HTTPX [Python] | Fast-performing and Robust Python Library
HTTPX is a Python library that prioritizes speed and efficiency for HTTP requests. It excels in handling high-traffic applications and large data transfers. Unlike others, HTTPX focuses on raw performance, making it ideal for building ultra-responsive web services and APIs.
When creating HTTP requests, there are a lot of potential variables that you can customize. If you are a developer who wishes to have more control over the requests you make, continue reading!
However, with Apidog, a comprehensive API platform, all that necessary studying can be skipped! Apidog's code generation feature allows users to create various programming codes within a few clicks of a button!
If you think Apidog can help you, start using Apidog for free by clicking the button below! 👇 👇 👇
What is HTTPX?
HTTPX is a powerful Python library designed to make HTTP requests with exceptional speed and efficiency. It caters to developers who prioritize performance and specific control over their communication channels.
HTTPX Key Features
HTTPX stands out as a powerful Python library designed for making HTTP requests with an emphasis on speed and efficiency. It becomes a useful asset for various web development tasks due to these key features:
1. Fast Performance:
- Asynchronous: HTTPX thrives on asynchronous operations (compatible with
asyncio
). This allows it to handle numerous HTTP requests concurrently, boosting performance for high-traffic web applications and APIs. - Connection Pooling: It efficiently manages connections to servers, eliminating the need to establish new connections for every request. This reduces overhead and improves response times.
2. Efficient data handling:
- Streaming: For large datasets or real-time data streams, HTTPX offers exceptional streaming capabilities. You can process data in chunks as it arrives, avoiding the need to download the entire response at once. This is particularly beneficial for real-time applications and large file downloads.
- Automatic decompression: HTTPX automatically handles compressed responses (like Gzip), simplifying data handling and reducing processing overhead.
3. Granular control for complex workflows:
Request Customization: HTTPX provides fine-grained control over request parameters. You can define various aspects like:
- Headers for specific information exchange with the server.
- Body content for sending complex data structures.
- Timeouts to prevent applications from hanging on unresponsive servers.
- Authentication methods for secure communication.
- Other advanced options for tailored request behavior.
4. Robust communication features:
- Retries and Timeouts: Configure retries for failed requests to handle temporary network issues gracefully. Set timeouts to avoid applications hanging on unresponsive servers.
- Interceptors and Hooks: Implement custom logic to modify request behavior or intercept responses for advanced control over communication.
5. Integration and ecosystem:
- Seamless Integration: HTTPX integrates well with other popular Python web development libraries. This makes it a versatile tool for building web applications with diverse functionalities.
HTTPX Real-world Use Case Scenarios
1. Building High-Traffic APIs:
- Scaling for Success: When your API needs to handle a massive number of concurrent requests efficiently, HTTPX's asynchronous nature and connection pooling come to the rescue. It ensures your API remains responsive and delivers fast results even under heavy load.
- Microservices Architecture: In microservices architectures where APIs communicate with each other frequently, HTTPX's speed becomes crucial. It minimizes communication overhead, leading to a more responsive and performant overall system.
2. Real-Time Applications:
- Minimizing Latency: For real-time applications like chat applications, stock tickers, or live sports updates, minimizing latency (delay) is critical. HTTPX's asynchronous nature and efficient data handling (including streaming) ensure real-time data flows smoothly with minimal delay.
- WebSocket Support (with Integration): While HTTPX doesn't handle WebSockets directly, it integrates well with libraries like WebSockets that enable real-time, two-way communication between clients and servers. This combined approach provides a powerful solution for building real-time applications in Python.
3. Data-Intensive Tasks:
- Large File Downloads: When downloading large files (e.g., media files, backups), HTTPX's streaming capabilities prove invaluable. You can process data in chunks as it arrives, reducing memory usage and improving download performance compared to libraries that download the entire file at once.
- Real-Time Data Feeds: Processing real-time data feeds from sensors, social media platforms, or financial markets often involves large data volumes. HTTPX's streaming support allows efficient processing of incoming data streams, enabling real-time analytics and insights.
4. Performance-Critical Applications:
- Performance Optimization: For applications where speed is a top priority, HTTPX delivers exceptional performance. Its asynchronous nature, connection pooling, and efficient data handling make it ideal for tasks like web scraping, API testing, or performance monitoring tools.
- Load Testing and Performance Benchmarking: HTTPX can be a valuable tool in load testing scenarios where you need to simulate high traffic volumes to assess an application's performance. Its efficiency allows you to send numerous requests quickly and analyze the results for performance bottlenecks.
HTTPX Code Examples
1. Basic GET request with response handling:
import httpx
async def fetch_data(url):
async with httpx.AsyncClient() as client:
response = await client.get(url)
if response.status_code == 200:
data = await response.text() # Read entire response as text
print(f"Data from {url}: {data[:100]}...") # Truncate for brevity
else:
print(f"Error: {response.status_code}")
asyncio.run(fetch_data("https://www.example.com"))
This code example defines an asynchronous function fetch_data
that takes a URL. It then uses httpx.AsyncClient
to make a GET request. The response is later processed based on the status code, where if successful, a data snippet is printed.
2. Handling large data transfers with streaming:
import httpx
async def download_file(url, filename):
async with httpx.AsyncClient() as client:
response = await client.get(url, stream=True)
if response.status_code == 200:
async with open(filename, 'wb') as f:
async for chunk in response.aiter_content(chunk_size=1024):
await f.write(chunk)
print(f"File downloaded: {filename}")
asyncio.run(download_file("https://large-file.com/data.zip", "data.zip"))
This code example demonstrates downloading a large file by streaming. The stream=True
argument allows processing 1024-byte data chunks, and the aiter_content
method allows iterating over the response content in manageable chunks that arelater written to the file.
3. Customizing requests with headers, timeouts, and authentication:
import httpx
async def make_authenticated_request(url, token):
headers = {'Authorization': f'Bearer {token}'}
timeout = httpx.Timeout(timeout=5, connect=2) # Set timeouts for request and connection
async with httpx.AsyncClient() as client:
try:
response = await client.get(url, headers=headers, timeout=timeout)
response.raise_for_status() # Raise exception for non-200 status codes
# Process successful response
except httpx.HTTPStatusError as e:
print(f"Error: {e}")
asyncio.run(make_authenticated_request("https://api.example.com/data", "your_access_token"))
The code example above demonstrates setting custom headers (for authorization) and timeouts for a request. It also utilizes raise_for_status
to raise an exception for non-successful response codes.
4. Advanced features: retries and interceptors:
from httpx import retries
async def fetch_data_with_retries(url):
async with httpx.AsyncClient(retries=retries.Retry(total=3, backoff_factor=1)) as client:
response = await client.get(url)
# Process response
async def logging_interceptor(request, response):
print(f"Request: {request.method} {request.url}")
print(f"Response: {response.status_code}")
async def main():
async with httpx.AsyncClient(interceptors=[logging_interceptor]) as client:
await fetch_data_with_retries("https://unreliable-api.com/data")
asyncio.run(main())
This code example demonstrates two advanced features:
- Retries: The
retries
argument configures automatic retries for failed requests with up to 3 attempts and a back-off strategy. - Interceptors: A custom
logging_interceptro
function is added using theinterceptors
argument, which logs information about the request and response during the communication process.
Apidog - Optimal API Development Tool for HTTPX App/API Development
Python's HTTPX library can be difficult to understand at first. Written in Python programming language, it may get confusing for first-timers despite the intuitive nature. For newer developers, consider using Apidog.
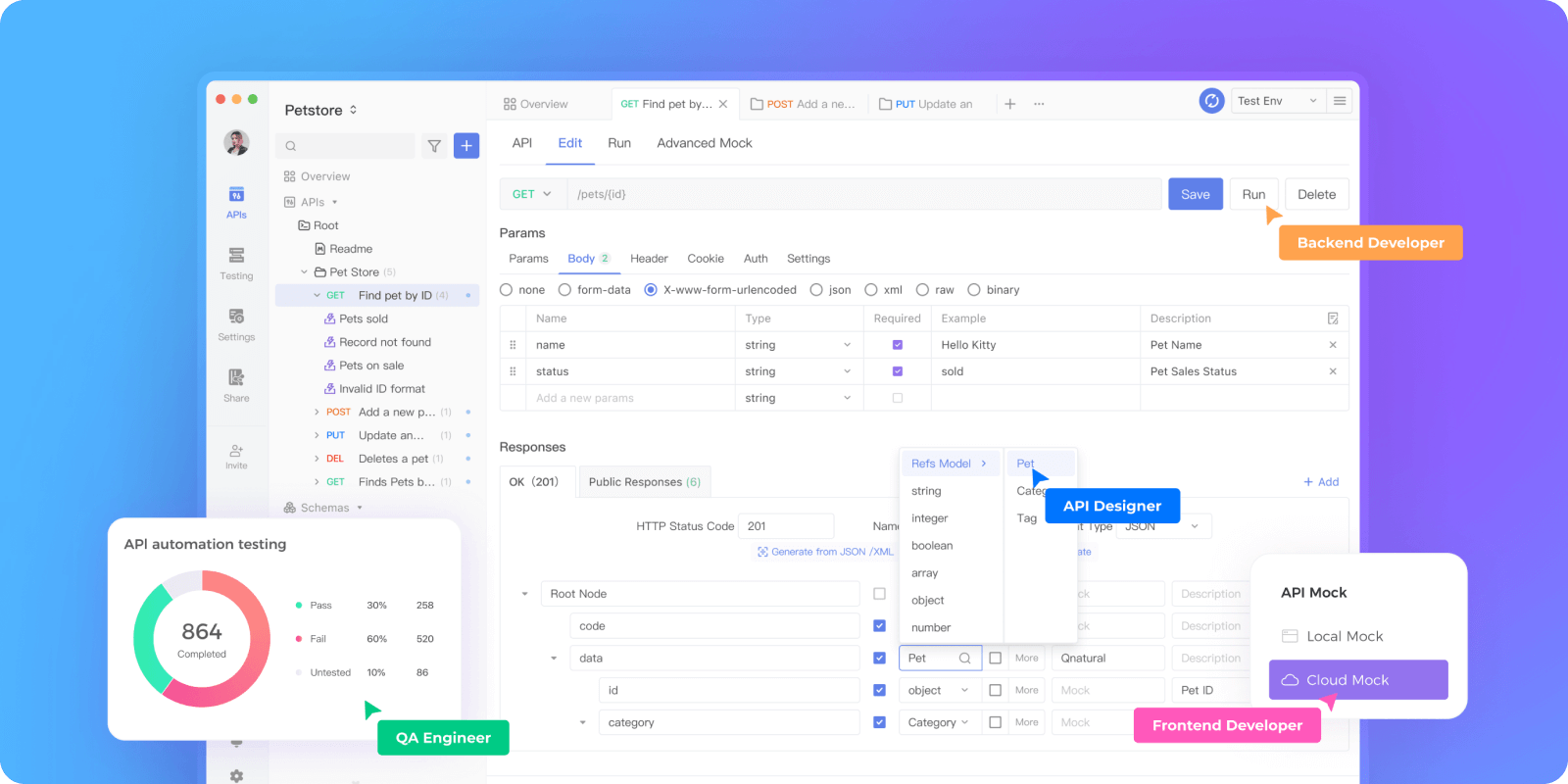
Generating Python Client Code Using Apidog
Apidog can fast-track anyone's API or app development processes with the help of its handy code generation feature. Within a few clicks of a button, you can have ready-to-use code for your applications - all you need to do is copy and paste it over to your working IDE!
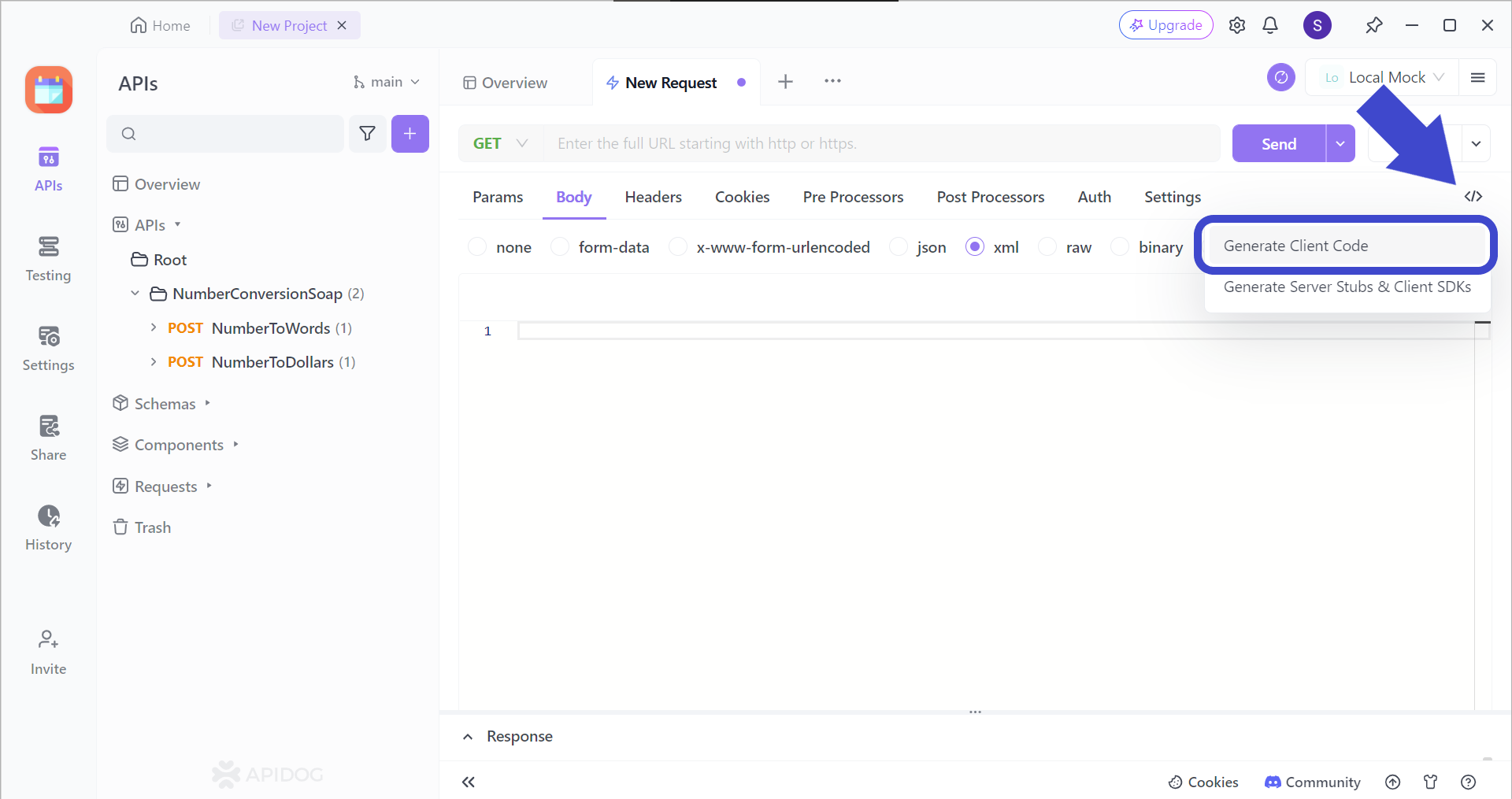
Firstly, locate this </>
button found on the top right corner of the screen when you are trying to create a new request. Then, select Generate Client Code
.
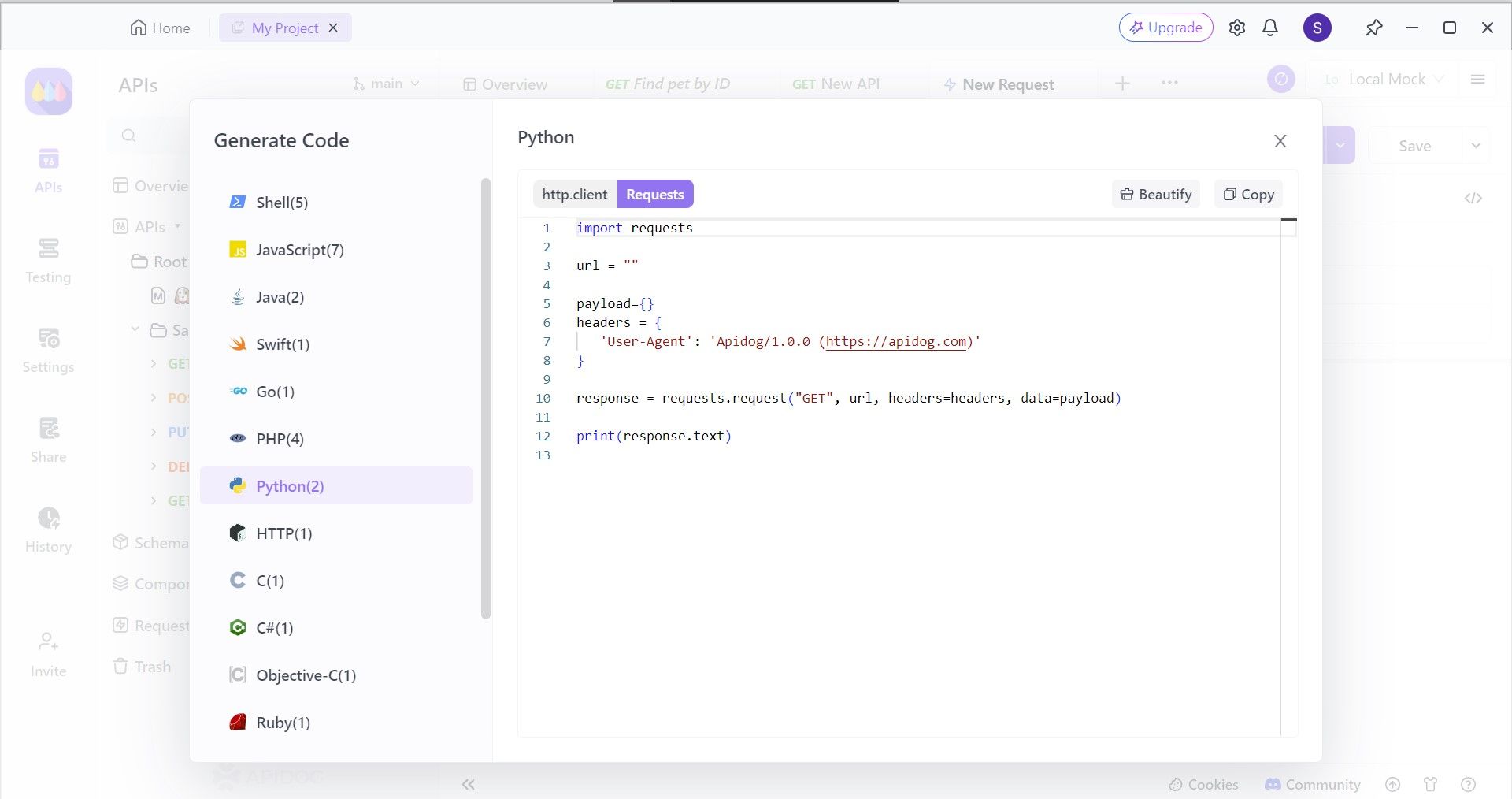
You can observe that Apidog supports code generation for a variety of other code languages as well. However, as we are trying to import Python client code, select Python. Copy and paste the code over to your IDE to begin completing your application.
Building APIs with Apidog
With Apidog, you can create APIs by yourself. You can create your API based on the design you intend for your application.
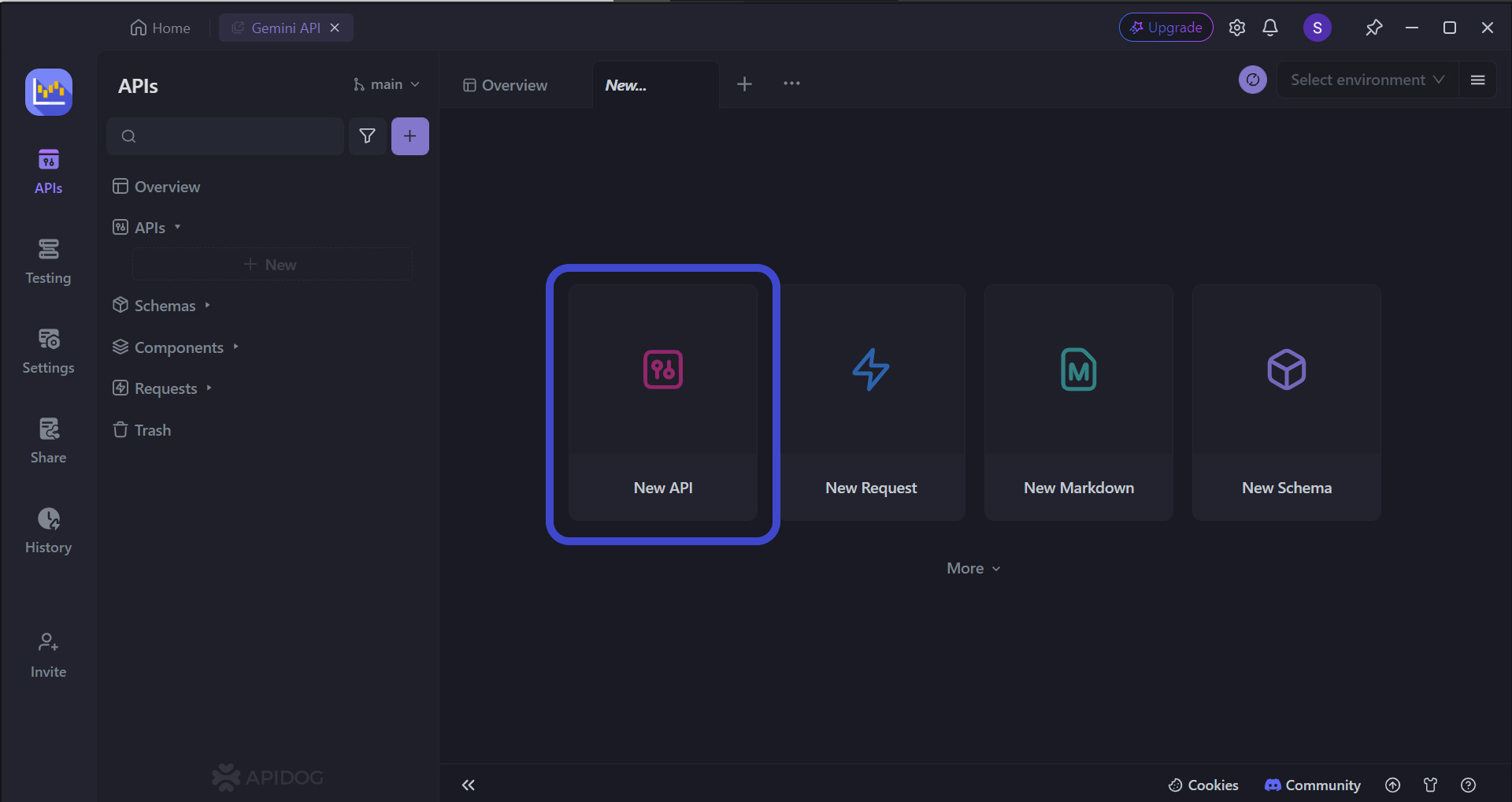
Begin by pressing the New API
button, as shown in the image above.
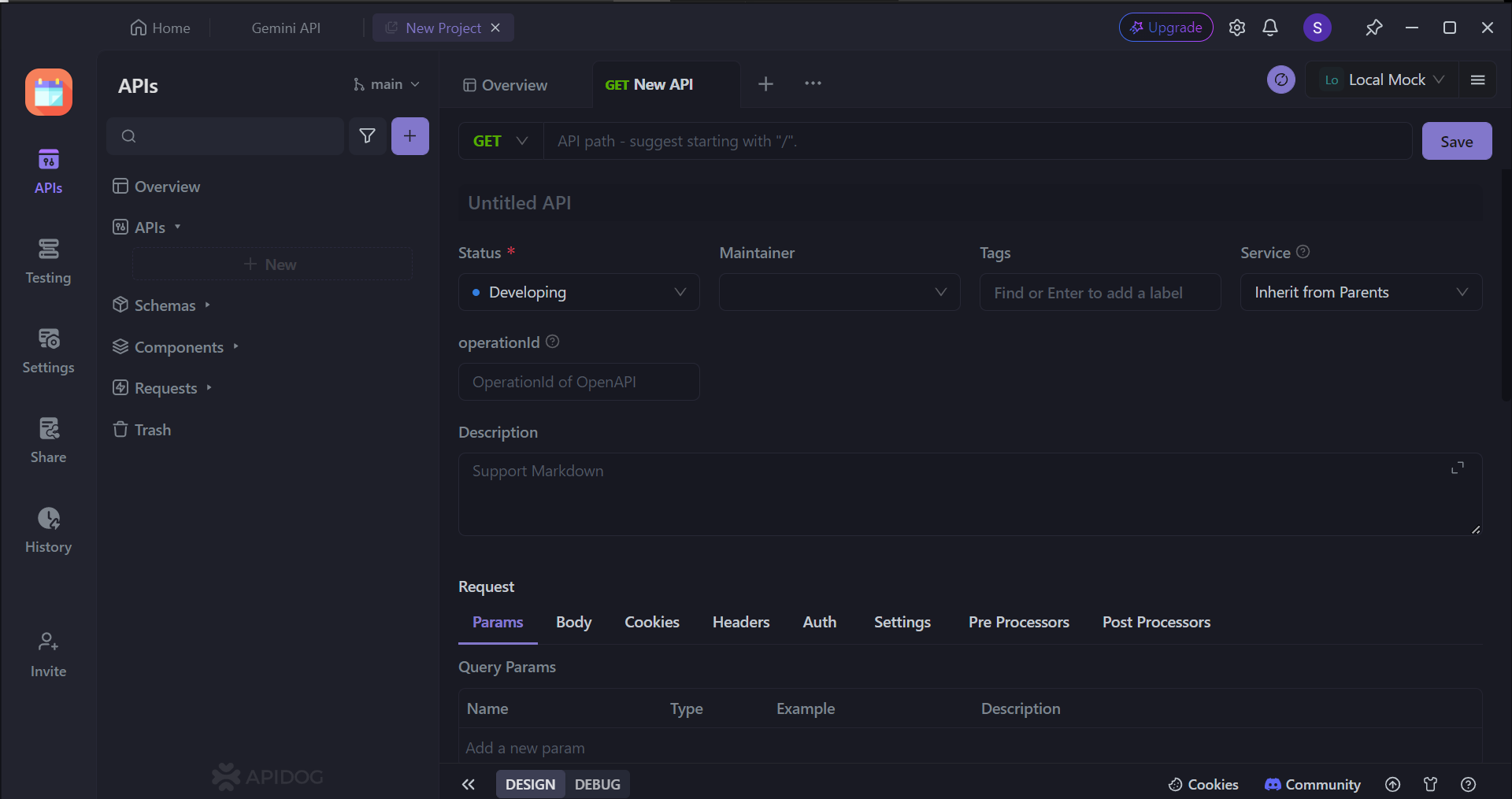
Next, you can select many of the API's characteristics. On this page, you can:
- Set the HTTP method (GET, POST, PUT, or DELETE)
- Set the API URL (or API endpoint) for client-server interaction
- Include one/multiple parameters to be passed in the API URL
- Provide a description of what functionality the API aims to provide. Here, you can also describe the rate limit you plan to implement on your API.
The more details you can provide to the designing stage, the more descriptive your API documentation will be, as shown in the next section of this article. You can also forewarn developers in the description that you are using the Python HTTPX library for creating this API.
To provide some assistance in creating APIs in case this is your first time creating one, you may consider reading these articles.
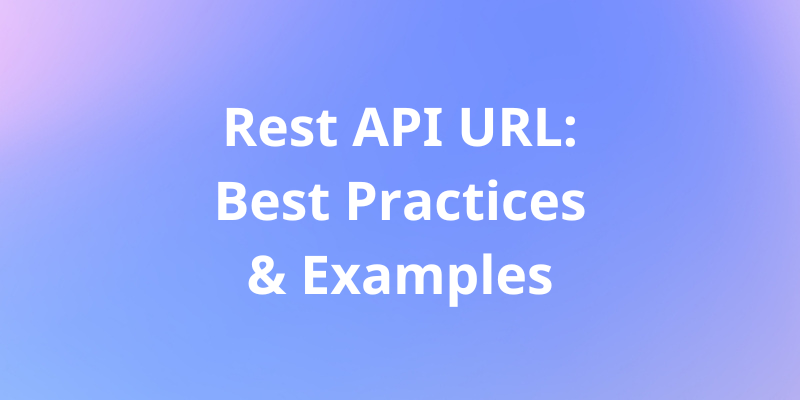
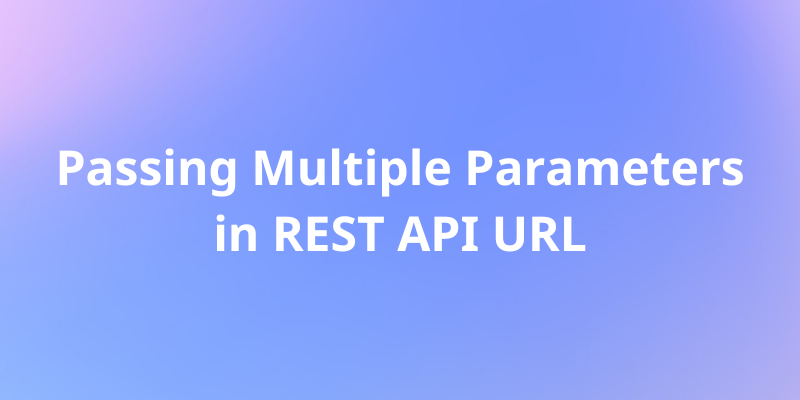
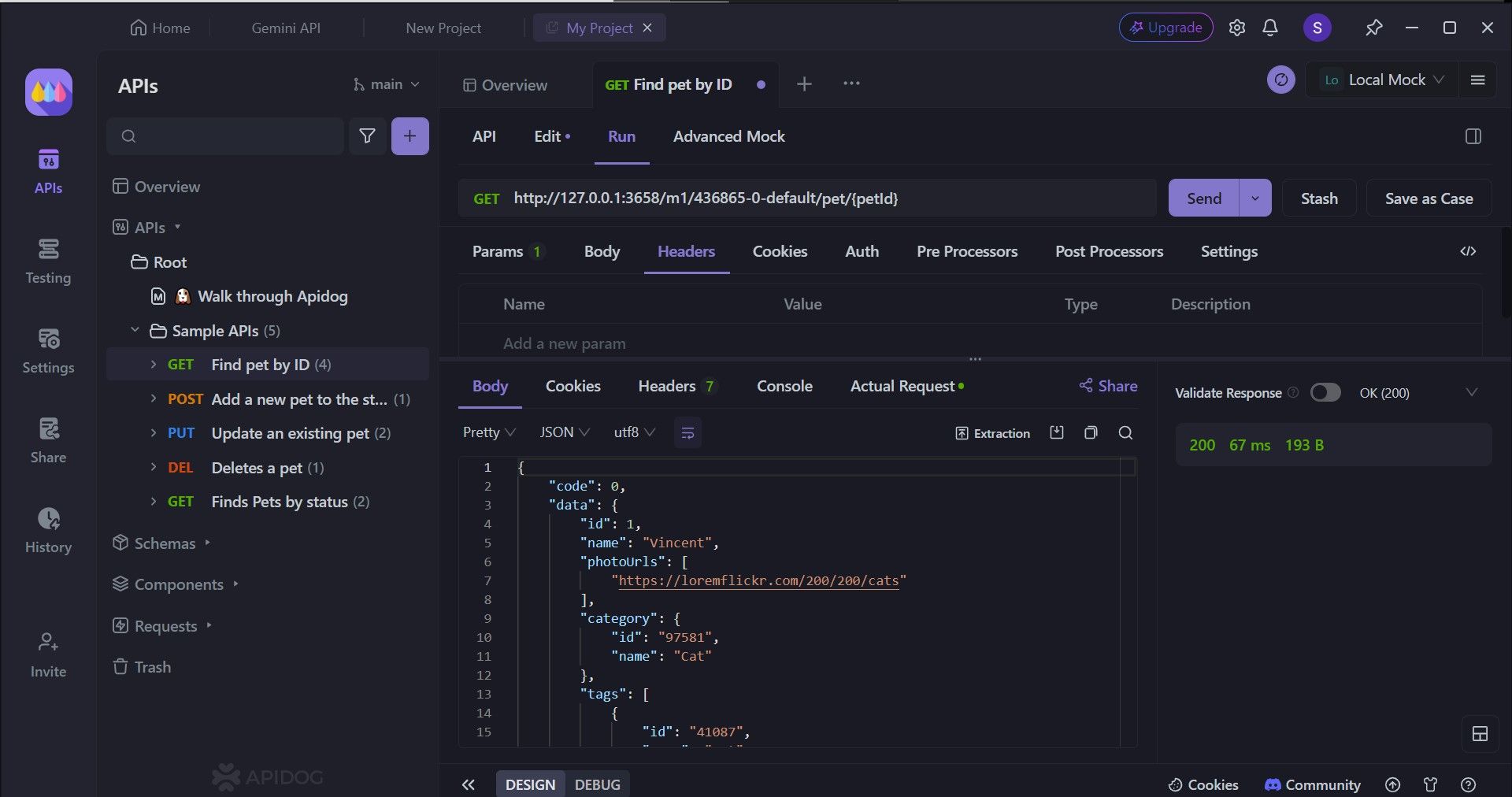
Once you have finalized all the basic necessities to make a request, you can try to make a request by clicking Send
. You should then receive a response on the bottom portion of the Apidog window, as shown in the image above.
The simple and intuitive user interface allows users to easily see the response obtained from the request. It is also important to understand the structure of the response as you need to match the code on both the client and server sides.
Generate Descriptive HTTPX API Documentation with Apidog
With Apidog, you can quickly create HTTPX API documentation that includes everything software developers need within just a few clicks.
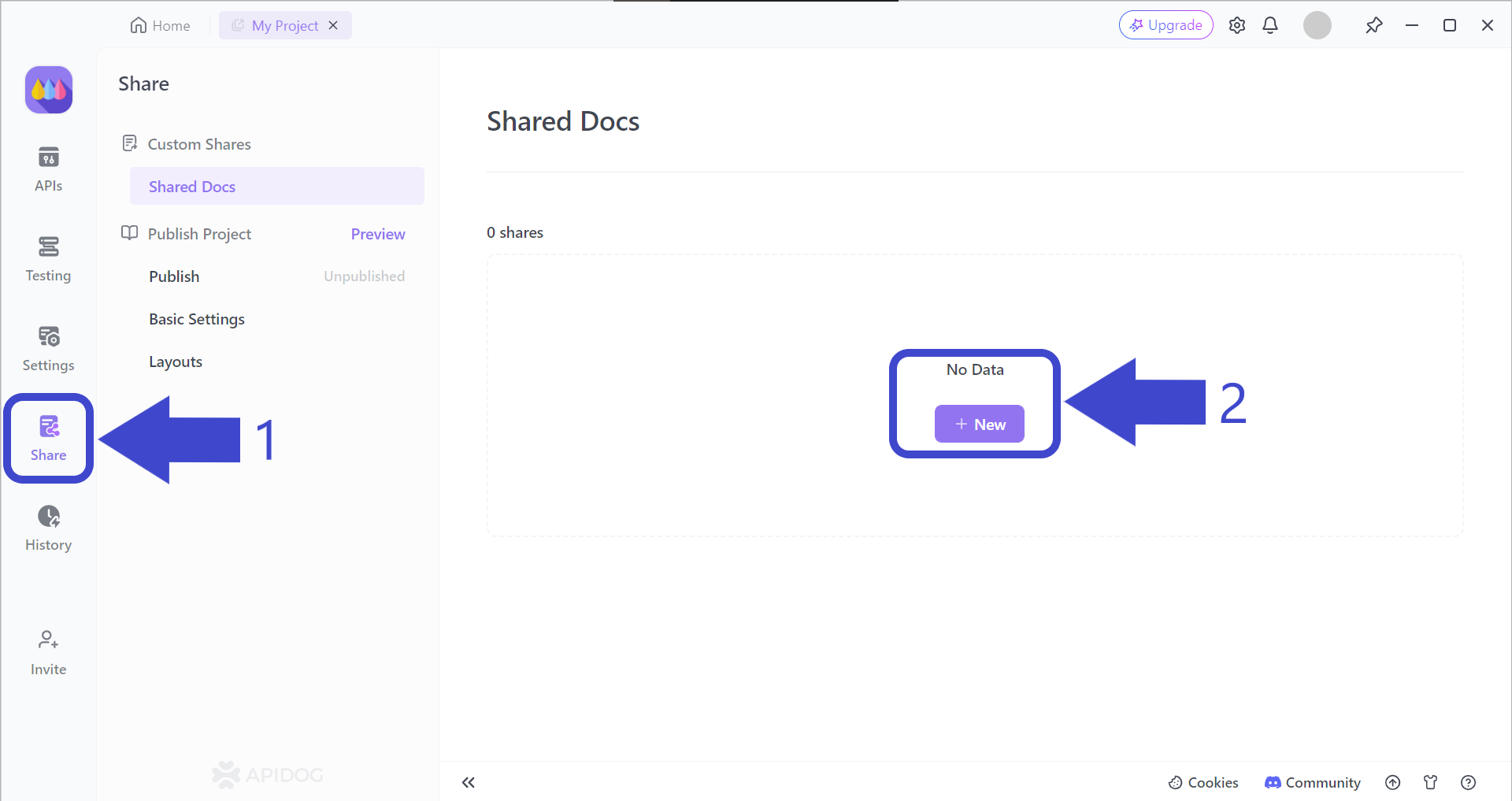
Arrow 1 - First, press the Share
button on the left side of the Apidog app window. You should then be able to see the "Shared Docs" page, which should be empty.
Arrow 2 - Press the + New
button under No Data
to begin creating your very first Apidog API documentation.
Select and Include Important API Documentation Properties
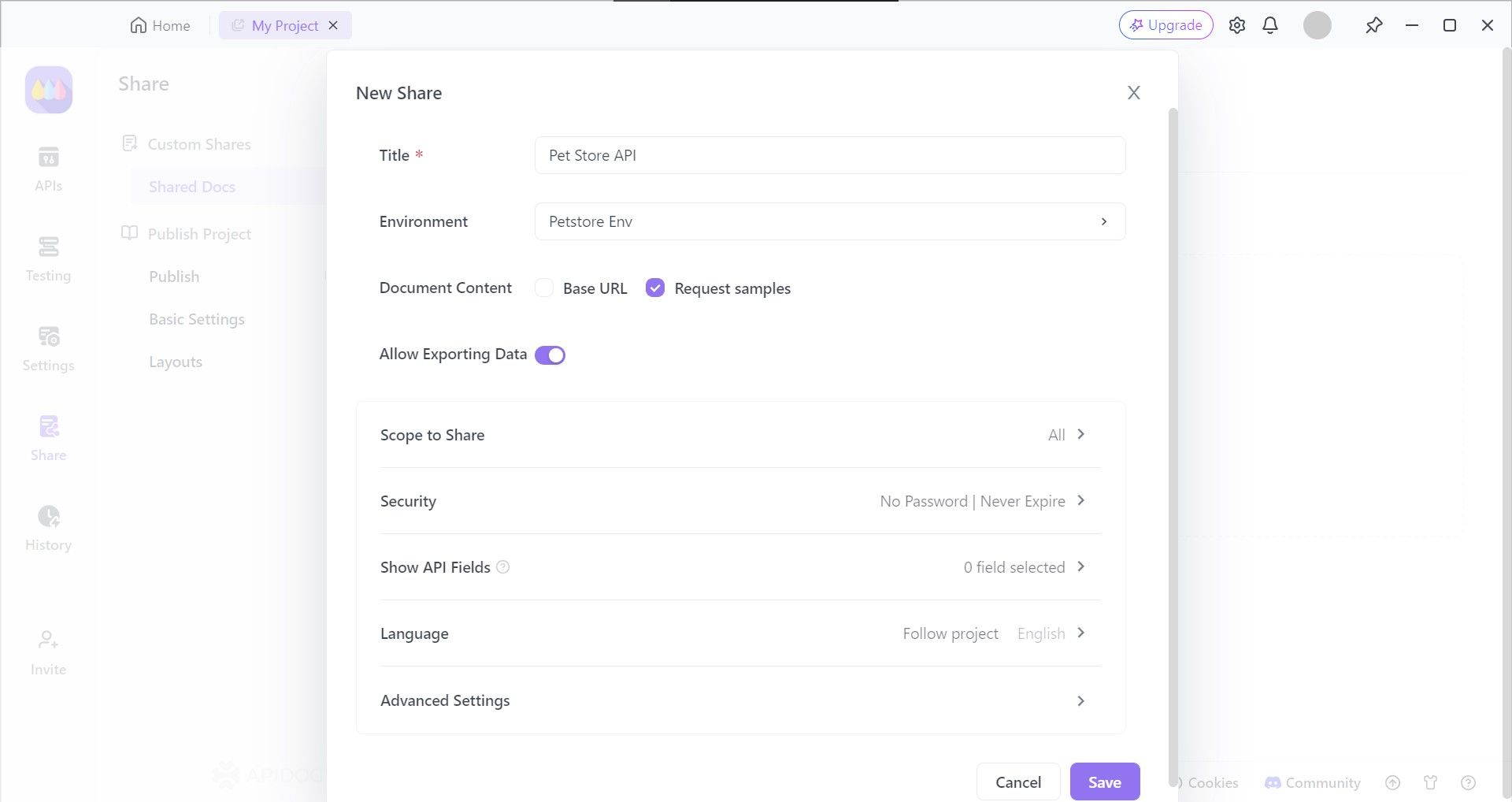
Apidog provides developers with the option of choosing the API documentation characteristics, such as who can view your API documentation and setting a file password, so only chosen individuals or organizations can view it.
View or Share Your API Documentation
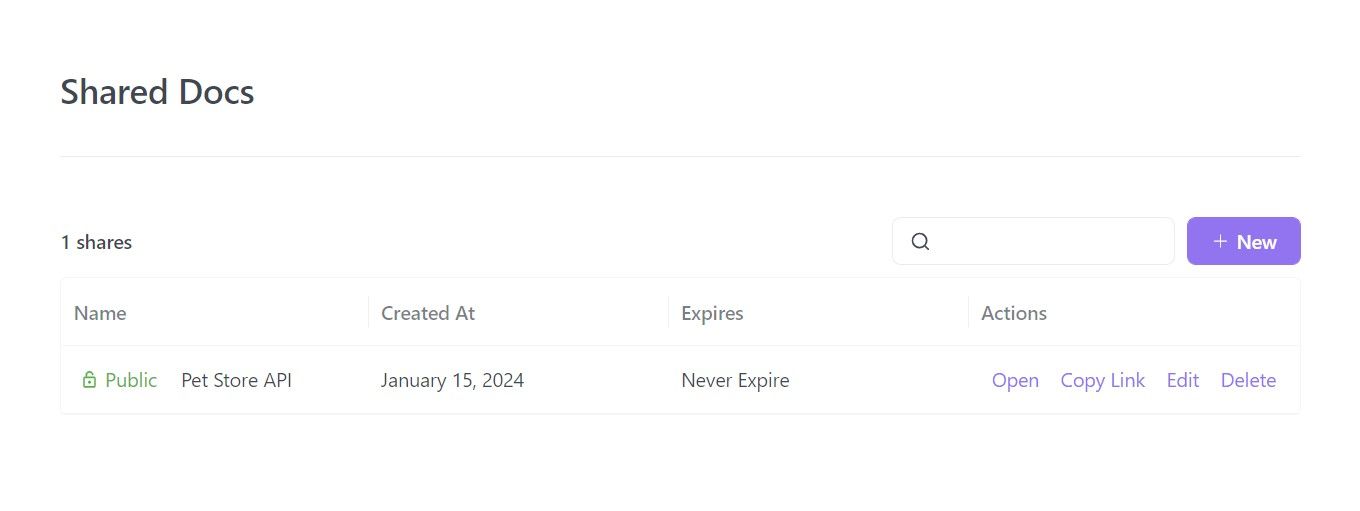
You can now share your HTTPX API documentation with anyone else. If you wish to distribute your documentation, you can copy and paste the URL to any messenger or email you wish. The other person will just have to open the link to access the HTTPX documentation!
If more details are required, read this article on how to generate API documentation using Apidog:
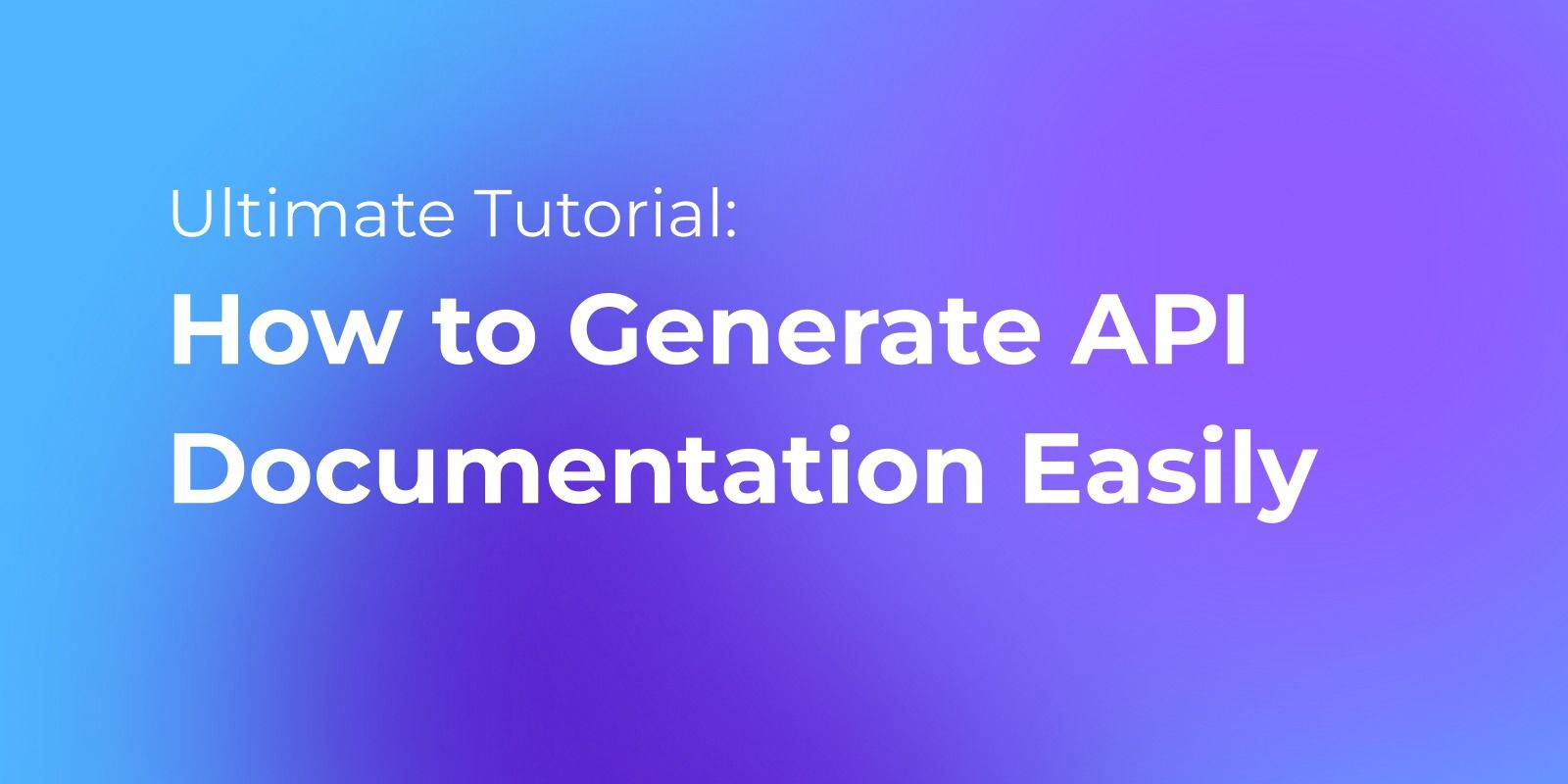
Conclusion
HTTPX emerges as a powerful Python library for crafting high-performance HTTP communication. Its asynchronous nature and emphasis on speed and efficiency make it a compelling choice for modern web development. When dealing with high-traffic applications, real-time data processing, or large data transfers, HTTPX shines.
Its capabilities like connection pooling, streaming support, and granular control over requests empower developers to build performant and scalable web applications. If your Python project prioritizes raw speed, efficient data handling, and fine-grained control over HTTP communication, HTTPX is the champion you've been looking for.
Apidog is more than capable of creating APIs that will be implemented in HTTPX-incorporated applications. On the other hand, if you wish to learn more about APIs, you can import various kinds of API file types over to Apidog to view, modify, mock and test them! All you need to do is download APidog via the button below.