Introduction
TypeScript enhances the development experience by adding static types to JavaScript, catching potential errors during development rather than at runtime. This is particularly valuable for API development, where maintaining data integrity and contract consistency is crucial. By the end of this tutorial, you'll have a well-structured, type-safe REST API ready for real-world use.
REST API Architecture, Explained
Before diving into implementation, let's clarify what makes an API truly RESTful:
Core REST Principles
- Statelessness: Each request from a client contains all information needed to process it. The server doesn't store client context between requests.
- Resource-Based: Resources are identified by URLs, and operations are performed using standard HTTP methods.
- Representation: Resources can have multiple representations (JSON, XML, etc.) that clients can request.
- Standard HTTP Methods: REST uses methods like GET, POST, PUT, DELETE, and PATCH to perform CRUD operations:
- GET: Retrieve resources
- POST: Create new resources
- PUT: Update existing resources (full update)
- PATCH: Partially update resources
- DELETE: Remove resources
Uniform Interface: Consistent resource identification and manipulation through URLs and HTTP methods.
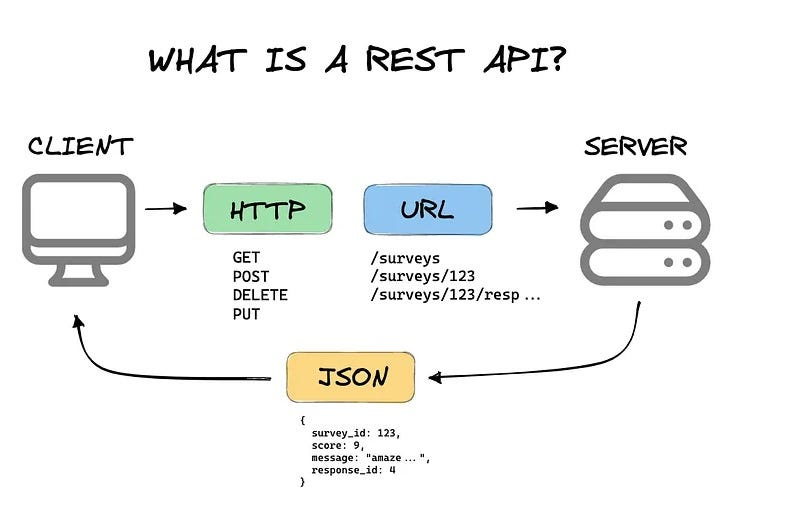
Why Use TypeScript for REST APIs?
TypeScript offers several advantages for API development:
- Static Type Checking: Catches type-related errors during development
- Enhanced IDE Support: Provides better autocompletion and documentation
- Interface Definitions: Allows clear contracts between client and server
- Code Organization: Facilitates better architecture through modules and decorators
- Easier Refactoring: Type safety makes large-scale changes safer
- Self-Documenting Code: Types serve as documentation for data structures
Here's a more contextual rewrite of the API testing section that flows better with your comprehensive TypeScript REST API tutorial:
Why You Should Test Your REST API with Apidog
As you develop your TypeScript REST API, Apidog provides a superior testing experience compared to Postman by deeply integrating with your development workflow. The tool's schema-first approach perfectly complements TypeScript's type safety, allowing you to:
Validate responses against your TypeScript interfaces
Apidog automatically checks that API responses match your defined schemas, mirroring TypeScript's compile-time type checking at the API level. This catches data type mismatches (like when a numeric ID is returned as a string) that could otherwise cause runtime errors in your TypeScript client code.
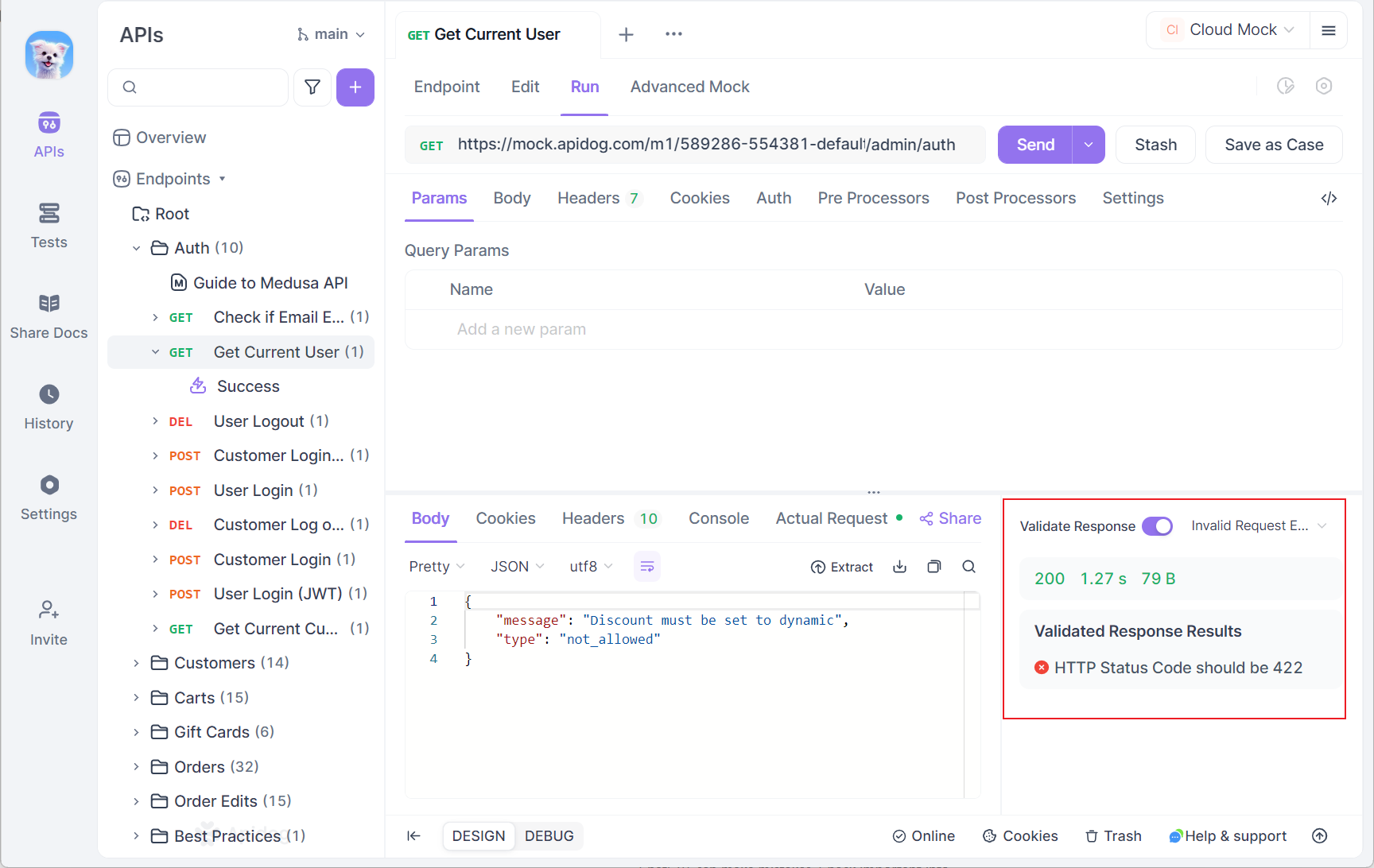
Generate realistic test data
Based on your TypeScript model definitions (like the Book
interface), Apidog can automatically create test cases with properly typed mock data, saving hours of manual test setup while ensuring your tests reflect real-world usage patterns.
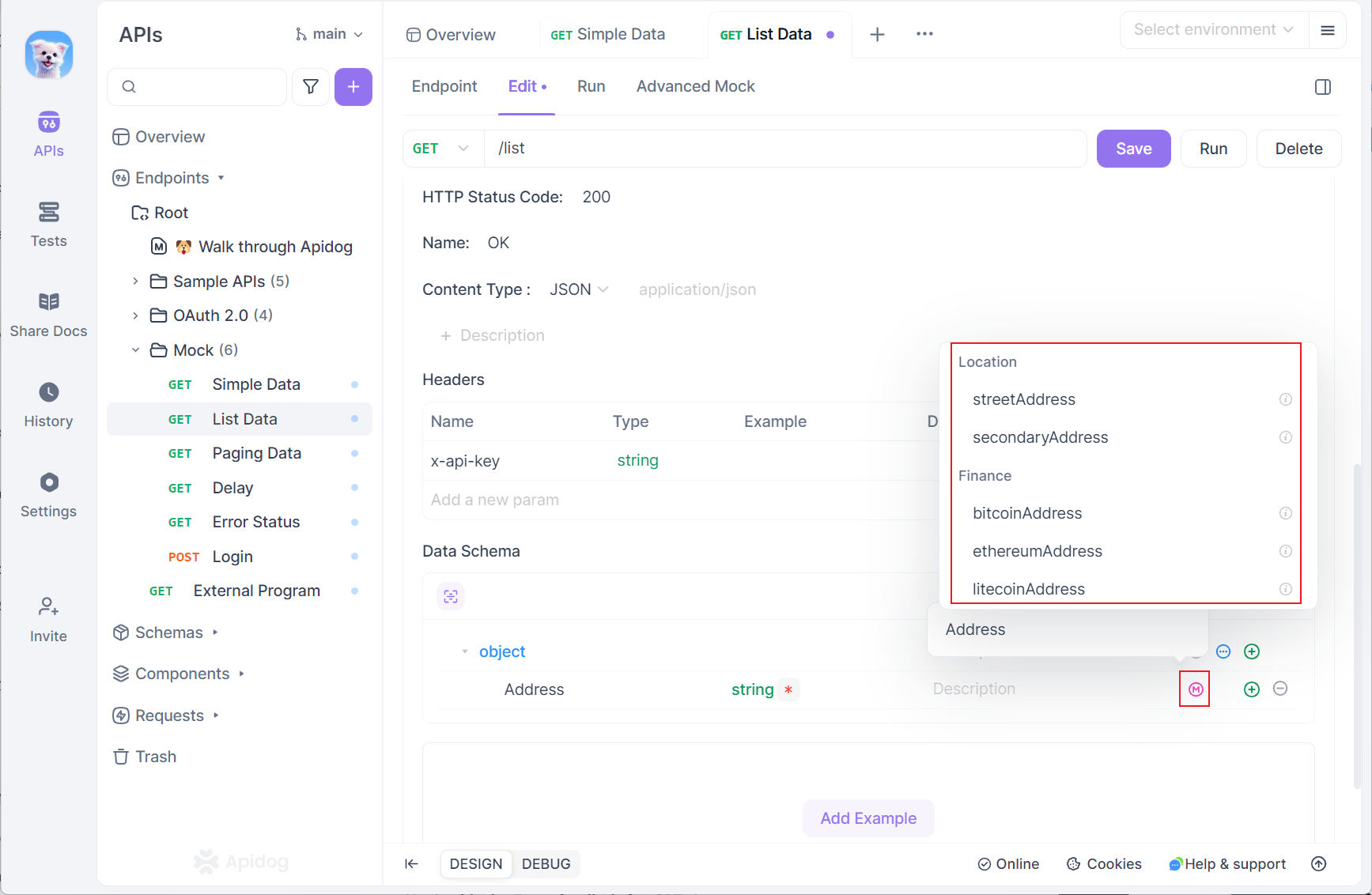
Maintain consistency across environments
The environment management system lets you define typed variables (like API base URLs) that work across development, staging, and production - similar to how TypeScript's config.ts
manages environment variables in your codebase.
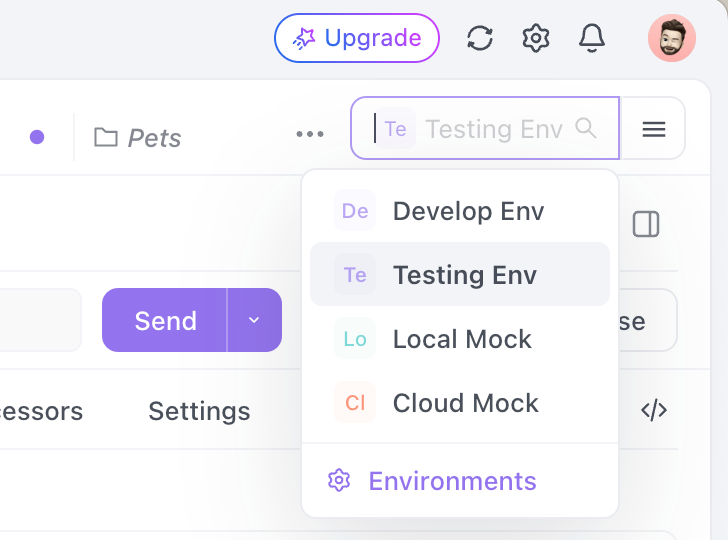
Automate complex test scenarios
Apidog's pre-processor scripts enable sophisticated test orchestration, allowing you to:
- Chain API calls while maintaining type safety
- Transform response data between requests
- Implement conditional test logic
All while leveraging TypeScript-like type hints and autocompletion.
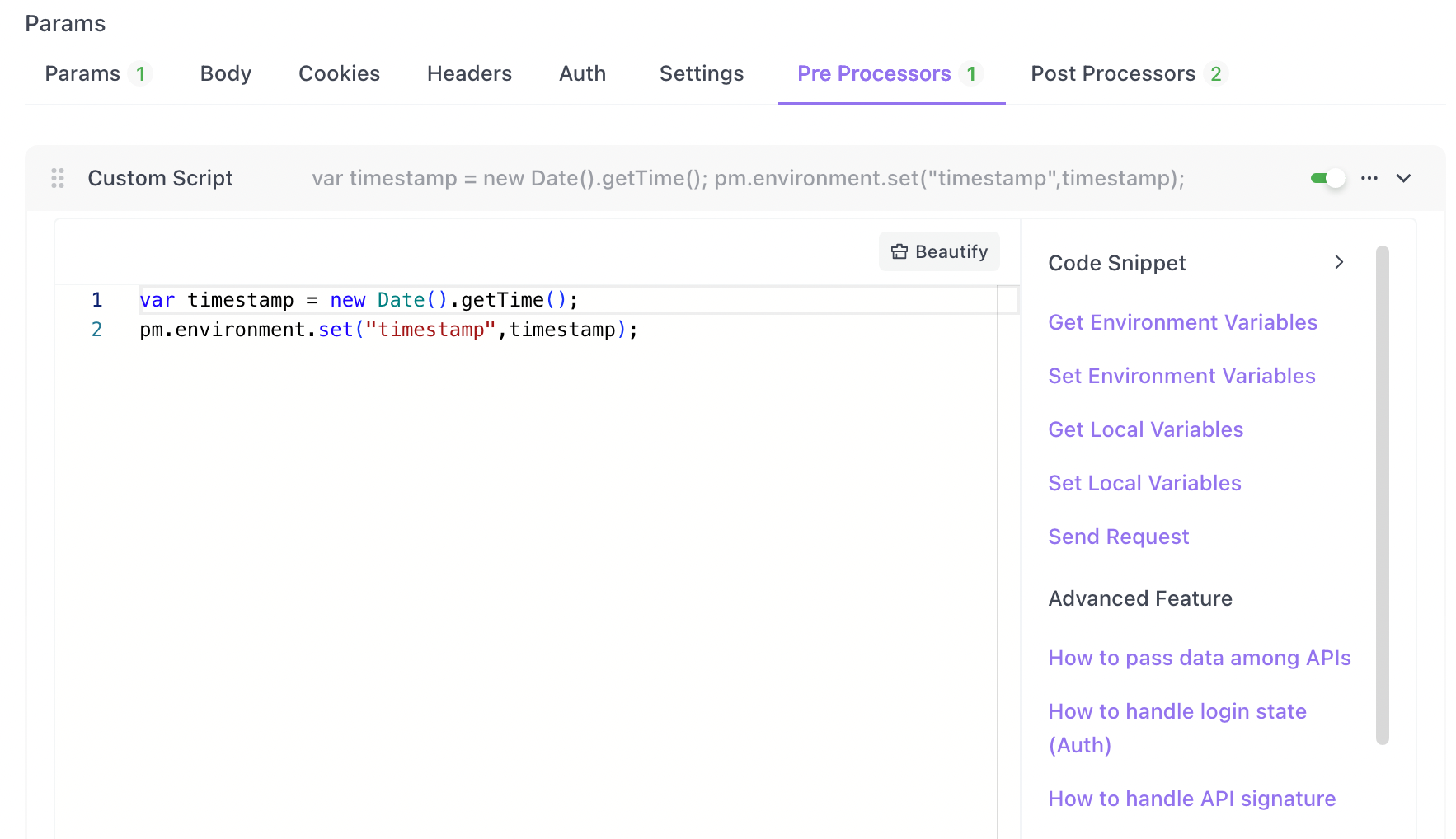
Integrated documentation
The automatically generated API documentation stays perfectly synchronized with your tests, eliminating the documentation drift that often plagues API projects. This is particularly valuable when working with TypeScript, as it ensures your runtime behavior matches your type definitions.
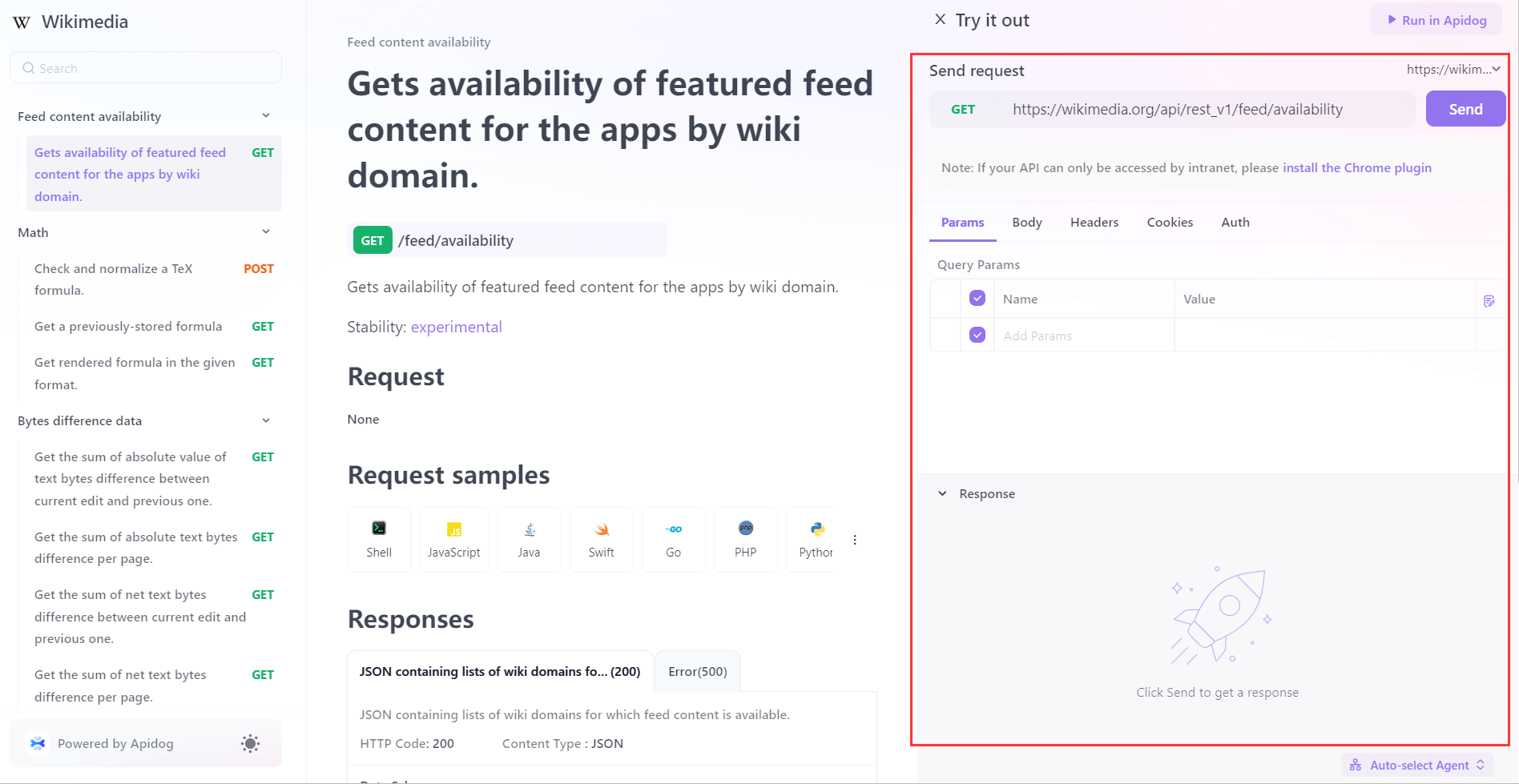
For teams building TypeScript APIs, Apidog's tight integration between design, testing, and documentation creates a more efficient workflow than Postman's fragmented approach. The ability to validate responses against TypeScript interfaces and generate type-safe test cases makes it an ideal companion for your type-driven development process.
Prerequisites
To follow this tutorial, you'll need:
- Node.js (v14+ recommended) installed
- A text editor or IDE (VS Code recommended for its TypeScript support)
- Basic knowledge of JavaScript and Node.js
- Familiarity with HTTP and REST concepts
- Command line/terminal access
Step 1: Project Setup and Initialization
Let's begin by creating a structured project environment:
# Create project directory
mkdir typescript-rest-api
cd typescript-rest-api
# Initialize package.json
npm init -y
Understanding package.json
The package.json
file is the heart of any Node.js project. It contains metadata about your project and manages dependencies. The -y
flag creates a default configuration that we'll customize shortly.
Step 2: Install Dependencies
Let's install the necessary packages for our TypeScript REST API:
# Install TypeScript and development tools
npm install typescript ts-node nodemon @types/node --save-dev
# Install Express and type definitions
npm install express
npm install @types/express --save-dev
# Install middleware for logging and parsing
npm install morgan cors helmet
npm install @types/morgan @types/cors --save-dev
# Install dotenv for environment variable management
npm install dotenv
Dependency Breakdown:
- typescript: The TypeScript compiler
- ts-node: Allows running TypeScript files directly
- nodemon: Monitors file changes and restarts the server
- express: Web framework for handling HTTP requests
- morgan: HTTP request logger middleware
- cors: Middleware for handling Cross-Origin Resource Sharing
- helmet: Security middleware that sets various HTTP headers
- dotenv: Loads environment variables from a .env file
Type definition packages (@types/*
) provide TypeScript type information for libraries written in JavaScript.
Step 3: Configure TypeScript
Initialize TypeScript configuration:
npx tsc --init
This creates a tsconfig.json
file with default settings. Let's customize it for our REST API:
{
"compilerOptions": {
"target": "ES6",
"module": "commonjs",
"outDir": "./dist",
"rootDir": "./src",
"strict": true,
"esModuleInterop": true,
"forceConsistentCasingInFileNames": true,
"skipLibCheck": true,
"sourceMap": true,
"resolveJsonModule": true
},
"include": ["src/**/*"],
"exclude": ["node_modules", "**/*.test.ts"]
}
Configuration Explanation:
- target: Specifies the ECMAScript target version (ES6 offers modern features)
- module: Defines the module system (CommonJS works well with Node.js)
- outDir: Where compiled JavaScript files will be placed
- rootDir: Source TypeScript files location
- strict: Enables all strict type checking options
- esModuleInterop: Allows default imports from modules with no default export
- sourceMap: Generates source map files for debugging
- resolveJsonModule: Allows importing JSON files as modules
Step 4: Create Environment Configuration
Create a .env
file in the root directory to store environment variables:
NODE_ENV=development
PORT=3000
API_VERSION=v1
Now create a .env.example
file with the same structure but without sensitive values. This will serve as a template for other developers:
NODE_ENV=development
PORT=3000
API_VERSION=v1
Add .env
to your .gitignore
file to prevent committing sensitive information:
node_modules
dist
.env
Step 5: Update Package.json Scripts
Enhance your package.json
with useful scripts:
"main": "dist/server.js",
"scripts": {
"start": "node dist/server.js",
"dev": "nodemon src/server.ts",
"build": "tsc",
"lint": "eslint . --ext .ts",
"test": "jest",
"clean": "rimraf dist"
}
These scripts provide commands for development, production builds, linting, testing, and cleaning compiled files.
Step 6: Create Project Structure
Create a well-organized directory structure:
typescript-rest-api/
├── src/
│ ├── config/ # Configuration files
│ │ └── index.ts # Central config exports
│ ├── controllers/ # Route handlers
│ ├── middleware/ # Custom middleware
│ ├── models/ # Data models/interfaces
│ ├── routes/ # Route definitions
│ │ └── v1/ # API version 1 routes
│ ├── services/ # Business logic
│ ├── utils/ # Utility functions
│ ├── types/ # Type definitions
│ ├── app.ts # Express application setup
│ └── server.ts # Server entry point
├── .env # Environment variables (gitignored)
├── .env.example # Example environment variables
├── .gitignore # Git ignore file
├── package.json # Project metadata and dependencies
└── tsconfig.json # TypeScript configuration
This structure follows the separation of concerns principle, making the codebase maintainable as it grows.
Step 7: Set Up Configuration
Create the configuration module in src/config/index.ts
:
import dotenv from 'dotenv';
import path from 'path';
// Load environment variables from .env file
dotenv.config({ path: path.resolve(__dirname, '../../.env') });
const config = {
env: process.env.NODE_ENV || 'development',
port: parseInt(process.env.PORT || '3000', 10),
apiVersion: process.env.API_VERSION || 'v1',
// Add other configuration variables as needed
};
export default config;
Step 8: Create the Express Application
First, let's create src/app.ts
to set up our Express application:
import express, { Express } from 'express';
import morgan from 'morgan';
import helmet from 'helmet';
import cors from 'cors';
import config from './config';
import routes from './routes';
import { errorHandler, notFoundHandler } from './middleware/error.middleware';
const app: Express = express();
// Middleware
app.use(helmet()); // Security headers
app.use(cors()); // Enable CORS
app.use(morgan(config.env === 'development' ? 'dev' : 'combined')); // Request logging
app.use(express.json()); // Parse JSON bodies
app.use(express.urlencoded({ extended: true })); // Parse URL-encoded bodies
// API Routes
app.use(`/api/${config.apiVersion}`, routes);
// Health check endpoint
app.get('/health', (_req, res) => {
res.status(200).json({ status: 'OK', timestamp: new Date() });
});
// Error Handling
app.use(notFoundHandler);
app.use(errorHandler);
export default app;
Next, create src/server.ts
as the entry point:
import app from './app';
import config from './config';
const startServer = () => {
try {
app.listen(config.port, () => {
console.log(`Server running on port ${config.port} in ${config.env} mode`);
console.log(`API available at <http://localhost>:${config.port}/api/${config.apiVersion}`);
console.log(`Health check available at <http://localhost>:${config.port}/health`);
});
} catch (error) {
console.error('Failed to start server:', error);
process.exit(1);
}
};
// Handle unhandled promise rejections
process.on('unhandledRejection', (reason, promise) => {
console.error('Unhandled Rejection at:', promise, 'reason:', reason);
});
startServer();
Step 9: Define Data Models and Types
Create base interfaces for your data in src/models/book.model.ts
:
export interface Book {
id: number;
title: string;
author: string;
publishYear?: number;
genre?: string;
isbn?: string;
createdAt: Date;
updatedAt: Date;
}
// DTO (Data Transfer Object) for creating new books
export interface CreateBookDTO {
title: string;
author: string;
publishYear?: number;
genre?: string;
isbn?: string;
}
// DTO for updating existing books (all fields optional)
export interface UpdateBookDTO {
title?: string;
author?: string;
publishYear?: number;
genre?: string;
isbn?: string;
}
// Response structure for API consistency
export interface BookResponse {
data: Book | Book[] | null;
message?: string;
success: boolean;
}
Step 10: Create Service Layer
The service layer contains business logic, separated from controllers. Create src/services/book.service.ts
:
import { Book, CreateBookDTO, UpdateBookDTO } from '../models/book.model';
// In-memory database (in a real app, you'd use a database)
let books: Book[] = [
{ id: 1, title: '1984', author: 'George Orwell', publishYear: 1949 },
{ id: 2, title: 'To Kill a Mockingbird', author: 'Harper Lee', publishYear: 1960 }
];
export class BookService {
async getAllBooks(): Promise<Book[]> {
return books;
}
async getBookById(id: number): Promise<Book | null> {
const book = books.find(b => b.id === id);
return book || null;
}
async createBook(bookData: CreateBookDTO): Promise<Book> {
const newId = books.length > 0 ? Math.max(...books.map(b => b.id)) + 1 : 1;
const newBook: Book = {
id: newId,
...bookData
};
books.push(newBook);
return newBook;
}
async updateBook(id: number, bookData: UpdateBookDTO): Promise<Book | null> {
const index = books.findIndex(b => b.id === id);
if (index === -1) {
return null;
}
books[index] = { ...books[index], ...bookData };
return books[index];
}
async deleteBook(id: number): Promise<boolean> {
const initialLength = books.length;
books = books.filter(b => b.id !== id);
return books.length !== initialLength;
}
}
export default new BookService();
Step 11: Refactor Controllers to Use Service Layer
Update the src/controllers/book.controller.ts
to use our new service layer:
import { Request, Response } from 'express';
import bookService from '../services/book.service';
export const getBooks = async (_req: Request, res: Response) => {
try {
const books = await bookService.getAllBooks();
return res.status(200).json(books);
} catch (error) {
return res.status(500).json({ message: 'Error retrieving books', error });
}
};
export const getBookById = async (req: Request, res: Response) => {
try {
const id: number = parseInt(req.params.id);
const book = await bookService.getBookById(id);
if (!book) {
return res.status(404).json({ message: 'Book not found' });
}
return res.status(200).json(book);
} catch (error) {
return res.status(500).json({ message: 'Error retrieving book', error });
}
};
export const createBook = async (req: Request, res: Response) => {
try {
const bookData = req.body;
// Validate request
if (!bookData.title || !bookData.author) {
return res.status(400).json({ message: 'Title and author are required' });
}
const newBook = await bookService.createBook(bookData);
return res.status(201).json(newBook);
} catch (error) {
return res.status(500).json({ message: 'Error creating book', error });
}
};
export const updateBook = async (req: Request, res: Response) => {
try {
const id: number = parseInt(req.params.id);
const bookData = req.body;
const updatedBook = await bookService.updateBook(id, bookData);
if (!updatedBook) {
return res.status(404).json({ message: 'Book not found' });
}
return res.status(200).json(updatedBook);
} catch (error) {
return res.status(500).json({ message: 'Error updating book', error });
}
};
export const deleteBook = async (req: Request, res: Response) => {
try {
const id: number = parseInt(req.params.id);
const success = await bookService.deleteBook(id);
if (!success) {
return res.status(404).json({ message: 'Book not found' });
}
return res.status(204).send();
} catch (error) {
return res.status(500).json({ message: 'Error deleting book', error });
}
};
Step 12: Add Request Validation
Create a validation middleware in src/middleware/validation.middleware.ts
:
import { Request, Response, NextFunction } from 'express';
export interface ValidationSchema {
[key: string]: {
required?: boolean;
type?: 'string' | 'number' | 'boolean';
minLength?: number;
maxLength?: number;
min?: number;
max?: number;
custom?: (value: any) => boolean;
message?: string;
};
}
export const validateRequest = (schema: ValidationSchema) => {
return (req: Request, res: Response, next: NextFunction) => {
const errors: string[] = [];
Object.entries(schema).forEach(([field, rules]) => {
const value = req.body[field];
// Check required fields
if (rules.required && (value === undefined || value === null || value === '')) {
errors.push(rules.message || `Field '${field}' is required`);
return;
}
// Skip further validation if field is not present and not required
if ((value === undefined || value === null) && !rules.required) {
return;
}
// Type validation
if (rules.type && typeof value !== rules.type) {
errors.push(rules.message || `Field '${field}' must be a ${rules.type}`);
}
// String validations
if (rules.type === 'string') {
if (rules.minLength !== undefined && value.length < rules.minLength) {
errors.push(rules.message || `Field '${field}' must be at least ${rules.minLength} characters long`);
}
if (rules.maxLength !== undefined && value.length > rules.maxLength) {
errors.push(rules.message || `Field '${field}' must not exceed ${rules.maxLength} characters`);
}
}
// Number validations
if (rules.type === 'number') {
if (rules.min !== undefined && value < rules.min) {
errors.push(rules.message || `Field '${field}' must be at least ${rules.min}`);
}
if (rules.max !== undefined && value > rules.max) {
errors.push(rules.message || `Field '${field}' must not exceed ${rules.max}`);
}
}
// Custom validation
if (rules.custom && !rules.custom(value)) {
errors.push(rules.message || `Field '${field}' failed validation`);
}
});
if (errors.length > 0) {
return res.status(400).json({ errors });
}
next();
};
};
Then create validation schemas in src/validators/book.validator.ts
:
import { ValidationSchema } from '../middleware/validation.middleware';
export const createBookSchema: ValidationSchema = {
title: {
required: true,
type: 'string',
minLength: 1,
maxLength: 100,
message: 'Title is required and must be between 1 and 100 characters'
},
author: {
required: true,
type: 'string',
minLength: 1,
maxLength: 100,
message: 'Author is required and must be between 1 and 100 characters'
},
publishYear: {
type: 'number',
min: 0,
max: new Date().getFullYear(),
message: `Publish year must be between 0 and ${new Date().getFullYear()}`
}
};
export const updateBookSchema: ValidationSchema = {
title: {
type: 'string',
minLength: 1,
maxLength: 100,
message: 'Title must be between 1 and 100 characters'
},
author: {
type: 'string',
minLength: 1,
maxLength: 100,
message: 'Author must be between 1 and 100 characters'
},
publishYear: {
type: 'number',
min: 0,
max: new Date().getFullYear(),
message: `Publish year must be between 0 and ${new Date().getFullYear()}`
}
};
Step 13: Update Routes to Include Validation
Let's update src/routes/book.routes.ts
to include our validation middleware:
import { Router } from 'express';
import { getBooks, getBookById, createBook, updateBook, deleteBook } from '../controllers/book.controller';
import { validateRequest } from '../middleware/validation.middleware';
import { createBookSchema, updateBookSchema } from '../validators/book.validator';
const router = Router();
// GET /api/books - Get all books
router.get('/', getBooks);
// GET /api/books/:id - Get a specific book
router.get('/:id', getBookById);
// POST /api/books - Create a new book
router.post('/', validateRequest(createBookSchema), createBook);
// PUT /api/books/:id - Update a book
router.put('/:id', validateRequest(updateBookSchema), updateBook);
// DELETE /api/books/:id - Delete a book
router.delete('/:id', deleteBook);
export default router;
Step 14: Add API Documentation
Install Swagger for API documentation:
npm install swagger-ui-express @types/swagger-ui-express swagger-jsdoc @types/swagger-jsdoc --save-dev
Create src/utils/swagger.ts
:
import swaggerJsdoc from 'swagger-jsdoc';
import swaggerUi from 'swagger-ui-express';
const options = {
definition: {
openapi: '3.0.0',
info: {
title: 'TypeScript REST API',
version: '1.0.0',
description: 'A simple REST API built with Express and TypeScript',
},
servers: [
{
url: '<http://localhost:3000/api>',
},
],
},
apis: ['./src/routes/*.ts'],
};
const specs = swaggerJsdoc(options);
export { specs, swaggerUi };
Add JSDoc comments to your routes file for Swagger documentation:
/**
* @swagger
* components:
* schemas:
* Book:
* type: object
* required:
* - title
* - author
* properties:
* id:
* type: number
* description: The auto-generated id of the book
* title:
* type: string
* description: The title of the book
* author:
* type: string
* description: The book author
* publishYear:
* type: number
* description: The year the book was published
*/
/**
* @swagger
* tags:
* name: Books
* description: The books managing API
*/
/**
* @swagger
* /books:
* get:
* summary: Returns the list of all the books
* tags: [Books]
* responses:
* 200:
* description: The list of the books
* content:
* application/json:
* schema:
* type: array
* items:
* $ref: '#/components/schemas/Book'
*/
// Add similar JSDoc comments for other endpoints
Update server.ts
to include Swagger:
import { specs, swaggerUi } from './utils/swagger';
// Add before your routes
app.use('/api-docs', swaggerUi.serve, swaggerUi.setup(specs));
Step 15: Add CORS Support
Add CORS middleware to allow cross-origin requests:
npm install cors @types/cors --save-dev
Update server.ts
:
import cors from 'cors';
// Add this before other middleware
app.use(cors());
// For more control over CORS options:
app.use(cors({
origin: ['<http://localhost:3000>', '<https://yourdomain.com>'],
methods: ['GET', 'POST', 'PUT', 'DELETE'],
allowedHeaders: ['Content-Type', 'Authorization']
}));
Step 16: Add Environment Configuration
Create a .env
file for environment variables:
PORT=3000
NODE_ENV=development
# Add other config variables here, like database connection strings
Install dotenv to load environment variables:
npm install dotenv --save
Create src/config/env.config.ts
:
import dotenv from 'dotenv';
import path from 'path';
// Load environment variables from .env file
dotenv.config({ path: path.resolve(__dirname, '../../.env') });
export default {
port: parseInt(process.env.PORT || '3000'),
nodeEnv: process.env.NODE_ENV || 'development',
// Add other environment variables here
};
Update server.ts
to use this configuration:
import config from './config/env.config';
const PORT: number = config.port;
Step 17: Final Integration and Testing
With all components in place, your file structure should look like:
typescript-rest-api/
├── src/
│ ├── config/
│ │ └── env.config.ts
│ ├── controllers/
│ │ └── book.controller.ts
│ ├── middleware/
│ │ ├── error.middleware.ts
│ │ └── validation.middleware.ts
│ ├── models/
│ │ └── book.model.ts
│ ├── routes/
│ │ ├── book.routes.ts
│ │ └── index.ts
│ ├── services/
│ │ └── book.service.ts
│ ├── utils/
│ │ └── swagger.ts
│ ├── validators/
│ │ └── book.validator.ts
│ └── server.ts
├── .env
├── tsconfig.json
└── package.json
Run your API:
npm run dev
Test the endpoints using a tool like Postman or access the Swagger documentation at http://localhost:3000/api-docs
.
Conclusion
You've now completed building a robust REST API with TypeScript. This API demonstrates best practices including:
- Type safety through TypeScript interfaces and models
- Clean architecture with separation of concerns (controllers, services, models)
- Input validation to ensure data integrity
- Error handling for robust API responses
- API documentation with Swagger for better developer experience
- CORS support for cross-origin requests
- Environment configuration for different deployment scenarios
This foundation provides an excellent starting point for more complex applications. As your API grows, consider implementing:
- Database integration with TypeORM, Prisma, or Mongoose
- Authentication middleware with JWT or OAuth
- Rate limiting to prevent abuse
- Logging with Winston or other logging libraries
- Testing with Jest, Mocha, or SuperTest
- Containerization with Docker for consistent deployment
The combination of TypeScript's static typing and Express's flexible architecture results in a maintainable, scalable API that can evolve alongside your application's needs.