How to Write Automated Test Scripts (Best 2 Ways)
This article will introduce 2 great ways to guide you on how to write automated testing scripts. Let's get it.
Automated test scripts have become increasingly essential in modern software development. As software projects continue to expand in size and complexity, manual testing proves to be time-consuming and error-prone.
To address these challenges, developers, and testers rely on automated test scripts to ensure the quality and stability of software systems. In this article, we will explore the two best approaches for writing automated test scripts. By leveraging these methods, developers, and testers can streamline their testing processes and achieve more efficient and effective results.
What is Automated Testing?
Automated testing is verifying and validating that software meets all user requirements and performs as expected using automated tools. It checks for bugs, problems, and other types of defects that occur during and after the product development phase. This type of software testing runs on programming scripts that are handled by the testing tool. There are multiple testing tools that either provide a code-based platform or a code-free option for QA.
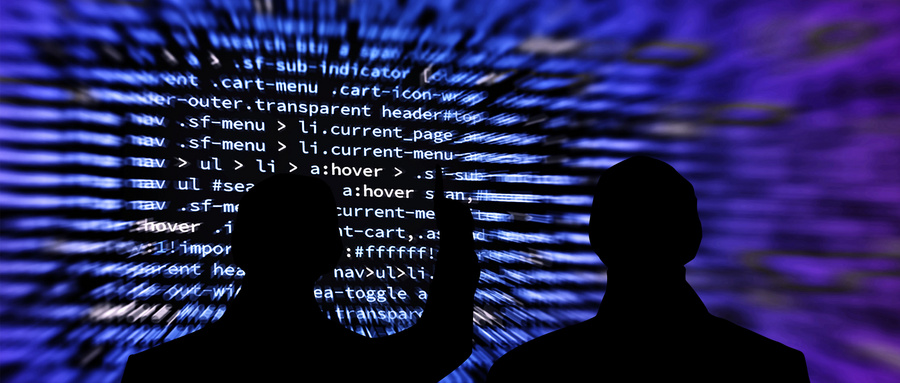
What is an Automation Test Script
Automation test scripting, also known as Testing Script, is the process of writing some script code to perform the functions of automated testing and can be written using and not limited to programming languages like javascript/java/python/PHP, etc.
How to Write Automated Testing Scripts Using Python and Selenium
Let's walk through a small example of what an automated test script really looks like and how to write it.
If you wanted to use Python + Selenium to test a small page to see if its input box search function works, it could write code like this:
library
# impoert the required library
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
# initialize browser
browser = webdriver.Firefox() # Firefox browser
# browser = webdriver.Chrome() # Chrome browser
# open the tested site
browser.get("http://www.example.com")
# excute test steps
search_box = browser.find_element_by_name("q")
search_box.send_keys("Hello World")
search_box.send_keys(Keys.RETURN)
# verify the test result
assert "No results found." not in browser.page_source
# close the browser
browser.close()
The code is actually an automated test script because after you write it, you can have it run hundreds or thousands of times and you don't even have to go back and make changes.
And the same goes for automation testing of APIs. You can also test APIs by writing code. We need to choose an API tool for automation testing. Today I choose Apidog to do this because Apidog supports automation testing.
Apidog: API Tool for Automation Testing
Now, we will explore the key features of Apidog and examine how it enhances the automation testing experience, empowering developers to write efficient and reliable test scripts. Apidog provides an intuitive, easy-to-use visual interface that enables testers to quickly create, edit and execute test cases.
- Apidog supports automation testing, which can quickly execute a large number of test cases and generate detailed test reports.
- Apidog also provides a Mock service, which can help testers simulate the behavior of API for better testing and debugging.
- Apidog can help testers better manage and organize test cases for better testing.
- Apidog provides powerful team collaboration features so that testers can better share test cases and test results, and collaborate to complete testing tasks.
How to Use Automation Test Scripts in Apidog
Creating Requests
We need to create a few requests and script the code in the latter part of the prescript in the request. The default scripting language for Apidog is javascript.
After filling in the path, method, and name, we need to write the custom script.
Writing Custom Scripts
If you are not very good at writing test scripts, don't worry, you can find that Apidog has prepared a lot of templates for us to check the script code.
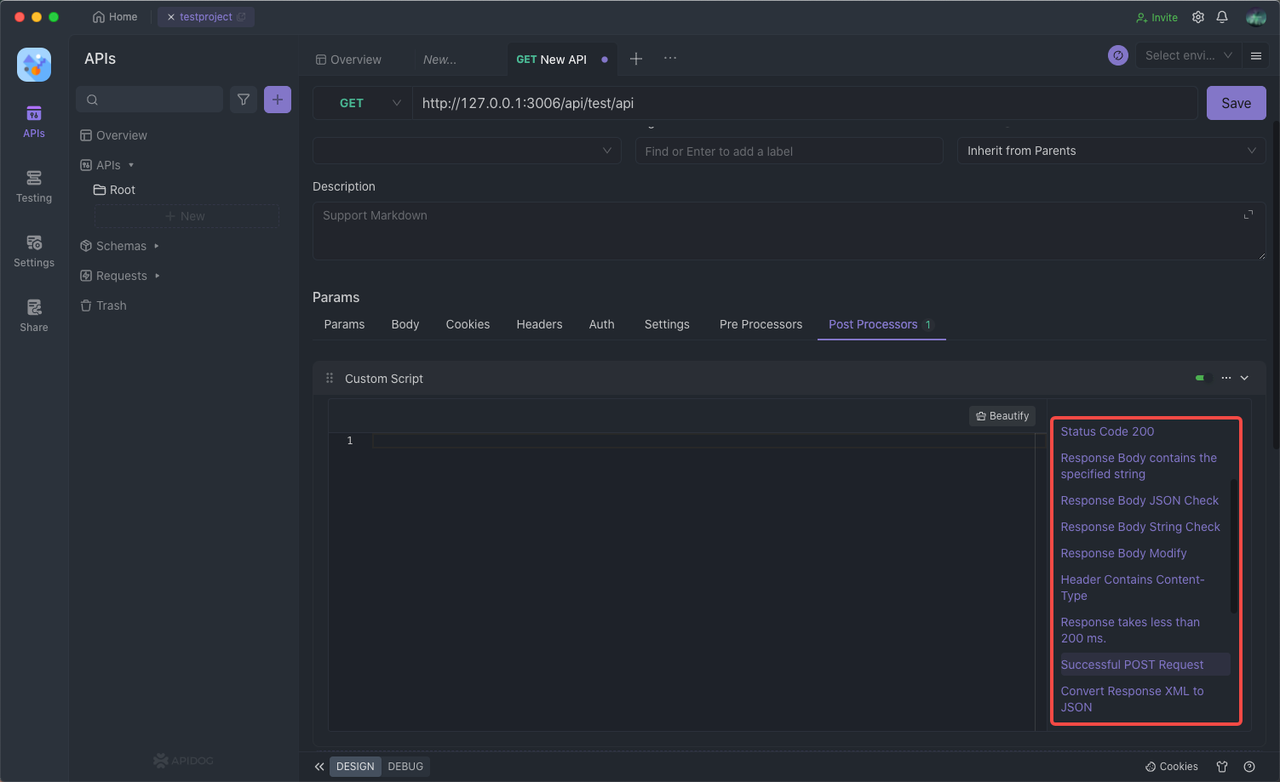
For example, the following script code:
// determine if the status code is 200
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
// determine if the body contains the target string
pm.test("Body matches string", function () {
pm.expect(pm.response.text()).to.include("string_you_want_to_search");
});
// body json check
pm.test("Your test name", function () {
var jsonData = pm.response.json();
pm.expect(jsonData.value).to.eql(100);
});
// body string check
pm.test("Body is correct", function () {
pm.response.to.have.body("response_body_string");
});
// change body
pm.response.setBody({});
// does header contain content-type
pm.test("Content-Type is present", function () {
pm.response.to.have.header("Content-Type");
});
// response time less than 200ms
pm.test("Response time is less than 200ms", function () {
pm.expect(pm.response.responseTime).to.be.below(200);
});
// post request successfully
pm.test("Successful POST request", function () {
pm.expect(pm.response.code).to.be.oneOf([201,202]);
});
You can set up multiple test checks for this request, for example by adding two here:
- Check if the return status code is 200
- if the request takes less than 200ms
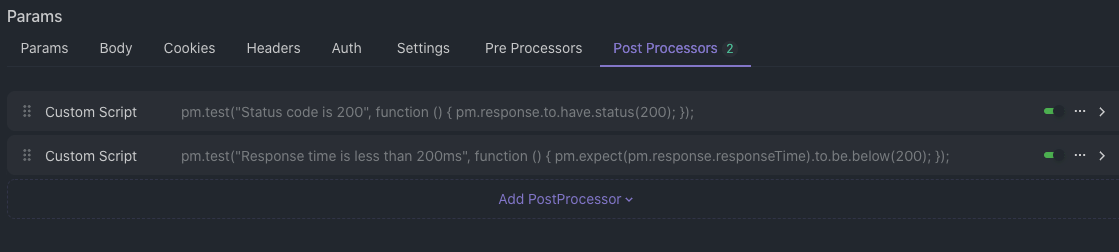
Click Save, you can run it first, and we can find that you get the expectation you want, and the verification passes.
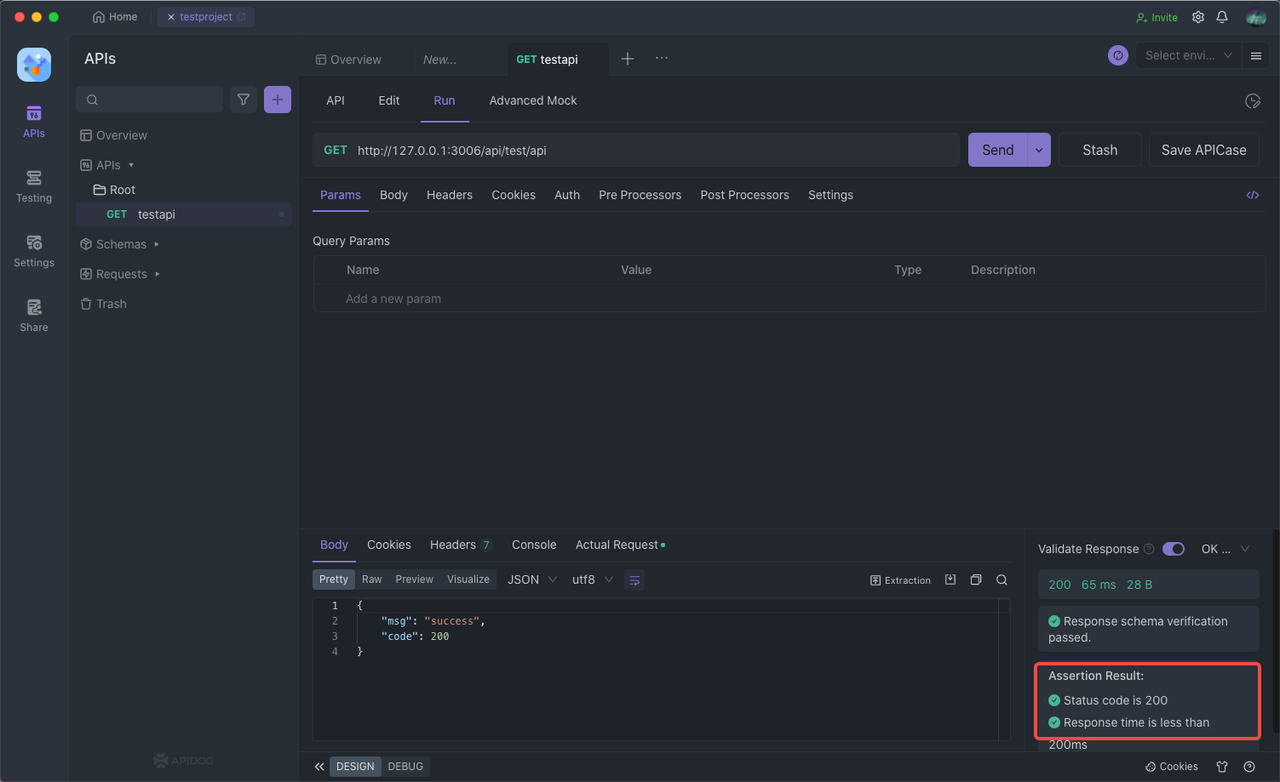
Apidog Automation Testing
Imagine if there are five APIs, it is impossible to send them one by one, so you need to use Apidog's automation testing function to help you save time.
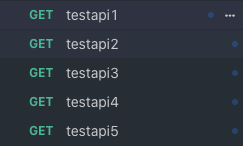
You need to enter the automation test interface and then import the corresponding interface.
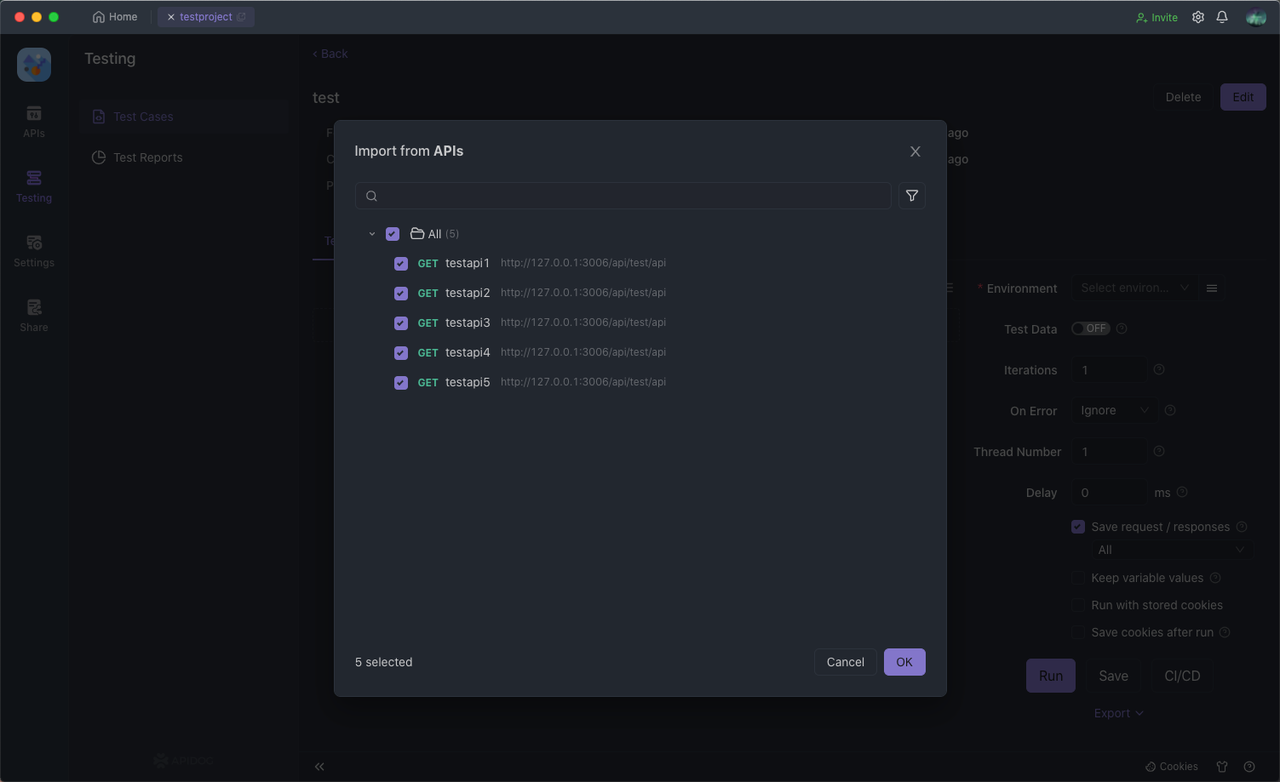
Finally, fill in the environment, number of loops, number of delays, etc., and run it.
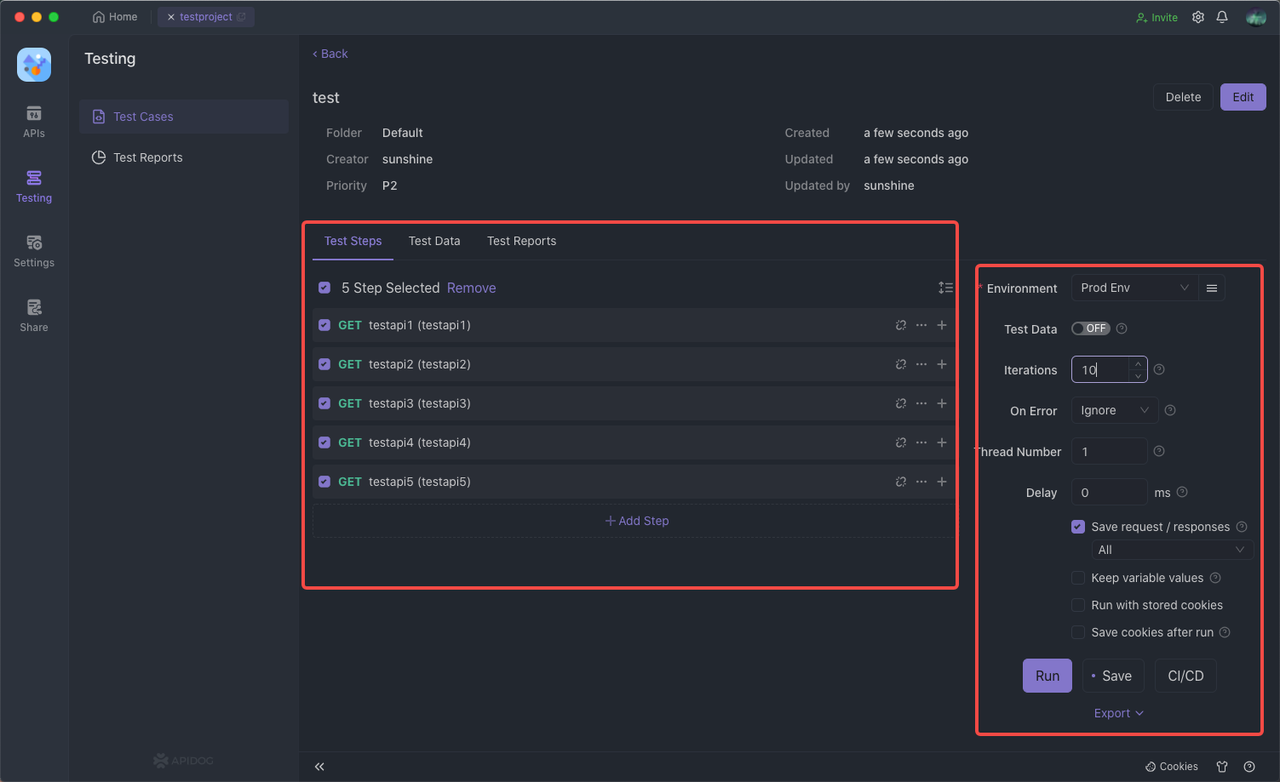
The result of the run can be viewed in terms of time spent, number of successes, number of failures, and other report parameters.
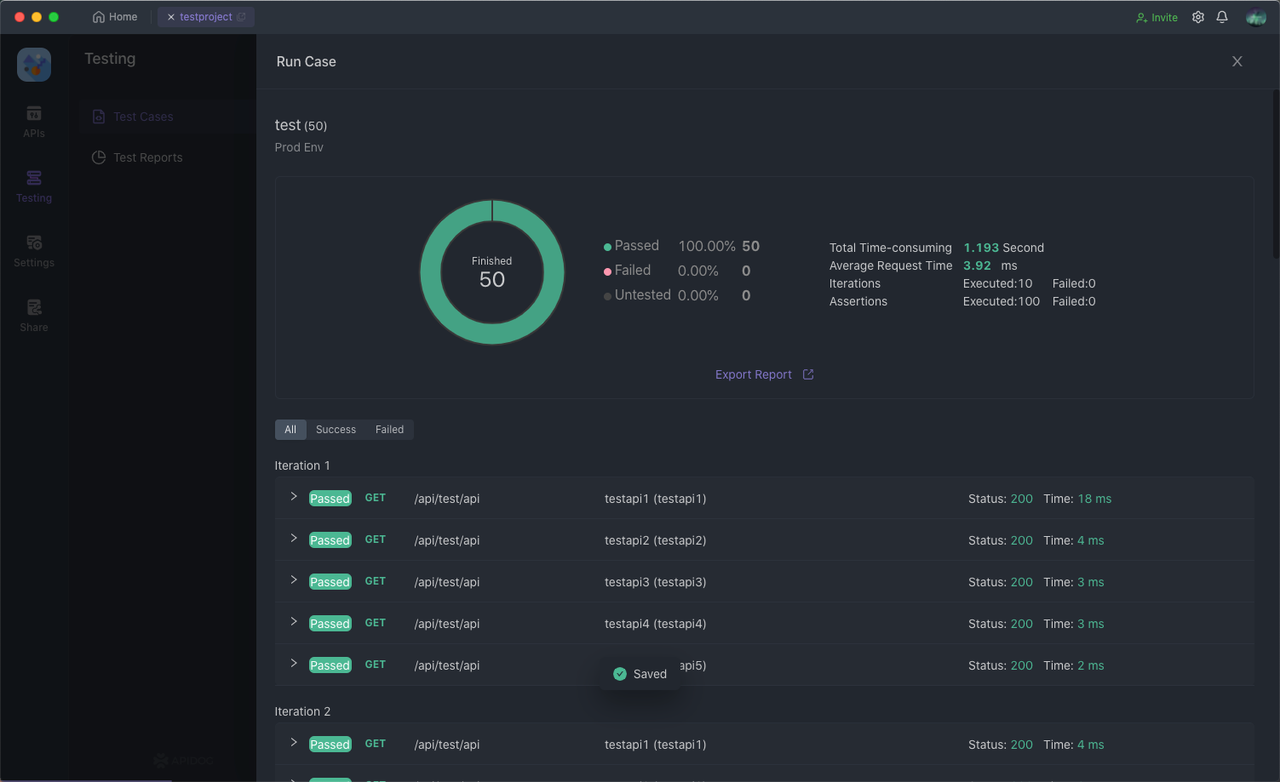
As you can see from the above, Apidog is a powerful and easy-to-use API testing tool. It can help users manage test cases, automation tests, simulation data, etc. It provides users with an intuitive and easy-to-use interface that helps testers better manage and organize test cases, and quickly execute and analyze test results. If you are looking for a powerful interface testing tool, we highly recommend you try it.
Bonus Tips: What are the Advantages of Automated Testing?
There are many reasons why automated testing is critical. The primary reason is the money and time it saves when executing manual test cases. But the benefits of automated testing go beyond this; it provides a gateway to perform complex testing processes, eliminate possible manual testing errors, and generate consistent, reliable results.
Where manual testing enables humans to analyze products and create test reports, automated testing is ideal for large projects that require iterative testing of features or that may have gone through the initial manual testing process.