If you've ever worked with APIs, you've probably come across JSON data. It’s everywhere, from web development to app integration. Python, being the versatile language it is, has powerful built-in libraries to handle JSON effortlessly. Whether you’re an API enthusiast, a web developer, or just curious about JSON, this guide is here to help you become proficient in handling JSON in Python.
What Is JSON?
Before we talk code, let’s cover the basics. JSON stands for JavaScript Object Notation. It’s a lightweight data format used primarily for transmitting data between a server and web applications. Despite its name, JSON isn’t limited to JavaScript—it’s widely used in many programming languages, including Python.
A simple JSON object looks like this:
{
"name": "Ashley",
"age": 99,
"languages": ["Python", "JavaScript", "C++"]
}
This data format is easy to read for humans and machines alike, which makes it so popular in API responses.
Why Should You Care About JSON?
In the world of APIs, JSON has become the go-to format for data exchange. When you're working with APIs in Python (or anywhere), you’ll frequently send or receive data in JSON format. Understanding how to work with JSON effectively will allow you to handle API responses, manipulate data, and even send structured data to APIs.
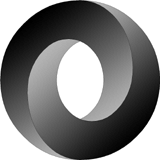
The JSON Library in Python
Python makes working with JSON incredibly easy, thanks to its built-in json
library. With just a few lines of code, you can convert JSON data into Python objects and vice versa. Let’s break this down step by step.
Importing the JSON Module
The first step is to import the json
module:
import json
Simple, right? Now let’s look at how we can use this module to work with JSON data.
Apidog: Simplifying JSON Integration in API Documentation
Apidog is a powerful tool designed to simplify the process of creating, sharing, and maintaining API documentation. It excels in handling JSON-based APIs and offers several key features that enhance the documentation process:
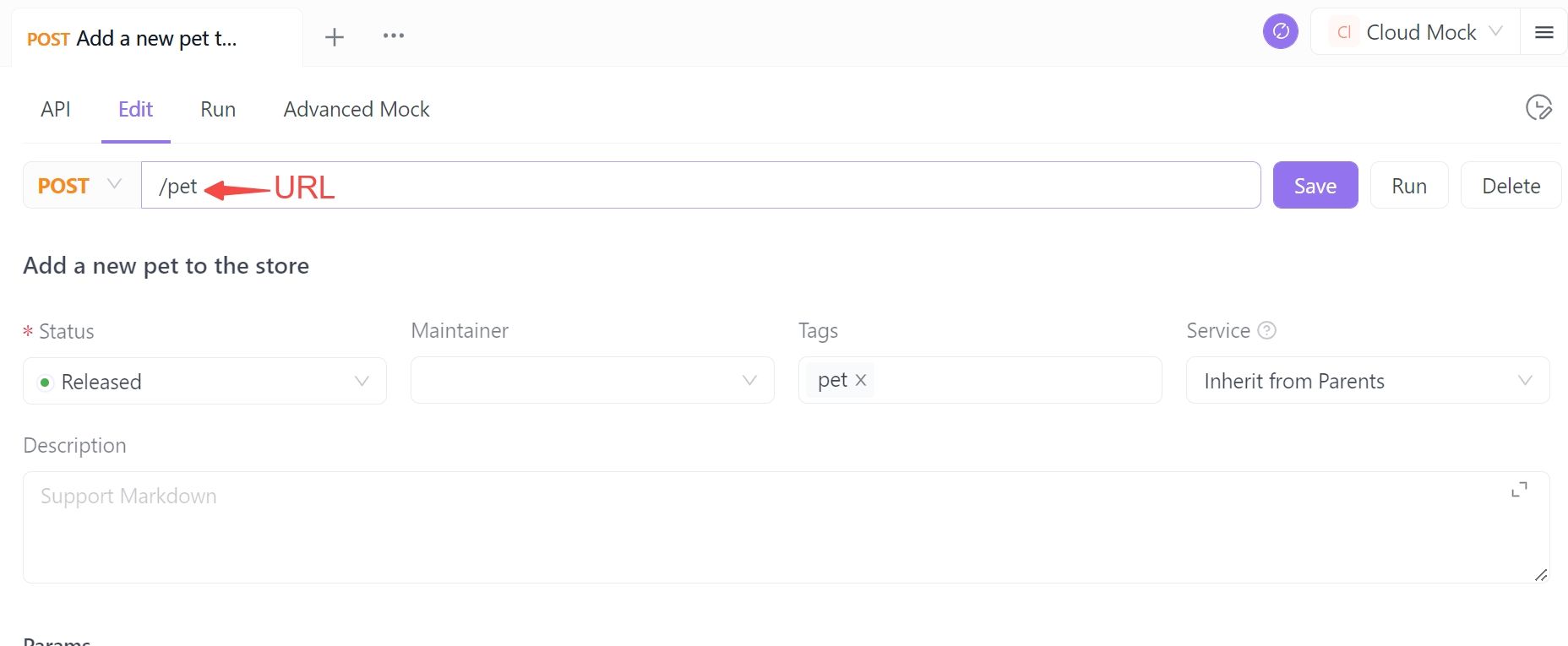
- Interactive Examples: Apidog allows developers to include interactive examples of JSON payloads in their documentation. This feature enables API consumers to visualize how requests should be structured and how responses will appear, facilitating a deeper understanding of API endpoints.
- Visual Data Modeling: With Apidog, you can create visual representations of JSON data structures. This feature is invaluable for showcasing complex JSON schemas and data models, making it easier for developers to work with APIs that have intricate data requirements.
- Automated Documentation Generation: Apidog automates the generation of API documentation from JSON schema files, reducing manual documentation efforts. By defining JSON schemas, you can effortlessly produce consistent and up-to-date API documentation.
- Team Collaboration: Collaboration among development teams and API consumers is simplified with Apidog. It offers seamless sharing and version control capabilities, ensuring that everyone is on the same page throughout the API integration process.
Parsing JSON Strings in Python
Parsing means converting a JSON string into a Python object. The most common JSON objects are dictionaries and lists. You can convert JSON into Python objects using the json.loads()
method.
Example 1: Converting a JSON String to a Python Dictionary
Let’s say you receive a JSON string from an API response. Here’s how you can convert it into a Python dictionary:
import json
json_string = '{"name": "Ashley", "age": 29, "languages": ["Python", "JavaScript", "C++"]}'
python_dict = json.loads(json_string)
print(python_dict)
Output:
{'name': 'Ashley', 'age': 99, 'languages': ['Python', 'JavaScript', 'C++']}
Now, python_dict
is a Python dictionary that you can work with like any other dictionary.
Accessing JSON Data in Python
Once your JSON string is converted into a Python dictionary, you can access its elements just like you would with any Python dictionary.
print(python_dict['name']) # Outputs: Ashley
print(python_dict['languages']) # Outputs: ['Python', 'JavaScript', 'C++']
This allows you to extract the data you need, making it easier to handle complex API responses.
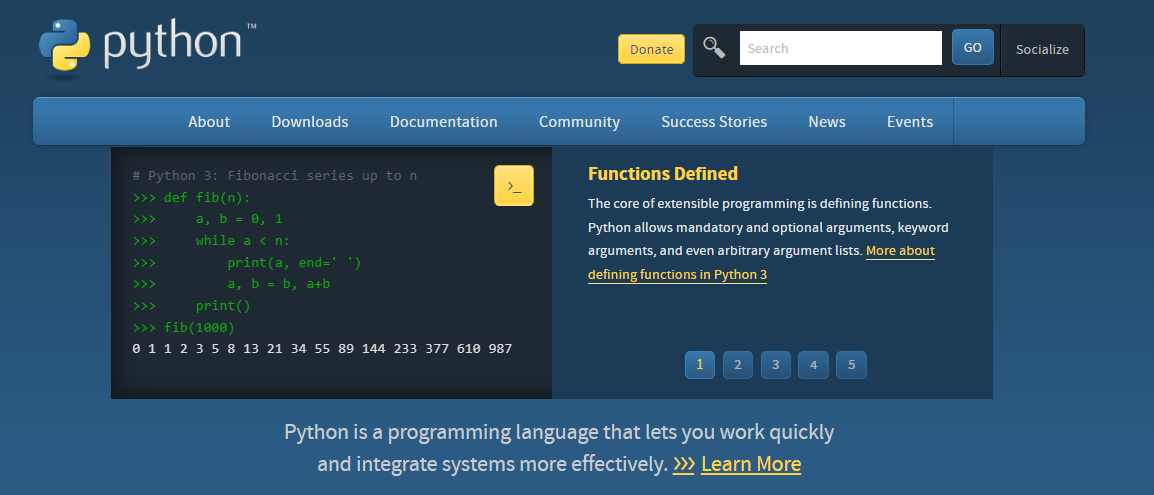
Converting Python Objects to JSON
Just like you can convert JSON strings into Python objects, you can also convert Python objects into JSON strings. This is useful when you’re sending data to an API or saving it to a file.
To convert a Python object into JSON, use the json.dumps()
method.
Example 2: Converting a Python Dictionary to a JSON String
python_data = {
"name": "Ashley",
"age": 99,
"languages": ["Python", "JavaScript", "C++"]
}
json_string = json.dumps(python_data)
print(json_string)
Output:
{"name": "Ashley", "age": 99, "languages": ["Python", "JavaScript", "C++"]}
As you can see, this outputs a valid JSON string that can be transmitted via APIs or stored as a file.
Writing JSON to a File in Python
Sometimes, you’ll want to store JSON data in a file. For example, you may be working with a large API response and need to save it for future use. Python makes it easy to write JSON data to a file.
Example 3: Writing JSON Data to a File
Here’s how you can save a Python dictionary as a JSON file:
with open('data.json', 'w') as json_file:
json.dump(python_data, json_file)
This will create a file named data.json
and write the JSON data into it. Simple, right?
Reading JSON from a File in Python
Of course, if you can write to a file, you should also be able to read from one. You can load JSON data from a file into a Python object using the json.load()
method.
Example 4: Reading JSON Data from a File
with open('data.json', 'r') as json_file:
data = json.load(json_file)
print(data)
This will read the JSON data from data.json
and convert it into a Python dictionary.
Working with JSON and APIs in Python
When dealing with APIs, you’ll often need to send and receive JSON data. This is where Python’s requests
library comes in handy.
Example 5: Sending JSON Data in a POST Request
Let’s say you’re working with an API that requires you to send JSON data. Here’s how you can do it using the requests
library:
import requests
import json
url = 'https://api.example.com/data'
payload = {
"name": "Ashley",
"age": 99
}
response = requests.post(url, json=payload)
print(response.status_code)
In this example, we’re sending a POST request with a JSON payload. The requests
library automatically converts the Python dictionary into JSON for you.
Handling API Responses with JSON
When you receive a response from an API, it’s often in JSON format. You can easily parse the JSON data and convert it into a Python object using the .json()
method from the requests
library.
Example 6: Parsing JSON from an API Response
response = requests.get('https://api.example.com/user/1')
data = response.json()
print(data)
This will print the JSON data received from the API as a Python dictionary. Now, you can work with this data as needed.
Working with Nested JSON Data
Sometimes, you’ll encounter more complex JSON objects that are nested. Python makes it easy to navigate through these structures.
Example 7: Accessing Nested JSON Data
Let’s say you have the following JSON object:
{
"name": "Ashley",
"age": 99,
"address": {
"city": "Nanjing",
"country": "China"
}
}
You can access nested data like this:
print(python_dict['address']['city']) # Outputs: Nanjing
This is particularly useful when working with API responses that include multiple layers of data.
Error Handling with JSON in Python
When working with JSON data, it’s important to handle errors, especially when dealing with API responses. Python provides tools to catch and handle JSON errors.
Example 8: Handling JSON Errors
If you try to load invalid JSON data, Python will raise a json.JSONDecodeError
. You can catch this error and handle it gracefully.
try:
json.loads('invalid json string')
except json.JSONDecodeError as e:
print(f"Error decoding JSON: {e}")
This prevents your program from crashing due to invalid JSON data.
Working with JSON and APIs: The Apidog Advantage
When dealing with JSON and APIs, efficiency is key. Apidog is an all-in-one API tool that simplifies the process of working with APIs. Whether you need to create, test, or debug an API, Apidog allows you to do it seamlessly. And because it supports JSON, managing your API data becomes a breeze.
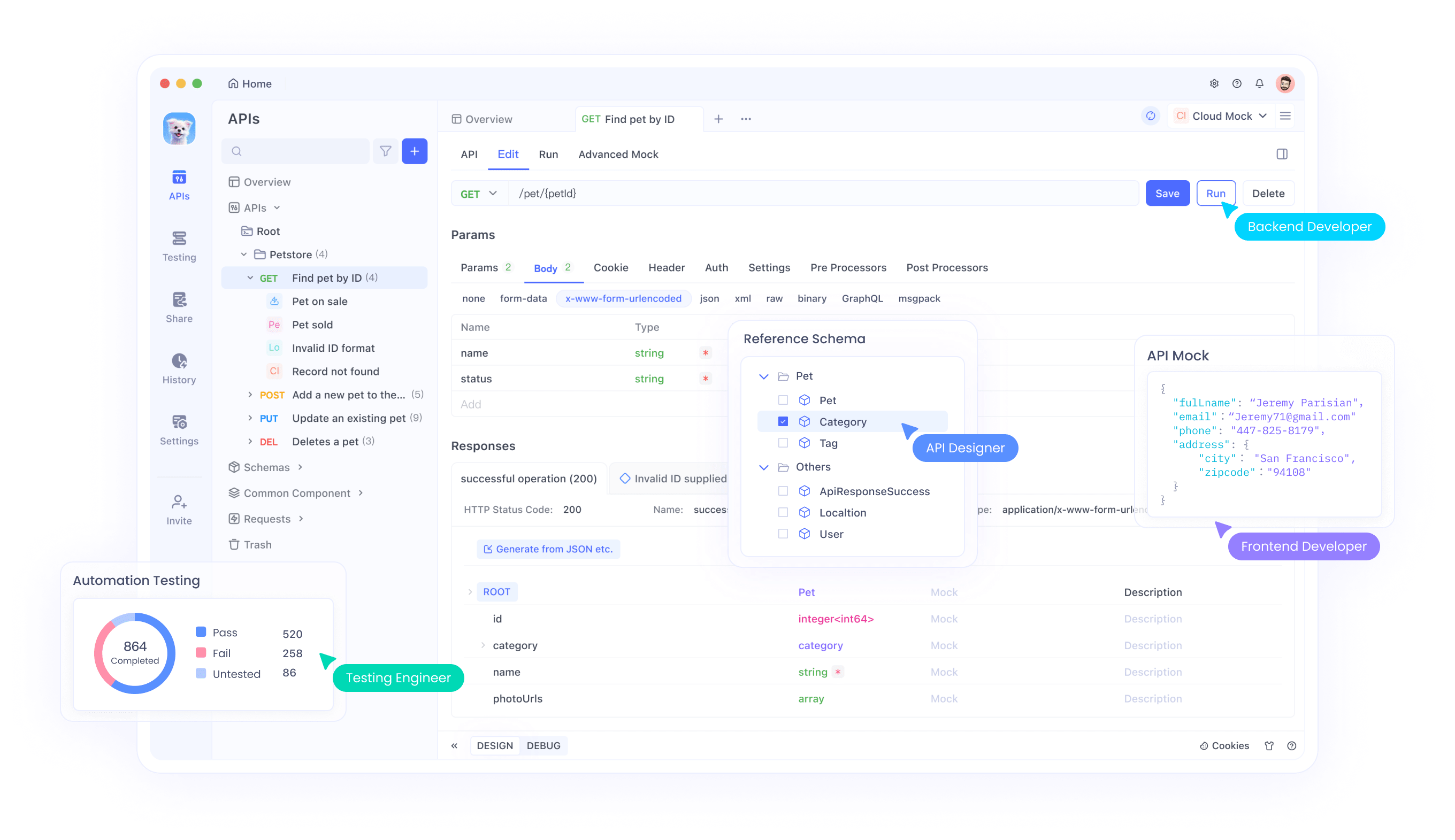
With Apidog, you can:
- Debug APIs: Send requests and view JSON responses effortlessly.
- Automate Testing: Use automated testing to ensure your APIs return valid JSON data.
- Collaborate with Teams: Share API documentation and data with your team easily.
The best part? You can download Apidog for free and start streamlining your API and JSON workflows today!
Test your Python API with Apidog
Testing your Python API with Apidog can streamline the process and ensure that your API functions as expected. Apidog is a tool that can help you design, develop, debug, and test your APIs.
- Open Apidog and create a new request.
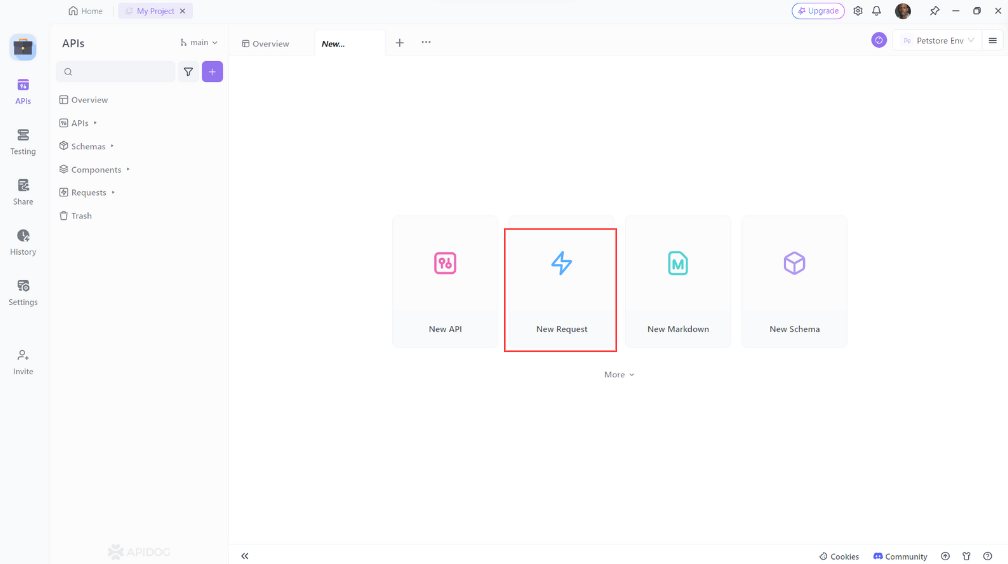
2. Set the request method to GET.
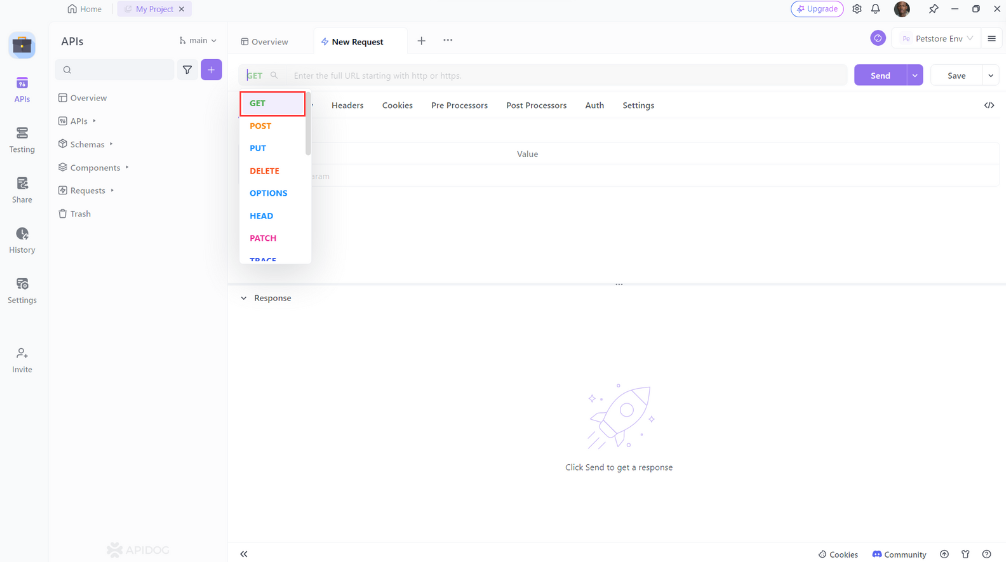
3. Enter the URL of the resource you wish to update. You can also add any additional headers or parameters you want to include, and then click the 'Send' button to send the request
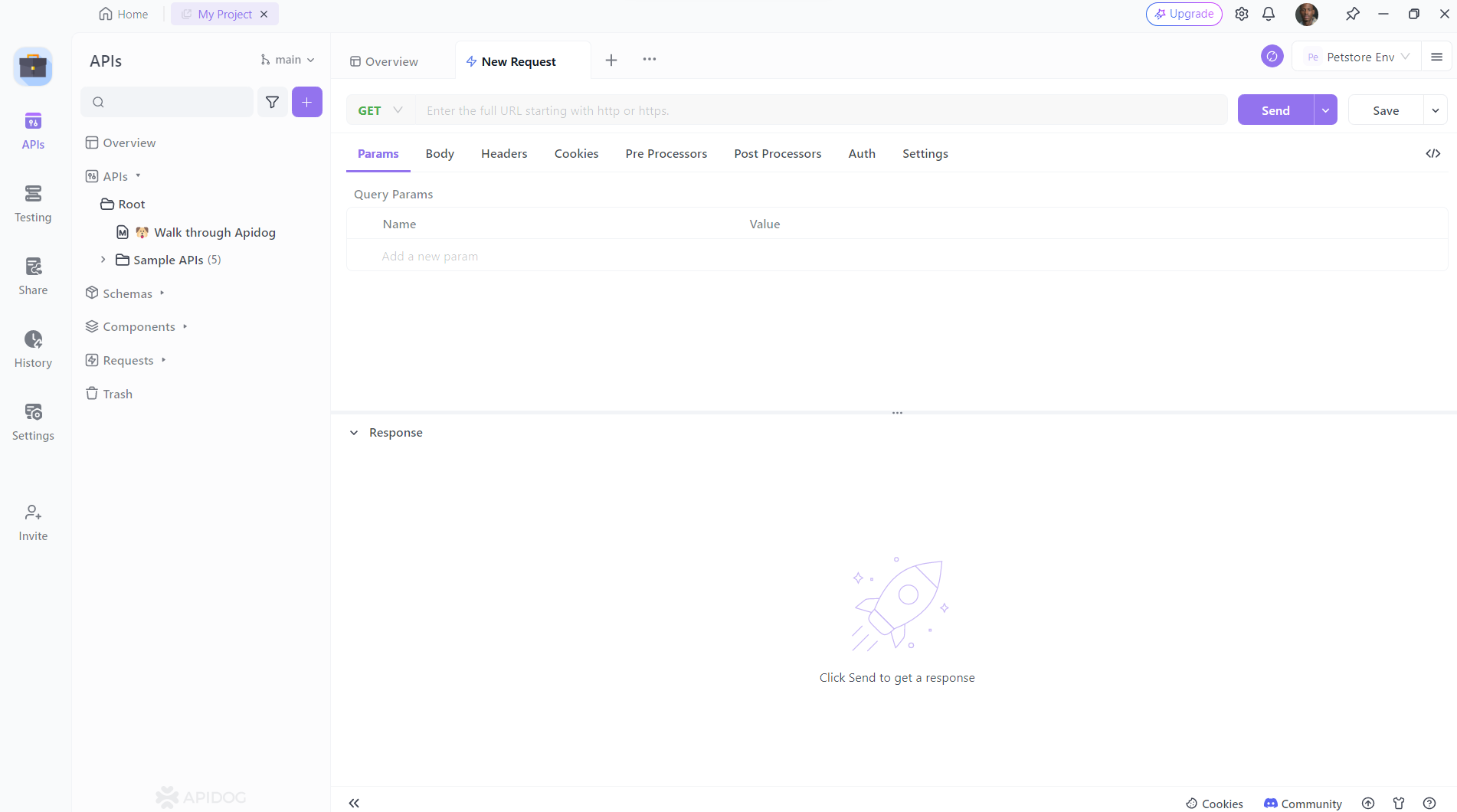
4. Confirm that the response matches your expectations.
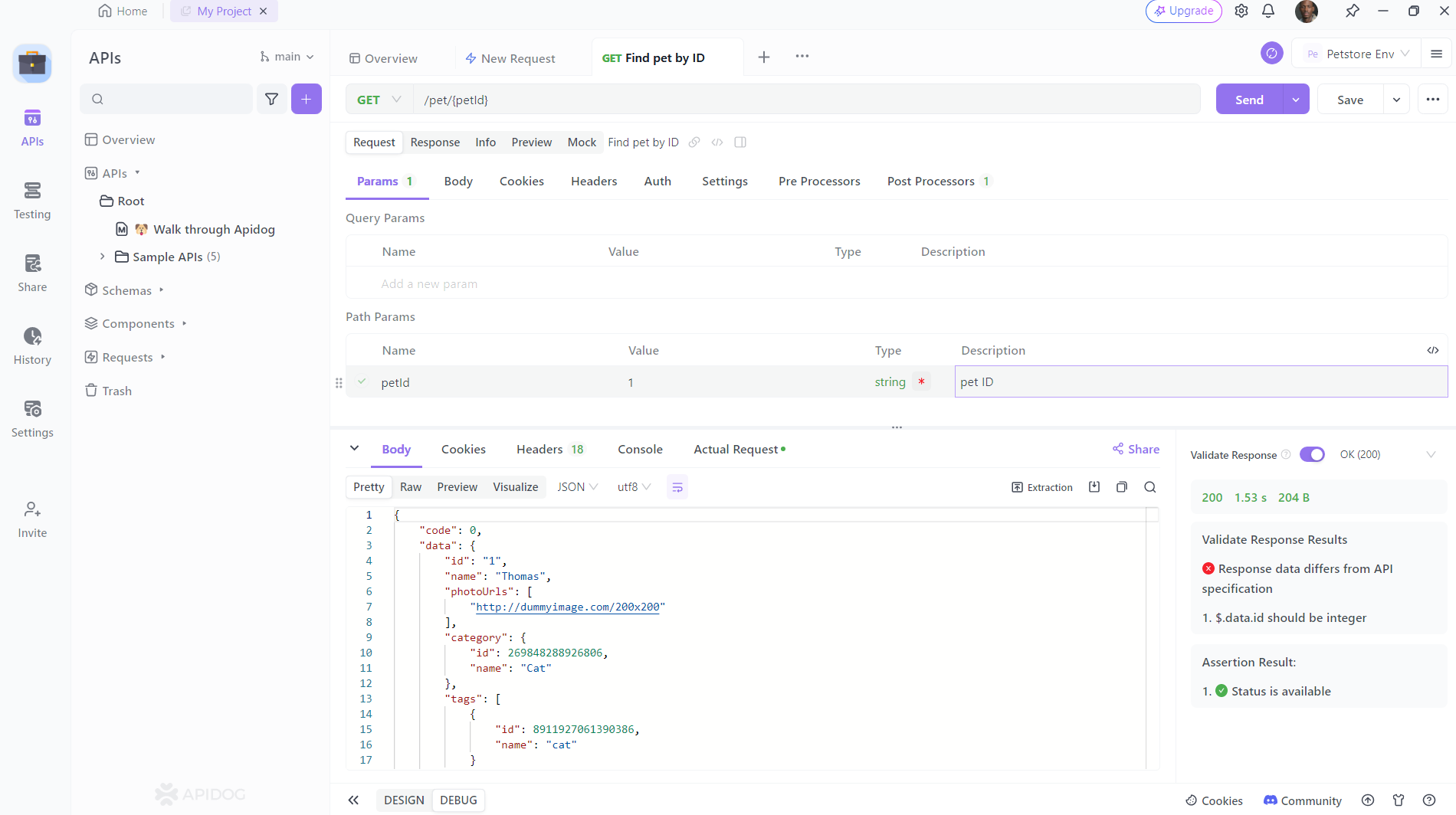
Apidog provides a comprehensive guide that explores leveraging its features to enhance your Python REST API development, making it faster, more efficient, and a pleasure to work with.
Conclusion
Working with JSON in Python is not only easy but essential for anyone dealing with APIs. Python’s json
library provides simple methods to parse, write, and manipulate JSON data. Whether you're a beginner or an experienced developer, mastering JSON in Python will make your API interactions much smoother.
And don’t forget, when you’re working with APIs and JSON, tools like Apidog can make your life a whole lot easier. Head over to Apidog and grab your free download today.