Types of APIs & Unique Features: You Should Know
In the ever-evolving tech landscape where applications are expected to connect seamlessly, APIs (Application Programming Interfaces) serve as the backbone for the exchange of data and services. From startups to tech giants, every business leverages different types of APIs to enhance functionality, streamline operations, and open new avenues for growth. To stay ahead in the digital game, developers and decision-makers must understand the nuances of API types and their underlying protocols. Let's dive into the world of APIs, exploring their varieties and key features, along with the core architectures that make inter-application communication possible.
Ready to elevate your API experience? Click the Download Button now!
How Does an API Work?
An API (Application Programming Interface) functions as an intermediary that enables different software applications to communicate and interact with each other. When an application sends a request to an API, the API forwards this request to the appropriate web server, processes the server’s response, and then delivers it back to the original application. This interaction is often conducted via standardized communication protocols like HTTP, allowing for the seamless exchange of data and functionality between disparate systems, thus enabling applications to leverage and extend their capabilities without direct linkage to each other.
Discovering the Variety of API Types
• Partner
• Internal
• Partner
Open APIs:
Open APIs, widely recognized as Public APIs, break down barriers to technological collaboration. By providing public access with minimal restrictions, Open APIs empower developers to build on top of existing platforms, fostering an ecosystem of innovation.
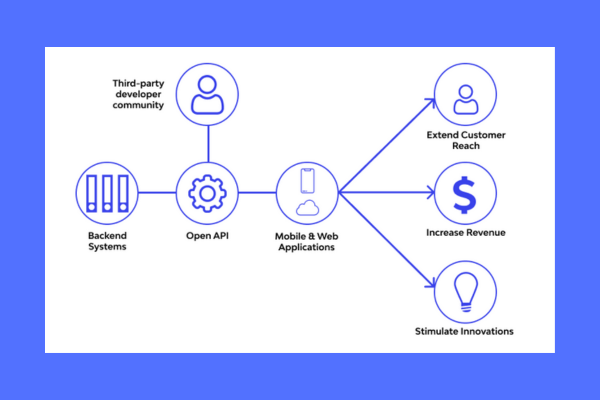
import requests
def get_weather(api_url, city):
response = requests.get(f"{api_url}/weather/{city}")
if response.status_code == 200:
return response.json()
else:
return "Error fetching weather data."
# Example usage
api_url = "http://api.weatherapi.com/v1"
city = "New York"
weather_data = get_weather(api_url, city)
print(weather_data)
Unique Features of Open APIs:
Accessibility: Open to developers, businesses, and the public, maximizing potential use cases and partnerships.
Transparency: Typically accompanied by comprehensive documentation for straightforward implementation.
Community Engagement: Encourage a developer community to create add-on value, expanding the parent service’s capabilities.
Partner APIs:
Exclusive to strategic partnerships, Partner APIs create a secure bridge between businesses, facilitating specialized interactions and seamless B2B integration.
def access_partner_api(api_url, api_key):
headers = {'Authorization': f'Bearer {api_key}'}
response = requests.get(api_url, headers=headers)
return response.json()
# Example usage
api_url = "https://partnerapi.example.com/data"
api_key = "YOUR_API_KEY"
partner_data = access_partner_api(api_url, api_key)
print(partner_data)
Unique Features of Partner APIs:
Controlled Accessibility: Access is provided selectively, ensuring secure data exchange.
B2B Integration: Perfect for creating a tight-knit ecosystem of partners with aligned business goals.
Monetization and Growth: A pathway to new revenue streams by extending services to trusted business partners.
Internal APIs:
Internal APIs, also known as Private APIs, are the unsung heroes of organizational efficiency. They serve internal purposes, helping various company departments interact more effectively with their tech stacks.
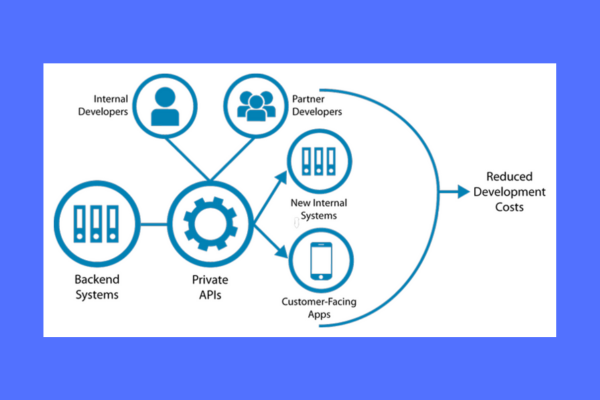
def get_employee_data(internal_api_url, employee_id):
response = requests.get(f"{internal_api_url}/employee/{employee_id}")
return response.json()
# Example usage
internal_api_url = "http://internalapi.company.com"
employee_id = 12345
employee_data = get_employee_data(internal_api_url, employee_id)
print(employee_data)
Unique Features of Internal APIs:
- Optimized Security: Restricted to internal networks, offering enhanced data protection.
- Streamlined Operations: Facilitate better intracompany communication and service utilization.
- Customization: Tailored to fit the specific needs of an organization, without external constraints.
Composite APIs:
When service integration complexity rises, Composite APIs come to the rescue. These APIs combine different service and data APIs to deliver a united output, simplifying client-side interactions.
def get_user_dashboard_data(user_id):
profile_data = requests.get(f"http://internalapi.company.com/profile/{user_id}")
project_data = requests.get(f"http://internalapi.company.com/projects/{user_id}")
if profile_data.status_code == 200 and project_data.status_code == 200:
return {
"profile": profile_data.json(),
"projects": project_data.json()
}
else:
return "Error fetching data."
# Example usage
user_id = 101
dashboard_data = get_user_dashboard_data(user_id)
print(dashboard_data)
Unique Features of Composite APIs:
Efficient Data Retrieval: Streamlines complex tasks by aggregating multiple service calls into one.
Performance Optimization: Reduces server load and client wait times, offering an improved user experience.
Microservices Friendly: Ideal for architectures requiring various services to be coordinated for a single user action.
API Architectures and Protocols
• REST
• GraphQL
• RPC
SOAP (Simple Objects Access Protocol):
SOAP standards ensure secure and transactional communication between web services, making it the choice for enterprises that prioritize strict security and transactional reliability.
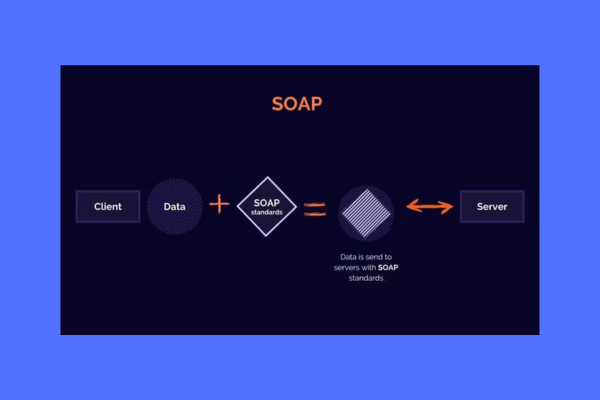
SOAP's Unique Features:
- Security and Standards: Inbuilt comprehensive WS-Security features.
- Reliability: Facilitates complex transactions with its ACID compliance.
- Statefulness: Allows for stateful operations, critical for certain scenarios.
REST (Representational State Transfer):
With its simplicity and compatibility with HTTP, REST remains the favorite amongst developers for creating APIs that are efficient, scalable, and easy to work with.
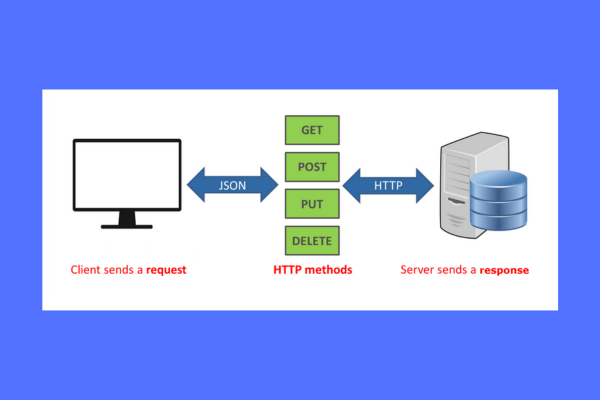
REST's Unique Features:
- Simplicity and Speed: A straightforward approach for API development.
- Flexibility: Supports multiple data formats beyond XML, such as JSON.
- Statelessness: Fosters scalability with stateless server communication.
GraphQL: The Query Language Revolutionizing Data Retrieval
GraphQL empowers clients to fetch exactly what they need, no more, no less. It's a game-changer for dynamic data needs and real-time applications.
Unique Features of GraphQL:
- Precise Data Fetching: Minimizes over and under-fetching of data.
- Efficient Networking: Reduces network traffic with tailored requests.
- Real-time Updates: Offers subscription services for live data updates.
gRPC (Google Remote Procedural Call):
gRPC, built on the modern HTTP/2 protocol and using Protocol Buffers, is optimized for high performance and low latency, valuable for microservices and inter-service communication.
Unique Features of gRPC's:
- Performance: Designed for speed and efficient communication.
- Streaming Capabilities: Supports bidirectional streaming and excellent real-time data transfer.
- Language Agnostic: Provides tools to work across languages and platforms.
RPC (Remote Procedural Call):
RPC is the protocol that keeps things traditional yet effective, abstracting the complexity of network communications as if they are local procedure calls.
Unique Features of RPC:
- Ease of Use: Simplifies network communication to resemble local functions.
- Diverse Implementations: Variations like JSON-RPC add flexibility to this method of communication.
Apache Thrift: The Efficient Cross-Language Service Framework
Born out of Facebook, Apache Thrift is the framework for efficient and consistent cross-language development, catering to the need for scalable and interoperable services.
Unique Features of Apache Thrift's :
- Cross-Language Services: Breaks the language barrier in service development.
- Optimized Protocols: Utilizes a compact binary protocol for data transfer efficiency.
- Scalability: A robust framework designed for growing service ecosystems.
How to Access in Apidog API Hub
Apidog API Hub is a comprehensive platform that provides a variety of APIs for developers. Whether you're a professional or a hobbyist, accessing these APIs can enhance your projects with new data and functionalities. The process is straightforward and user-friendly, enabling you to quickly integrate diverse APIs into your applications.
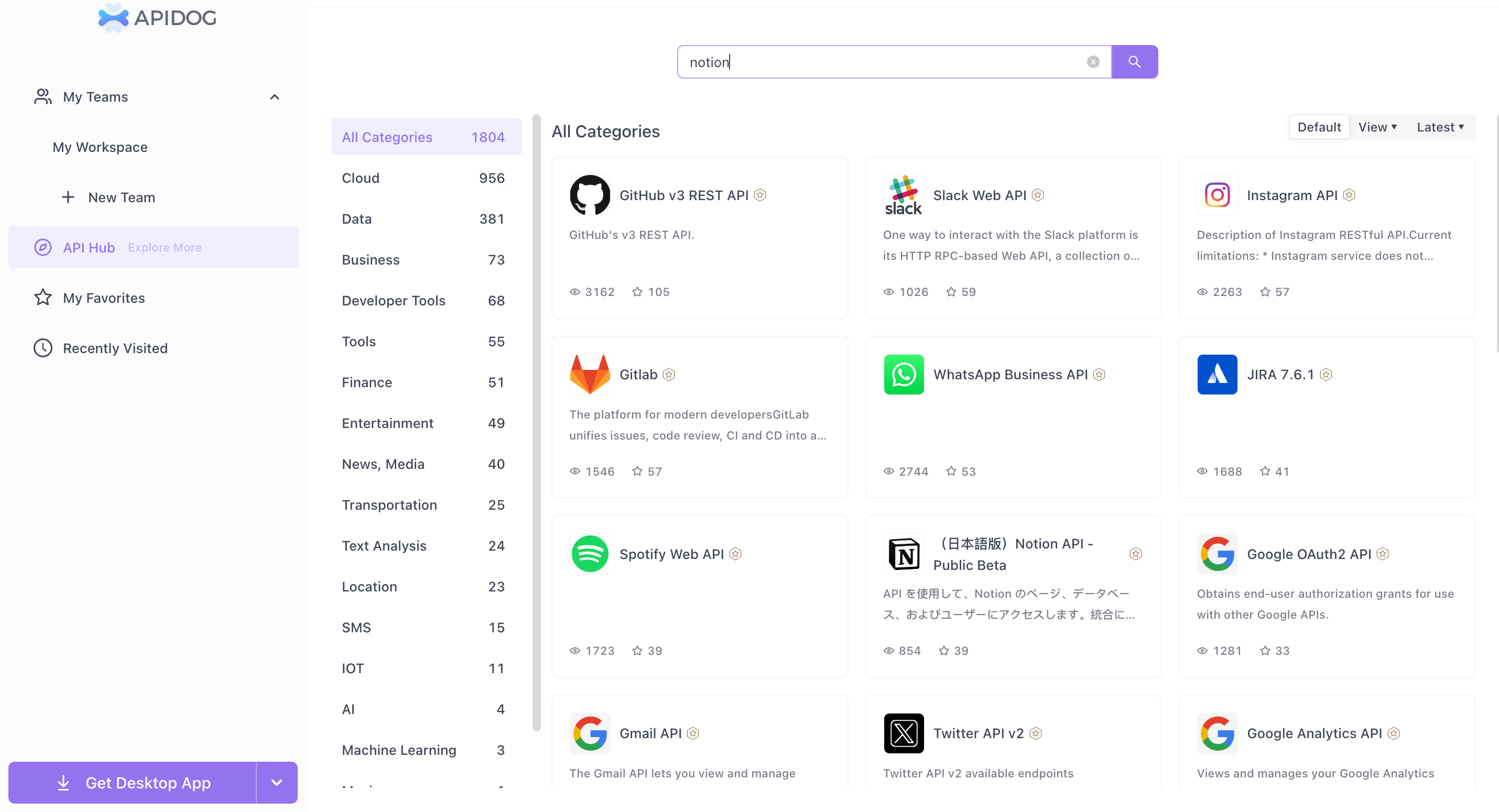
5 Simple Steps to Access APIs in Apidog API Hub
Step 1: Create an Account
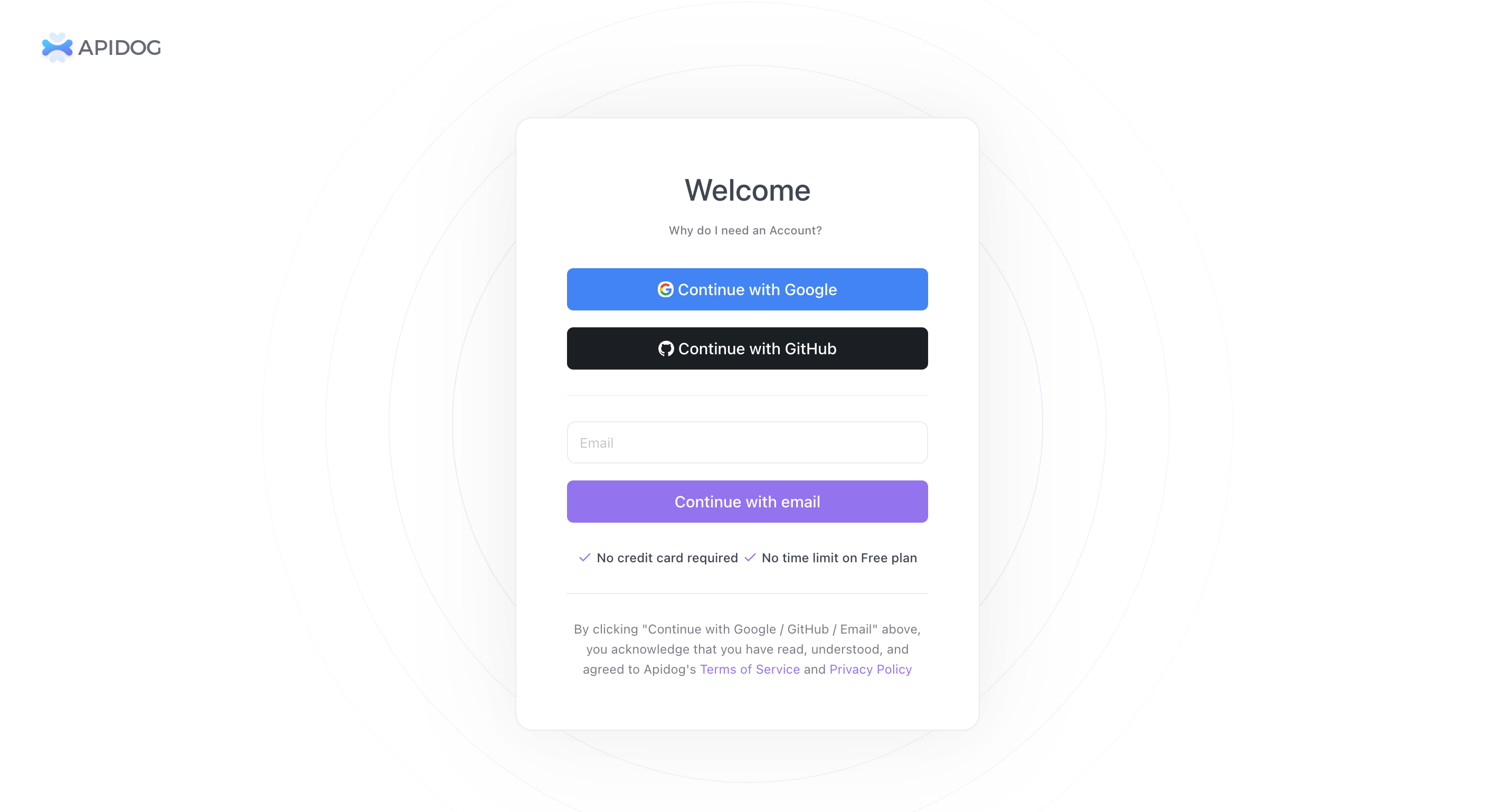
- Visit Apidog API Hub: Go to the Apidog API Hub website.
- Sign Up: Register for an account by filling in the required details.
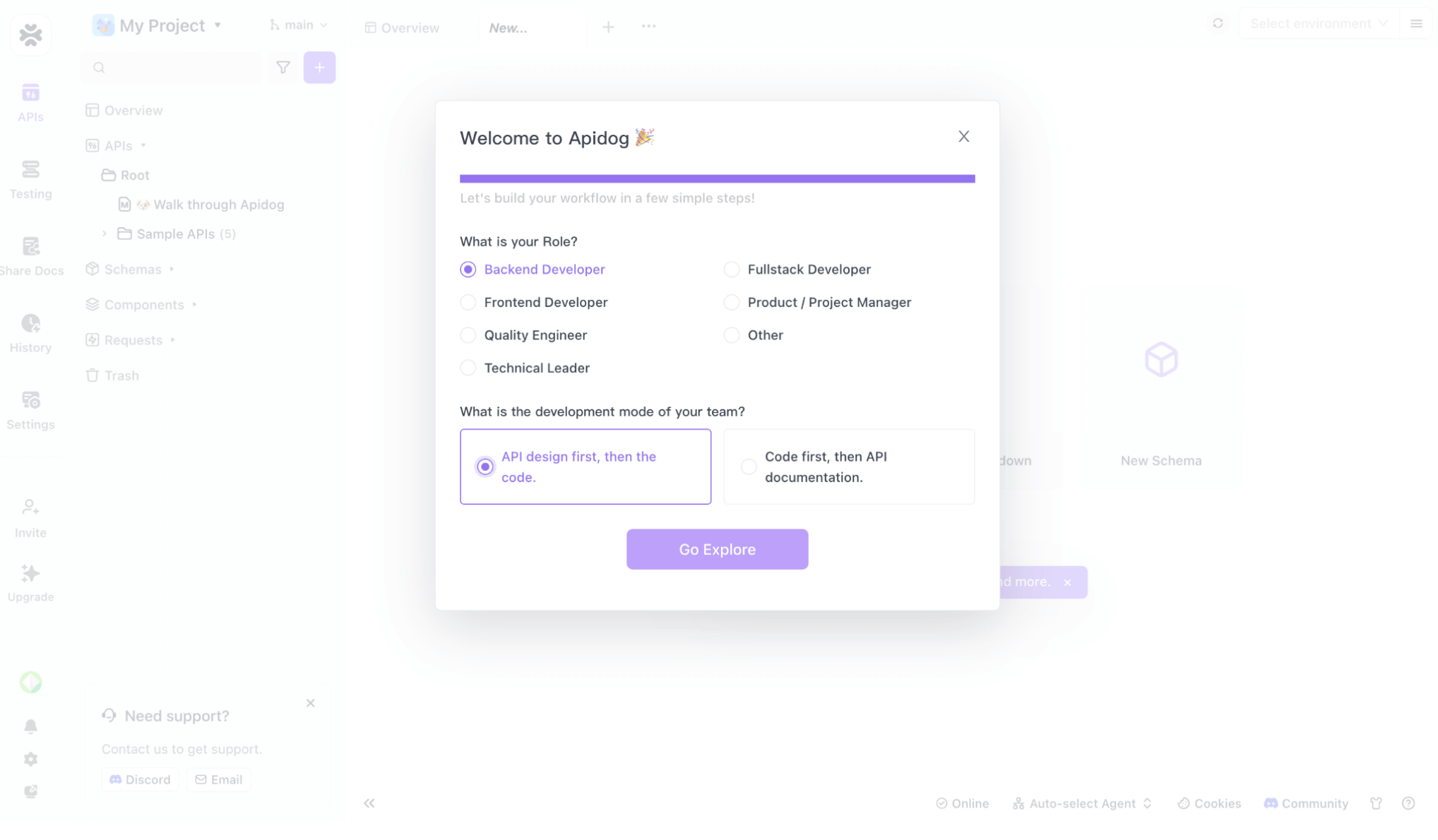
Step 2: Explore the APIs
- Log In: Access your Apidog account.
- Browse: Navigate through the platform to discover various available APIs.
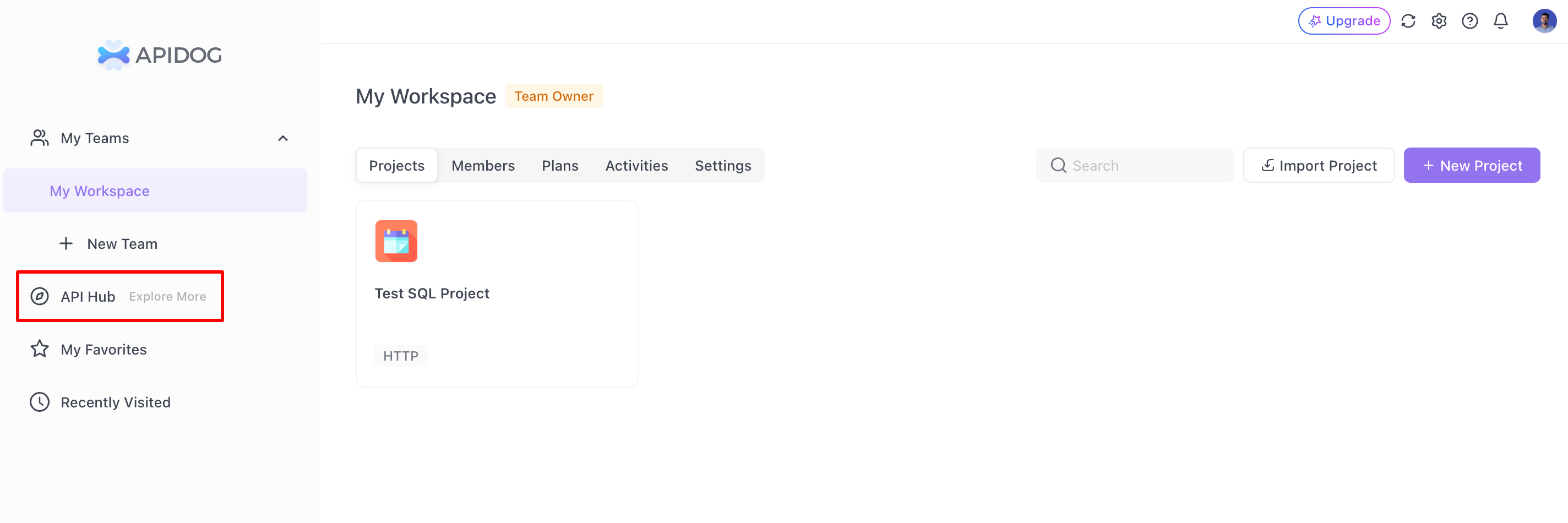
Step 3: Select Your Desired API
- Identify: Choose an API that suits your project's needs.
- Read Documentation: Familiarize yourself with its documentation for proper usage guidelines.
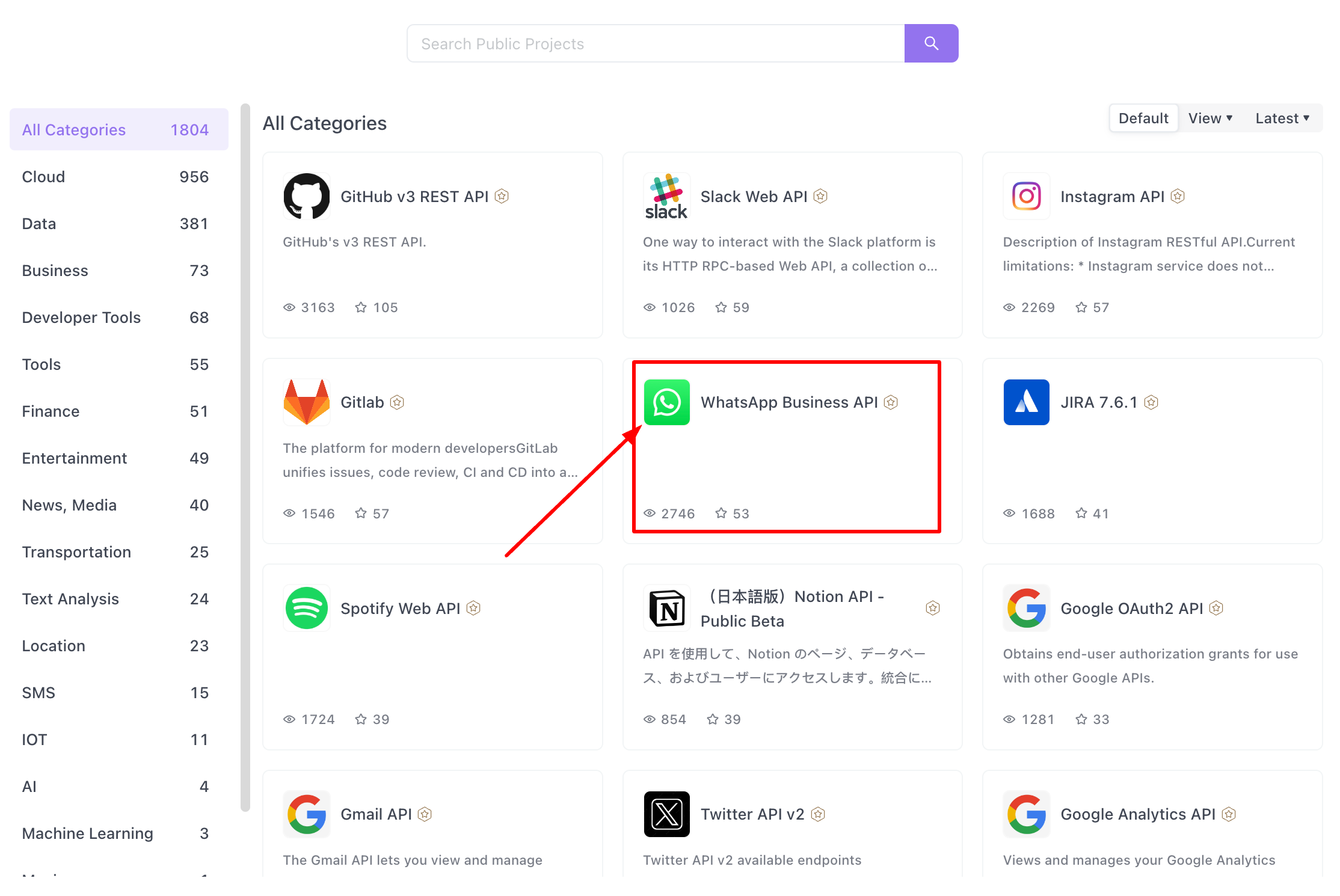
Step 4: Obtain API Key
- Register for API Key: Some APIs may require an API key, which you can typically obtain by registering for it on the API’s page.
- Store API Key Securely: Remember to keep your API key confidential and safe.
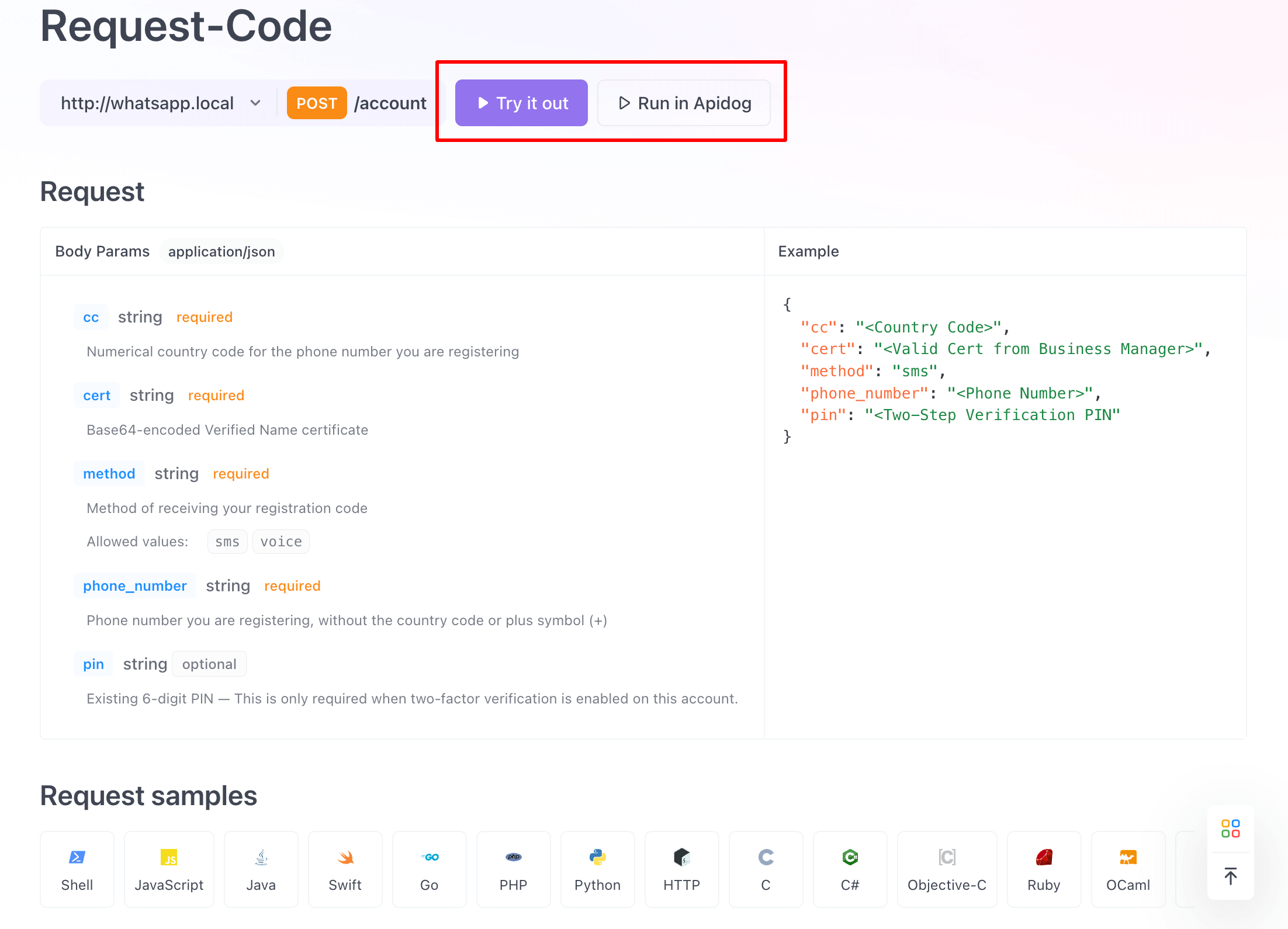
Step 5: Make Your First API Call
- Set Up: Prepare your environment for API calls, using tools or programming languages like Python.
- Execute: Make your first API request using the obtained API key, following the guidelines provided in the API documentation.
Conclusion
Understanding the range of API types, their unique features, and the distinctive architectures that support them is not only beneficial—it's essential for success in today's interconnected digital environment. Whether enhancing public engagement, nurturing partner ecosystems, sharpening internal operations, or managing complex service integrations, APIs hold the key to unlocking seamless digital experiences and opportunities for innovation. With this knowledge, developers and businesses can make informed decisions, driving technological advancements and achieving strategic objectives.