A Beginner Guide to Telegram Bot API
If you're looking to build your next bot or automate a process, the Telegram API is a fantastic choice that offers all the tools you need to get started.
Telegram is not just another messaging app; it's a powerful platform that offers developers a robust API for creating bots that can interact with users in real-time. Known for its security, speed, and feature-rich environment, Telegram provides a versatile API that enables developers to build a wide range of applications, from simple messaging bots to complex automated systems that integrate with third-party services.
In this article, we'll review Telegram Bot, how we can build a simple Bot using telegram bot, and how we can make API calls to Telegram.
Benefits of using Telegram
Telegram has become a popular choice for both casual users and developers due to its unique set of features:
- Telegram stands out for several reasons. First, it is designed with a focus on privacy and security, offering end-to-end encryption for private chats to ensure that user data remains protected. This commitment to security makes Telegram a reliable choice for developers looking to build applications that handle sensitive information.
- Telegram's decentralized infrastructure means it is not only fast but also highly reliable, providing consistent performance even on low-bandwidth networks. This makes it ideal for use cases that require real-time communication or need to operate smoothly in regions with varying internet connectivity.
- Telegram’s open and free API is a major attraction for developers. Unlike some other messaging platforms that impose restrictions or fees, Telegram’s API is open to all developers, offering a level playing field to innovate without barriers. This openness particularly benefits startups and independent developers who want to build and deploy applications quickly without worrying about costs.
The real power of Telegram lies in its bot API. Telegram bots are automated programs that can perform various tasks and interact with users, making them a versatile tool for developers. Setting up a bot on Telegram is straightforward. With the help of the BotFather, Telegram’s bot management interface, developers can create a new bot in just a few steps and receive an API token that grants them access to the Telegram API. This ease of setup allows developers to quickly prototype and deploy bots without needing a deep understanding of the underlying infrastructure.
Telegram Bots: The Real Powerhouse
While Telegram's general features are impressive, its bot API is where the platform truly shines for developers. A Telegram bot is an automated program that can perform predefined tasks and interact with users. Bots can send messages, respond to user queries, handle multimedia content, and even manage entire conversations autonomously.
Features of Telegram Bots
- Ease of Use and Setup: Creating a bot on Telegram is straightforward and requires minimal setup. Using the BotFather (Telegram's bot management bot), developers can create a new bot in just a few steps and receive an API token that allows them to interact with the Telegram API.
- Rich Media Support: Telegram bots can handle all kinds of content—text, images, videos, documents, and even location data. This flexibility allows developers to create engaging, interactive experiences for users.
- Webhook Support for Real-Time Updates: Telegram bots can use webhooks to receive updates in real-time, allowing for instant interaction with users. This is ideal for applications that require low-latency communication, such as customer service bots, gaming bots, or real-time notification systems.
- Custom Commands and Keyboards: Developers can create custom commands and interactive keyboards to enhance user interaction. Inline keyboards and custom replies can guide users through complex workflows or provide quick access to frequently used features.
- Extensive API Documentation and Support: Telegram offers comprehensive documentation and an active community of developers, making it easier to find support and resources when building bots.
- No Limit on Bot Count: Unlike some messaging platforms, Telegram allows developers to create and deploy an unlimited number of bots, offering scalability for any project size.
By leveraging these features, developers can build powerful and dynamic applications that extend beyond simple messaging. Whether it’s for customer service, marketing, education, or entertainment, Telegram bots offer a versatile and efficient way to interact with users.
Setting Up the Telegram Bot
Before you can use the Telegram API, you need to create a Telegram bot. Here's how to do it:
Open Telegram and search for the BotFather.
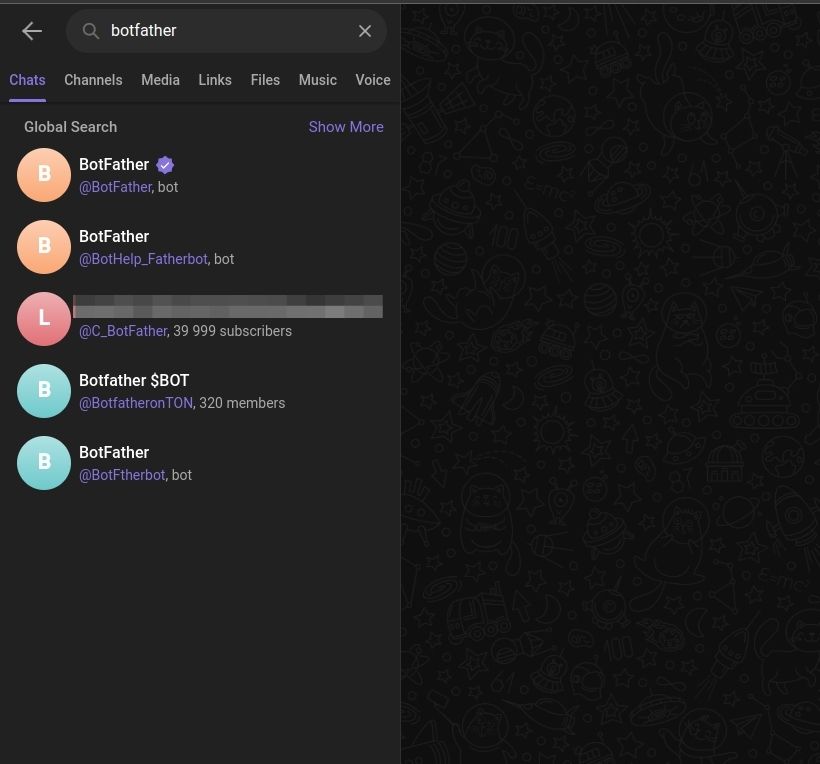
Start a chat with BotFather and use the /newbot
command to create a new bot. After that you'll be asked to choose a username for your bot. Make sure to choose one that matches your business name.
After creating your bot, BotFather will provide an API token. Keep this token secure; you'll need it to interact with the Telegram API.
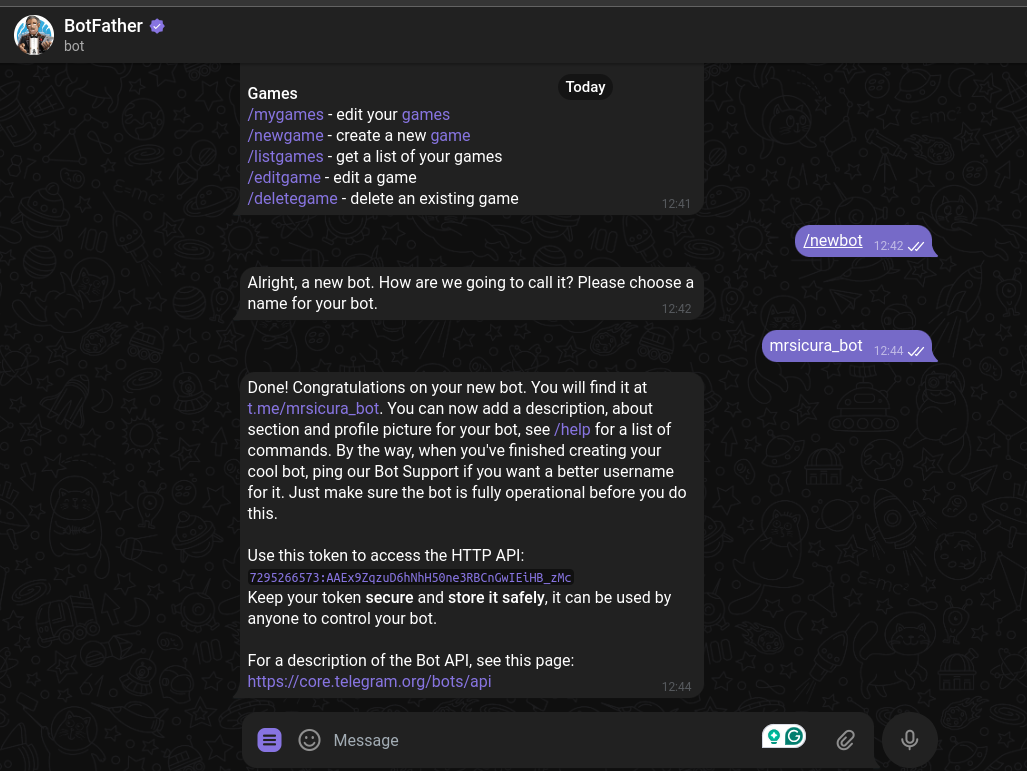
As you can see, my token has been generated for me. And if you're wondering why I exposed my token, it's simply because the Bot will be deleted, and the access token will be useless :).
Now that the Bot has been created, we can interact with it, and even send the link to our users/customers so that they can chat with it.
Using the Telegram API to Send Messages in 2 Ways
To get started with using the API to send messages or anything else, you'll need to get your chat_id
. The chat_id
is needed so that the API can know who it's sending the messages to.
Here's an article that explains how to get your chat_id.
This is another article too :)
Telegram has different endpoints that we can use to interact with our Bot, but we'll only focus on the SendMessage
endpoint for this guide.
https://api.telegram.org/bot<bot_id>/sendMessage
Use Apidog to Send A Test Request:
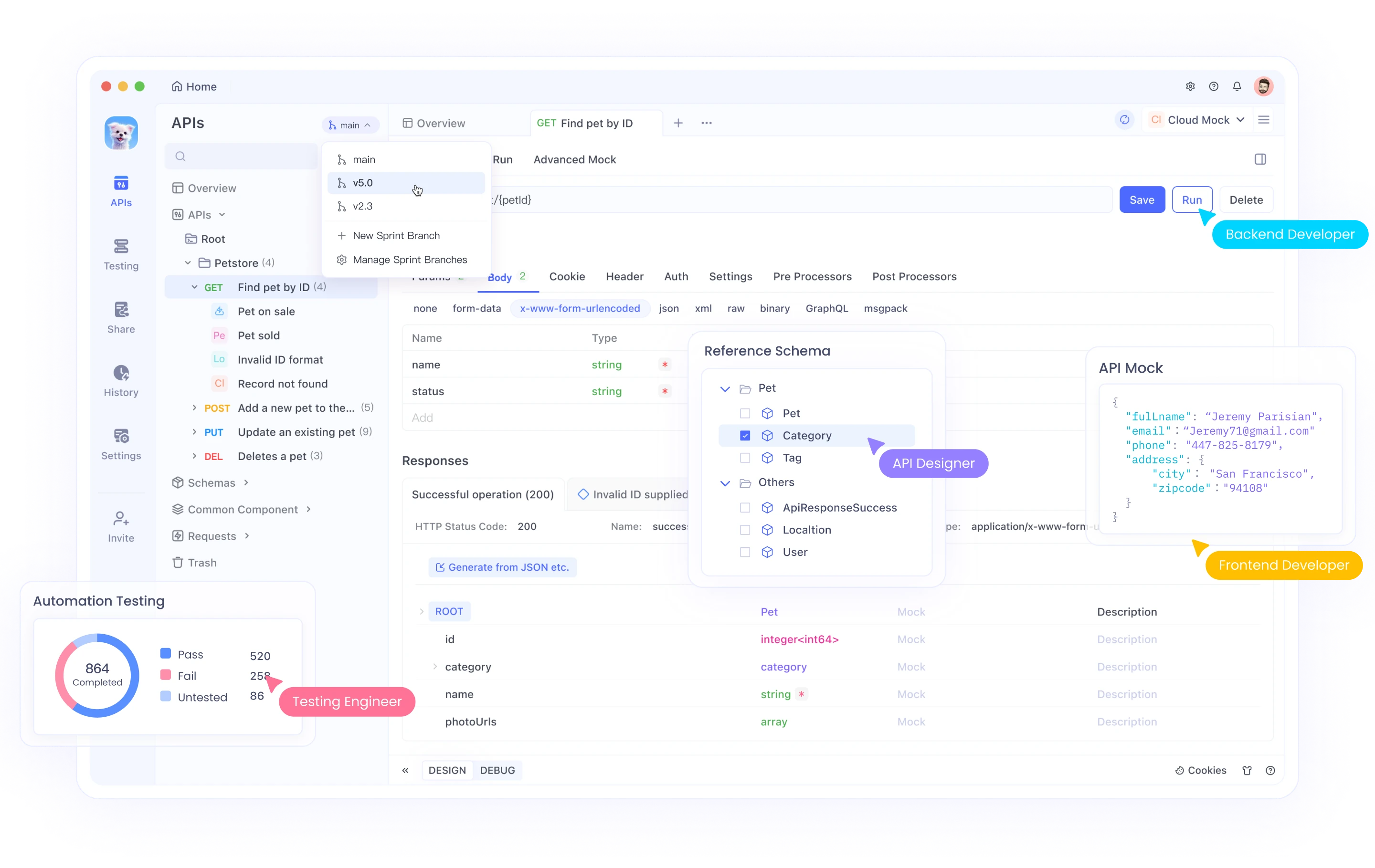
Apidog enhances API security by offering robust documentation, automated testing, and real-time monitoring. Apidog also aids in compliance with industry standards like GDPR and HIPAA, ensuring your APIs protect user data effectively.
Additionally, Apidog supports team collaboration, fostering a security-focused development environment. By integrating Apidog, you can build secure, reliable, and compliant APIs, protecting your data and users from various security threats.
Once you have Apidog or the web version installed, you can start by creating a new project and sending your first request.
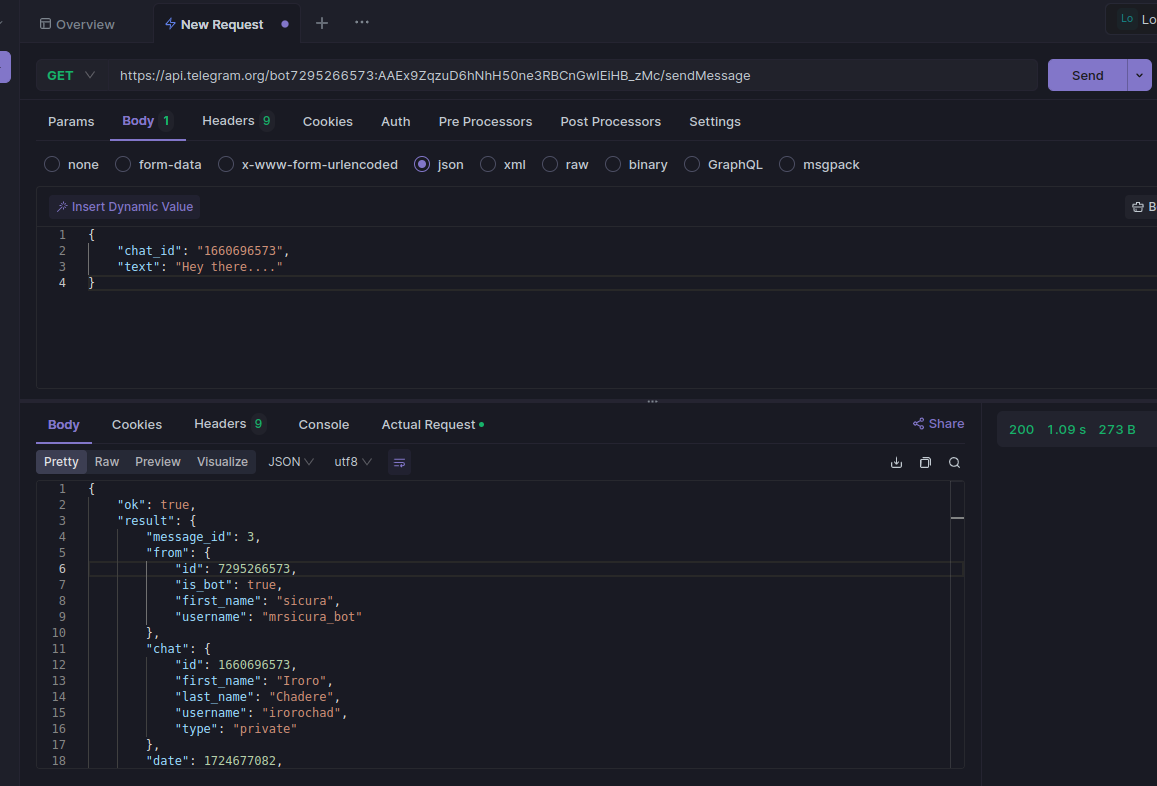
In this case, I sent a simple "Hey there" message to the bot we've created, passing the chat_id
. The response was OK, and you can see the result from the response.
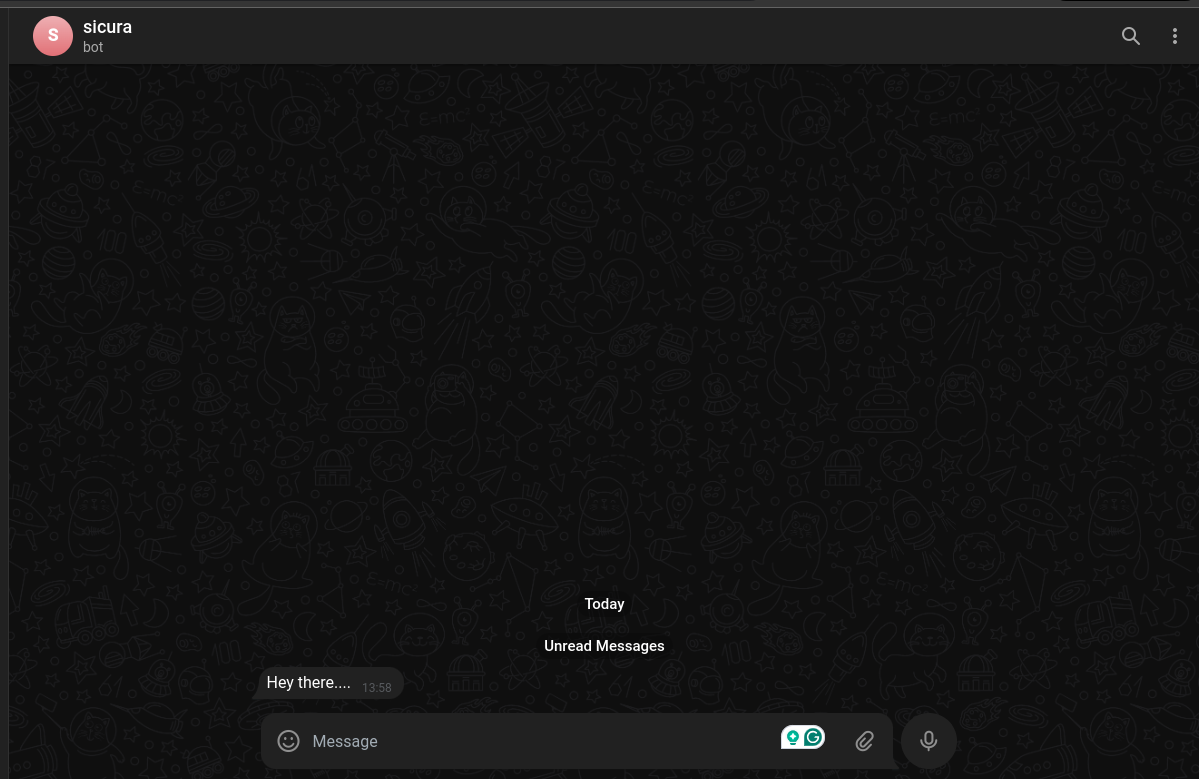
Using React.js
You can also use Javascript to send the request, and get a response too. This is useful if you're building a web interface where you want people to contact you with your bot.
import React, { useState } from 'react';
import axios from 'axios';
const TelegramBot = () => {
const [message, setMessage] = useState(''); // State to store user input
const [isLoading, setIsLoading] = useState(false); // State to manage loading status
const [feedback, setFeedback] = useState(''); // State to provide user feedback
const sendMessage = async () => {
if (!message.trim()) {
setFeedback('Message cannot be empty.');
return;
}
setIsLoading(true); // Set loading to true when sending a request
setFeedback(''); // Clear any previous feedback
const botToken = 'YOUR_API_TOKEN'; // Replace with your Telegram Bot API token
const chatId = 'CHAT_ID'; // Replace with the chat ID to which you want to send the message
const url = `https://api.telegram.org/bot${botToken}/sendMessage`;
try {
const response = await axios.post(url, {
chat_id: chatId,
text: message
});
if (response.data.ok) {
setFeedback('Message sent successfully!');
setMessage(''); // Clear the input field after successful send
} else {
setFeedback(`Error: ${response.data.description}`);
}
} catch (error) {
setFeedback(`Error: ${error.message}`);
} finally {
setIsLoading(false); // Reset loading status
}
};
return (
<div>
<h1>Send a Message via Telegram Bot</h1>
<input
type="text"
value={message}
onChange={e => setMessage(e.target.value)}
placeholder="Enter your message"
disabled={isLoading}
/>
<button onClick={sendMessage} disabled={isLoading}>
{isLoading ? 'Sending...' : 'Send Message'}
</button>
{feedback && <p>{feedback}</p>} {/* Display feedback message */}
</div>
);
};
export default TelegramBot;
Conclusion
In this article, we've explored the powerful capabilities of the Telegram API, particularly focusing on how developers can leverage it to build dynamic and engaging bots. We started with an overview of Telegram's unique features, such as its emphasis on privacy, speed, and open access, which make it an ideal platform for bot development. We then delved into the core functionality of Telegram bots, including their ease of setup, support for rich media, real-time updates with webhooks, and extensive customization options.
If you're looking to build your next bot or automate a process, the Telegram API is a fantastic choice that offers all the tools you need to get started.