Webhook vs WebSocket: Details Comparison You Should Know
The digital age has brought a paradigm shift in how applications communicate over the web. Two pivotal technologies facilitating this are Webhooks and WebSockets. Both serve distinct purposes in the web development ecosystem and understanding their nuances is key to optimizing web application performance and user experience.
Click the Download button and elevate your WebSocket debugging experience!
In-Depth Overview: Webhook vs WebSocket
What is Webhooks?
A Webhook, in the simplest terms, is a method for augmenting or altering the behavior of a web page or web application with custom callbacks. These callbacks may be maintained, modified, and managed by third-party users and developers who may not necessarily be affiliated with the originating website or application.
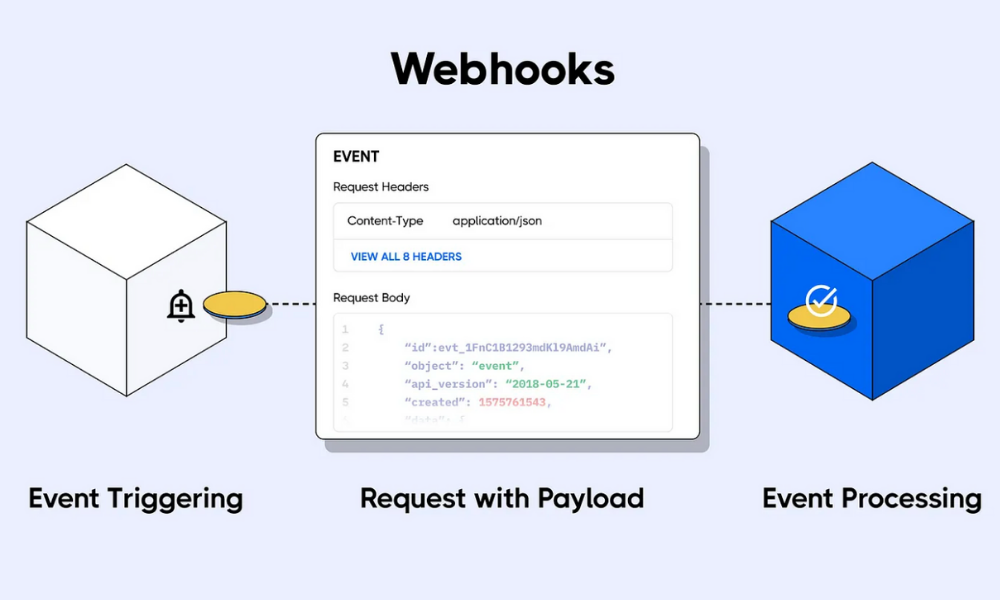
How Webhooks Works:
- Event Monitoring: Webhooks are configured to monitor events within a system or application.
- Trigger Activation: When the specified event occurs, the Webhook is triggered.
- HTTP POST Execution: The Webhook reacts by sending an HTTP POST request to a specified URL.
- Payload Delivery: This request includes a payload, which typically contains information about the event that triggered the Webhook.
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
app.use(bodyParser.json()); // for parsing application/json
// Webhook endpoint listening for POST requests
app.post('/webhook', (req, res) => {
console.log('Received Webhook:', req.body);
// Process the Webhook payload
// e.g., trigger an action based on the event
res.status(200).send('Webhook received!');
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => console.log(`Webhook listener running on port ${PORT}`));
Advantages:
- Efficient Notification System: Enables applications to receive real-time updates.
- Customizable: Can be tailored to specific events and needs.
- Independence from Polling: Reduces the need for constant checking or polling for updates.
Disadvantages:
- Limited to Predefined Events: Only effective for the events they are set to monitor.
- Security Risks: Exposed endpoints may be targets for malicious attacks.
- Dependence on External Services: Reliability depends on the external server's uptime and performance.
What is WebSockets?
WebSocket is a communication protocol that provides a full-duplex communication channel over a single TCP connection. It enables interaction between a web browser (or other client application) and a web server with lower overheads, facilitating real-time data transfer.
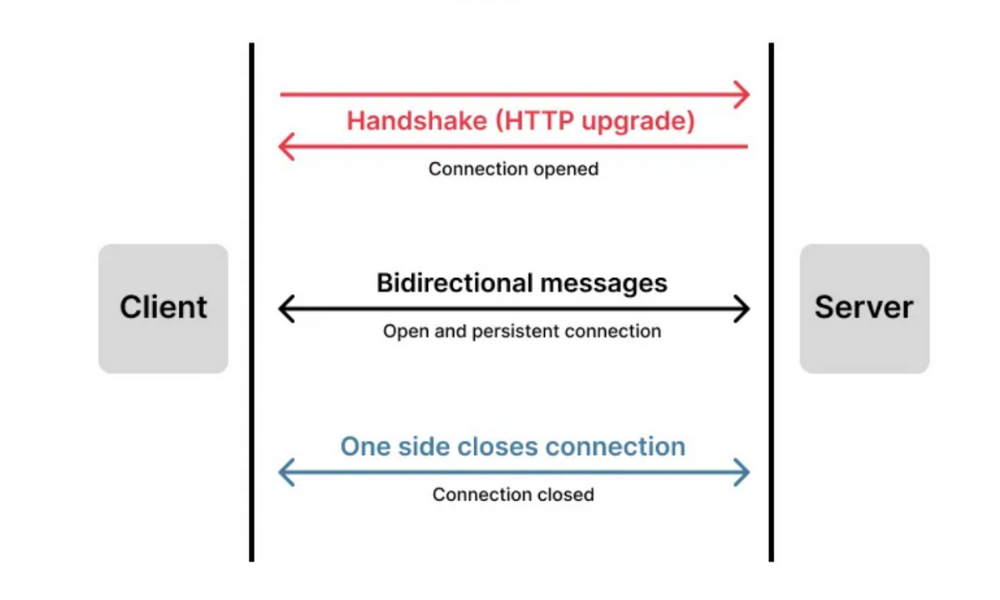
How WebSockets Works:
- Connection Establishment: Initiated with an HTTP handshake, which is then upgraded to a WebSocket connection.
- Persistent Connection: Unlike HTTP, the WebSocket connection remains open, allowing ongoing communication.
- Data Transfer: Messages can be sent from client to server and vice versa at any time, enabling real-time, bidirectional communication.
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', (ws) => {
console.log('New client connected');
ws.on('message', (message) => {
console.log(`Received message: ${message}`);
// Echo the received message back to the client
ws.send(`Echo: ${message}`);
});
ws.on('close', () => {
console.log('Client has disconnected');
});
});
Advantages:
- Real-Time Communication: Ideal for applications requiring live data feeds.
- Reduced Overheads: Eliminates the need for repeatedly opening and closing connections.
- Full Duplex: Simultaneous two-way communication is possible.
Disadvantages:
- Complex Implementation: More intricate to implement compared to traditional HTTP requests.
- Scalability Challenges: Managing numerous open connections simultaneously can be demanding.
- Resource Intensity: Maintains constant connections, which can consume more server resources.
Comparative Analysis Webhook vs WebSocket
Communication Model
- Webhook: Operates on a one-way, event-driven model. It is essentially a stateless communication method.
- WebSocket: Provides a two-way, persistent communication channel. It's stateful and maintains context over its open connection.
Ideal Use Cases
- Webhook: Best suited for scenarios where specific events trigger actions, such as notifications, integrations, or automated workflows.
- WebSocket: Ideal for applications requiring real-time data exchange, interactive interfaces, and live updates.
Implementation and Maintenance
- Webhook: Easier to set up with minimal coding. Less resource-intensive but requires robust security measures.
- WebSocket: More complex to implement and maintain. Requires more server resources and careful handling of open connections.
Scalability and Performance
- Webhook: Scalable for event-driven communication, with limited impact on performance.
- WebSocket: Offers superior performance for real-time communication but can encounter scalability issues with high user volumes.
Comparison Table: Webhook vs WebSocket
Feature | Webhook | WebSocket |
---|---|---|
Definition | A method for sending automated messages (HTTP POST requests) to a specific URL when an event occurs in an application. | A communication protocol providing a full-duplex communication channel over a single, persistent TCP connection. |
Communication Type | Asynchronous, one-way (server to client). | Synchronous, bi-directional (client to server and vice versa). |
Connection | A Stateless, connection is made only when an event triggers the Webhook. | Stateful, maintains a persistent connection once established. |
Data Transfer | Data is sent only when certain events occur. | Continuous data transfer, with the ability to send and receive messages at any time. |
Use Cases |
|
|
Pros |
|
|
Cons |
|
|
Implementation | Relatively easy and quick to set up. | Requires more complex setup and ongoing maintenance. |
Scalability | Easily handles large numbers of events. | Can face challenges with many open, concurrent connections. |
Resource Utilization | Low, as it operates only upon events. | High, due to maintaining open connections. |
Why Choose Apidog for WebSocket Debugging?
First off, why pick Apidog? Well, it's a bit like having a Swiss Army knife for API debugging. It's versatile and user-friendly, and it gets the job done. Whether you're a seasoned pro or just dipping your toes into the world of WebSocket services, Apidog has got your back.
Step-by-Step Guide to Debugging with Apidog
Now, onto the main event. Here's how you get your WebSocket service and Apidog to have a meaningful conversation:
- Initiate Contact: Launch Apidog and look for that friendly "+" button. Give it a click, and you're on your way.
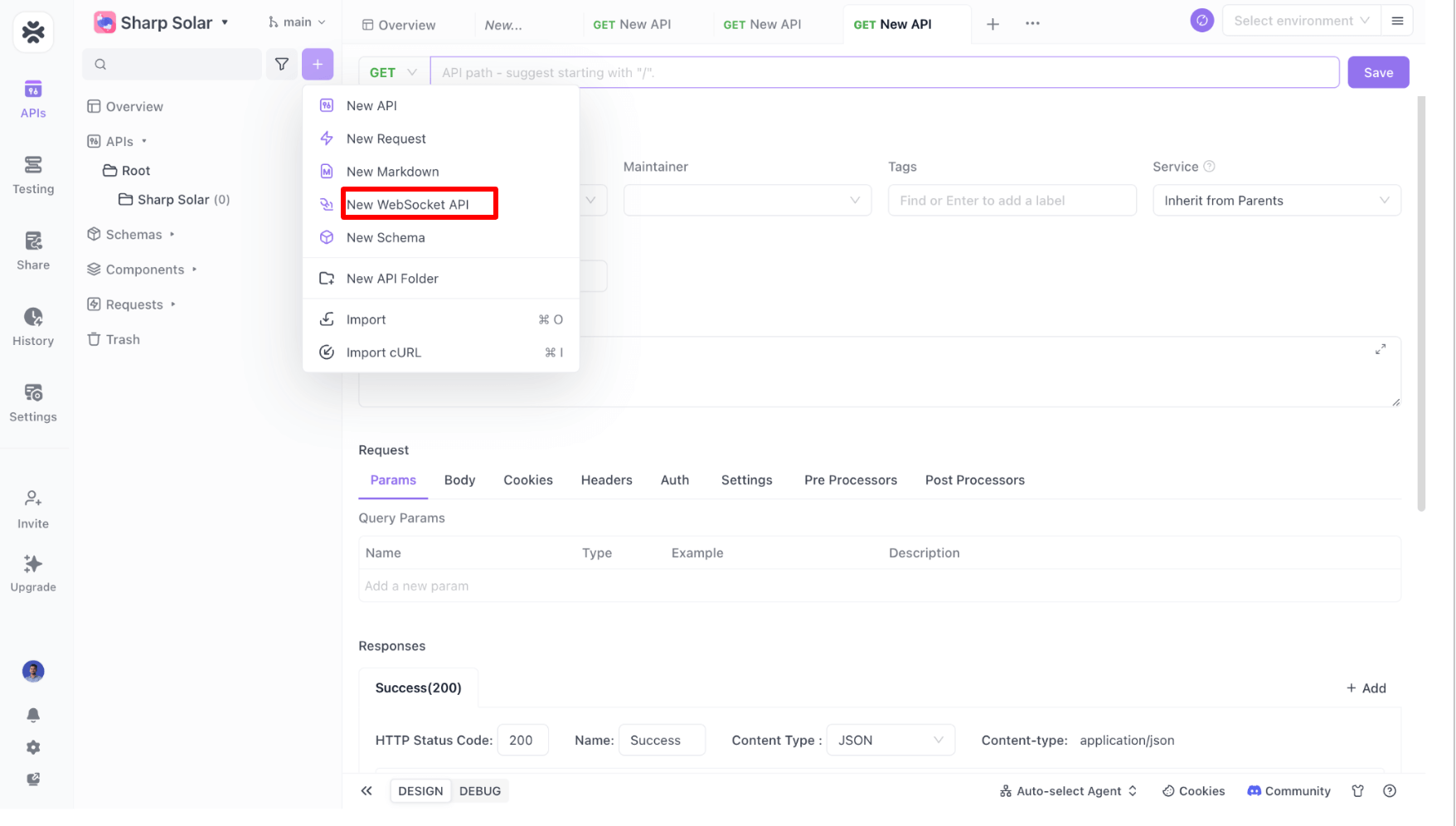
2. Dialing the Right Number: Enter your WebSocket service's address. Think of it like putting the right address into your GPS – you need to know where you're going!
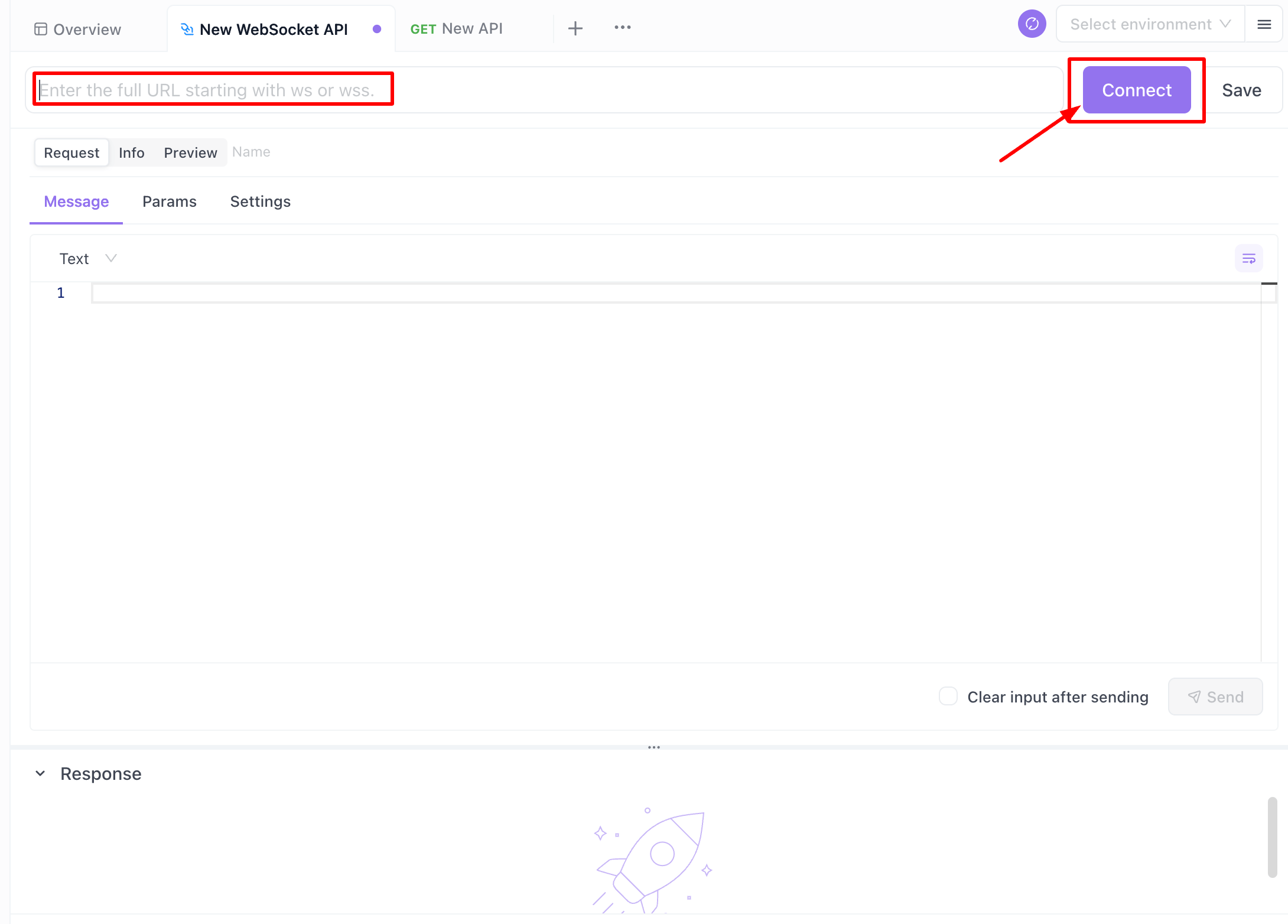
3. The Extras: If you're feeling fancy, fill in the "Message" and "Params" fields. It's like adding toppings to your pizza – not always necessary, but often makes things better.
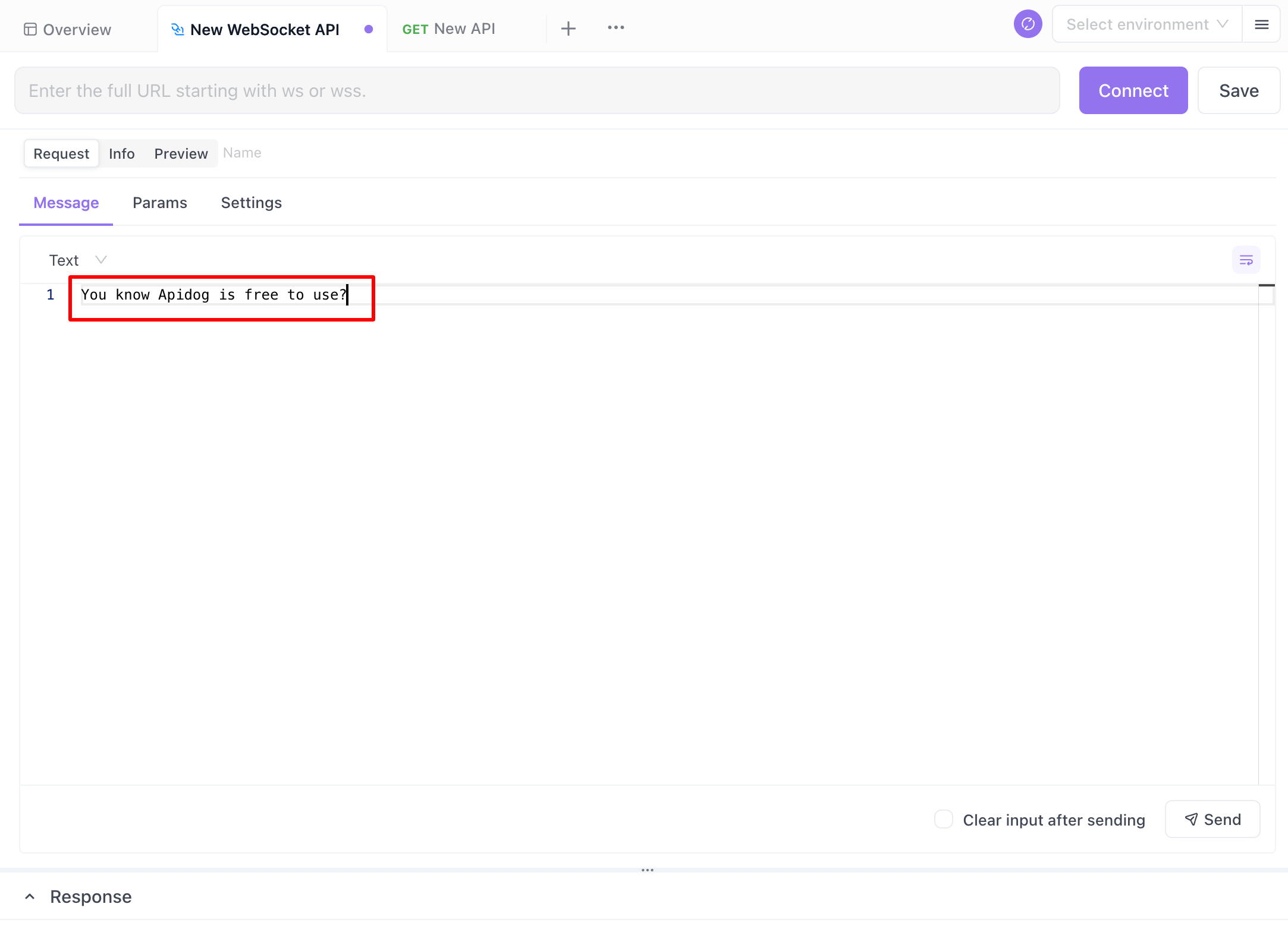
4. Save for Later: Hit the "Save" button. It's like bookmarking your favorite recipe. You'll thank yourself later.
5. The Handshake: Click "Connect" and watch as Apidog reaches out to your WebSocket service. It's like making a new friend.
6. Chit-chat: Now that you're connected, use the "Send" button to chat with your server. Think of it as texting – but for debugging.
Conclusion
Webhooks and WebSockets serve distinct but equally important roles in modern web development. The choice between them hinges on the specific requirements of the application. Webhooks are ideal for applications requiring asynchronous, event-driven communication. On the other hand, WebSockets are the go-to solution for applications that demand real-time, synchronous, and interactive communication. Understanding and leveraging the strengths of each technology can significantly enhance the functionality and user experience of web applications.