Hey there, Python enthusiasts! Whether you're a seasoned developer or just starting out, working with APIs is something you can't avoid in today's tech landscape. One crucial aspect of interacting with APIs is knowing how to check the status of your requests. Not only does this help you debug issues, but it also ensures your application behaves as expected. In this blog post, we'll dive deep into checking request statuses in Python. We'll cover everything from the basics to advanced techniques, and by the end, you'll be a pro at handling API responses. Plus, we'll introduce you to Apidog, a fantastic tool to streamline your API development process. Ready? Let's get started!
What are API Requests?
API requests are the way our applications communicate with other services. Think of APIs (Application Programming Interfaces) as bridges that connect different software systems, allowing them to share data and functionality. When you make an API request, you're essentially asking another service to provide you with some information or perform a certain action.
Setting Up Your Python Environment
Before we dive into checking request statuses, let's set up our Python environment. You'll need to have Python installed on your machine. If you haven't done that yet, head over to the official Python website and download the latest version.
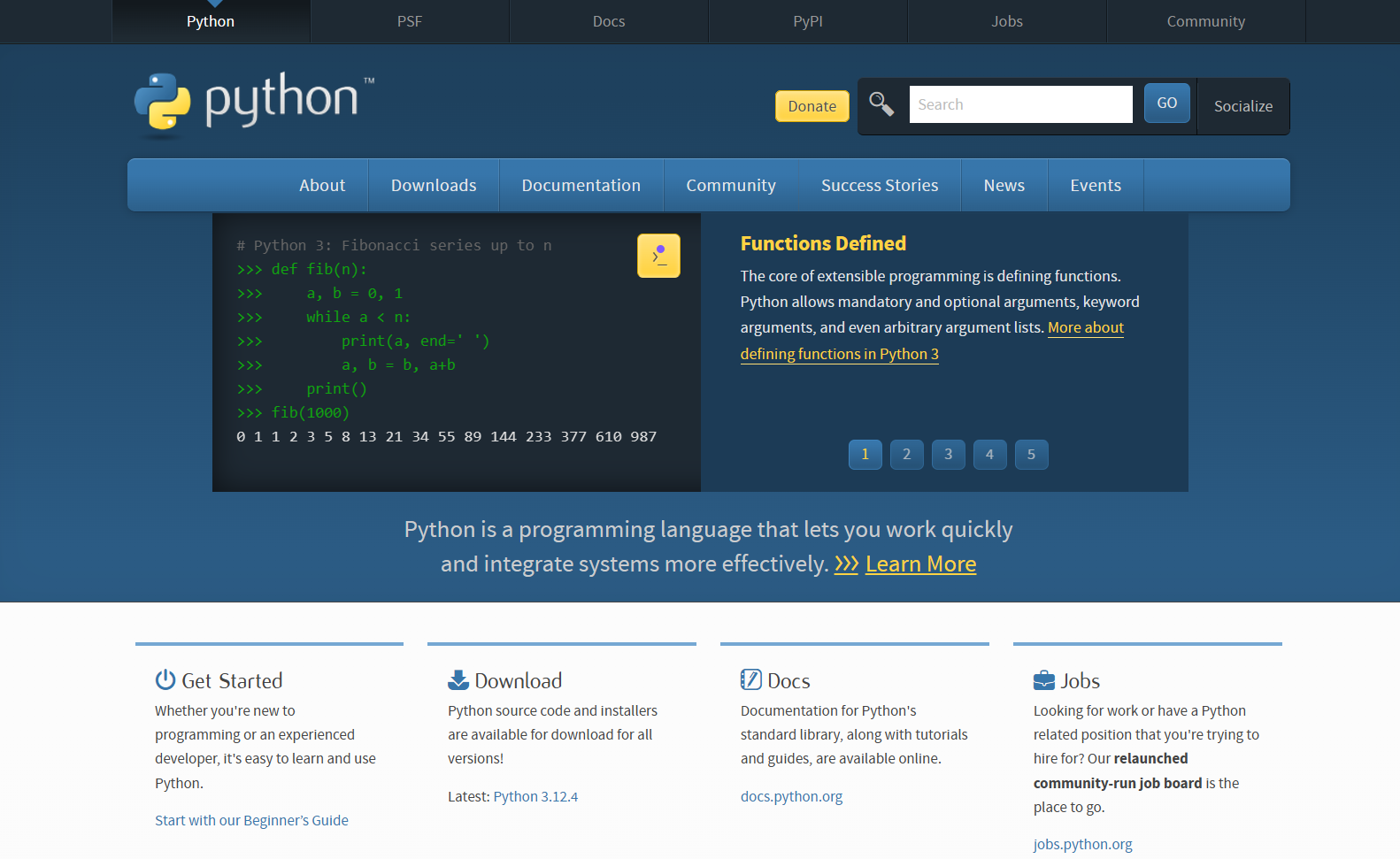
Next, you'll need to install the requests
library. This library makes it incredibly easy to send HTTP requests using Python. Open your terminal or command prompt and run the following command:
pip install requests
Great! Now you're ready to start making API requests.
Making Your First API Request
Let's kick things off with a simple API request. We'll use a public API that provides random jokes. Here's a small snippet of code to get you started:
import requests
response = requests.get('https://official-joke-api.appspot.com/random_joke')
print(response.json())
Run this code, and you should see a random joke printed out. Pretty cool, right?
Checking Request Status
Using the requests
Library
Now, let's focus on the main topic: checking the status of our requests. Every time you make an API request, the server responds with a status code. This code tells you whether your request was successful or if something went wrong.
The requests
library makes it easy to check the status code of a response. Here's how you can do it:
import requests
response = requests.get('https://official-joke-api.appspot.com/random_joke')
print(response.status_code)
This will print out the status code of the response. A status code of 200
means everything went smoothly, while other codes indicate various issues.
Understanding HTTP Status Codes
Let's take a quick look at some common HTTP status codes and what they mean:
- 200 OK: The request was successful, and the server returned the requested data.
- 201 Created: The request was successful, and a new resource was created.
- 400 Bad Request: The server could not understand the request due to invalid syntax.
- 401 Unauthorized: The client must authenticate itself to get the requested response.
- 404 Not Found: The server could not find the requested resource.
- 500 Internal Server Error: The server encountered an unexpected condition that prevented it from fulfilling the request.
Handling Different Status Codes
Understanding how to handle different status codes is crucial for building robust applications. Let's go through some examples.
200 OK
When you receive a 200 OK
status code, it means your request was successful. Here's how you can handle it:
if response.status_code == 200:
print("Request was successful!")
print(response.json())
else:
print("Something went wrong!")
404 Not Found
A 404 Not Found
status code indicates that the requested resource could not be found. You might want to handle this gracefully in your application:
if response.status_code == 404:
print("Resource not found.")
else:
print("Something went wrong!")
500 Internal Server Error
A 500 Internal Server Error
status code means something went wrong on the server side. Here's how you can deal with it:
if response.status_code == 500:
print("Internal server error. Please try again later.")
else:
print("Something went wrong!")
Advanced Techniques for Checking Request Status
Using try-except
for Error Handling
To make your code more robust, you can use try-except
blocks to handle exceptions. This way, your application won't crash if something goes wrong:
try:
response = requests.get('https://official-joke-api.appspot.com/random_joke')
response.raise_for_status() # Raises an HTTPError if the status is 4xx, 5xx
print(response.json())
except requests.exceptions.HTTPError as err:
print(f"HTTP error occurred: {err}")
except Exception as err:
print(f"Other error occurred: {err}")
Implementing Retries for Failed Requests
Sometimes, requests might fail due to temporary issues. Implementing retries can help improve the reliability of your application. The requests
library doesn't support retries out of the box, but you can use the urllib3
library to achieve this:
from requests.adapters import HTTPAdapter
from requests.packages.urllib3.util.retry import Retry
session = requests.Session()
retry = Retry(
total=3, # Total number of retries
backoff_factor=0.1, # Wait time between retries
status_forcelist=[500, 502, 503, 504] # Retry for these status codes
)
adapter = HTTPAdapter(max_retries=retry)
session.mount('http://', adapter)
session.mount('https://', adapter)
try:
response = session.get('https://official-joke-api.appspot.com/random_joke')
response.raise_for_status()
print(response.json())
except requests.exceptions.HTTPError as err:
print(f"HTTP error occurred: {err}")
except Exception as err:
print(f"Other error occurred: {err}")
Integrating Apidog for Better API Management
Now that you know how to check request statuses in Python, let's talk about Apidog. Apidog is an amazing tool that simplifies API development. It provides a suite of features for designing, testing, and managing APIs. With Apidog, you can:
How to send Python API request using Apidog
- Open Apidog and click on the "New Request" button to create a new request.
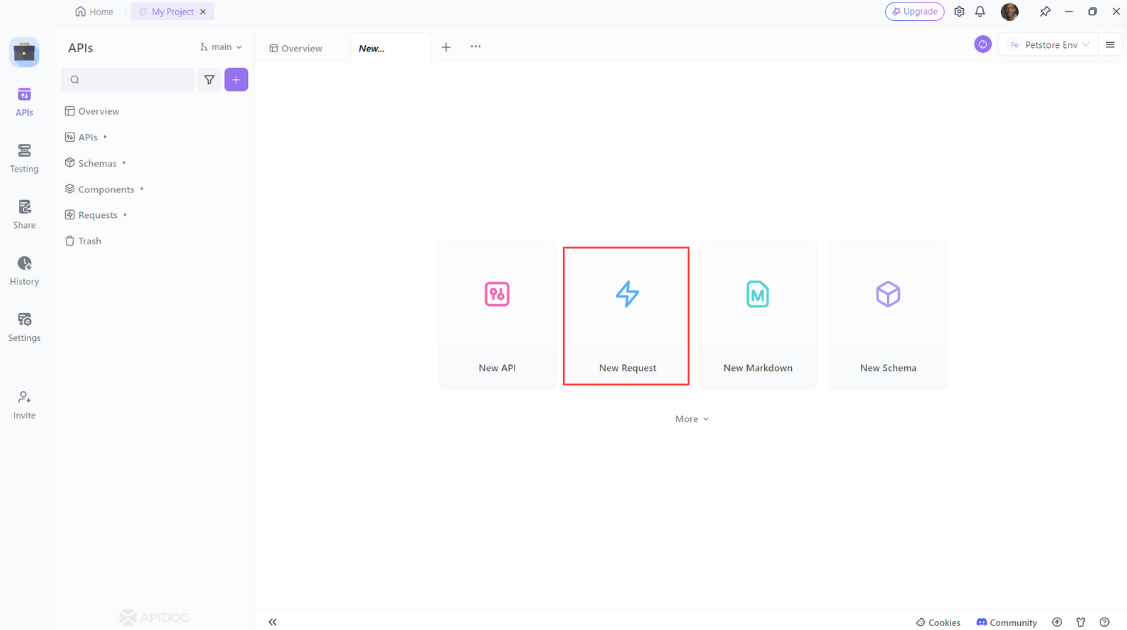
2. Select "GET" as the method of the request.
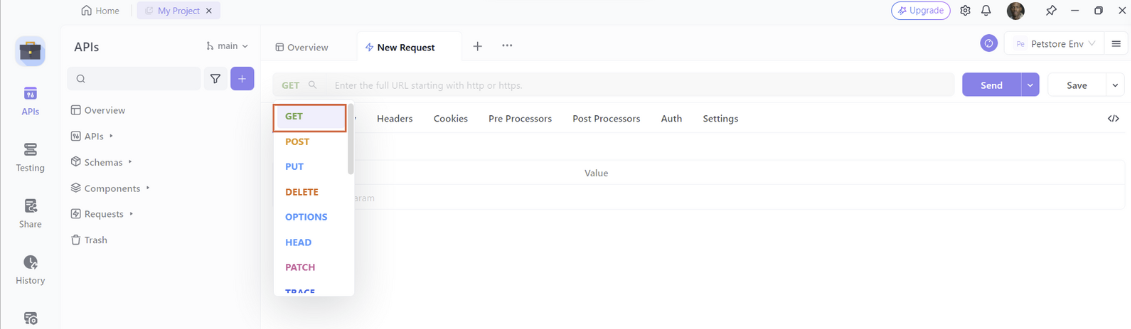
3. Enter the URL of the API endpoint
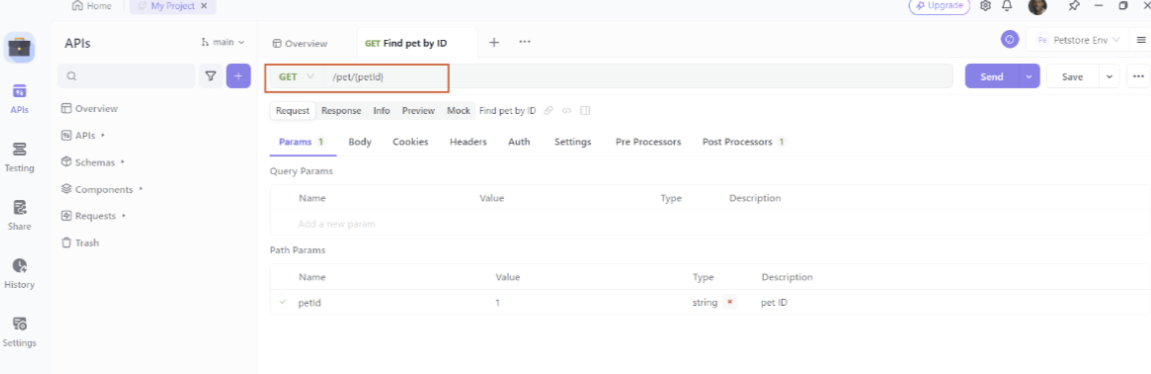
Then click on the “Send” button to send the request to the API.
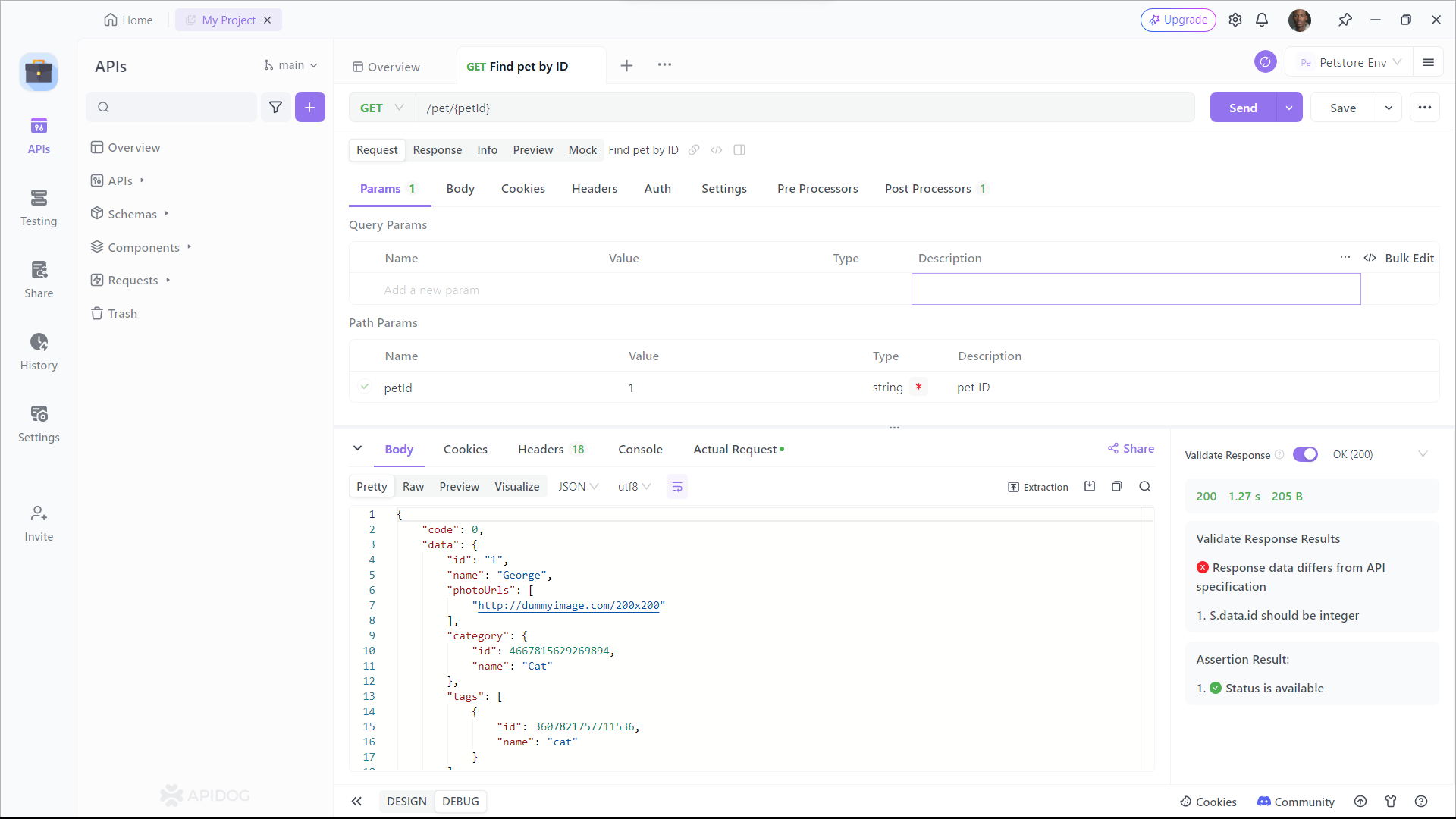
As you can see, Apidog shows you the URL, parameters, headers, and body of the request, and the status, headers, and body of the response. You can also see the response time, size, and format of the request and response, and compare them with different web APIs.
How to make Python automation testing using Apidog
Here’s a step-by-step guide on how to automate API testing using Apidog:
Open your Apidog Project and switch to the testing interface
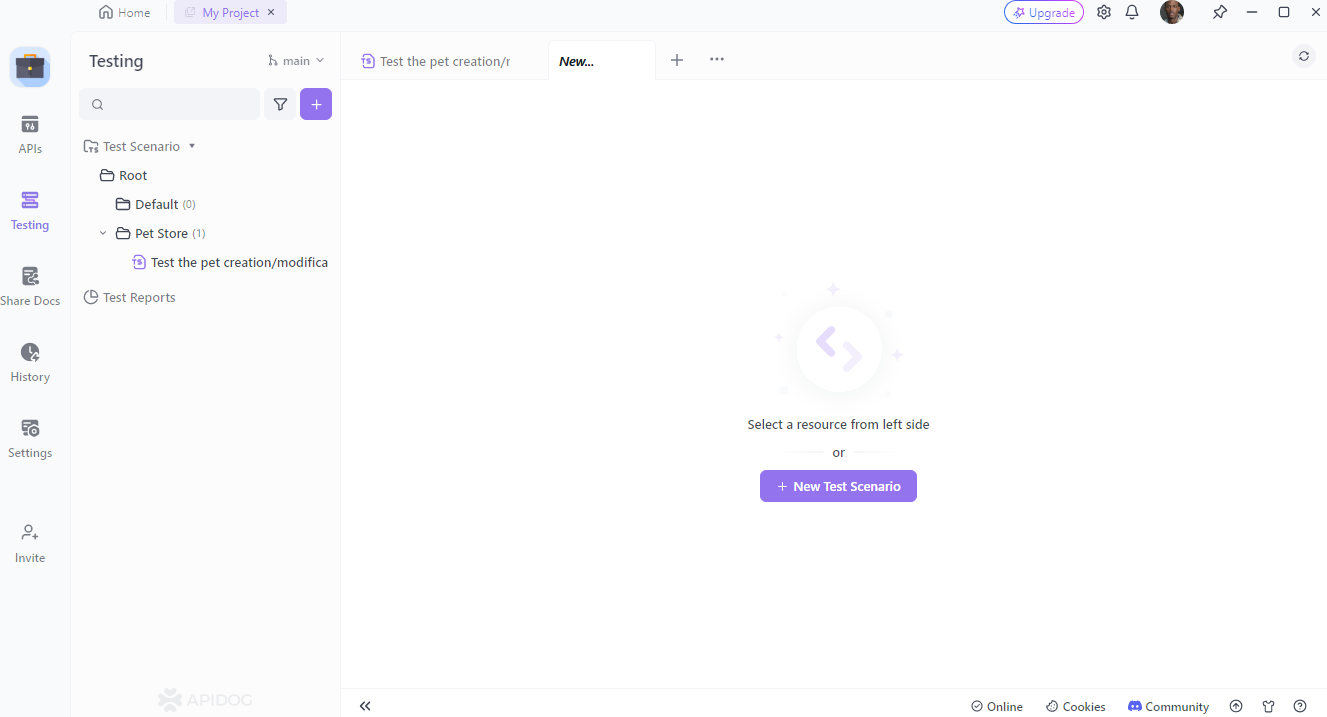
Design Your Test Scenarios: You can design your test scenarios in Apidog.
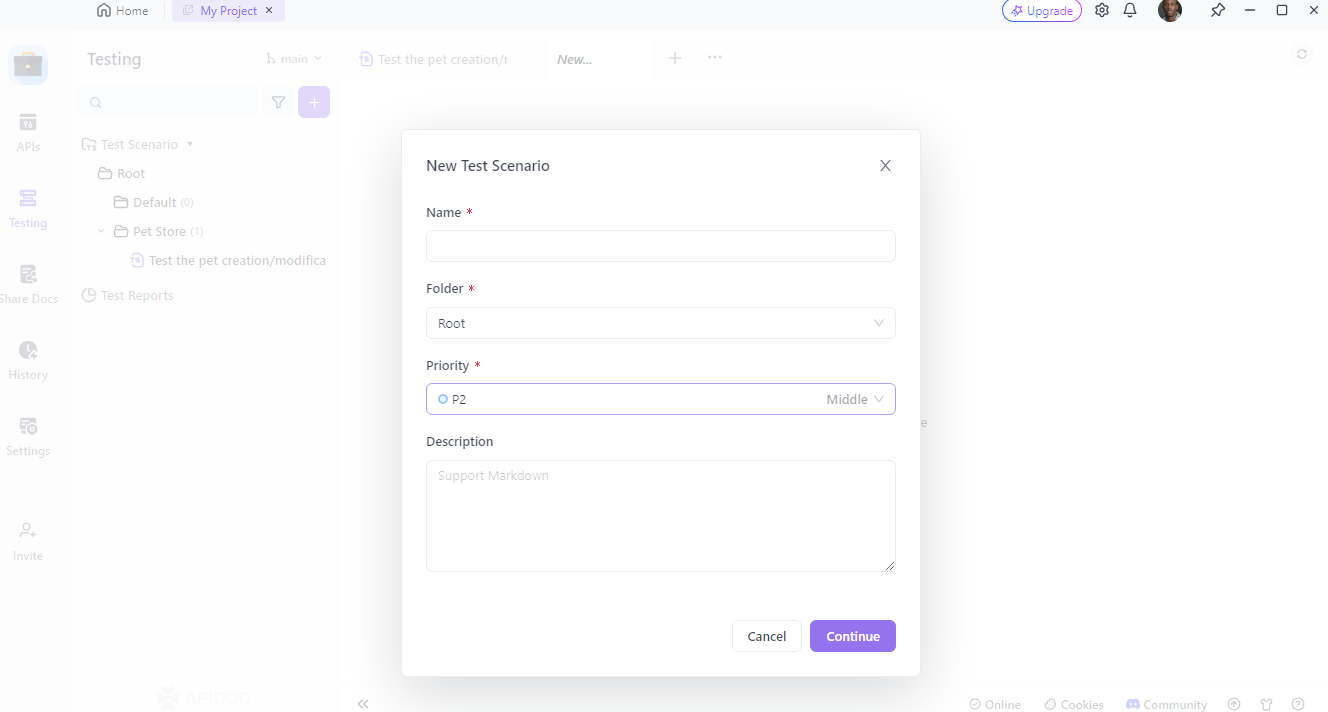
Run Your Tests: You can run your tests in Apidog.
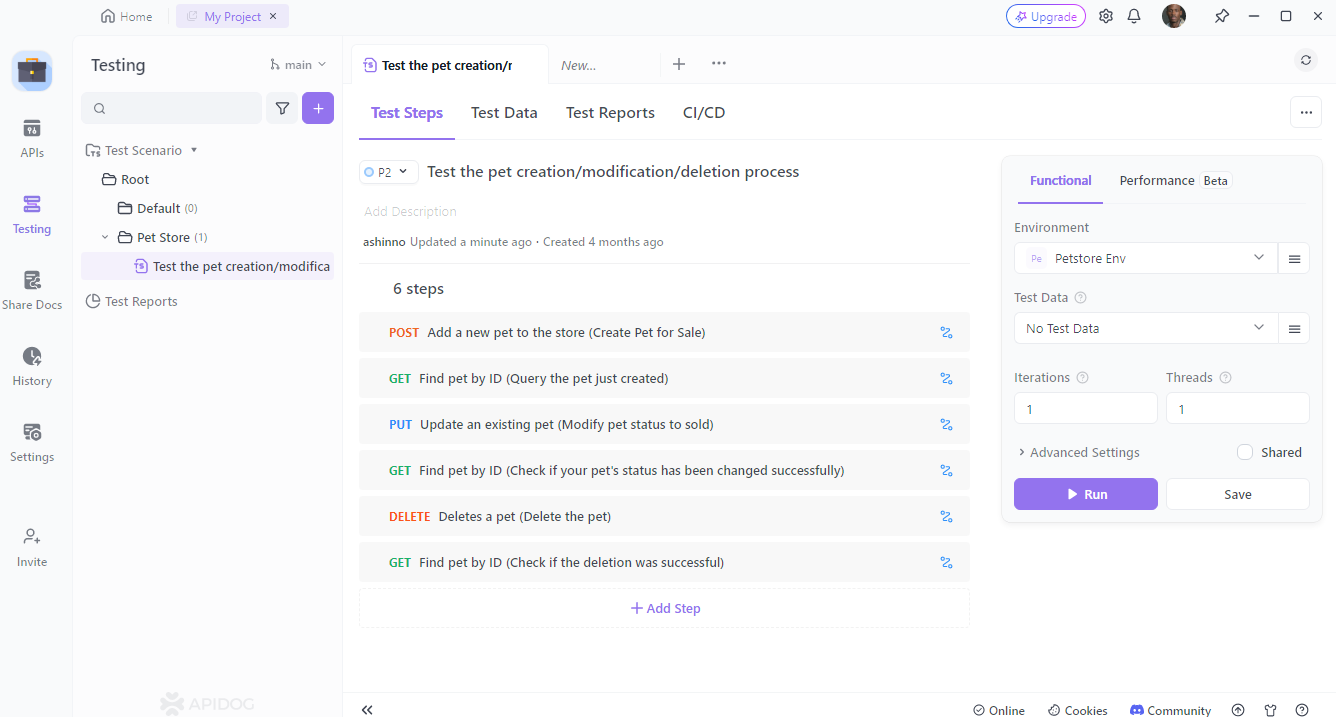
Analyze Test Results and Optimize: After running your tests, you can analyze the test results and optimize your tests accordingly.
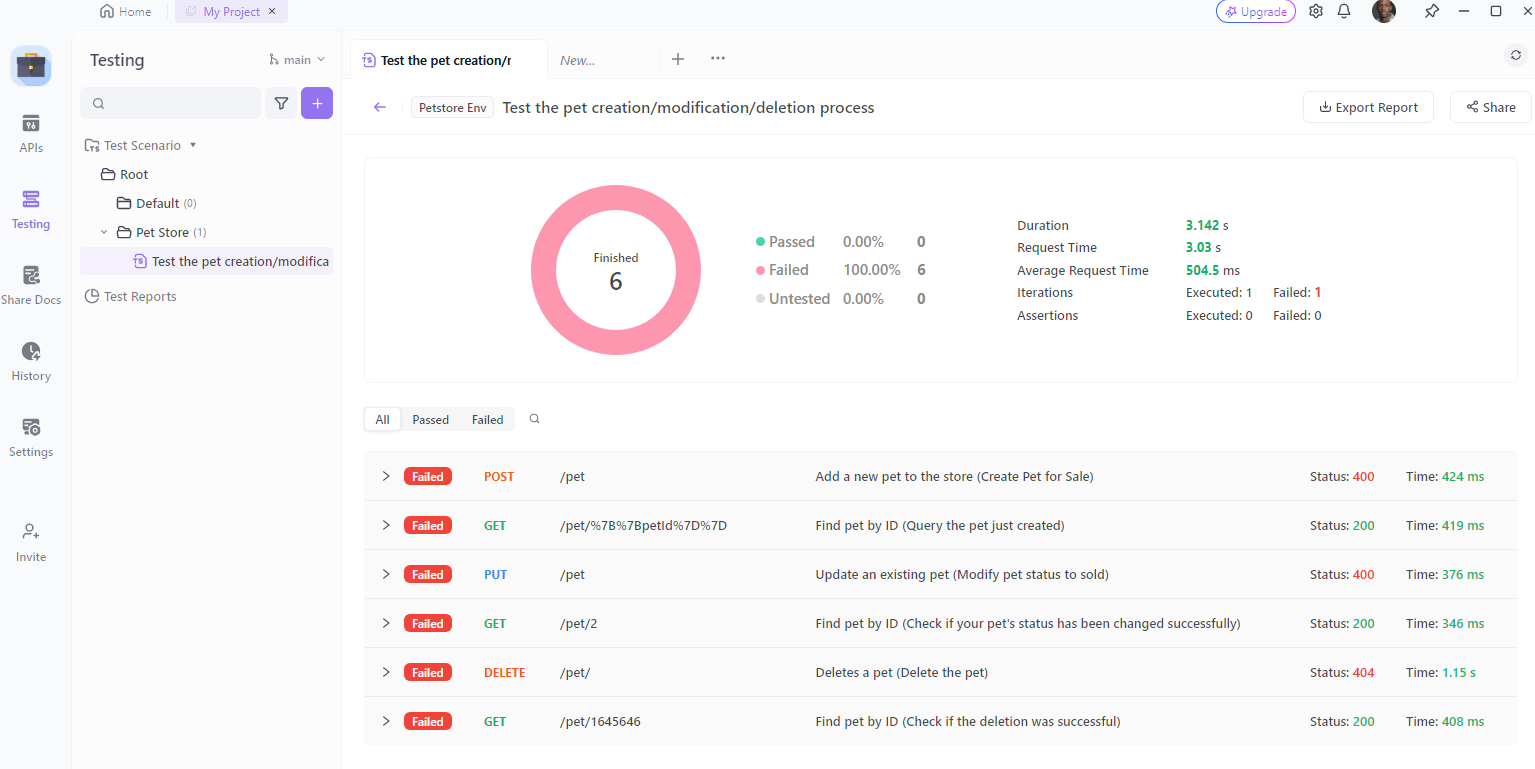
Integrating Apidog into your workflow can save you time and help you avoid common pitfalls in API development.
Conclusion
In this blog post, we've covered the essentials of checking request statuses in Python. From making your first API request to handling different status codes and implementing advanced error-handling techniques, you're now equipped with the knowledge to build more robust and reliable applications.
Remember, using tools like Apidog can further streamline your API development process, making it easier to manage and debug your APIs. So, don't hesitate to give it a try!
Happy coding!