What Does Await Do in Playwright? Clearly Explained!
Discover the role of await in Playwright and learn how to effectively use async operations in your web testing. This comprehensive guide covers practical examples, common pitfalls, and the integration of Playwright with Apidog.
Playwright is a powerful automation framework for web browsers that allows developers to write robust and efficient tests for web applications. One of the key features of Playwright is its asynchronous nature, which enables it to handle multiple operations concurrently. At the heart of this asynchronous behavior is the await
keyword, which plays a crucial role in managing the flow of asynchronous operations in Playwright scripts.
The Basics of Asynchronous Programming
Before diving into the specifics of await
in Playwright, it's essential to understand the basics of asynchronous programming in JavaScript.
In traditional synchronous programming, operations are executed one after another, with each operation blocking the execution of the next until it completes. This can lead to performance issues, especially when dealing with time-consuming tasks like network requests or file operations.
Asynchronous programming allows multiple operations to be initiated without waiting for the previous ones to complete. This non-blocking approach improves performance and responsiveness, particularly in scenarios involving I/O operations or external resources.
What is Playwright?
Before diving into await
and its role, let's briefly touch upon what Playwright is. Playwright is an open-source Node.js library developed by Microsoft for automating web browsers. It supports Chromium, Firefox, and WebKit, making it a versatile tool for testing web applications across different browsers.
Playwright enables developers and testers to write high-fidelity, reliable tests. It’s particularly known for its ability to handle multiple tabs, iframes, and even intercept network requests. But to leverage its full potential, understanding asynchronous programming and the await
keyword is essential.
Promises and Async/Await
JavaScript uses Promises to handle asynchronous operations. A Promise represents a value that may not be available immediately but will be resolved at some point in the future. The async/await
syntax, introduced in ECMAScript 2017, provides a more readable and manageable way to work with Promises.
- An
async
function always returns a Promise. - The
await
keyword can only be used inside anasync
function. await
pauses the execution of theasync
function until the Promise is resolved.
The Role of await
in Playwright
In Playwright, many operations are asynchronous by nature. These include navigating to a page, interacting with elements, and waiting for specific conditions to be met. The await
keyword is used extensively in Playwright scripts to handle these asynchronous operations effectively.
Here's what await
does in Playwright:
Pauses Execution: When await
is used before a Playwright action or assertion, it pauses the execution of the script until that action or assertion is complete.
Resolves Promises: It waits for the Promise returned by a Playwright method to resolve, allowing you to work with the resolved value directly.
Ensures Proper Sequencing: It helps maintain the correct order of operations, ensuring that one action is completed before moving on to the next.
Handles Errors: If the awaited Promise is rejected, await
throws an exception, allowing you to catch and handle errors effectively.
Practical Examples of await
in Playwright
Let's explore some common scenarios where await
is used in Playwright scripts:
1. Navigation
test('Navigate to a page', async ({ page }) => {
await page.goto('https://example.com');
// The script waits until the page is loaded before proceeding
});
In this example, await
ensures that the navigation is complete before the test continues.
2. Interacting with Elements
test('Click a button', async ({ page }) => {
await page.click('#submit-button');
// The script waits for the click action to complete
});
Here, await
ensures that the click action is fully executed before moving on.
3. Waiting for Elements
test('Wait for element to be visible', async ({ page }) => {
await page.waitForSelector('#dynamic-content');
// The script pauses until the element is visible
});
In this case, await
pauses the script until the specified element becomes visible on the page.
4. Assertions
test('Check page title', async ({ page }) => {
await expect(page).toHaveTitle('Expected Title');
// The assertion is evaluated asynchronously
});
await
is used with assertions to ensure they are evaluated properly, waiting for the condition to be met or timing out if it's not.
The Importance of await
in Playwright
Using await
correctly in Playwright is crucial for several reasons:
Synchronization: It helps synchronize asynchronous operations, preventing race conditions and ensuring that actions are performed in the intended order.
Reliability: By waiting for operations to complete, await
makes tests more reliable and less prone to flakiness.
Error Handling: It allows for proper error propagation, making it easier to identify and debug issues in your tests.
Readability: await
makes asynchronous code more readable and easier to understand, as it allows you to write asynchronous code in a more linear, synchronous-looking manner.
Common Pitfalls and Best Practices
While await
is powerful, there are some common pitfalls to avoid and best practices to follow:
1. Forgetting to Use await
One of the most common mistakes is forgetting to use await
before an asynchronous operation. This can lead to unexpected behavior and race conditions.
Incorrect:
test('Incorrect usage', async ({ page }) => {
page.click('#button'); // This is incorrect
expect(page.locator('#result')).toBeVisible();
});
Correct:
test('Correct usage', async ({ page }) => {
await page.click('#button');
await expect(page.locator('#result')).toBeVisible();
});
2. Overusing await
While it's important to use await
for asynchronous operations, overusing it can lead to unnecessary sequential execution, reducing the benefits of asynchronous programming.
Less Efficient:
test('Sequential execution', async ({ page }) => {
await page.fill('#field1', 'value1');
await page.fill('#field2', 'value2');
await page.fill('#field3', 'value3');
});
More Efficient:
test('Parallel execution', async ({ page }) => {
const [field1, field2, field3] = await Promise.all([
page.fill('#field1', 'value1'),
page.fill('#field2', 'value2'),
page.fill('#field3', 'value3')
]);
});
3. Using await
Outside of Async Functions
Remember that await
can only be used inside async
functions. Using it outside will result in a syntax error.
Incorrect:
test('Incorrect usage', ({ page }) => {
await page.goto('https://example.com'); // This will cause an error
});
Correct:
test('Correct usage', async ({ page }) => {
await page.goto('https://example.com');
});
4. Handling Errors
Always use try-catch blocks or .catch()
methods to handle potential errors when using await
.
test('Error handling', async ({ page }) => {
try {
await page.click('#non-existent-button');
} catch (error) {
console.error('An error occurred:', error);
}
});
Advanced Usage of await
in Playwright
As you become more proficient with Playwright, you'll encounter more advanced scenarios where await
plays a crucial role:
1. Custom Assertions
When creating custom assertions, you may need to use await
to handle asynchronous operations within the assertion logic.
expect.extend({
async toHaveCorrectApiResponse(page, expectedResponse) {
const response = await page.evaluate(() => fetchApiData());
return {
pass: JSON.stringify(response) === JSON.stringify(expectedResponse),
message: () => `Expected API response to match ${expectedResponse}`
};
}
});
test('Custom assertion', async ({ page }) => {
await expect(page).toHaveCorrectApiResponse({ status: 'success' });
});
2. Handling Multiple Promises
In complex scenarios, you might need to handle multiple promises concurrently. The Promise.all()
method, combined with await
, is useful in such cases.
test('Handle multiple promises', async ({ page }) => {
const [navigationPromise, dialogPromise] = await Promise.all([
page.waitForNavigation(),
page.waitForEvent('dialog'),
page.click('#submit-button')
]);
await dialogPromise.accept();
await navigationPromise;
});
3. Conditional Waiting
Sometimes, you may need to wait for a condition that may or may not occur. You can use try-catch
with a timeout to handle such scenarios.
test('Conditional waiting', async ({ page }) => {
try {
await page.waitForSelector('#popup', { timeout: 5000 });
await page.click('#close-popup');
} catch (error) {
// Popup didn't appear, continue with the test
}
});
Integrating Playwright with Apidog
Integrating Playwright with Apidog can take your testing to the next level. Apidog provides robust API design, documentation, and testing tools that can complement your Playwright tests, ensuring your web application functions correctly both on the frontend and backend.
Sending Your API with Apidog
Now that you have a working API and a React frontend, it's time to test your API. Apidog is a fantastic tool for this.
Step 1: Open Apidog and create a new request.
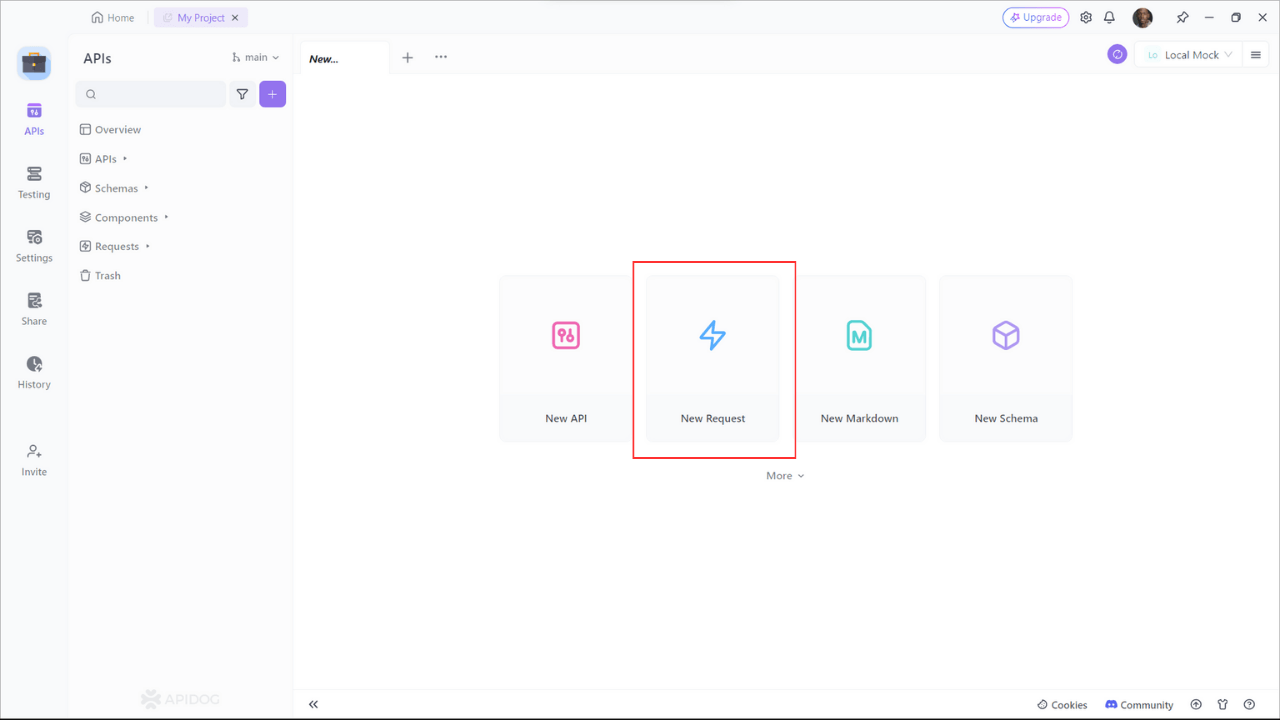
Step 2: In the test editor, enter the URL of your API endpoint, select the HTTP method, and add any headers, parameters, or body data that you need. For example, you can test the route that returns a simple message that we created earlier:
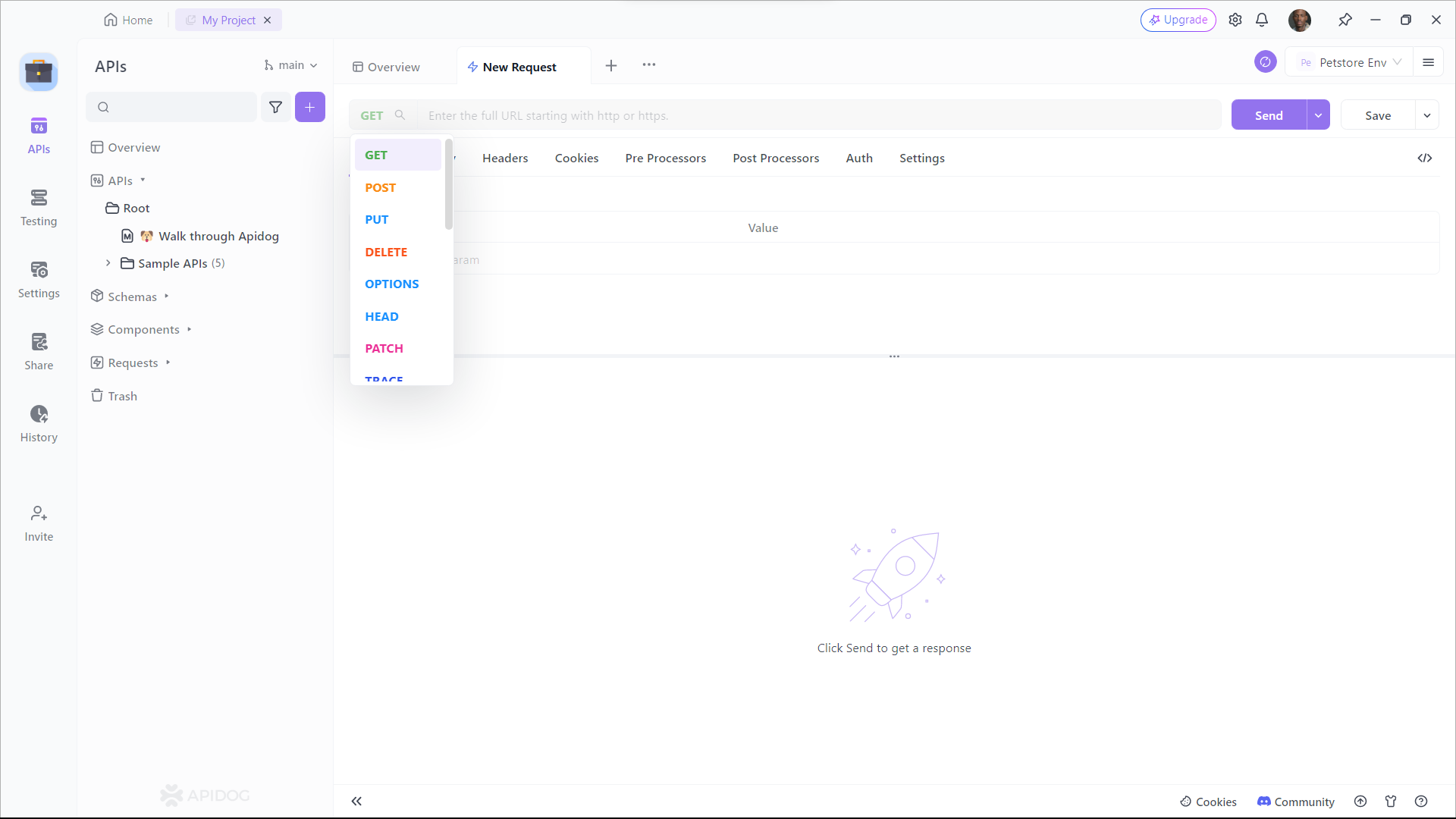
Step 3: Click on the Send button and see the result of your test. You should see the status code, the response time, and the response body of your API. For example, you should see something like this:
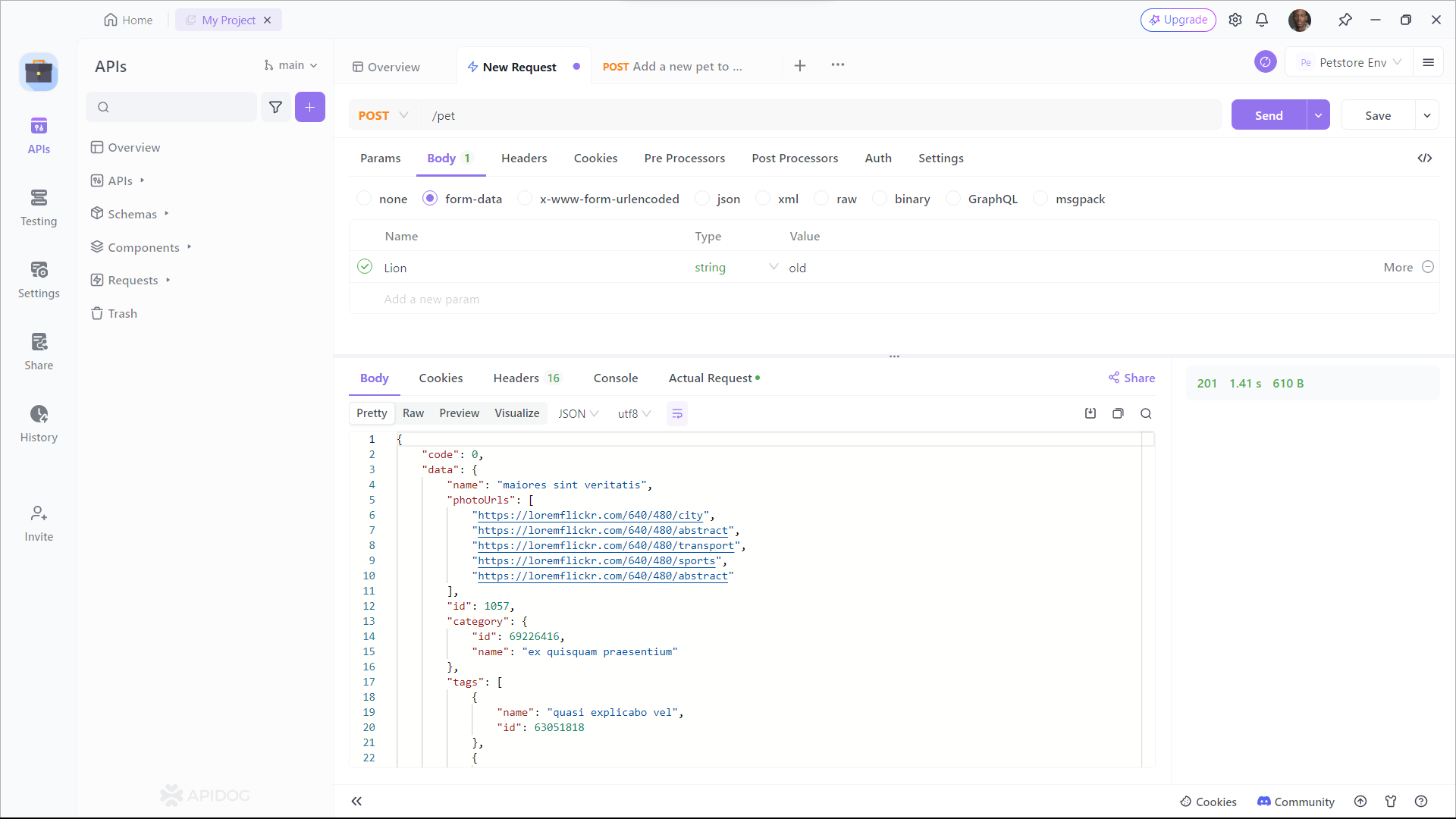
Apidog is a great tool for testing your APIs, as it helps you ensure the quality, reliability, and performance of your web services. It also saves you time and effort, as you don’t need to write any code or install any software to test your APIs. You can just use your browser and enjoy the user-friendly interface and features of Apidog.
Conclusion
Understanding what await
does in Playwright is crucial for writing effective and reliable tests. By leveraging async/await, you can ensure that each step of your test script executes in the correct order, preventing potential issues and improving the maintainability of your tests.
Remember to download Apidog for free to enhance your testing toolkit further. With Playwright and Apidog combined, you'll have a comprehensive solution for both frontend and backend testing.