The Slack Model Context Protocol (MCP) Server provides a seamless way to integrate AI assistants like Claude into your Slack workspace, transforming them into active participants in your team's daily communications. Built with a robust TypeScript implementation, this server allows AI to post messages, retrieve user information, and interact with various workspace elements in real time. By bridging the gap between artificial intelligence and team collaboration, Slack MCP Server enhances productivity, streamlines workflows, and enables smarter, more efficient workplace interactions—all within the familiar Slack environment.
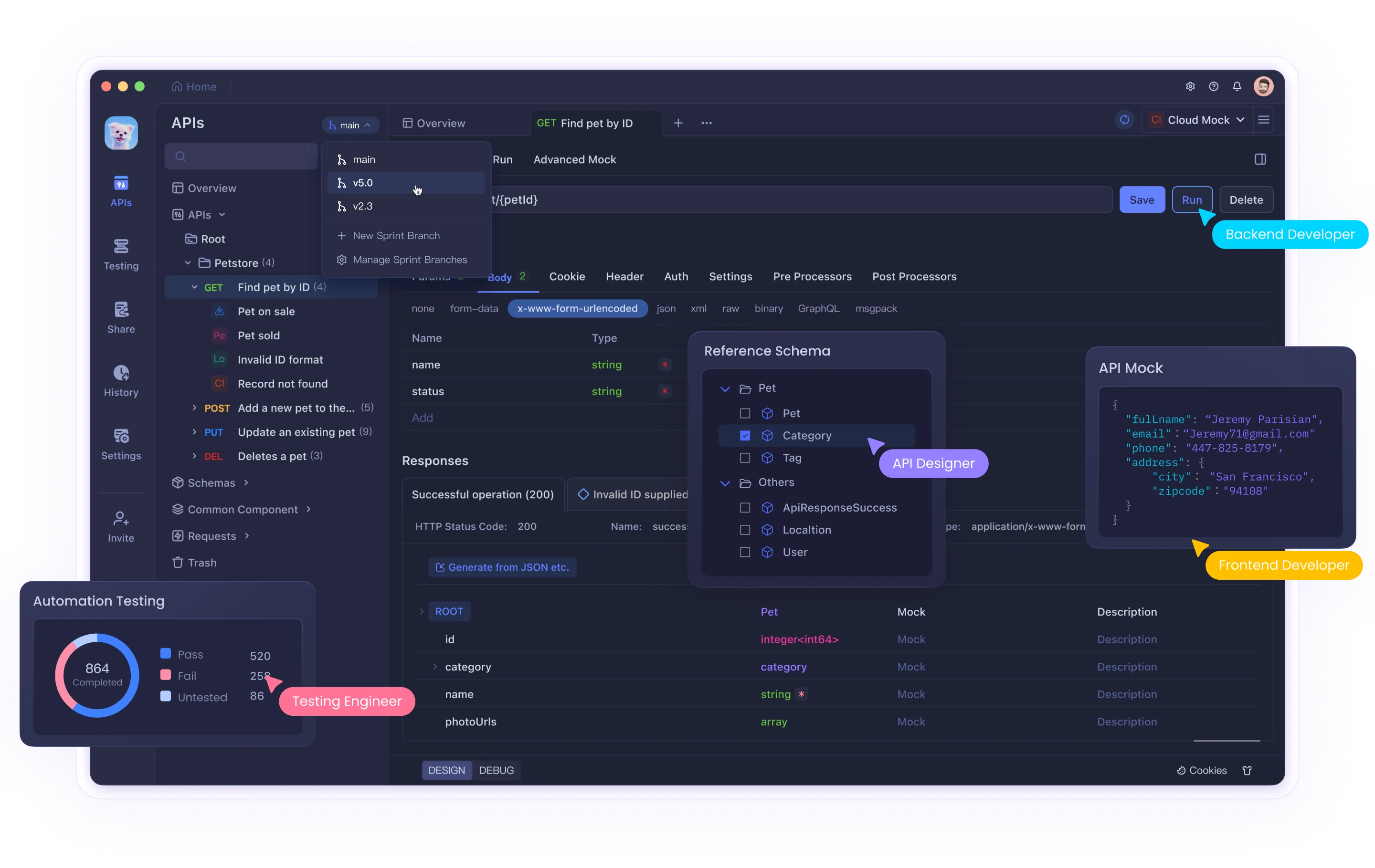
Understanding the Model Context Protocol
Before diving into the specifics of the Slack MCP Server, it's important to understand what the Model Context Protocol represents. MCP is a standardized interface that allows AI models to interact with external systems and services. It provides a structured way for AI assistants to access tools, retrieve information, and take actions beyond their training data. In the context of Slack integration, the MCP server functions as an interpreter between the AI assistant's requests and the Slack API's requirements, handling authentication, formatting, and response processing.
Comprehensive Feature Set
The Slack MCP Server implementation offers an extensive suite of capabilities that mirror the most essential functions of the native Slack interface:
Channel Management and Navigation
- Channel Listing: The server can retrieve a comprehensive list of all public channels in the workspace, including details such as member counts, topics, and creation dates. This allows AI assistants to understand the workspace structure and recommend appropriate channels for specific discussions.
- Channel History Access: AI assistants can retrieve recent message history from channels, providing context for ongoing conversations and enabling more relevant responses.
Robust Messaging Capabilities
- Message Posting: The server enables AI assistants to compose and send formatted messages to any channel where the Slack bot has been added, supporting Slack's markdown-like formatting options for rich text display.
- Threaded Replies: Beyond simple posting, the server supports replying to specific message threads, allowing AI assistants to maintain conversational context and participate in focused discussions.
- Scheduled Messages: For time-sensitive communications, the server can schedule messages to be delivered at specific times, supporting proactive reminders and announcements.
Enhanced User Engagement
- Emoji Reactions: The server allows AI assistants to add emoji reactions to messages, providing a lightweight way to acknowledge or respond to content without cluttering conversation threads.
- User Profile Retrieval: AI assistants can access detailed user profile information, including display names, email addresses (where permitted), time zones, and custom profile fields, enabling personalized interactions.
- Workspace Demographics: The ability to retrieve comprehensive user listings helps AI assistants understand team composition and tailor communications appropriately.
Technical Implementation
The Slack MCP Server is built on a modern stack designed for reliability and performance:
- TypeScript Foundation: The entire codebase leverages TypeScript for enhanced type safety and developer experience, reducing runtime errors and improving code maintainability.
- Asynchronous Architecture: The server implements asynchronous communication patterns to handle concurrent requests efficiently, preventing bottlenecks during high-volume interactions.
- Rate Limit Management: Built-in mechanisms respect Slack API rate limits, implementing intelligent retry strategies to ensure reliable operation even during intensive usage periods.
- Secure Token Handling: The implementation follows security best practices for managing authentication tokens, preventing exposure of sensitive credentials.
- Comprehensive Error Handling: Detailed error management provides actionable feedback when issues occur, simplifying troubleshooting and maintenance.
Detailed Installation Guide
Setting up the Slack MCP Server requires careful attention to both the Slack application configuration and the server deployment. This comprehensive guide walks through each step of the process:
Creating and Configuring Your Slack Application
Create a New Slack App:
- Navigate to the Slack API Applications page
- Click "Create New App" and select "From scratch"
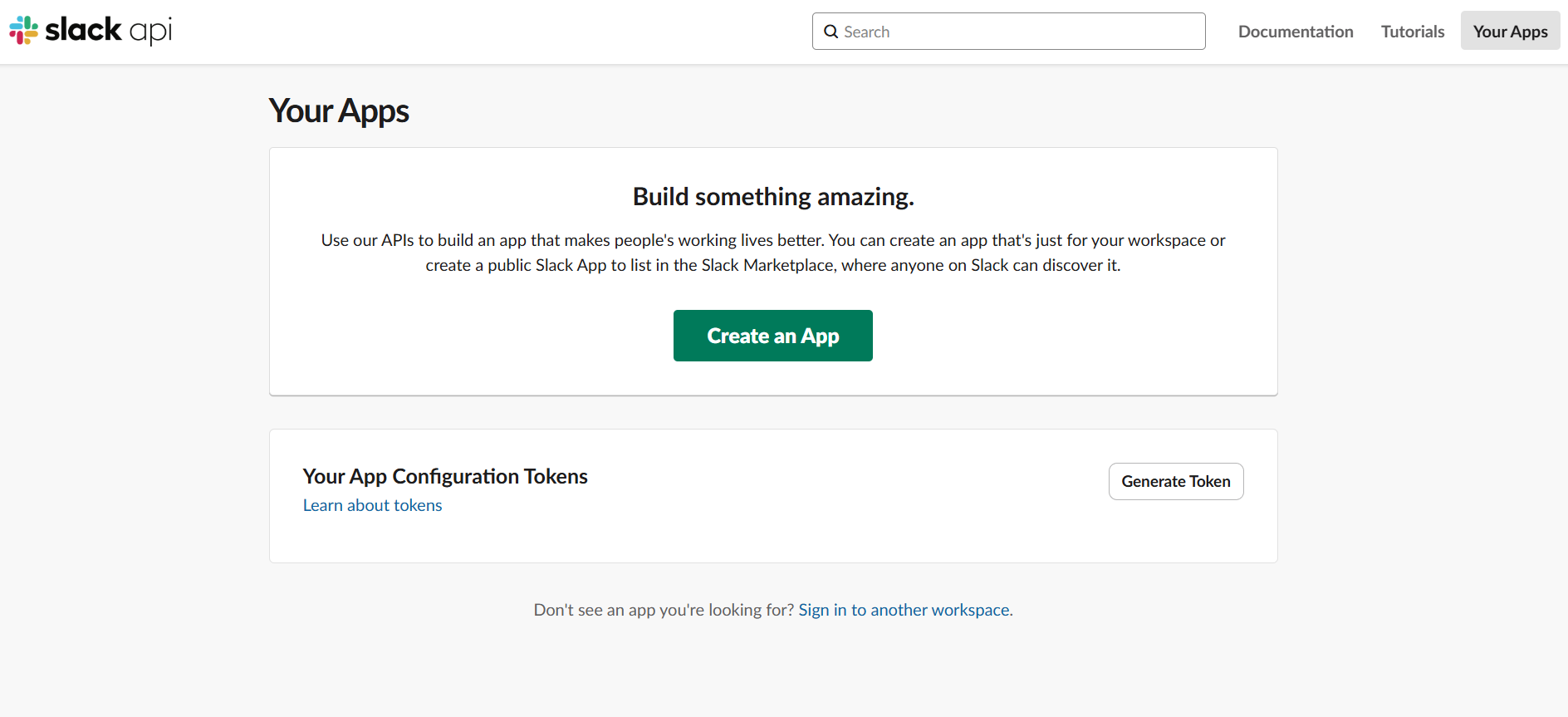
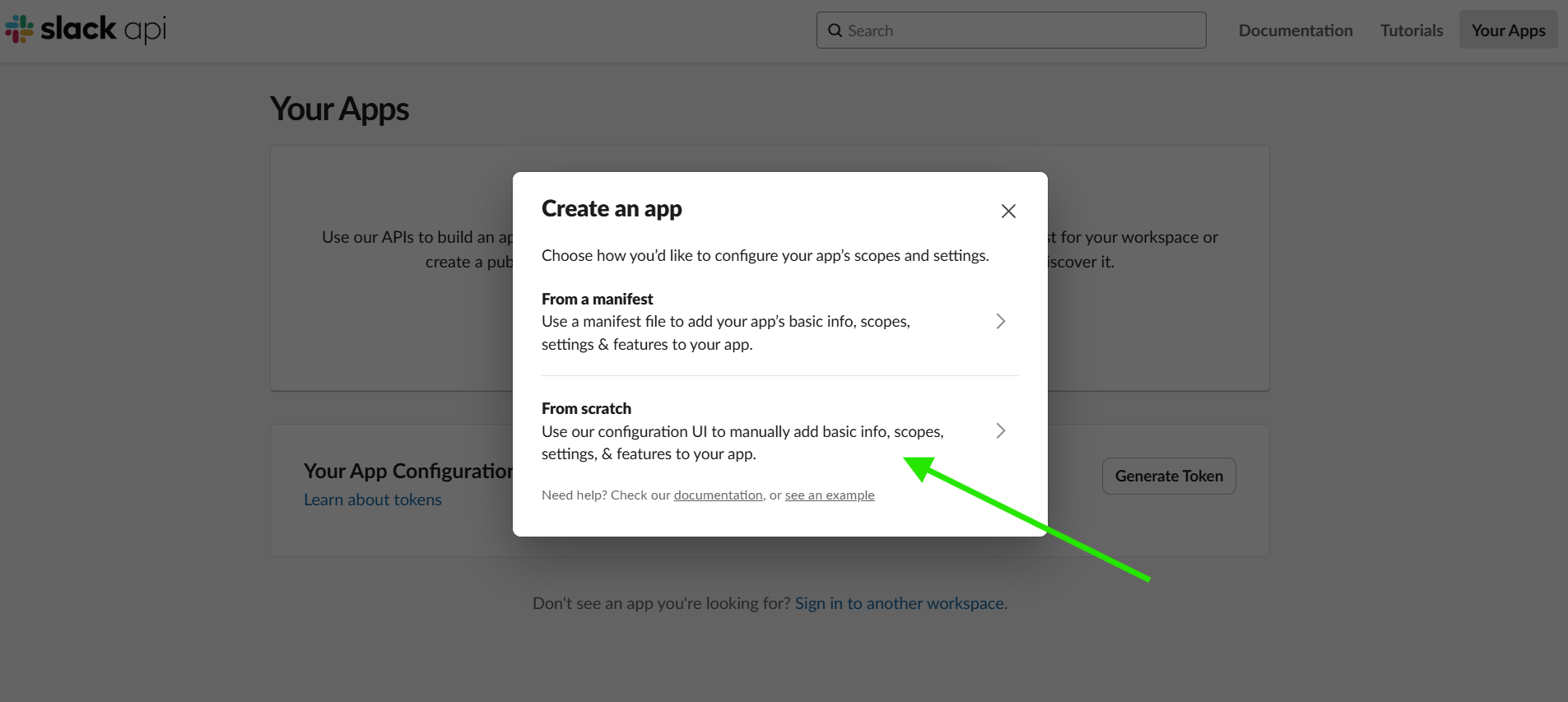
- Provide a meaningful name for your application and select the target workspace
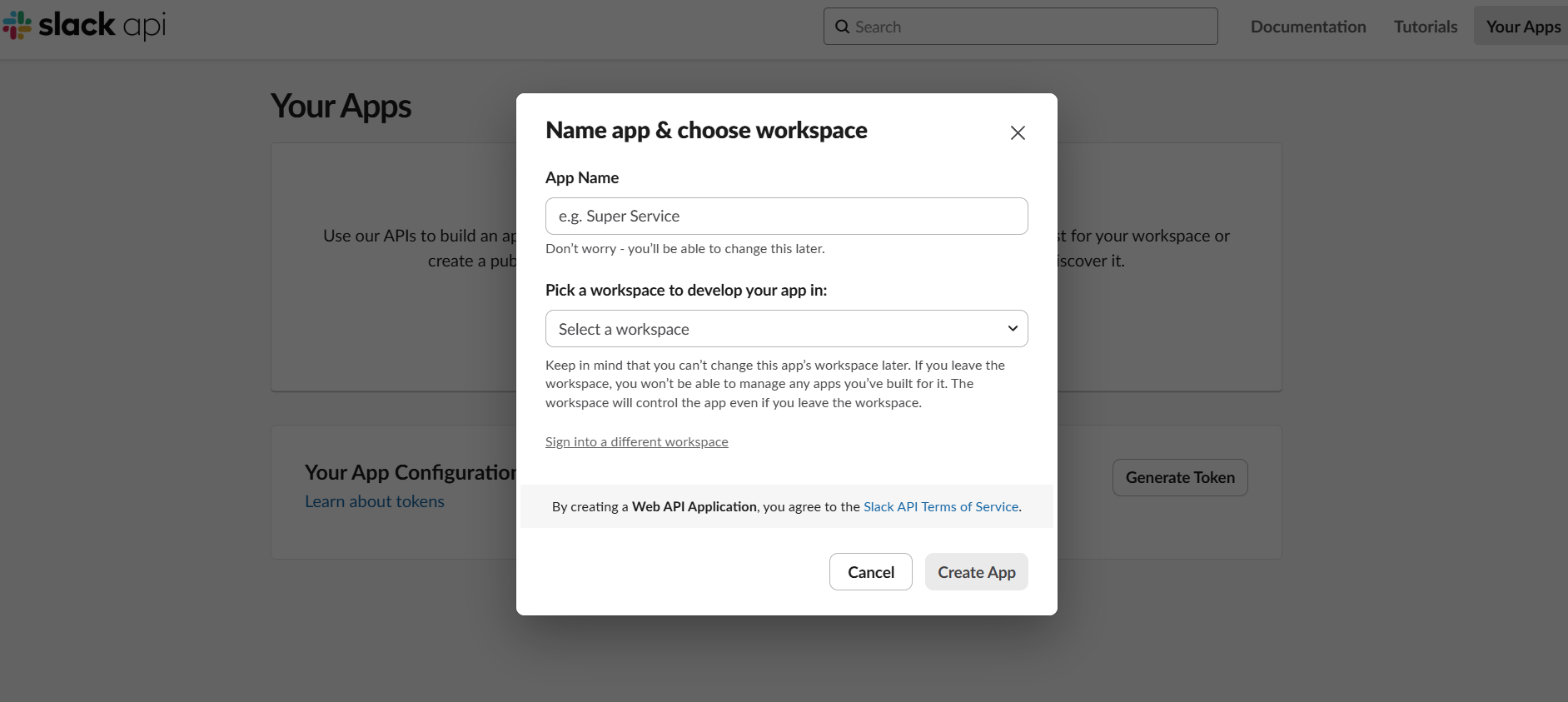
- Take note of your Application ID for future reference
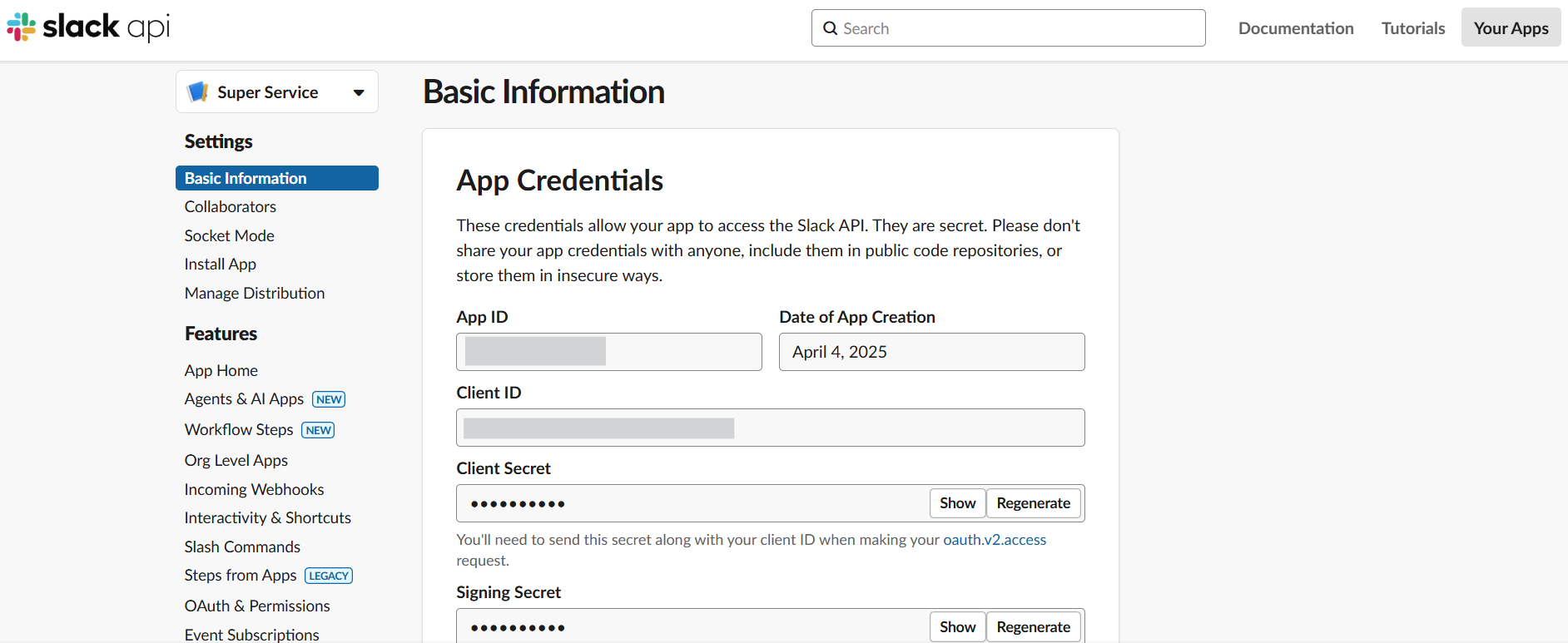
Configure OAuth Scopes:
The server requires specific permissions to function properly:
- Navigate to "OAuth & Permissions" in your app's sidebar
- Under "Bot Token Scopes", add the following scopes:
channels:history
- Allows viewing messages and content in public channelschannels:read
- Enables access to basic information about channelschat:write
- Grants permission to send messages as the applicationreactions:write
- Permits adding emoji reactions to messagesusers:read
- Allows viewing basic information about workspace usersusers:read.email
- (Optional) Enables access to user email addresses
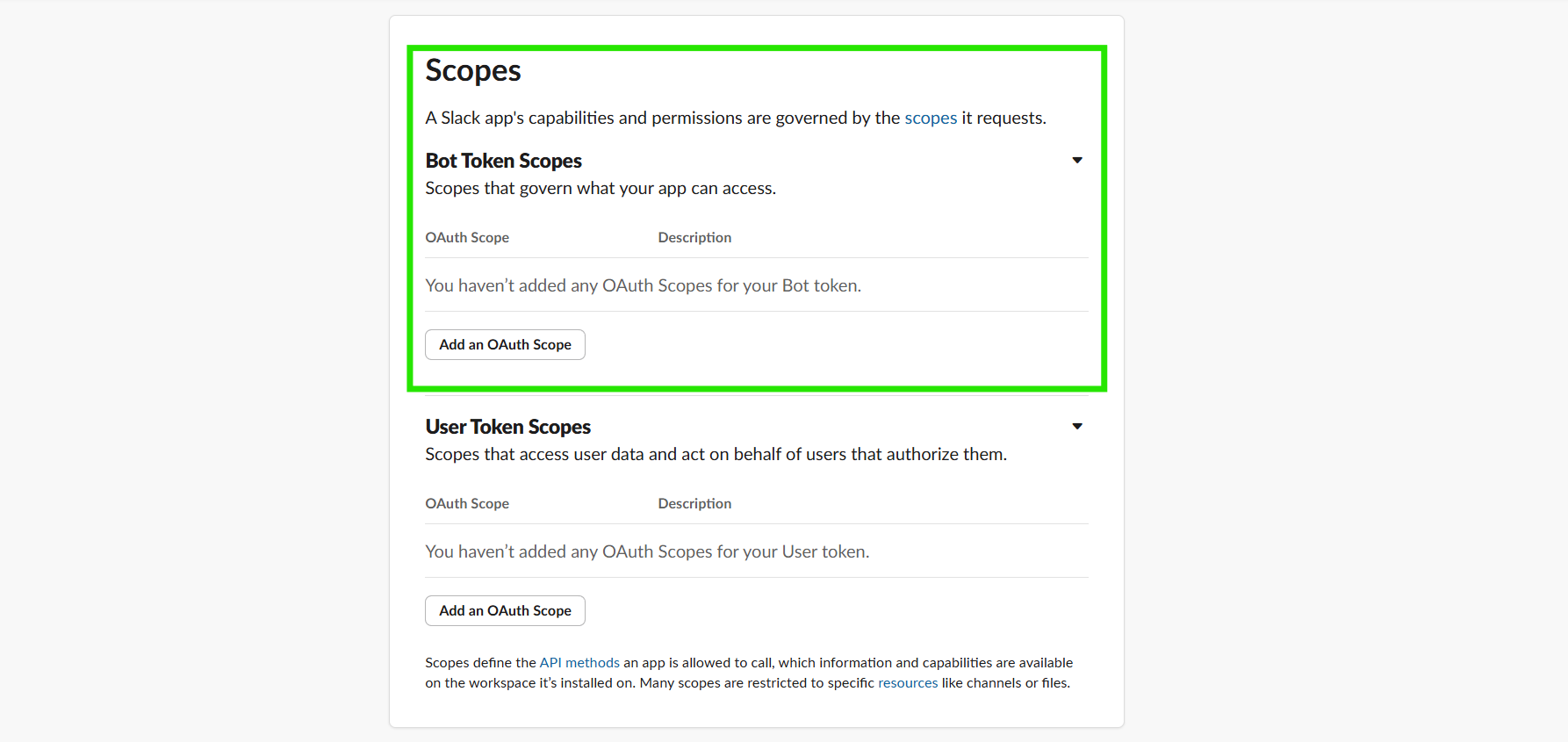
Install to Workspace:
- Click the "Install to Workspace" button in the OAuth section
- Review and approve the requested permissions
- After approval, you'll receive a "Bot User OAuth Token" that starts with
xoxb-
- Securely store this token as it will be needed for server configuration
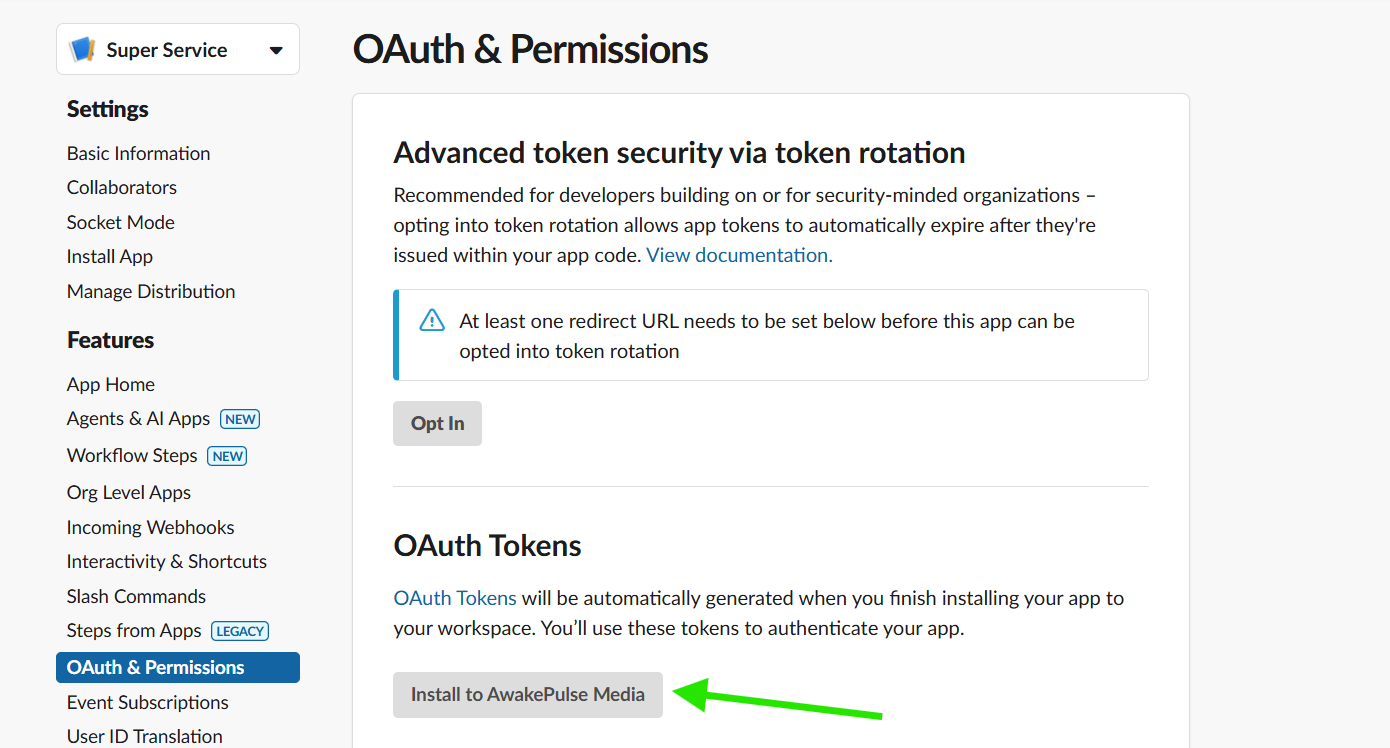
Retrieve Team ID:
- Your Team ID is required for certain API calls
- This ID typically starts with "T" followed by alphanumeric characters
- You can find this in the URL when logged into your Slack workspace or through the API
Deployment Options
The Slack MCP Server offers multiple deployment methods to accommodate various infrastructure preferences:
NPX Deployment (Recommended for Quick Setup)
This method leverages NPM's package execution functionality for a streamlined setup:
{
"mcpServers": {
"slack": {
"command": "npx",
"args": [
"-y",
"@modelcontextprotocol/server-slack"
],
"env": {
"SLACK_BOT_TOKEN": "xoxb-your-bot-token",
"SLACK_TEAM_ID": "T01234567"
}
}
}
}
Docker Container Deployment
For environments where containerization is preferred:
{
"mcpServers": {
"slack": {
"command": "docker",
"args": [
"run",
"-i",
"--rm",
"-e",
"SLACK_BOT_TOKEN",
"-e",
"SLACK_TEAM_ID",
"mcp/slack"
],
"env": {
"SLACK_BOT_TOKEN": "xoxb-your-bot-token",
"SLACK_TEAM_ID": "T01234567"
}
}
}
}
To build the Docker image locally:
docker build -t mcp/slack -f src/slack/Dockerfile .
Source Deployment
For those who prefer direct control over the source code:
Clone the repository:
git clone https://github.com/modelcontextprotocol/server-slack.git
Install dependencies:
npm install
Build the TypeScript project:
npm run build
Start the server:
SLACK_BOT_TOKEN=xoxb-your-token SLACK_TEAM_ID=T01234567 node dist/index.js
Integration with AI Assistant Platforms
Claude Desktop Configuration
To connect the Slack MCP Server with Claude Desktop:
- Locate your Claude Desktop configuration file
- Add the MCP server configuration, adjusting paths and tokens as needed:
{
"mcpServers": {
"slack": {
"command": "node",
"args": ["/path/to/project/dist/index.js"],
"env": {
"SLACK_BOT_TOKEN": "xoxb-your-bot-token",
"SLACK_TEAM_ID": "T01234567"
}
}
}
}
Cursor Integration
Cursor is a modern AI-powered code editor that supports MCP servers. You can integrate the Slack MCP Server either globally or per-project:
Global Integration:
- Navigate to Cursor Settings > MCP
- Click "Add new global MCP server"
- Add the server configuration to
~/.cursor/mcp.json
Project-Specific Integration:
Create or edit .cursor/mcp.json
in your project directory with the appropriate configuration.
Detailed Tool Reference
The Slack MCP Server exposes eight primary tools for interaction with Slack:
slack_list_channels
Lists available public channels in the workspace.
- Optional Parameters:
limit
(default: 100, max: 200): Maximum number of channels to returncursor
: Pagination cursor for retrieving additional pages- Returns: Array of channel objects containing IDs, names, member counts, and metadata
slack_post_message
Posts new messages to specified channels.
- Required Parameters:
channel_id
: The ID of the target channeltext
: The message content to post- Returns: Confirmation object with message timestamp and channel information
slack_reply_to_thread
Posts replies to existing message threads.
- Required Parameters:
channel_id
: The channel containing the threadthread_ts
: Timestamp of the parent messagetext
: The reply content- Returns: Confirmation object with reply timestamp and thread information
slack_add_reaction
Adds emoji reactions to messages.
- Required Parameters:
channel_id
: The channel containing the target messagetimestamp
: The message timestamp to react toreaction
: Emoji name without colons (e.g., "+1" for 👍)- Returns: Confirmation of successful reaction addition
slack_get_channel_history
Retrieves recent messages from a channel.
- Required Parameters:
channel_id
: The target channel ID- Optional Parameters:
limit
(default: 10): Number of messages to retrieve- Returns: Array of message objects with content, timestamps, and metadata
slack_get_thread_replies
Retrieves all replies in a specific message thread.
- Required Parameters:
channel_id
: The channel containing the threadthread_ts
: Timestamp of the parent message- Returns: Array of reply messages with content and metadata
slack_get_users
Lists all workspace users with basic profile information.
- Optional Parameters:
cursor
: Pagination cursor for next pagelimit
(default: 100, max: 200): Maximum users to return- Returns: Array of user objects with IDs, names, and basic profile data
slack_get_user_profile
Retrieves detailed profile information for a specific user.
- Required Parameters:
user_id
: The target user's ID- Returns: Comprehensive user profile object with all available fields
When implementing the Slack MCP Server, several common issues may arise:
Authentication Problems
- Symptom: "Not authorized" errors in server logs
- Solution: Verify that the Bot Token is correctly copied and has not expired
- Prevention: Periodically rotate tokens following security best practices
Permission Limitations
- Symptom: "Permission denied" when attempting certain operations
- Solution: Review the OAuth scopes configured for your Slack app and add any missing permissions
- Prevention: Document required permissions in your deployment guide
Channel Access Issues
- Symptom: Unable to post to specific channels
- Solution: Ensure the Slack bot has been invited to the channels it needs to access
- Prevention: Create an onboarding script that automatically invites the bot to essential channels
Rate Limiting
- Symptom: Failed requests with rate limit notifications
- Solution: Implement exponential backoff for retries and consider batching requests when possible
- Prevention: Monitor API usage patterns and optimize high-volume operations
By establishing this bridge between AI assistants and your Slack workspace, teams can unlock new possibilities for automation, information retrieval, and collaborative workflows. The Slack MCP Server represents an important step toward truly integrated AI assistance in the modern workplace, offering both the technical foundation and practical tools needed for meaningful AI participation in team communications.