Webrtc vs WebSocket: What's the Differences
In the intricate world of web communication technologies, WebRTC and WebSocket stand out as two pivotal players. While both are instrumental in the realm of modern web-based communication, they cater to distinct needs and operate under different paradigms. Let's delve deeper into these technologies to understand their nuances and explore their key differences in more detail.
Elevate your Debugging experience today – Checkout Download Button Below 👇👇👇
Comparison Table: Webrtc vs WebSocket
Aspect |
WebRTC |
WebSocket |
---|---|---|
Communication Type | Peer-to-peer | Client-server |
Data Types | Audio, video, and arbitrary data | Text, binary data (e.g., images, custom formats) |
Connection | Direct between users | Through a server |
Latency | Very low due to direct connection | Low, but higher than WebRTC |
Complexity | High (NAT traversal, signaling) | Lower |
Security | End-to-end encryption | Dependent on implementation |
Use Cases | Video/audio calls, live streaming, file sharing | Real-time chats, gaming, stock updates |
Browser Integration | Native, no plugins required | Native, no plugins required |
Customization | High (codec selection, data channels) | Moderate (protocol constraints) |
Scalability | Moderate (peer-to-peer can be resource-intensive) | High (efficient with server-client model) |
Typical Implementations | Video conferencing apps, peer-to-peer file sharing | Chat applications, live sports updates |
What Exactly is WebRTC?
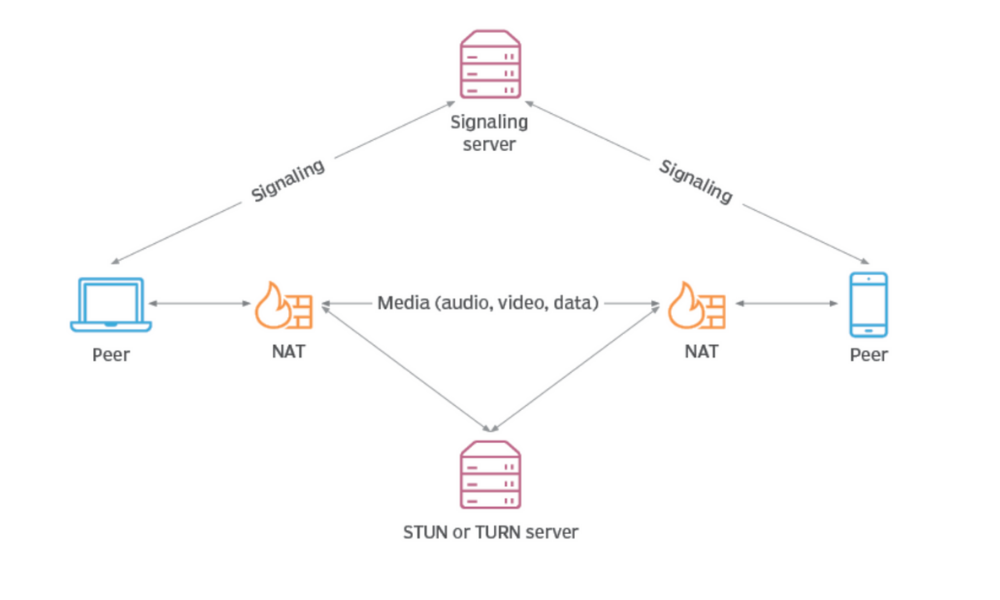
WebRTC, or Web Real-Time Communication, is a groundbreaking technology that facilitates direct, real-time communication between web browsers and devices. It's akin to a digital bridge, enabling users to share audio, video, and data in real-time, without the need for additional plugins or software.
The Standout Features of WebRTC
- Real-Time Communication: Offers live, instantaneous audio and video communication.
- Peer-to-Peer Connection: Creates direct links between users, which reduces latency and boosts the quality of interactions.
- Data Channels: Supports the sharing of various forms of data, enhancing the versatility of communication.
- Encryption and Security: Ensures secure communication with mandatory encryption for all data transfers.
- Browser-Based: Operates natively in browsers, eliminating the need for external installations.
// Sample code for setting up a simple WebRTC connection
// Getting local media stream
navigator.mediaDevices.getUserMedia({ video: true, audio: true })
.then(stream => {
const localVideo = document.getElementById('localVideo');
localVideo.srcObject = stream;
})
.catch(error => {
console.error('Error accessing media devices.', error);
});
// WebRTC peer connection setup
const peerConnection = new RTCPeerConnection(configuration);
Exploring WebSocket
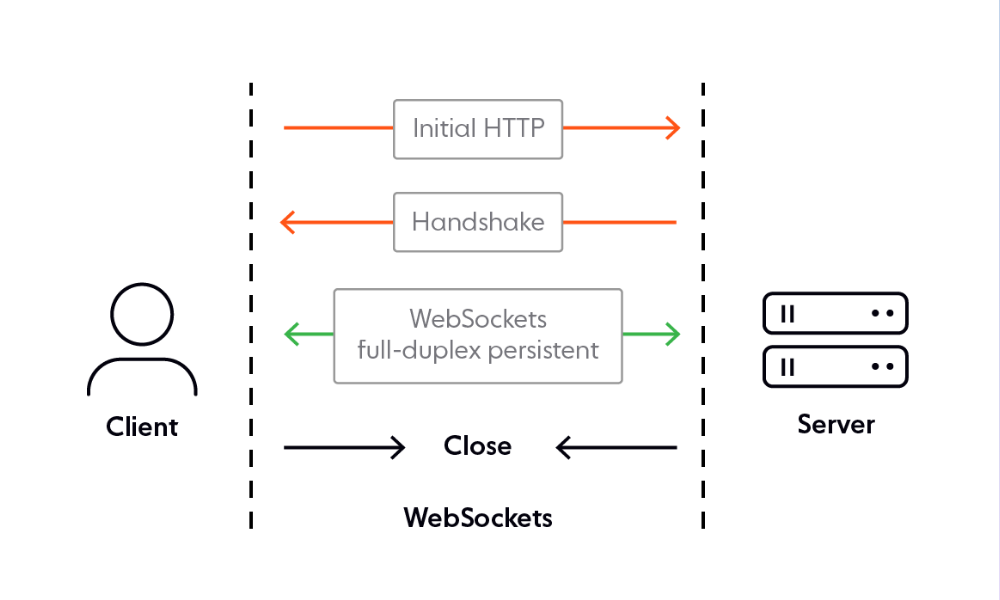
WebSocket, in contrast, is a protocol that enables ongoing, two-way communication between a client (like a web browser) and a server. It's like a digital pipeline that remains open, allowing continuous and instantaneous data flow in both directions.
WebSocket's Key Attributes
- Full-Duplex Communication: Enables two-way data exchange simultaneously.
- Low Latency: Maintains an open connection for faster data transfer compared to traditional HTTP connections.
- Versatility in Data Handling: Capable of transmitting a wide variety of data formats, including text and binary.
- Reduced Overheads: More efficient data transfer post-initial handshake, minimizing resource usage.
- Broad Compatibility: Supported across most modern web browsers.
// Example: Establishing a basic WebSocket connection
const socket = new WebSocket('ws://example.com');
socket.onopen = function(event) {
console.log("Connection opened");
};
socket.onmessage = function(event) {
console.log("Received message: " + event.data);
// Handle incoming messages...
};
socket.onerror = function(error) {
console.error("WebSocket Error: " + error);
};
// Sample function to send data through WebSocket
function sendData(data) {
socket.send(data);
}
Key Differences: WebRTC vs WebSocket
Communication Nature
- WebRTC: Specialized in direct, peer-to-peer communication, it's the go-to for real-time audio and video interactions. It bypasses servers, facilitating direct data transfer between users.
- WebSocket: Focused on client-server communication, it's ideal for situations where constant, two-way data exchange is necessary, such as in chat applications or live feed updates.
Data Handling
- WebRTC: Primarily optimized for high-quality audio and video streaming. It also supports arbitrary data sharing, but its forte is real-time, rich media communication.
- WebSocket: Exhibits a broader range in data handling, efficiently managing both text and binary data. This versatility makes it suitable for various applications, from text-based chats to complex binary data transfers like image sharing.
Connection Dynamics
- WebRTC: Operates through a direct connection between peers. This setup significantly reduces latency, making it ideal for applications where real-time interaction is crucial, like video conferencing or live gaming.
- WebSocket: Although it provides a persistent connection, it does so through a server. This architecture can introduce some latency compared to direct peer-to-peer connections but remains much more efficient than traditional HTTP polling.
Use Cases and Applications
- WebRTC: Best suited for applications that require immediate, interactive communication, such as video and audio conferencing, live broadcasting, and peer-to-peer file sharing.
- WebSocket: Excellently suited for applications where users need to maintain a continuous line of communication with a server, like real-time notifications, multiplayer online games, and collaborative editing tools.
Complexity and Deployment
- WebRTC: This tends to be more complex to implement due to the need for handling NAT traversal, signaling for connection establishment, and dealing with various media formats.
- WebSocket: Generally simpler in terms of setup and deployment. It requires handling the initial handshake and maintaining a stable connection but lacks the complexity of direct peer-to-peer communication management.
Debugging WebSocket connections using Apidog can be streamlined into five key steps. This approach simplifies the process, allowing you to effectively identify and resolve issues with your WebSocket implementation.
How to Debug WebSocket With Apidog
Debugging WebSocket connections using Apidog can be streamlined into five key steps. This approach simplifies the process, allowing you to effectively identify and resolve issues with your WebSocket implementation.
Step 1: Set Up Apidog Workspace
- Create an Apidog Account: If you don't already have one, sign up for an Apidog account.
- Initialize a Workspace: Once logged in, create a new workspace. This will be your primary area for managing WebSocket debugging and other API testing tasks.
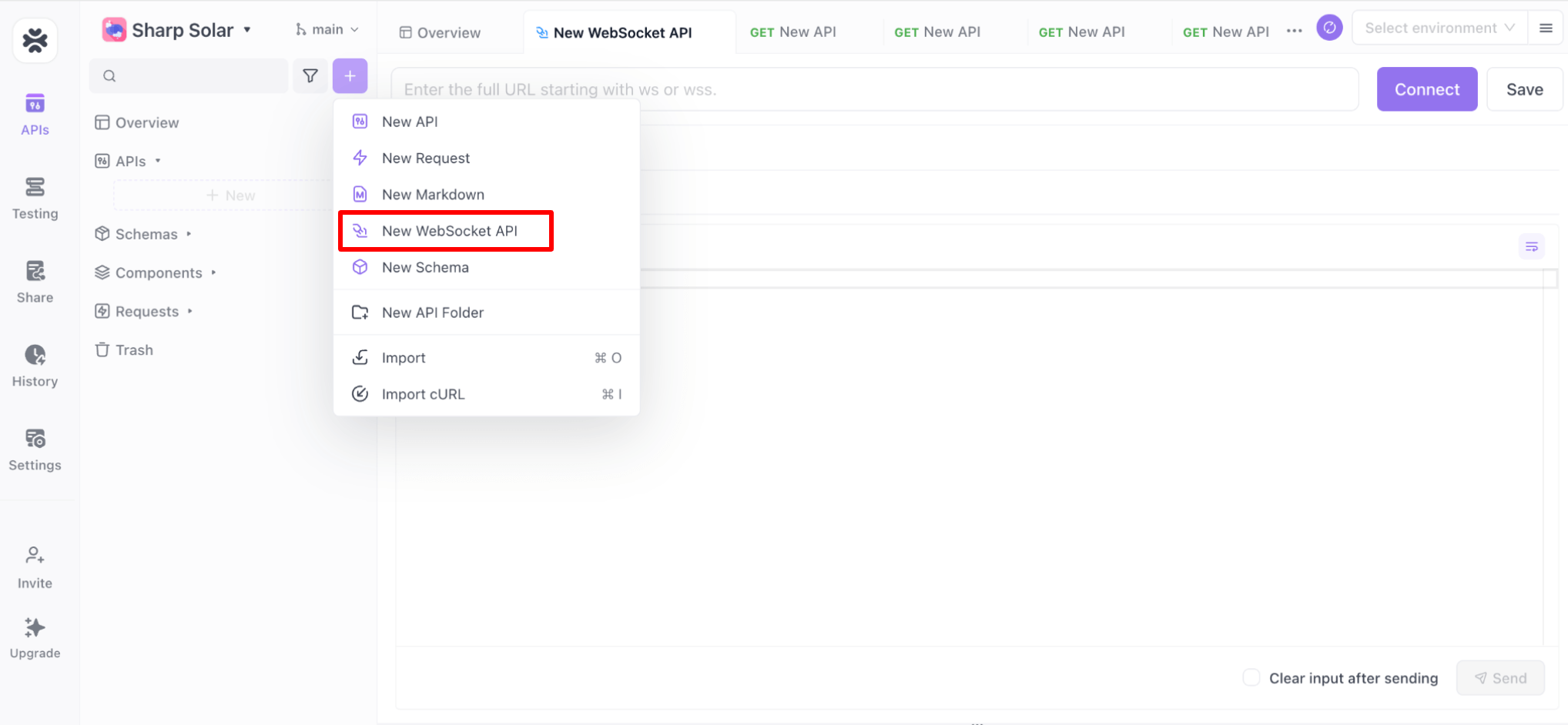
Step 2: Configure Your WebSocket Endpoint
- Add a New Project: Within your workspace, start a new project specifically for WebSocket debugging.
- Define WebSocket Service: In this project, add a new service for your WebSocket. You'll need to provide the WebSocket URL (
ws://
orwss://
) and configure any necessary headers or authentication details.
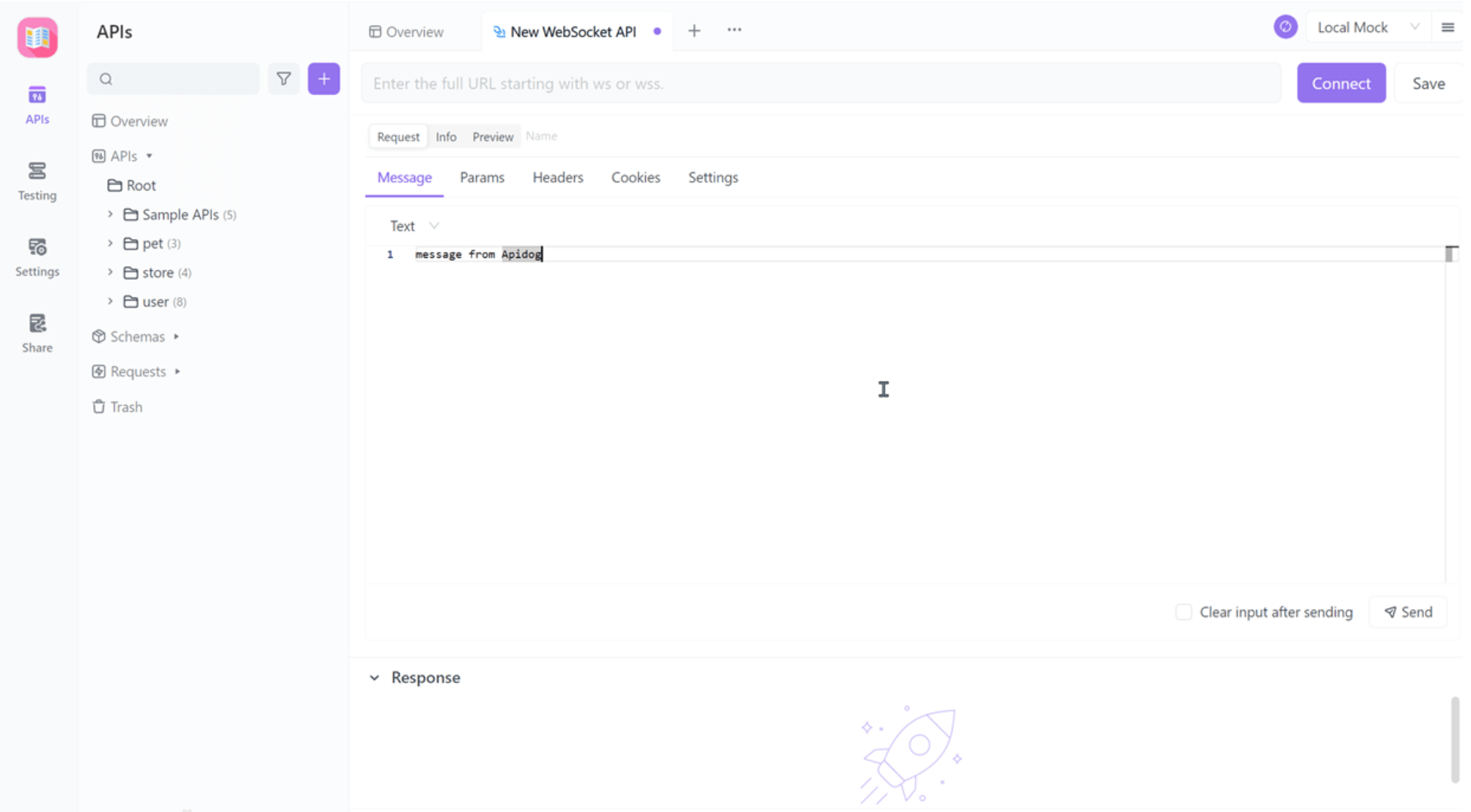
Step 3: Establish and Monitor WebSocket Connection
- Connect to Your WebSocket Server: Utilize Apidog's interface to establish a connection with your WebSocket server. Ensure that the server is online and reachable.
- Observe Connection Status: Apidog will display the connection status, helping you to verify whether the WebSocket connection is successful or if there are initial connection issues.
Step 4: Test with Messages and Analyze Responses
- Send Test Requests: Through the Apidog interface, send various test messages to your WebSocket server. This can include different scenarios you want to test.
- Review Server Responses: Monitor how your server responds to each request. Apidog will display incoming messages, enabling you to analyze the server's behavior and response patterns.
Step 5: Debug and Optimize
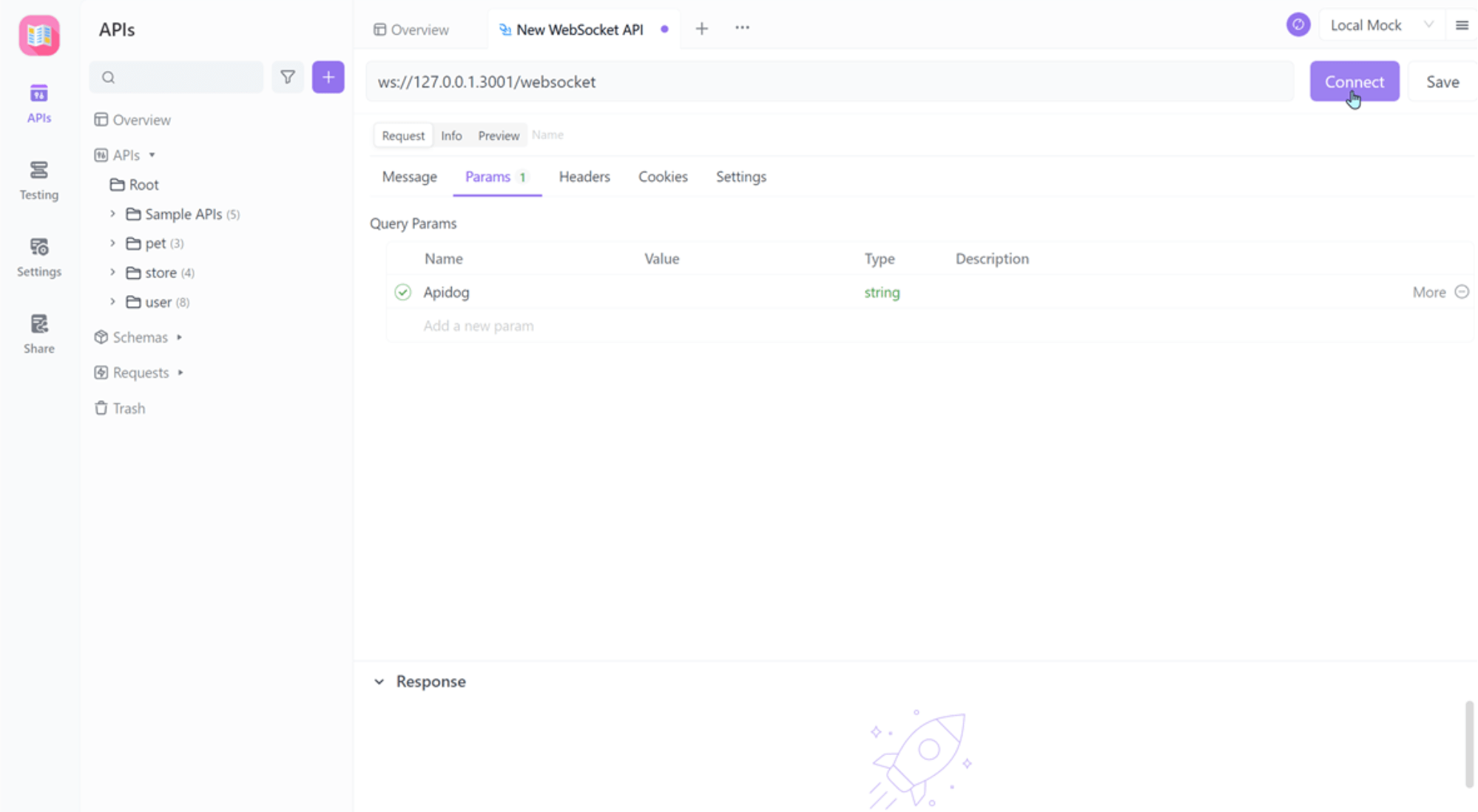
- Analyze Interaction Data: Examine the details of the messages exchanged (both sent and received) for unexpected behavior or errors.
- Iterative Debugging: Based on the insights gained, modify your server code or WebSocket request as needed and repeat the testing process until the desired performance and reliability are achieved.
Conclusion
WebRTC and WebSocket, while serving the umbrella purpose of enhanced web communication, cater to distinctly different needs. WebRTC excels in facilitating real-time, high-quality communication directly between users, making it a cornerstone technology in applications like video conferencing and live streaming. WebSocket, on the other hand, is the backbone of continuous client-server communication, playing a crucial role in chat applications, online gaming, and real-time data feeds. Understanding these key differences allows developers and businesses to choose the right technology based on their specific communication requirements and application needs, leading to more efficient and effective digital solutions.