A Closer Look Into REST API Calls
REST API calls are an important concept for any web developer to master. To keep things simple, REST API calls can be though of as the bridge that enables data exchange between users (like us) and servers (databases). Come and take a closer look at how you can create REST API calls yourself.
Have you worked with REST APIs before? If you have, then chances are you have seen the phrase "REST API Calls"!
If you wish to try out Apidog, all you need to do is click the button below! 👇 👇 👇
The Essence of REST API Calls
The phrase "REST API calls" refers to messages sent between two applications over the internet with the intent of requesting or exchanging data. This is the way for computer programs to "talk" to one another, except they conform to the REST architecture.
Dissecting REST API Calls
REST API Calls can be separated into three single entities (and each of them is worthy of an article!). The breakdown looks something like this:
REST: REST is the acronym for Representational State Transfer. REST is a set of architectural principles made so that APIs are built to be consistent, scalable, and easily learned. It is also responsible for how the API is structured and how the corresponding requests and responses are formatted.
API: Also known as Application Programming Interface, it is a tool software and application developers may use to enable data exchange between two programs or applications, defining their method of interaction.
Calls: In APIs, calls are the messages that are sent between the applications through the API. They will include useful information, such as what kind of data is requested and what action is desired.
Step-by-step Explanation of How REST API Calls Work
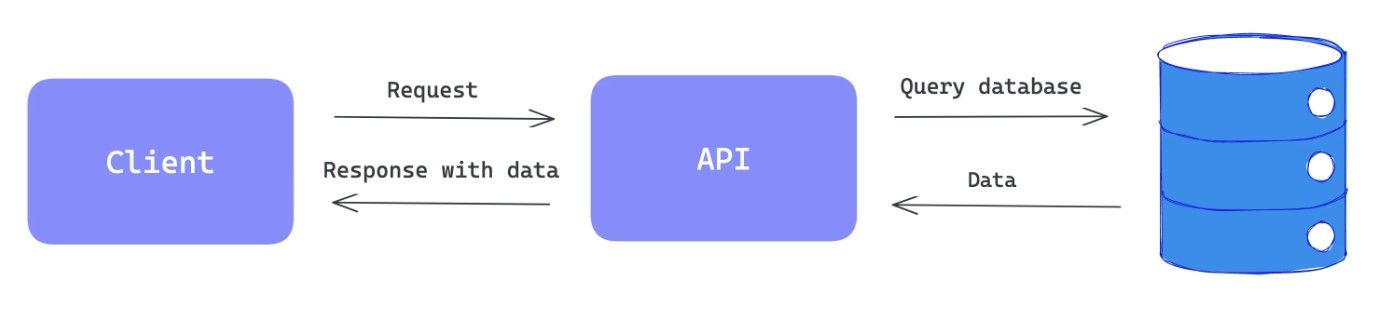
- Client (your application): Makes a REST API call by sending a request message to the server (the application providing the data or service) via the API. This request includes details like the specific resource it wants (e.g., a user profile), the desired action (e.g., retrieve data), and any necessary data (e.g., authentication credentials).
- Server: With the help of the API as a means of communication, the server receives the request and processes it. It retrieves the requested data, performs the desired action, or sends an error message if there's an issue.
- Server: Sends a response message back to the client through the API, containing the requested data, confirmation of the action, or an error message.
- Client: Receives the response and interprets it based on the pre-defined format (often JSON). It then uses the received information for its intended purpose. It could be a list of vegetables starting with the letter
S
, or perhaps viewing all soccer matches happening in July.
Examples of REST API Calls Written in Different Client Languages
Here are a few REST APIs that you can make using different client languages - please do note that the code samples provided in this article are not 100% functioning, as they require further modification to fit your API requirements!
GET request to retrieve a user profile (Python):
import requests
url = "https://api.example.com/users/123"
response = requests.get(url)
if response.status_code == 200:
# Successful request, access data in response.json()
user_data = response.json()
print(f"User name: {user_data['name']}")
print(f"Email: {user_data['email']}")
else:
print(f"Error: {response.status_code}")
The GET request above retrieves a profile through the https://api.example.com/users/123
URL, where if it receives the Status Code 200
, it will print out the response that includes the username and email of the person's profile. Else, it will print out Error: Status Code xxx
where xxx
could be any status code denoting an error has occurred (unsuccessful request).
POST request to create a new product (JavaScript):
fetch("https://api.example.com/products", {
method: "POST",
headers: {
"Content-Type": "application/json"
},
body: JSON.stringify({
name: "New Product",
price: 19.99,
description: "This is a new product!"
})
})
.then(response => response.json())
.then(data => {
console.log("Product created successfully:", data);
})
.catch(error => {
console.error("Error creating product:", error);
});
Through the https://api.example.com/products
URL, this POST request wishes to add a new record to the database. If the response received corresponds to a successful status code, it will print out Product created successfully: insert product name
. Else, there will be an error message displayed: Error creating product: insert error message
.
PUT request to update a user's address (Java):
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
public class UpdateUserAddress {
public static void main(String[] args) throws Exception {
String url = "https://api.example.com/users/123/address";
String newAddress = "123 Main St, Anytown, CA";
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create(url))
.PUT(HttpRequest.BodyPublishers.ofText(newAddress))
.header("Content-Type", "text/plain")
.build();
HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
if (response.statusCode() == 200) {
System.out.println("Address updated successfully!");
} else {
System.err.println("Error updating address: " + response.statusCode());
}
}
}
This PUT request updates a user's address with the help of path parameters (the URL found in the code sample is: https://api.example.com/users/123/address
. If done successfully, the message Address updated successfully!
appears, otherwise, it will print the error message Error updating address:
insert error status code
.
DELETE request to remove a product (Python):
import requests
url = "https://api.example.com/products/10"
response = requests.delete(url)
if response.status_code == 204:
print("Product deleted successfully!")
else:
print(f"Error deleting product: {response.status_code}")
DELETE requests like the one above remove a record from the database via the URL https://api.example.com/products/10
. If the response code matches 204
, you will receive the message saying Product deleted successfully!
. If not, you will receive the error message: Error deleting product: insert error status code
.
Initializing Your REST API Call Using Apidog
Apidog is an API development tool for API developers. It is loaded with all the specifications and modifications required of an API developer, be it a new or experienced developer! Furthermore, you can also craft your API request to initiate your REST API call.
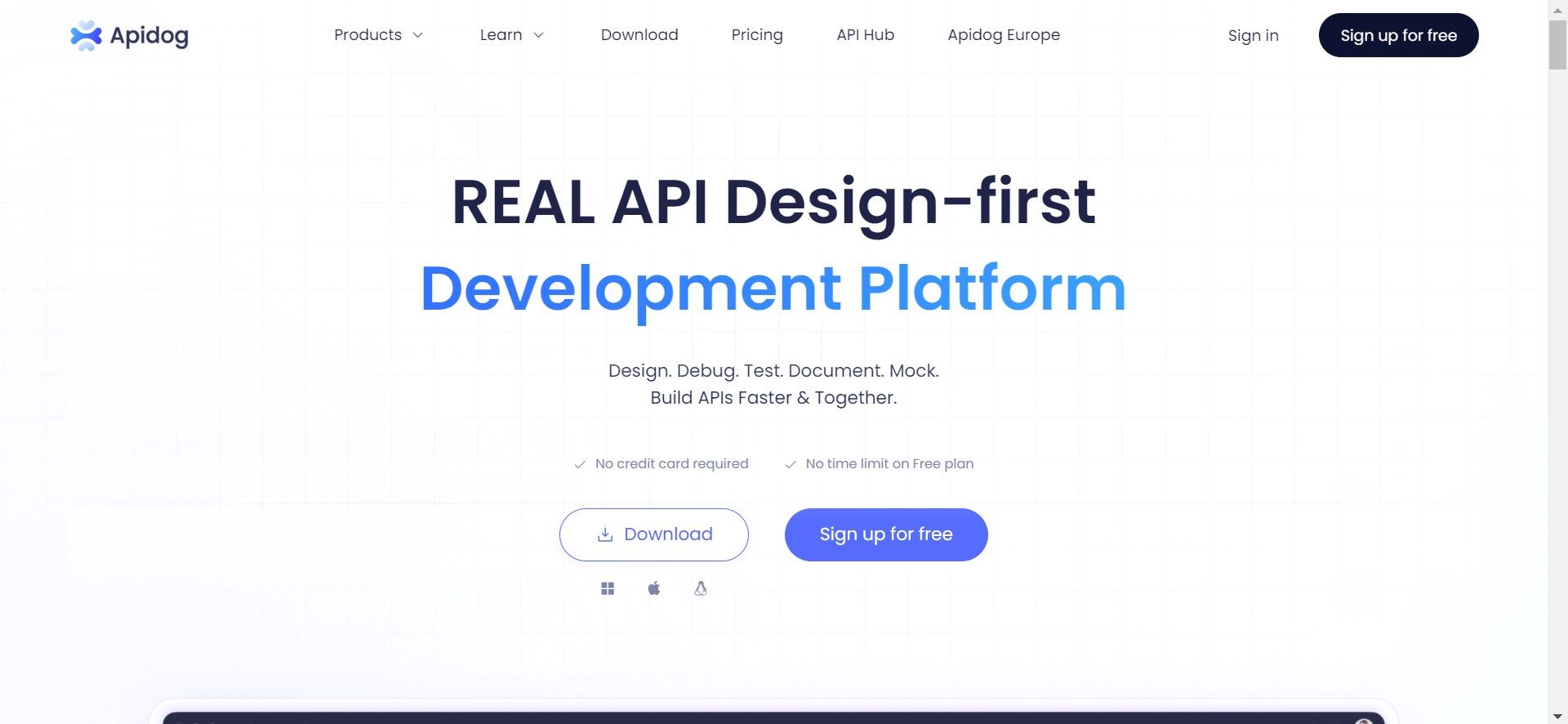
The next section will explain further how you can create your API request to start the whole REST API call process.
Configuring an API Request on Apidog
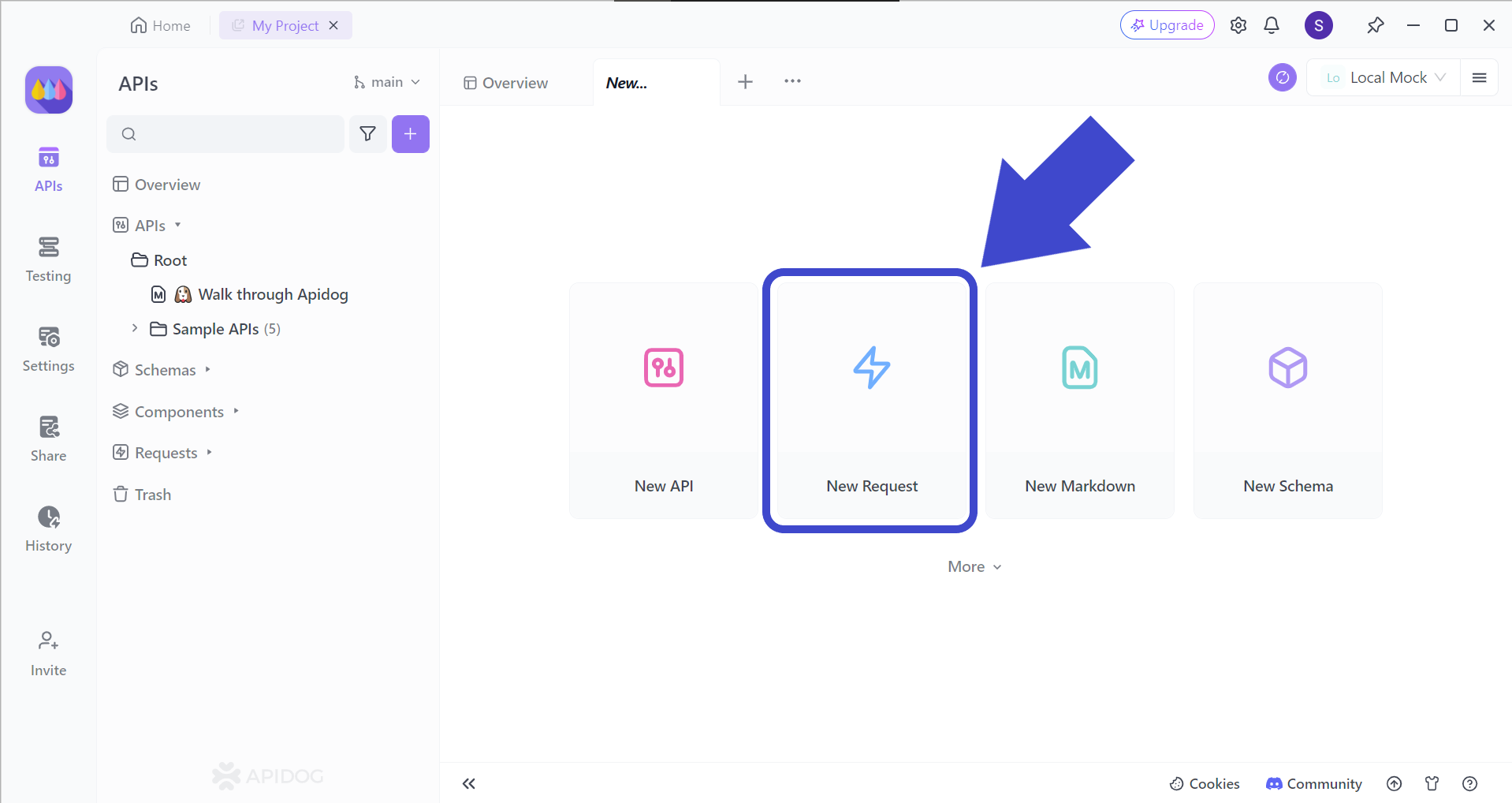
Start by creating a new project, followed by creating a new request to set up your REST API call.
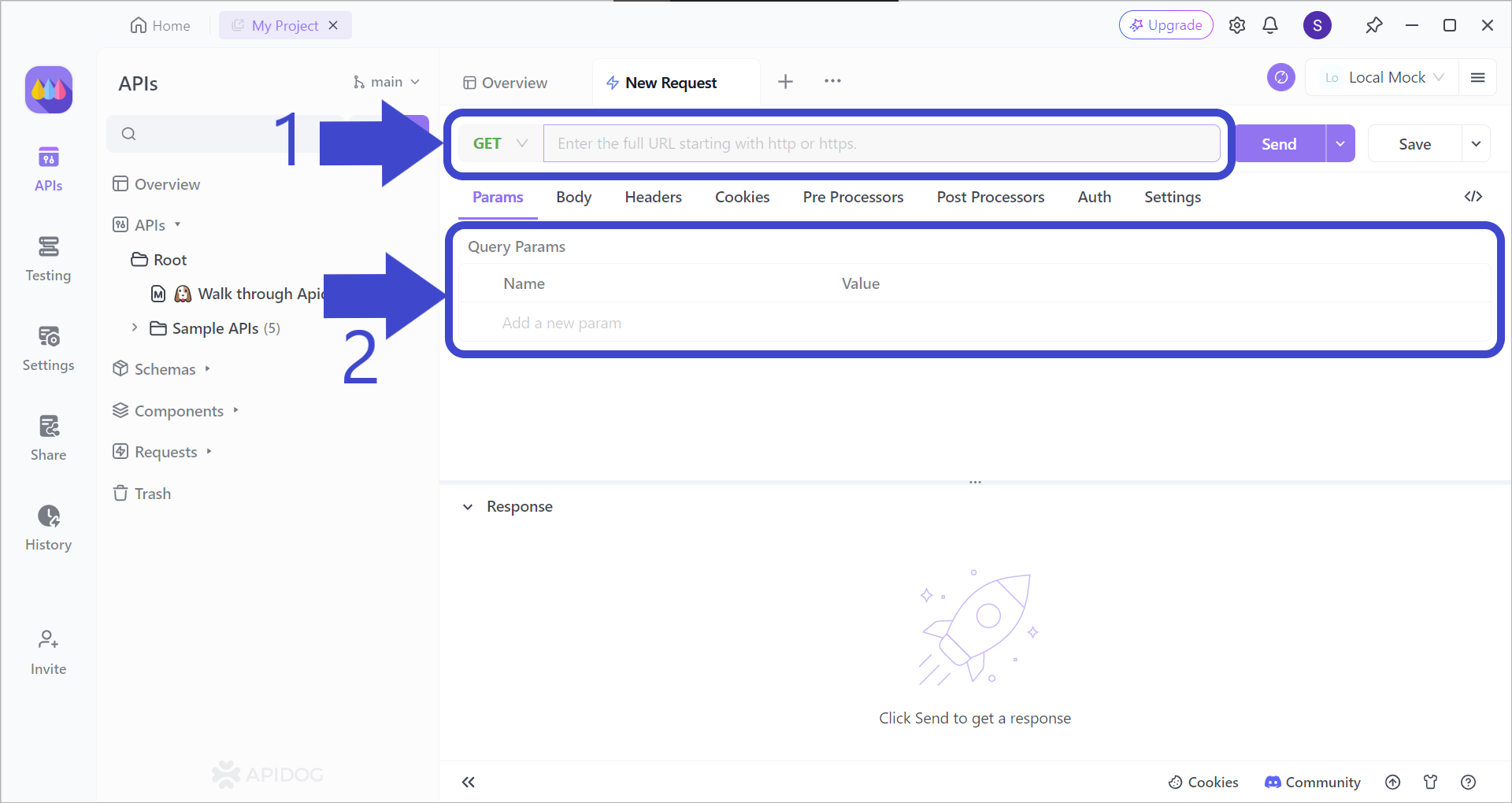
Next, ensure that you craft an appropriate REST API URL for your API request, and make sure to select the right HTTP method. If you wish to include parameters, you can also define them in this section.
Once you have filled in all the required fields, you can press run to send a response to your application, and observe the response you receive!
Use Apidog to Generate Client Language Code
If you require some assistance with coding, Apidog can help with that!
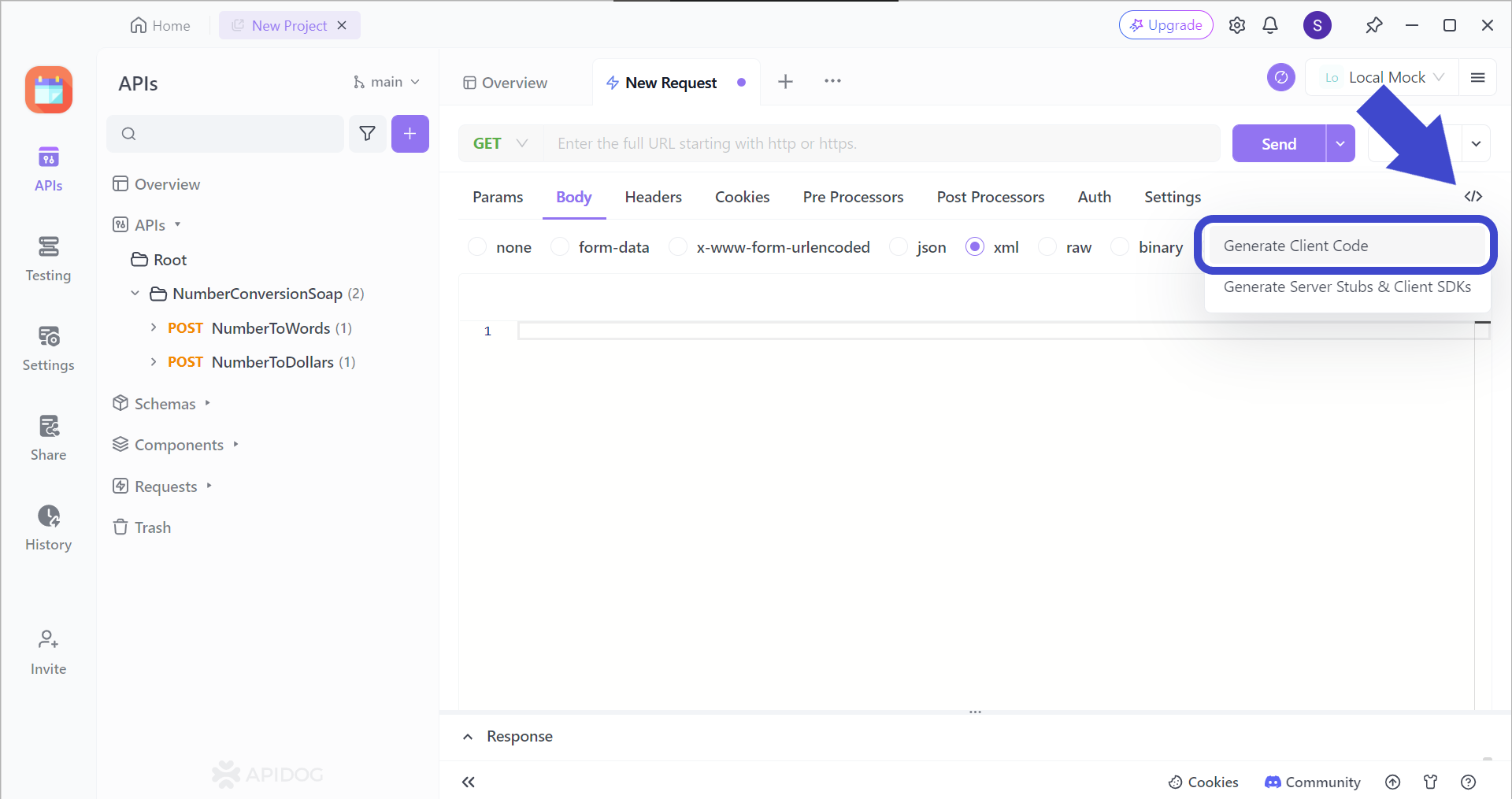
Around the top right corner of the Apidog window, locate and press the </>
button, as pointed out by the arrow in the image above.
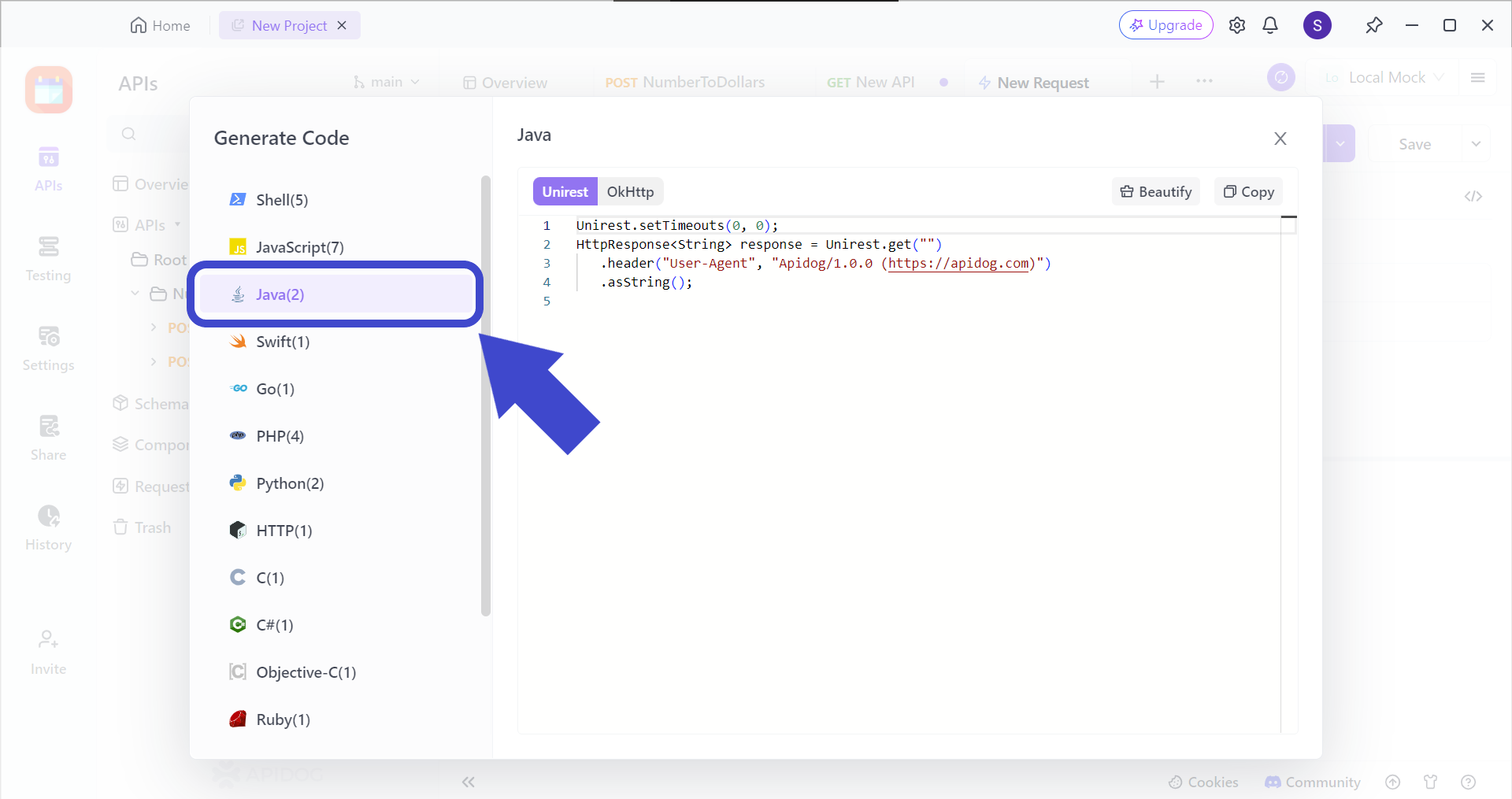
You can then select which client language you require help in. The image above shows an example of the Java client language.
Conclusion
REST API calls are extremely powerful tools for developers to utilize. They allow software and web developers to create fun, interesting, and most importantly, useful applications for us to use. By bridging together the client (user-side) to the server (database-side), data exchange has become much easier and effortless!
Apidog is a suitable option for API developers looking for a platform to create REST API calls. Isn't it much more useful to have an all-in-one API platform that allows you to test, debug, modify, and mock your API requests so that you can perfect your API? If Apidog has your attention, it is not too late to try. Go now and download Apidog - it is free for everyone to use.