How to Convert XML to JSON: A Step-by-Step Guide
Converting XML (eXtensible Markup Language) to JSON (JavaScript Object Notation) is a common task in web development and data interchange.
Converting XML to JSON is a common task in web development and data interchange. While XML and JSON serve similar purposes, their structures differ, and converting between them can be necessary for compatibility with various systems and applications.
In this guide, we'll explore a step-by-step process for converting XML to JSON using different tools like Apidog and programming languages.
What is XML and JSON?
XML (Extensible Markup Language) is a markup language used to define data with a self-describing structure. XML uses human-readable tags to organize and transmit data. It is widely used in various scenarios, such as configuration files, electronic data interchange (EDI), and more.
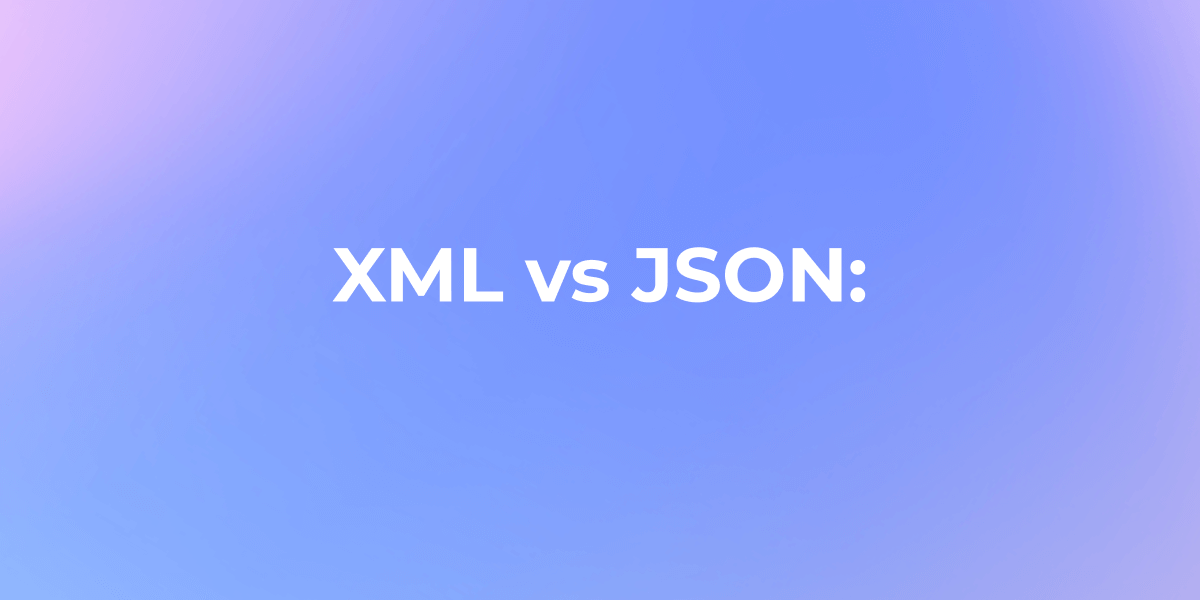
JSON (JavaScript Object Notation) is a lightweight data interchange format. It uses text that is easy for humans to read and write, while also being easy for machines to parse and generate. JSON organizes data in key-value pairs, and it is commonly used for data transmission between servers and web applications.
The main differences between the two are:
- XML focuses on data transmission and storage, primarily used for file transfers. JSON emphasizes data exchange and is widely used for network transmission.
- XML is a pure text format that can be parsed by both humans and machines. JSON is also a pure text format, but it is more lightweight and concise.
- XML has a strict hierarchical structure, while JSON's structure is relatively more flexible.
- XML supports multiple data types by default, while JSON only supports basic types like numbers, strings, and booleans.
Understand XML Data Structures:
Before diving into the conversion process, it's essential to understand the basic structures of both XML. XML (Extensible Markup Language) is a markup language designed to store and transport data. It uses a tree-like structure to represent data, with elements, attributes, and text content as the building blocks.
Elements: Elements are the fundamental building blocks of XML documents. They are represented by tags enclosed in angle brackets, such as <element>
. Elements can contain other elements, attributes, and text content.
Attributes: Attributes provide additional information about an element. They are defined within the start tag of an element, with a name and value pair separated by an equal sign, like <element attribute="value">
.
Text Content: Text content is the actual data stored within an element. It is the content between the opening and closing tags of an element, e.g., <element>This is the text content</element>
.
Root Element: Every well-formed XML document must have a single root element that contains all other elements.
Hierarchical Structure: XML documents are structured hierarchically, with elements nested within other elements, forming a tree-like structure. This structure allows for the representation of complex data relationships.
Tags: XML uses opening and closing tags to define elements. Opening tags are represented by <element>
, while closing tags are represented by </element>
. Elements must be properly nested and closed.
Here's an example of an XML document representing a book catalog:
<?xml version="1.0" encoding="UTF-8"?>
<catalog>
<book id="1">
<title>To Kill a Mockingbird</title>
<author>Harper Lee</author>
<year>1960</year>
</book>
<book id="2">
<title>Pride and Prejudice</title>
<author>Jane Austen</author>
<year>1813</year>
</book>
</catalog>
In this example, the root element is <catalog>
, which contains two <book>
elements. Each <book>
element has a unique id
attribute and contains child elements like <title>
, <author>
, and <year>
.
XML's hierarchical structure and ability to represent complex data relationships make it suitable for various applications, such as data exchange, configuration files, and document markup.
How to Convert XML to JSON in Python
Here we will show you how to convert XML to JSON with a built-in Python module with detailed steps.
- Import the Required Modules
First, you need to import the necessary modules. For this task, we'll be using the xml.etree.ElementTree
module to parse the XML data and the json
module to convert the parsed data to JSON.
import xml.etree.ElementTree as ET
import json
2. Parse the XML Data
Next, you need to parse the XML data using the ElementTree
module. You can either read the XML data from a file or provide it as a string.
From a file:
tree = ET.parse('path/to/your/file.xml')
root = tree.getroot()
From a string:
xml_string = """
<root>
<child1>Value 1</child1>
<child2>Value 2</child2>
</root>
"""
root = ET.fromstring(xml_string)
3. Convert the Parsed XML to a Python Dictionary
The ElementTree
module provides a convenient way to convert the parsed XML data into a Python dictionary using the ElementTree.tostringdict()
function.
xml_dict = {root.tag: {child.tag: child.text for child in root}}
This code creates a dictionary where the root tag is the key, and its value is another dictionary containing the child tags as keys and their corresponding text values.
4. Convert the Dictionary to JSON
Finally, you can use the json.dumps()
function to convert the dictionary to a JSON string.
json_data = json.dumps(xml_dict)
print(json_data)
This will output the JSON data:
{"root": {"child1": "Value 1", "child2": "Value 2"}}
Here's the complete code:
import xml.etree.ElementTree as ET
import json
# Parse the XML data
xml_string = """
<root>
<child1>Value 1</child1>
<child2>Value 2</child2>
</root>
"""
root = ET.fromstring(xml_string)
# Convert the parsed XML to a Python dictionary
xml_dict = {root.tag: {child.tag: child.text for child in root}}
# Convert the dictionary to JSON
json_data = json.dumps(xml_dict)
print(json_data)
Note that this approach assumes a relatively simple XML structure. If your XML data has a more complex structure (e.g., nested elements, attributes), you may need to modify the dictionary conversion step accordingly.
Apidog Supports XML and JSON Formats
Apidog is a versatile API documentation tool that enables users to upload and work with API documentation in XML and JSON formats. It facilitates comprehensive documentation, making it convenient for developers to describe, share, and interact with their APIs using either XML or JSON specifications.
This flexibility accommodates diverse preferences and project requirements in the API documentation process.
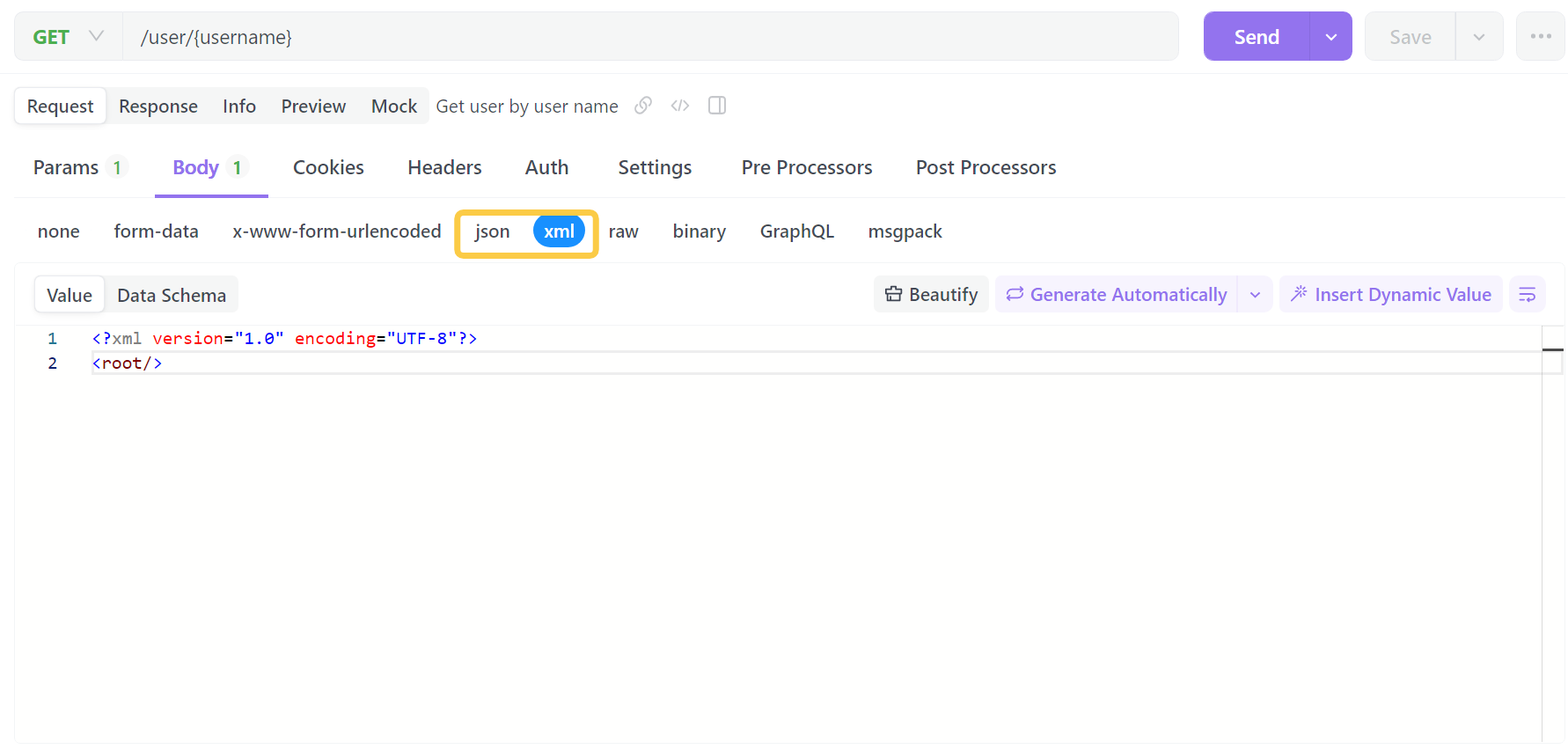
Conclusion:
Converting XML to JSON is a crucial task for ensuring compatibility and interoperability between different systems. By understanding the structures of both XML and JSON and utilizing the appropriate tools or programming languages, you can seamlessly perform this conversion, making your data more versatile and compatible across various platforms.