If you are a web developer, you probably know how important it is to communicate with APIs. APIs are the interfaces that allow different applications to exchange data and perform actions. One of the most common ways to interact with APIs is by using HTTP requests. HTTP requests are messages that follow a specific format and protocol, and they can be used to send and receive data from a server.
There are different types of HTTP requests, such as GET, POST, PUT, PATCH, and DELETE. Each one has a different purpose and meaning. In this blog post, we will focus on the DELETE request, which is used to remove a resource from a server. We will learn how to make DELETE request in Node.js, a popular JavaScript runtime environment that allows you to run JavaScript code on the server-side.
By the end of this blog post, you will have a solid understanding of how to make delete request in Node.js and how to work with APIs effectively. Let's get started!
What is a DELETE Request and When to Use It
A DELETE request is one of the HTTP methods that are used to communicate with APIs. As the name suggests, a DELETE request is used to delete a resource from a server. A resource can be anything that is identified by a unique URL, such as a user, a post, a comment, a file, etc.
A DELETE request is usually sent with a URL that specifies the resource to be deleted. For example, if you want to delete a user with the id of 123 from an API, you might send a DELETE request to the URL https://example.com/api/users/123. The server will then process the request and return a response indicating whether the deletion was successful or not.
A DELETE request is typically used when you want to remove something that is no longer needed or relevant. For example, you might use a DELETE request to:
- Delete a user account that is inactive or spammy
- Delete a post or a comment that is inappropriate or offensive
- Delete a file that is outdated or corrupted
- Delete a record that is duplicated or erroneous
However, you should be careful when using a DELETE request, as it is a destructive operation that cannot be undone. Once you delete a resource, it is gone forever. Therefore, you should always make sure that you have the proper authorization and permission to delete a resource, and that you have a backup or a confirmation mechanism in case something goes wrong.
What is Node.Js?
Node.js is a JavaScript runtime environment that allows you to run JavaScript code outside of a web browser. Node.js is based on the Google Chrome V8 JavaScript engine, and it is used for building web applications, especially data-intensive and real-time ones. Node.js also has a large library of modules and packages that you can use to add functionality to your projects.
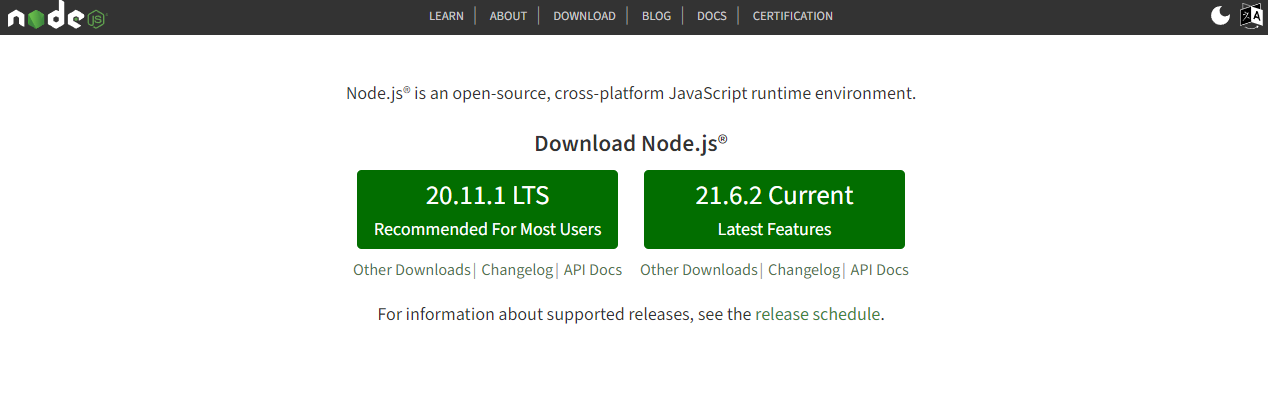
Some of the benefits of Node.js are:
- It is fast and scalable, thanks to its asynchronous and event-driven nature.
- It is cross-platform and open-source, meaning you can run it on various operating systems and contribute to its development.
- It is consistent and unified, as you can use the same language for both the front-end and the back-end of your web application.
How to Make A DELETE Request in Node.js Using the Built-in Http Module
One of the ways to make a DELETE request in Node.js is by using the built-in http module. The http module is a core module that provides low-level functionality for working with HTTP requests and responses. To use the http module, you need to require it in your code, like this:
const http = require('http');
To make a DELETE request using the http module, you need to use the http.request() method. This method takes two arguments: an options object and a callback function. The options object contains the details of the request, such as the method, the hostname, the port, the path, the headers, etc. The callback function is executed when the request is completed, and it receives a response object as a parameter.
For example, let’s say we want to make a DELETE request to the URL https://jsonplaceholder.typicode.com/posts/1, which is a fake API that returns dummy data for testing purposes. We can use the following code to do that:
const http = require('http');
// Define the options object
const options = {
method: 'DELETE', // Specify the HTTP method
hostname: 'jsonplaceholder.typicode.com', // Specify the hostname
port: 443, // Specify the port (443 for HTTPS)
path: '/posts/1', // Specify the path
};
// Make the request
const req = http.request(options, (res) => {
// Handle the response
console.log('Status code:', res.statusCode); // Print the status code
console.log('Headers:', res.headers); // Print the headers
// Get the data from the response
let data = '';
res.on('data', (chunk) => {
data += chunk; // Concatenate the chunks
});
// When the response is finished, print the data
res.on('end', () => {
console.log('Data:', data); // Print the data
});
});
// Handle any errors
req.on('error', (err) => {
console.error('Error:', err.message); // Print the error message
});
// End the request
req.end();
How to Make A DELETE Request in Node.js Using the Axios Library
Another way to make a delete request in Node.js is by using the axios library. Axios is a popular and easy-to-use library that allows you to make HTTP requests from Node.js or the browser. Axios has many features and options that make working with APIs simpler and more convenient. To use axios, you need to install it in your project using npm or yarn, like this:
npm install axios
# or
yarn add axios
To make a DELETE request using Axios, you need to use the axios.delete() method. This method takes two arguments: a URL and an optional config object. The URL is the same as the one you would use for the http module, and the config object can contain additional parameters and headers for the request. The axios.delete() method returns a promise, which you can handle with the then() and catch() methods.
For example, let’s use the same URL as before, https://jsonplaceholder.typicode.com/posts/1, and make a delete request using axios. We can use the following code to do that:
const axios = require('axios');
// Make the request
axios
.delete('https://jsonplaceholder.typicode.com/posts/1')
.then((res) => {
// Handle the response
console.log('Status code:', res.status); // Print the status code
console.log('Headers:', res.headers); // Print the headers
console.log('Data:', res.data); // Print the data
})
.catch((err) => {
// Handle any errors
console.error('Error:', err.message); // Print the error message
});
If you run this code, you should see something similar to this in the console:
Status code: 200
Headers: {
date: 'Tue, 05 Mar 2024 12:45:47 GMT',
'content-type': 'application/json; charset=utf-8',
'content-length': '2',
connection: 'close',
'x-powered-by': 'Express',
'x-ratelimit-limit': '1000',
'x-ratelimit-remaining': '998',
'x-ratelimit-reset': '1614938747',
vary: 'Origin, Accept-Encoding',
'access-control-allow-credentials': 'true',
'cache-control': 'no-cache',
pragma: 'no-cache',
expires: '-1',
'access-control-expose-headers': 'X-Json-Placeholder-User-Id',
'x-json-placeholder-user-id': '1',
etag: 'W/"2-vyGp6PvFo4RvsFtPoIWeCReyIC8"',
via: '1.1 vegur',
'cf-cache-status': 'DYNAMIC',
'cf-request-id': '08a9f9c9f40000e6b4b3f0a000000001',
'expect-ct': 'max-age=604800, report-uri="https://report-uri.cloudflare.com/cdn-cgi/beacon/expect-ct"',
'report-to': '{"group":"cf-nel","endpoints":[{"url":"https:\\/\\/a.nel.cloudflare.com\\/report?s=Q%2BZL0U%2FJ9%2F5i2coL0wvYcQLLmZvIML2Zzjwqk3Iw0%2B3U%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F
How to Make A DELETE Request in Node.js Using the Apidog
Apidog is an API tool that simplifies the process of creating and testing APIs in Node.js. It allows you to define your API endpoints using a declarative syntax, and it automatically generates documentation, validation, and testing your API.
Here is how you can use Apidog to test your DELETE request:
- Open Apidog and create a new request.
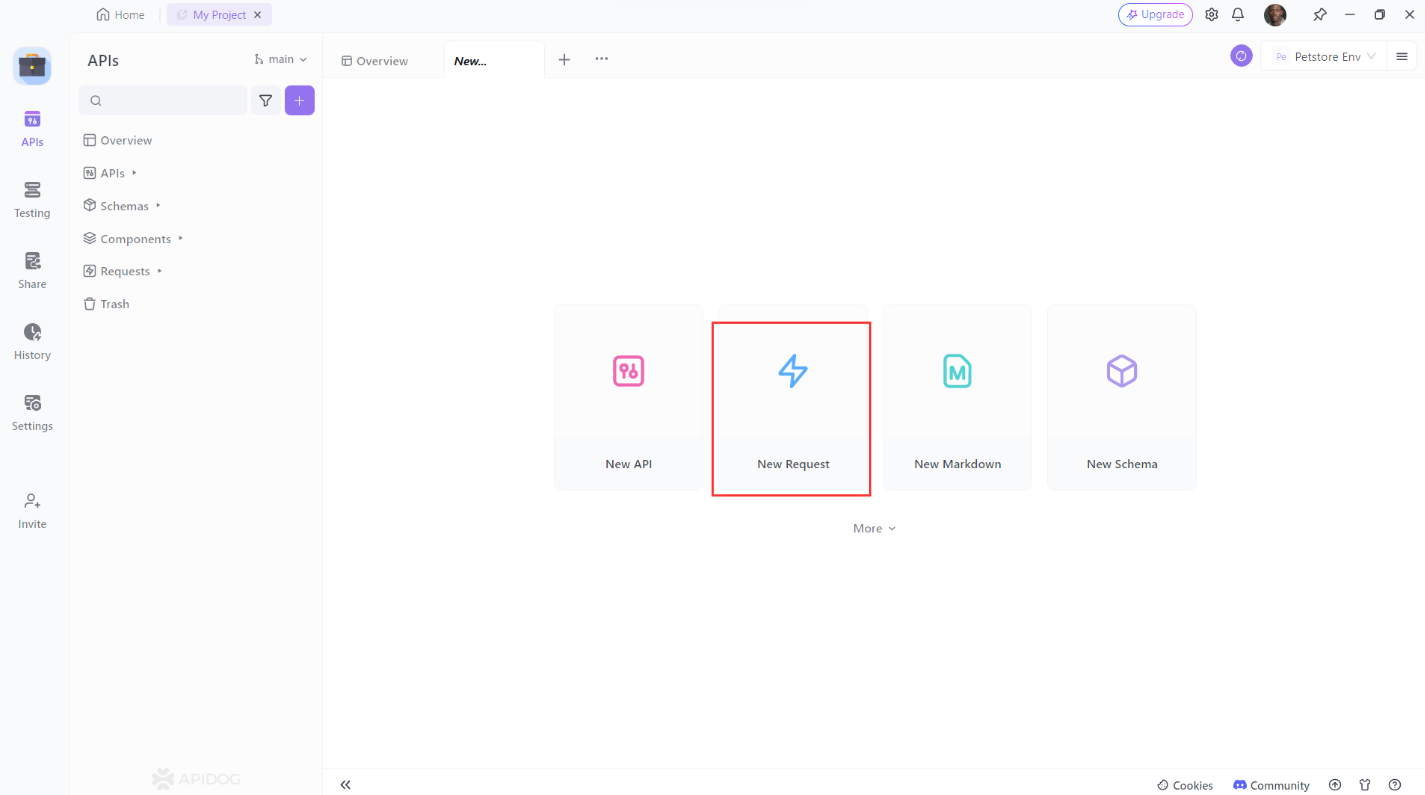
2. Set the request method to HTTP DELETE .
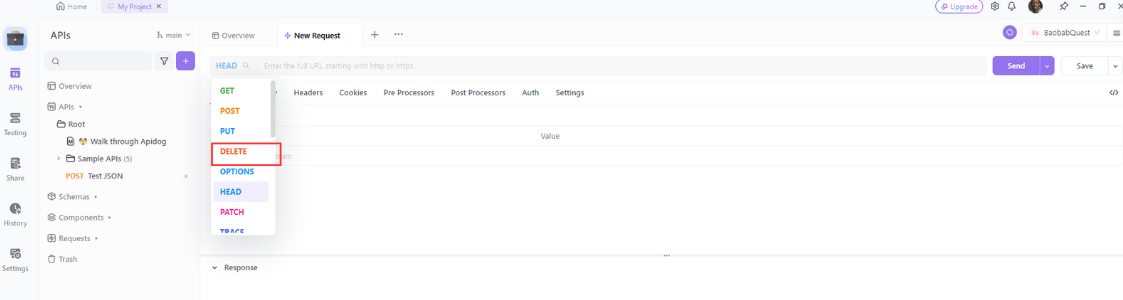
3. Enter the URL of the resource you want to update. Add any additional headers or parameters you want to include then click the “Send” button to send the request.

4. Verify that the response is what you expected.
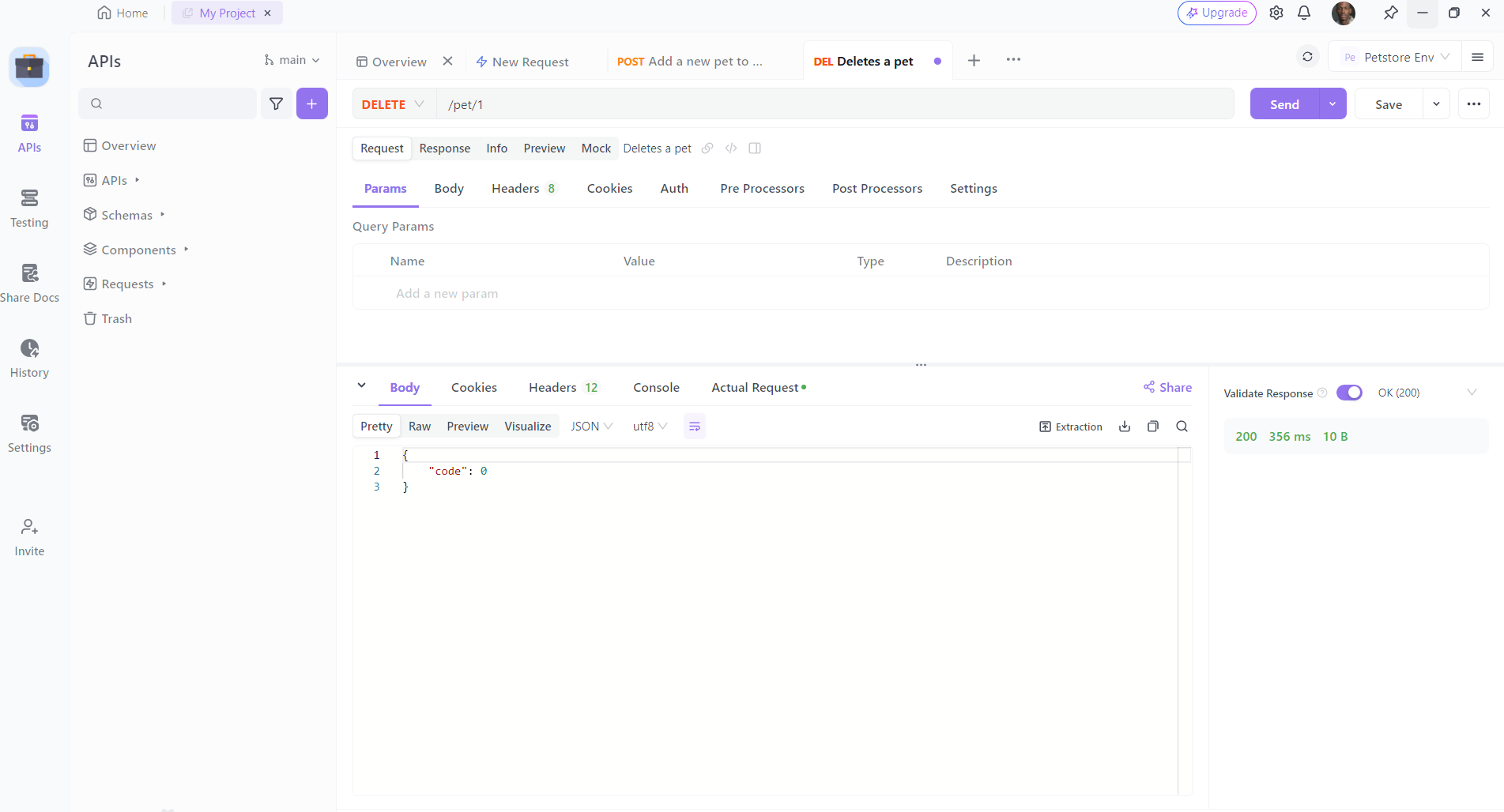
Conclusion
In this blog post, we have learned how to make DELETE request in Node.js using three different ways: the built-in http module, the Axios library, and the Apidog library. We have seen the advantages and disadvantages of each method, and how to handle errors and responses from a DELETE request. We have also learned some tips and best practices for making DELETE requests in Node.js and working with APIs effectively.