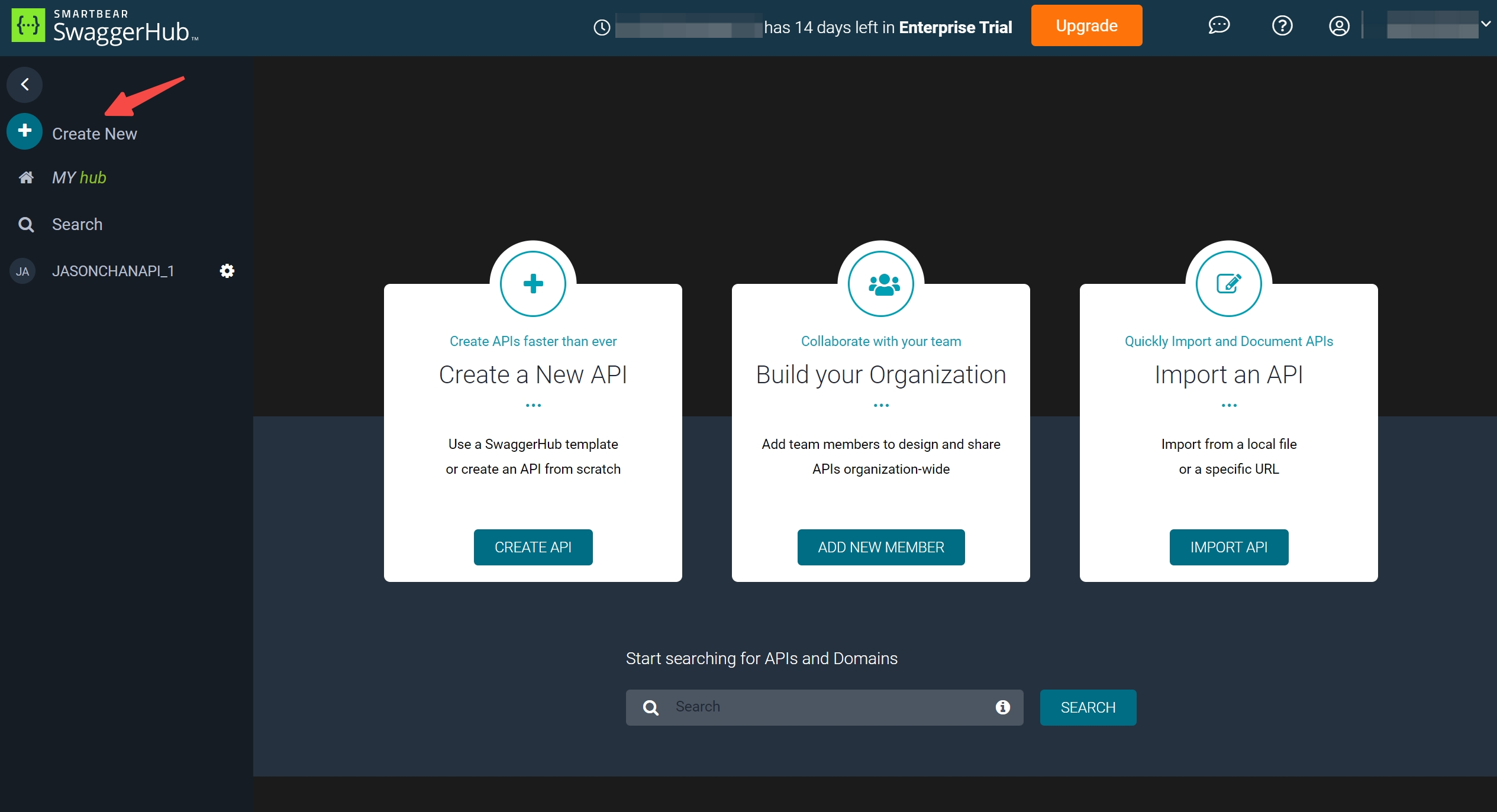
Step 2. Put all the necessary information here, such as owner, specification, template, visibility, etc. Please pay attention to your team organization.
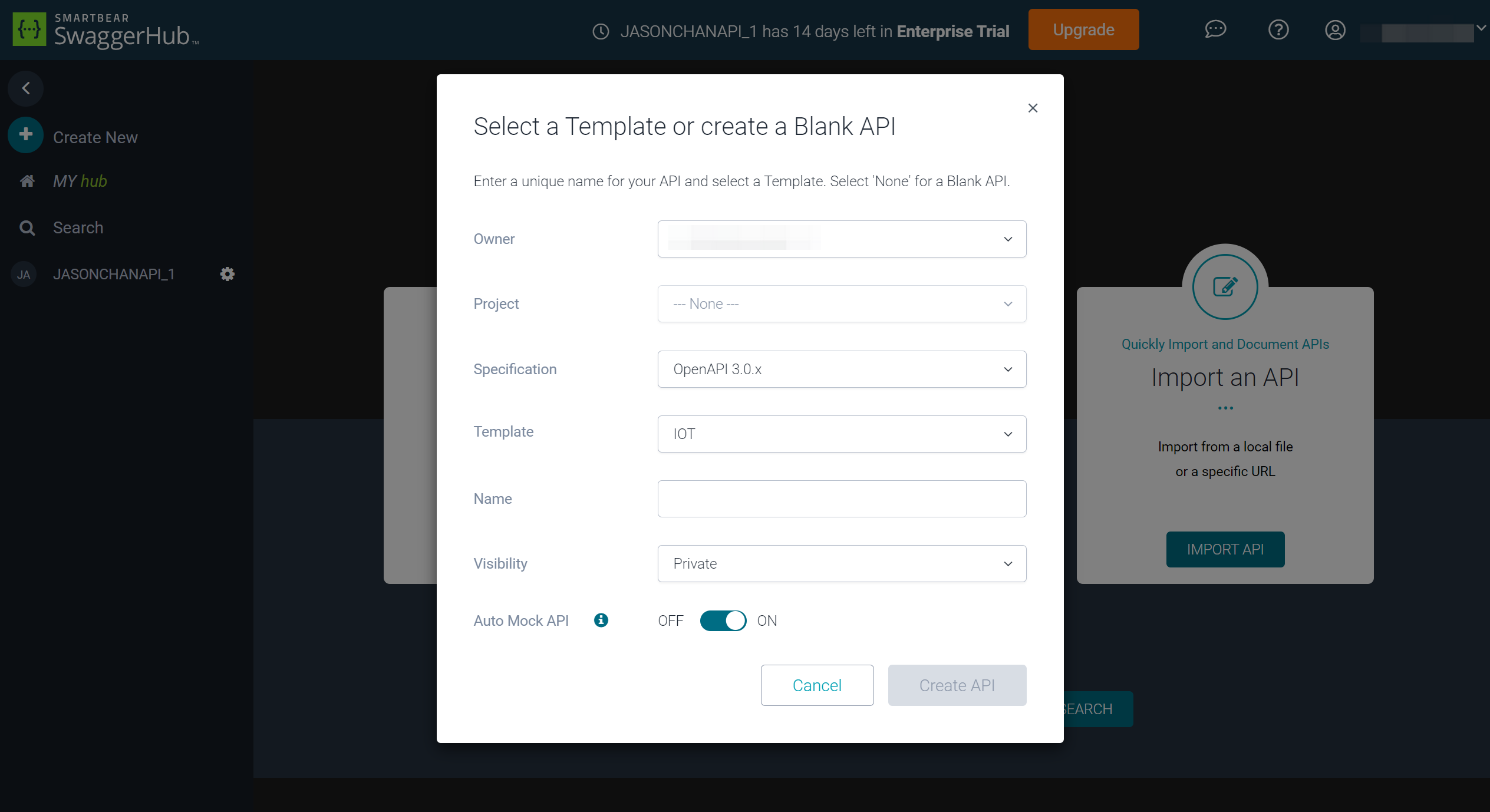
Step 3. Select the OpenAPI specification that you desire. Select the "OpenAPI 2.0" and "OAuth 2.0 Access Code" here.
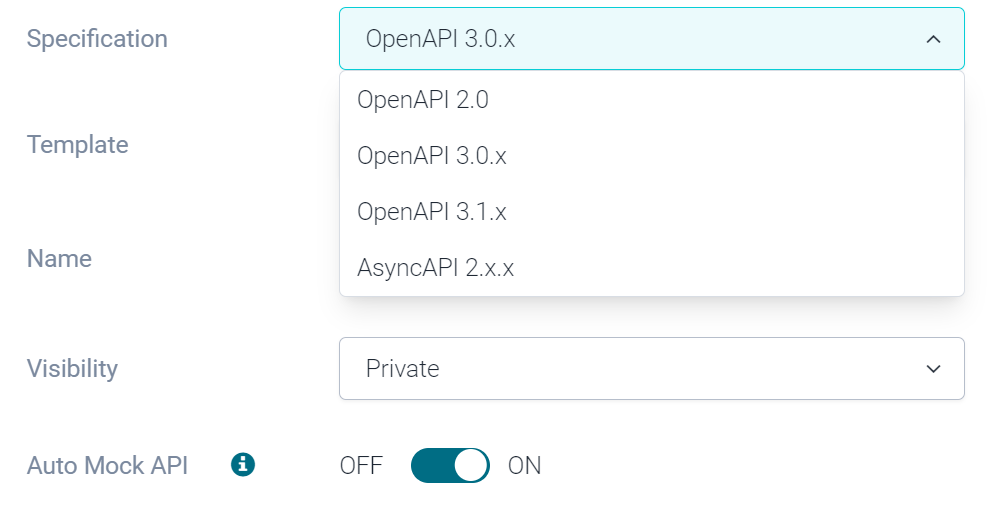
About the template, you can choose to generate API documentation from an empty document. However, if you are a beginner, Swagger also provides many templates for you. Just pick the one you prefer. On the contrary, you can also customize the API documentation template for yourself.
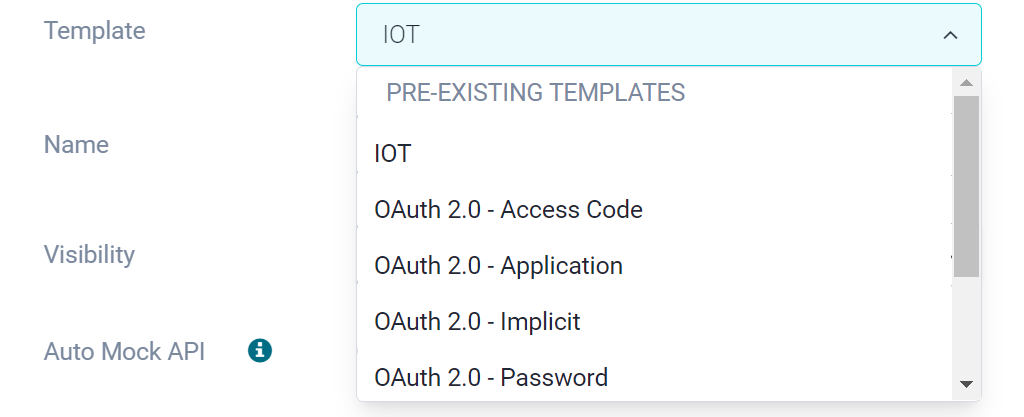
Step 4. Generate Your New API Documentation in one click. You can get the documentation below. If you also want to export the Swagger documentation, click here to learn more.
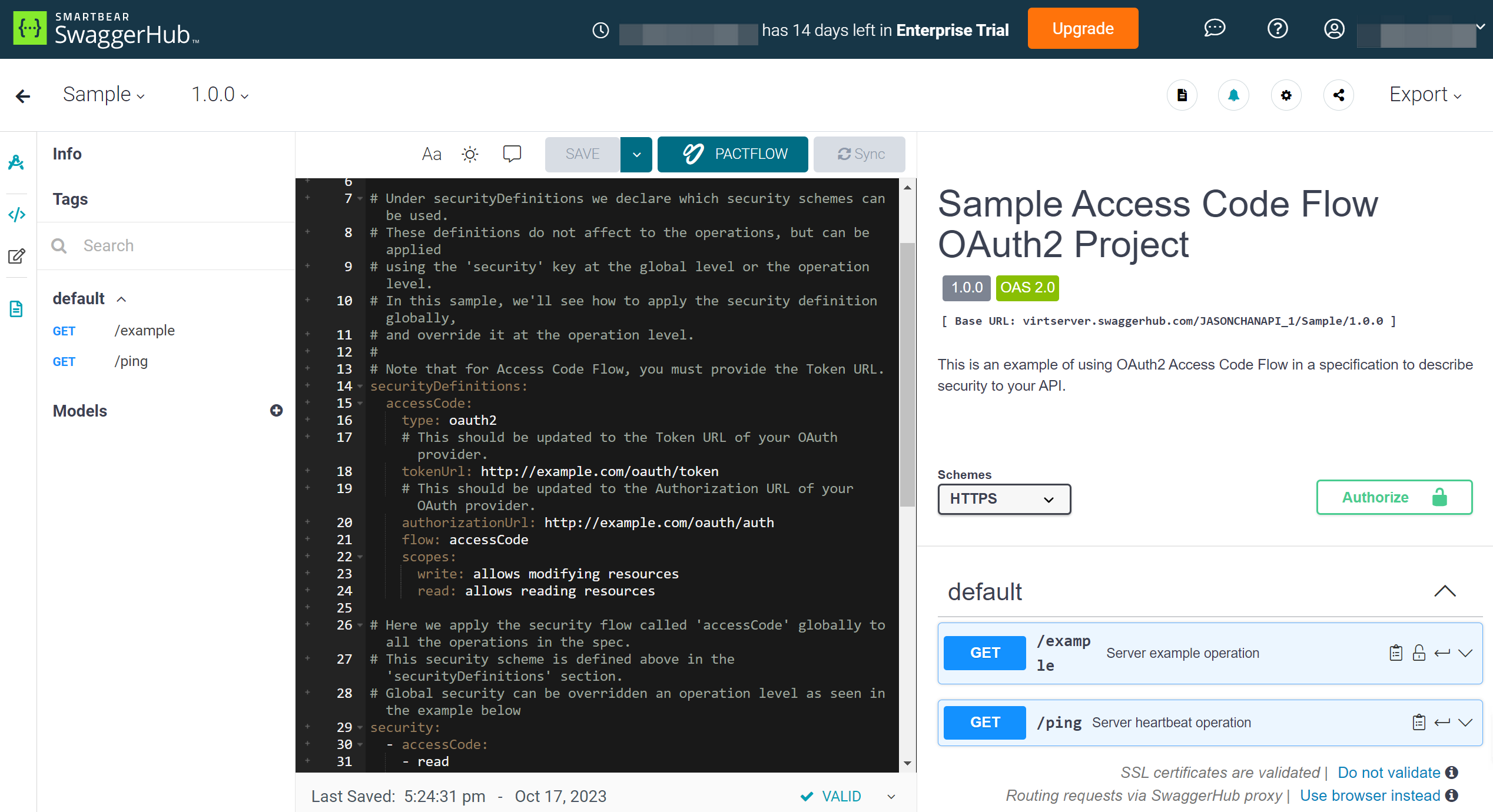
Apidog: Swagger Alternative for Writing API Documentation
While Swagger is a widely used tool for creating API documentation, it's important to be aware of alternative solutions that can make the process even more efficient and user-friendly. One such alternative is Apidog. Apidog is a comprehensive API documentation tool that not only helps you create Swagger documentation but also makes the process more efficient and user-friendly.
Key Features of Apidog
It offers a range of features that can simplify the documentation process and enhance the overall quality of your API documentation:
- Auto-generate Documentation: Apidog can automatically generate documentation for your API based on code comments, annotations, or existing Swagger specifications.
- Interactive Documentation: With Apidog, you can create interactive documentation that allows users to try out API requests directly within the documentation interface.
- Version Control Integration: Apidog integrates with popular version control systems, making it easy to keep your documentation up-to-date as your API evolves.
- Collaboration and Review: Apidog provides collaboration features, allowing multiple team members to work on the documentation simultaneously and review changes before publication.
- Real-time Synchronization: Apidog can sync with your codebase in real-time, ensuring that your API documentation is always accurate and up-to-date.
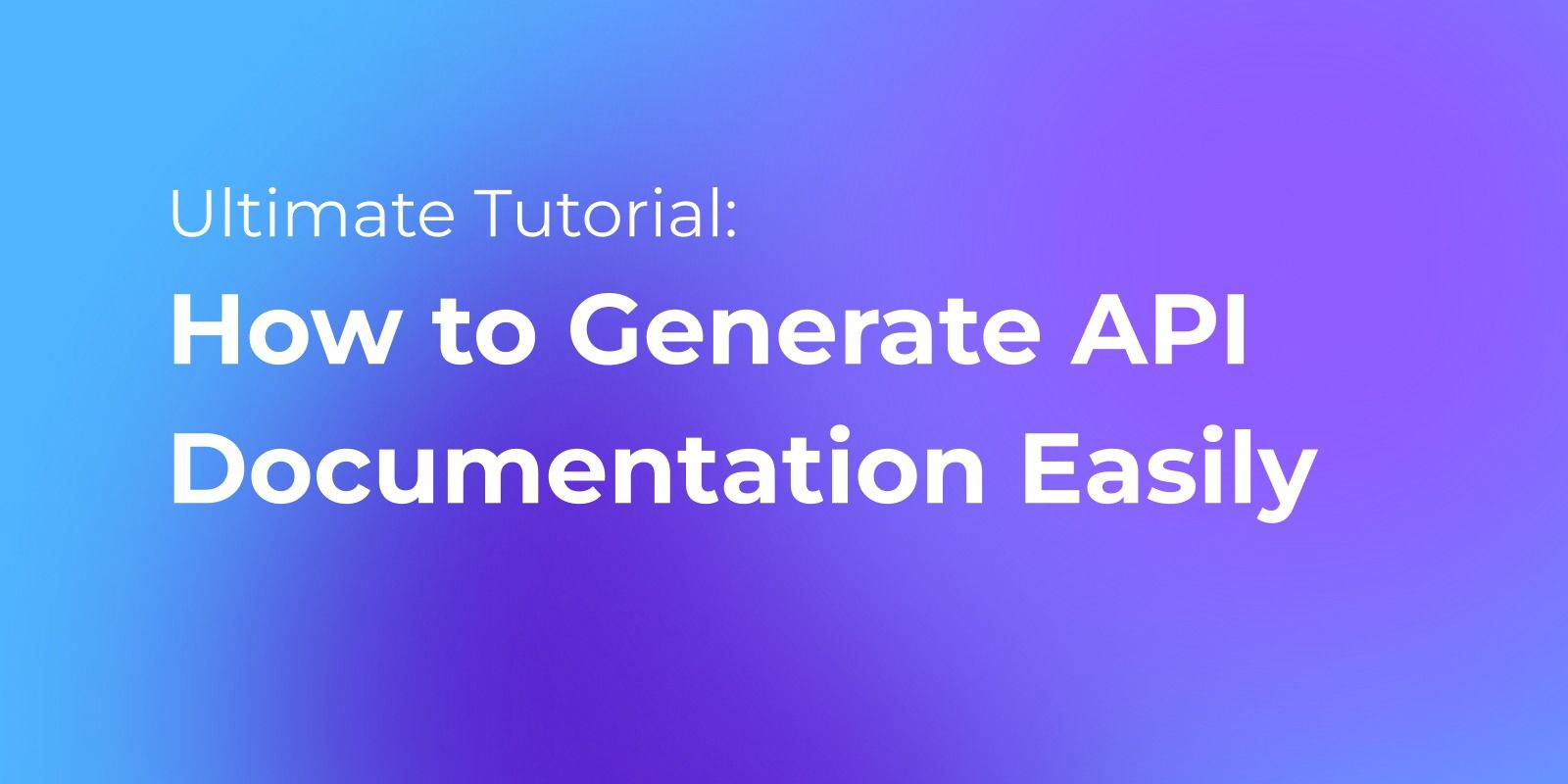
Conclusion
In conclusion, writing Swagger documentation is a crucial step in ensuring that your API is accessible and user-friendly. A well-documented API saves developers time and effort, resulting in a smoother integration process. While Swagger is an excellent choice for API documentation, tools like Apidog can greatly simplify the process and offer numerous additional benefits.