Why Flask?
When it comes to building APIs, especially for smaller or medium-sized applications, Flask is like the perfect assistant, it’s minimalist, flexible, and lets you focus on what truly matters. Unlike heavier frameworks, Flask doesn’t throw everything at you from the get-go. It gives you just enough to get started, and from there, you can add features as needed.
Flask lets developers have a sense of creative freedom.
Want to quickly spin up a RESTful API?
Flask can handle it without any unnecessary bloat.
Need to scale later?
No problem! You can easily integrate more complex setups with Flask extensions.
High-Level Flask Application Architecture:
The chart outlines the structure of a Flask app, showing how routes, controllers, models, and templates interact to handle a request.
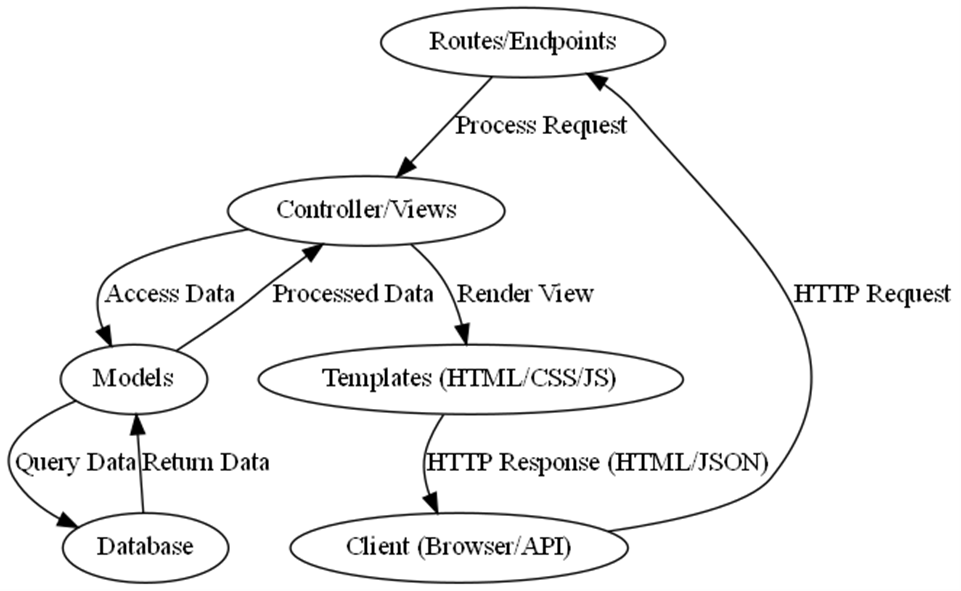
API Request-Response Flow Chart
The flowchart shows how a client request travels through the Flask server, route, logic, and database, before returning a response.
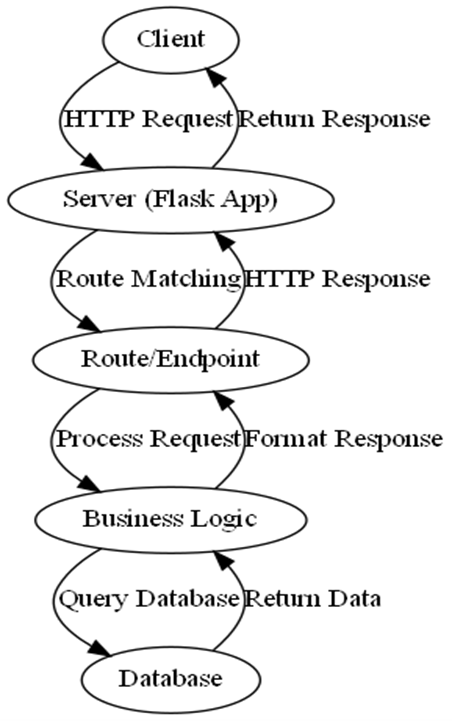
Endpoint Interaction Map
• The diagram illustrates different API endpoints and the HTTP methods used to interact with them (GET, POST, PUT, DELETE).
•The diagram further shows how different API endpoints (GET, POST, PUT, DELETE) are related to the base Flask API.
•Each node represents an endpoint and HTTP method, showing their interaction with the Flask app.

API Workflow with Swagger Documentation
The infographic shows how API endpoints can be auto-documented with Swagger, highlighting its integration into the Flask app.
•The flow demonstrates how the client interacts with the Flask API, and how Swagger automatically generates documentation for the API endpoints.
•Swagger UI is presented as an intermediary that allows users to interact with and view the documentation for the API endpoints.
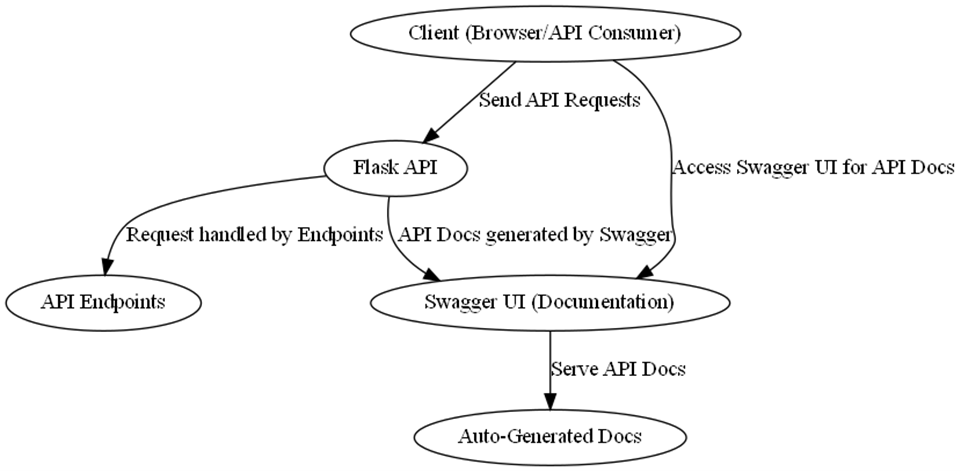
Flask API Security Diagram
Security practices involve using authentication with Flask-JWT or OAuth;
1. JWT Authentication Flow:
The JWT Authentication flow shows the steps in a typical JWT-based authentication flow as illustrated in the diagram;
• The client sends login credentials to the API.
• The API validates the credentials by checking the user information in the database.
• The API generates a JWT token and sends it back to the client.
• The client includes the JWT token in future requests, and the API validates the token to authorize the client.
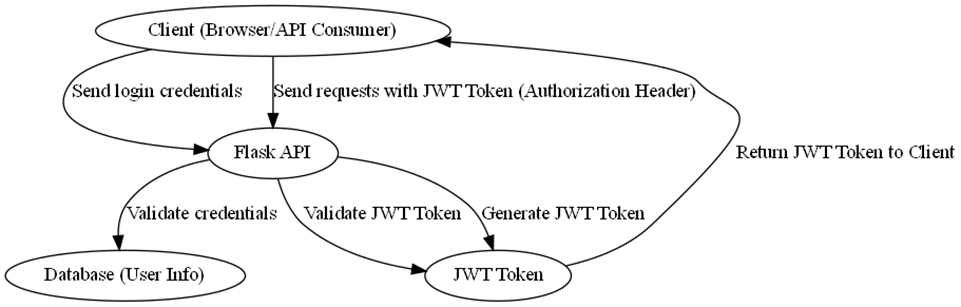
2. OAuth Flow
In the OAuth flow;
• The client requests authorization from the authorization server.
• The authorization server returns an authorization code to the client.
• The client exchanges the code for an access token.
• The client uses the access token in future requests to the API server, and the API server validates the token.
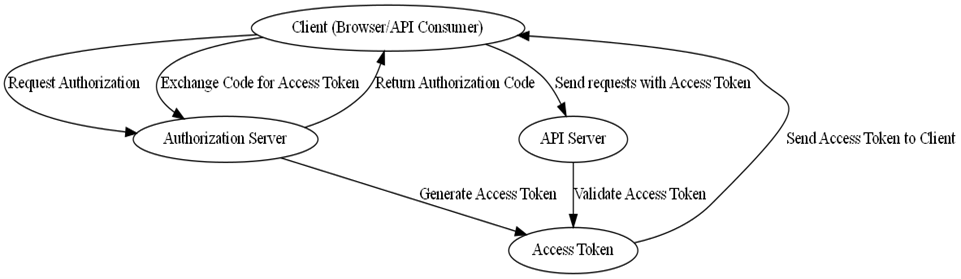
Now before we dive into the Beauty of Flask API Documentation, let’s pause for a moment and talk about an essential part of the development process—collaboration tools. Whether you're working alone or with a team, having the right tools makes all the difference.
Here’s where APIDog comes in. Think of it as your go-to solution that offers all the powerful features you love from Postman’s paid version, but at a fraction of the cost. And trust me, switching over is effortless! With just a few clicks, APIDog handles the migration for you—no hassle, no complicated steps.
But that's not all.
APIDog boasts a clean, user-friendly interface that gets you up and running in no time, especially if you're already familiar with Postman. The sleek design isn’t just visually appealing; it’s built for collaboration, with intuitive workflows that keep your productivity high.
Oh, and did I mention it has Dark Mode? Now you can code and collaborate comfortably, day or night!
Why waste time struggling with clunky tools when you can have an elegant, easy-to-use solution? APIDog makes your API testing and documentation process seamless, saving you time and making your development smoother.
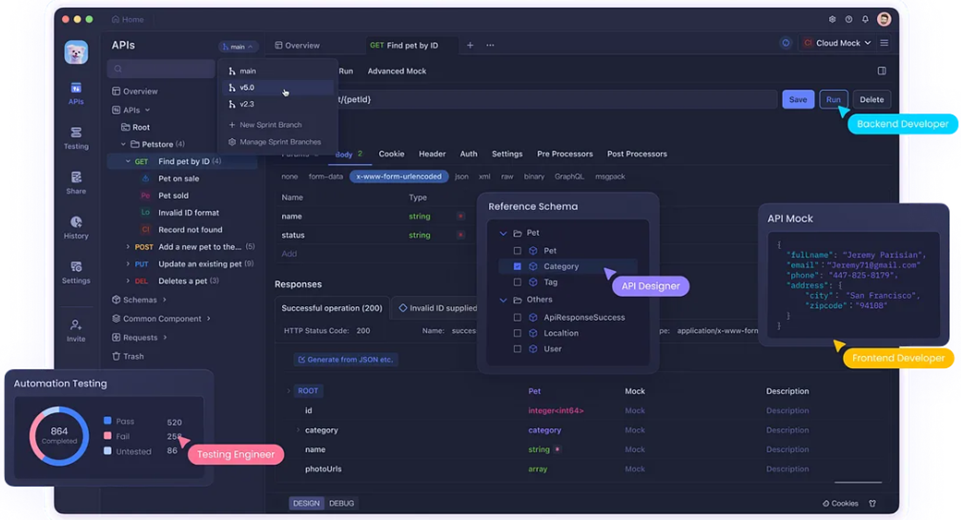
The Beauty of Flask API Documentation
Let’s face it, documentation can be a deal-breaker. A great API can lose its charm if the docs are hard to navigate. This is where Flask’s ease of use translates beautifully into how APIs are documented. A couple of things make Flask API documentation a smooth experience:
Simplicity: Flask itself is easy to understand, so documenting an API built with it is equally straightforward. The framework has a clean and readable structure, which means less confusion when describing routes, parameters, and methods.
Flask-RESTful & Swagger: You can use powerful extensions like Flask-RESTful to handle your API routes, and you can integrate Flask-Swagger to automatically generate interactive, user-friendly API documentation. So your API not only functions well, but the documentation will also look professional without requiring tons of extra work.
Why You Should Use Flask for Your API Documentation
Building an API is one thing. Getting others to use it? That’s where good documentation is crucial. Flask’s flexibility means you can document your API just as easily as you build it. Here's why Flask shines in this department:
Auto-documentation: Using tools like Flask-RESTPlus or Flask-Swagger, your documentation can be auto-generated. Imagine having your routes documented without much manual effort.
Interactive Docs: With Swagger UI, your API docs are more than just text—they’re interactive. Users can test endpoints directly from the documentation page, making their lives (and yours) a lot easier.
OpenAPI Integration: Flask plays well with OpenAPI, the industry-standard format for describing APIs. This allows you to take advantage of a broad ecosystem of tools for API management, testing, and collaboration.
Ultimately,it's prudent to choose Flask for a Smooth API Experience;
In a world where APIs are the backbone of communication between systems, having well-documented, easy-to-understand APIs is vital. Flask makes this process not only possible but also pleasurable.
Its simplicity, combined with its flexibility and integration options, allows developers to not only build but also clearly explain their APIs. And with tools like Swagger making documentation interactive, you’re giving your users an API experience that’s both powerful and delightful.
Setting Up a Flask Project: A Simple, Step-by-Step Guide
If you're looking to dive into API development with Flask, you’re in for a smooth ride. Flask is lightweight, flexible, and perfect for getting your APIs up and running quickly without unnecessary overhead. Let’s walk through the process of setting up a basic Flask project and creating your first API in just a few steps.
Step 1: Install Flask
First things first—if you don’t already have Flask installed, let’s get that sorted. Installing Flask is as easy as running a simple command.
Here’s how to do it:
pip install Flask
Boom! That’s it. Flask is now ready to go. If you haven't already, I recommend setting up a virtual environment for your project to keep your dependencies isolated.
Step 2: Create Your Flask Project
Next up, we’ll create a basic project structure. In your project directory, let’s create a Python file—say, app.py. This will be the heart of your API.
mkdir flaskproject
cd flaskproject
touch app.py
In app.py, we’ll set up a super basic Flask application. Don’t worry; you’ll see how simple this is!
from flask import Flask
app = Flask(name)
@app.route('/')
def home():
return "Welcome to my Flask API!"
if name == 'main':
app.run(debug=True)
So, what’s happening here?
• Flask(name) initializes the app.
• @app.route('/') defines a route—a URL endpoint. When you visit the home URL (/), the app will trigger the home function and return a message.
• app.run(debug=True) starts your app with debug mode turned on so you can see detailed error messages.
Step 3: Run Your Flask App
Now that you’ve got your app set up, let’s see it in action. In your terminal, run:
python app.py
- Running on http://127.0.0.1:5000/
Visit http://127.0.0.1:5000/ in your browser, and you should see “Welcome to my Flask API!” Awesome, right? You've just set up a basic Flask project!
Step 4: Define API Endpoints
Now, let’s expand this by adding a couple of API endpoints. APIs are all about sending and receiving data, so let’s create routes that can do just that. For example, let’s say we want to create an endpoint to return a list of users in JSON format.
Here’s how you can do it:
from flask import Flask, jsonify
app = Flask(name)
# Home route
@app.route('/')
def home():
return "Welcome to my Flask API!"
# API route to get users
@app.route('/users', methods=['GET'])
def get_users():
users = [
{"id": 1, "name": "John Njiru"},
{"id": 2, "name": "Jane Kagiri"},
{"id": 3, "name": "David Simiyu"}
]
return jsonify(users)
if name == 'main':
app.run(debug=True)
• @app.route('/users', methods=['GET']) defines an API route that responds to GET requests.
• jsonify(users) converts the users list into a JSON response, which is the standard format for API data exchange.
Step 5: Test the API
Now, if you run the app again and visit http://127.0.0.1:5000/users, you should see a list of users returned as JSON:
[
{"id": 1, "name": "John Njiru"},
{"id": 2, "name": "Jane Kagiri"},
{"id": 3, "name": "David Simiyu"}
]
See how easy that was? In just a few steps, you’ve installed Flask, set up a project, and created a working API that serves JSON data.
Final Thoughts
**You're Just Getting Started!**
That’s all it takes to get started with Flask. This is just the tip of the iceberg; you can build on this to create robust, scalable APIs that power your applications. Flask’s simplicity and flexibility are its greatest strengths, allowing you to scale from small apps to larger projects with ease.
**What’s next?** Well, you might want to look into more advanced topics like:
• Using Flask-RESTful for better API structure.
• Adding authentication and security.
• Auto-generating API documentation with Swagger.
But for now, congratulations! You’ve officially set up your first Flask API project, and you’re ready to build some amazing things.
**1.Building RESTful API Endpoints in Flask**
Creating RESTful API endpoints with Flask is one of the most satisfying experiences for developers. Whether you're building a simple app or scaling up to a more complex system, Flask offers a flexible and intuitive way to manage your API.
Let’s walk through some examples of HTTP methods and learn how to create clean, efficient API endpoints. By the end of this guide, you’ll be ready to build your own API with ease!
**2. Understanding HTTP Methods**
APIs primarily interact using the following HTTP methods:
**GET:** Retrieve data from the server.
**POST:** Submit new data to the server.
**PUT:** Update existing data.
**DELETE:** Remove data.
Flask makes these operations effortless with its route decorator, which you can use to define different actions for each method.
**3. Creating a Simple Flask API with HTTP Methods**
Here’s a quick example of how you can build a RESTful API using Flask:
from flask import Flask, jsonify, request
app = Flask(__name__)
**# Dummy data to simulate a simple database**
books = [
{'id': 1, 'title': 'Flask for Beginners', 'author': 'John Njiru'},
{'id': 2, 'title': 'Learning Python', 'author': 'Jane Kagiri'}
]
**# GET method: Retrieve all books**
@app.route('/books', methods=['GET'])
def get_books():
return jsonify(books)
**# GET method: Retrieve a single book by ID**
@app.route('/books/<int:book_id>', methods=['GET'])
def get_book(book_id):
book = next((book for book in books if book['id'] == book_id), None)
return jsonify(book) if book else jsonify({'message': 'Book not found'}), 404
**# POST method: Add a new book**
@app.route('/books', methods=['POST'])
def add_book():
new_book = request.json
books.append(new_book)
return jsonify(new_book), 201
**# PUT method: Update a book's information**
@app.route('/books/<int:book_id>', methods=['PUT'])
def update_book(book_id):
book = next((book for book in books if book['id'] == book_id), None)
if book:
book.update(request.json)
return jsonify(book)
return jsonify({'message': 'Book not found'}), 404
**# DELETE method: Remove a book by ID**
@app.route('/books/<int:book_id>', methods=['DELETE'])
def delete_book(book_id):
global books
books = [book for book in books if book['id'] != book_id]
return jsonify({'message': 'Book deleted'}), 204
if __name__ == '__main__':
app.run(debug=True)
**Let’s break this down:**
• GET /books: Fetch all available books.
• GET /books/<book_id>: Retrieve a specific book by its ID.
• POST /books: Add a new book to the collection.
• PUT /books/<book_id>: Update an existing book's details.
• DELETE /books/<book_id>: Delete a book by its ID.
**4. Handling JSON Data**
Flask provides a very developer-friendly approach to handling JSON data. In the example above, you can see how request.json is used to receive JSON input from the client, while jsonify() ensures the data sent back to the client is in JSON format.
**5. Why Flask is Perfect for RESTful APIs**
Flask's simplicity makes it ideal for developers of all levels. Whether you're just starting out or looking to quickly prototype, Flask’s flexibility lets you focus on writing clean, readable code. Its native support for JSON, combined with its ability to scale, makes it a solid choice for RESTful API development.
With Flask, you can quickly build out robust endpoints to serve your application needs.
Ready to start building your own?
**Using Flask-RESTful**
When it comes to developing APIs with Flask, there’s an extension that can take your API-building game to the next level: Flask-RESTful. It simplifies the whole process, making it faster and easier to structure, develop, and maintain your APIs—especially as they grow in complexity.
Let’s dive into what Flask-RESTful is all about and how you can use it to scale larger APIs efficiently!
**1. What is Flask-RESTful?**
Flask-RESTful is an extension for Flask that adds support for quickly building REST APIs. It provides tools that streamline the entire process—from routing to serialization. If you’re looking to avoid the repetitive work of manually setting up routes and responses for each endpoint, Flask-RESTful is here to help.
With this extension, you get a more organized way to manage your resources, define HTTP methods, and structure your API responses. The result? Cleaner code, faster development, and a much smoother experience overall.
**2. Why You Should Use Flask-RESTful**
Building APIs without structure can get chaotic fast, especially when your project scales. Flask-RESTful allows you to:
• Organize your API into manageable chunks (resources).
• Automatically handle serialization of your objects.
• Keep your code cleaner, avoiding redundant route setups and methods.
• Easily manage error handling and validation.
Essentially, it turns your project from “spaghetti code” into a well-oiled machine that’s easier to maintain and expand.
**3. Structuring and Scaling with Flask-RESTful**
Let’s start with a simple example of how Flask-RESTful can make API development more efficient. Imagine you’re building an API to manage a library of books.
Here’s how you could do it with Flask-RESTful:
First, install the extension:
*pip install Flask-RESTful*
from flask import Flask, request
from flask_restful import Resource, Api
app = Flask(__name__)
api = Api(app)
**# the data**
books = [
{'id': 1, 'title': 'Flask-RESTful Guide', 'author': 'John Njiru'},
{'id': 2, 'title': 'Advanced Flask APIs', 'author': 'Jane Kagiri'}
]
**# Resource to handle books**
class Book(Resource):
def get(self, book_id=None):
if book_id:
book = next((book for book in books if book['id'] == book_id), None)
return book if book else {'message': 'Book not found'}, 404
return books
def post(self):
new_book = request.json
books.append(new_book)
return new_book, 201
def put(self, book_id):
book = next((book for book in books if book['id'] == book_id), None)
if book:
book.update(request.json)
return book
return {'message': 'Book not found'}, 404
def delete(self, book_id):
global books
books = [book for book in books if book['id'] != book_id]
return {'message': 'Book deleted'}, 204
**# Adding the resource to the API**
api.add_resource(Book, '/books', '/books/<int:book_id>')
if __name__ == '__main__':
app.run(debug=True)
###Let’s break it down:
**• Resource Class:** Instead of defining routes for each HTTP method, we define a resource class (Book) and manage everything in one place. The get, post, put, and delete methods correspond to the HTTP methods.
**• Dynamic Routing:** Using Flask-RESTful, you can easily create dynamic routes. In this case, the route /books handles the list of books, while /books/<book_id> handles individual books based on their ID.
**• Error Handling:** Flask-RESTful makes it easy to return standardized HTTP response codes like 404 Not Found or 201 Created, helping you stay consistent with RESTful practices.
**4. Scaling Larger APIs with Flask-RESTful**
As your API grows, keeping things organized becomes crucial. Flask-RESTful excels here because it naturally encourages you to break your API into reusable, modular resources. This means that even if you have dozens of endpoints, your code remains clean and scalable.
**For larger projects, you can:**
• Organize resources by categories (e.g., users, books, orders) and place them in separate files.
• Implement authentication and permissions on a resource level.
• Handle complex relationships between resources (like linking books to authors or users to orders).
By using Flask-RESTful, the scalability of your API becomes easier to manage, even as it grows from a simple prototype to a production-level system.
**5. Why Choose Flask-RESTful?**
Flask-RESTful takes the tedious parts of building REST APIs and automates them for you, allowing you to focus on the functionality rather than boilerplate code. It's lightweight, flexible, and perfect for projects of any size.
As your API evolves, Flask-RESTful will help you manage its complexity without losing sight of maintainability.
In short, Flask-RESTful is a game-changer for anyone serious about developing clean, scalable APIs. It saves you time, energy, and lets you focus on building the features that matter! Ready to give it a try?
**USimplify Your API Development**
Hey there! Let's dive into how Flask-RESTful can make your API development a breeze. If you’re familiar with Flask, you know it's a great framework for building web apps, but when it comes to creating RESTful APIs, things can get a bit complex. That’s where Flask-RESTful comes in.
**What is Flask-RESTful?**
Flask-RESTful is an extension for Flask that simplifies the process of creating REST APIs. It provides tools and abstractions that make API development more intuitive, allowing you to focus on building your application rather than getting bogged down by boilerplate code.
**Structuring and Scaling with Flask-RESTful**
With Flask-RESTful, you can structure your API by creating resource classes. Each class represents a RESTful resource and maps to a URL endpoint. This modular approach helps keep your code organized and scalable.
**Here’s a quick example to illustrate:**
from flask import Flask
from flask_restful import Api, Resource
app = Flask(__name__)
api = Api(app)
class HelloWorld(Resource):
def get(self):
return {'hello': 'world'}
api.add_resource(HelloWorld, '/')
if __name__ == '__main__':
app.run(debug=True)
In this example, HelloWorld is a resource with a single GET endpoint. As your application grows, you can add more resources, manage them efficiently, and keep your API clean and maintainable.
**API Documentation with Swagger: The Why and How?**
**Why Document Your API?**
**Imagine this:** you’ve built a fantastic API, but your users have no idea how to interact with it. That’s where API documentation comes into play.
Proper documentation helps users understand how to use your API, what endpoints are available, and what responses to expect. It’s crucial for a seamless developer experience.
**Introducing Swagger for Flask**
Swagger is a powerful tool for auto-generating API documentation. With Flask-Swagger or Flask-APISpec, you can integrate Swagger UI into your Flask project to provide interactive, user-friendly documentation.
Here’s how you can get started:
**1.Install Flask-RESTful and Flask-Swagger:**
pip install flask-restful flask-swagger-ui
**2.Integrate Swagger UI:**
from flask import Flask
from flask_restful import Api
from flask_swagger_ui import get_swaggerui_blueprint
app = Flask(__name__)
api = Api(app)
SWAGGER_URL = '/swagger'
API_URL = '/static/swagger.json'
swagger_ui_blueprint = get_swaggerui_blueprint(
SWAGGER_URL,
API_URL,
config={'app_name': "My Flask API"}
)
app.register_blueprint(swagger_ui_blueprint, url_prefix=SWAGGER_URL)
if __name__ == '__main__':
app.run(debug=True)
from flask import Flask
from flask_restful import Api
from flask_swagger_ui import get_swaggerui_blueprint
app = Flask(__name__)
api = Api(app)
SWAGGER_URL = '/swagger'
API_URL = '/static/swagger.json'
swagger_ui_blueprint = get_swaggerui_blueprint(
SWAGGER_URL,
API_URL,
config={'app_name': "My Flask API"}
)
app.register_blueprint(swagger_ui_blueprint, url_prefix=SWAGGER_URL)
if __name__ == '__main__':
app.run(debug=True)
This will serve a Swagger UI at /swagger where users can interactively explore your API.
**Error Handling and Validation: Keeping Things Smooth**
**Handling Errors Gracefully**
No one likes errors, but they’re inevitable. Proper error handling ensures that your API communicates issues clearly, which is crucial for a good user experience. Use Flask’s error handling to catch exceptions and return meaningful messages:
from flask import Flask, jsonify
app = Flask(__name__)
@app.errorhandler(404)
def not_found_error(error):
return jsonify({'error': 'Not found'}), 404
if __name__ == '__main__':
app.run(debug=True)
**Input Validation**
Ensuring that incoming data is valid is vital. Libraries like marshmallow or Flask-Validator can help with this. Here’s a simple example using marshmallow:
from flask import Flask, request, jsonify
from marshmallow import Schema, fields, ValidationError
app = Flask(__name__)
class ItemSchema(Schema):
name = fields.Str(required=True)
price = fields.Float(required=True)
item_schema = ItemSchema()
@app.route('/items', methods=['POST'])
def create_item():
json_data = request.get_json()
try:
item = item_schema.load(json_data)
except ValidationError as err:
return jsonify(err.messages), 400
return jsonify(item), 201
if __name__ == '__main__':
app.run(debug=True)
**Securing Your API: Keeping It Safe**
**Security Practices**
Protecting your API is crucial. You can use Flask-JWT or OAuth for authentication. Token-based authentication ensures that only authorized users can access certain endpoints.
Here’s an example using Flask-JWT:
from flask import Flask, request, jsonify
from flask_jwt import JWT, jwt_required, current_identity
app = Flask(__name__)
app.config['SECRET_KEY'] = 'your_secret_key'
def authenticate(username, password):
# Authenticate user (dummy function here)
return User(username)
def identity(payload):
user_id = payload['identity']
return User(user_id)
jwt = JWT(app, authenticate, identity)
@app.route('/protected', methods=['GET'])
@jwt_required()
def protected():
return jsonify({'hello': 'world', 'user': current_identity.username})
if __name__ == '__main__':
app.run(debug=True)
**Testing APIs: Ensuring Quality**
**Testing with Postman and curl**
Testing is a crucial part of API development. Tools like Postman or curl allow you to send requests and check responses. Postman provides a user-friendly interface, while curl is great for command-line testing.
**Writing Unit Tests**
Use Flask’s built-in testing tools to write unit tests for your API endpoints:
import unittest
from app import app
class FlaskTestCase(unittest.TestCase):
def setUp(self):
self.app = app.test_client()
self.app.testing = True
def test_protected(self):
response = self.app.get('/protected')
self.assertEqual(response.status_code, 200)
if __name__ == '__main__':
unittest.main()
**Deploying Your Flask API: Going Live**
**Deployment Guide**
Ready to take your API live? Deploy it on platforms like Heroku or AWS.
Here’s a quick guide for deploying on Heroku:
**1. Create a Procfile:**
*web: gunicorn app:app*
**2. Deploy with Heroku CLI:**
*heroku create
git push heroku main*
**Deployment Pipeline**
A deployment pipeline helps you move from local development to production smoothly. Automate testing and deployment with CI/CD tools for a seamless process.
With these insights and tools, you’re well on your way to mastering Flask API development. Dive in, and happy coding!
Stay tuned!