How to Check If WebSocket Server is Running
Ensuring the proper functioning of a WebSocket server is essential for seamless real-time communication in web applications. In this guide, we'll explore simple steps to check if your W
Ensuring the proper functioning of a WebSocket server is essential for seamless real-time communication in web applications. In this guide, we'll explore simple steps to check if your WebSocket server is running, addressing potential issues and providing practical solutions.
Why Use WebSocket?
WebSocket is a communication protocol known for its real-time, bidirectional capabilities. Its advantages include low latency, efficient resource utilization, bi-directional communication, event-driven architecture, reduced network traffic, cross-domain support, and broad API compatibility. These features make WebSocket a preferred choice for applications requiring instant updates, such as chat applications, online gaming, financial platforms, and more.
How to Check If WebSocket Server is Running
When initializing a WebSocket connection to a server, you may encounter errors if the server has not finished starting up yet. For example:
var webSocket = new WebSocket("ws://localhost:8025/myContextRoot");
This may result in an error:
WebSocket Error: Network Error 12029, A connection with the server could not be established
The solution to Check WebSocket Server is Running
There are a few ways to handle this:
- Retry connecting multiple times
You can wrap the WebSocket initialization in a function that retries connecting a certain number of times before throwing an error:
function connectWithRetries(url) {
let tries = 3;
let ws = new WebSocket(url);
ws.onerror = () => {
if(tries 0) {
tries--;
setTimeout(() => connectWithRetries(url), 5000);
} else {
throw new Error("Maximum retries reached");
}
};
return ws;
}
let webSocket = connectWithRetries("ws://localhost:8025/myContextRoot");
This will attempt connecting 3 times before giving up.
2. Use Promises
You can also return a Promise from the connect function to handle success and failure:
function connect(url) {
return new Promise((resolve, reject) => {
let ws = new WebSocket(url);
ws.onopen = () => resolve(ws);
ws.onerror = () => reject(new Error("Unable to connect"));
});
}
connect("ws://localhost:8025/myContextRoot")
.then(ws => {
// use ws
})
.catch(err => {
// unable to connect
});
This provides more control flow using Promises.
3. Check if the server is running another way
If possible, you can have the server write a file or provide an endpoint that indicates it is up and running. Then check this from the client before initializing the WebSocket.
For example, have the server write a status.txt file. The client can then check this file first using XMLHttpRequest before creating the WebSocket.
In summary, retries, promises, and checking server status another way can help prevent WebSocket errors from initiating too early before the server is ready. This allows more robust connection handling.
Source: https://stackoverflow.com/questions/42112919/check-if-websocket-server-is-running-on-localhost
How to Close WebSocket Connection
Having explored the advantages of using WebSocket for seamless real-time communication, it's equally important to understand how to manage these connections effectively. One crucial aspect is knowing how to close a WebSocket connection when necessary.
Whether you're dealing with resource optimization, ending a session, or implementing specific functionalities, proper closure ensures the graceful termination of connections. Let's delve into the intricacies of closing WebSocket connections
To properly close a WebSocket connection:
On the client:
webSocket.close();
On the server:
webSocket.close(1000, "Normal closure");
The close() method accepts a numeric status code and a human-readable string indicating the reason. Some common status codes:
- 1000 - Normal closure, the default
- 1001 - Going away
- 1002 - Protocol error
- 1003 - Unsupported data
- 1005 - No status received
It's important to properly close WebSockets when finished, to clean up resources and avoid errors. Make sure to add close handling when the user navigates away or the component unmounts.
WebSockets Connection in Apidog
Apidog's extended WebSocket support elevates API testing by seamlessly integrating real-time communication testing. With a user-friendly interface, Apidog simplifies WebSocket testing, featuring automatic mocking of human-friendly data for efficient debugging.
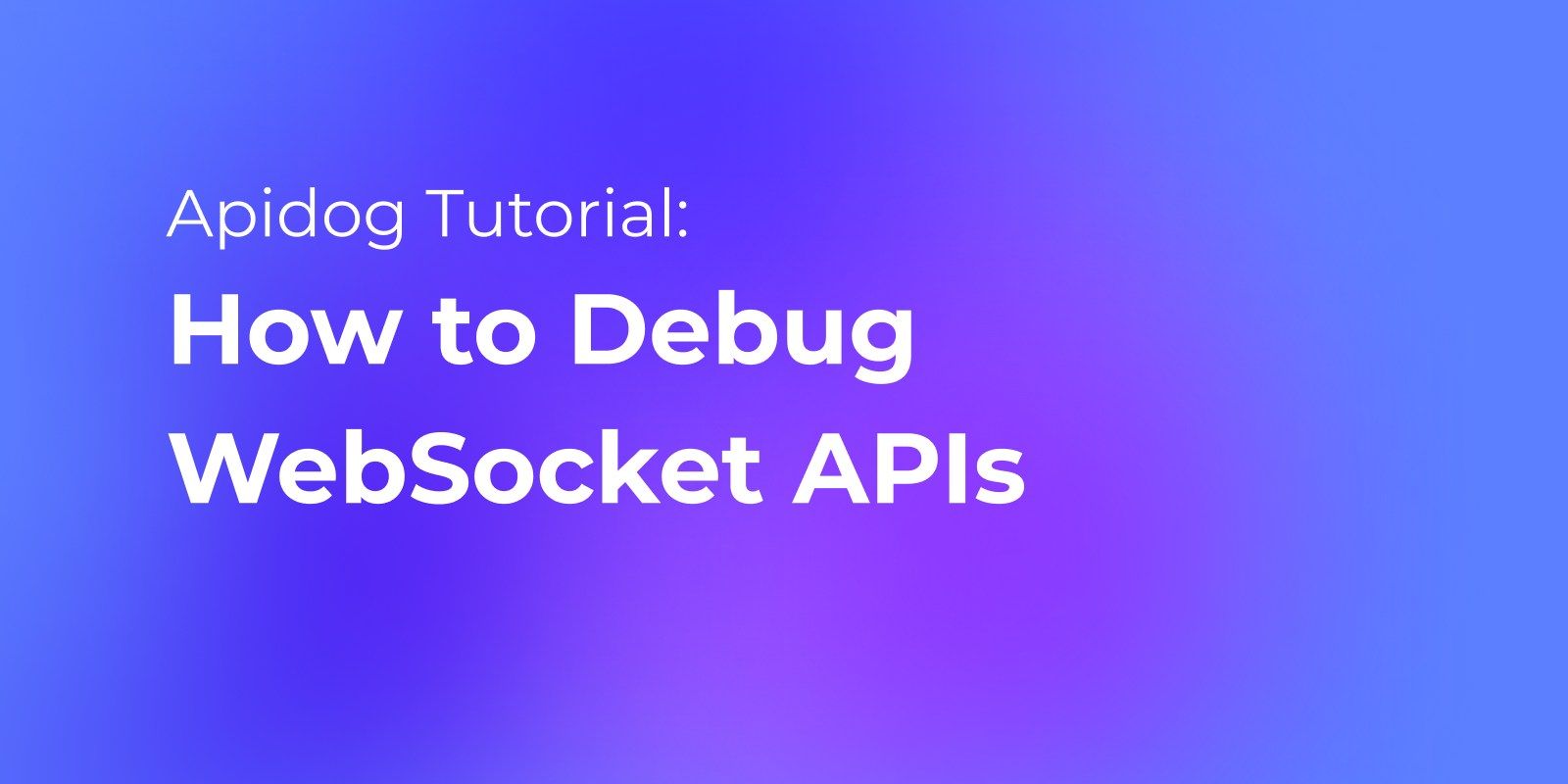
As a versatile API tool, Apidog offers developers a comprehensive solution, addressing the evolving needs of modern API development, including the intricacies of WebSocket integration.
Conclusion
In conclusion, ensuring the WebSocket server's proper functioning is crucial for seamless real-time communication, and this guide offers practical steps to check and handle potential issues. WebSocket's advantages, including low latency and bidirectional capabilities, make it indispensable for applications like chat platforms and online gaming.
Additionally, understanding how to close WebSocket connections is essential for efficient resource management, and proper closure guarantees graceful termination. This comprehensive guide equips developers with insights into WebSocket functionality and effective connection management.