In today’s fast-paced development world, automated testing has become indispensable. But while Selenium is often hailed as the go-to tool for UI testing, did you know it can also play a critical role in API automation testing? Let’s take a look at how Selenium can be a key player in building robust, automated API tests and why you should consider it for your testing strategy.
Why Automate API Testing?
Before we dive into how to automate APIs using Selenium, let’s talk about the “why.” APIs serve as the backbone for many modern applications, connecting different systems and services. Ensuring their functionality, reliability, and performance is vital. Manual API testing can be time-consuming and prone to human error. That’s where automation comes in — it offers speed, consistency, and efficiency.
Testing Workflow Diagram for API automation testing using Selenium
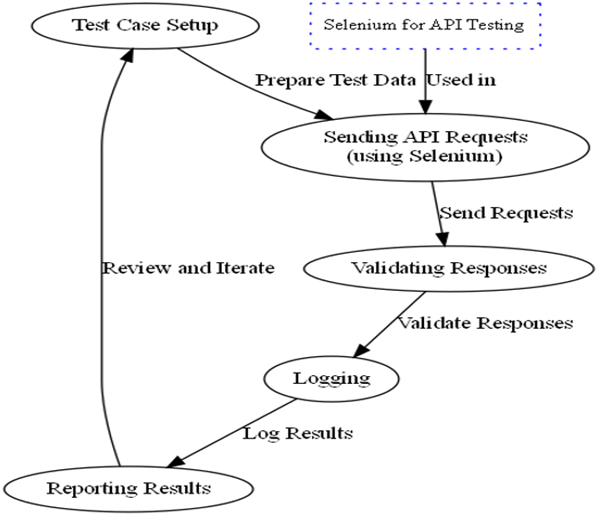
Let’s walk through the steps of a typical testing workflow:
1. Test Case Setup: Think of this as getting your tools and data ready before starting. You’ll need to prepare the environment and any data you'll use during the tests.
2. Sending API Requests: Now it’s time to put your tools to work! You’ll execute API requests, often using automation tools like Selenium, to interact with your API.
3. Validating Responses: Here’s where you check if everything’s working as expected. You’ll compare the responses you get from the API against the outcomes you were hoping for.
**4. Logging: **This is like keeping a diary of your test journey. Record what happens during testing, including any successes and hiccups along the way.
5. Reporting Results: Finally, you’ll wrap things up by generating reports based on your test results. These reports help you understand what went well and what needs fixing.
When you automate API testing, you’re in for some great benefits:
• Increase Testing Speed: Automation speeds up the process, so you spend less time running the same tests over and over.
• Enhance Test Coverage: It’s easier to test a variety of scenarios and environments with automation, making sure you cover more ground.
• Ensure Consistency: Automated tests run the same way every time, which means your testing approach is consistent and reliable.
• Catch Issues Early: By integrating automated tests into your CI/CD pipeline, you can catch issues before they make it to production, saving time and effort in the long run.
In short, automated API testing not only boosts efficiency but also helps you maintain a higher quality of your software!
Why Use Selenium for API Testing?
Selenium is typically known for browser automation, but with a bit of ingenuity, it can be extended for API testing. Why choose Selenium over traditional API testing tools? One significant advantage is the integration of both API and UI tests in the same framework. This approach helps developers and testers avoid switching between different testing tools, streamlining the process.
Here’s how Selenium can be leveraged in API automation testing:
Flexibility: You can use Selenium WebDriver to create HTTP requests and validate responses.
Integration with Testing Frameworks: You can integrate your API tests with popular testing frameworks like JUnit or TestNG, making it easier to manage and run tests.
Parallel Testing: Selenium’s parallel execution capabilities can also be applied to API tests, allowing you to test different API endpoints simultaneously.
How to Set Up API Automation Testing with Selenium
Let’s look at the basic steps to automate API testing using Selenium:
1.Set up Selenium WebDriver: Start by setting up the Selenium WebDriver environment. You can use programming languages like Java, Python, or C#.
2. Send HTTP Requests: Instead of using Selenium WebDriver’s usual browser control features, you can use its capabilities to send GET, POST, PUT, or DELETE HTTP requests to your API endpoints.
3. Verify Responses: After sending the request, you’ll capture the API response (in JSON or XML format) and validate it against expected results using assertions.
4. Integrate with Other Tools: Selenium’s versatility allows you to integrate with tools like Rest-Assured (for Java) or Python’s requests library to enhance API test functionalities.
How to Code for API Testing with Selenium (Python)
import requests
import unittest
class APITest(unittest.TestCase):
def test_get_request(self):
# Replace with actual endpoint from APIDog.com
response = requests.get("https://apidog.com/api/v1/data")
self.assertEqual(response.status_code, 200)
# Assuming 'expected_data' is part of the response JSON
self.assertIn("expected_data", response.json())
def test_post_request(self):
# Replace with actual endpoint from APIDog.com
data = {"key": "value"}
response = requests.post("https://apidog.com/api/v1/post", json=data)
self.assertEqual(response.status_code, 201)
# Assuming 'success' is part of the response JSON
self.assertIn("success", response.json())
if name == "main":
unittest.main()
Explanation:
APIDog.com Integration: Now, instead of using generic API endpoints, this code interacts with real endpoints from APIDog.com. You can easily replace the provided endpoints with your actual setup on APIDog, making it a breeze to switch.
GET Request: The code sends a simple GET request to the API. It checks for a status code of 200, meaning success, and ensures the JSON response includes an expected key, like expected_data.
POST Request: This part sends a POST request with some JSON data to the API. If successful, it checks for a 201 status code and validates that the key success appears in the response.
These checks make sure your API is doing what it should—quick, effective, and easy to manage with APIDog!
The process involved in an API call
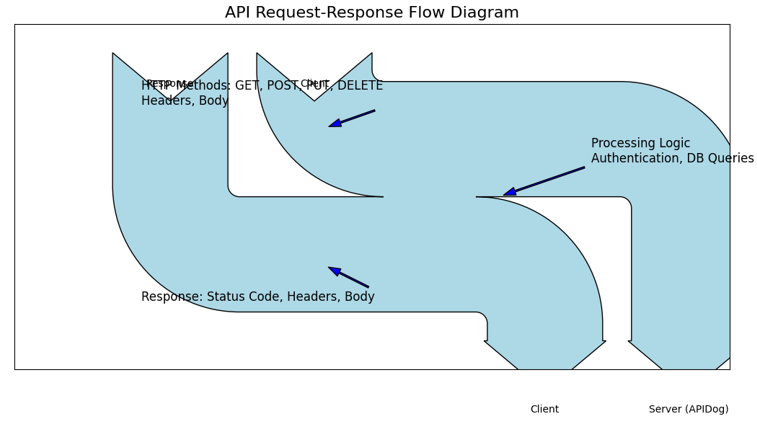
Let’s break down what this diagram is all about:
1. Sankey Diagram: Imagine this as a detailed map of how your data moves. It shows the journey of an API request from the client to the server and then back to the client.
You’ll see labels like “Client,” “Server (APIDog),” “Response,” and “Back to Client” highlighting each part of this journey.
2. Arrows and Annotations: Think of these as helpful notes along the way. They’ll point out:
• The different HTTP methods you’re using, like GET, POST, PUT, and DELETE.
• What’s happening behind the scenes on the server side, including the headers and body of the request.
• How the server processes this request and what it sends back, including the status code, headers, and the response body.
In a nutshell, this diagram will give you a clear, visual representation of how an API request flows from start to finish. It’s like a step-by-step guide that makes understanding the API call process a breeze!
Discover APIDog, the Ultimate Postman Alternative!
Hey there, API enthusiasts! 🚀
If you’ve been on the hunt for a Postman alternative that offers a game-changing feature, then let me introduce you to APIDog—your new best friend in API testing.
Why APIDog?
You might have heard that Insomnia recently unveiled an exciting update allowing for Unlimited Collection Runners. It’s a fantastic feature, especially for those who’ve felt constrained by Postman’s limits. But let’s dive into why APIDog could be the superior choice for your API testing needs.
Unlimited Collection Runners:
With Postman, the constraints on collection runners can sometimes put a damper on your testing workflow. But guess what? APIDog takes it to the next level by offering unlimited collection runners. This means you can run as many collections as you need without hitting any limits—perfect for scaling your testing operations effortlessly.
Why Choose APIDog?
• Seamless Transition: Switching from Postman to APIDog is a breeze. With its intuitive design, you can migrate your existing collections with just a few clicks, leaving you more time to focus on what really matters—your API tests.
• Elegant and User-Friendly: APIDog boasts a sleek, user-friendly GUI that makes navigating through your API tests a pleasure. Plus, it comes with a Dark Mode for those late-night coding sessions.
• Cost-Effective: Why pay more when you can get all the powerful features of Postman’s paid version at a fraction of the cost? APIDog delivers premium functionality without breaking the bank.
• Collaborative Features: Whether you’re working solo or in a team, APIDog’s collaborative tools ensure everyone stays on the same page, making teamwork a breeze.
So if you’re tired of running into limitations and want to elevate your API testing game, give APIDog a try. It’s not just an alternative—it’s a smarter, more efficient way to handle your API testing needs. 🐶✨
Ready to make the switch? Explore APIDog today!
Integration Selenium with Testing Frameworks
Integrating Selenium with popular testing frameworks like unittest, pytest, or TestNG is essential for automating the testing process efficiently and maintaining a structured, scalable test suite. Let’s break down how to achieve this and why it’s beneficial.
Why Integrate Selenium with Testing Frameworks?
Testing frameworks provide a robust foundation for writing and managing test cases, assertions, and test suites. They help in:
• Organizing Tests: With frameworks like pytest or unittest, you can group tests logically into test suites.
• Reusability: You can create setup and teardown methods that initialize and close the browser, making your code DRY (Don’t Repeat Yourself).
• Assertions: Testing frameworks allow you to assert that the results of your test match the expected outcomes, which is crucial for automated validation.
• Parallel Execution: Some frameworks (like pytest) support parallel test execution, significantly speeding up the testing process.
The diagram (figure 3) depicts the integration of Selenium with Python (or other languages), and its interaction with RESTful APIs, including components like test scripts, API endpoints, request methods, assertions and test reports
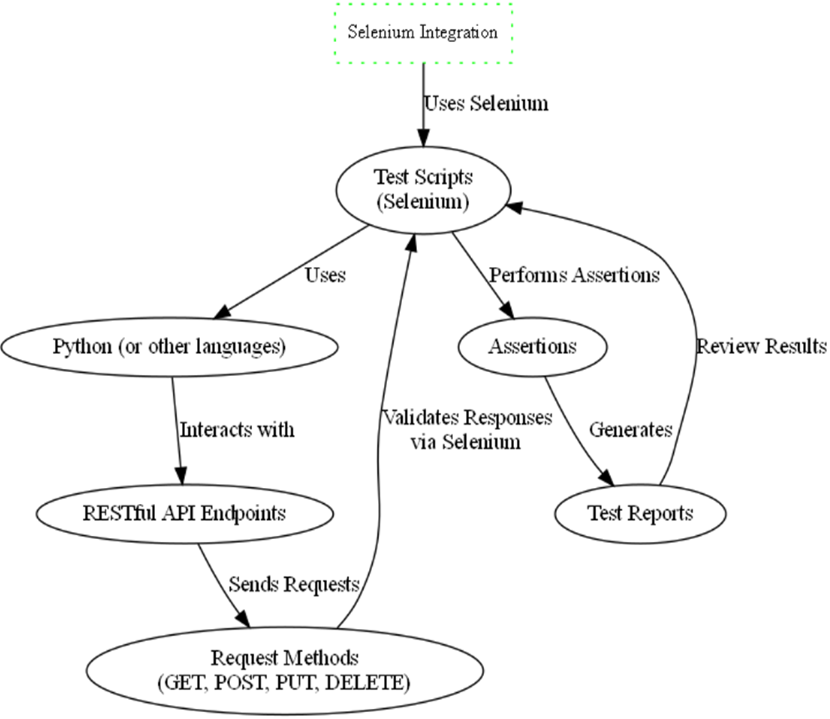
Let’s dive into the diagram, which beautifully illustrates how everything comes together in API testing with Selenium. Here’s a friendly breakdown of each part:
• Test Scripts (Selenium): Think of this as your playbook. These are the scripts you write using Selenium to test your API. They’re the instructions for what you want to test.
• Python (or other languages): This is the language you use to write your test scripts. Whether it’s Python or another language, it’s the tool you use to bring your tests to life.
• RESTful API Endpoints: These are like the different doors you knock on in your API. Each endpoint represents a different part of the API you interact with.
• Request Methods (GET, POST, PUT, DELETE): Imagine these as different ways to knock on those doors. GET, POST, PUT, and DELETE are the methods you use to communicate with the API.
• Assertions: This is where you check if the API is responding as expected. It’s like checking if your letter was answered correctly—did you get what you were looking for?
• Test Reports: After running your tests, you get a report. This is your
summary of how everything went, including what worked and what didn’t.
So, the diagram shows how all these components interact: Selenium writes the test scripts in Python, which then communicates with the API endpoints using different request methods.
The results are checked through assertions, and finally, the outcomes are compiled into test reports. It’s a well-orchestrated dance of technology that helps ensure your API is running smoothly.
How to Integrating Selenium with unittest
unittest is a built-in Python module for organizing tests and asserting expected results. Here’s how you can integrate Selenium with unittest to automate browser tests.
Steps:
1. Install Selenium: Ensure you have Selenium installed:
pip install selenium
2. Write the Test Case: Below is a simple test case using unittest where we open a webpage, interact with elements, and assert that the title matches the expected value.
import unittest
from selenium import webdriver
class TestGoogleSearch(unittest.TestCase):
@classmethod
def setUpClass(cls):
# Set up the WebDriver (e.g., using ChromeDriver)
cls.driver = webdriver.Chrome(executable_path='path/to/chromedriver')
cls.driver.implicitly_wait(10)
def test_search_in_google(self):
# Open the Google homepage
self.driver.get('https://www.google.com')
# Find the search box and enter a search term
search_box = self.driver.find_element_by_name('q')
search_box.send_keys('Selenium WebDriver')
search_box.submit()
# Assert that the title contains 'Selenium WebDriver'
self.assertIn('Selenium WebDriver', self.driver.title)
@classmethod
def tearDownClass(cls):
# Close the browser
cls.driver.quit()
if name == "main":
unittest.main()
Key Points:
• setUpClass: This method initializes the Selenium WebDriver before running any tests.
• tearDownClass: This closes the browser after all tests are completed.
• assertIn: We use an assertion to check that the page title contains the expected text.
Integrating Selenium with pytest
pytest is a flexible and powerful testing framework. Here's how to integrate Selenium with pytest:
1. Install pytest:
pip install pytest
2. Write a Test Using pytest:
import pytest
from selenium import webdriver
@pytest.fixture(scope="class")
def setup(request):
# Set up the WebDriver (e.g., using ChromeDriver)
driver = webdriver.Chrome(executable_path='path/to/chromedriver')
driver.implicitly_wait(10)
request.cls.driver = driver
yield
driver.quit()
@pytest.mark.usefixtures("setup")
class TestGoogleSearch:
def test_google_search(self):
self.driver.get('https://www.google.com')
search_box = self.driver.find_element_by_name('q')
search_box.send_keys('Selenium WebDriver')
search_box.submit()
assert 'Selenium WebDriver' in self.driver.title
Key Points:
• Fixtures: pytest uses fixtures to manage setup and teardown. In this case, we initialize the WebDriver before running tests and close it afterward.
• Test Assertions: We use Python’s assert statement to validate the test result.
Managing Test Suites
Testing frameworks allow you to organize your tests into suites for better scalability. In unittest, for example, you can create a suite of tests like this:
def suite():
suite = unittest.TestSuite()
suite.addTest(TestGoogleSearch('test_search_in_google'))
return suite
if name == "main":
runner = unittest.TextTestRunner()
runner.run(suite())
For pytest, test discovery is automatic, and you can run multiple tests by simply using:
Pytest
THerefore,integrating Selenium with testing frameworks like unittest and pytest enables you to scale your automated tests, structure them better, and get more reliable results through assertions and test suites.
This integration is key for any test automation project, as it allows you to reuse code, parallelize test runs, and get comprehensive reports on test coverage.
Handling Authentication in API Automation Tests
When automating API tests, handling authentication is critical, as many APIs require you to authenticate using various methods like Basic Authentication, OAuth, or JWT (JSON Web Tokens).
Here's how you can handle different types of authentication and integrate them into your tests effectively.
Basic Authentication
Basic authentication involves sending a username and password encoded in base64 within the request headers. Many APIs still support this simple form of authentication.
TheCode:
import requests
from requests.auth import HTTPBasicAuth
# URL of the API endpoint
url = 'https://apidog.com/api/resource'
# Sending a request with basic authentication
response = requests.get(url, auth=HTTPBasicAuth('username', 'password'))
# Print the response
print(response.status_code)
print(response.json())
In this example, the credentials are passed using HTTPBasicAuth. The server checks the credentials and responds accordingly.
OAuth Authentication
OAuth (Open Authorization) is a token-based authentication standard, commonly used when APIs need to access resources on behalf of a user. It involves retrieving an access token through an authorization server and using that token in the API requests.
The Code :
import requests
# First, obtain the OAuth token
auth_url = 'https://apidog.com/oauth/token'
client_id = 'your_client_id'
client_secret = 'your_client_secret'
data = {
'grant_type': 'client_credentials',
'client_id': client_id,
'client_secret': client_secret
}
# Get the access token
response = requests.post(auth_url, data=data)
token = response.json()['access_token']
# Use the token in your API request headers
api_url = 'https://apidog.com/api/resource'
headers = {
'Authorization': f'Bearer {token}'
}
response = requests.get(api_url, headers=headers)
# Print the response
print(response.status_code)
print(response.json())
Here, you first authenticate to get an OAuth token, which you then include in the Authorization header for subsequent API requests.
JWT (JSON Web Token) Authentication
JWT is a common token-based authentication method that provides a signed token representing the user’s identity. The token is passed in the Authorization header as a Bearer token.
The Code:
import requests
# Example of API request with JWT authentication
token = 'your_jwt_token_here'
# Setting the headers with the JWT token
headers = {
'Authorization': f'Bearer {token}',
'Content-Type': 'application/json'
}
# Send the request with authentication
api_url = 'https://apidog.com/api/protected-resource'
response = requests.get(api_url, headers=headers)
# Print the response
print(response.status_code)
print(response.json())
In this example, the JWT token is used in the Authorization header. The server verifies the token and allows access if the token is valid.
Test Data Parameterization
When automating API tests, it's essential to test the API with different sets of data to ensure it works under various conditions. Data-driven testing allows you to pass multiple inputs to a single test case using external data sources like CSV, JSON, or databases. This is particularly useful for validating API behavior with varying inputs.
Using CSV Files for Data-Driven Testing
You can store different test data in a CSV file and use it in your API requests by reading it dynamically.
The Code:
import csv
import requests
# Read test data from a CSV file
with open('test_data.csv', mode='r') as file:
reader = csv.DictReader(file)
for row in reader:
api_url = 'https://apidog.com/api/resource'
headers = {
'Authorization': f'Bearer {row["token"]}',
'Content-Type': 'application/json'
}
response = requests.post(api_url, headers=headers, json={'data': row["data"]})
# Print the response for each test case
print(f"Status: {response.status_code}, Response: {response.json()}")
CSV File (test_data.csv):
token,data
jwt_token_1,{"key": "value1"}
jwt_token_2,{"key": "value2"}
jwt_token_3,{"key": "value3"}
In this example, the test is iterating over the rows in the CSV file, passing each token and data set to the API. This is a scalable way to test multiple inputs without hardcoding them into your test scripts.
Using JSON Files for Data-Driven Testing
You can also use a JSON file to store your test data, especially when dealing with complex data structures.
The Code:
import json
import requests
# Load test data from a JSON file
with open('test_data.json', 'r') as file:
test_data = json.load(file)
# Iterate over test cases and send requests
for test_case in test_data['test_cases']:
api_url = 'https://apidog.com/api/resource'
headers = {
'Authorization': f'Bearer {test_case["token"]}',
'Content-Type': 'application/json'
}
response = requests.post(api_url, headers=headers, json=test_case["data"])
# Print the response for each test case
print(f"Status: {response.status_code}, Response: {response.json()}")
JSON File (test_data.json):
{
"test_cases": [
{
"token": "jwt_token_1",
"data": {"key": "value1"}
},
{
"token": "jwt_token_2",
"data": {"key": "value2"}
},
{
"token": "jwt_token_3",
"data": {"key": "value3"}
}
]
}
This approach allows for greater flexibility in defining test cases and handling more complex data types.
We therefore note that handling authentication and test data parameterization are essential for comprehensive API testing.
Whether you're using Basic Auth, OAuth, or JWT for authentication, you need to ensure your test scripts handle tokens correctly.
By incorporating data-driven testing with CSV, JSON, or databases, you can significantly improve the coverage and reliability of your API tests. These techniques will help you create robust, scalable tests that can handle multiple scenarios with ease.
Validating APIs and Why Assertions Matter
When testing APIs, you need solid assertions to ensure the responses you're getting are exactly what you expect. Imagine you’re sending a request to a server, and you need to check if the API is delivering the right data or returning the expected status code. Assertions are the way to do that! They allow you to compare the actual response with the expected outcome, instantly alerting you if something’s off.
What Should You Be Validating?
• Status Codes: Every API request responds with a status code. It tells you what happened—whether the request was successful, created something, or if something went wrong. For instance, a 200 OK means the request was a success, while a 500 Internal Server Error indicates that the server couldn't process it.
import requests
response = requests.get('https://apidog.com/api/resource')
assert response.status_code == 200, f"Expected 200, but got {response.status_code}"
• JSON Body: API responses often contain a JSON object. Verifying that this body is structured correctly and contains the right data is crucial. For example, if you're testing a login feature, you need to ensure that the API returns a user token in the JSON body.
data = response.json()
assert 'token' in data, "Key 'token' not found in response"
• Response Time: Let’s not forget about performance! A great API is a fast API. Use assertions to confirm that your APIs are responding in a timely manner.
assert response.elapsed.total_seconds() < 2, "Response took too long"
These simple checks go a long way in ensuring the reliability and speed of your APIs.
Continuous Integration (CI)
One of the most impactful things you can do is automate your tests as part of a CI/CD pipeline. Tools like Jenkins or GitLab CI let you automatically run your tests after each code change. This means you’ll get instant feedback if something breaks, preventing bugs from slipping through the cracks.
Setting Up Jenkins for API Testing
With Jenkins, your tests can run automatically, and you can even generate detailed reports that provide insights into test performance.
Here’s a simple Jenkinsfile example to get you started:
pipeline {
agent any
stages {
stage('Test') {
steps {
sh 'pytest --html=report.html'
}
}
stage('Report') {
steps {
archiveArtifacts artifacts: 'report.html', fingerprint: true
}
}
}
}
Imagine never having to manually run API tests again! Every time there’s a code push, Jenkins will test the APIs for you. You’ll also have the option to create beautiful test reports using tools like Allure, which gives you a clear picture of what's working and what's not.
Handling Authentication Like a Pro
If you’re testing secure APIs, handling authentication is critical. Many APIs require authentication tokens, such as JWT or OAuth, to allow access. You’ll need to ensure that your automated tests can correctly send these tokens in the headers of the API requests.
Here’s how you can handle authentication tokens in your requests:
headers = {
'Authorization': 'Bearer your_jwt_token_here'
}
response = requests.get('https://apidog.com/api/secure-endpoint', headers=headers)
Integrating token-based authentication ensures that your tests mirror real-world scenarios, especially for APIs behind a login.
Test Data Parameterization: Make Your Tests More Dynamic
Running tests with hardcoded data is a big no-no. You need data-driven testing, where you can test multiple scenarios by feeding different sets of data into the same test. This approach significantly reduces the time spent writing redundant test cases.
Whether you’re using CSV, JSON, or even a database, parameterizing your tests ensures you cover more ground with less effort. For example:
import csv
with open('test_data.csv', newline='') as csvfile:
reader = csv.DictReader(csvfile)
for row in reader:
response = requests.post('https://apidog.com/api/create', json=row)
assert response.status_code == 201
Now, you're not just testing one case—you’re testing dozens with a single script!
Integrating API Testing into the CI/CD Pipeline
Imagine being able to deploy code with complete confidence, knowing that your API tests will automatically catch any issues. By integrating API tests into your CI/CD pipeline, you can create a seamless testing environment that ensures quality at every stage.
Tools like Jenkins or GitLab CI are perfect for automating the execution of your tests. Once the tests run, you can configure them to generate test reports that visualize performance, success rates, and areas for improvement.
Error Handling & Debugging: Stay Ahead of the Curve
Automated testing isn’t foolproof—errors can happen. Your job is to catch these errors before they catch you. From timeouts to unexpected status codes or malformed JSON responses, there are many potential pitfalls in API testing.
A flowchart illustrating error handling and reporting in automation testing with Selenium (figure 4)
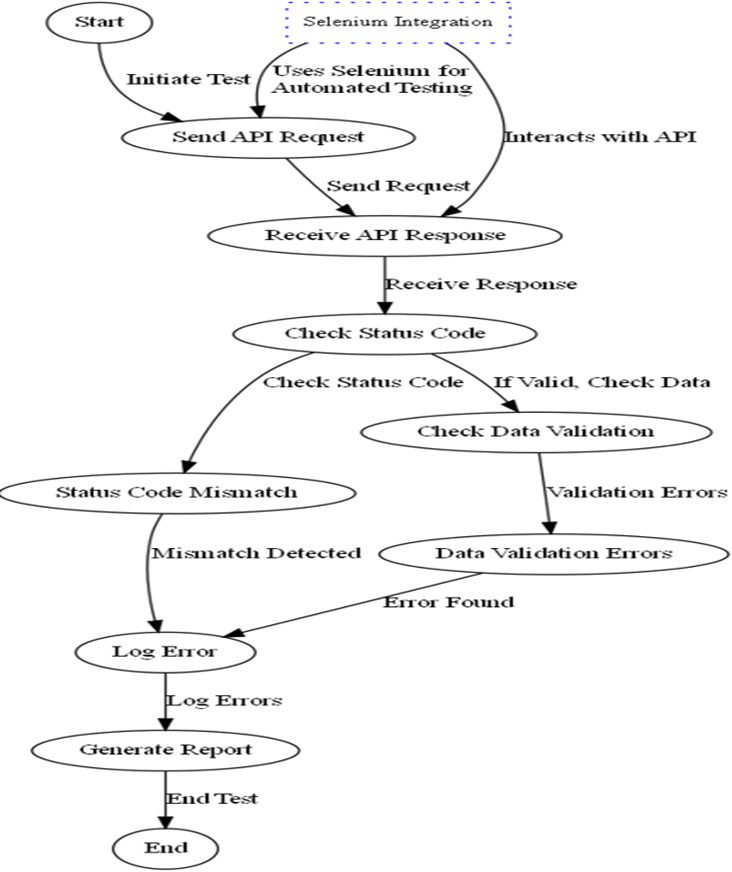
Let's break down the nodes in our error handling and reporting flowchart. Here’s a friendly guide to each step:
• Start: This is where your testing journey begins. You’re all set to dive into the process!
• Send API Request: Imagine this as sending a letter. You’re putting your request out there to the API.
• Receive API Response: The API gets back to you. It’s like getting a reply to your letter.
• Check Status Code: Here, you’re checking the return address on the letter to ensure everything is as expected.
• Status Code Mismatch: If the return address is incorrect or doesn’t match what you anticipated, that’s an error. It means something went wrong.
• Check Data Validation: Now you’re scrutinizing the content of the letter (response data) to ensure it’s valid and meets your expectations.
• Data Validation Errors: If the content of the letter is off or doesn’t make sense, that’s a data validation error.
• Log Error: This step involves jotting down the error details. Think of it as noting down the issues so you can refer to them later.
• Generate Report: Based on the errors you’ve logged, you create a report. This report helps you summarize what went wrong and why.
• End: You’ve reached the end of the testing process. Time to review and act on the insights from your report!
Tips for Smoother Error Handling:
• Log Everything: Make sure to keep a record of all requests, responses, and errors. This way, if something goes wrong, you can track down what happened easily.
• Retry Logic: Sometimes, tests fail because of temporary issues like network hiccups. Implementing a retry logic can help your tests handle these minor glitches without failing.
for attempt in range(3):
try:
response = requests.get('https://apidog.com/api/resource')
if response.status_code == 200:
break
except requests.exceptions.RequestException:
print("Request failed, retrying...")
Proper error handling allows you to create reliable, consistent API tests that won’t break the moment something goes wrong.
We can therefore take note that API automation testing using Selenium is a game-changer for ensuring the quality and performance of your APIs. By mastering assertions, integrating with CI/CD pipelines, handling authentication, and using data-driven testing, you’ll be well on your way to creating a seamless testing environment.
Remember, this isn’t just about ensuring that your API works—it’s about building trust with your stakeholders and users by delivering a reliable, high-quality product. Integrating automation testing into your development workflow ensures that your APIs continue to function flawlessly, even as your codebase evolves.
By leveraging tools like Jenkins, Allure, and data-driven testing, you’re setting yourself up for success and saving countless hours of manual work. So, get started on your API automation journey today—and ensure that your APIs are always one step ahead of potential issues.
A comparison diagram between manual API testing and automated API testing with Selenium (figure 5)
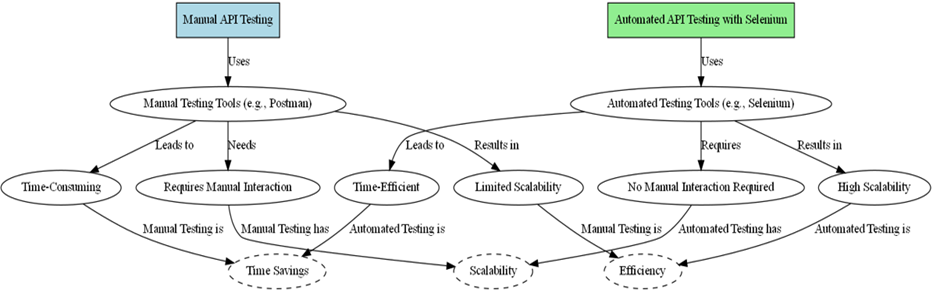
Figure 5 provides a clear comparison between manual and automated API testing. Here’s a breakdown of what each part represents:
Manual API Testing:
• Manual Testing Tools (e.g., Postman): Think of these as the go-to tools you use when you’re testing APIs by hand. Postman is a classic example.
• Time-Consuming: This node highlights that manual testing can take quite a bit of time—each test requires your direct attention.
• Limited Scalability: It’s a bit of a bottleneck. Manual testing doesn’t handle growth well, meaning as your API grows, testing becomes increasingly cumbersome.
• Requires Manual Interaction: This just means that every step of the testing process needs your hands-on involvement.
Automated API Testing with Selenium:
• Automated Testing Tools (e.g., Selenium): These are the tools that do the heavy lifting for you. Selenium, for example, automates the testing process so you don’t have to do it manually.Automated testing is a real time-saver. It handles tests quickly, letting you focus on other tasks.
• High Scalability: This approach scales effortlessly with your API. As your API grows, automated testing can keep up without missing a beat.
• No Manual Interaction Required: The beauty of automation here is that it runs on its own—no need for constant human input.
Comparison Attributes:
• Time Savings: This attribute showcases how much time you save with automation compared to doing things manually.
• Efficiency: Here, we compare how effectively each method works overall.
• Scalability: This is all about how well each method adapts to growth and increased workload.
In essence, this diagram not only highlights the key differences between manual and automated testing but also provides a clear view of how automation with tools like Selenium can make your life a lot easier. By automating your tests, you’re not just saving time—you’re also ensuring your testing process is scalable and efficient.
The Role of Selenium in Continuous Testing
Automating API tests with Selenium can easily fit into your continuous integration (CI) and continuous deployment (CD) pipelines. Since Selenium is compatible with Jenkins, Travis CI, and other CI/CD tools, you can run your API tests whenever new code is pushed, ensuring quick feedback and higher test reliability.
Wrapping It All Up
Automating API tests is crucial to maintaining a robust and scalable application. While there are specialized tools for API testing, Selenium offers flexibility, allowing you to unify your API and UI testing within a single framework.
This can streamline your testing process, enhance productivity, and provide more
comprehensive test coverage.
By integrating Selenium into your API testing strategy, you can reduce testing time, improve accuracy, and ensure smoother, more reliable releases — all essential ingredients in delivering quality software.
Ready to take your API testing to the next level with Selenium? Let’s start automating!
Enjoy!