API testing has become a crucial part of software development. As the world leans more toward microservices and interconnected systems, ensuring that APIs are robust and reliable is paramount. Python, with its simplicity and extensive library support, has emerged as a preferred language for API testing. In this post, we will explore everything you need to know about API testing with Python.Plus, we'll show you how to get started with Apidog for free.
What is API Testing?
Before diving into the specifics of Python API testing, let's understand what API testing entails. An Application Programming Interface (API) is a set of rules and protocols for building and interacting with software applications. API testing involves verifying that these interactions are as expected. It includes sending requests to various endpoints and validating the responses against expected outcomes.
Why Python for API Testing?
You might wonder, "Why Python?" Well, Python is known for its simplicity and readability, which makes it a fantastic choice for scripting tests. Moreover, Python boasts a rich ecosystem of libraries and tools tailored for API testing. Whether you're a seasoned developer or a beginner, Python offers a gentle learning curve and powerful capabilities.
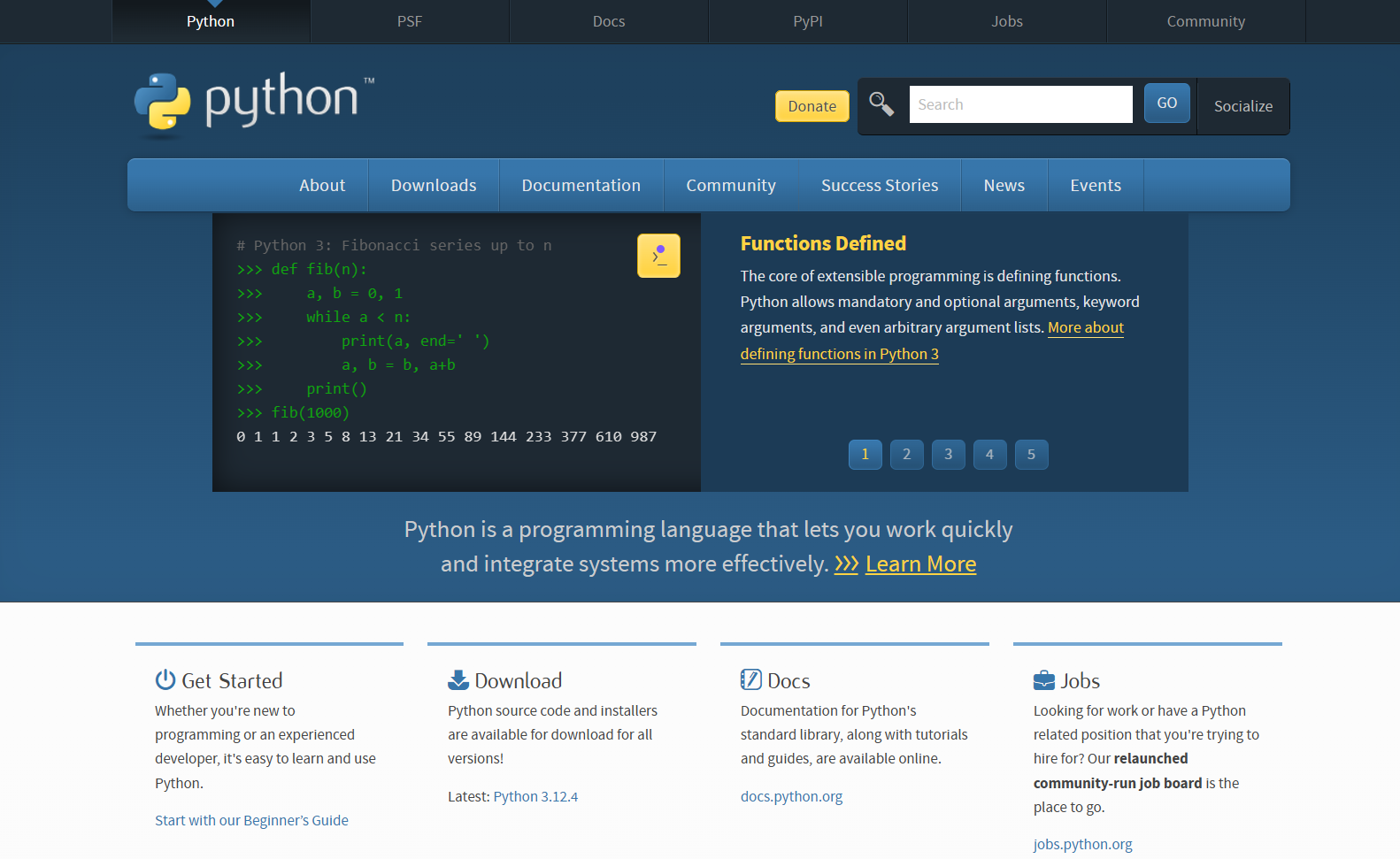
Getting Started with Python API Testing
Ready to jump into Python API testing? Let's start with the basics.
Setting Up Your Environment
First, you'll need to set up your Python environment. If you haven't already, download and install Python from the official website. Once installed, verify the installation by running:
python --version
You'll also need to install some essential libraries. Open your terminal and run:
pip install requests pytest
Understanding HTTP Methods
APIs communicate via HTTP methods. The most common ones include:
- GET: Retrieve data from the server.
- POST: Send data to the server to create a resource.
- PUT: Update an existing resource on the server.
- DELETE: Remove a resource from the server.
Understanding these methods is crucial for effective API testing.
Your First API Test with Python
Let's write our first API test using the requests
library.
import requests
def test_get_request():
response = requests.get('https://jsonplaceholder.typicode.com/posts/1')
assert response.status_code == 200
assert response.json()['id'] == 1
if __name__ == '__main__':
test_get_request()
print("Test Passed!")
This simple script sends a GET request to a sample API and checks if the response status is 200 and the ID of the returned post is 1.
Advanced API Testing with Pytest
While simple scripts are great for getting started, real-world applications require more robust testing frameworks. This is where pytest
comes in. pytest
is a powerful testing framework that makes it easy to write scalable test suites.
Installing and Setting Up Pytest
Install pytest
using pip:
pip install pytest
Writing Your First Pytest Test
Here's how you can integrate pytest
into your API testing:
import requests
import pytest
def test_get_request():
response = requests.get('https://jsonplaceholder.typicode.com/posts/1')
assert response.status_code == 200
assert response.json()['id'] == 1
if __name__ == '__main__':
pytest.main()
To run your tests, simply execute:
pytest
Organizing Tests with Fixtures
Fixtures in pytest
are a way to set up some context before running tests. They help in avoiding repetitive code and making the tests cleaner.
import requests
import pytest
@pytest.fixture
def base_url():
return 'https://jsonplaceholder.typicode.com'
def test_get_request(base_url):
response = requests.get(f'{base_url}/posts/1')
assert response.status_code == 200
assert response.json()['id'] == 1
Testing Different HTTP Methods
Let's dive deeper into testing various HTTP methods. Testing different methods ensures that your API handles various types of requests correctly.
Testing POST Requests
A POST request is used to create a new resource. Here's how you can test it:
def test_post_request(base_url):
payload = {
"title": "foo",
"body": "bar",
"userId": 1
}
response = requests.post(f'{base_url}/posts', json=payload)
assert response.status_code == 201
assert response.json()['title'] == "foo"
Testing PUT Requests
A PUT request updates an existing resource. Let's see an example:
def test_put_request(base_url):
payload = {
"id": 1,
"title": "foo updated",
"body": "bar updated",
"userId": 1
}
response = requests.put(f'{base_url}/posts/1', json=payload)
assert response.status_code == 200
assert response.json()['title'] == "foo updated"
Testing DELETE Requests
Finally, a DELETE request removes a resource:
def test_delete_request(base_url):
response = requests.delete(f'{base_url}/posts/1')
assert response.status_code == 200
Handling Authentication in API Testing
Many APIs require authentication. This section will cover how to handle different authentication methods.
Basic Authentication
Basic Authentication requires a username and password encoded in the request header.
def test_basic_auth():
response = requests.get('https://api.example.com/secure-endpoint', auth=('user', 'pass'))
assert response.status_code == 200
Token-Based Authentication
Token-based authentication uses a token, typically in the header, to authenticate requests.
def test_token_auth():
token = 'your_token_here'
headers = {'Authorization': f'Bearer {token}'}
response = requests.get('https://api.example.com/secure-endpoint', headers=headers)
assert response.status_code == 200
Error Handling in API Testing
Handling errors gracefully is crucial in API testing. This ensures that your API responds correctly to invalid requests.
Testing for 404 Errors
A 404 error indicates that the requested resource was not found.
def test_404_error(base_url):
response = requests.get(f'{base_url}/posts/999999')
assert response.status_code == 404
Testing for 400 Errors
A 400 error indicates a bad request, often due to invalid input.
def test_400_error(base_url):
response = requests.post(f'{base_url}/posts', json={})
assert response.status_code == 400
Automating API Testing
Automating your API tests can save time and ensure consistency. You can set up Continuous Integration (CI) pipelines to run your tests automatically.
Using GitHub Actions for CI
GitHub Actions is a powerful CI/CD tool. Here's a simple configuration to run your pytest
tests:
Create a .github/workflows/python-app.yml
file with the following content:
name: Python application
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: '3.x'
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install pytest requests
- name: Run tests
run: |
pytest
This configuration will run your tests every time you push code to your repository.
Tools and Libraries for API Testing
Several tools and libraries can make your API testing process more efficient. Here are some popular ones:
Apidog
Apidog is a comprehensive API development and testing tool that provides a collaborative platform for API documentation, testing, and debugging. It integrates seamlessly with various stages of the API lifecycle, making it an invaluable tool for developers.
Postman
Postman is a powerful API testing tool with a user-friendly interface. You can create, manage, and automate tests using Postman.
Requests
The requests
library in Python is perfect for making HTTP requests and handling responses.
Pytest
pytest
is a robust testing framework that simplifies writing and running tests.
Responses
The responses
library allows you to mock API responses for testing purposes.
Locust
Locust is a performance testing tool that helps you identify performance issues in your APIs.
Integrating Apidog for Seamless API Testing
Remember our mention of Apidog at the beginning? Let’s take a closer look at how this tool can simplify your API testing process.
Why Apidog?
Apidog is an all-in-one API platform that provides tools for designing, testing, and documenting APIs. It’s designed to streamline the entire API development lifecycle, making it easier for developers to create reliable and well-documented APIs.
Key Features of Apidog
- API Design: Create and manage your API specifications with an intuitive interface.
- API Testing: Easily create and run automated tests for your APIs.
- API Documentation: Generate and maintain comprehensive API documentation.
- Collaboration: Work seamlessly with your team on API projects.
How to send Python API request using Apidog
- Open Apidog and click on the "New Request" button to create a new request.
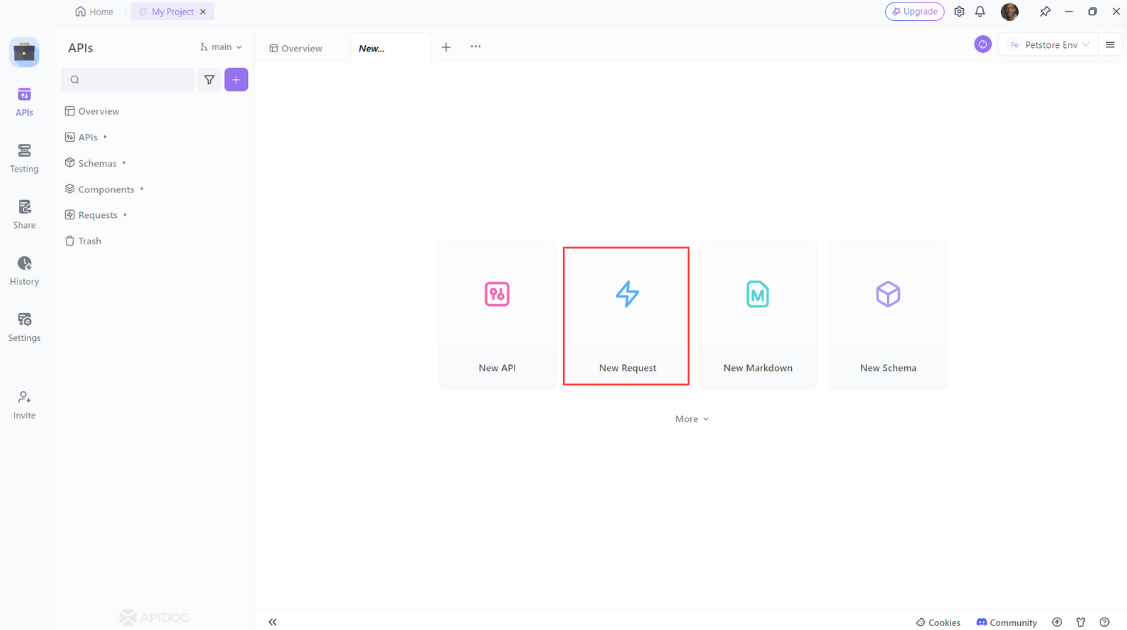
2. Select "GET" as the method of the request.
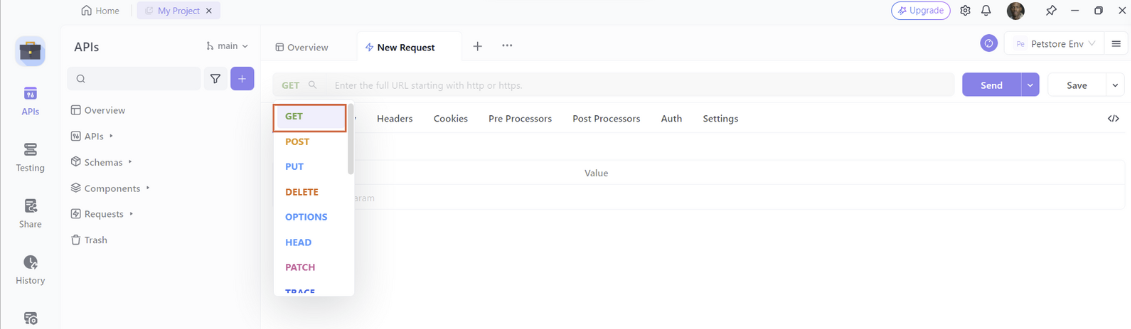
3. Enter the URL of the API endpoint
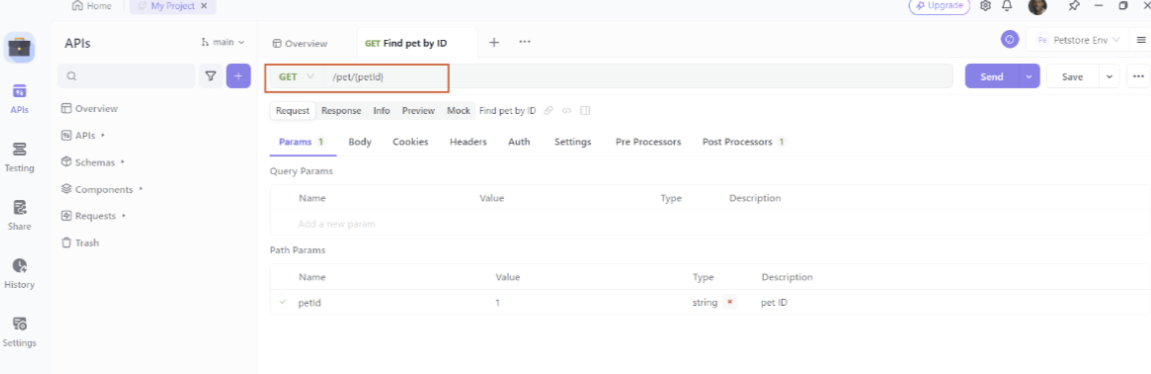
Then click on the “Send” button to send the request to the API.
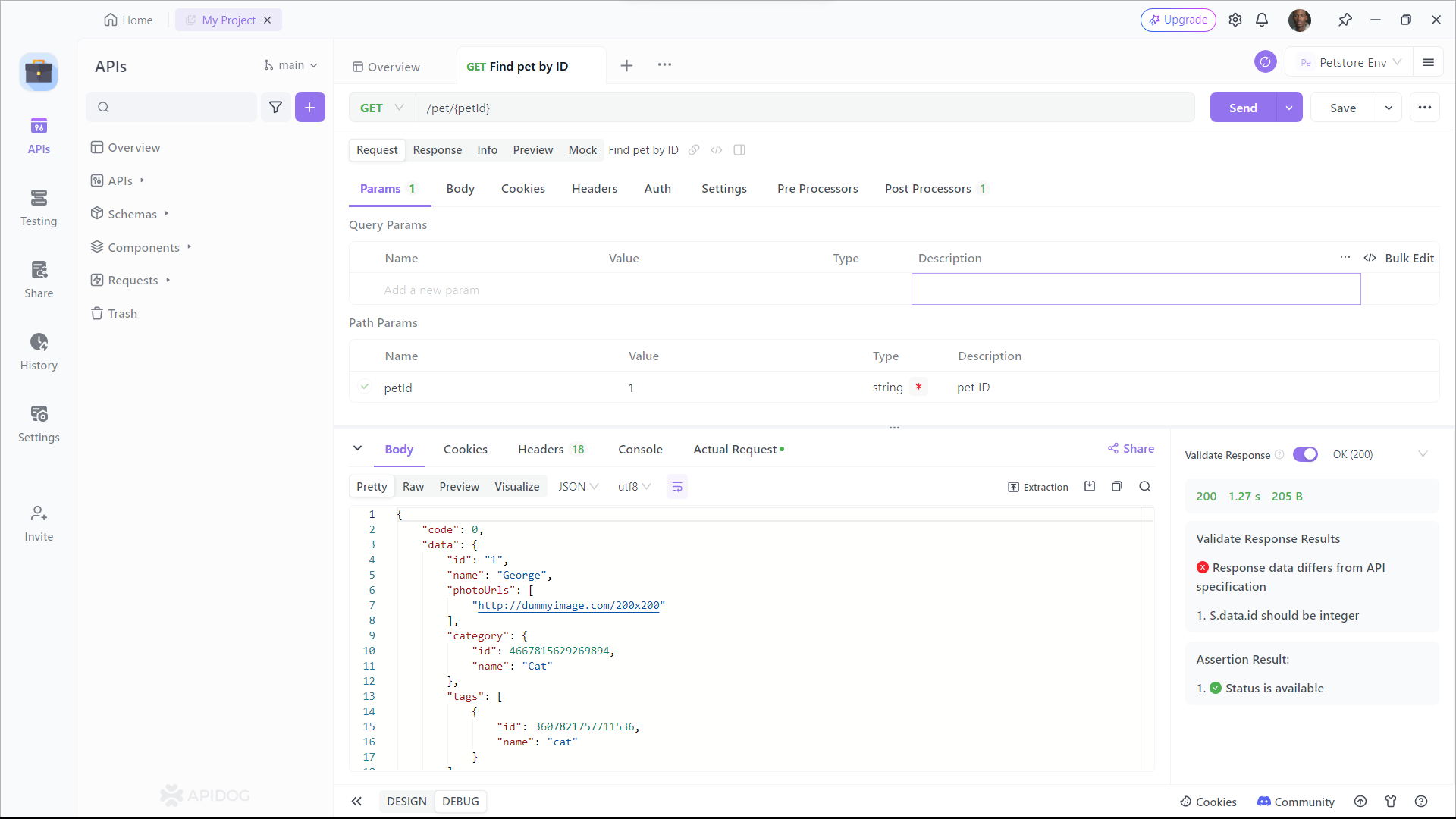
As you can see, Apidog shows you the URL, parameters, headers, and body of the request, and the status, headers, and body of the response. You can also see the response time, size, and format of the request and response, and compare them with different web APIs.
How to make Python automation testing using Apidog
Here’s a step-by-step guide on how to automate API testing using Apidog:
Open your Apidog Project and switch to the testing interface
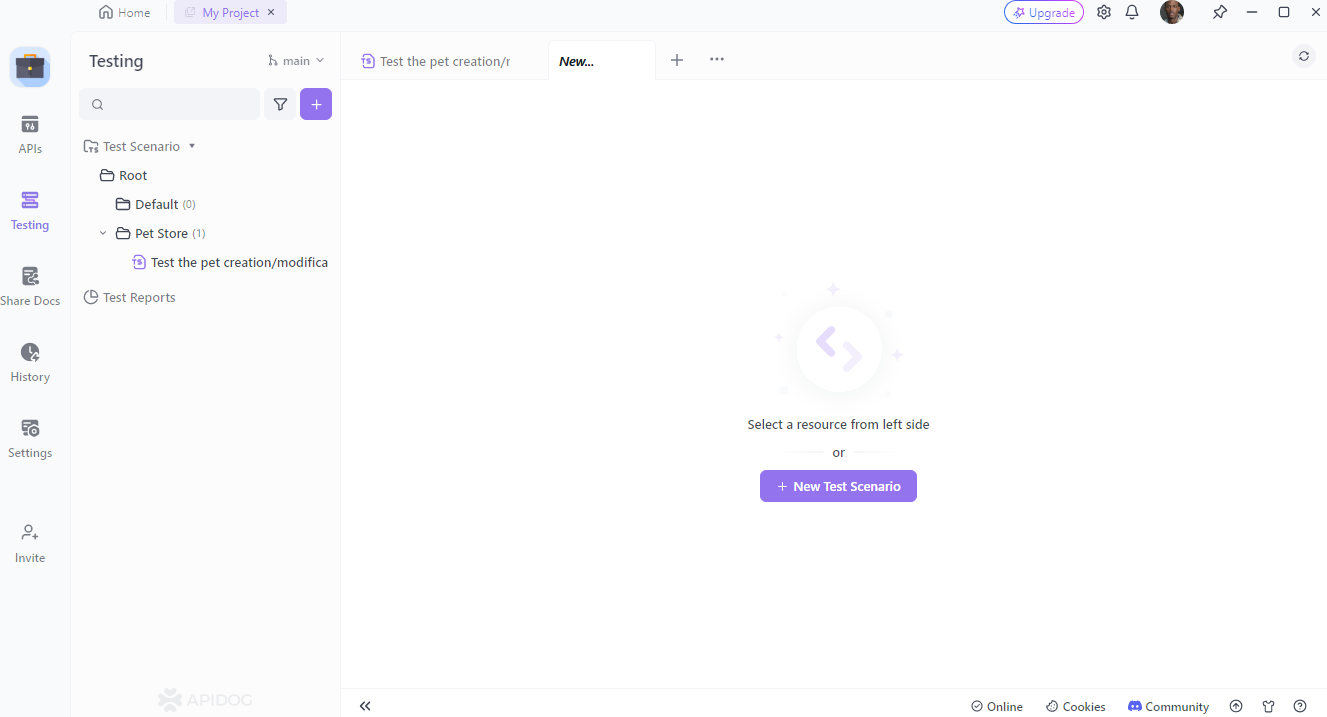
Design Your Test Scenarios: You can design your test scenarios in Apidog.
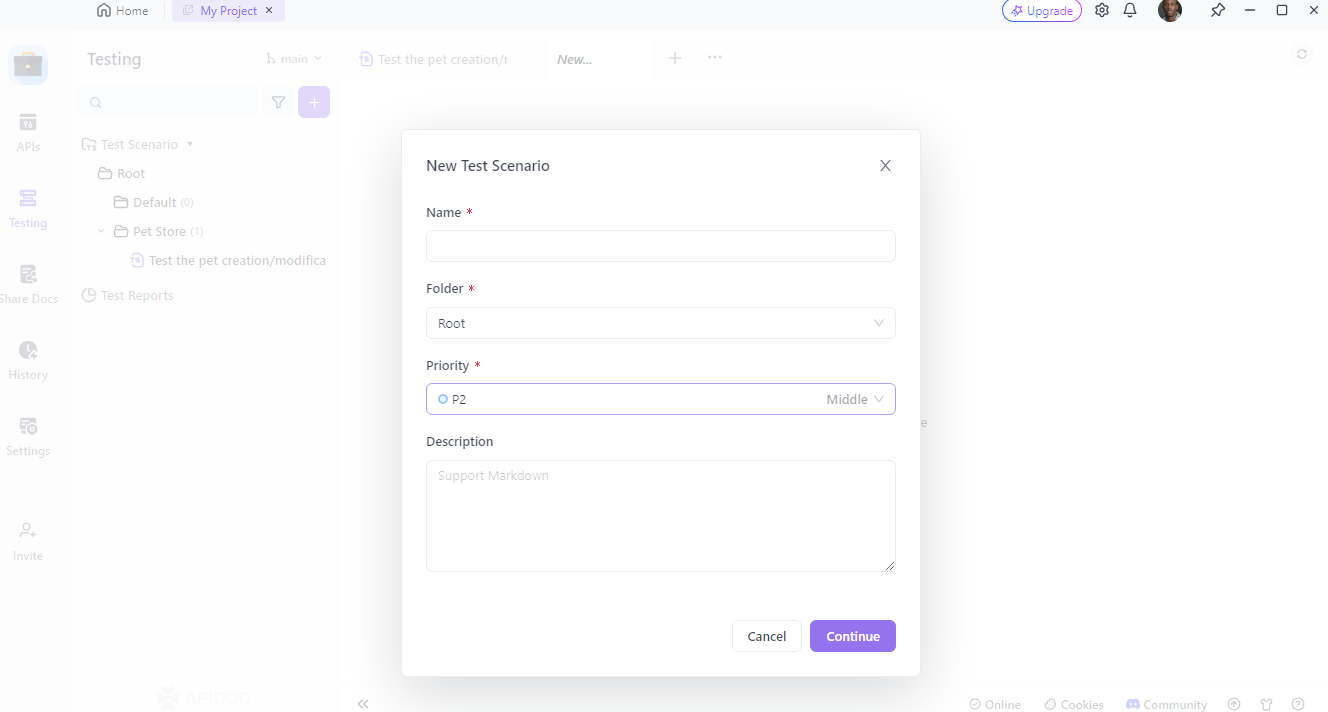
Run Your Tests: You can run your tests in Apidog.
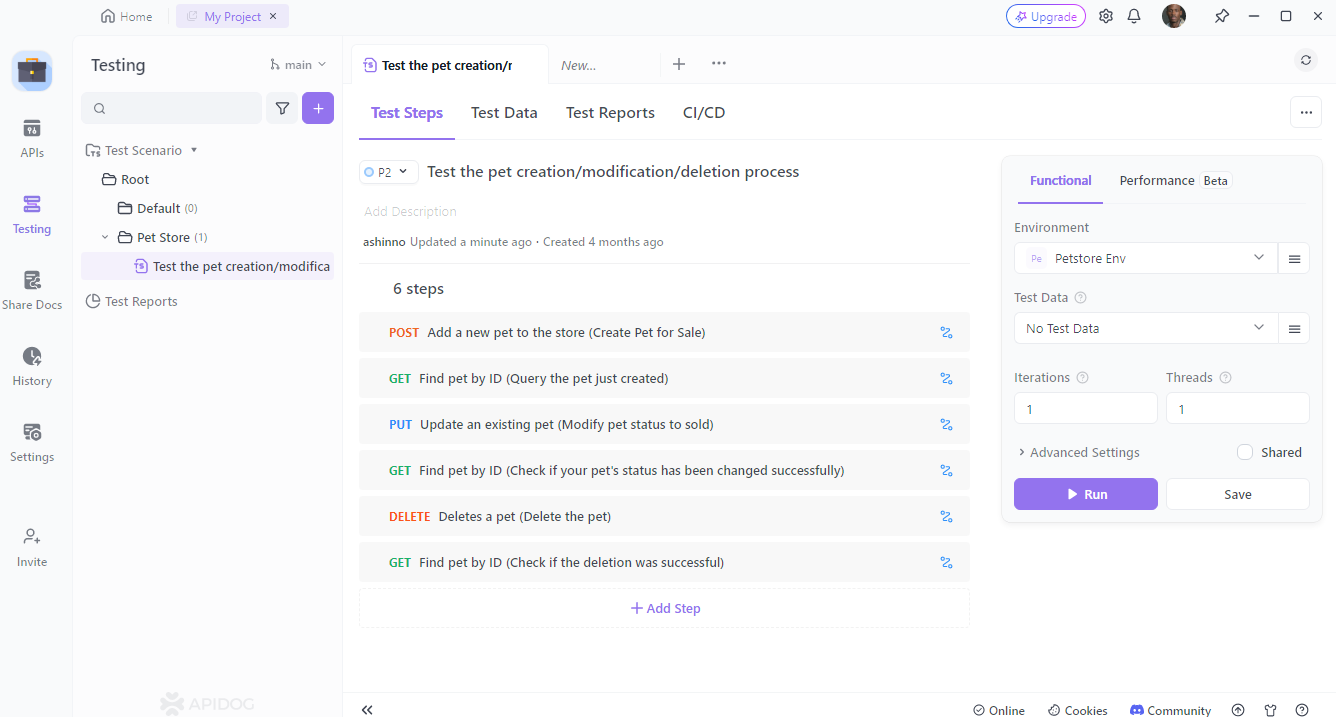
Analyze Test Results and Optimize: After running your tests, you can analyze the test results and optimize your tests accordingly.
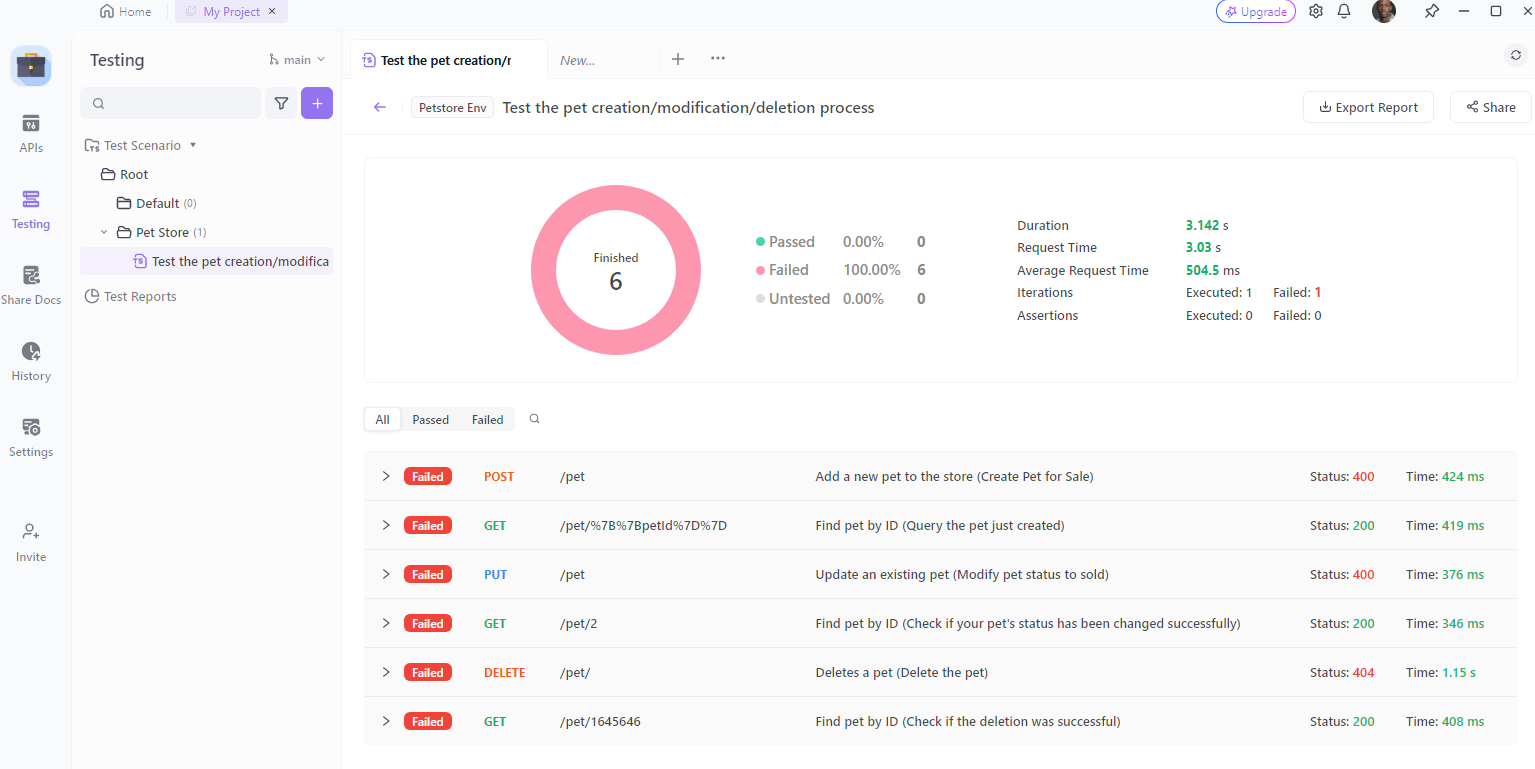
Remember, Apidog is an all-in-one toolkit for API development. The whole team can work on it together to produce APIs efficiently and conveniently. Each role in the team can use it to solve their own problems.
Best Practices for API Testing
To wrap up, here are some best practices for API testing:
Use Clear and Descriptive Test Names
Your test names should clearly describe what they are testing. This makes it easier to understand what went wrong when a test fails.
Test Both Positive and Negative Scenarios
Ensure you test both valid and invalid inputs to cover all possible scenarios.
Mock External Services
When testing APIs that depend on external services, use mocking to simulate these dependencies. This ensures your tests are reliable and don't fail due to issues with external services.
Maintain Clean and Organized Code
Keep your test code clean and organized. Use fixtures, helper functions, and proper structure to maintain readability and manageability.
Conclusion
API testing with Python is both powerful and straightforward. With tools like requests
and pytest
, you can write comprehensive tests that ensure your APIs are robust and reliable. By following best practices and leveraging automation, you can streamline your testing process and deliver high-quality software.
Happy testing!