Binary is an information encoding method based on binary numbers. It uses binary value representation (usually 0 and 1) to store and process all types of data, including text, images, audio, etc.
The binary system is the most basic data representation method in computer technology because the underlying computer hardware (such as logic gates and flip-flops) can only recognize two states: on (1) and off (0).
What is Axios?
Axios is a popular JavaScript library used for making HTTP requests from web browsers or Node.js environments. It provides a simple and consistent interface for sending asynchronous HTTP requests to REST endpoints and handling the responses.
What is a Binary File?
A binary file is a type of computer file that contains data in a binary format, which consists of a sequence of binary digits (bits) representing various kinds of data, such as executable code, images, audio, or video files. Unlike text files, which store data in human-readable characters (e.g., ASCII or Unicode), binary files store data in a format that is optimized for computer processing and storage.
When you upload a binary file, you transfer the exact sequence of binary data from your local machine to a remote server or another destination. This process is commonly used in software development for uploading compiled program files, libraries, or other resources required for the operation of a software application.
Binary upload is often contrasted with text-based upload, where the data being uploaded consists of human-readable characters encoded in a specific character set, such as ASCII or UTF-8. Text-based upload is more suitable for transferring textual content, such as configuration files, source code, or documents. Let's we will simply describe the concept of a server binary file.
Why Use Binary Format for Uploading?
The main reasons for using binary data to send to the server are as follows:
- Efficient data transmission: Binary data is compact, reducing transmission size and bandwidth usage.
- Faster data processing: Servers parse binary data quicker than text, improving processing efficiency.
- Enhanced data integrity: Binary data is less prone to errors and can be verified for integrity during transmission.
- Versatile support: Binary format accommodates various data types, making it flexible for different types of uploads.
Sending binary data to the server can improve the efficiency of data transmission and processing, protect the integrity of the data, and support multiple data types. It is a commonly used data representation form in network communications. Let's use Apidog to further understand the upload of Binary format data.
How to Upload Binary Files with a Visual Way
In Apidog, we need to send binary data like images, audio, or videos. The primary method for this is through file upload. When selecting Binary as the Body type, you'll typically have the option to upload a file instead of entering binary data directly. This is because inputting binary data directly into a text box, especially for large files, is inconvenient.
To send binary data via Apidog:
- Open Apidog and create a new request. After creating an HTTP project, click the "+" icon to create a new request. Set the request method (e.g., POST) and fill in the requested URL.
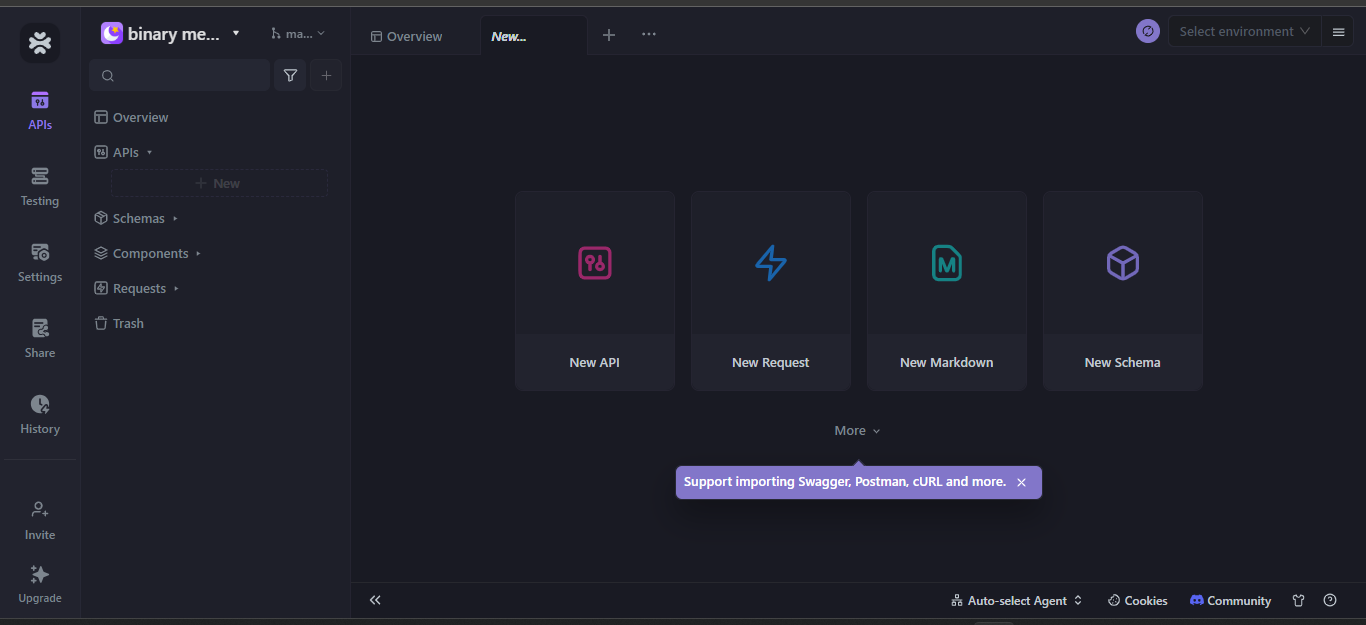
2. Select the Body type as Binary
In the request editing interface, select the "Body" tab, select "binary" from the "Binary" option under the "Body" tab, and save it after the configuration is completed.
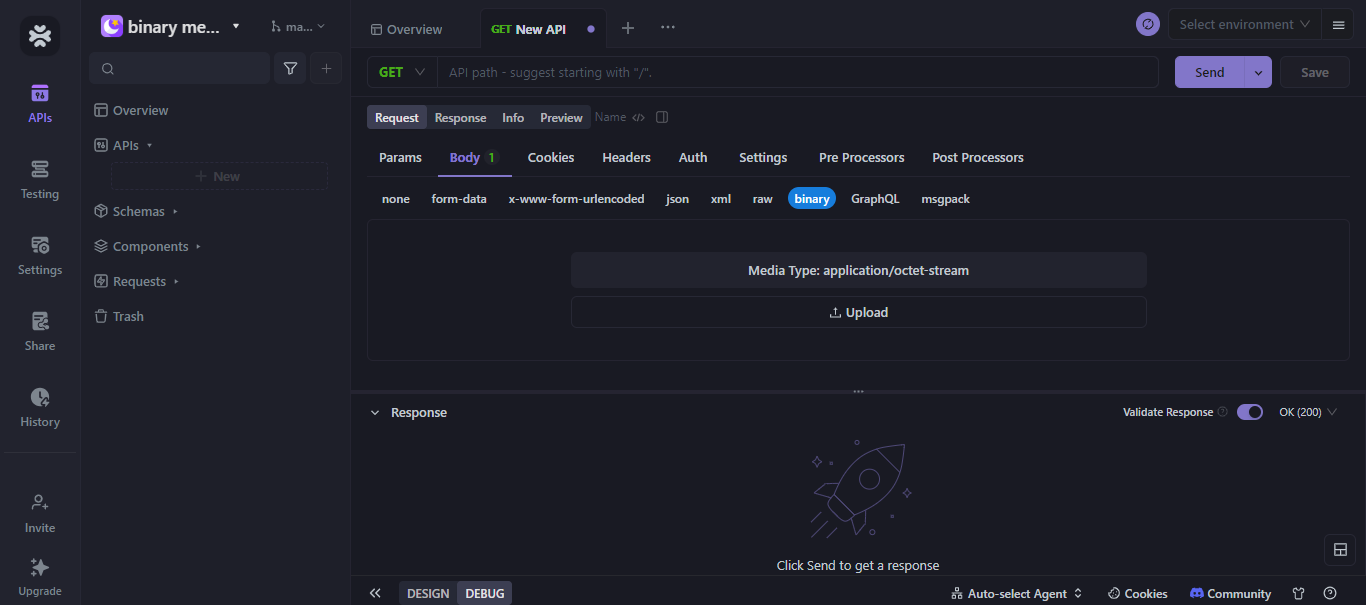
3. You can choose any media type, like an image, video, or audio. Choose the Request type PUT, input your API, choose your environment, change the base URL to your server address, save your request, and click the send button.
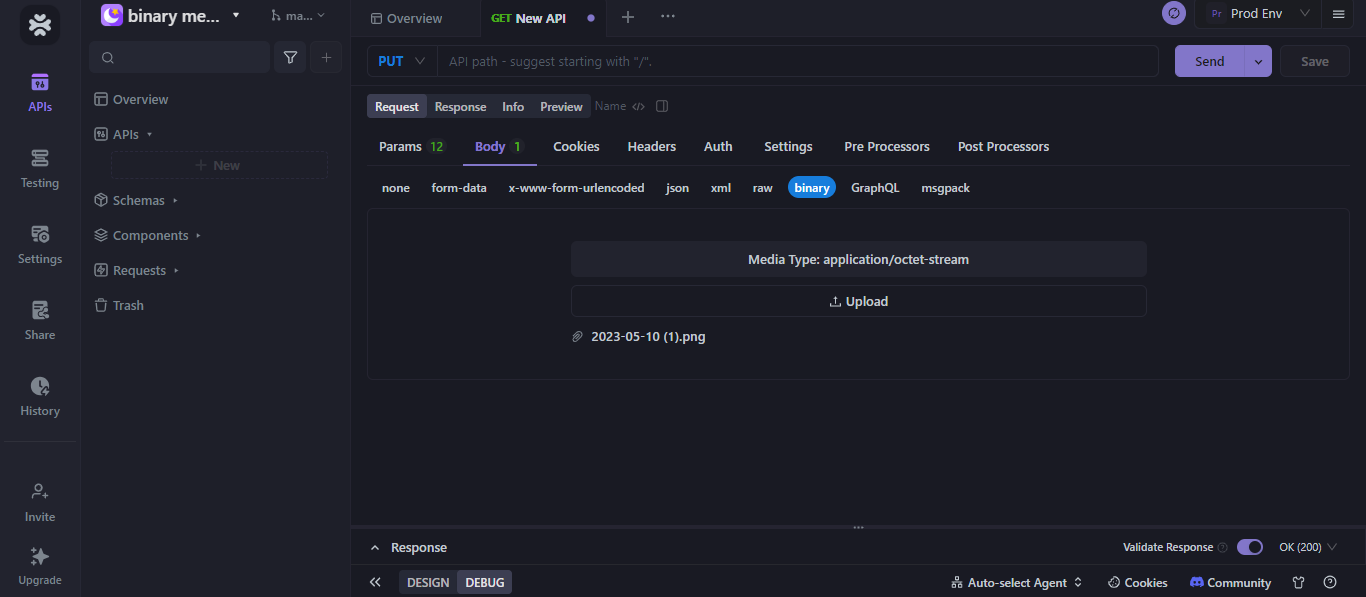
4. You can check the response obtained from your request.
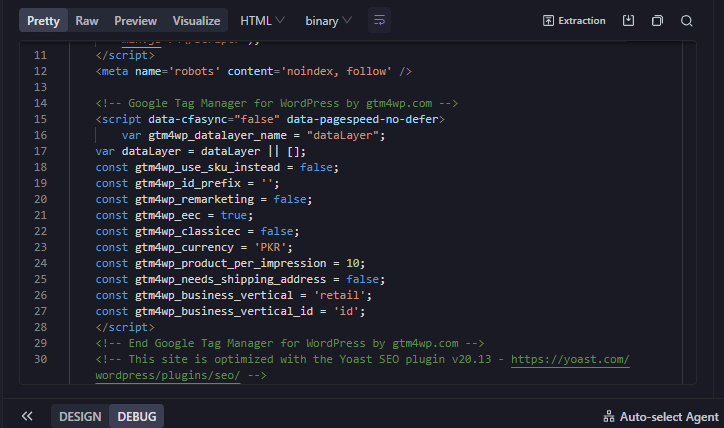
How to Upload and Send Binary File in Axios
Uploading data in binary format to the server, such as uploading files, can also be achieved through the Axios library. Here is a step-by-step breakdown:
1. Create a FormData object
First, you need to add your binary data to the FormData object. FormData is a method used to encode data into a message and send it to the server in a way that simulates the form submission behavior.
let formData = new FormData();
// Assume you have a file input element or a Blob object to upload
formData.append('file', binaryData, 'filename.ext');
Here 'file' is the field name of the file you get on the server side, binaryData is the binary data you want to upload (which can be a file obtained from <input type="file">
or any Blob object), and 'filename.ext' is the intended file name to save on the server.
2. Configure Axios
Next, you need to configure the Axios request, specifically the headers. Since you're uploading a file, it's useful to have the Content-Type header set to 'multipart/form-data'. In most cases, the browser handles this automatically when you use FormData.
const config = {
headers: {
// `Content-Type` will be automatically set by the browser
'Accept': 'application/json', // Just an example, adjust as needed
},
};
3. Send a request
Finally, use Axios to send the formData object.
axios.post('your-server-endpoint', formData, config)
.then(response => {
// Handle successful response
console.log(response.data);
})
.catch(error => {
// Handle errors
console.error(error);
});
Here 'your-server-endpoint' is the server endpoint to which you wish to upload files.
Uploading binary data to the server involves creating a FormData object, adding your files (or other binary data) to it, and then sending the object through Axios. Make sure that Axios' configuration is set up correctly, especially the config headers section, and then handle responses or errors.
Note that the server also needs to be configured correctly to receive multipart/form-data requests and handle uploaded files. This usually involves setting up request body parsing, file storage logic, etc., which will not be described in detail.
Conclusion
Through the above steps, we can easily send binary data in Apidog, whether it is pictures, audio, video, or other types of files. Using the file upload function provided by Apidog, we can easily send local file content to the target API to realize binary data transmission and testing. This provides developers with convenience when developing and testing APIs, while also improving work efficiency.