In this article, we will explore the concept of API caching, discuss the creation of a custom caching component in React, and examine various tools and packages that simplify the process. Whether you're looking to implement a basic caching solution or leverage advanced tools like React Query and SWR, this guide will provide the insights and practical steps needed to optimize your React applications with efficient API response caching.
Why Caching API Response in React?
API response caching is a critical technique in modern web development that can significantly enhance the performance and efficiency of applications. As applications grow more complex, they increasingly rely on external APIs to fetch data, making efficient data management a critical concern. One powerful technique to optimize performance and enhance user experience is API response caching. By storing responses from API calls, applications can significantly reduce server load, minimize data retrieval times, and deliver a seamless experience to users.
Imagine a scenario where a user revisits a page they've previously accessed—rather than making a fresh request to the server and waiting for the data to be fetched again, the application can serve the stored response almost instantaneously. This not only saves precious time but also ensures a smoother and more responsive interface.
Creating a Custom Caching Component
Creating a custom caching component in React allows for precise control over how data is cached and managed. This section provides a step-by-step guide to building such a component, along with a discussion on its downsides.
Component Setup
First, set up a basic React component that will be responsible for fetching and caching data.
import React, { useState, useEffect } from 'react';
const useCustomCache = (apiUrl) => {
const [data, setData] = useState(null);
const [cache, setCache] = useState({});
useEffect(() => {
if (cache[apiUrl]) {
setData(cache[apiUrl]);
} else {
fetch(apiUrl)
.then(response => response.json())
.then(result => {
setCache(prevCache => ({ ...prevCache, [apiUrl]: result }));
setData(result);
});
}
}, [apiUrl, cache]);
return data;
};
export function App(props) {
const apiUrl = 'https://jsonplaceholder.typicode.com/users';
const data = useCustomCache(apiUrl);
if (!data) return <div>Loading...</div>;
return (
<div className='App'>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
};
State Management
The useState hook is used to manage both the cached data and the current data being fetched. This allows the component to determine whether to serve cached data or make a new API call.
Fetching and Caching Data
The useEffect hook contains the logic to check the cache before making a network request. If the data is found in the cache, it is used directly; otherwise, a fetch request is made, and the result is stored in the cache.
Data Expiry
To handle cache invalidation, you can set a time-to-live (TTL) for cached data. This ensures that data is periodically refreshed and stays up-to-date.
import React, { useState, useEffect } from 'react';
const useCustomCache = (apiUrl, ttl = 60000) => {
const [data, setData] = useState(null);
const [cache, setCache] = useState({});
useEffect(() => {
const cachedData = cache[apiUrl];
if (cachedData && (Date.now() - cachedData.timestamp < ttl)) {
setData(cachedData.data);
} else {
fetch(apiUrl)
.then(response => response.json())
.then(result => {
setCache(prevCache => ({ ...prevCache, [apiUrl]: { data: result, timestamp: Date.now() } }));
setData(result);
});
}
}, [apiUrl, cache, ttl]);
return data;
};
export function App(props) {
const apiUrl = 'https://jsonplaceholder.typicode.com/users';
const data = useCustomCache(apiUrl);
if (!data) return <div>Loading...</div>;
return (
<div className='App'>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
};
Downsides of Custom Caching
While creating a custom caching component offers flexibility, it also comes with several downsides:
- Complexity: Custom caching logic can add significant complexity to the codebase, making it harder to maintain and debug.
- Maintenance: Regular updates and maintenance are required to ensure the caching logic continues to work correctly as the application evolves.
- Scalability Issues: As the application grows, managing custom caching solutions can become increasingly difficult, potentially leading to performance bottlenecks.
By understanding these challenges, developers can make informed decisions about when to implement custom caching and when to leverage more advanced tools
Using NPM Packages for Caching
Leveraging NPM packages can greatly simplify the implementation of caching in React applications. Here are a few popular packages that can help you get started:
axios-cache-adapter
axios-cache-adapter
integrates caching capabilities into axios, a popular HTTP client.
Installation:
npm install axios axios-cache-adapter
Usage:
// Import dependencies
import axios from 'axios'
import { setupCache } from 'axios-cache-adapter'
// Create `axios-cache-adapter` instance
const cache = setupCache({
maxAge: 15 * 60 * 1000
})
// Create `axios` instance passing the newly created `cache.adapter`
const api = axios.create({
adapter: cache.adapter
})
// Send a GET request to some REST api
api({
url: 'http://some-rest.api/url',
method: 'get'
}).then(async (response) => {
// Do something fantastic with response.data \o/
console.log('Request response:', response)
// Interacting with the store, see `localForage` API.
const length = await cache.store.length()
console.log('Cache store length:', length)
})
lru-cache
lru-cache
implements a least-recently-used (LRU) cache.
Installation:
npm install lru-cache
Usage:
import LRU from 'lru-cache';
const cache = new LRU({ max: 100 }); // Maximum number of items in cache
const fetchData = async (url) => {
if (cache.has(url)) {
return cache.get(url);
}
const response = await fetch(url);
const data = await response.json();
cache.set(url, data);
return data;
};
idb-keyval
idb-keyval
simplifies storing key-value pairs in IndexedDB, a low-level API for client-side storage.
Installation:
npm install idb-keyval
Usage:
import { get, set } from 'idb-keyval';
const fetchData = async (url) => {
const cachedData = await get(url);
if (cachedData) {
return cachedData;
}
const response = await fetch(url);
const data = await response.json();
await set(url, data);
return data;
};
These packages offer a straightforward way to implement caching in your React applications, reducing the complexity and effort required to manage cached data manually. By using these tools, you can focus more on developing your application's features while ensuring efficient data retrieval and improved performance.
Introduction to Advanced Tools for API Caching
When it comes to caching API responses in React, advanced tools like React Query and SWR offer robust, feature-rich solutions that surpass the capabilities of custom-built caching mechanisms. These tools are designed to simplify state management, enhance data fetching, and ensure data consistency, all while providing a seamless developer experience.
TanStack Query
TanStack Query (FKA React Query is a powerful data-fetching library for React applications that abstracts away the complexities of managing server-side data. React Query transforms how you handle server-state in your React applications. It offers built-in caching, background updates, and out-of-the-box support for error handling, making it easier to manage data-fetching logic without the need to manually handle caching and state synchronization.
Benefits Over Custom Solutions:
Simplified State Management:
React Query handles the intricacies of caching and state management, allowing developers to focus on application logic. It abstracts the complexities of caching, invalidating, and refetching data, providing a clean and intuitive API.
Automatic Background Refresh:
React Query can automatically refetch data in the background to keep it up-to-date. This ensures that your application always displays the most current data without the need for manual refresh triggers.
Optimistic Updates:
With optimistic updates, React Query can immediately reflect changes in the UI before the server confirms the update. This provides a smooth and responsive user experience, as users can see their actions reflected instantly.
Example:
npm install react-query // install the package
import { QueryClient, QueryClientProvider, useQuery } from 'react-query'
const queryClient = new QueryClient()
export default function App() {
return (
<QueryClientProvider client={queryClient}>
<Example />
</QueryClientProvider>
)
}
function Example() {
const { isPending, error, data } = useQuery({
queryKey: ['repoData'],
queryFn: () =>
fetch('https://jsonplaceholder.typicode.com/users').then((res) =>
res.json(),
),
})
console.log(data)
if (isPending) return 'Loading...'
if (error) return 'An error has occurred: ' + error.message
return (
<div>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
)
}
The code above is from the official docs page, and it explains the basic way of fetching data. As you can see from the above codes, TanStack Query makes it easy for us to fetch data, and also cache those data easily.
SWR (Stale-While-Revalidate)
SWR, developed by Vercel, is another popular data-fetching library for React that emphasizes simplicity and efficiency. The name "SWR" stands for "stale-while-revalidate," a caching strategy that serves stale data while fetching fresh data in the background.
SWR provides a highly intuitive API for data fetching and caching. It ensures your application displays data immediately (stale data) and then revalidates by fetching fresh data in the background. This strategy provides a balance between performance and data freshness.
Benefits Over Custom Solutions:
Ease of Use:
SWR’s API is simple and easy to use, making it accessible for developers of all skill levels. It handles data fetching, caching, synchronization, and error handling with minimal configuration.
Data Consistency:
SWR ensures that your application data is always fresh. It automatically revalidates data in the background, providing users with the most up-to-date information without requiring manual refreshes.
Error Handling:
Built-in error handling in SWR makes it easy to manage errors during data fetching. It provides hooks to display error messages or fallback UI components when data fetching fails.
Example:
npm install swr // install the package
import useSWR from 'swr';
const fetcher = (url) => fetch(url).then((res) => res.json());
export default Example = () => {
const apiUrl = 'https://jsonplaceholder.typicode.com/users';
const { data, error } = useSWR(apiUrl, fetcher);
if (!data) return <div>Loading...</div>;
if (error) return <div>Error: {error.message}</div>;
return (
<div>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
};
Both React Query and SWR provide comprehensive solutions for managing server-side data in React applications. They simplify the process of fetching, caching, and synchronizing data, offering powerful features that reduce the need for custom caching logic. By leveraging these tools, developers can ensure their applications are efficient, performant, and deliver a seamless user experience.
Working With Apidog
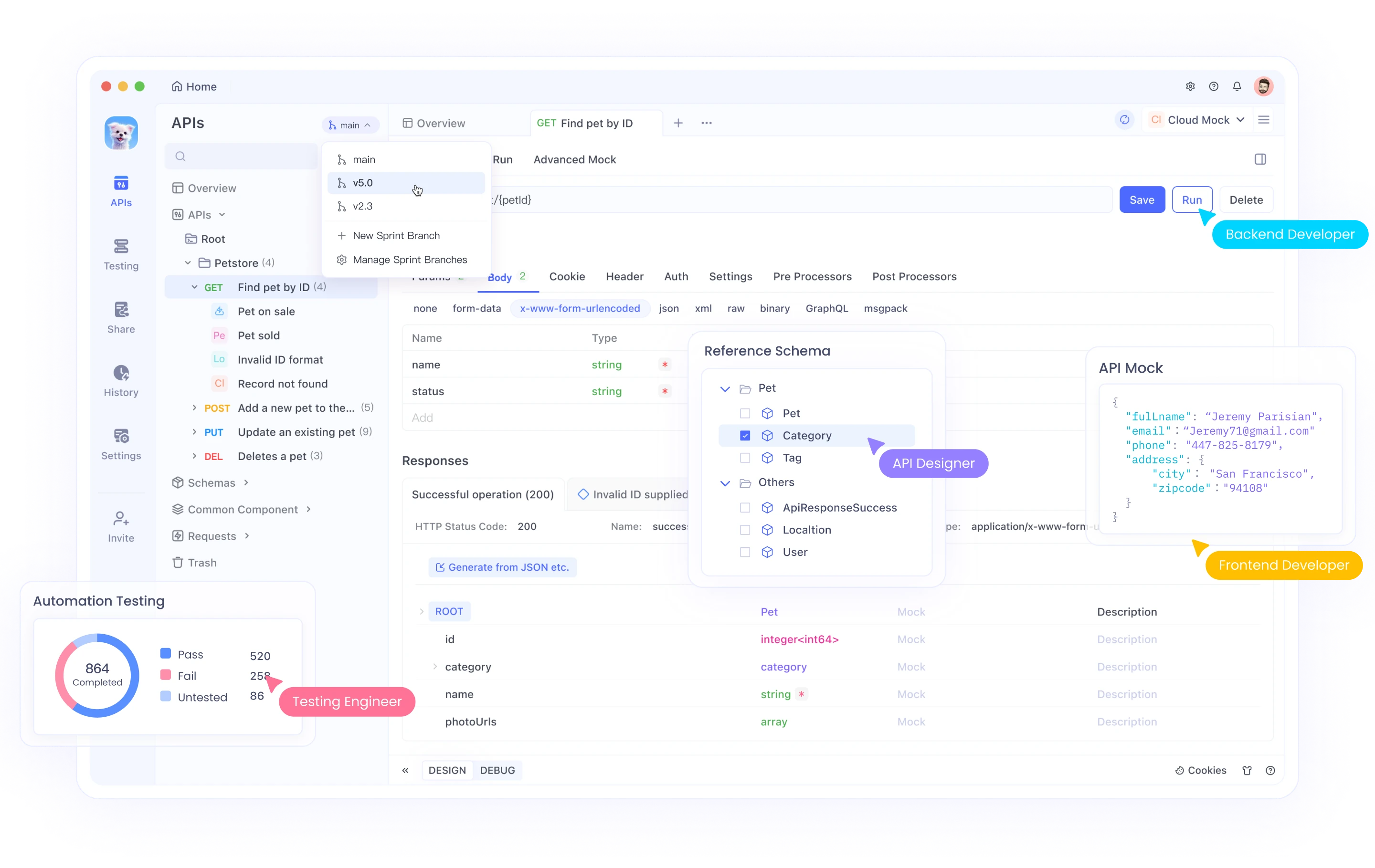
Apidog enhances API security by offering robust documentation, automated testing, and real-time monitoring. Apidog also aids in compliance with industry standards like GDPR and HIPAA, ensuring your APIs protect user data effectively.
Additionally, Apidog supports team collaboration, fostering a security-focused development environment. By integrating Apidog, you can build secure, reliable, and compliant APIs, protecting your data and users from various security threats.
Conclusion
Optimizing API response times is crucial for enhancing the performance and user experience of modern web applications. Caching API responses can significantly reduce server load and improve data retrieval speeds. While building custom caching components in React offers flexibility, leveraging advanced tools like React Query and SWR can streamline the process and provide additional features like automatic background updates and error handling.
Furthermore, using NPM packages such as axios-cache-adapter
, lru-cache
, and idb-keyval
can simplify caching implementations and reduce the complexity of managing cached data. For comprehensive API management, tools like Apidog offer robust solutions for API design, testing, and monitoring, ensuring reliability and efficiency throughout the development lifecycle.
By incorporating these tools and strategies, developers can create responsive and performant applications that deliver a seamless user experience.