Python Tutorial: Working with SOAP-based Web Services
Python is a popular programming language used with and for SOAP-based web services.
There are a variety of client languages that you can learn to make web applications or servers for anyone to use. A popular client language that many people use these days is Python. Whether you're a software engineer or a university student during your semester, Python is a solid language choice to pick up for your coding arsenal.
Python - Popular Programming Language
If this is your first time seeing Python, it is a computer programming language well known for its readability and simplicity. It is a lot more user-friendly compared to other existing programming languages. It can be a very suitable language to pick up if you're interested in learning more about web development!
Other common use cases for Python are in data analysis, artificial intelligence, and automation, so if you're planning to go into these fields of study, it is the perfect time to start familiarizing yourself with Python!
SOAP-based Web Services
What is SOAP in "SOAP-based"?
SOAP, short form for Simple Object Access Protocol, is a protocol for exchanging structured information amongst web services, systems, and applications.
By complying with the SOAP protocol, you are following a set of rules for structuring messages in XML (eXtensible Markup Language), allowing programs running on different operating systems and technologies to communicate with one another.
Key Components of a Typical SOAP Message
Envelope
: The outermost element that encapsulates the entire SOAP message.
Header
: An optional section containing additional information for processing the message.
Body
: The main part of the message containing the actual data or the request/response information.
Fault
: An optional section for reporting errors.
WSDL File That Comes with the SOAP Service
Documentation is a developer's best friend when you are trying out something new for the first time. For SOAP web services or SOAP APIs, the instruction manual comes in the form of a WSDL file.
WSDL stands for Web Services Description Language, which provides descriptions of the functionalities of the web service, methods supported, data types used, and protocols followed.
Why Consider Using Python for Working With SOAP Web Services?
Using Python for working with SOAP-based web services provides several advantages for developers. Here are a few reasons why you should consider using and learning Python:
- Simple and Easy: Python's syntax is very clear and easy to read. This allows writing, understanding, and maintaining Python code to be a lot simpler than other programming languages.
- Extensive Library: With Python, countless library sets and modules can work with and for web services - some even specializing in streamlining SOAP service consumption by reducing the complexity of the Python code needed to interact with the SOAP-based web services.
- Versatility: Python is used in many different domains, ranging from web development to data science and machine learning. With a good understanding of Python and web services, you can easily integrate SOAP-based web services into applications in the above-mentioned fields of work.
- Community Support: Python developers all around the world contribute to the community, producing resources such as tutorials and guides for newer developers to work with the Python programming language, and this includes working with SOAP-based web services too.
- Cross-Platform Support: Python programming language can be run on various operating systems, such as Windows, macOS, Linux, and more. It allows Python applications to be a lot more maintainable across different working environments.
Interaction Examples of Python and SOAP-based Web Services Using "Suds-Jurko" Library
Python requires the "suds-jurko" library to interact with SOAP-based web services, so before you do anything, make sure to run the following line on Bash:
pip install suds-jurko
Simple SOAP Service Interaction
from suds.client import Client
# Step 1: Create a SOAP Client
url = 'https://www.example.com/soap-service?wsdl'
client = Client(url)
# Step 2: Explore the Service
print(client)
# Step 3: Call a SOAP Method
result = client.service.say_hello('John')
# Step 4: Handle Response
print(result)
This example uses a SOAP service that has a method named say_hello
, which takes a parameter and returns a greeting. This code is functional by replacing the URL https://www.example.com/soap-service?wsdl
with the actual WSDL file URL of your SOAP service to start working with it in Python!
Using Python to Handle Complex Data Types
from suds.client import Client
# Step 1: Create a SOAP Client
url = 'https://www.example.com/complex-service?wsdl'
client = Client(url)
# Step 2: Explore the Service
print(client)
# Step 3: Call a SOAP Method with Complex Type
person_data = {'name': 'Alice', 'age': 30, 'city': 'Wonderland'}
result = client.service.process_person_data(person_data)
# Step 4: Handle Response
print(result)
The Python code example above demonstrates a SOAP service with a method called process_person_data
which takes a complex type as a parameter.
Creating SOAP-based Web Services Using Python
You can create your very own SOAP-based web service (or SOAP API) with the Python language. Begin by installing the spyne
library on Bash
pip install spyne
Next, you should create a new Python file. As this article's example, the file name would be soap_service.py
. Notice that the SOAP API will be defined by sypne
.
from spyne import Application, rpc, ServiceBase, Iterable, Integer, Unicode
class HelloWorldService(ServiceBase):
@rpc(Unicode, Integer, _returns=Iterable(Unicode))
def say_hello(ctx, name, times):
for i in range(times):
yield f"Hello, {name}!"
# Create the application with the specified service
application = Application([HelloWorldService], tns='my_namespace', in_protocol=None, out_protocol=None)
To ensure that your API can run, add the following code below to the file you have made in the previous step.
from wsgiref.simple_server import make_server
if __name__ == '__main__':
server = make_server('0.0.0.0', 8000, application)
server.serve_forever()
Lastly, check if it is working by running the Python script on Bash.
python soap_service.py
This SOAP API should be running on a simple HTTP server with the URL http://0.0.0.0:8000
.
Testing the Python SOAP API Using Apidog
Wondering if the SOAP API you made with Python works? Try using Apidog - a powerful API platform that allows you not only to test but also to debug and create APIs.
In this article, a demonstration of how to test Python SOAP APIs will be provided (Note: This request is different from Python requests!).
Prerequisites for Testing
To test the Python SOAP API, make sure that:
- The Python SOAP API is running already
- Install Apidog by clicking on the button below!
You will also have to choose which account you prefer to log into Apidog.
Steps to Test Python SOAP API on Apidog
Step 1: Create a New Request in Apidog
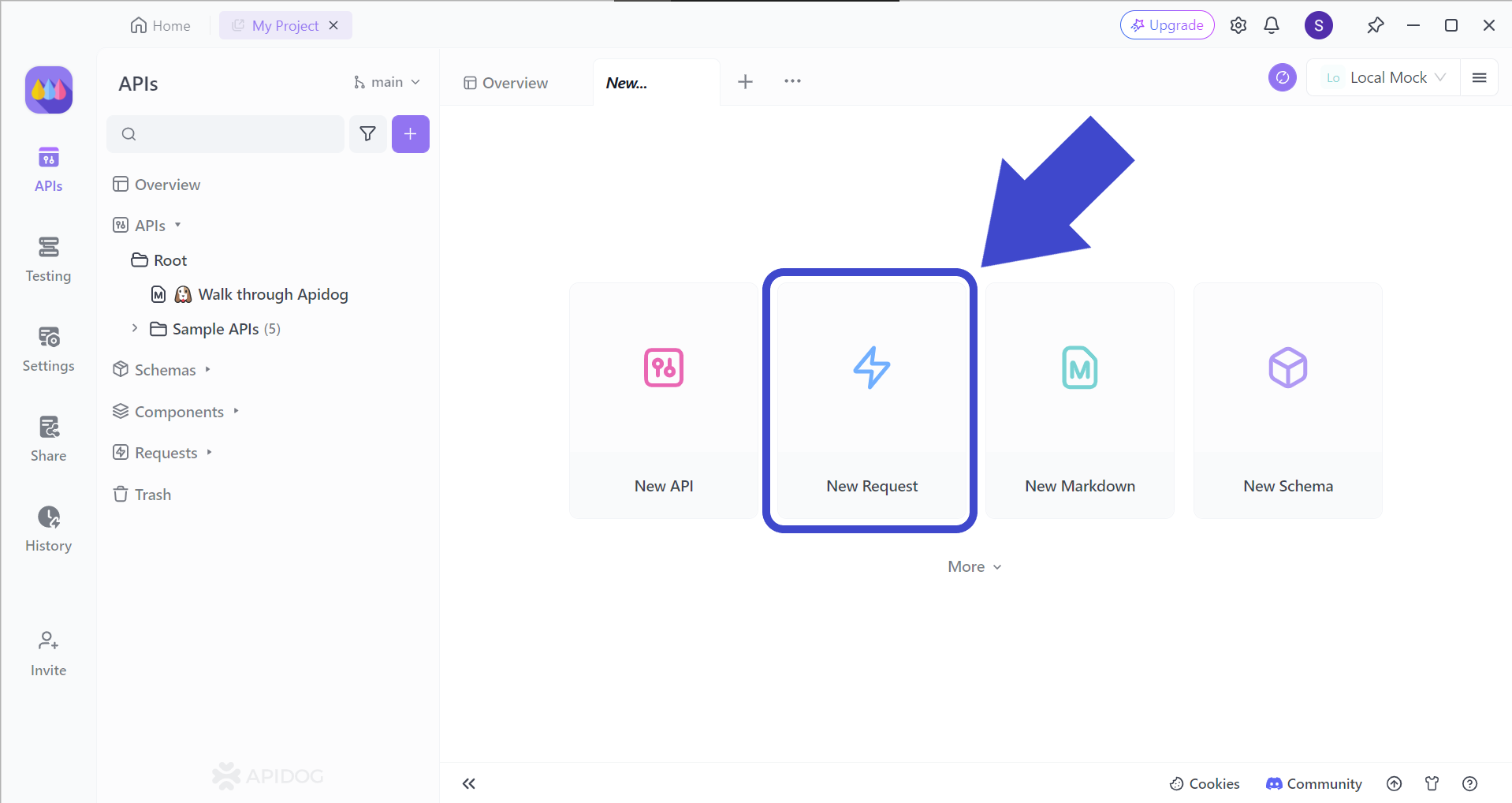
Select the button as shown in the image above.
Step 2: Configure the New Request
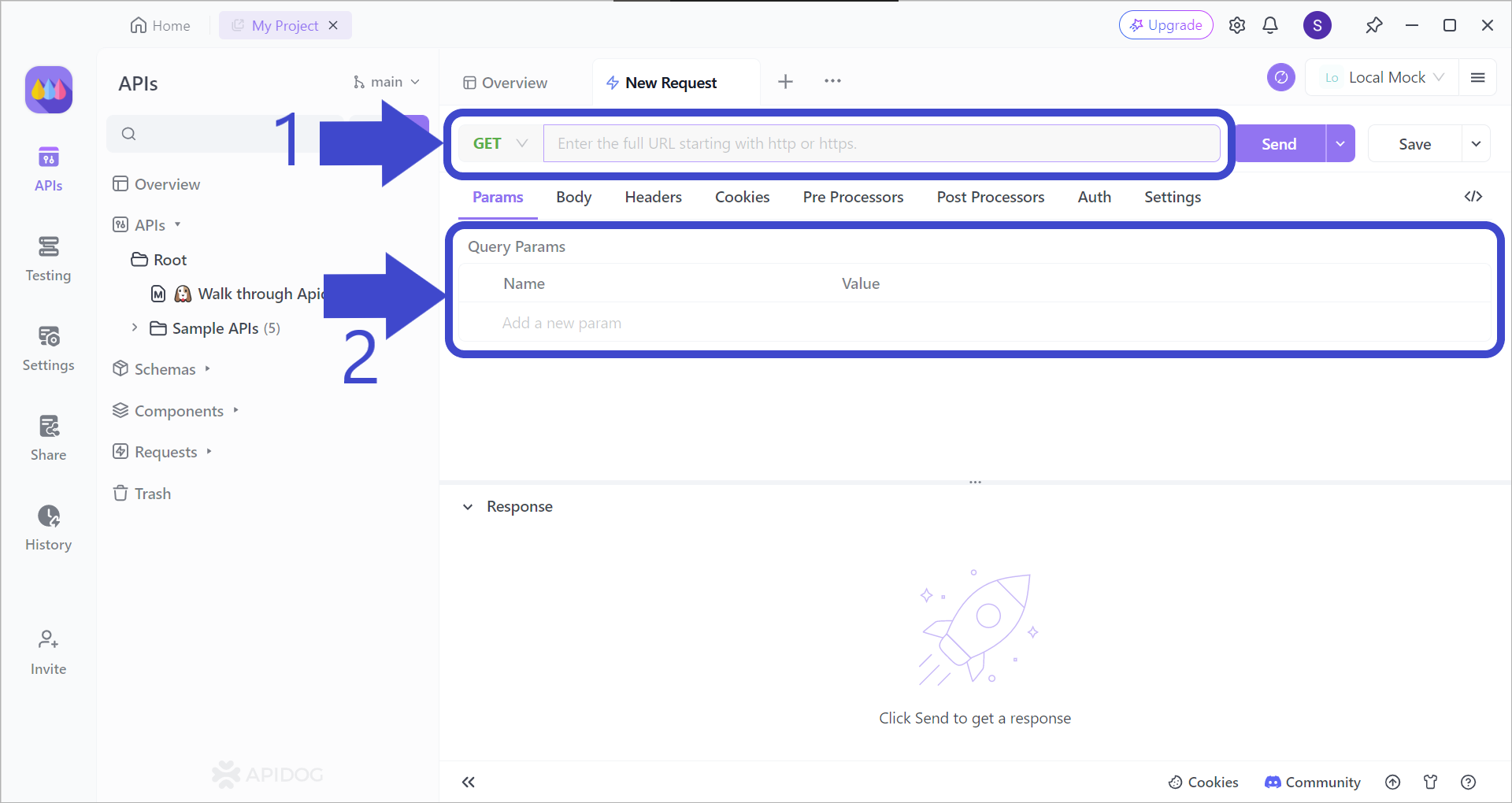
Arrow 1 - Make sure to set the request type to POST as SOAP requests are usually sent via the HTTP POST method. Here, you should also set the Request URL to the endpoint of your running Python SOAP API. An example of this request URL can be http://localhost:8000/
.
Arrow 2 - Add any additional parameters to match your SOAP API.
Step 3 - Include Headers
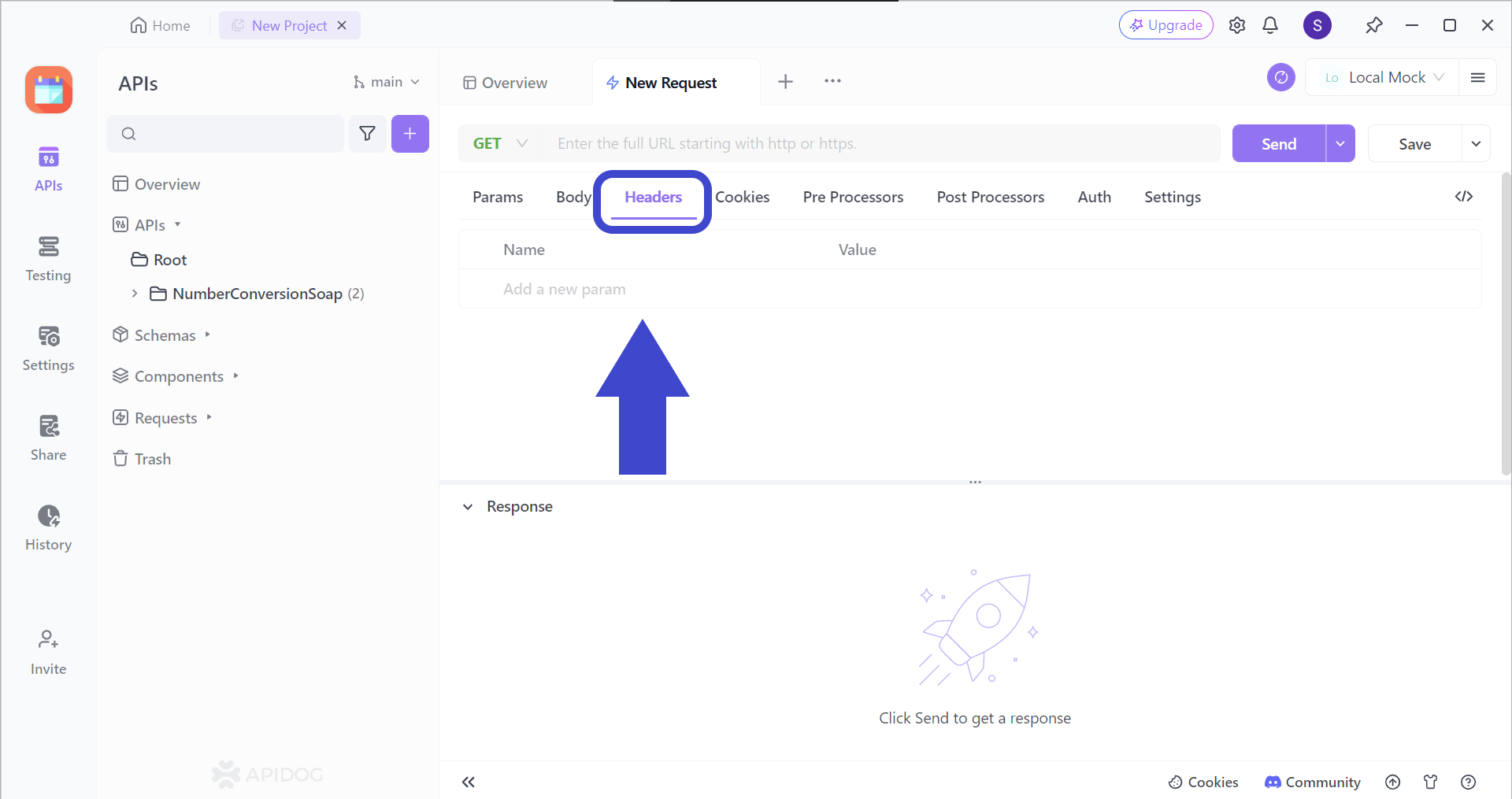
To get to the section above, click the "Headers" button and add the two headers to your request:
Content-Type
: Set this totext/xml
.SOAPAction
: Set this to the SOAP action or method you want to invoke.
Step 4 - Set Request Body
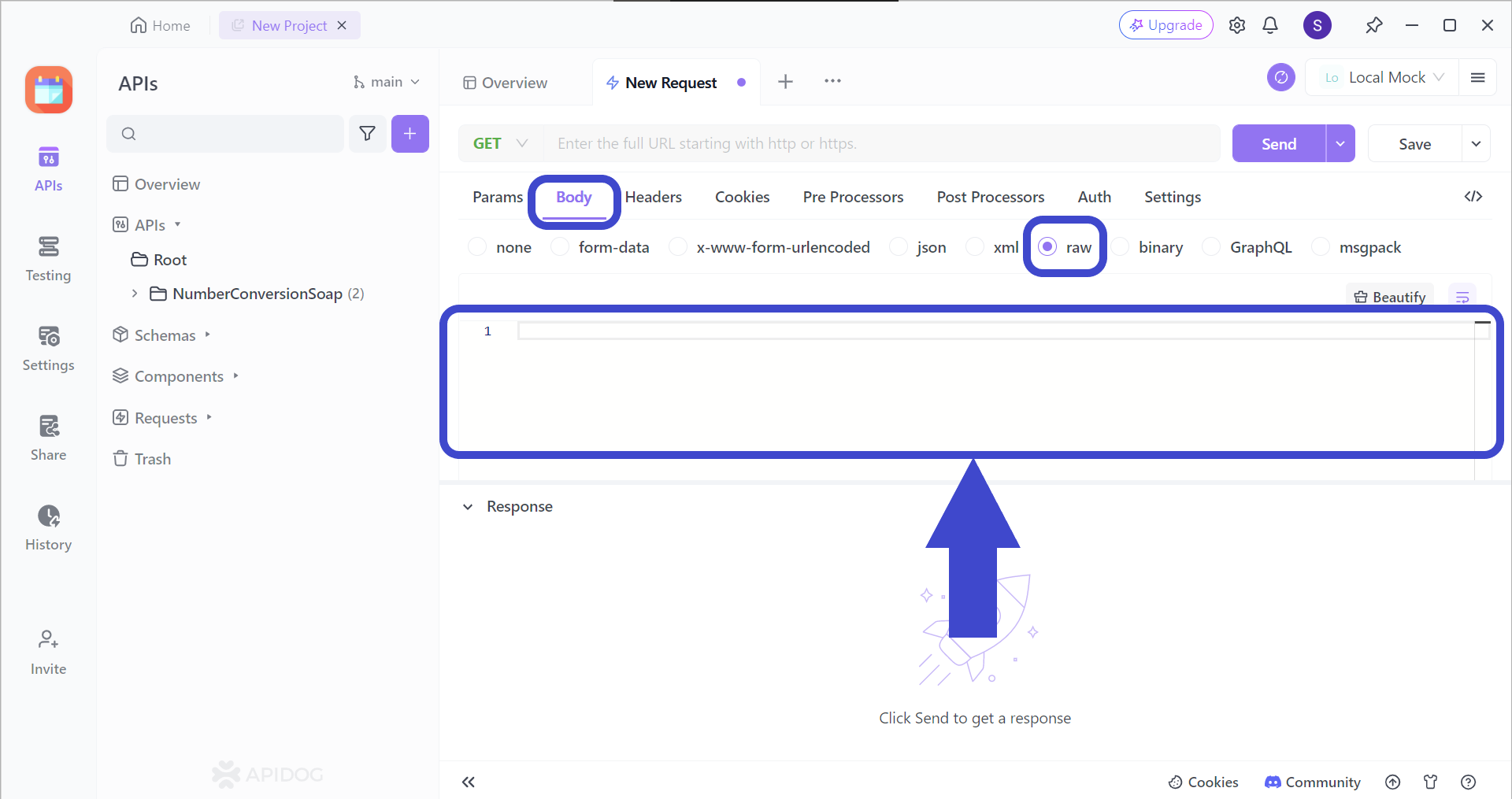
- Under the "Body" section, select the
raw
option. - Construct a SOAP request using the XML format that matches the service method you want to call in the blank space pointed out by the arrow.
An example SOAP request for a method called say_hello
can be added, like such:
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/" xmlns:web="my_namespace">
<soapenv:Header/>
<soapenv:Body>
<web:say_hello>
<name>John</name>
<times>3</times>
</web:say_hello>
</soapenv:Body>
</soapenv:Envelope>
Just make sure that if the code sample above is used, that the namespace (my_namespace
) and method parameters are replaced with your actual service details.
Step 5 - Send Request and Check Response
Once everything is ready, press the "Send" button found around the top right corner of the screen!
If it is successful, you should be able to view the response received. Double-check if the response is what you expect it to be.
Conclusion
Python is a recommended programming that offers lots of flexibility for new and old programmers alike. All you need to do to select an API platform (like Apidog), and you can start testing out your SOAP API's functionality. However, you can of course use Apidog to test Python REST APIs too!