Sending data to a server is a common task for web developers, and there are several methods to do so. One of the most efficient ways to update existing resources on a server is by using the PUT request. Today, we'll dive deep into how to send PUT requests with JSON data, ensuring you have a clear understanding of the process. Plus, we'll show you how to leverage tools like API, and Apidog to streamline your workflow.
Understanding PUT Requests
To begin, let's clarify what a PUT request is. In the world of APIs, HTTP methods are used to perform actions on resources. PUT is one of these methods, and it's specifically designed to update an existing resource or create a new one if it doesn’t exist.
The Basics of PUT Requests
Imagine you're working with a user profile system. You might have a user resource with details like name, email, and address. If you want to update the user's address, you'd use a PUT request.
Here’s the general structure of a PUT request:
- URL: The endpoint where the resource is located.
- Headers: Metadata about the request, including content type.
- Body: The actual data you want to send, often in JSON format.
Why JSON?
JSON (JavaScript Object Notation) is the most common format for sending data over the web. It's lightweight, easy to read, and supported by most programming languages. When you send a PUT request with JSON data, you're essentially updating the resource with new information formatted as a JSON object.
Setting Up for PUT Requests
Before we dive into coding, you need a few things in place:
- API Endpoint: The URL where you'll send your PUT request.
- API Key (if needed): Some APIs require authentication.
- Tool or Library: To send HTTP requests, like Apidog, Postman, or a programming language with HTTP capabilities.
Tools You’ll Need
While you can use any HTTP client, Apidog is a fantastic tool that simplifies API testing and development. If you haven’t already, download Apidog for free and install it on your machine. It will make your life easier when dealing with PUT requests.
Sending PUT Requests with Apidog
Let’s start by sending a PUT request using Apidog. Follow these steps:
- Open Apidog: Launch the application and Create a New Request
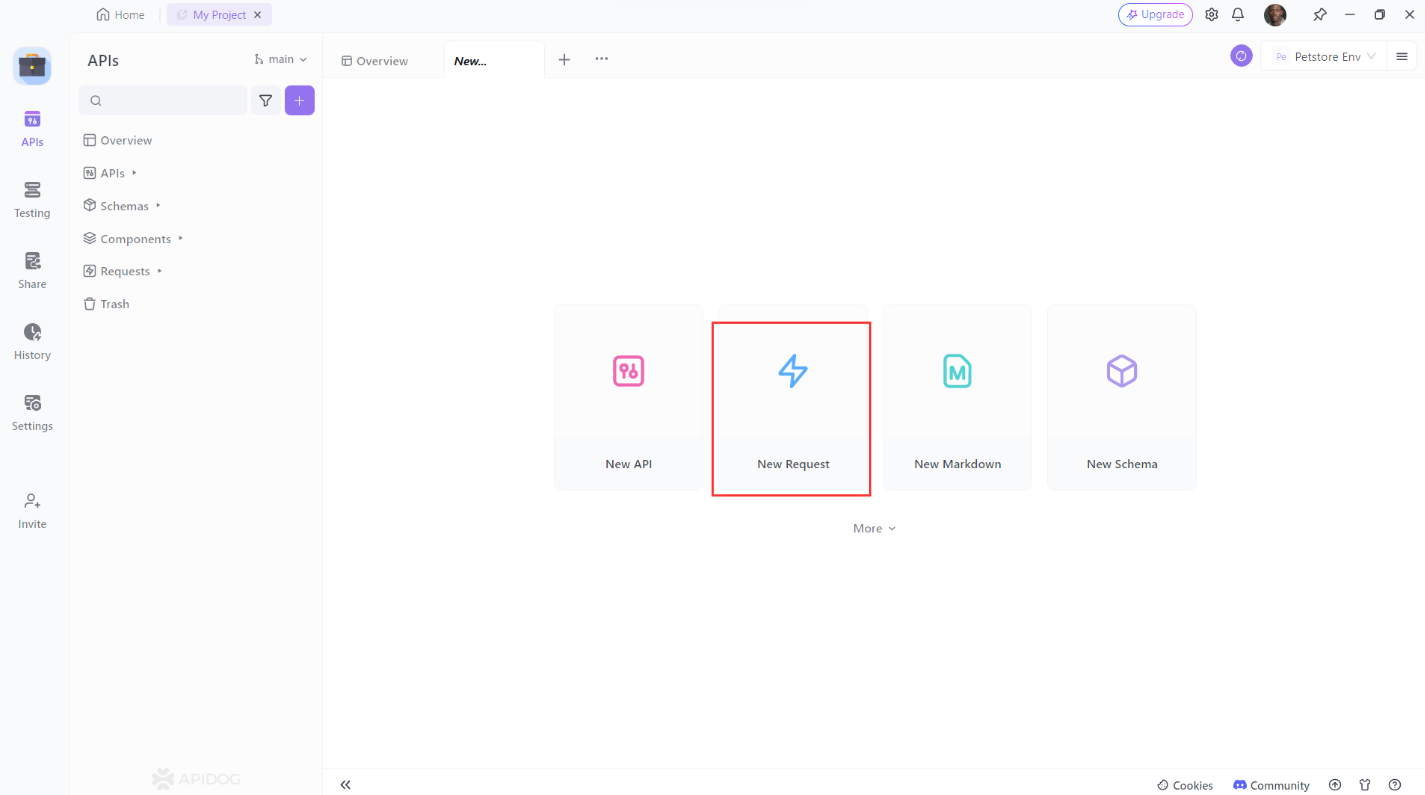
2. Set the Method to PUT: Choose PUT from the dropdown menu.
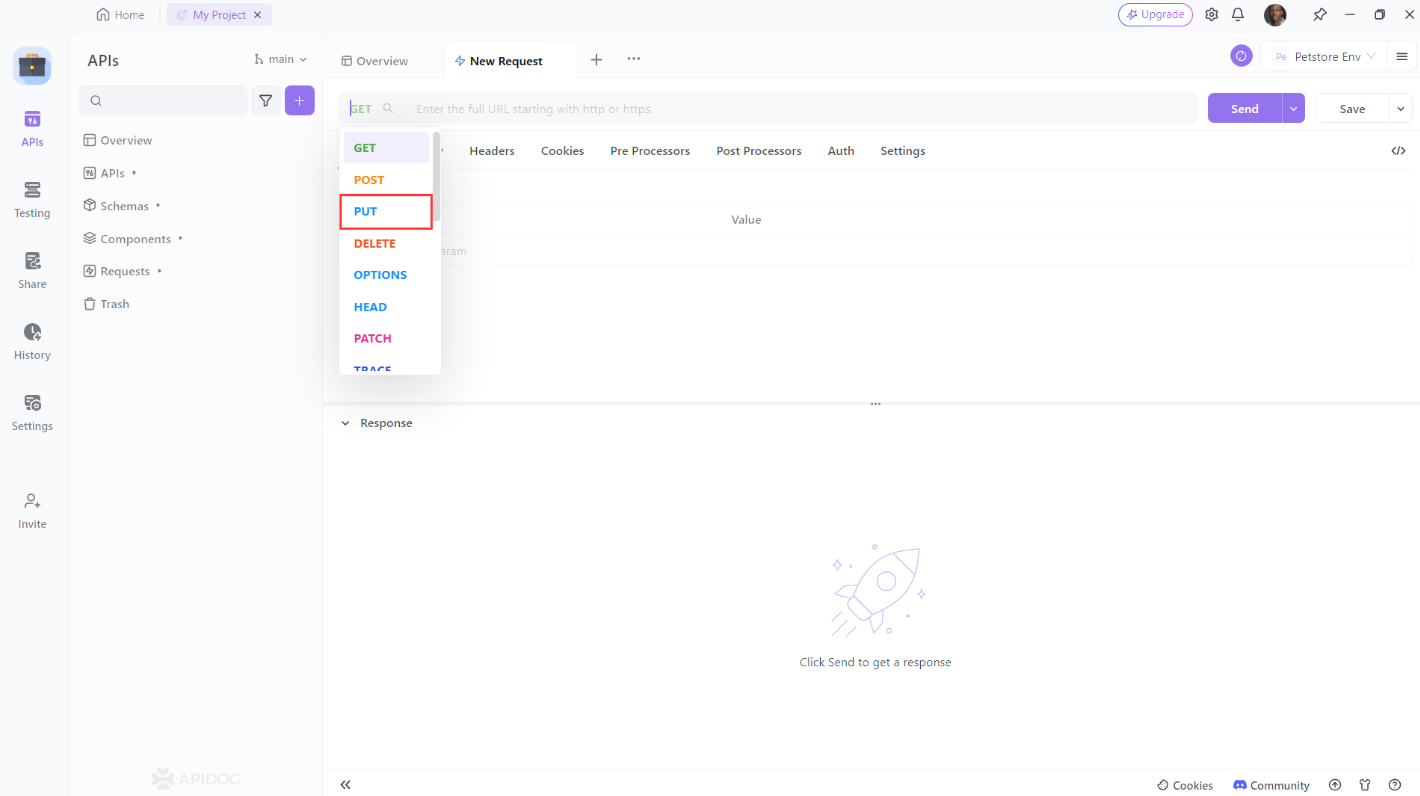
3. Enter the URL: Type in the API endpoint.
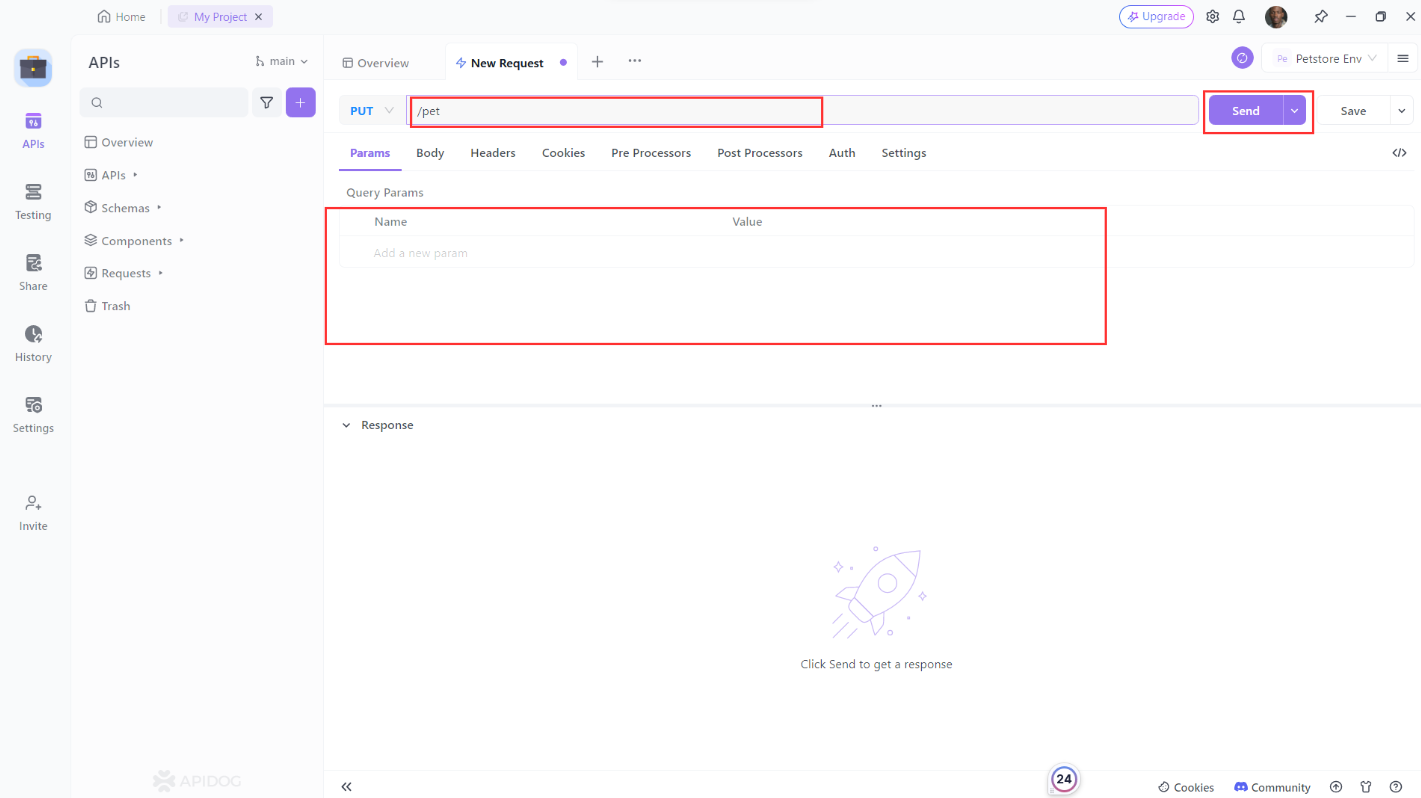
4. Add JSON Data: In the body section, input your JSON data.
Here’s an example of a PUT request to update a user’s address:
{
"name": "John Doe",
"email": "john.doe@example.com",
"address": "1234 Main St, Anytown, USA"
}
When you send this data, the server will update the user resource with the new address information.
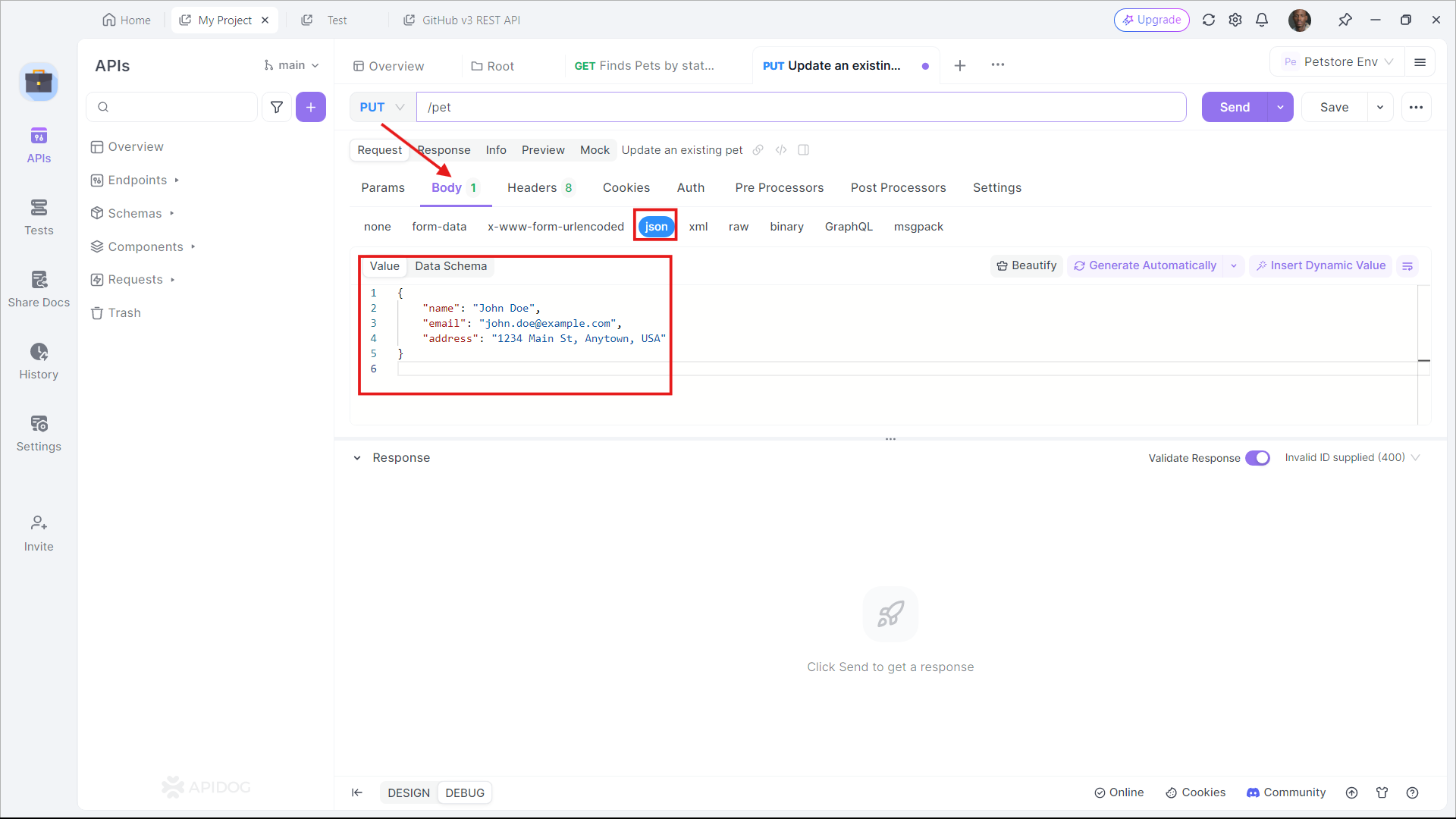
5. Send the Request: Click the “Send” button and review the response.

Writing PUT Requests in Code
While tools like Apidog are great for testing, you’ll often need to send PUT requests directly from your application code. Let’s look at how to do this in a few popular programming languages.
Using JavaScript (Fetch API)
JavaScript’s Fetch API is a modern way to make HTTP requests. Here’s how to send a PUT request with JSON data:
const url = 'https://api.example.com/user/1';
const data = {
name: 'John Doe',
email: 'john.doe@example.com',
address: '1234 Main St, Anytown, USA'
};
fetch(url, {
method: 'PUT',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(data)
})
.then(response => response.json())
.then(data => console.log('Success:', data))
.catch(error => console.error('Error:', error));
Using Python (Requests Library)
Python’s Requests library is another excellent tool for making HTTP requests. Here’s how to do it:
import requests
import json
url = 'https://api.example.com/user/1'
data = {
'name': 'John Doe',
'email': 'john.doe@example.com',
'address': '1234 Main St, Anytown, USA'
}
response = requests.put(url, headers={'Content-Type': 'application/json'}, data=json.dumps(data))
print(response.status_code)
print(response.json())
Using Java (HttpURLConnection)
Java’s HttpURLConnection is a bit more verbose, but it gets the job done:
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import java.nio.charset.StandardCharsets;
public class PutRequestExample {
public static void main(String[] args) {
try {
URL url = new URL("https://api.example.com/user/1");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("PUT");
connection.setRequestProperty("Content-Type", "application/json; utf-8");
connection.setDoOutput(true);
String jsonInputString = "{\"name\": \"John Doe\", \"email\": \"john.doe@example.com\", \"address\": \"1234 Main St, Anytown, USA\"}";
try (OutputStream os = connection.getOutputStream()) {
byte[] input = jsonInputString.getBytes(StandardCharsets.UTF_8);
os.write(input, 0, input.length);
}
int responseCode = connection.getResponseCode();
System.out.println("Response Code: " + responseCode);
// Read the response (if needed)
} catch (Exception e) {
e.printStackTrace();
}
}
}
Handling Responses
When you send a PUT request, you’ll get a response from the server. This response will let you know if your request was successful or if there were any errors. Here are some common response codes:
- 200 OK: The request was successful, and the resource was updated.
- 201 Created: The resource was created successfully (if it didn’t exist).
- 400 Bad Request: The server couldn’t understand the request (e.g., invalid JSON).
- 401 Unauthorized: Authentication is required and has failed or not been provided.
- 404 Not Found: The requested resource couldn’t be found.
- 500 Internal Server Error: Something went wrong on the server.
Example Response Handling in JavaScript
Let’s revisit our JavaScript example and add response handling:
fetch(url, {
method: 'PUT',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(data)
})
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok ' + response.statusText);
}
return response.json();
})
.then(data => console.log('Success:', data))
.catch(error => console.error('Error:', error));
Tips for Sending PUT Requests with JSON Data
Now that you know the basics, here are some tips to ensure your PUT requests are effective:
Validate Your JSON
Before sending your JSON data, validate it to ensure it’s correctly formatted. There are many online JSON validators available.
Use Proper Headers
Always set the Content-Type
header to application/json
when sending JSON data. This tells the server to expect JSON in the request body.
Handle Errors Gracefully
Implement error handling in your code to manage potential issues like network errors or invalid responses. This will make your application more robust.
Test with Tools
Use tools like Apidog to test your PUT requests before implementing them in your code. This can save you a lot of debugging time.
Conclusion
Sending PUT requests with JSON data is a fundamental skill for any web developer. Whether you’re updating user profiles, modifying resources, or syncing data, understanding how to craft these requests is crucial.
Remember to download Apidog for free to make your API testing smoother and more efficient. With the right tools and knowledge, you'll be well-equipped to handle any API interaction.
Happy coding!