Introduction to Rest Assured API Testing for Beginners
Learn the basics of REST Assured API testing with this beginner-friendly guide. Understand how to automate API testing, write your first test scripts, and improve your testing efficiency with REST Assured.
In the world of software development, APIs (Application Programming Interfaces) have become the backbone of modern applications. As these APIs grow in complexity and importance, the need for robust and efficient testing methodologies has never been more critical. Enter Rest Assured, a Java-based library that has revolutionized the way we approach API testing.
Rest Assured has gained immense popularity among developers and QA professionals for its simplicity and power in automating REST API tests. Its expressive syntax and seamless integration with Java make it an ideal choice for teams looking to implement comprehensive API testing strategies.
In this complete guide, we'll delve deeper into Rest Assured API testing, covering everything from basic setup to advanced techniques, and even explore how it compares to other tools in the API testing landscape.
What is Rest Assured in API Testing?
Rest Assured is a Java-based library specifically designed for testing RESTful APIs. It provides a domain-specific language (DSL) for making HTTP requests and validating responses. Key features include:
- Fluent API for easy test writing
- Support for XML and JSON parsing
- Integration with Java ecosystem tools (TestNG, JUnit, etc.)
- Extensive validation capabilities
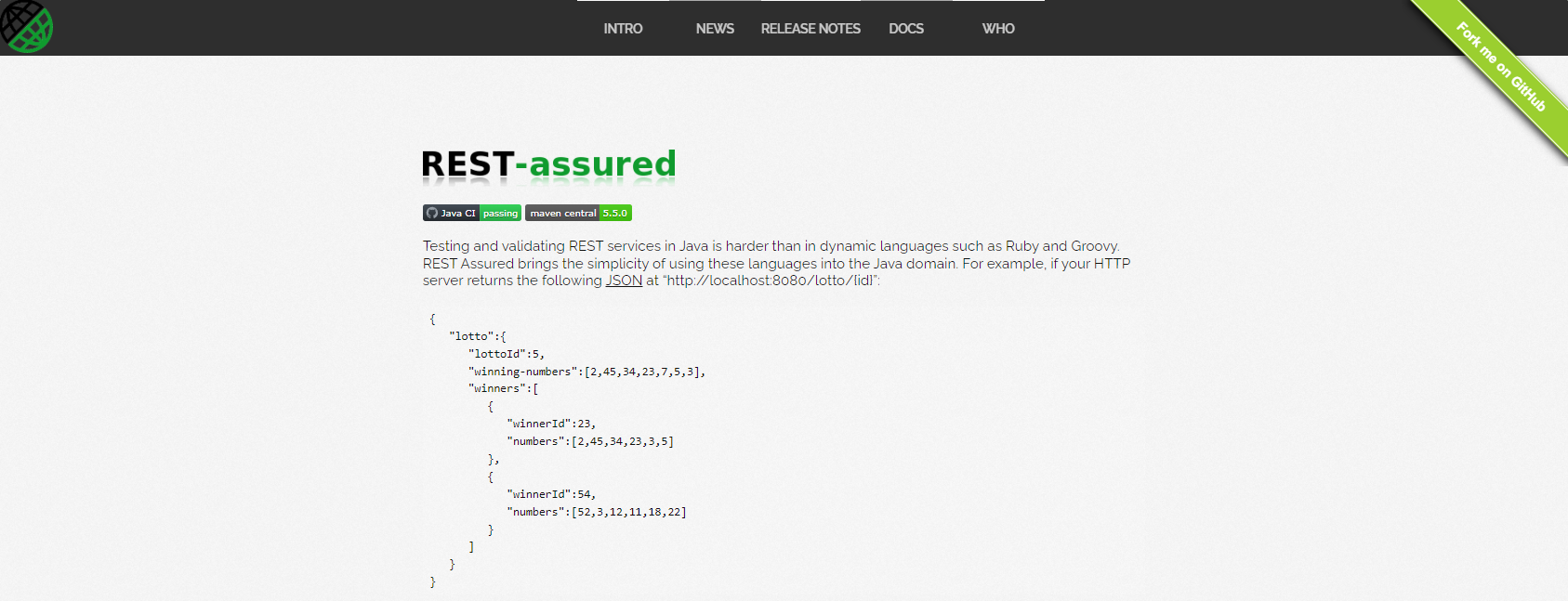
What is REST API in Testing?
REST (Representational State Transfer) API is an architectural style for designing networked applications. In the context of testing, REST API testing involves:
- Verifying correct handling of HTTP methods (GET, POST, PUT, DELETE, etc.)
- Validating response status codes
- Checking response payload structure and content
- Testing API behavior under different scenarios (valid/invalid inputs, authentication, etc.)
- Verifying state changes after API operations
What is the Difference Between Postman and Rest Assured?
While both tools are used for API testing, they serve different purposes:
Feature | Postman | Rest Assured |
---|---|---|
Interface | GUI-based | Code-based |
Language | JavaScript | Java |
Learning Curve | Lower | Steeper |
Automation | Possible with Newman | Native |
CI/CD Integration | Requires additional setup | Seamless |
Scripting Flexibility | Limited to Postman's scripting | Full Java ecosystem |
Rest Assured is typically preferred when you need to integrate API tests into your Java-based test automation framework or when you require more complex test scenarios.
What is the Difference Between TestNG and Rest Assured?
TestNG and Rest Assured serve different purposes but are often used together:
Feature | TestNG | Rest Assured |
---|---|---|
Primary Purpose | Test framework | API testing library |
Scope | General-purpose testing | Specific to API testing |
Features | Test organization, parallel execution, reporting | HTTP requests, response validation |
Language Support | Java | Java |
You can use TestNG to structure and run your Rest Assured tests, combining the strengths of both tools. For example:
import org.testng.annotations.BeforeClass;
import org.testng.annotations.Test;
import static io.restassured.RestAssured.*;
import static org.hamcrest.Matchers.*;
public class CombinedTest {
@BeforeClass
public void setup() {
baseURI = "https://api.example.com";
basePath = "/v1";
}
@Test(groups = "smoke")
public void testGetUser() {
given()
.pathParam("id", 1)
.when()
.get("/users/{id}")
.then()
.statusCode(200)
.body("name", notNullValue());
}
@Test(groups = "regression")
public void testCreateUser() {
String newUser = "{\"name\":\"John Doe\",\"email\":\"john@example.com\"}";
given()
.contentType("application/json")
.body(newUser)
.when()
.post("/users")
.then()
.statusCode(201)
.body("id", notNullValue());
}
}
This example uses TestNG annotations to organize the tests and Rest Assured for the actual API testing logic.
Getting Started with Rest Assured API Testing
Before we dive into the intricacies of Rest Assured, let's set up our development environment to ensure we're ready to start testing.
Setting Up Your Environment
Install Java: Rest Assured requires Java 8 or higher. Download and install the latest JDK from Oracle's website or use OpenJDK.
Choose an IDE: While you can use any text editor, an Integrated Development Environment (IDE) can significantly boost your productivity. Popular choices include:
- IntelliJ IDEA
- Eclipse
- Visual Studio Code with Java extensions
Set up a Maven project: Maven will help manage our dependencies. Create a new Maven project in your IDE or use the command line:
mvn archetype:generate -DgroupId=com.example -DartifactId=rest-assured-demo -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
Add Rest Assured dependency: Open your pom.xml
file and add the following dependency:
<dependencies>
<dependency>
<groupId>io.rest-assured</groupId>
<artifactId>rest-assured</artifactId>
<version>4.4.0</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.4.0</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.hamcrest</groupId>
<artifactId>hamcrest</artifactId>
<version>2.2</version>
<scope>test</scope>
</dependency>
</dependencies>
This includes Rest Assured, TestNG for test organization, and Hamcrest for additional matchers.
Update Maven: Run mvn clean install
to download the dependencies.
With these steps completed, you're now ready to start writing Rest Assured tests!
Basic Rest Assured API Testing Concepts
Rest Assured follows a Given-When-Then syntax, inspired by behavior-driven development (BDD). This structure makes tests readable and intuitive, even for those not familiar with the codebase.
The Given-When-Then Structure
- Given: Set up the test preconditions (e.g., parameters, headers, authentication)
- When: Perform the API action (GET, POST, PUT, DELETE, etc.)
- Then: Assert the response (status code, body content, headers)
Let's break down a simple example:
import static io.restassured.RestAssured.*;
import static org.hamcrest.Matchers.*;
import org.testng.annotations.Test;
public class SimpleTest {
@Test
public void testGetRequest() {
given()
.baseUri("https://api.example.com")
.when()
.get("/users")
.then()
.statusCode(200)
.body("data.size()", greaterThan(0))
.body("data[0].id", notNullValue())
.body("data[0].email", containsString("@"));
}
}
This test does the following:
- Sets the base URI for the API
- Sends a GET request to the "/users" endpoint
- Asserts that:
- The status code is 200
- The response contains a non-empty data array
- The first user in the array has a non-null ID
- The first user's email contains an "@" symbol
Understanding Rest Assured Syntax
Rest Assured uses a fluent interface, allowing you to chain method calls. Here's a breakdown of some common methods:
given()
: Starts the test specificationbaseUri()
,basePath()
: Set the base URL and path for the requestparam()
,queryParam()
: Add query parametersheader()
,headers()
: Set request headersbody()
: Set the request bodywhen()
: Marks the start of the request sectionget()
,post()
,put()
,delete()
: HTTP methodsthen()
: Starts the validation sectionstatusCode()
: Asserts the response status codebody()
: Validates the response body
Advanced Rest Assured API Testing Techniques
As you become more comfortable with Rest Assured, you'll want to explore its more advanced features to create robust and comprehensive test suites.
Handling Authentication in Rest Assured API Testing
Many APIs require authentication. Rest Assured supports various authentication methods:
Basic Authentication
given()
.auth().basic("username", "password")
.when()
.get("/secure-endpoint")
.then()
.statusCode(200);
OAuth 2.0
given()
.auth().oauth2("your_access_token")
.when()
.get("/oauth2-protected-endpoint")
.then()
.statusCode(200);
Custom Authentication
For APIs with custom authentication schemes, you can add headers manually:
given()
.header("X-API-Key", "your-api-key")
.when()
.get("/custom-auth-endpoint")
.then()
.statusCode(200);
Parameterizing Tests in Rest Assured API Testing
Parameterized tests allow you to run the same test with different inputs, increasing your test coverage:
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
public class ParameterizedTest {
@DataProvider(name = "userIds")
public Object[][] createUserIds() {
return new Object[][] {{1}, {2}, {3}, {4}, {5}};
}
@Test(dataProvider = "userIds")
public void testMultipleUsers(int userId) {
given()
.pathParam("id", userId)
.when()
.get("https://api.example.com/users/{id}")
.then()
.statusCode(200)
.body("id", equalTo(userId))
.body("name", notNullValue());
}
}
This test will run five times, once for each user ID provided by the data provider.
Validating JSON Responses in Rest Assured API Testing
Rest Assured provides powerful JSON parsing capabilities using JSONPath:
given()
.when()
.get("https://api.example.com/users/1")
.then()
.statusCode(200)
.body("name", equalTo("John Doe"))
.body("email", endsWith("@example.com"))
.body("roles", hasItems("user", "admin"))
.body("address.city", equalTo("New York"))
.body("phoneNumbers.size()", greaterThanOrEqualTo(1));
This test validates various aspects of the JSON response, including nested objects and arrays.
Handling XML Responses
While JSON is more common, some APIs still use XML. Rest Assured can handle XML responses as well:
given()
.when()
.get("https://api.example.com/data.xml")
.then()
.statusCode(200)
.body("root.data.name", equalTo("Test Name"))
.body("root.data.value", equalTo("100"));
File Upload and Download
Rest Assured can handle file operations:
File Upload
File fileToUpload = new File("path/to/file.txt");
given()
.multiPart("file", fileToUpload)
.when()
.post("/upload")
.then()
.statusCode(200)
.body("message", equalTo("File uploaded successfully"));
File Download
byte[] downloadedFile = given()
.when()
.get("/download/file.pdf")
.then()
.statusCode(200)
.extract().asByteArray();
// Now you can write the byte array to a file or process it further
Using Apidog with Rest Assured API Testing
While Rest Assured is powerful on its own, you can enhance your API testing workflow by incorporating Apidog, an API documentation and testing platform. Here's how you can use Apidog alongside Rest Assured:
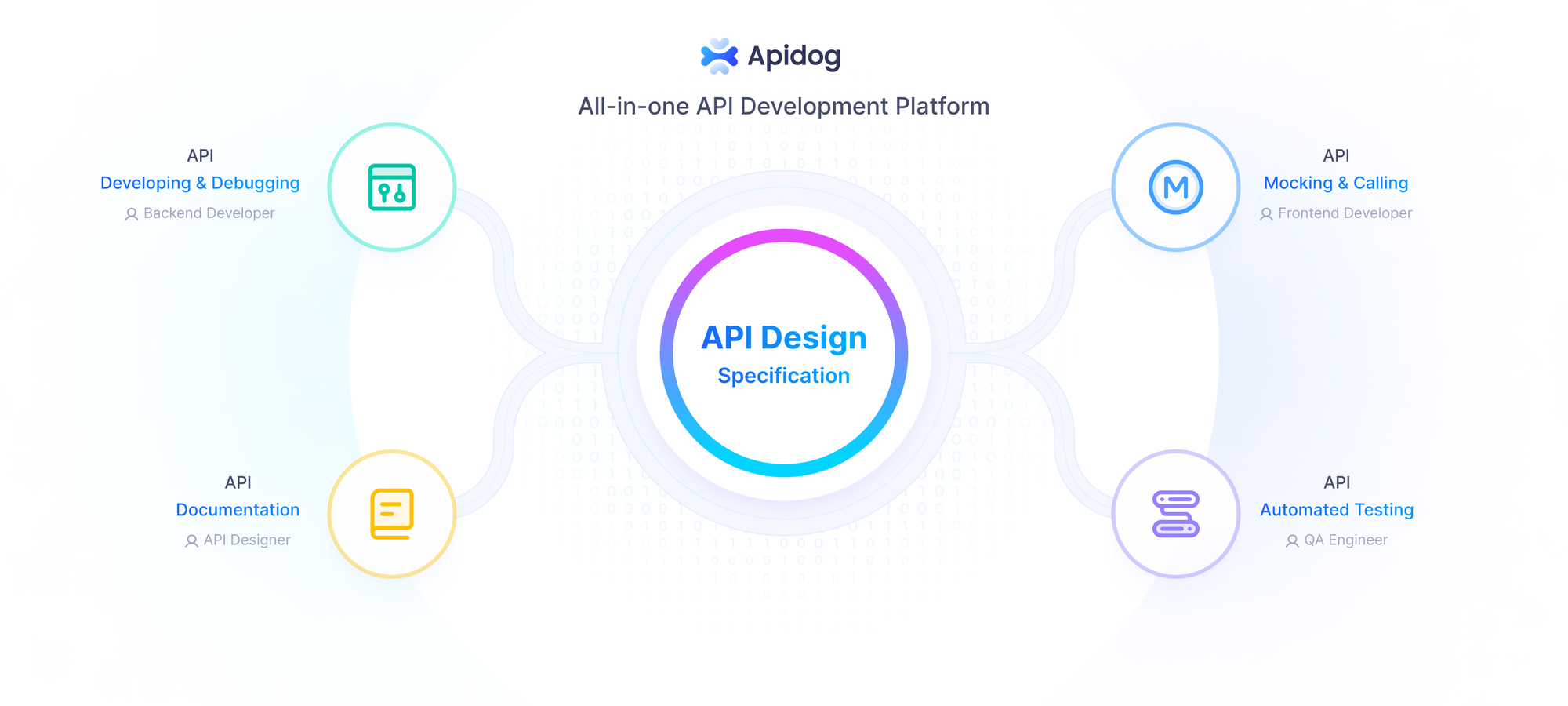
Design APIs: Use APIdog to design and document your APIs before implementation. This helps in creating a clear specification for developers and testers.
Generate Tests: APIdog can generate test cases based on your API specifications. While these aren't directly Rest Assured tests, they can serve as a blueprint for what to test.
Implement Tests: Translate the APIdog-generated test cases into Rest Assured tests. This ensures your tests cover all specified behaviors.
Collaborate: Share API specifications and test results with your team through APIdog's collaborative features. This keeps everyone aligned on the API's expected behavior.
Maintain Documentation: As you update your Rest Assured tests, ensure the APIdog documentation stays in sync. This helps maintain accurate, up-to-date API documentation.
How to Integrate REST API with Apidog
Integrating a REST API with Apidog involves a few basic steps. Here's a detailed step-by-step process to integrate REST API with Apidog:
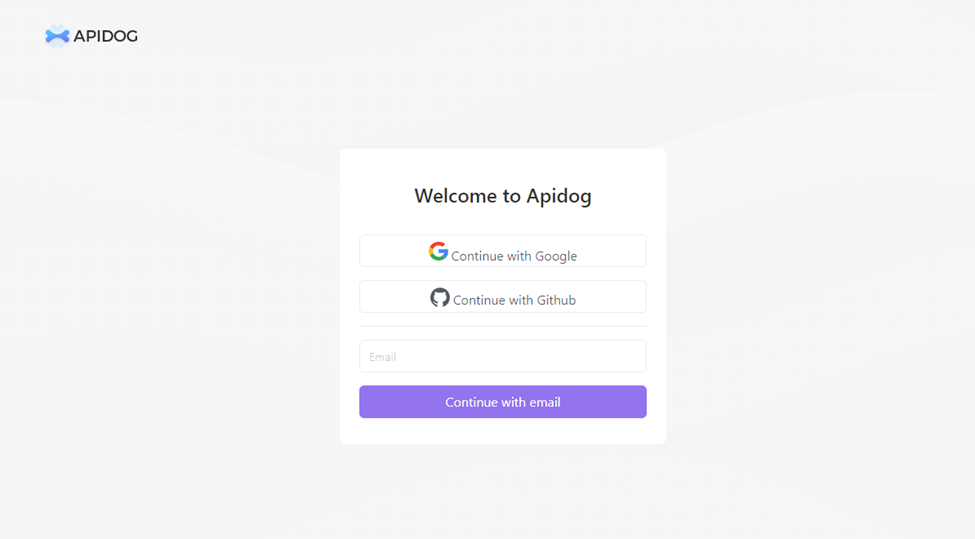
2. Click on "New Project" and give your project a name.
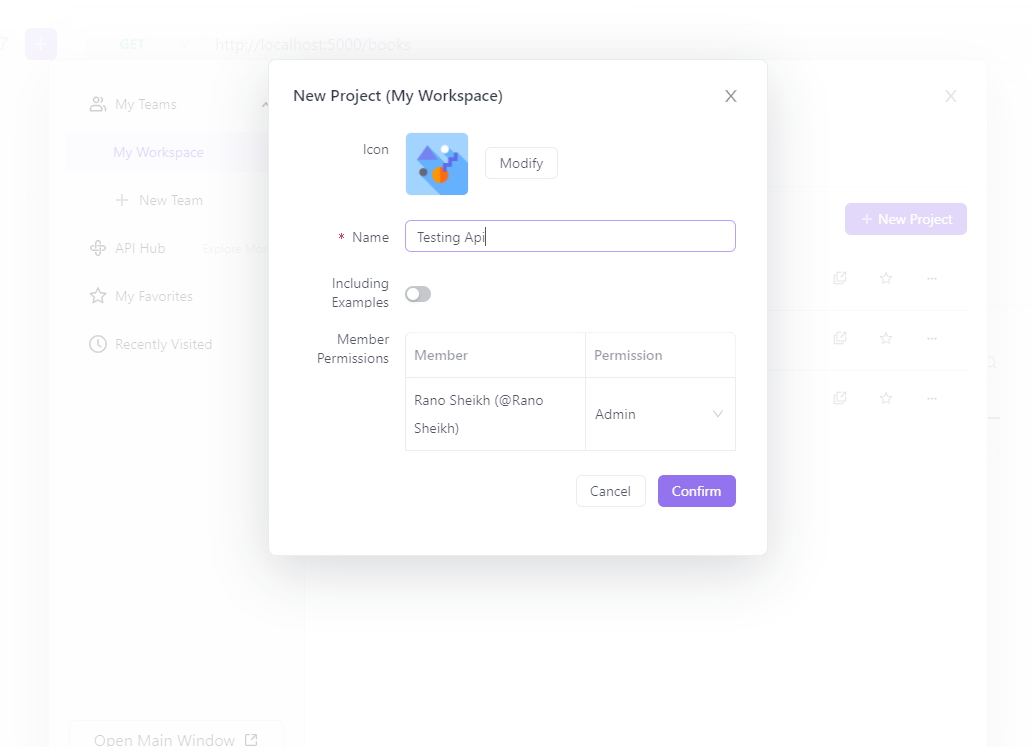
3. Create a New API.
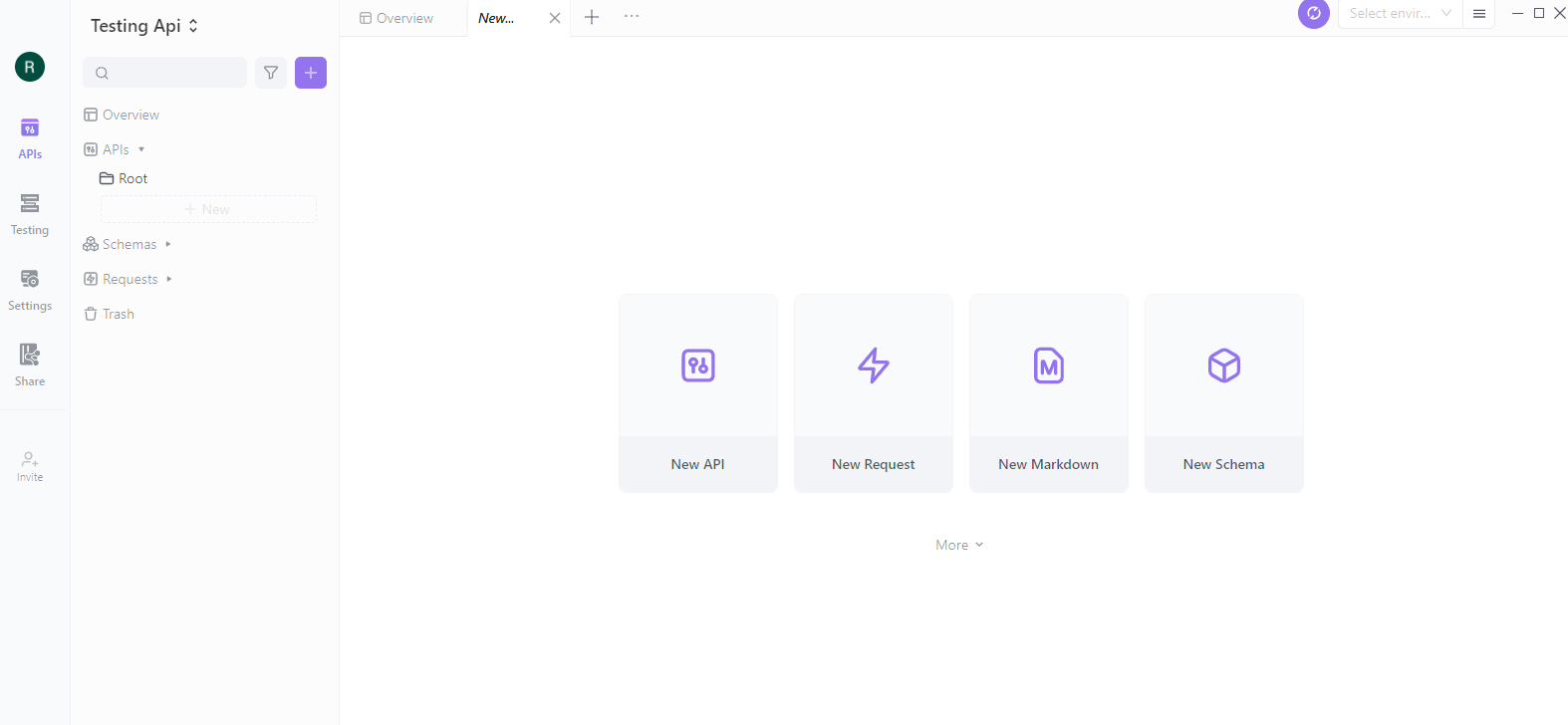
4. Now click on the "Add Endpoint" button and fill in the following details for the "Get all books" endpoint: In this case,
URL: http://localhost:5000/books
Method: GET
Endpoint name: Get all books
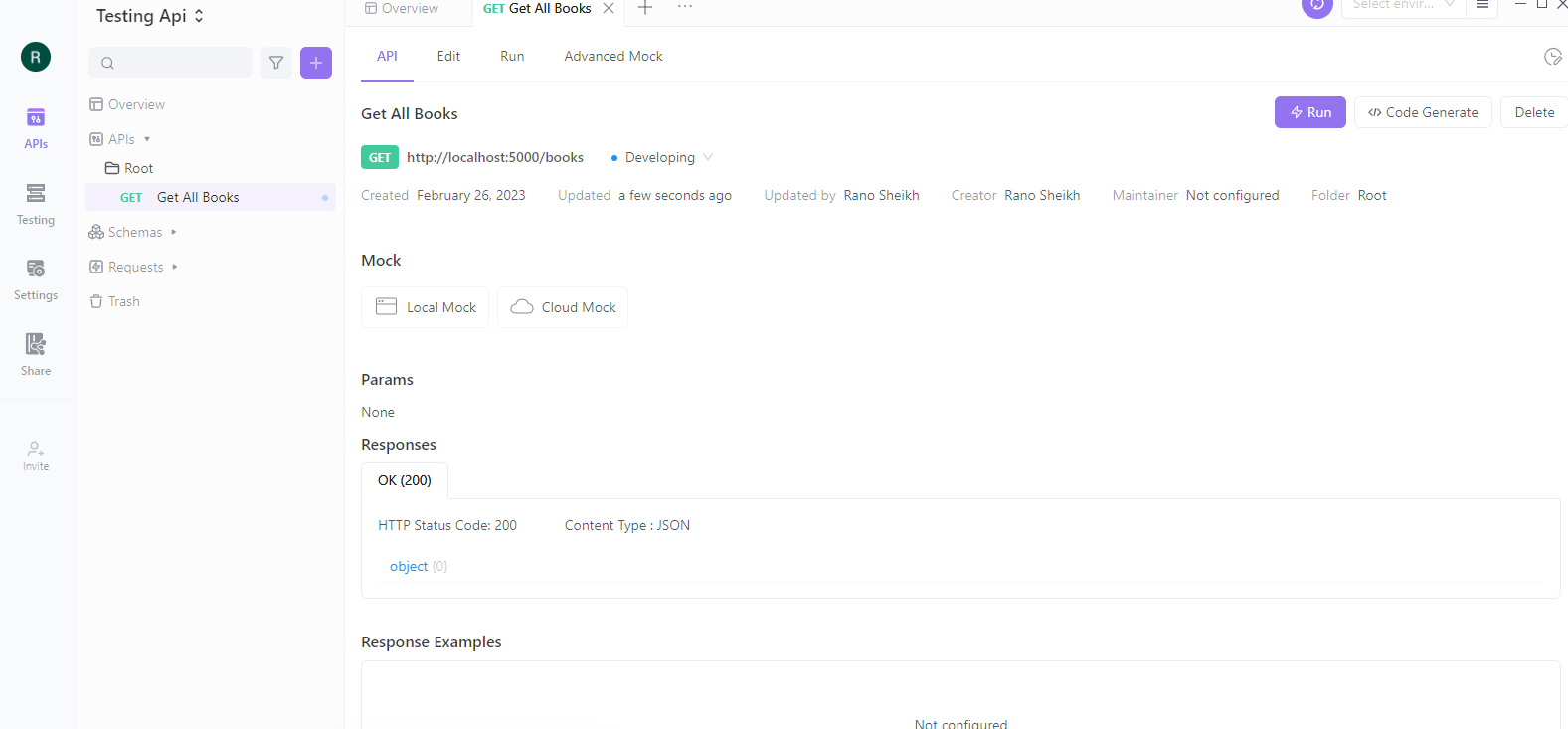
5. Specify any query parameters or headers that your endpoint may require by clicking on the "Add parameter" or "Add header" buttons.
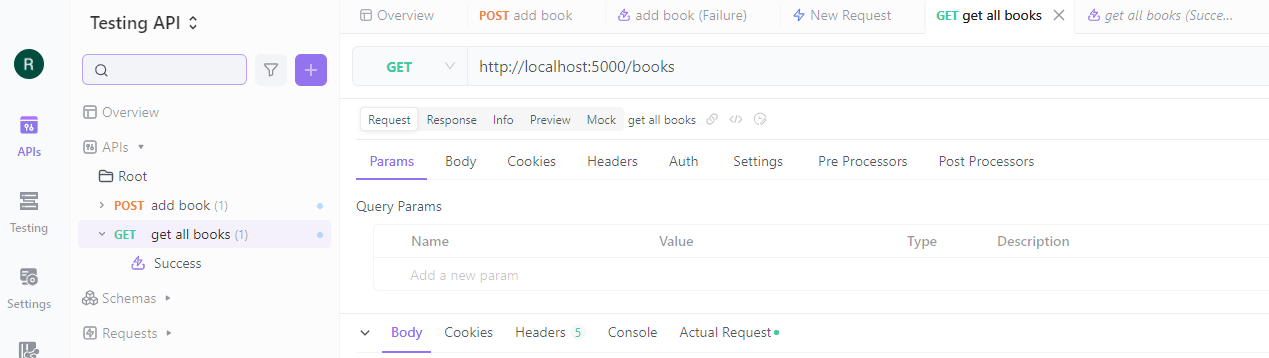
6. Click on the "Send" button to test your endpoint and ensure that it is working properly. Once your endpoint is working as expected, click on the "Save APICase" button to add it to your Apidog project.
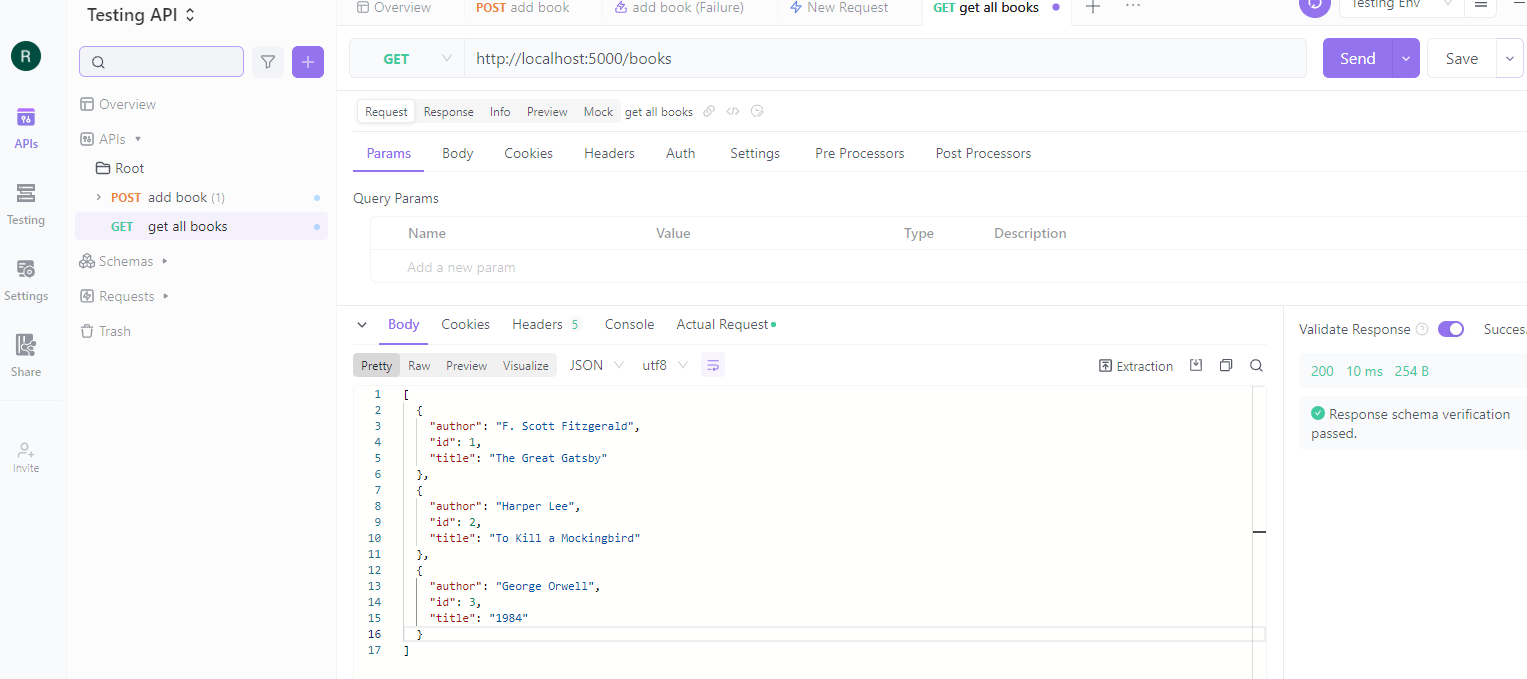
7. You can now use Apidog to test your endpoint and generate documentation for your Flask API.
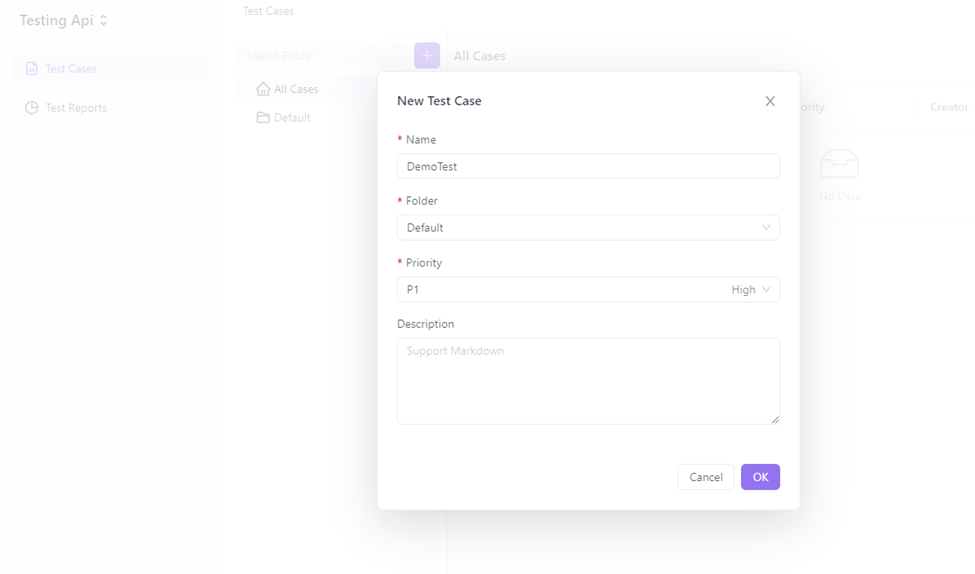
8. Define the test steps of your test case and select the endpoints you want to include in testing. Customize the Test Cases as per your needs.
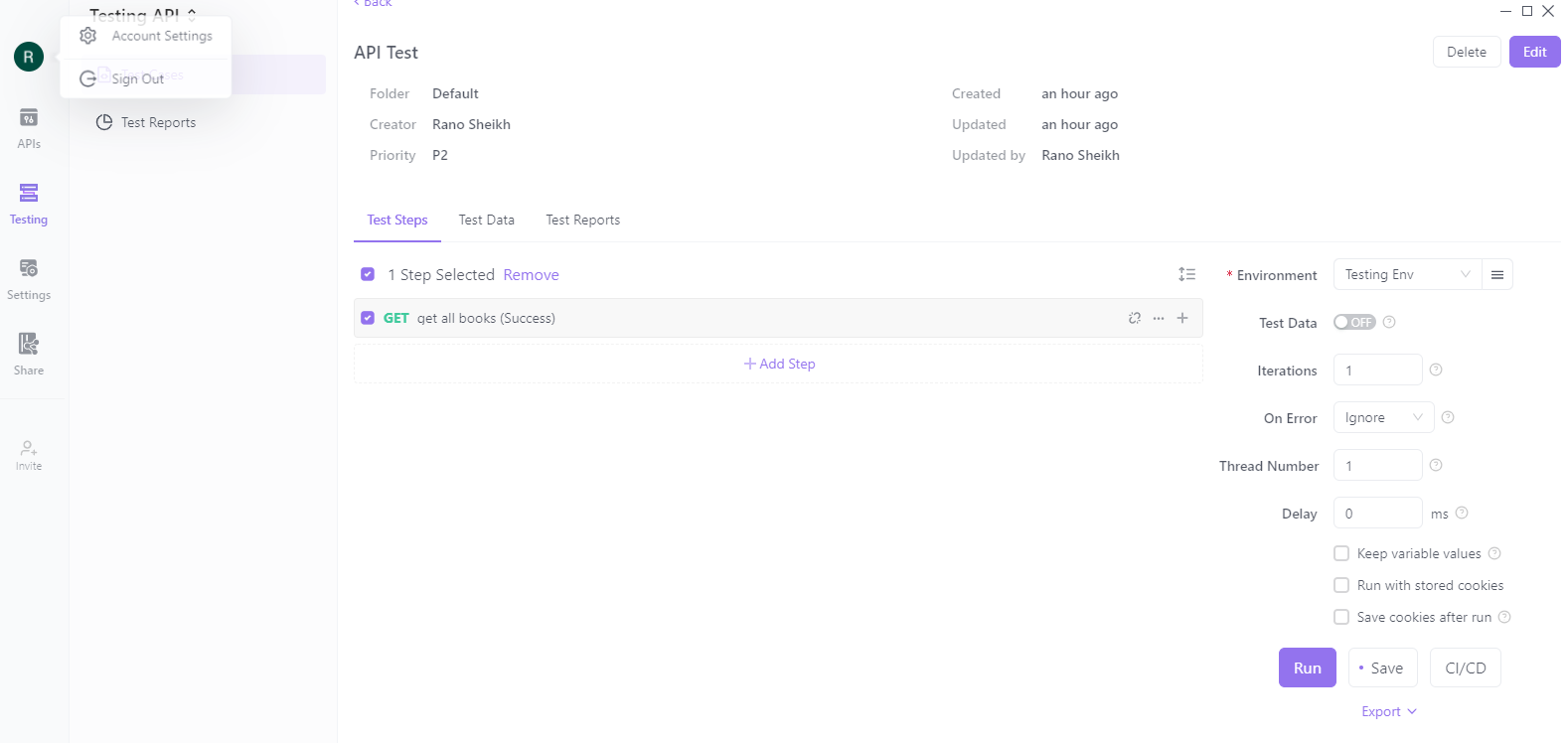
9. Once you test the cases, you can publish them on the web or export them to a PDF or Markdown file.
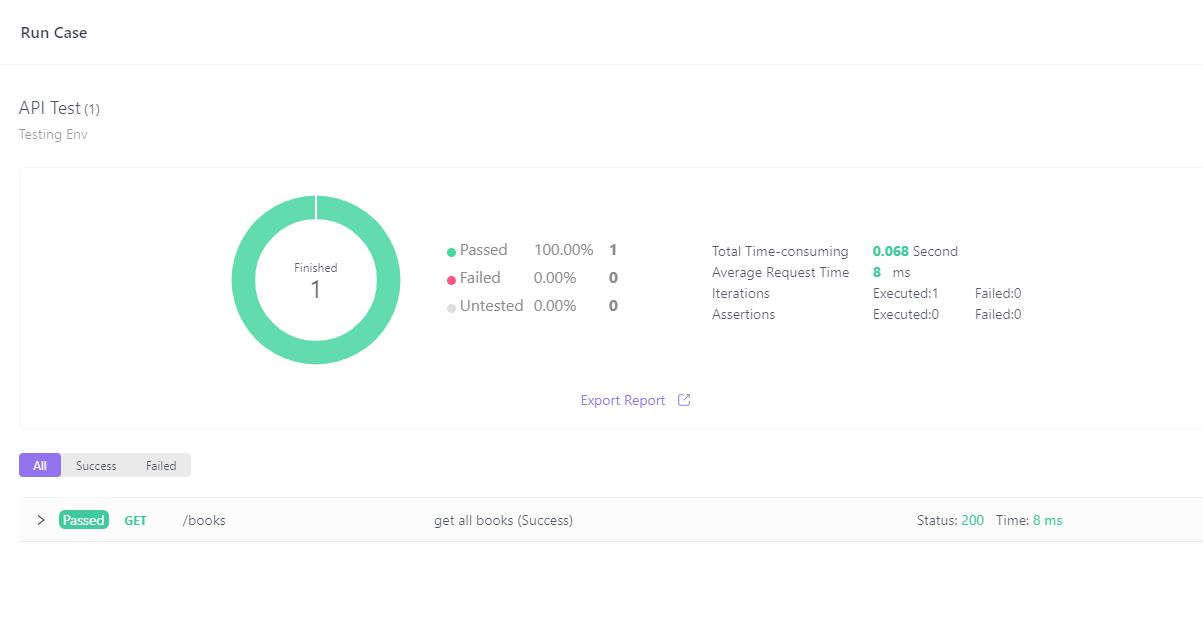
Apidog offers numerous customization options to assist users in utilizing and testing their APIs in accordance with their specific requirements.
Best Practices for Rest Assured API Testing
To get the most out of Rest Assured API testing, consider these best practices:
Organize your tests: Use TestNG or JUnit to structure your tests logically. Group related tests together and use appropriate annotations for setup and teardown.
Reuse code: Create utility methods for common operations to keep your tests DRY (Don't Repeat Yourself). For example:
public class TestUtils {
public static RequestSpecification getBaseRequestSpec() {
return given()
.baseUri("https://api.example.com")
.contentType(ContentType.JSON)
.accept(ContentType.JSON);
}
}
Then use it in your tests:
@Test
public void testSomething() {
TestUtils.getBaseRequestSpec()
.when()
.get("/endpoint")
.then()
.statusCode(200);
}
Use logging: Enable logging to debug issues more easily. Rest Assured provides various logging options:
given()
.log().all() // Log all request details
.when()
.get("/endpoint")
.then()
.log().ifValidationFails() // Log response if assertion fails
.statusCode(200);
Validate schemas: Use JSON Schema validation to ensure response structures are correct:
given()
.when()
.get("/users")
.then()
.assertThat()
.body(matchesJsonSchemaInClasspath("user-schema.json"));
Handle environment-specific data: Use properties files or environment variables to manage different environments:
public class Config {
public static String getBaseUrl() {
return System.getProperty("api.baseUrl", "https://api.example.com");
}
}
Then use this in your tests:
@Test
public void testEndpoint() {
given()
.baseUri(Config.getBaseUrl())
.when()
.get("/users")
.then()
.statusCode(200);
}
Use response extraction: For complex validations, extract the response and perform assertions:
Response response = given()
.when()
.get("/users")
.then()
.extract().response();
JsonPath jsonPath = response.jsonPath();
List<String> names = jsonPath.getList("name");
Assert.assertTrue(names.contains("John Doe"));
Implement custom matchers: For specific validations, create custom Hamcrest matchers:
public class CustomMatchers {
public static Matcher<String> isValidEmail() {
return new TypeSafeMatcher<String>() {
@Override
protected boolean matchesSafely(String item) {
return item.matches("^[A-Za-z0-9+_.-]+@(.+)$");
}
@Override
public void describeTo(Description description) {
description.appendText("should be a valid email");
}
};
}
}
Use it in your tests:
given()
.when()
.get("/users/1")
.then()
.body("email", CustomMatchers.isValidEmail());
Use data providers for comprehensive testing: Leverage TestNG's data providers to test multiple scenarios:
@DataProvider(name = "userRoles")
public Object[][] userRoles() {
return new Object[][] {
{"admin", 200},
{"user", 403},
{"guest", 401}
};
}
@Test(dataProvider = "userRoles")
public void testAccessControl(String role, int expectedStatus) {
given()
.auth().oauth2(getTokenForRole(role))
.when()
.get("/admin-endpoint")
.then()
.statusCode(expectedStatus);
}
Implement retry mechanism: For flaky tests or unreliable networks, implement a retry mechanism:
@Test(retryAnalyzer = RetryAnalyzer.class)
public void testWithRetry() {
// Your test code here
}
public class RetryAnalyzer implements IRetryAnalyzer {
private int retryCount = 0;
private static final int MAX_RETRY_COUNT = 3;
@Override
public boolean retry(ITestResult result) {
if (retryCount < MAX_RETRY_COUNT) {
retryCount++;
return true;
}
return false;
}
}
Use specifications: For consistent request/response expectations, use specifications:
RequestSpecification requestSpec = new RequestSpecBuilder()
.setBaseUri("https://api.example.com")
.setContentType(ContentType.JSON)
.build();
ResponseSpecification responseSpec = new ResponseSpecBuilder()
.expectStatusCode(200)
.expectContentType(ContentType.JSON)
.build();
@Test
public void testWithSpecs() {
given()
.spec(requestSpec)
.when()
.get("/users")
.then()
.spec(responseSpec);
}
Conclusion
Rest Assured API testing offers a powerful and flexible way to automate your API tests. By combining it with tools like TestNG and APIdog, you can create a comprehensive API testing strategy that integrates seamlessly with your Java-based projects.
The key advantages of using Rest Assured include:
- Simplicity: Its fluent API makes writing tests intuitive and readable.
- Power: It provides extensive capabilities for request building and response validation.
- Integration: It works well with the Java ecosystem, including build tools and CI/CD pipelines.
- Flexibility: From simple GET requests to complex authentication and file handling, Rest Assured can handle it all.
As APIs continue to play a crucial role in modern software architecture, robust API testing becomes increasingly important. Rest Assured equips you with the tools to ensure your APIs are reliable, performant, and behave as expected.
Remember, effective API testing is not just about finding bugs; it's about ensuring the quality and reliability of your entire system. With Rest Assured, you have a versatile tool at your disposal to thoroughly test your APIs and catch issues before they reach production.
As you continue your journey with Rest Assured, keep exploring its features, stay updated with the latest versions, and don't hesitate to contribute to the open-source community. Happy testing!