So, you want to build your Web automation, and simulate the human-like typing, right? Congratulate, you've come to the right place!
While Selenium's send_keys()
method efficiently accomplishes this, it often does so at a speed that's far from human-like. This can sometimes trigger anti-bot measures or fail to properly interact with dynamic web elements. Enter the concept of "Selenium send keys with delay" – a technique that adds a more natural, human-like rhythm to automated text input.
Why Do You Need to Delayed Input in Selenium
Before diving into the implementation, it's crucial to understand why we might need to add delays between keystrokes:
Mimicking Human Behavior: Humans don't type at lightning speeds. Adding delays makes the input appear more natural.
Avoiding Anti-Bot Detection: Many websites have measures to detect and block automated inputs. Slowing down the input can help bypass these measures.
Allowing for Dynamic Content: Some web applications load content dynamically as the user types. Rapid input might outpace these loading processes.
Debugging and Visualization: Slowed-down input makes it easier to visually debug your automation scripts.
Now that we understand the 'why', let's explore the 'how'.
How to Implement Delayed Send Keys in Selenium
There are several approaches to implementing delayed send keys in Selenium. We'll cover the most common and effective methods, starting with the simplest and progressing to more advanced techniques.
Method 1: Basic Loop with Time.sleep()
The most straightforward method involves using a simple loop and Python's time.sleep()
function.
from selenium import webdriver
from selenium.webdriver.common.by import By
import time
driver = webdriver.Chrome()
driver.get("https://example.com")
input_element = driver.find_element(By.ID, "input-field")
text_to_input = "Hello, World!"
for character in text_to_input:
input_element.send_keys(character)
time.sleep(0.1) # 100ms delay between each character
This method is simple but has some drawbacks:
- It uses a fixed delay, which isn't very realistic.
- The
time.sleep()
function can sometimes be imprecise, especially for very short delays.
Method 2: Random Delays
To make the typing appear more human-like, we can introduce random delays between keystrokes.
import random
# ... (previous setup code)
min_delay = 0.05
max_delay = 0.3
for character in text_to_input:
input_element.send_keys(character)
time.sleep(random.uniform(min_delay, max_delay))
This approach adds variability to the typing speed, making it appear more natural.
Method 3: Using ActionChains
Selenium's ActionChains class provides a more sophisticated way to handle user interactions, including typing with delays.
from selenium.webdriver.common.action_chains import ActionChains
# ... (previous setup code)
actions = ActionChains(driver)
for character in text_to_input:
actions.send_keys(character)
actions.pause(random.uniform(min_delay, max_delay))
actions.perform()
This method is more efficient as it builds up a chain of actions before executing them all at once.
Method 4: Custom JavaScript Function
For more precise control, we can inject and execute a custom JavaScript function:
js_code = """
function typeWithDelay(element, text, minDelay, maxDelay) {
var i = 0;
var interval = setInterval(function() {
if (i < text.length) {
element.value += text.charAt(i);
i++;
} else {
clearInterval(interval);
}
}, Math.floor(Math.random() * (maxDelay - minDelay + 1) + minDelay));
}
"""
driver.execute_script(js_code)
driver.execute_script("typeWithDelay(arguments[0], arguments[1], arguments[2], arguments[3])",
input_element, text_to_input, 50, 200)
This JavaScript approach gives us fine-grained control over the typing behavior and can be more reliable across different browsers.
Advanced Techniques for Delaying Keys with Selemium
While the methods above cover the basics, there are several advanced techniques and considerations to keep in mind when implementing delayed send keys in Selenium.
Handling Special Keys and Modifiers
When simulating human-like typing, it's important to consider special keys and modifiers. For instance, you might want to simulate the use of Shift for capital letters or punctuation marks.
from selenium.webdriver.common.keys import Keys
# ... (previous setup code)
text_to_input = "Hello, World!"
for char in text_to_input:
if char.isupper():
actions.key_down(Keys.SHIFT)
actions.send_keys(char.lower())
actions.key_up(Keys.SHIFT)
else:
actions.send_keys(char)
actions.pause(random.uniform(min_delay, max_delay))
actions.perform()
This approach simulates the pressing and releasing of the Shift key for uppercase letters, adding another layer of realism to the typing simulation.
Implementing Typing Mistakes and Corrections
To make the typing even more human-like, you can introduce occasional typing mistakes followed by corrections:
def simulate_typing_with_mistakes(text, mistake_probability=0.05):
result = []
for char in text:
if random.random() < mistake_probability:
# Simulate a typo
wrong_char = random.choice('abcdefghijklmnopqrstuvwxyz')
result.append(wrong_char)
result.append(Keys.BACKSPACE)
result.append(char)
return result
text_to_input = "Hello, World!"
characters_to_type = simulate_typing_with_mistakes(text_to_input)
for char in characters_to_type:
actions.send_keys(char)
actions.pause(random.uniform(min_delay, max_delay))
actions.perform()
This function introduces random typos and immediate corrections, further enhancing the human-like quality of the input.
Adapting to Dynamic Web Elements
Sometimes, web elements might change or become stale during the typing process. To handle this, you can implement a retry mechanism:
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
def send_keys_with_retry(element, text, max_retries=3):
for attempt in range(max_retries):
try:
for char in text:
element.send_keys(char)
time.sleep(random.uniform(min_delay, max_delay))
return # Success, exit the function
except StaleElementReferenceException:
if attempt < max_retries - 1:
# Wait for the element to become available again
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID, "input-field"))
)
else:
raise # Re-raise the exception if max retries reached
# Usage
try:
send_keys_with_retry(input_element, text_to_input)
except Exception as e:
print(f"Failed to input text after multiple attempts: {e}")
This function attempts to type the text multiple times, handling cases where the element might become stale during the typing process.
How to Make Selenium Performance Faster with send_keys()
While adding delays to send_keys()
can make your automation more robust and realistic, it's important to consider the performance implications:
Increased Execution Time: Obviously, adding delays will increase the overall execution time of your tests or automation scripts.
Resource Usage: Long-running scripts with many delayed inputs might consume more system resources over time.
Timeout Handling: You may need to adjust timeout settings in your WebDriverWait calls to account for the additional time taken by delayed inputs.
To mitigate these issues, consider the following strategies:
- Use delayed input only where necessary, such as on login forms or other sensitive inputs.
- Implement a configuration system that allows you to easily enable or disable delayed input for different scenarios or environments.
- Use more efficient methods like ActionChains or custom JavaScript functions for better performance.
Introducing Apidog: Your API Companion
While we're on the topic of enhancing your automation and development workflow, let's take a moment to introduce Apidog. If you're working with APIs, having top-notch documentation is essential. Apidog is a powerful tool designed to make API documentation easy and efficient.
Key Features of Apidog
- Interactive Documentation: Test API endpoints directly within the documentation.
- Auto-Generated Docs: Automatically generate documentation from your API specifications.
- Collaboration Tools: Work with your team in real-time to create and update documentation.
- API Testing: Built-in tools for testing API endpoints.
- Customizable Templates: Create documentation that matches your brand and style.
Why Choose Apidog?
Apidog stands out because it combines ease of use with powerful features. Whether you're a solo developer or part of a large team, Apidog provides the tools you need to create top-notch API documentation. The ability to test APIs directly from the documentation is a huge time-saver. And the best part? You can download Apidog for free and start improving your API documentation today.
How to Send Independent Request with Apidog
Move the mouse to the left directory, click the +
buttom next to the search box on the left, select New Request
to create a new quick request. New Request
from the drop-down list.
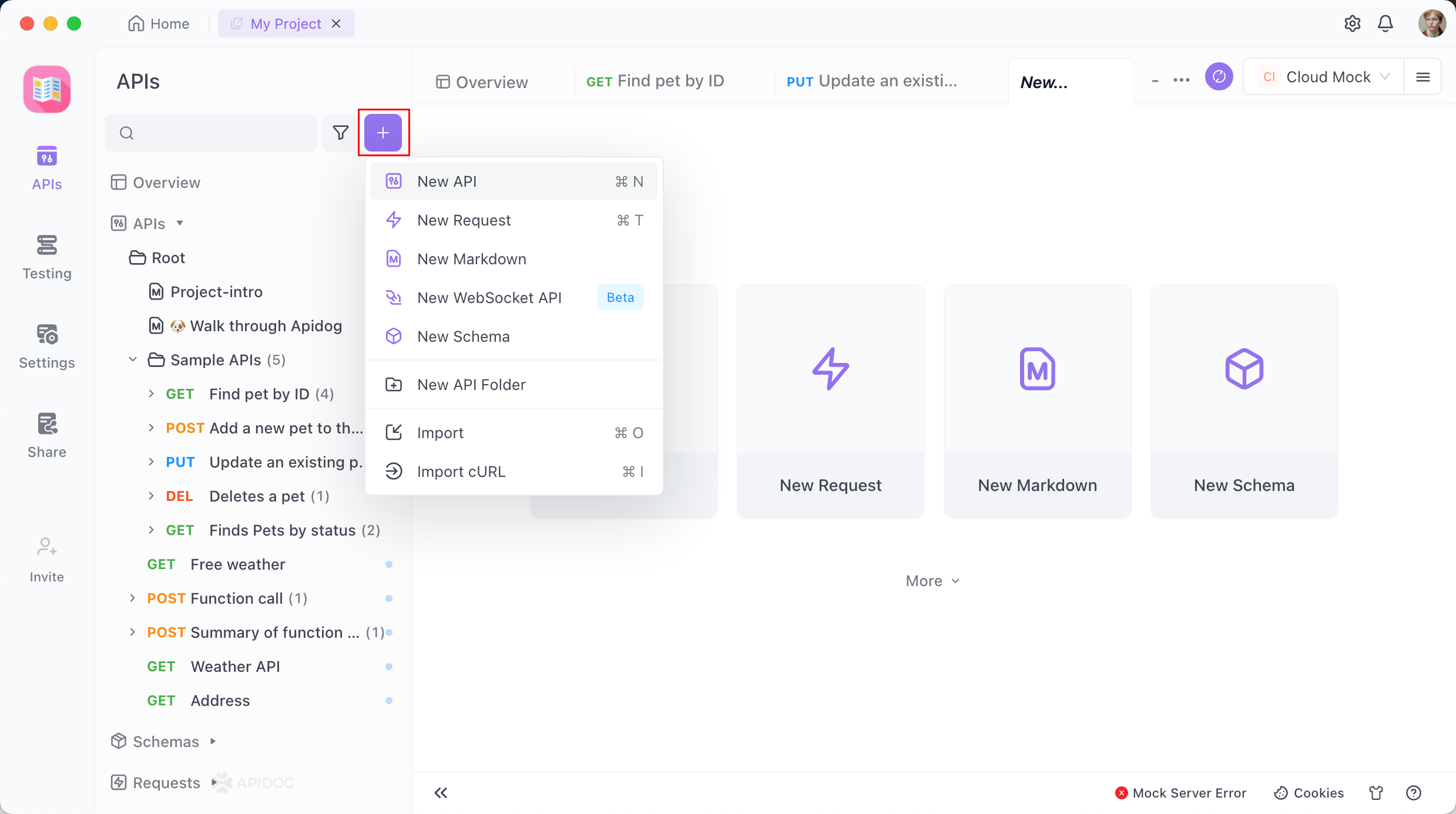
Optionally Move the mouse to the left directory, click the +
button on the right side of New Request
to create a new quick request.
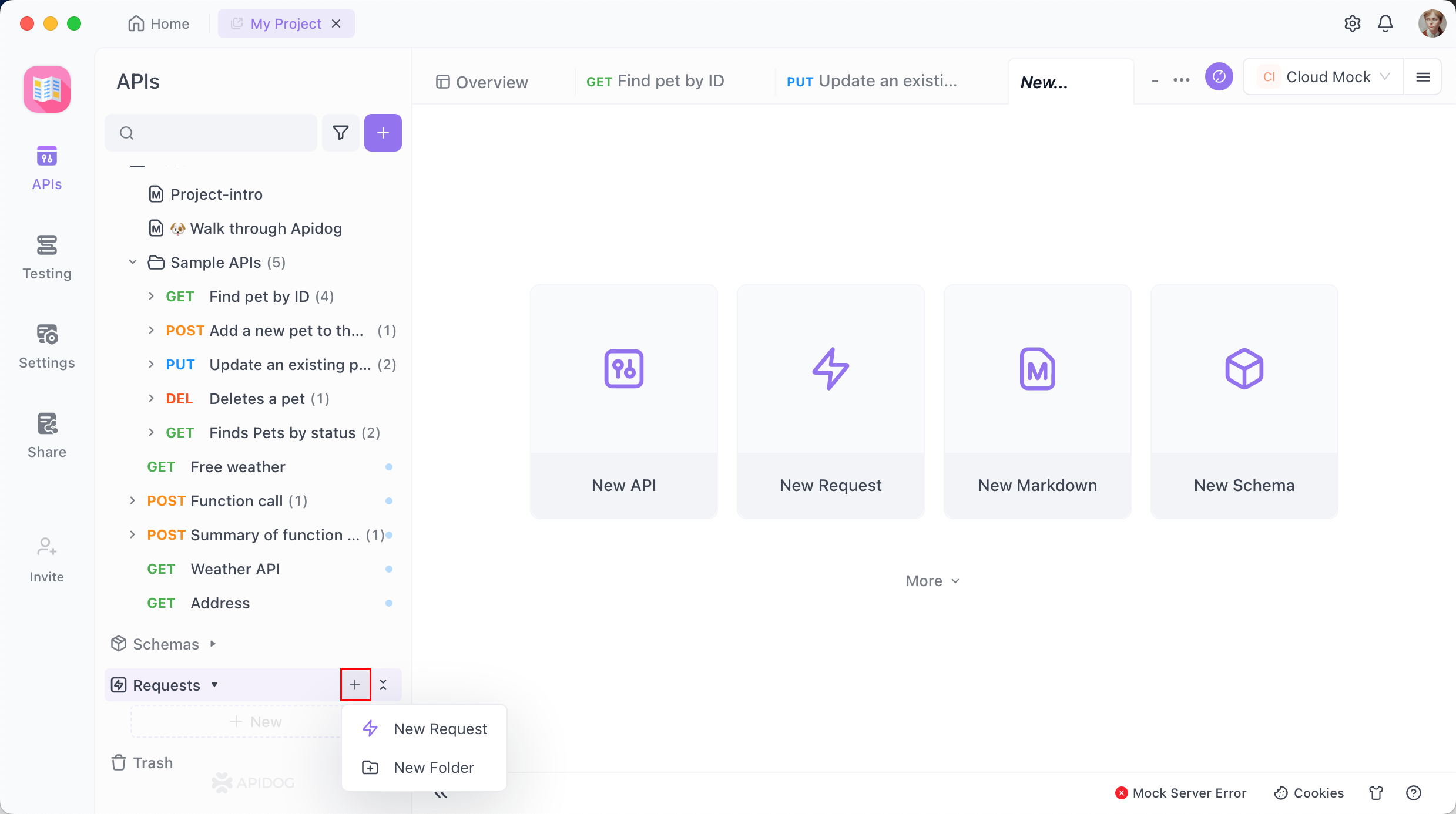
Enter the API URL and parameters to quickly request the API.
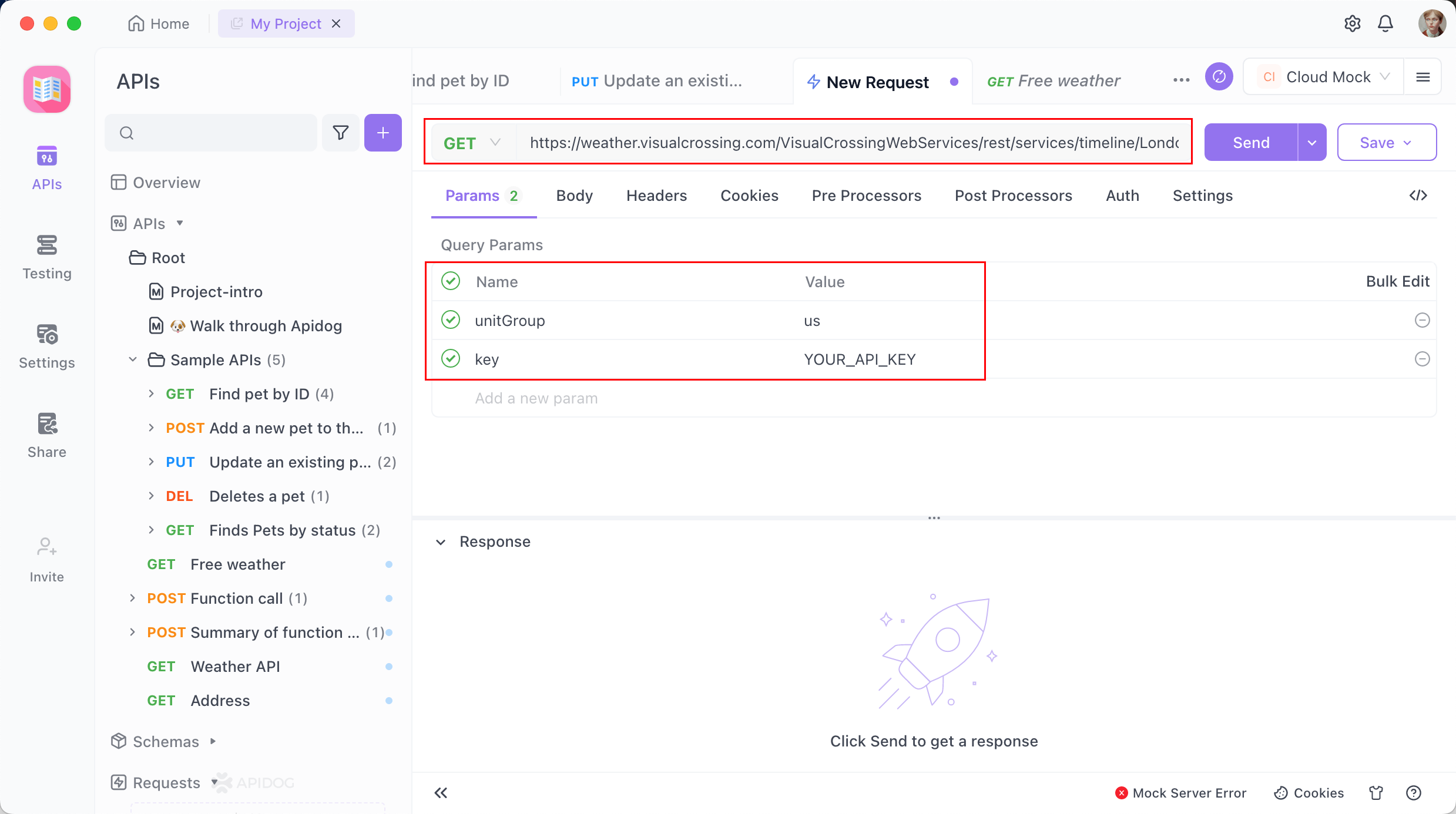
After debugging, the request can be saved as a New Request or API Documentation.
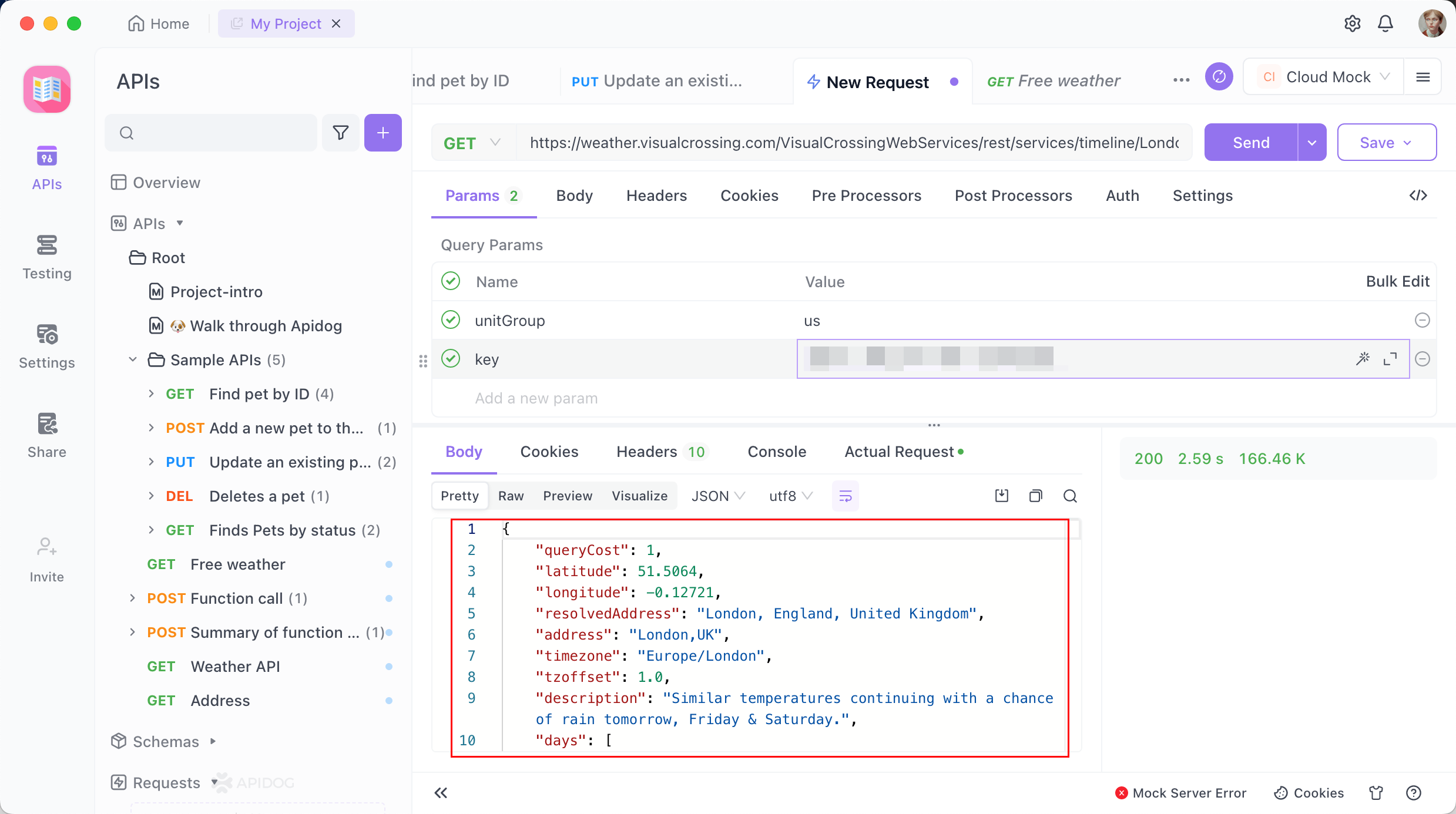
Saved New Request
can be managed in the left directory.
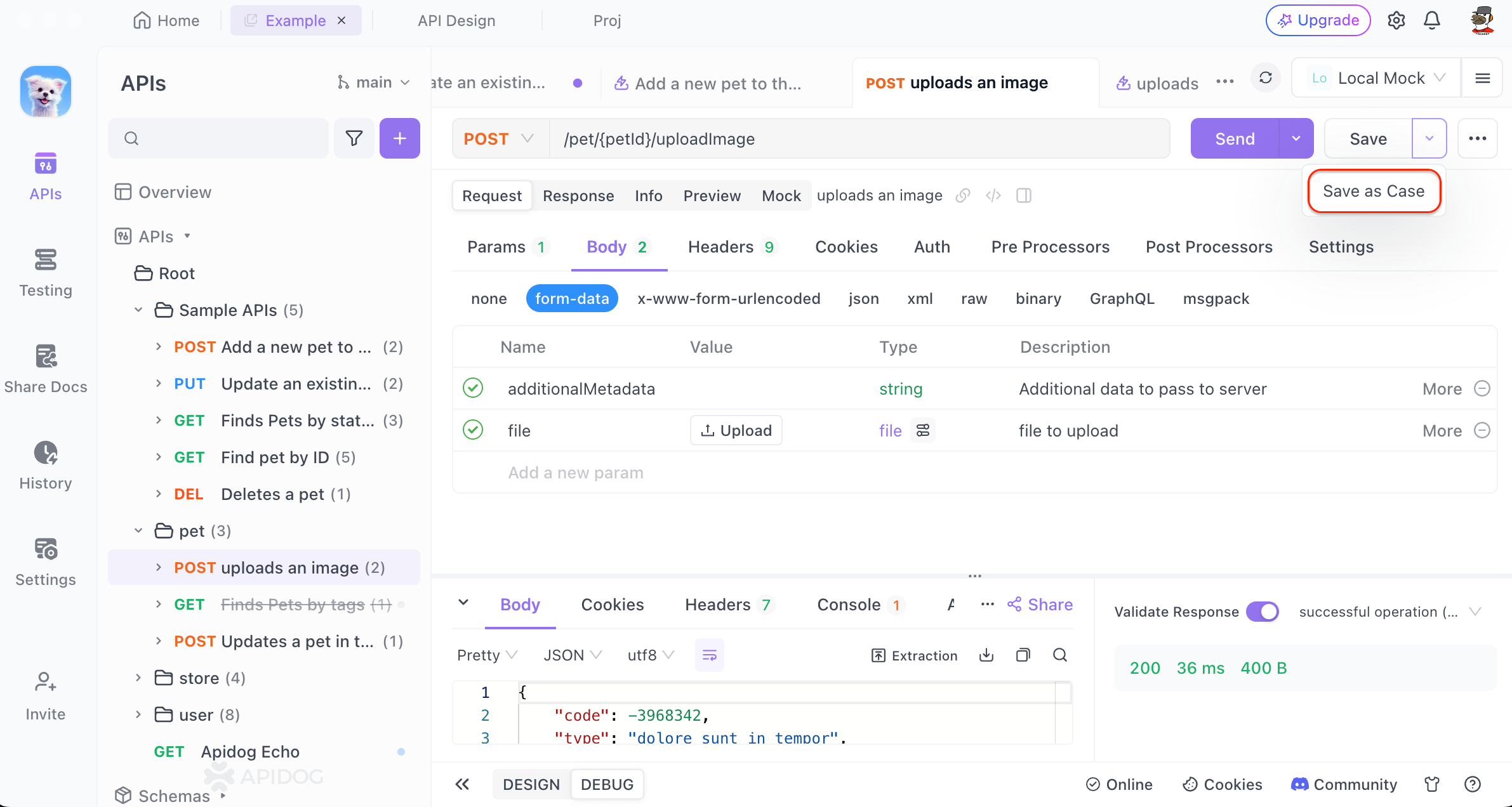
Best Practices for send_keys() with Selemium
To make the most of delayed send keys in Selenium, keep these best practices in mind:
Variability is Key: Avoid using fixed delays. Randomize the delay times within a reasonable range to make the input appear more natural.
Context-Aware Delays: Consider the context of what's being typed. For instance, you might use shorter delays for familiar words and longer delays for complex terms or email addresses.
Observe Real User Behavior: If possible, study the typing patterns of real users on your application to inform your delay strategies.
Test Different Approaches: Experiment with different methods (Python loops, ActionChains, JavaScript) to see which works best for your specific use case.
Stay Updated: Keep your Selenium and browser driver versions up to date, as improvements in these tools might affect how delayed inputs are handled.
Document Your Approach: Clearly document why and how you're implementing delayed send keys in your project, so other team members understand the rationale and implementation.
Conclusion
Implementing delayed send keys in Selenium is a powerful technique for creating more realistic and robust web automation scripts. By simulating human-like typing patterns, you can improve the reliability of your tests, bypass certain anti-bot measures, and create more authentic interactions with web applications.
Whether you choose a simple Python-based approach, leverage Selenium's ActionChains, or implement custom JavaScript solutions, the key is to find the right balance between realism and efficiency for your specific use case. Remember that while adding delays can solve many problems, it's not a one-size-fits-all solution. Always consider the specific requirements of your project and the behavior of the web application you're interacting with.
As web technologies continue to evolve, so too will the techniques for automating interactions with them. Stay curious, keep experimenting, and always be on the lookout for new and improved ways to make your Selenium scripts more effective and reliable. And don't forget to check out Apidog to take your API documentation to the next level!