In software development, optimizing the performance and user experience of applications is crucial. One common challenge is handling frequent API calls, especially when users interact rapidly with the interface, such as typing in a search box. This can lead to performance issues and unnecessary server load. Debouncing is a powerful technique to address this problem. In this article, we'll explore what debouncing is, why it's important, and how to implement it in a React application.
What is Debouncing?
Debouncing is a programming practice used to limit the rate at which a function is executed. It ensures that a function is only called once within a specified period of time, regardless of how many times it was triggered. This is particularly useful in scenarios where an action can be performed multiple times in quick succession, such as user input events.
For instance, when a user types in a search box, debouncing can help ensure that an API call is only made after the user has stopped typing for a short period, rather than on every keystroke.
Why Use Debouncing?
Debouncing offers several benefits:
- Performance Improvement: Reduces the number of unnecessary API calls, thereby improving the application's performance.
- Server Load Reduction: Minimizes the load on the server by preventing excessive requests.
- Enhanced User Experience: This creates a smoother experience for the user, as the application responds more predictably and efficiently.
Let's delve deeper into each of these benefits.
Performance Improvement
When a user interacts with an application, especially in real-time scenarios, the application can quickly become overwhelmed with tasks. For instance, without debouncing, each keystroke in a search bar might trigger an API call. If the user types quickly, this can result in a flurry of requests being sent to the server. This not only slows down the application but can also make it unresponsive.
By implementing debouncing, you ensure that the application waits for a pause in the user’s activity before making the API call. This significantly reduces the number of calls and allows the application to perform more efficiently. The user perceives the application as faster and more responsive.
Server Load Reduction
Every API call consumes server resources. When multiple unnecessary API calls are made, it can lead to increased server load, which might affect not only the performance of the current application but also other applications relying on the same server. High server load can result in slower response times, server crashes, or even increased costs if the server is scaled based on usage.
Debouncing helps mitigate this by ensuring that only necessary API calls are made. This leads to more efficient use of server resources, reduced operational costs, and better overall performance.
Enhanced User Experience
Users today expect applications to be fast and responsive. An application that lags or behaves unpredictably can lead to frustration and a poor user experience. Debouncing helps create a smoother user experience by reducing lag and making the application behave more predictably. When users type in a search box, they see the results after a brief pause, which feels natural and intuitive.
Implementing Debouncing in React
Let's dive into how you can implement debouncing in a React application. We'll start with a basic example of a search component that makes API calls.
Step 1: Set Up a React Application
First, ensure you have a React application set up. If not, you can create one using Create React App:
npx create-react-app debounce-example
cd debounce-example
npm start
Step 2: Create a Search Component
Create a new component called SearchComponent.js
:
import React, { useState, useEffect, useCallback } from 'react';
const SearchComponent = () => {
const [query, setQuery] = useState('');
const [results, setResults] = useState([]);
const fetchResults = async (searchQuery) => {
if (searchQuery) {
const response = await fetch(`https://api.example.com/search?q=${searchQuery}`);
const data = await response.json();
setResults(data.results);
}
};
const handleChange = (e) => {
setQuery(e.target.value);
};
useEffect(() => {
fetchResults(query);
}, [query]);
return (
<div>
<input type="text" value={query} onChange={handleChange} placeholder="Search..." />
<ul>
{results.map((result, index) => (
<li key={index}>{result.name}</li>
))}
</ul>
</div>
);
};
export default SearchComponent;
In this basic implementation, the fetchResults
function is called on every change to the input field, which can lead to excessive API calls.
Step 3: Implement Debouncing
To debounce the API calls, we'll use a custom hook. This hook will delay the execution of the fetchResults
function until the user stops typing for a specified duration.
Create a new file called useDebounce.js
:
import { useState, useEffect } from 'react';
const useDebounce = (value, delay) => {
const [debouncedValue, setDebouncedValue] = useState(value);
useEffect(() => {
const handler = setTimeout(() => {
setDebouncedValue(value);
}, delay);
return () => {
clearTimeout(handler);
};
}, [value, delay]);
return debouncedValue;
};
export default useDebounce;
This custom hook accepts a value and a delay, and returns the debounced value. It uses setTimeout
to update the debounced value only after the specified delay.
Step 4: Integrate the Debounce Hook
Update the SearchComponent
to use the useDebounce
hook:
import React, { useState, useEffect, useCallback } from 'react';
import useDebounce from './useDebounce';
const SearchComponent = () => {
const [query, setQuery] = useState('');
const [results, setResults] = useState([]);
const debouncedQuery = useDebounce(query, 500);
const fetchResults = useCallback(async (searchQuery) => {
if (searchQuery) {
const response = await fetch(`https://api.example.com/search?q=${searchQuery}`);
const data = await response.json();
setResults(data.results);
}
}, []);
useEffect(() => {
fetchResults(debouncedQuery);
}, [debouncedQuery, fetchResults]);
const handleChange = (e) => {
setQuery(e.target.value);
};
return (
<div>
<input type="text" value={query} onChange={handleChange} placeholder="Search..." />
<ul>
{results.map((result, index) => (
<li key={index}>{result.name}</li>
))}
</ul>
</div>
);
};
export default SearchComponent;
In this updated component, the debouncedQuery
is derived using the useDebounce
hook. The fetchResults
function is now only called when the debouncedQuery
changes, effectively debouncing the API calls.
Advanced Debouncing Techniques
While the above implementation is sufficient for many cases, there are scenarios where more advanced debouncing techniques are beneficial.
Immediate Execution
In some cases, you might want the function to execute immediately on the first trigger and debounce subsequent calls. This can be achieved with minor adjustments to the custom hook.
Modify the useDebounce
hook to execute the function immediately on the first call:
import { useState, useEffect, useRef } from 'react';
const useDebounce = (value, delay) => {
const [debouncedValue, setDebouncedValue] = useState(value);
const firstCall = useRef(true);
useEffect(() => {
if (firstCall.current) {
firstCall.current = false;
setDebouncedValue(value);
return;
}
const handler = setTimeout(() => {
setDebouncedValue(value);
}, delay);
return () => {
clearTimeout(handler);
};
}, [value, delay]);
return debouncedValue;
};
export default useDebounce;
With this modification, the function will execute immediately on the first call and debounce subsequent calls.
Canceling Debounced Calls
There might be situations where you need to cancel a debounced function call. For example, if the component unmounts before the debounce delay is over, you might want to cancel the pending API call.
To achieve this, you can extend the useDebounce
hook to return a function for canceling the debounced call:
import { useState, useEffect, useRef } from 'react';
const useDebounce = (value, delay) => {
const [debouncedValue, setDebouncedValue] = useState(value);
const timeoutRef = useRef(null);
const cancel = () => {
if (timeoutRef.current) {
clearTimeout(timeoutRef.current);
}
};
useEffect(() => {
timeoutRef.current = setTimeout(() => {
setDebouncedValue(value);
}, delay);
return () => {
cancel();
};
}, [value, delay]);
return [debouncedValue, cancel];
};
export default useDebounce;
In this version, the useDebounce
hook returns both the debounced value and a cancel function. The cancel function clears the timeout, effectively canceling the debounced call
Example Usage
Here's how you can use the extended useDebounce
hook in your SearchComponent
:
import React, { useState, useEffect, useCallback } from 'react';
import useDebounce from './useDebounce';
const SearchComponent = () => {
const [query, setQuery] = useState('');
const [results, setResults] = useState([]);
const [debouncedQuery, cancelDebounce] = useDebounce(query, 500);
const fetchResults = useCallback(async (searchQuery) => {
if (searchQuery) {
const response = await fetch(`https://api.example.com/search?q=${searchQuery}`);
const data = await response.json();
setResults(data.results);
}
}, []);
useEffect(() => {
fetchResults(debouncedQuery);
return () => {
cancelDebounce();
};
}, [debouncedQuery, fetchResults, cancelDebounce]);
const handleChange = (e) => {
setQuery(e.target.value);
};
return (
<div>
<input type="text" value={query} onChange={handleChange} placeholder="Search..." />
<ul>
{results.map((result, index) => (
<li key={index}>{result.name}</li>
))}
</ul>
</div>
);
};
export default SearchComponent;
In this implementation, the debounced call is canceled when the component unmounts, ensuring no unnecessary API calls are made.
Best Practices for Debouncing in Javascript/React
To make the most out of debouncing in your React applications, consider the following best practices:
Choose an Appropriate Delay: The delay duration is critical. It should balance responsiveness and performance. A delay that's too short may not effectively debounce, while a delay that's too long might make the application feel sluggish.
Use Callbacks Wisely: When using debounced functions, it's essential to ensure that the function references remain stable. Use useCallback
to memoize functions that are passed as dependencies to hooks.
Test Thoroughly: Test the debouncing behavior under different conditions. Ensure that the application behaves as expected when users interact with it at different speeds.
Optimize for Performance: While debouncing helps reduce unnecessary calls, it's also important to optimize the debounced function itself. Ensure that the function performs efficiently and avoids unnecessary computations.
Handle Errors Gracefully: When making API calls, always handle potential errors gracefully. This includes network errors, server errors, and invalid responses. Provide appropriate feedback to the user.
Working With Apidog
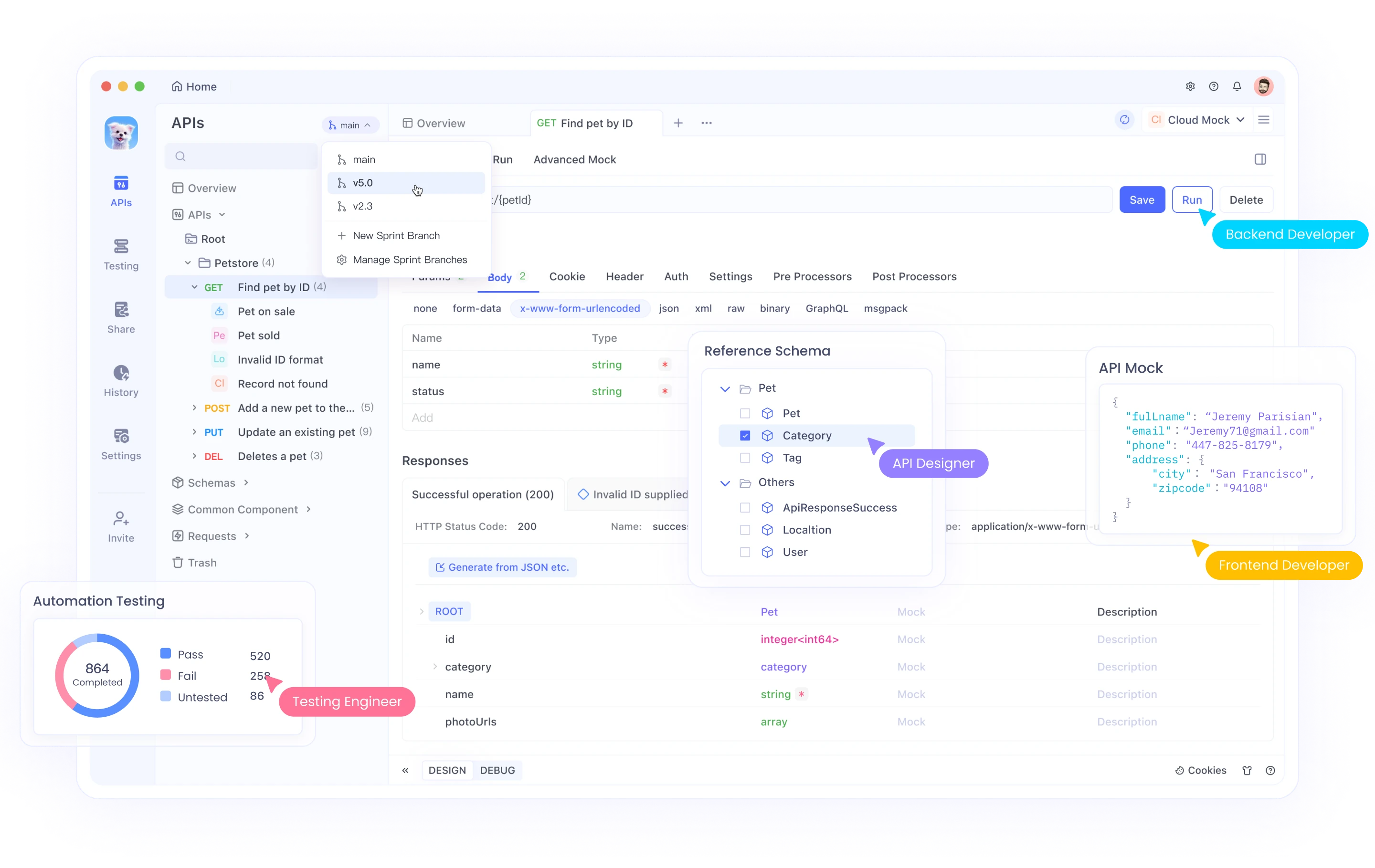
Apidog enhances API security by offering robust documentation, automated testing, and real-time monitoring. Apidog also aids in compliance with industry standards like GDPR and HIPAA, ensuring your APIs protect user data effectively.
Additionally, Apidog supports team collaboration, fostering a security-focused development environment. By integrating Apidog, you can build secure, reliable, and compliant APIs, protecting your data and users from various security threats.
Conclusion
Debouncing is an essential technique for optimizing performance and enhancing user experience in web applications. By implementing debouncing for API calls in React, you can significantly reduce unnecessary server load and improve the responsiveness of your application. The custom useDebounce
hook provides a flexible and reusable solution for debouncing any value or function in your React components.
By understanding and applying debouncing, you can create more efficient and user-friendly applications, ensuring a smoother experience for your users. Whether you’re working on a simple search component or a complex form, debouncing is a valuable tool in your development toolkit.
Happy coding!