Welcome to the ultimate guide on building APIs with Python! If you're excited to dive into creating APIs that are powerful and flexible, you're in the right place. We'll walk you through everything you need to know to get started, from setting up your environment to deploying your API. Along the way, we'll introduce you to some exciting tools that can make your life easier, including a fantastic alternative to Postman—APIDog. Let’s jump in!
1. Understanding APIs and Why They Matter
What is an API?
An API (Application Programming Interface) allows different software systems to communicate with each other. Think of it as a bridge that enables one application to request and use the services or data of another application without needing to know its internal workings.
Why Build an API?
APIs are crucial for modern software development because they:
• Enable Integration: APIs allow different systems to work together.
• Promote Reusability: One API can be used by multiple clients.
• Improve Scalability: APIs help in scaling applications by separating concerns between different components.
2. Setting Up Your Development Environment
Before we start coding, let’s set up your development environment to ensure everything runs smoothly.
1. Install Python
First things first—make sure Python is installed on your system. You can download it from python.org.
2. Create a Virtual Environment
Virtual environments are essential for managing dependencies. Here’s how to create one:
source myenv/bin/activate # On Windows use: myenv\Scripts\activate
3. Install Flask
Flask is a lightweight framework perfect for building APIs:
pip install Flask
4. Install Flask-RESTful (Optional but recommended)
For more advanced API functionalities:
pip install Flask-RESTful
3. Building Your First API with Flask
Let’s build a basic API with Flask to get started.
1. Create Your Flask App (app.py)
from flask import Flask, jsonify, request
app = Flask(__name__)
@app.route('/api', methods=['GET'])
def get_data():
data = {"message": "Hello, World!"}
return jsonify(data)
@app.route('/api/data', methods=['POST'])
def post_data():
content = request.json
return jsonify({"you_sent": content}), 201
if __name__ == '__main__':
app.run(debug=True)
2. Run Your Flask Application
python app.py
3. Test Your Endpoints
Use Postman or cURL to test your API endpoints.
GET Request:
python app.py
3. Test Your Endpoints
Use Postman or cURL to test your API endpoints.
GET Request:
curl http://127.0.0.1:5000/api
POST Request:
curl -X POST http://127.0.0.1:5000/api/data -H "Content-Type: application/json" -d '{"key": "value"}'
4. Enhancing Your API with Flask-RESTful
1. Add Flask-RESTful to Your Project
from flask_restful import Api, Resource
api = Api(app)
class HelloWorld(Resource):
def get(self):
return {'hello': 'world'}
api.add_resource(HelloWorld, '/hello')
2. Create More Resources
class User(Resource):
def get(self, user_id):
return {'user_id': user_id}
api.add_resource(User, '/user/<int:user_id>')
5. Securing Your API
1. Install Flask-HTTPAuth
pip install Flask-HTTPAuth
2. Add Basic Authentication
from flask_httpauth import HTTPBasicAuth
auth = HTTPBasicAuth()
@auth.verify_password
def verify_password(username, password):
return username == 'admin' and password == 'password'
@app.route('/secure')
@auth.login_required
def secure_data():
return jsonify({'message': 'This is a secure endpoint'})
6. Documenting Your API
Good documentation is crucial. Let’s use Swagger to make your API documentation easy to access.
1. Install Flask-Swagger-UI
pip install flask-swagger-ui
2. Set Up Swagger UI
from flask_swagger_ui import get_swaggerui_blueprint
SWAGGER_URL = '/swagger'
API_URL = '/static/swagger.json'
swagger_ui = get_swaggerui_blueprint(SWAGGER_URL, API_URL, config={'app_name': "My API"})
app.register_blueprint(swagger_ui, url_prefix=SWAGGER_URL)
3. Create a Swagger JSON File (swagger.json)
{
"swagger": "2.0",
"info": {
"title": "My API",
"version": "1.0.0"
},
"paths": {
"/api": {
"get": {
"summary": "Returns a hello message",
"responses": {
"200": {
"description": "A hello message"
}
}
}
}
}
}
7. Testing Your API
1. Write Unit Tests
Testing ensures that your API behaves as expected. Here’s how to test using unittest:
import unittest
from app import app
class TestAPI(unittest.TestCase):
def setUp(self):
self.app = app.test_client()
self.app.testing = True
def test_get_data(self):
response = self.app.get('/api')
self.assertEqual(response.status_code, 200)
self.assertIn(b'Hello, World!', response.data)
def test_post_data(self):
response = self.app.post('/api/data', json={'key': 'value'})
self.assertEqual(response.status_code, 201)
self.assertIn(b'{"you_sent": {"key": "value"}}', response.data)
if __name__ == '__main__':
unittest.main()
8. Deploying Your API
To make your API available online, consider using Heroku for deployment:
1. Create a requirements.txt File
pip freeze > requirements.txt
2. Create a Procfile
web: python app.py
3. Deploy to Heroku
heroku create
git push heroku main
heroku open
9. Using APIDog for testing APIs
Now that you’ve put in the effort to build your API, it’s time to ensure it performs just as you’ve designed it to. This is where APIDog shines as the ultimate alternative to Postman. While Postman has been a go-to tool for many developers, you might have noticed some recent limitations on collection runs that can be quite restrictive.
That’s where APIDog steps in to make a real difference. Imagine having the freedom to test your APIs thoroughly without any of those pesky limits. With APIDog's unlimited collection runner, you can dive deep into your testing process, explore every edge case, and ensure your API is robust and reliable. Let’s explore how APIDog can transform your API testing experience and help you achieve the seamless performance you’re aiming for.
Key Features of APIDog:
• Unlimited Collection Runner: Test as many collections as you want without restrictions.
• User-Friendly Interface: Easy to navigate and set up, even for beginners.
• Robust Reporting: Detailed reports on your test runs to help you track performance and issues.
• Integration with CI/CD Pipelines: Seamlessly integrate APIDog into your continuous integration and deployment workflows.
How APIDog Enhances Your Workflow:
• Efficient Testing: No more hitting limits on your tests.
• Streamlined Development: Focus on building great APIs without worrying about tool constraints.
• Enhanced Collaboration: Share your API test results effortlessly with your team.
Try Apidog now!
Start with API tests on Apidog for Free and see the difference immediately
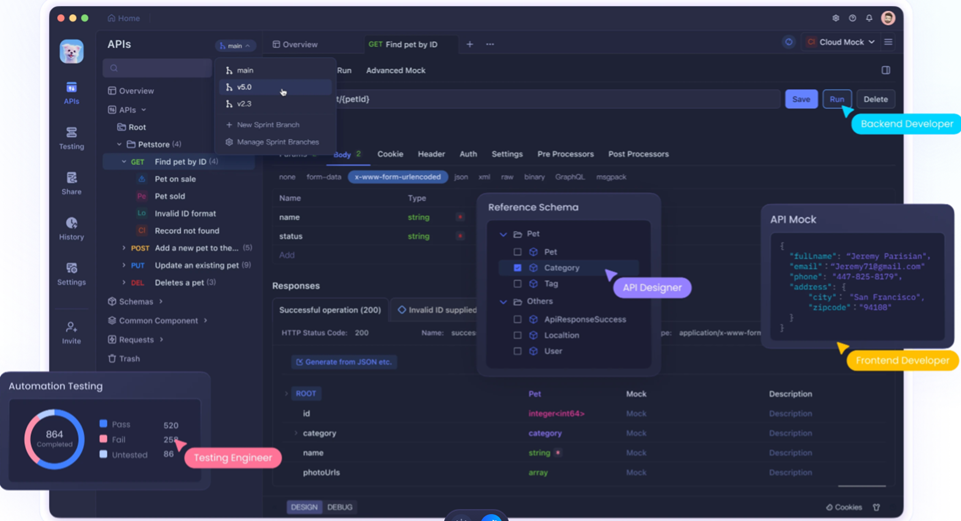
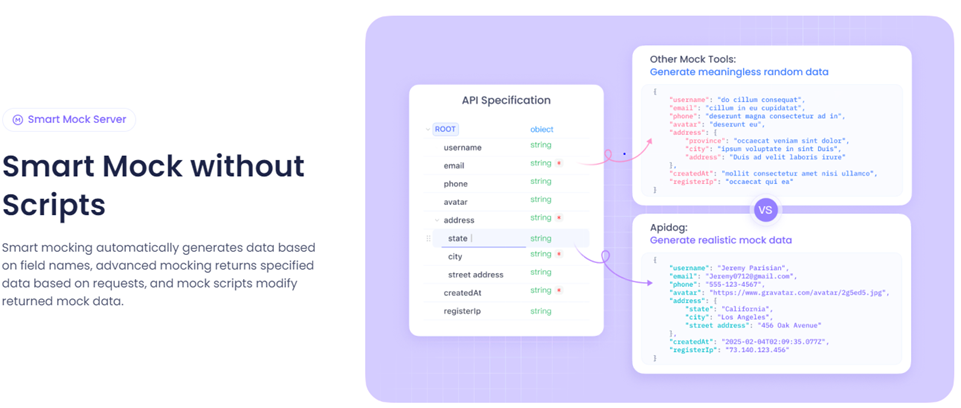
Your API Testing Process with APIDog
To illustrate how APIDog fits into your workflow, consider a diagram showing the integration of APIDog for testing your API endpoints.
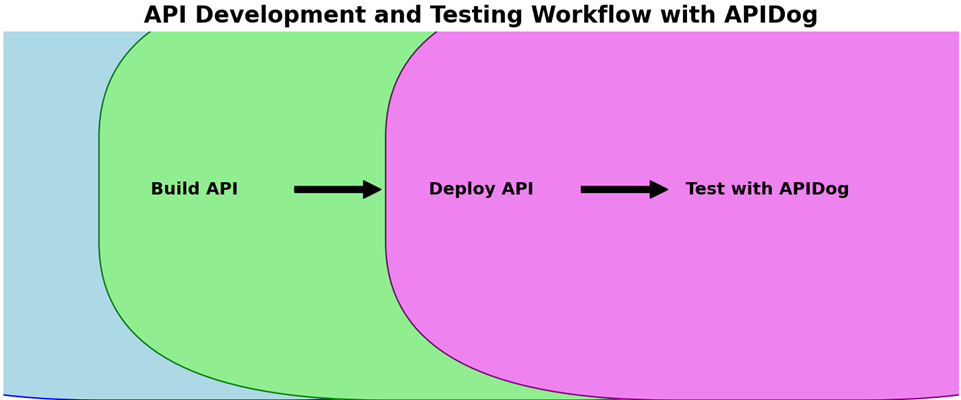
A call to action
In conclusion, mastering API development in Python is not just a technical skill—it's a gateway to creating sophisticated, high-performance applications that stand out in today’s competitive landscape. As you fine-tune your APIs, consider integrating APIDog into your workflow. Its unlimited collection runner and advanced testing capabilities can transform your approach, ensuring your APIs are both robust and reliable.
Why does this matter? Because delivering high-quality, well-tested APIs is crucial for the success of your applications and the satisfaction of your users. Don’t let limitations hold you back—embrace the power of APIDog to unlock new levels of efficiency and accuracy in your testing process.
Ready to elevate your API development game? Dive into the features of APIDog today and see firsthand how it can enhance your testing strategy. Start integrating these tools into your projects and experience the difference.
Your users—and your future self—will thank you for it.
Enjoy!