Protobuf vs JSON: Choosing the Right Data Format for Your API
Dive into the world of APIs with our in-depth comparison of Protobuf and JSON. Understand the nuances of each data format and learn how to choose the best for your API needs. Perfect for developers and API enthusiasts alike.
When it comes to APIs, the data format you choose can make a world of difference. It’s like picking the right vehicle for a road trip; you want something reliable, efficient, and suited to the journey ahead. In the world of API data formats, two contenders often come up: Protobuf and JSON. But which one should you use? Let’s chat about that.
Understanding the Basics
Hey there, fellow developers! Let's kick things off by laying the foundation for our epic showdown between Protobuf and JSON. You've probably heard these terms tossed around in the API world, but what do they really mean?
What is JSON?
JSON, or JavaScript Object Notation, is a lightweight data-interchange format that's incredibly easy for humans to read and write. It's essentially a way to represent data using key-value pairs and arrays. JSON has become the de facto standard for APIs across the web, and chances are you've already had your fair share of JSON wrangling.

What is Protobuf?
Enter Protobuf, give code illustration, or Protocol Buffers, Google’s brainchild for serializing structured data. Think of it as the secret agent of data formats – it’s not as ubiquitous as JSON, but it packs a punch with efficiency and speed, especially when dealing with large amounts of data.
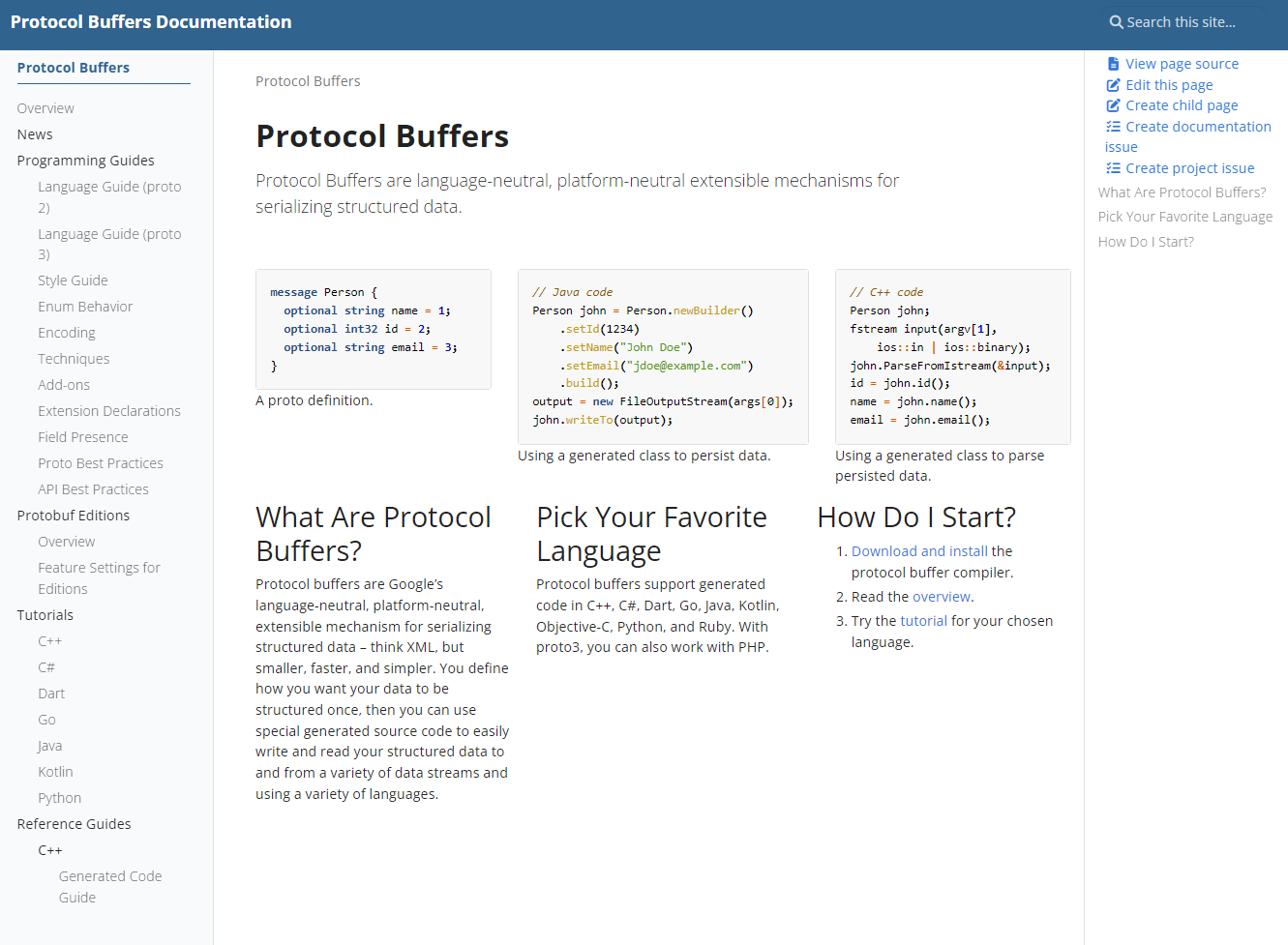
The role of data formats in APIs
In the world of APIs, data formats play a crucial role in how information is transmitted between clients and servers. They define the structure and representation of data, ensuring that both parties can communicate effectively. Whether you choose Protobuf or JSON, the data format you select can have a significant impact on the performance, scalability, and maintainability of your API.
The Battle of Performance
Alright, let's dive into one of the most important aspects of any API: performance. When it comes to speed and efficiency, Protobuf and JSON have some distinct differences that might sway your decision.
Speed and efficiency in data serialization
Serialization is the process of converting data structures into a format that can be transmitted over a network or stored in a file. In the realm of APIs, efficient serialization can mean the difference between lightning-fast responses and sluggish, unresponsive systems.
Protobuf's binary format gives it a significant edge in terms of speed and efficiency. Since it doesn't have to deal with the overhead of parsing human-readable text like JSON, Protobuf can encode and decode data much faster, leading to quicker response times for your API.
Comparing the size: Protobuf vs JSON
Another key factor in API performance is the size of the data payloads being transmitted. Smaller payloads mean less bandwidth consumption and faster data transfer, which is especially crucial for mobile apps or APIs with high-traffic demands.
Here's where Protobuf really shines. Thanks to its compact binary format, Protobuf can often achieve smaller payload sizes compared to JSON. This can translate into significant performance gains, especially for APIs that deal with large amounts of data or have high-throughput requirements.
Code Illustration: Protobuf vs JSON in Action
Now, let’s put theory into practice with a bit of code. Below is a simple Python example that illustrates the serialization and deserialization processes for both JSON and Protobuf. This hands-on comparison will give you a clearer picture of how each format operates under the hood.
# JSON Serialization
import json
# Define a simple data structure
data = {
"name": "Copilot",
"type": "AI Assistant",
"features": ["Chat", "Assist", "Learn"]
}
# Serialize to JSON
json_data = json.dumps(data)
print("JSON Serialized Data:", json_data)
# Deserialize from JSON
deserialized_data = json.loads(json_data)
print("Deserialized Data:", deserialized_data)
# Protobuf Serialization
from google.protobuf.json_format import MessageToJson, Parse
# Assuming we have a Protobuf schema defined as follows:
# message Assistant {
# string name = 1;
# string type = 2;
# repeated string features = 3;
# }
# Create a new instance of the Assistant message (defined in Protobuf)
assistant = Assistant(name="Copilot", type="AI Assistant", features=["Chat", "Assist", "Learn"])
# Serialize to Protobuf
protobuf_data = assistant.SerializeToString()
print("Protobuf Serialized Data:", protobuf_data)
# Deserialize from Protobuf
new_assistant = Assistant()
new_assistant.ParseFromString(protobuf_data)
print("Deserialized Data:", MessageToJson(new_assistant))
This example demonstrates how to serialize and deserialize data using both JSON and Protobuf in Python. Note that for Protobuf, you need to have a predefined schema and the generated classes from that schema to serialize and deserialize your data. JSON, on the other hand, is more flexible and doesn’t require a predefined structure.
Remember, this is just a basic illustration. In real-world applications, you would have more complex data structures and additional considerations for performance, security, and compatibility.
Ease of Use and Flexibility
While performance is undoubtedly important, we can't ignore the human aspect of API development. After all, developers are the ones who'll be working with these data formats on a daily basis.
The learning curve for developers
Let's be real: JSON is a walk in the park for most developers. Its straightforward syntax and key-value pairs make it incredibly easy to read, write, and understand. Even if you're new to the game, you can probably grasp JSON in a matter of minutes.
Protobuf, on the other hand, comes with a bit of a learning curve. You'll need to define your data structures using Protobuf's schema language, which can take some getting used to. However, once you've got the hang of it, Protobuf's strong typing and well-defined structure can make your life a whole lot easier in the long run.
Language support and ecosystem
Another factor to consider is the ecosystem and language support for each data format. JSON has been around for ages and has excellent support across virtually every programming language and environment. Whether you're working with Python, JavaScript, Java, or any other language, you'll find a plethora of JSON libraries and tools at your disposal.
Protobuf, while widely adopted by tech giants like Google, Facebook, and Dropbox, has a more niche following. That said, it does have official support for popular languages like Java, C++, Python, and Go, as well as third-party libraries for languages like JavaScript and Ruby.
API documentation with Apidog
Speaking of developer experience, let's talk about API documentation. Clear, well-organized documentation can make or break the adoption of your API, and that's where tools like Apidog come into play.
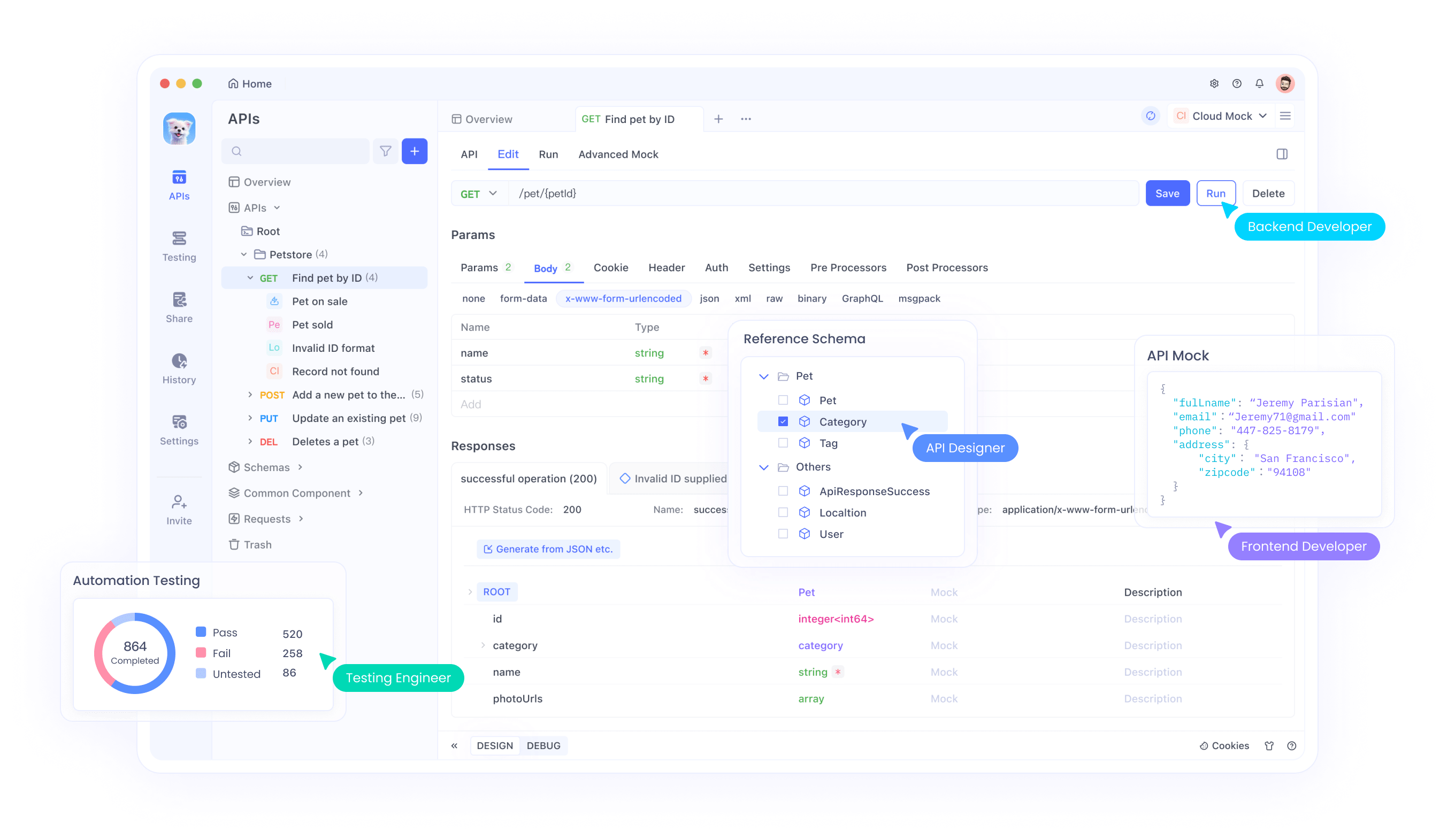
Apidog is a powerful platform that helps you design, test, and document your APIs with ease. With Apidog, you can quickly prototype and experiment with different data formats, test your APIs against various scenarios, and generate beautiful documentation – all in one place. Whether you're team Protobuf or team JSON, Apidog has got your back, making it easier for developers to understand and work with your API.
Reliability and Maintenance
In the world of APIs, reliability and maintainability are key. After all, you want your API to be rock-solid and able to evolve with changing needs over time.
Error handling and data integrity
One area where Protobuf really shines is error handling and data integrity. Thanks to its strong typing and schema-based approach, Protobuf can catch potential data issues during development, before they ever make it to production.
With JSON, on the other hand, you're more likely to encounter runtime errors or data integrity issues, since there's no built-in type checking or schema validation. This can lead to more bugs and harder-to-catch issues down the line.
Versioning and backward compatibility
Another important consideration is how well each data format handles versioning and backwards compatibility. In the API world, you'll often need to evolve your data structures over time, and you'll want to do so without breaking existing clients or servers.
Protobuf's schema-based approach and backwards compatibility features make it easier to evolve your API over time. You can add or remove fields from your data structures without breaking existing clients or servers, as long as you follow Protobuf's versioning guidelines.
JSON, on the other hand, lacks built-in versioning mechanisms, which means you'll need to implement your own versioning strategies to ensure backwards compatibility. This can be a pain point for APIs with complex data structures or frequent updates.
Security Considerations
Last but certainly not least, let's talk about security. In the world of APIs, ensuring the confidentiality and integrity of your data is paramount.
Data encryption and security features
Both Protobuf and JSON can be used in combination with various encryption and security protocols to ensure secure API communication. However, Protobuf does have some built-in security features that can make life a little easier.
For example, Protobuf supports encryption at the message level, which means you can encrypt individual messages or fields within a message. This can be useful for scenarios where you need to selectively encrypt sensitive data, while leaving non-sensitive data in plaintext for performance reasons.
Best practices for secure API communication
Regardless of which data format you choose, it's important to follow best practices for secure API communication. This includes using secure protocols like HTTPS, implementing proper authentication and authorization mechanisms, and following industry-standard encryption practices.
It's also a good idea to regularly audit your API for potential security vulnerabilities and stay up-to-date with the latest security best practices and guidelines.
So, there you have it, folks! We've covered the basics of Protobuf and JSON, their performance characteristics, ease of use, reliability, and security considerations. By now, you should have a pretty good idea of which data format might be the best fit for your API needs.
Remember, there's no one-size-fits-all solution. The best approach is to carefully evaluate your requirements, weigh the pros and cons of each format, and make an informed decision that aligns with your project goals and constraints.
And if you're still feeling a little lost in the API wilderness, don't worry! Tools like Apidog are here to help you design, test, and document your APIs with ease, regardless of whether you're team Protobuf or team JSON.
Conclusion
Choosing between Protobuf and JSON for your API is a strategic decision that depends on your specific needs. Whether you prioritize performance, ease of use, or security, both formats have their merits. Remember, the right choice is the one that aligns with your API’s roadmap and ensures a smooth ride for your data.
With APIdog, you can quickly prototype and experiment with different data formats, test your APIs against various scenarios, and generate beautiful documentation – all in one place.
So, whether you're team Protobuf or team JSON, APIdog has got your back. Check it out and take the hassle out of API development!