How to make REST API calls with Axios
REST APIs are a way for web services to communicate with each other. They use HTTP requests to GET, POST, PUT, and DELETE data. REST APIs are important because they allow developers to access data from a variety of sources, including social media platforms, e-commerce sites, and more.
In this blog post, I will show you how to use Axios to make REST API calls. I will also introduce you to Apidog, a tool that helps you test and debug your REST API calls.
So, are you ready to learn how to make awesome REST API calls with Axios and Apidog? Let's get started!
Why REST APIs are the Future of Web Services
REST APIs are a powerful way to interact with web services and data. They allow developers to access and manipulate data from a variety of sources, including social media platforms, e-commerce sites, and more. REST APIs are important because they provide a standardized way for web services to communicate with each other.
What is Axios?
Axios is a JavaScript library that allows you to make HTTP requests from both the browser and Node.js. It is promise-based, which means that you can use async/await or promises to handle the results of your requests.
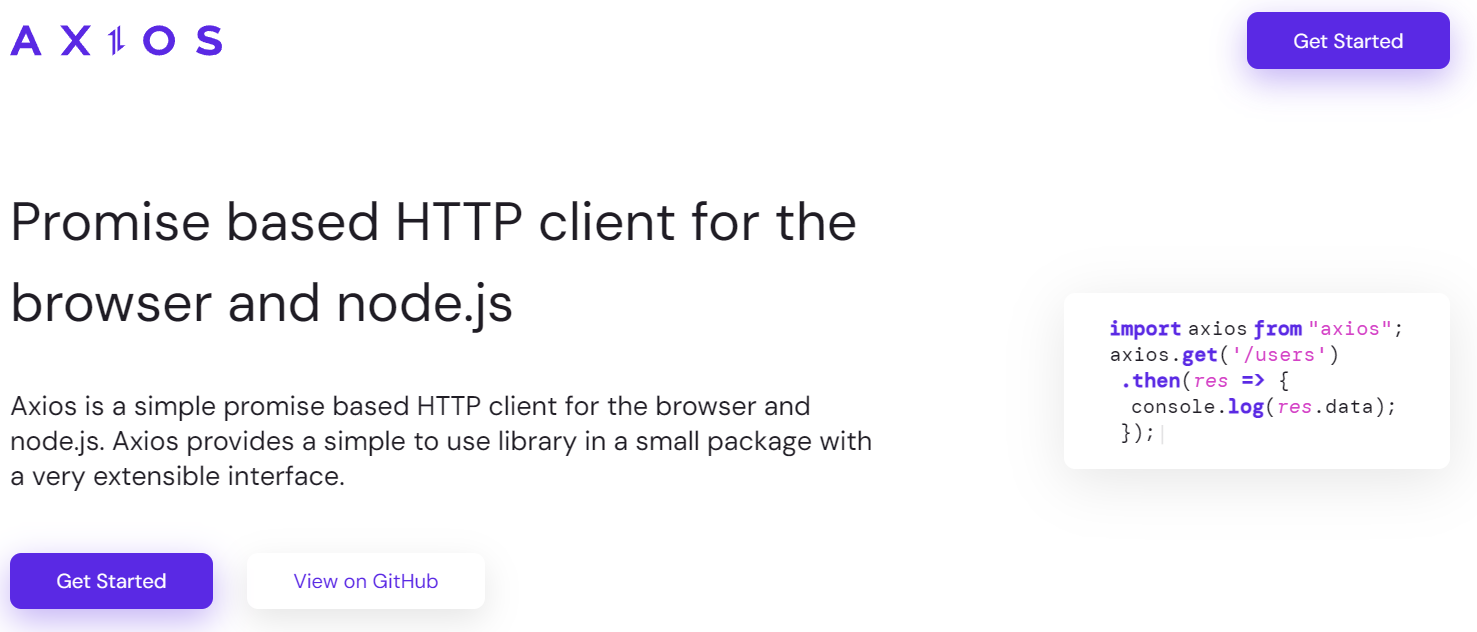
Axios has many advantages over the native Fetch API or jQuery's $.ajax() function. Some of the benefits of using Axios are:
- It supports older browsers, unlike Fetch, which requires a polyfill
- It automatically transforms JSON data, unlike Fetch, which requires you to call .json() on the response
- It allows you to set a timeout for your requests, unlike Fetch, which does not have a timeout option
- It protects you from cross-site request forgery (XSRF) attacks, unlike jQuery, which does not have built-in XSRF protection
- It allows you to monitor the progress of your requests, unlike jQuery, which does not have a progress option
- It allows you to cancel your requests, unlike jQuery, which does not have a cancel option
- It has a more concise and readable syntax, unlike jQuery, which can be verbose and complex
As you can see, Axios has many features that make it a great choice for making HTTP requests in JavaScript. But how do you use Axios? Let's find out!
How to Install Axios
To use Axios, you need to install it first. There are several ways to install Axios, depending on your environment and preference. You can install Axios using:
- npm:
npm install axios
- Yarn:
yarn add axios
- pnpm:
pnpm add axios
- Bower:
bower install axios
- CDN:
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
Once you have installed Axios, you can import it in your JavaScript file and start using it. For example, if you are using Node.js, you can import Axios like this:
const axios = require('axios');
If you are using a module bundler like Webpack or Rollup, you can import Axios like this:
import axios from 'axios';
If you are using a CDN, you can access Axios from the global variable axios
.
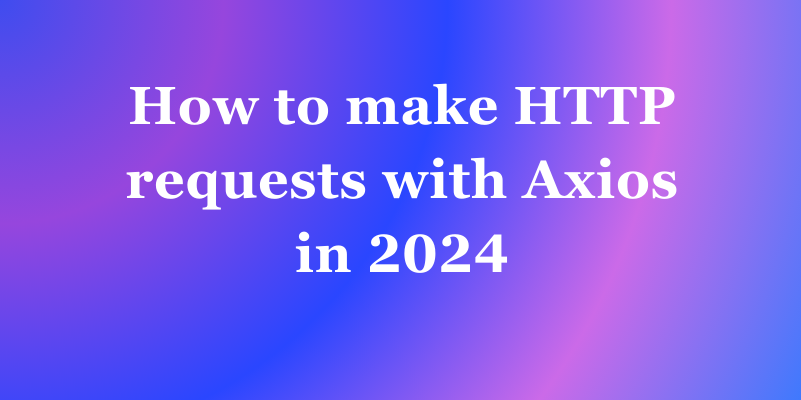
REST APIs are a powerful way to interact with web services and data. They allow developers to access and manipulate data from a variety of sources, including social media platforms, e-commerce sites, and more. Axios is a popular JavaScript library that makes it easy to work with REST APIs. In this blog post, we’ll explore how to use Axios to make REST API calls.
Making Your First REST API Call with Axios
In this section, we’ll show you how to make your first REST API call with Axios. We’ll cover how to install Axios, how to make a simple GET request, and how to handle errors.
To get started with Axios, you’ll need to install it using npm or yarn. Once you’ve installed Axios, you can make a simple GET request like this:
axios.get('https://api.example.com/data')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error(error);
});
This code makes a GET request to https://api.example.com/data and logs the response data to the console. If there’s an error, it logs the error to the console instead.
Sending Data to the Server with Axios
In this section, we’ll show you how to send data to the server with Axios. We’ll cover how to make a POST request, how to send data in the request body, and how to set headers.
To make a POST request with Axios, you can use the axios.post()
method. Here’s an example:
axios.post('https://api.example.com/data', {
name: 'John Doe',
email: 'john.doe@example.com'
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error(error);
});
This code sends a POST request to https://api.example.com/data with the data { name: 'John Doe', email: 'john.doe@example.com' }
in the request body. If there’s an error, it logs the error to the console instead.
Modifying Requests and Responses with Axios Interceptors
In this section, we’ll show you how to use Axios interceptors to modify requests and responses. We’ll cover how to add interceptors to your Axios instance, how to modify requests and responses, and how to handle errors.
Axios interceptors allow you to intercept requests or responses before they are handled by the then()
or catch()
methods. This can be useful for adding authentication headers, modifying requests or responses, or handling errors.
Here’s an example of how to add an interceptor to your Axios instance:
const axiosInstance = axios.create();
axiosInstance.interceptors.request.use(config => {
// Add authentication headers here
return config;
});
axiosInstance.interceptors.response.use(response => {
// Modify response data here
return response;
}, error => {
// Handle errors here
return Promise.reject(error);
});
This code creates a new Axios instance and adds an interceptor to the request and response pipelines. The request interceptor adds authentication headers to the request, and the response interceptor modifies the response data. If there’s an error, it rejects the Promise with the error.
Dealing with Errors in REST API Calls with Axios
Axios provides a simple and intuitive API for handling errors. You can use the catch()
method to handle network errors, HTTP errors, and custom errors.
Here’s an example:
axios.get('https://api.example.com/data')
.then(response => {
console.log(response.data);
})
.catch(error => {
if (error.response) {
// The request was made and the server responded with a status code
// that falls out of the range of 2xx
console.log(error.response.data);
console.log(error.response.status);
console.log(error.response.headers);
} else if (error.request) {
// The request was made but no response was received
console.log(error.request);
} else {
// Something happened in setting up the request that triggered an Error
console.log('Error', error.message);
}
console.log(error.config);
});
This code makes a GET request to https://api.example.com/data and logs the response data to the console. If there’s an error, it logs the error to the console instead. The catch()
method handles the error and logs the appropriate message based on the type of error.
Best practices for optimizing REST API calls with Axios
Axios is a popular JavaScript library that makes it easy to work with REST APIs. It provides a simple and intuitive API for making HTTP requests, and it supports features like request and response interceptors, automatic transforms, and more. Here are some tips for optimizing your REST API calls with Axios:
- Use Interceptors Judiciously: While Axios interceptors are powerful, use them sparingly to avoid making code overly complex. Interceptors can be used to add authentication headers, modify requests or responses, or handle errors. However, using too many interceptors can make your code difficult to read and maintain.
- Global Error Handling: Implement a global error-handling mechanism for Axios requests. This can help you catch errors early and provide a consistent error-handling experience for your users. You can use the
axios.interceptors.response
property to add a global error handler. - Modular Configurations: If your application interacts with multiple APIs with different configurations, create separate Axios instances with their own settings to keep your code organized. This can help you avoid conflicts between different API configurations and make your code easier to read and maintain.
- Use Cancel Tokens: Cancel tokens can be used to cancel a request before it completes. This can be useful if the user navigates away from a page or if the request is taking too long to complete. You can use the
axios.CancelToken
property to create a cancel token. - Use Timeouts: Timeouts can be used to limit the amount of time a request can take. This can help you avoid long wait times and improve the user experience. You can use the
timeout
property to set a timeout for your requests. - Optimize Your Data: When sending data in your requests, optimize it to reduce the amount of data being sent. This can help you reduce the load on your server and improve the performance of your application. You can use tools like Apidog to generate optimized data structures for your requests.
- Use SEO-Friendly URLs: When designing your REST API, use SEO-friendly URLs to make it easier for search engines to crawl and index your content. This can help you improve your search engine rankings and drive more traffic to your site.
- Use Caching: Caching can be used to store frequently accessed data in memory or on disk. This can help you reduce the load on your server and improve the performance of your application. You can use tools like Redis or Memcached to implement caching in your application.
- Use Compression: Compression can be used to reduce the size of your requests and responses. This can help you reduce the load on your server and improve the performance of your application. You can use tools like Gzip or Brotli to implement compression in your application.
- Use Load Balancing: Load balancing can be used to distribute the load across multiple servers. This can help you improve the performance and reliability of your application. You can use tools like NGINX or HAProxy to implement load balancing in your application.
By following these best practices, you can optimize your REST API calls with Axios and improve the performance and reliability of your application. Remember to always test your code thoroughly and monitor your application’s performance to ensure that it’s running smoothly.
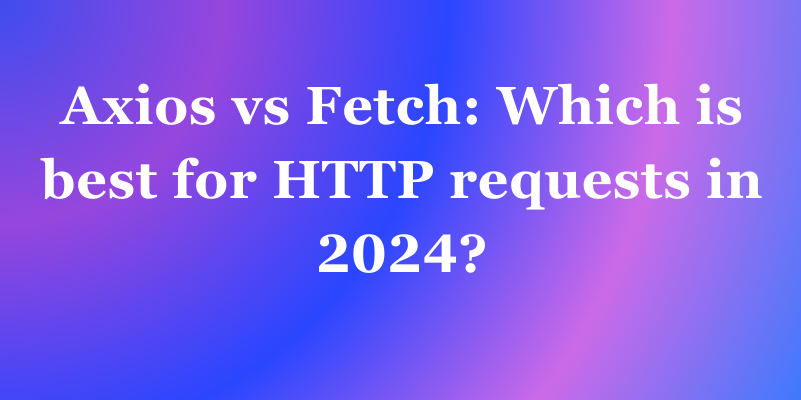
Use Apidog to test and debug your REST API calls
Apidog is an all-in-one collaborative API development platform that provides a variety of tools for API design, documentation, mocking, automated testing, and more. With Apidog, you can streamline your REST API development workflow and ensure that your APIs are reliable and performant. To get started with Apidog, you can sign up for free and download the app for Mac or Linux.
To make an API call with Apidog, create a new request and enter the complete request address and request parameters.
Step 1: Open Apidog and create a new request.
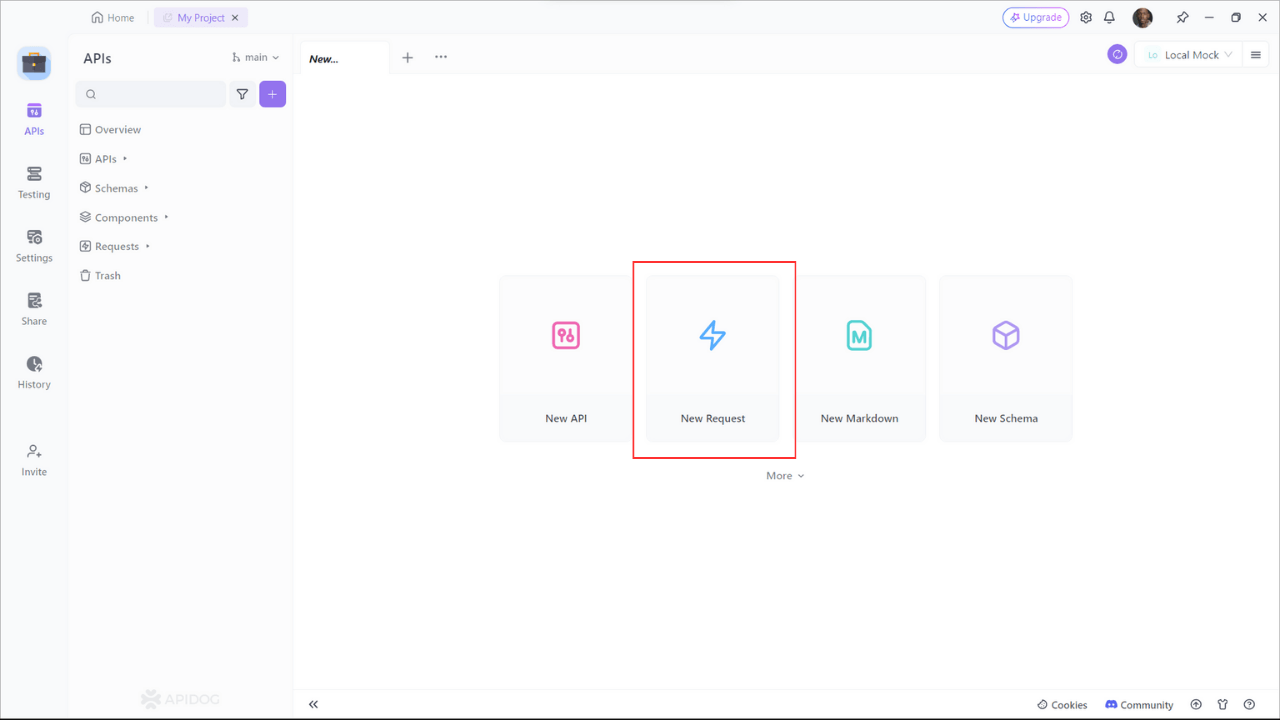
Step 2: Find or manually input the API details for the POST request you want to make.
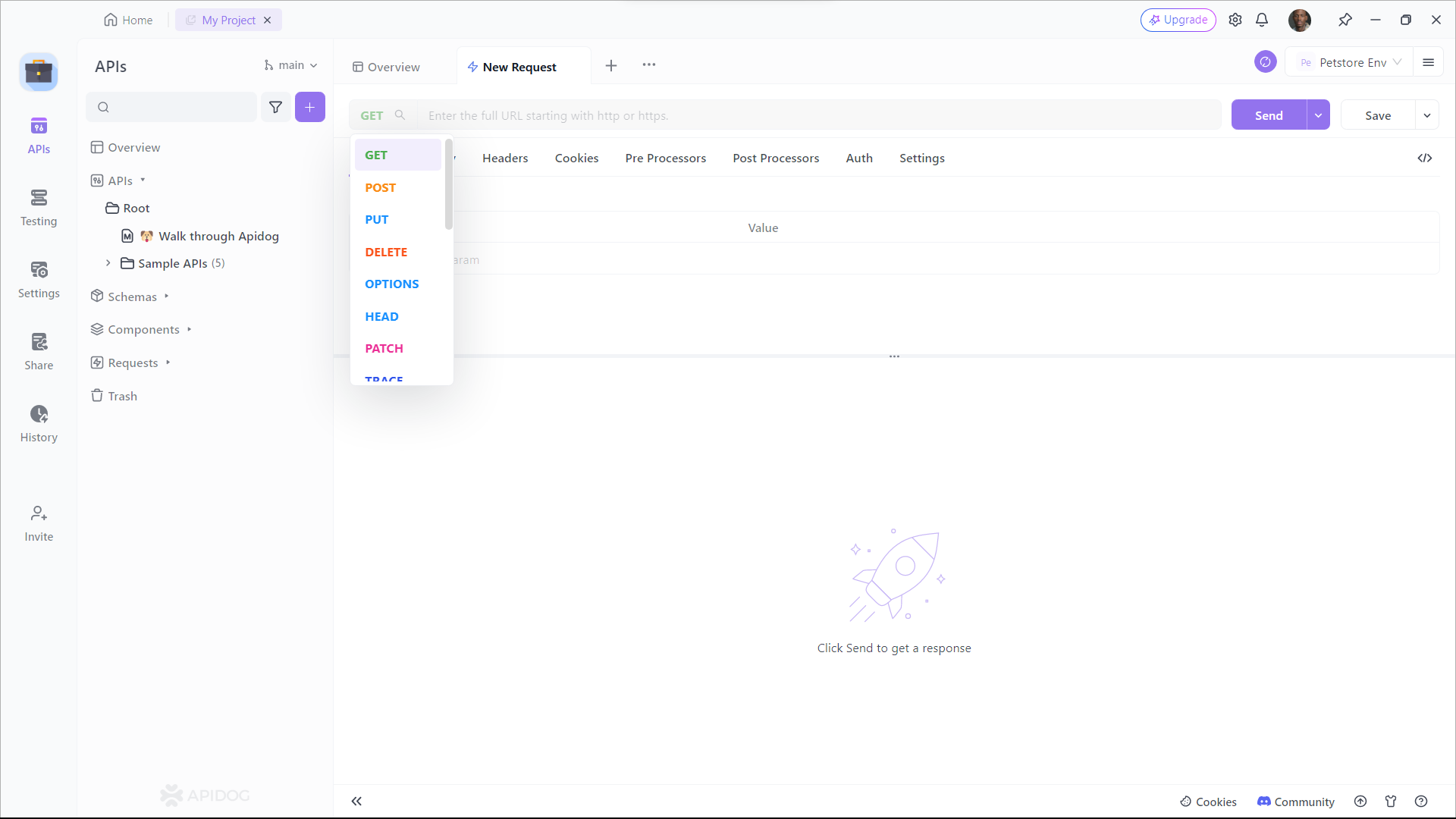
Step 3: Fill in the required parameters and any data you want to include in the request body.
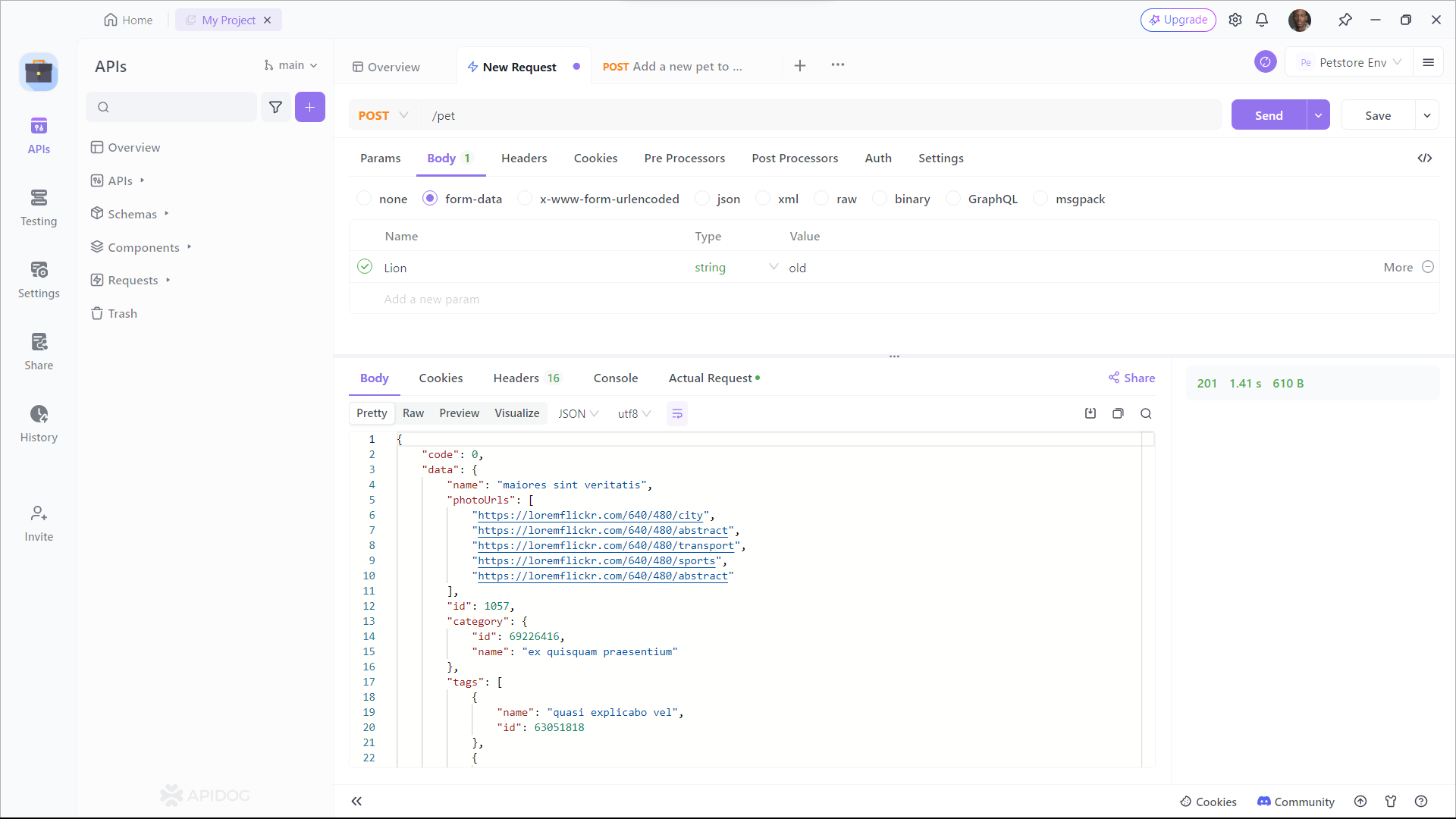
By using Apidog to test and debug your REST API calls, you can streamline your development workflow and ensure that your APIs are reliable and performant. Remember to always test your code thoroughly and monitor your application’s performance to ensure that it’s running smoothly.
Conclusion
In this post, we learned how to make REST API calls with Axios, a popular JavaScript library for fetching data from servers.
Axios is a powerful and easy-to-use tool for building modern web applications that rely on data from various sources, it's a great choice for making REST API calls because it’s easy to use and provides a lot of flexibility. By following the examples and tips in this post, you can start using Axios in your own projects and enjoy its benefits. Happy coding!