A Comprehensive Guide to API Keys
Organizations can gain valuable insights into API key usage trends, identify potential security incidents, and optimize resource utilization with precision and efficiency
Introduction:
In today's interconnected digital landscape, the term "API keys" has become popular in software development circles. These seemingly innocuous strings of characters serve as the pillar of secure communication between applications, enabling seamless integration and functionality. In this comprehensive guide, we delve deep into the realm of API keys, uncovering their definition, unraveling their significance in software development, and elucidating their overarching purpose.
Definition of API Keys:
At its core, an API (Application Programming Interface) key is a unique identifier assigned to a user, application, or device accessing an API. Essentially, it acts as a credential, granting authorized access to specific functionalities or resources within an application or service. Think of it as a digital key that unlocks the door to a wealth of data and functionality, while simultaneously safeguarding against unauthorized entry.
Importance of API Keys in Software Development:
In the dynamic landscape of software development, where interoperability and integration reign supreme, API keys play a pivotal role in facilitating seamless communication between disparate systems. They serve as the cornerstone of security and access control, ensuring that only authorized entities can interact with an application's API endpoints. Moreover, API keys empower developers to implement granular access controls, thereby safeguarding sensitive data and mitigating potential security risks.
Purpose of API Keys:
The primary purpose of API keys transcends mere authentication; they serve as a mechanism for enforcing access policies and tracking usage metrics. By leveraging API keys, developers can monitor and regulate the flow of data between systems, enabling efficient resource management and performance optimization. Additionally, API keys enable organizations to enforce usage quotas, preventing abuse and ensuring equitable distribution of resources among consumers.
Understanding API Keys:
Now that we've gotten a clear introduction to what an API key is all about - let's familiarize ourselves with some concepts of API keys.
How API Keys Work:
The mechanics of API keys revolve around the concept of token-based authentication. When a client application requests access to an API endpoint, it must include its API key in the request header or query parameters. Upon receiving the request, the API server validates the provided API key against its registry of authorized keys. If the key is valid and authorized for the requested action, the server processes the request and returns the corresponding response.
Types of API Keys:
API keys can be broadly categorized into two distinct types: public keys and private keys. Each type serves a unique purpose and is associated with specific access privileges and security considerations
- Public Keys: Public API keys, as the name implies, are intended for widespread distribution and consumption. These keys are typically used for accessing public APIs or resources that do not require stringent access controls. While public keys enable broad accessibility, they also pose a higher risk of exposure and abuse if not adequately protected.
- Private Keys: Contrary to public keys, private API keys are meant to be kept confidential and shared only with trusted entities. These keys grant access to sensitive functionalities or privileged resources within an API, such as administrative endpoints or premium features. As such, stringent measures must be implemented to safeguard private keys against unauthorized access or exploitation.
Security Concerns with API Keys:
Despite their inherent utility, API keys are not immune to security vulnerabilities and risks. From potential exposure through insecure storage mechanisms to unauthorized usage by malicious actors, API keys pose a myriad of security concerns that demand meticulous attention and proactive mitigation strategies. Common security threats associated with API keys include API key leakage, brute force attacks, and unauthorized key usage, underscoring the critical importance of robust security measures and best practices.
In the next part, we will delve deeper into implementing, securing, managing, and monitoring API keys to ensure robust security and optimal performance in software development environments.
Implementing API Keys:
A. Generating API Keys:
The first step in implementing API keys is generating unique identifiers that will serve as credentials for accessing your API. Most API providers offer straightforward mechanisms for generating API keys through their developer portals or administrative interfaces. Typically, API keys are generated as alphanumeric strings of a specific length, ensuring randomness and uniqueness. Let's consider an example of generating API keys in node.js using a fictional API provider's developer portal:
const axios = require('axios');
// Function to generate API key
let apiKey;
async function generateAPIKey() {
try {
// Make a POST request to the API provider's endpoint for generating API keys
const response = await axios.post('https://api.example.com/generate_key');
if (response.status === 201) {
// Extract the generated API key from the response
let apiKey = response.data.apiKey;
return apiKey;
} else {
// Handle error response
console.error('Failed to generate API key. Status code:', response.status);
return null;
}
} catch (error) {
// Handle request error
console.error('Error generating API key:', error.message);
return null;
}
}
I chose to use JavaScript for this example because JavaScript is one of the most popular and widely used programming languages by developers. If you understand what the code above does, that's cool - well done! If you don't, let's break it down a little.
First of all, we're using axios
- a javascript library for data fetching. We created a generateAPIKey
function and we sent a POST
request using axios
to generate our API keys from the example
website.
If the response from the POST
request is 201, it means the API was created, and we store it in a variable where we can access it and pass it to other functions if needed.
B. Integrating API Keys into Applications:
Once you have obtained the API key, the next step is to integrate it into your application's codebase. Depending on the programming language and framework you're using, the integration process may vary. However, the fundamental principle remains consistent: include the API key in your HTTP requests to authenticate with the API server. Here's a basic example of integrating an API key into a Javascript application using the requests library:
const axios = require('axios');
// Function to make API request with API key
async function fetchDataWithAPIKey(apiKey) {
const endpoint = 'https://api.example.com/data';
try {
// Make a GET request to the API endpoint with the API key included in the headers
const response = await axios.get(endpoint, {
headers: {
'Authorization': `Bearer ${apiKey}`
}
});
// Process the API response
if (response.status === 200) {
const data = response.data;
// Process the retrieved data
console.log(data);
} else {
console.error('Failed to fetch data from the API. Status code:', response.status);
}
} catch (error) {
// Handle request error
console.error('Error fetching data from the API:', error.message);
}
}
Again, we're using Axios to send the requests. This time around, we're sending a request and appending our API key in the request header, by passing it as a Bearer
method.
Testing API Keys using Apidog:
Now that we've covered the integration of API keys into applications, it's essential to ensure that our APIs are functioning as expected and delivering the intended functionalities. One effective way to achieve this is through API testing tools, which enable developers to simulate API requests, analyze responses, and validate the behavior of their APIs.
One such tool that stands out in the realm of API testing is Apidog. Apidog provides a user-friendly interface for testing APIs, similar to popular tools like Postman. With its intuitive design and comprehensive feature set, Apidog simplifies the process of API testing and debugging, allowing developers to streamline their testing workflows and identify potential issues with ease.
By leveraging Apidog, developers can:
- Send HTTP requests: Apidog.com enables users to craft and send various types of HTTP requests, including GET, POST, PUT, DELETE, and more, to interact with their APIs.
- Customize request parameters: Developers can specify request headers, query parameters, request bodies, and other parameters to simulate different scenarios and test edge cases.
- Inspect responses: Apidog.com provides detailed insights into API responses, including status codes, headers, and response bodies, allowing developers to validate the correctness of API behavior and troubleshoot any issues encountered.
- Save and organize requests: Users can save their API requests, organize them into collections, and share them with team members, facilitating collaboration and knowledge sharing within development teams.
By incorporating Apidog into their API testing toolkit, developers can expedite the testing process, identify potential bugs or inconsistencies early in the development lifecycle, and ensure the reliability and performance of their APIs before deployment.
So now, How do we use Apidog to test the functionality of our API keys?
The first thing is, if you're yet to create an account, please create an account and follow this guide to learn how to use Apidog at its core.
The next thing is to start sending a request. Click on the New Request button as shown below from the Apidog's dashboard;
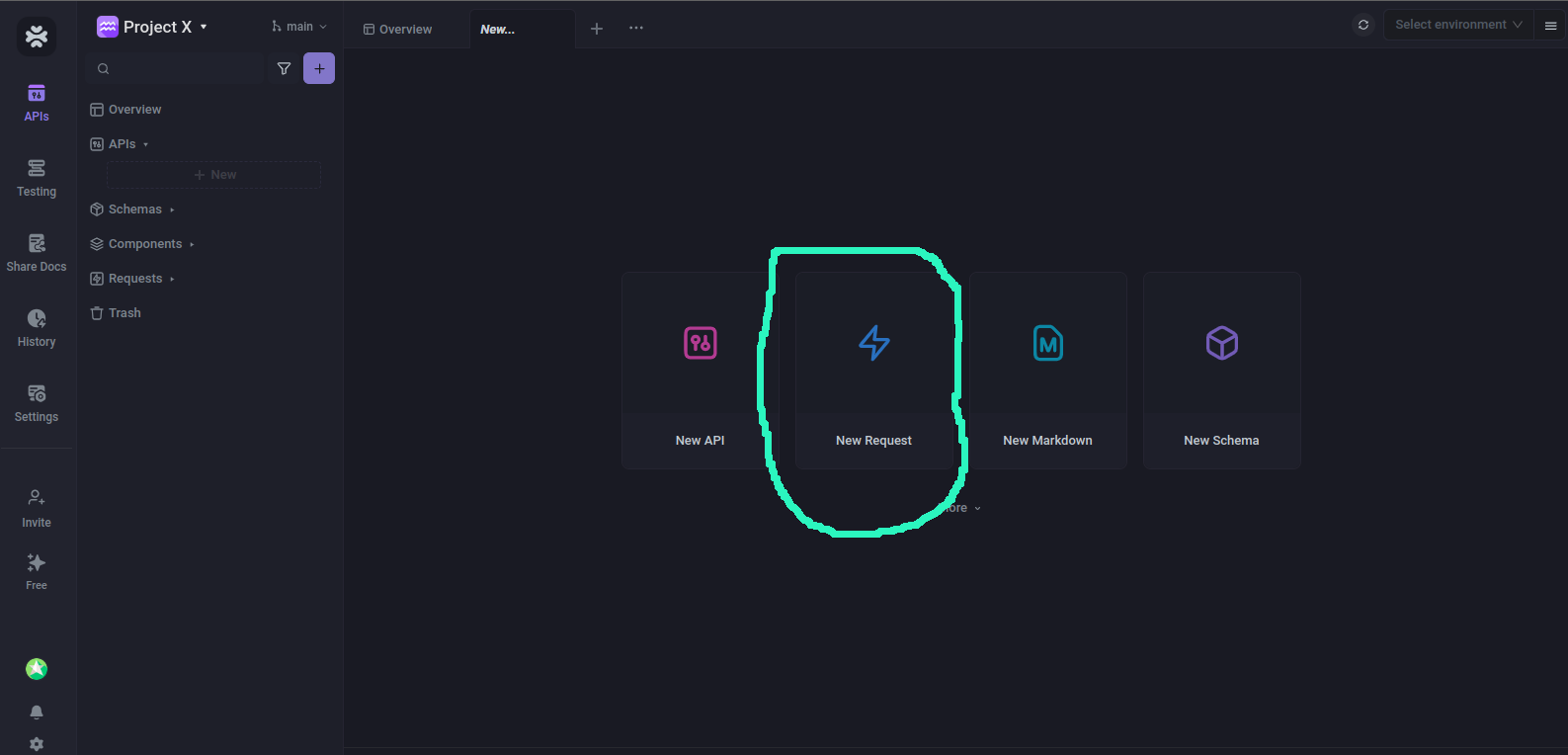
Then you'll be taken to a page where you can enter the URL and your API key to test things out.
For this tutorial, I'll be using The Cat API. You can check them out, or use your own API if you have a server running.
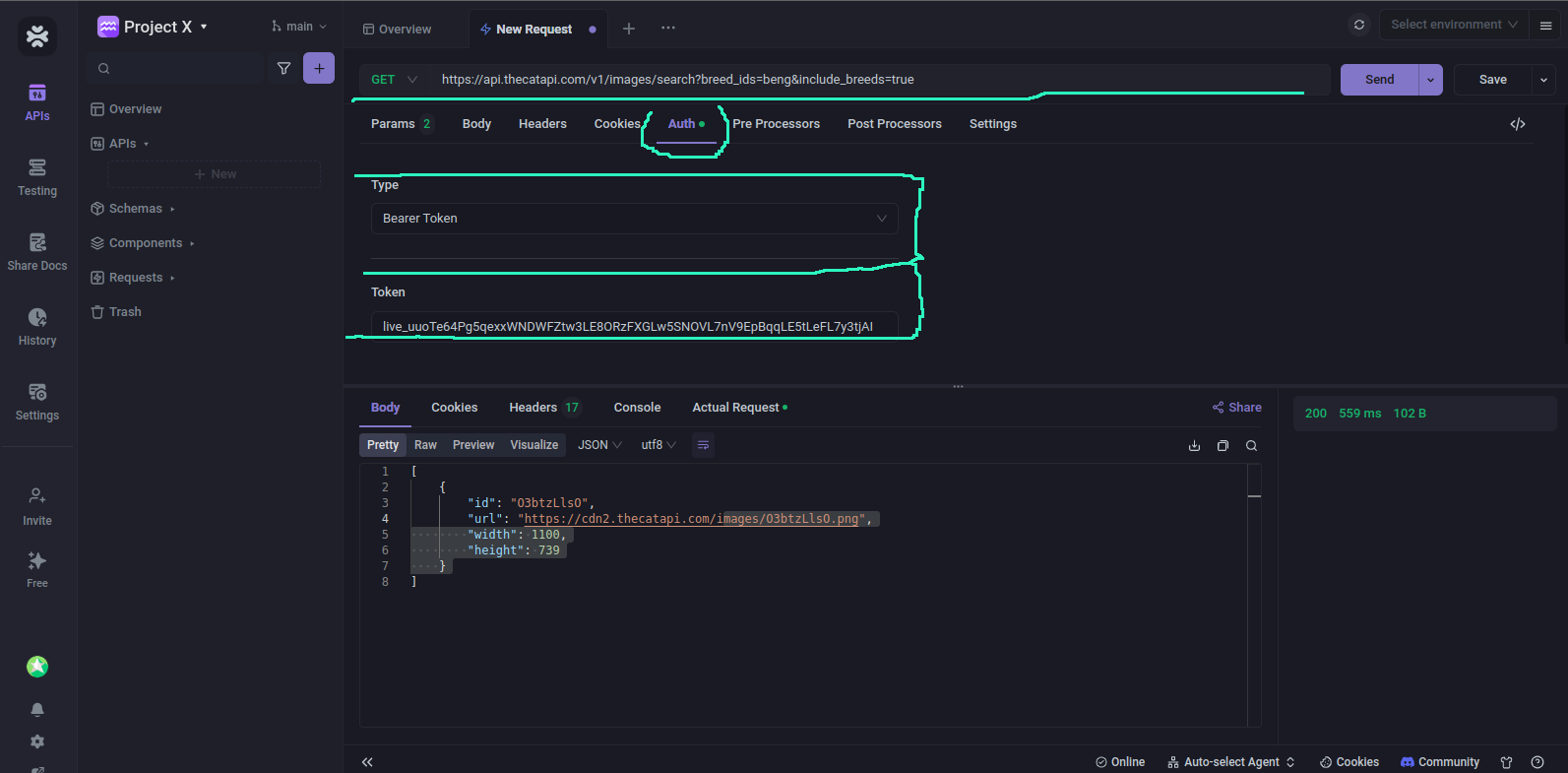
From the above image, all I needed to do was to provide the URL, the auth type, in this case, Bearer
and the token
. Apidog makes it super easy to do this.
Apidog also gives us the ability to choose different API key types;
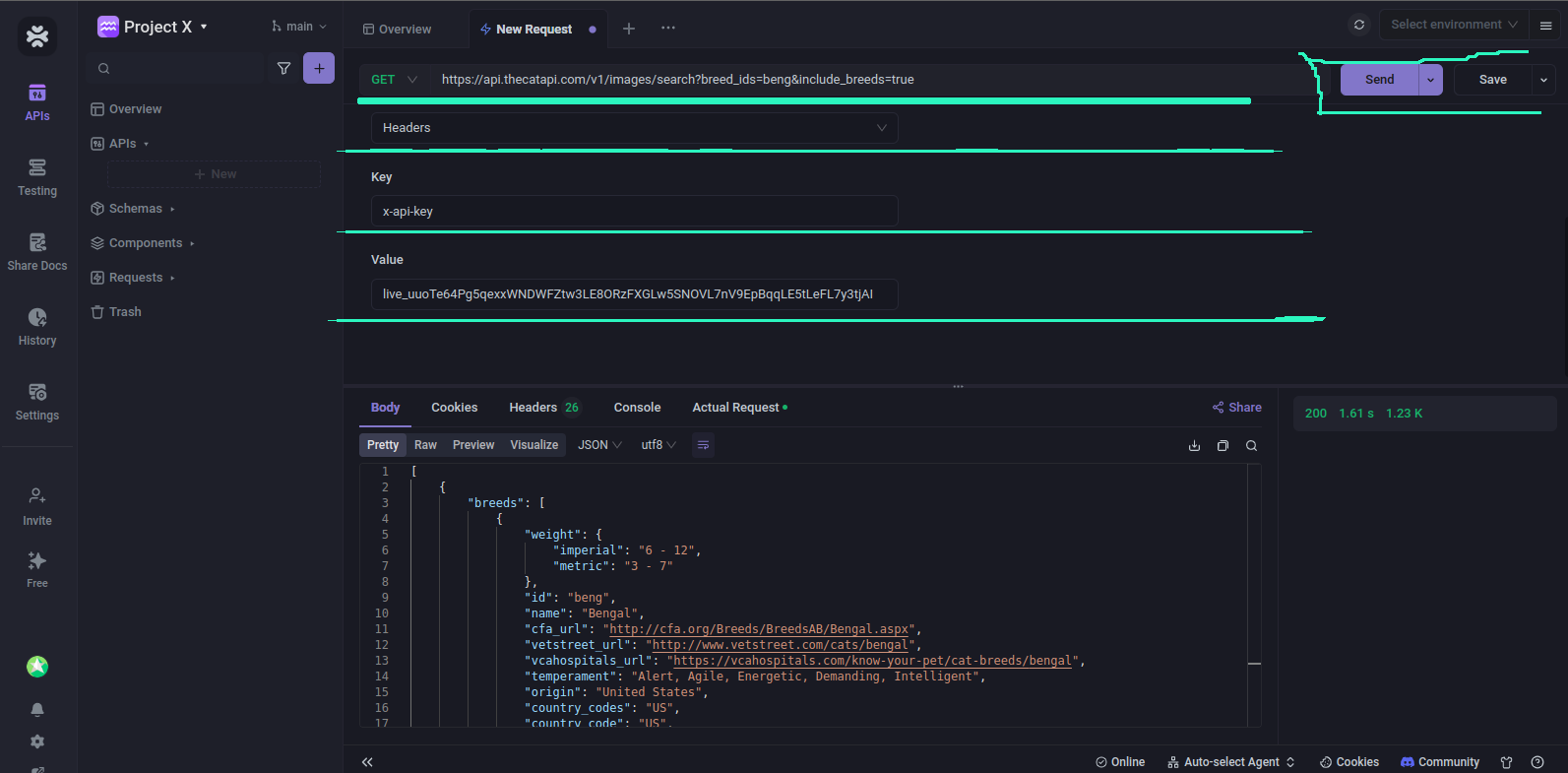
From the above screenshot, you can see that I used the x-api-key
type and it still worked. If you have different ways or types to use your API key, Apidog got you covered!
If you didn't add the api key
to requests that require it, the response would therefore be 401 unauthorized
.
C. Best Practices for API Key Management:
- Key Rotation: Regularly rotate API keys to mitigate the risk of unauthorized access and potential exposure. Implement a scheduled key rotation mechanism to generate new keys and invalidate old ones.
- Secure Storage: Store API keys securely, utilizing encryption and access controls to prevent unauthorized access. Avoid hardcoding API keys in your source code or storing them in plaintext files.
- Usage Policies: Enforce usage policies to govern the usage of API keys, including rate limits, access restrictions, and usage quotas. Monitor key usage metrics to identify anomalies and potential misuse.
D. Case Studies of API Key Implementation
Let's explore two real-world case studies of API key implementation:
- Google Maps API: Google Maps API utilizes API keys for authentication and access control. Developers generate API keys through the Google Cloud Console and integrate them into their applications to access mapping and geolocation services.
- Stripe API: Stripe API provides payment processing services to businesses worldwide, relying on API keys for secure authentication and authorization. Developers generate API keys through the Stripe Dashboard and incorporate them into their platforms to facilitate secure transactions.
You can check out these docs to see how to use the Stripe API.
By examining these case studies, we gain valuable insights into the practical implementation of API keys in real-world scenarios, underscoring their importance in enabling secure and seamless integration between applications and services.
Securing API Keys:
In an era marked by heightened cybersecurity threats and evolving regulatory frameworks, securing API keys has emerged as a critical imperative for organizations across industries. In this section, we delve into the multifaceted realm of API key security, exploring encryption techniques, access control mechanisms, environmental considerations, and strategies for mitigating vulnerabilities.
A. Encryption of API Keys:
Encryption of API keys is a crucial security measure aimed at protecting these sensitive credentials from unauthorized access and interception.
API keys, being unique identifiers that grant access to resources or functionalities within an application's API, are valuable assets for both legitimate users and malicious actors. However, if transmitted or stored in plaintext, API keys are vulnerable to interception by adversaries who could exploit them for unauthorized access to sensitive data or services.
Encryption involves transforming plaintext API keys into ciphertext using cryptographic algorithms. This process ensures that even if intercepted, the API keys are unintelligible to unauthorized parties without the corresponding decryption key.
By encrypting API keys, organizations add an additional layer of security to their systems, safeguarding these critical credentials from eavesdropping attacks and unauthorized access during transmission or storage. Advanced encryption standards such as AES (Advanced Encryption Standard) are commonly employed to encrypt API keys, providing robust protection against interception and exploitation.
In essence, encryption of API keys serves as a fundamental safeguard against unauthorized access and interception, bolstering the overall security posture of an organization's API-driven applications and services.
B. Access Control and Authorization:
Effective access control and authorization mechanisms are indispensable for enforcing granular access policies and mitigating unauthorized usage of API keys. Organizations must implement robust authentication mechanisms, such as OAuth 2.0 or JWT (JSON Web Tokens), to verify the identity of API key holders and authorize access to specific resources or functionalities based on predefined permissions. Role-based access control (RBAC) frameworks can further enhance security by delineating access privileges based on user roles and responsibilities.
C. API Key Security in Different Environments:
The security considerations surrounding API keys vary across different deployment environments, including web, mobile, and cloud-based infrastructures. In web environments, organizations must implement HTTPS (Hypertext Transfer Protocol Secure) to encrypt data transmission and mitigate interception attacks. Mobile environments necessitate additional safeguards, such as secure key storage mechanisms and runtime permission enforcement, to protect API keys from compromise through device tampering or application reverse engineering. Cloud-based environments demand stringent access controls and encryption protocols to safeguard API keys stored in distributed systems and infrastructure-as-a-service (IaaS) platforms.
D. Mitigating API Key Vulnerabilities:
Despite diligent security measures, API keys remain susceptible to various vulnerabilities and exploitation vectors. Organizations must proactively identify and mitigate potential vulnerabilities to fortify their API key security posture. Common vulnerability mitigation strategies include:
- Key Rotation: Regularly rotate API keys to limit their exposure window and mitigate the risk of unauthorized access. Automated key rotation mechanisms can streamline the process and ensure continuous security.
- Least Privilege Principle: Adhere to the principle of least privilege by granting API keys only the minimum permissions required to fulfill their intended purpose. Restrict access to sensitive resources and functionalities to mitigate the impact of potential breaches.
- Secure Key Storage: Employ robust encryption techniques and secure key storage mechanisms, such as hardware security modules (HSMs) or key management services (KMS), to protect API keys from unauthorized access and theft.
- Security Monitoring and Incident Response: Implement comprehensive security monitoring capabilities to detect anomalous API key activity and potential security incidents in real time. Develop a proactive incident response plan to swiftly mitigate security breaches and minimize their impact on business operations.
By adopting a holistic approach to API key security encompassing encryption, access control, environmental considerations, and vulnerability mitigation strategies, organizations can bolster their defenses against emerging threats and safeguard their digital assets from exploitation.
API Key Usage Tracking:
The landscape of API key management is replete with a myriad of tools, platforms, and services designed to streamline key provisioning, access control, and monitoring processes. From cloud-based key management services (KMS) to enterprise-grade API management platforms, organizations have access to a diverse array of solutions tailored to their unique requirements. Some popular tools and services for API key management include:
- Amazon Web Services (AWS) Key Management Service (KMS): A fully managed service for creating and controlling encryption keys used to encrypt data and manage access to AWS services and resources.
- Google Cloud Key Management Service (KMS): A cloud-hosted key management service that allows users to generate, use, rotate, and destroy cryptographic keys for encrypting data.
- Auth0: An identity and access management platform that offers robust API key management capabilities, including key generation, revocation, and monitoring, as part of its comprehensive authentication and authorization solutions.
- Kong: An open-source API gateway and microservices management platform that provides built-in API key authentication and authorization features, along with analytics and monitoring functionalities for managing API keys at scale.
By leveraging these tools and services, organizations can streamline API key management processes, enhance security posture, and optimize operational efficiency, thereby empowering developers and administrators to focus on innovation and value creation.
Conclusion:
As we draw to a close, let's recap the key points discussed in this comprehensive guide, emphasize the importance of proper API key management, and explore future directions in API key security.
A. Recap of Key Points
Throughout this guide, we've explored the intricate facets of API key management and security, covering topics ranging from the fundamentals of API keys to advanced techniques for securing, managing, and monitoring them. Key points include:
- Definition and significance of API keys in software development.
- Understanding API keys, including their types and security concerns.
- Best practices for implementing, securing, and managing API keys.
- Strategies for mitigating API key vulnerabilities and enhancing security posture.
- Tools and services available for streamlining API key management processes.
- The importance of encryption, access control, and monitoring in API key security.
- The role of logging, auditing, and usage tracking in maintaining transparency and accountability.
B. Importance of Proper API Key Management
Proper API key management is critical to ensuring the security, reliability, and compliance of modern software applications and services. API keys serve as the primary mechanism for authentication and access control, enabling seamless integration and interoperability between disparate systems. By adopting best practices for API key management, organizations can:
- Safeguard sensitive data and resources from unauthorized access and exploitation.
- Enhance transparency, accountability, and traceability throughout the API key lifecycle.
- Optimize resource utilization, performance, and scalability through efficient key provisioning and usage tracking.
- Demonstrate compliance with regulatory requirements and industry standards governing data privacy and security.
C. Future Directions in API Key Security
Looking ahead, the landscape of API key security is poised for continuous evolution and innovation in response to emerging threats, technological advancements, and evolving regulatory frameworks. Future directions in API key security may include:
- Integration of advanced authentication mechanisms, such as biometrics and multifactor authentication (MFA), to enhance API key security and mitigate identity-related risks.
- Adoption of decentralized identity frameworks, such as blockchain-based self-sovereign identity (SSI), to empower users with greater control over their digital identities and access credentials.
- Continued advancements in encryption techniques, access control models, and security protocols to address evolving threats and ensure robust protection of API keys and sensitive data.
- Collaboration and knowledge sharing among industry stakeholders to develop standardized best practices, guidelines, and frameworks for API key management and security.
By embracing these future directions and staying abreast of emerging trends and technologies, organizations can fortify their defenses against evolving threats and maintain a proactive stance towards API key security.
In conclusion, proper API key management is not merely a matter of compliance or best practice; it is a cornerstone of modern software development, underpinning the security, reliability, and trustworthiness of digital ecosystems. By adhering to the principles outlined in this guide and embracing a culture of continuous improvement and innovation, organizations can navigate the complexities of API key security with confidence and resilience.
Thanks for reading. Don't forget to reach out if you have any question, or need some help!