How to Use Foreach Loop (An Ultimate Tutorial)
Loops are essential for effectively carrying out repetitive operations in the vast programming field. One such loop recognized for its adaptability and simplicity is the 'foreach' loop.
Loops are essential for effectively carrying out repetitive operations in the vast programming field. One such loop recognized for its adaptability and simplicity is the 'foreach' loop.
Widely used in many programming languages, the foreach loop provides an advanced method for iterating across collections of elements. This article will examine the syntax of the foreach loop, its various implementations in programming languages, and its application in Apidog.
Understanding the ForEach Loop:
Programmers frequently utilize the ForEach loop construct to iterate through components inside a collection, providing a simple and expressive way to handle data sequences. The ForEach loop eliminates the need to manage indices or counters, allowing it to focus on the components instead of typical loops.
Syntax of Foreach Loop in Different Programming Languages:
Programming languages might differ significantly in the syntax of a foreach loop, but the basic structure is always the same. Here is a generic representation:
for element in iterable:
foreach ($iterable as $element) {
}
for (dataType element : iterable) {
}
iterable.forEach(function(element) {
});
Explanation of key components in the code:
Element: Depicts the iteration's active element.
Iterable: Describes the set of items being accessed.
Code block: The series of instructions carried out for every iterable element.
Let's use PHP to explore various applications and demonstrate the usefulness and adaptability of the 'foreach' loop. You might be wondering why I chose PHP over other languages. The explanation is simple: PHP is an excellent programming language for processing data. It is widely used, has a concise syntax, and its 'foreach' loop makes it easier to iterate over arrays.
Applications of Foreach Loop PHP
List iteration:
$programming_languages = ["Python", "Java", "JavaScript"];
foreach ($programming_languages as $language) {
echo $language . "\n";
}
Explanation:
List iteration utilizing a foreach loop to traverse a variety of programming languages is demonstrated in the provided PHP code. The array $programming_languages, which contains three different programming languages, is defined in the first line. Then, the foreach loop assigns the current language to the loop variable $language for each iteration as it works efficiently through all of the elements in the array.
The foreach loop's usefulness for efficient array iteration and output is then illustrated by the echo $language. "\n"; instruction, which outputs each programming language on a novel line. As demonstrated by this example, the foreach loop makes processing array items easier to understand and simpler.
Dictionary iteration:
$programming_language = ["name" => "Python", "release_year" => 1991, "creator" => "Guido van Rossum"];
foreach ($programming_language as $key => $value) {
echo "$key: $value\n";
}
Explanation:
This PHP code demonstrates how to efficiently iterate through the key-value pairs in an associative array using a `foreach` loop, providing an understanding of programming language properties. The first line introduces the associative array $programming_language, which contains the essential attributes such as language name, release year, and creator.
The `foreach` loop then iteratively goes over every key-value pair in the array. The loop variables ‘$key’ and `$value’ automatically assume each iteration's current key and value. The output of each key-value pair is organized and formatted elegantly in the following `echo "$key: $value\n";` command, producing an organized representation.
The output echoes each attribute after execution, highlighting how readable and efficient the `foreach` loop is. This code exemplifies how such a loop construct facilitates fast data handling in programming, making traversing and showing data in associative arrays easier.
File iteration:
$filename = "example.txt";
$file = fopen($filename, "r");
if ($file) {
foreach (file($filename) as $line) {
echo trim($line) . "\n";
}
fclose($file);
} else {
echo "Unable to open file: $filename";
}
Explanation:
The above PHP code uses a `foreach` loop to illustrate a basic file reading procedure. The code opens a file called "example.txt" for reading in the first few lines, and if that succeeds, it uses a `foreach` loop to run over each line in the file. The current line's content is sent to the loop variable $line for each iteration, and the ‘echo trim($line)’ is executed. The "\n" statement ensures a tidy representation by outputting the line without leading or trailing whitespaces.
A check is included in the code to ensure that the file is opened successfully. An error message detailing the problem is printed if the file cannot be opened. This short yet effective PHP script illustrates how to read and display a file's contents using a `foreach` loop, illustrating a typical file handling scenario.
Foreach Loop in Testing: The New Feature of Apidog
Apidog is an integrated platform that facilitates the entire API lifecycle. It is known for its adaptability and user-friendly interface.
Apidog recently introduced a new testing feature called ‘ForEach Loop.’ One crucial aspect of this feature is that it simplifies the process of iterating through lists of items. In this article, we'll explore what the ForEach loop does and how it enhances the testing experience within the Apidog platform.
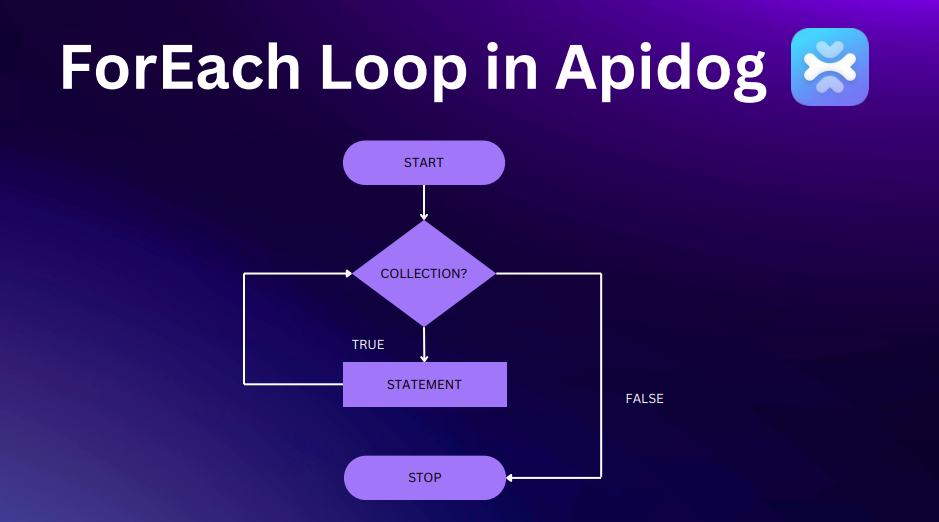
Enhancements in Apidog's Automation Testing
Apidog has recently improved its automated testing capabilities by adding ForEach Loop to improve testing processes.
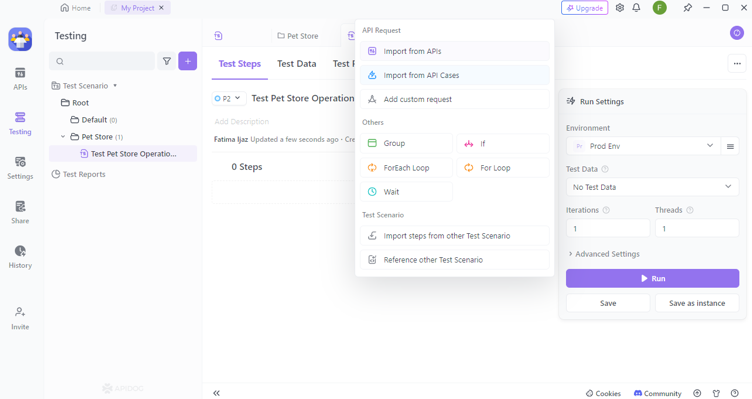
Redesigned Automated Testing Module:
A substantial modification was made to the automated testing module. Most notably, "test cases" are now called "test scenarios," and "test suites" were eliminated. The testing procedure is intended to be improved and streamlined by this reorganization.
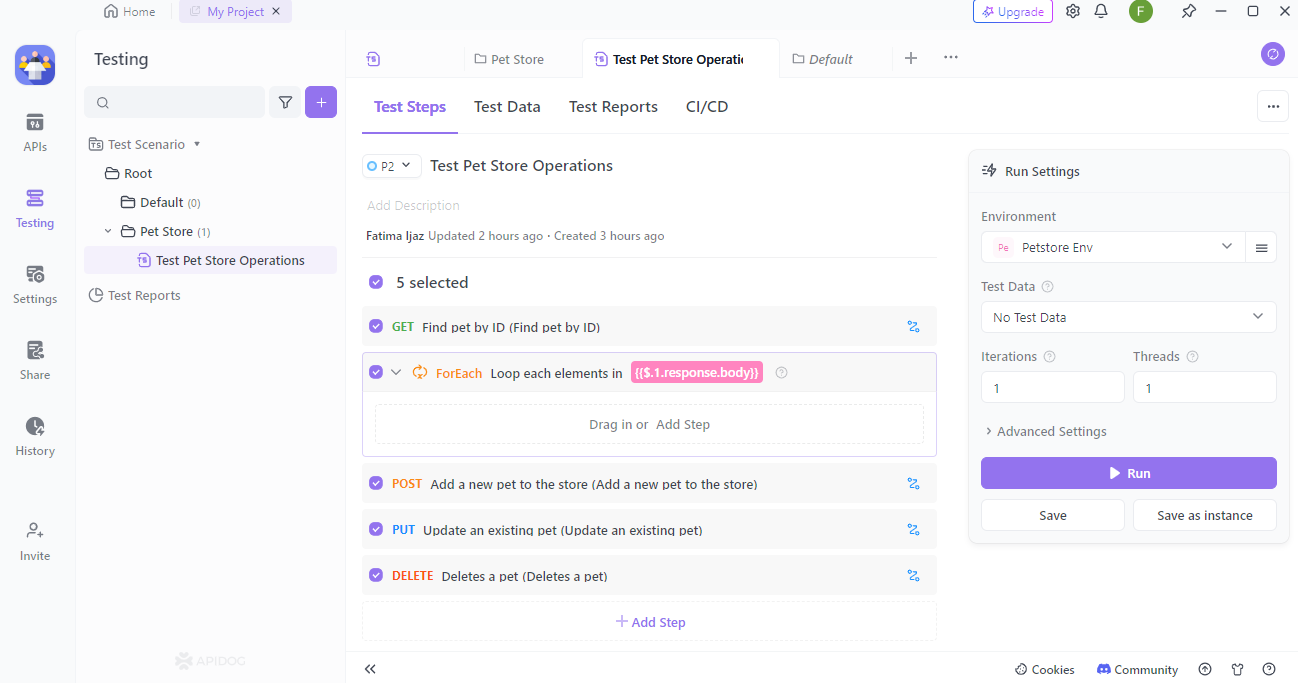
Improved Organization with Directory Tree and Tabbed Pages:
An easier and more structured interface is now provided by presenting test scenarios in a directory tree style with tabbed pages.
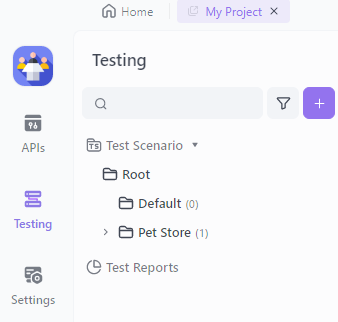
Multiple Test Data Set Support:
Multiple test data sets may now be configured inside a single scenario due to scenario instances, giving testing scenarios more flexibility.
Enhanced Integration of CI/CD:
The code for configuration files for well-known tools like Jenkins and GitHub Actions is now automatically generated by the CI/CD integration. This simplifies the integration process by eliminating the need for manual pipeline coding.
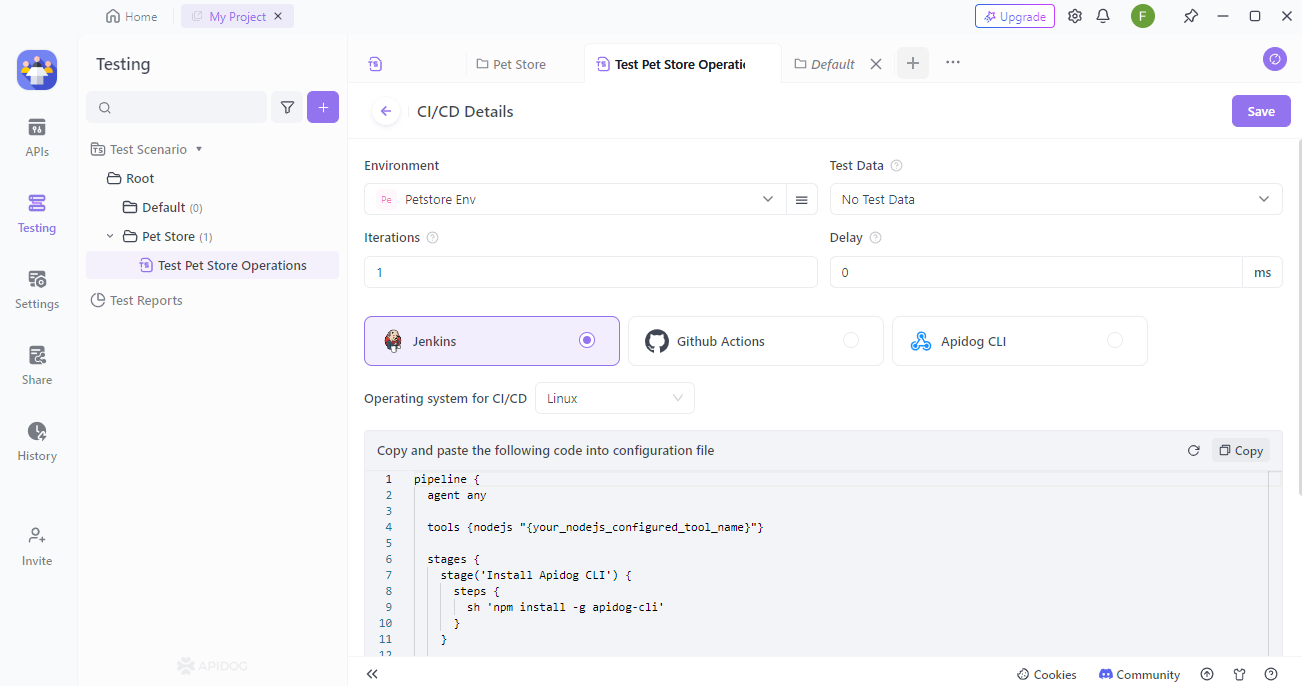
How to Create Test Scenarios in Apidog?
Now, we’ll create a test scenario on apidog and will also use ForEach Loop in this.
A thorough comprehension of the differences between test cases and scenarios is necessary to create effective test scenarios. To create test cases and scenarios, consider the following steps:
Prerequisite:
Apidog is installed on your computer.
Step 1: Log in to your Apidog account and click the '+' button on the test scenario within the 'Testing' page.
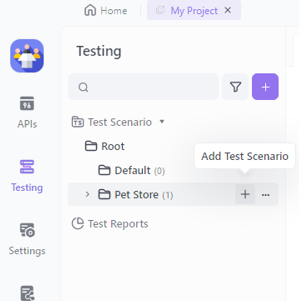
Step 2: Set up the New Test Scenario's parameters, such as the folder name, test scenario name, and priority.
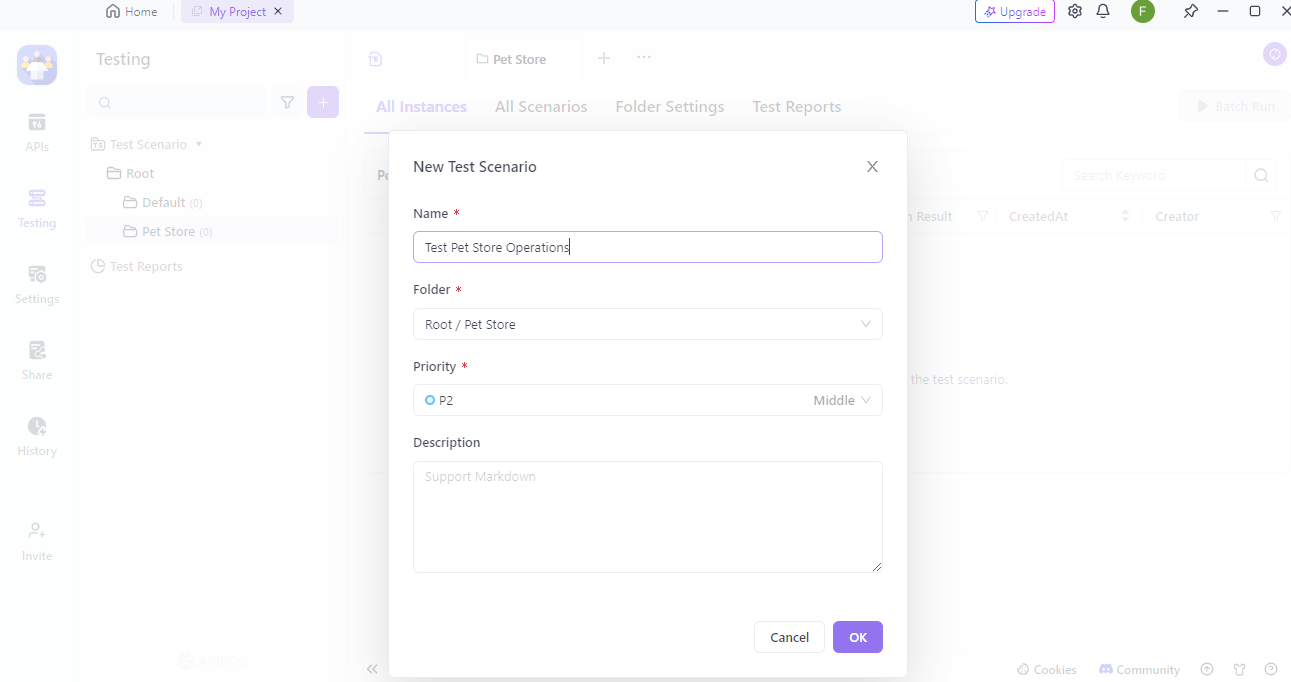
Step 3: Add the test case by clicking on ‘Import from API.’
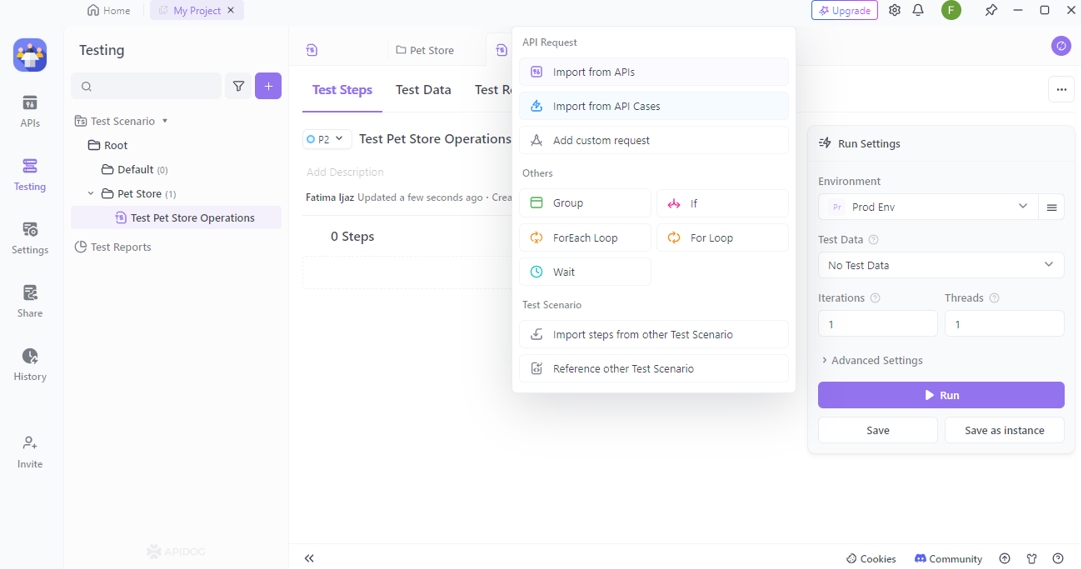
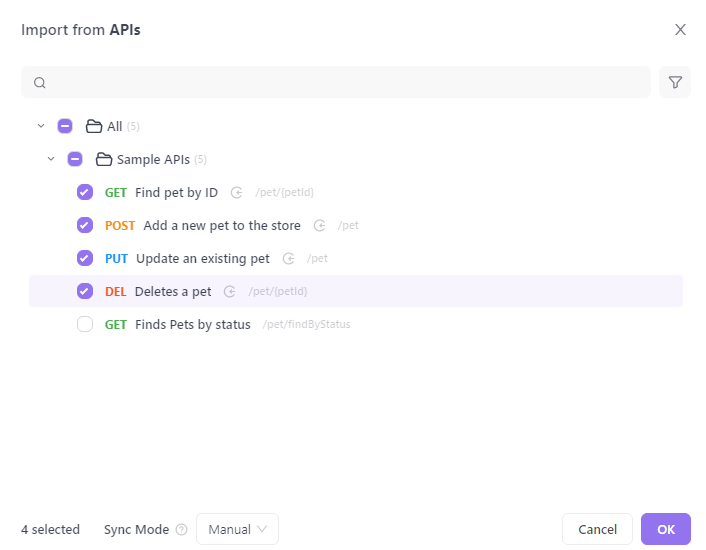
Step 4: Before starting the test, create thorough test cases with all necessary circumstances, steps, input data, intended outcomes, and preconditions or postconditions by clicking on ‘Add Step.’
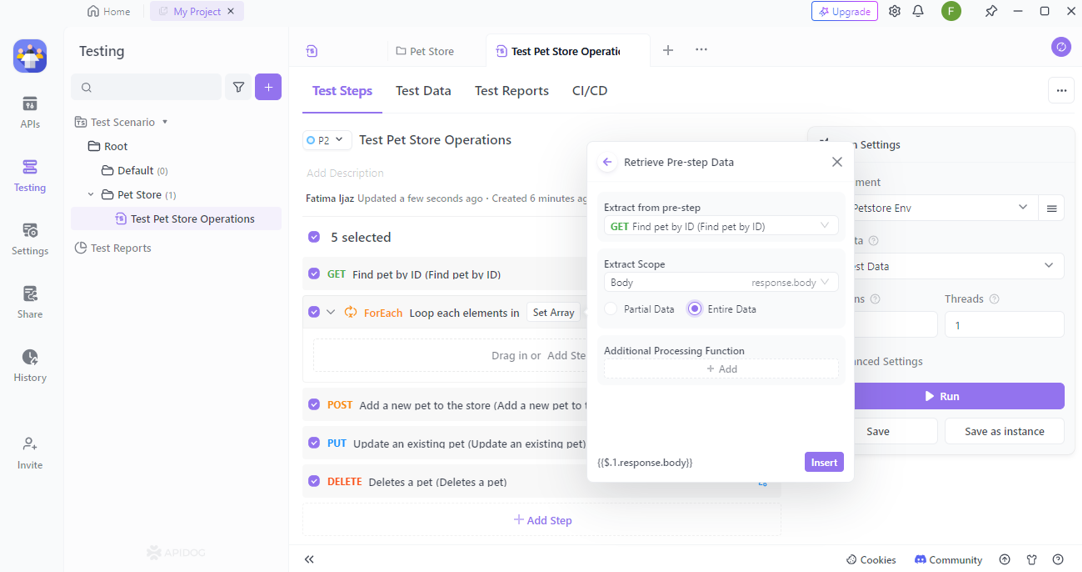
Step 5: Examine the test cases and scenarios to ensure they cover all necessary features and functions. Make sure the test cases accurately reflect the goals of the test scenarios by verifying them.
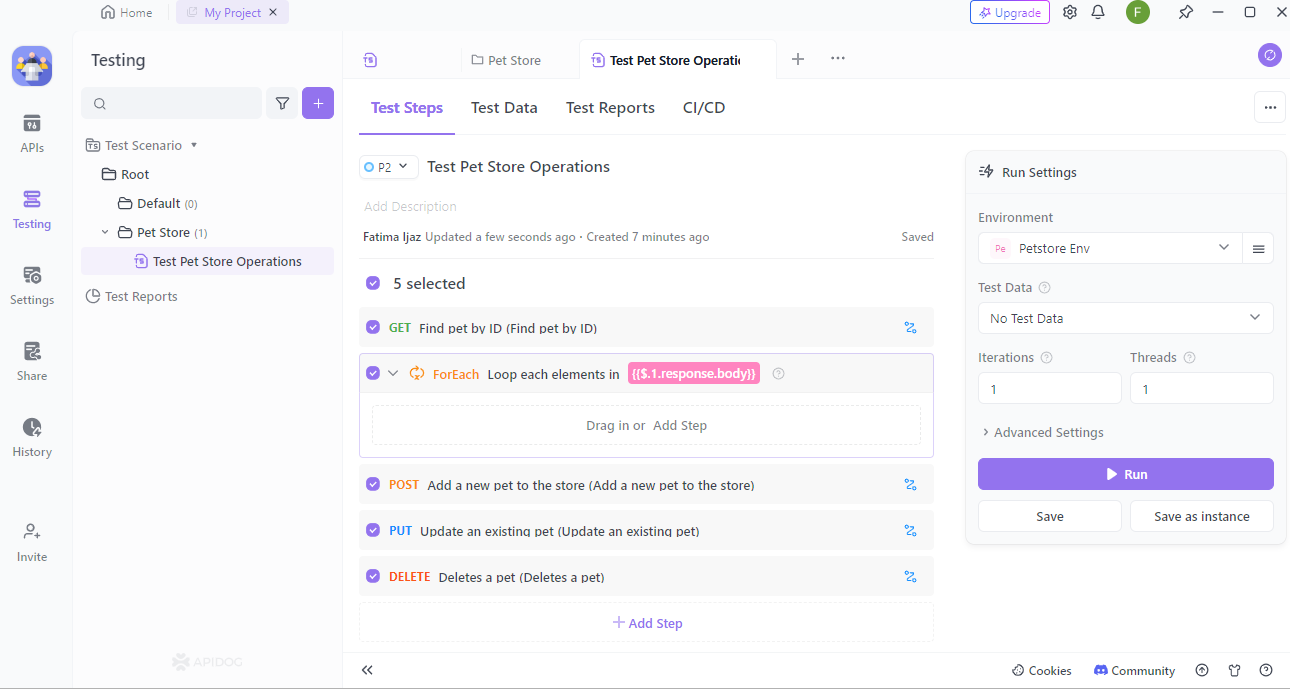
Step 6: Run the test scenarios and track the results. Keep track of the defects or problems found throughout the testing process.
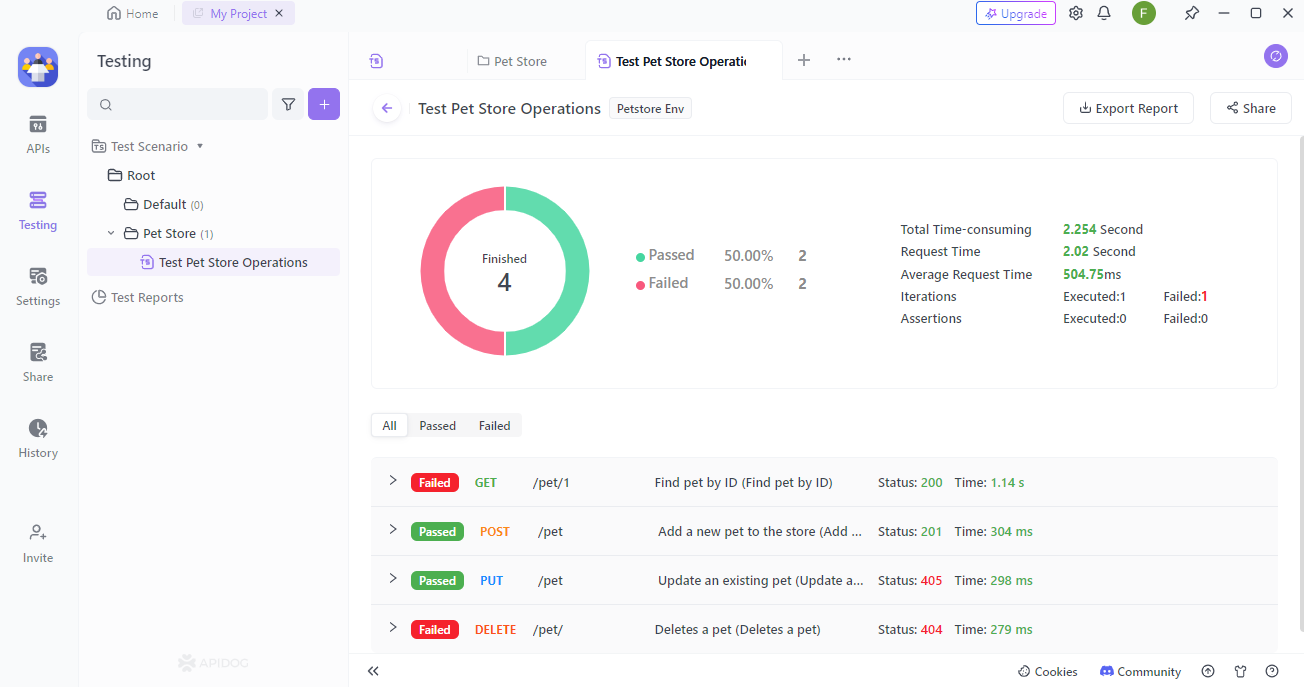
Benefits of ForEach Loop:
In many programming languages, the `foreach` loop is a useful technique that improves code readability, simplicity, and performance. Now, let's explore the advantages of utilizing a `foreach` loop:
Readability: One of the main benefits of utilizing the `foreach’ loop is its improved readability. Because much of the syntax is clear and simple to comprehend, even developers with less programming experience can effortlessly access the code.
Simplicity: Unlike other loop types, the `foreach` loop abstracts away the complexity of keeping indices or counters. This simplicity makes the code easier to understand and less prone to errors by lowering the possibility of off-by-one errors.
Ease in utilizing collections: For iterating over collections, such as arrays, lists, or other iterable objects, the `foreach` loop works very well. It eliminates the need to manually manage indices and iterators, making it easier to traverse the elements of a collection.
Avoidance of Off-by-One errors: The probability of introducing off-by-one errors considerably decreases since the loop automatically manages the iteration and termination conditions.
Adaptability to various data structures: A variety of data structures, such as arrays, lists, sets, maps, and more, can be iterated using the `foreach` loop. This adaptability makes it possible to iterate across various collection types consistently and simply.
Maintainability: Code utilizing `foreach` loops is often easier to maintain over time. The `foreach` loop frequently requires minimum adjustment if the size or structure of a collection changes, making the code easy to extend and maintain.
Clarity of Intent: The code's intent is made unambiguous using a `foreach’ loop to iterate over collection elements. This makes the codebase easier to read and manage overall.
Decreased Boilerplate Code: The ‘foreach’ loop frequently involves less boilerplate code than standard loops, which also entail initializing counters, testing conditions, and incrementing indices. Implementations become clearer and clearer as a result of eliminating unnecessary code.
Consistent Performance: The `foreach` loop in several programming languages is optimized for the data structure it works with, leading to reliable and effective performance. This is particularly valid for iterable collections such as arrays.
Compatibility Across Languages: The `foreach` loop is present in several programming languages with minor syntactic variations and is not exclusive to any of them. Because of this uniformity, developers may transition between languages more easily, and there isn't a big learning curve for fundamental loop constructions.
Conclusion:
In conclusion, testing experts must fully understand the complexities of creating test scenarios, including the innovative use of the ForEach Loop in Apidog. Basic concepts, including well-defined goals and a thorough comprehension of user behavior, have been covered in this tutorial. Adopting the ForEach Loop feature allows testers to traverse through lists of objects more quickly and effectively, which improves the overall testing experience on the Apidog platform.