What is Web API Security? Best Practices for APIs Protecting
In today's digital age, Web APIs (Application Programming Interfaces) serve as the backbone of much of the web's functionality, enabling systems to communicate with each other seamlessly. However, as the reliance on these APIs grows, so does the need for robust security measures. Web API security refers to the strategies, technologies, and practices designed to protect APIs from being exploited by unauthorized parties. Let's dive deep into the realm of API security, outlining its importance and best practices to ensure your APIs remain protected.
Click the Download button below to explore the world's largest API empire.
What is Web API Security?
At its core, Web API security is about safeguarding the integrity, confidentiality, and availability of the web APIs that applications use to interact. This involves preventing attacks, data breaches, and unauthorized access, which could compromise sensitive information or disrupt services. Given the critical role APIs play in the modern internet ecosystem, ensuring their security is not just a technical requirement but a business imperative.
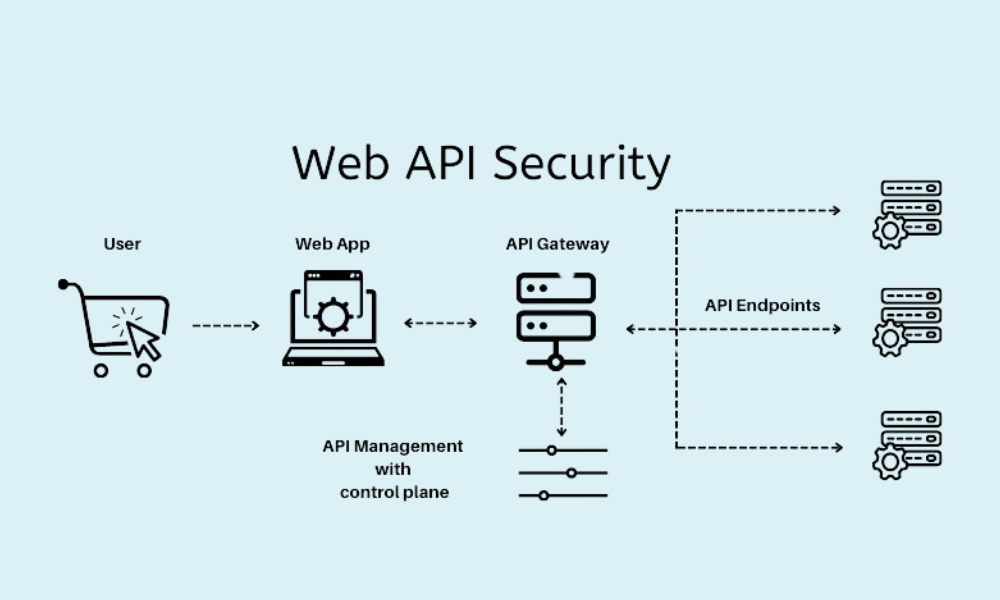
The Importance of Securing Web APIs
Securing Web APIs is not just a best practice—it's a necessity in today's interconnected digital landscape. As the glue that binds different web services together, APIs, if left unprotected, can become prime targets for cyberattacks. This can lead to dire consequences, not just for the service provider, but also for end-users who rely on these digital services daily. The importance of implementing robust security measures for Web APIs cannot be overstated. Here are five critical reasons why securing your Web APIs should be a top priority:
- Protect Sensitive Data: Ensuring that personal, financial, or confidential information remains secure from unauthorized access.
- Maintain Service Integrity: Keeping APIs functioning as intended without interference from malicious entities.
- Compliance and Legal Obligations: Adhering to data protection and privacy laws to avoid legal repercussions and fines.
- Prevent Data Breaches: Minimizing the risk of incidents that can lead to significant financial losses and reputational damage.
- Ensure Service Availability: Guarding against attacks, such as DDoS, which aim to disrupt service availability to users.
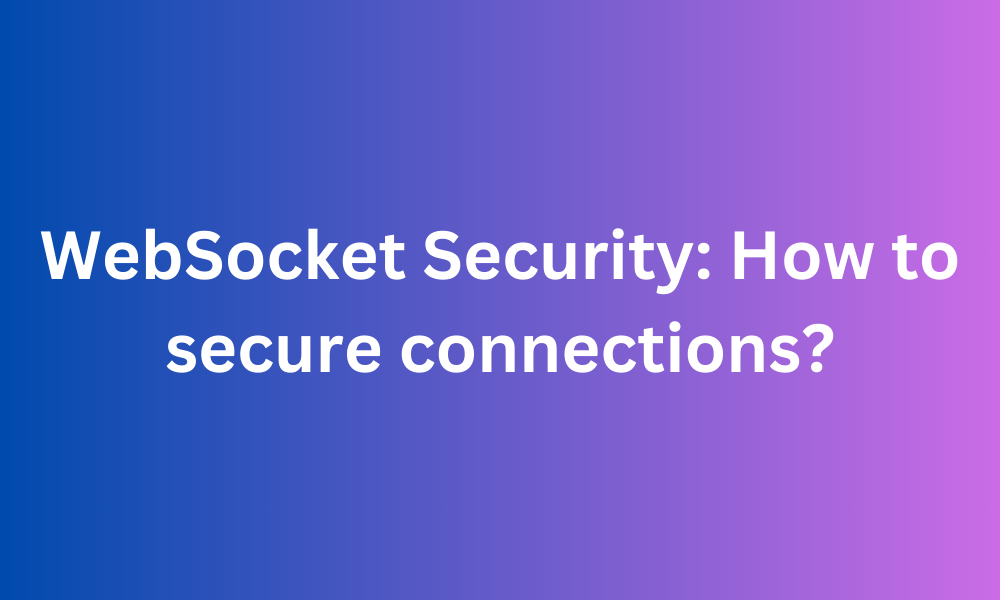
7 Best Practices for Protecting Your APIs
Protecting your APIs is like building a fortress around your digital assets. It requires a combination of strategies, tools, and practices. Here are some of the best practices to fortify your API security.
Use Authentication and Authorization Mechanisms
Implement Strong Authentication
Ensure that only authenticated users can access your API. Techniques like OAuth2, API keys, and JSON Web Tokens (JWT) provide robust frameworks for authenticating API requests, acting as the gatekeepers to your digital fortress.
const jwt = require('jsonwebtoken');
const express = require('express');
const app = express();
// Secret key for JWT signing and encryption
const SECRET_KEY = 'your_secret_key_here';
// Middleware to validate JWT
const authenticateToken = (req, res, next) => {
const authHeader = req.headers['authorization'];
const token = authHeader && authHeader.split(' ')[1]; // Bearer TOKEN
if (token == null) return res.sendStatus(401); // If no token, return unauthorized
jwt.verify(token, SECRET_KEY, (err, user) => {
if (err) return res.sendStatus(403); // If token is not valid, return forbidden
req.user = user;
next();
});
};
app.get('/api/resource', authenticateToken, (req, res) => {
// Resource access logic here
res.json({ message: "Access granted to protected resource", user: req.user });
});
app.listen(3000, () => console.log('Server running on port 3000'));
Authorization Checks
Once a user is authenticated, determine what resources they are allowed to access. This is where authorization comes into play. Implement role-based access control (RBAC) or attribute-based access control (ABAC) to ensure users can only interact with resources appropriate to their permissions.
Secure Data Transmission
Utilize HTTPS
Always use HTTPS (Hypertext Transfer Protocol Secure) for data in transit. This ensures that data between the client and server is encrypted, making it much harder for eavesdroppers to intercept sensitive information.
Securing data in transit using HTTPS is crucial. For a Node.js application, ensuring your server supports HTTPS could look like this:
const https = require('https');
const fs = require('fs');
const options = {
key: fs.readFileSync('path/to/your/private.key'),
cert: fs.readFileSync('path/to/your/certificate.crt')
};
https.createServer(options, (req, res) => {
res.writeHead(200);
res.end('Hello Secure World!\n');
}).listen(443);
Validate Input to Prevent Injection Attacks
Validate all input data to ensure it meets the expected format. This helps prevent SQL injection, command injection, and other injection attacks, which exploit security vulnerabilities to run malicious code.
Implement Rate Limiting and Throttling
Rate limiting controls how many requests a user can make to your API within a certain timeframe. Throttling, on the other hand, limits the number of API calls to prevent overuse. Both practices help mitigate DDoS (Distributed Denial of Service) attacks and ensure that your API remains available to users.
Manage Sensitive Data Carefully
Encrypt Sensitive Data
When storing sensitive information, encryption is crucial. Whether it's passwords, personal information, or financial data, encryption ensures that even if data is accessed, it remains unreadable without the decryption key.
Encryption of sensitive data can be handled in various ways. Here's an example using the crypto
module in Node.js for AES encryption:
const crypto = require('crypto');
const algorithm = 'aes-256-cbc';
const password = 'password'; // Use a secure password and environment variable in production
const key = crypto.scryptSync(password, 'salt', 32);
const iv = crypto.randomBytes(16);
function encrypt(text) {
let cipher = crypto.createCipheriv(algorithm, key, iv);
let encrypted = cipher.update(text, 'utf8', 'hex');
encrypted += cipher.final('hex');
return { iv: iv.toString('hex'), encryptedData: encrypted };
}
Use Tokenization
Tokenization replaces sensitive data elements with non-sensitive equivalents, known as tokens, which have no exploitable value. This is particularly useful for protecting payment information and other sensitive data.
Regular Security Audits and Penetration Testing
Conducting regular security audits and penetration testing is like having regular check-ups for your API's health. These practices help identify vulnerabilities before attackers can exploit them, allowing you to fortify your defenses accordingly.
Monitor and Log API Activity
Keep a close eye on your API's activity by logging and monitoring requests and responses. This not only helps in debugging issues but also in detecting and responding to potential security threats in real time.
Stay Updated on Security Practices
The digital landscape is constantly evolving, and so are the threats. Staying informed about the latest security trends, vulnerabilities, and protective measures is crucial for maintaining robust API security.
Use Apidog to Ensure Web API Security
Apidog is a powerful tool for Web API security, offering a broad spectrum of authentication methods to meet diverse security needs. It supports Bearer Token for stateless authentication, Basic Auth for simple and fast implementations, and Digest Auth for enhanced security by hashing user credentials. For more complex access requirements, Apidog provides OAuth 1.0, along with Hawk Authentication for secure HTTP authentication without credential distribution. It also facilitates AWS Signature for secure requests to AWS services, NTLM Authentication for Windows-based domain authentication, and Akamai EdgeGrid for secure API calls to Akamai's platform. Leveraging Apidog enables developers to secure their APIs effectively, ensuring data protection and compliance with various authentication standards.
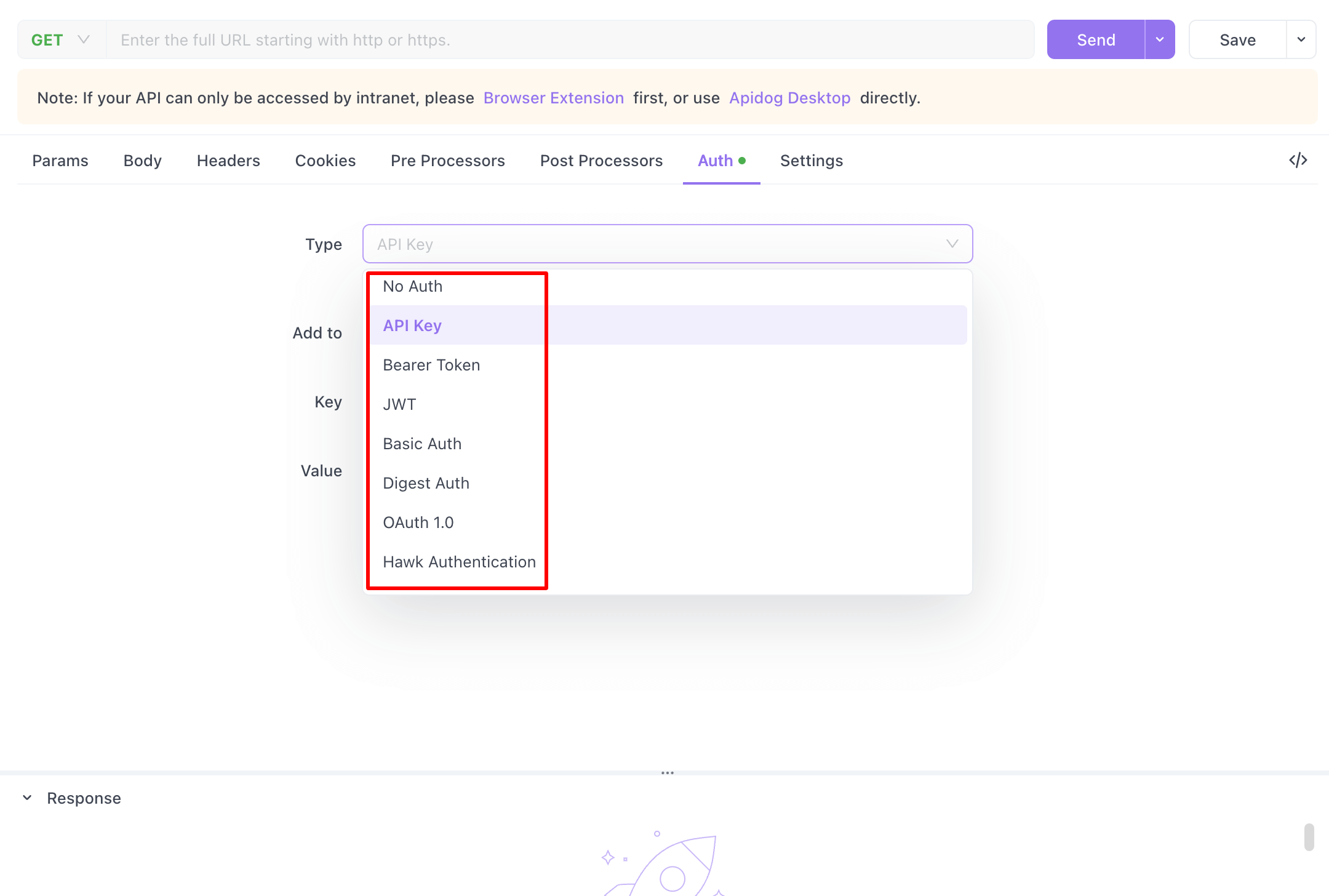
Conclusion
In a nutshell, Web API security is a critical component of modern web development, akin to the shields and armor in a knight's arsenal. By implementing these best practices, developers and organizations can significantly reduce their vulnerability to attacks, ensuring that their APIs—and the users who rely on them—remain secure. Remember, in the realm of digital security, vigilance, and proactive measures are your best allies.
What are some best practices for Web API security?
Key practices include using authentication and authorization mechanisms, securing data transmission with HTTPS, validating input to prevent injection attacks, implementing rate limiting and throttling, managing sensitive data carefully, conducting security audits, and monitoring API activity.
How does JWT work in Apidog?
In Apidog, JWT provides a secure and efficient way to manage user authentication and authorization, automatically generating tokens upon login and seamlessly integrating them into API requests for robust security.
How can I ensure my API's data transmission is secure?
Always use HTTPS to encrypt data in transit, ensuring that information exchanged between the client and server is protected against eavesdropping.
What is rate limiting and why is it important?
Rate limiting restricts the number of API requests a user can make within a specific timeframe, crucial for preventing abuse and mitigating DDoS attacks.
What is the difference between encryption and tokenization?
Encryption converts data into a coded format to protect it from unauthorized access, while tokenization replaces sensitive data with non-sensitive tokens that have no exploitable value, offering an additional layer of security.
How often should security audits and penetration testing be conducted?
Regularly, at least annually, or following significant changes to the API or its environment, to identify and mitigate potential vulnerabilities.
Why is monitoring API activity important?
A9: Monitoring helps in detecting unusual patterns or potential security threats in real-time, allowing for quick response to protect the API.
How can I stay updated on Web API security practices?
Follow reputable cybersecurity news sources, participate in security forums, and attend related conferences or webinars to stay informed about the latest trends and best practices.